Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
Merge pull request #16 from Elius94/Elius94/issue13
Elius94/issue13 ## NEW DRAWING ALGORYTM 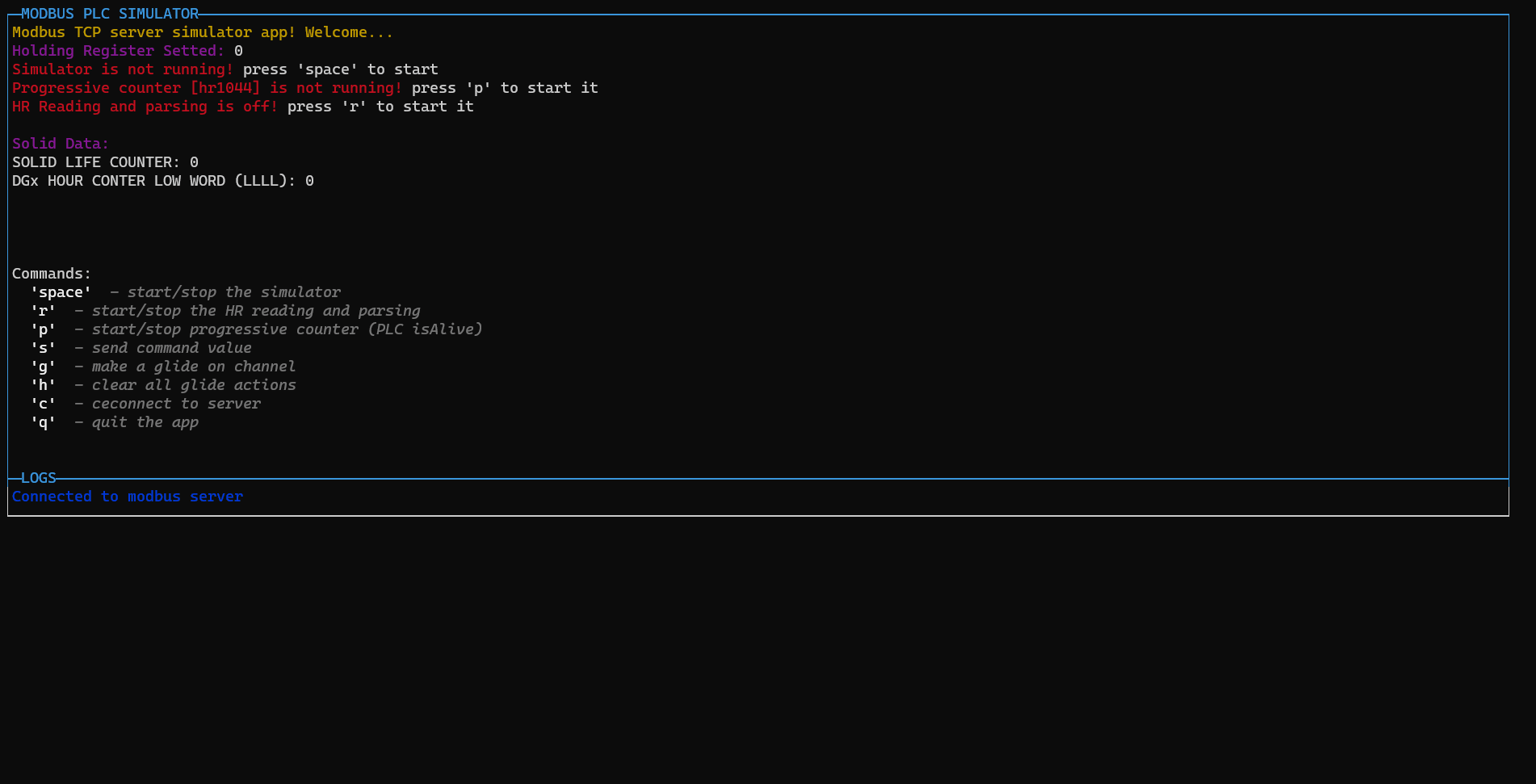 Now there's no more flickering in the screen! all the page is prerendered before printing on the console. Introduced new styling design pattern: Each page need to be created with the new class ```js const p = new PageBuilder() ``` and to add a styled row it's neccessary to call: ```js p.addRow({ text: ` 'm'`, color: 'gray', bold: true }, { text: ` - Select simulation mode`, color: 'white', italic: true }) ``` The arguments of that function is an array of object (function arguments syntax, no []!), so in a row you can add different phrases with different styles. The styles are converted to the Chalk modificator: ### colors: - black - red - green - yellow - blue - magenta - cyan - white - blackBright (alias: gray, grey) - redBright - greenBright - yellowBright - blueBright - magentaBright - cyanBright - whiteBright ### Background colors ('bg') - bgBlack - bgRed - bgGreen - bgYellow - bgBlue - bgMagenta - bgCyan - bgWhite - bgBlackBright (alias: bgGray, bgGrey) - bgRedBright - bgGreenBright - bgYellowBright - bgBlueBright - bgMagentaBright - bgCyanBright - bgWhiteBright ### Formatters (Each is a prop): - italic - bold - dim - underline - overline - inverse - hidden - strikethrough eg: ```js p.addRow({ text: `TCP messages sent:`, color: 'green', bg: 'bgRed', bold: true, italic: true, underline: true }, { text: ` ${tcpCounter}`, color: 'white' }) ``` And so, we can add the PageBuilder to the home page ```js GUI.setHomePage(p) ``` The new Screen class is used internally by the ConsoleManager.
- Loading branch information