diff --git a/.github/ISSUE_TEMPLATE/custom.md b/.github/ISSUE_TEMPLATE/custom.md
new file mode 100644
index 000000000..48d5f81fa
--- /dev/null
+++ b/.github/ISSUE_TEMPLATE/custom.md
@@ -0,0 +1,10 @@
+---
+name: Custom issue template
+about: Describe this issue template's purpose here.
+title: ''
+labels: ''
+assignees: ''
+
+---
+
+
diff --git a/.github/ISSUE_TEMPLATE/feature_request.md b/.github/ISSUE_TEMPLATE/feature_request.md
index bbcbbe7d6..3f3ef4501 100644
--- a/.github/ISSUE_TEMPLATE/feature_request.md
+++ b/.github/ISSUE_TEMPLATE/feature_request.md
@@ -2,7 +2,7 @@
name: Feature request
about: Suggest an idea for this project
title: ''
-labels: ''
+labels: question
assignees: ''
---
diff --git a/.gitignore b/.gitignore
index efb9828ad..87ac5adcb 100644
--- a/.gitignore
+++ b/.gitignore
@@ -1,4 +1,6 @@
.idea/
.vscode/
-
+automate_git.bat
+auto_commit.sh
+auto_commit.bat
*.iml
\ No newline at end of file
diff --git a/README.md b/README.md
index 47a9d4474..cb4bd1c19 100644
--- a/README.md
+++ b/README.md
@@ -2,4 +2,7 @@
Php-based LeetCode algorithm problem solutions, regularly updated.
-
+
+
+
+
diff --git a/algorithms/000040-combination-sum-ii/README.md b/algorithms/000040-combination-sum-ii/README.md
new file mode 100644
index 000000000..ac01d0ea1
--- /dev/null
+++ b/algorithms/000040-combination-sum-ii/README.md
@@ -0,0 +1,56 @@
+40\. Combination Sum II
+
+**Difficulty:** Medium
+
+**Topics:** `Array`, `Backtracking`
+
+Given a collection of candidate numbers (`candidates`) and a target number (`target`), find all unique combinations in `candidates` where the candidate numbers sum to `target`.
+
+Each number in `candidates` may only be used **once** in the combination.
+
+**Note:** The solution set must not contain duplicate combinations.
+
+**Example 1:**
+
+- **Input:** candidates = [10,1,2,7,6,1,5], target = 8
+- **Output:** [[1,1,6], [1,2,5], [1,7], [2,6]]
+
+**Example 2:**
+
+- **Input:** candidates = [2,5,2,1,2], target = 5
+- **Output:** [[1,2,2], [5]]
+
+**Constraints:**
+
+- 1 <= candidates.length <= 100
+- 1 <= candidates[i] <= 50
+- 1 <= target <= 30
+
+
+**Solution:**
+
+
+We can use a backtracking approach. The key idea is to sort the array first to handle duplicates easily and then explore all possible combinations using backtracking.
+
+Let's implement this solution in PHP: **[40. Combination Sum II](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/000040-combination-sum-ii/solution.php)**
+
+### Explanation:
+
+1. **Sorting**: The candidates array is sorted to handle duplicates easily and to ensure combinations are formed in a sorted order.
+2. **Backtracking**: The `backtrack` function is used to explore all possible combinations.
+ - If the `target` becomes zero, we add the current combination to the result.
+ - We iterate over the candidates starting from the current index. If a candidate is the same as the previous one, we skip it to avoid duplicate combinations.
+ - We subtract the current candidate from the target and recursively call the `backtrack` function with the new target and the next index.
+ - The recursive call continues until we either find a valid combination or exhaust all possibilities.
+3. **Pruning**: If the candidate exceeds the target, we break out of the loop early since further candidates will also exceed the target.
+
+This code will output all unique combinations that sum up to the target while ensuring that each candidate is used only once in each combination.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
diff --git a/algorithms/000040-combination-sum-ii/solution.php b/algorithms/000040-combination-sum-ii/solution.php
new file mode 100644
index 000000000..063901cf8
--- /dev/null
+++ b/algorithms/000040-combination-sum-ii/solution.php
@@ -0,0 +1,44 @@
+backtrack($candidates, $target, 0, [], $result);
+ return $result;
+ }
+
+ /**
+ * @param $candidates
+ * @param $target
+ * @param $start
+ * @param $currentCombination
+ * @param $result
+ * @return void
+ */
+ function backtrack($candidates, $target, $start, $currentCombination, &$result) {
+ if ($target == 0) {
+ $result[] = $currentCombination;
+ return;
+ }
+
+ for ($i = $start; $i < count($candidates); $i++) {
+ if ($i > $start && $candidates[$i] == $candidates[$i - 1]) {
+ continue; // Skip duplicates
+ }
+
+ if ($candidates[$i] > $target) {
+ break; // No need to continue if the candidate exceeds the target
+ }
+
+ self::backtrack($candidates, $target - $candidates[$i], $i + 1, array_merge($currentCombination, [$candidates[$i]]), $result);
+ }
+ }
+
+}
\ No newline at end of file
diff --git a/algorithms/000075-sort-colors/README.md b/algorithms/000075-sort-colors/README.md
index 8b4d2bf7f..49660628a 100644
--- a/algorithms/000075-sort-colors/README.md
+++ b/algorithms/000075-sort-colors/README.md
@@ -2,6 +2,8 @@
Medium
+**Topics:** `Array`, `Two Pointers`, `Sorting`
+
Given an array `nums` with `n` objects colored red, white, or blue, sort them [in-place](https://en.wikipedia.org/wiki/In-place_algorithm) so that objects of the same color are adjacent, with the colors in the order red, white, and blue.
We will use the integers `0`, `1`, and `2` to represent the color red, white, and blue, respectively.
@@ -24,4 +26,81 @@ You must solve this problem without using the library's sort function.
- 1 <= n <= 300
- `nums[i]` is either `0`, `1`, or `2`.
-**Follow-up:** Could you come up with a one-pass algorithm using only constant extra space?
\ No newline at end of file
+**Follow-up:** Could you come up with a one-pass algorithm using only constant extra space?
+
+**Hint:**
+1. A rather straight forward solution is a two-pass algorithm using counting sort.
+2. Iterate the array counting number of 0's, 1's, and 2's.
+3. Overwrite array with the total number of 0's, then 1's and followed by 2's.
+
+
+**Solution:**
+
+
+To solve this problem, we can follow these steps:
+
+The goal is to sort the array `nums` with elements representing the colors red (0), white (1), and blue (2) in one pass, using constant extra space.
+
+### Approach:
+
+The most efficient way to solve this problem is by using the Dutch National Flag algorithm, which is a one-pass algorithm with constant extra space. The idea is to use three pointers:
+- `low` to track the position for the next 0 (red),
+- `mid` to traverse the array,
+- `high` to track the position for the next 2 (blue).
+
+### Algorithm:
+1. Initialize three pointers:
+ - `low` at the start (0),
+ - `mid` at the start (0),
+ - `high` at the end (n-1) of the array.
+
+2. Traverse the array with `mid`:
+ - If `nums[mid]` is `0` (red), swap `nums[mid]` with `nums[low]`, then increment both `low` and `mid`.
+ - If `nums[mid]` is `1` (white), move `mid` to the next element.
+ - If `nums[mid]` is `2` (blue), swap `nums[mid]` with `nums[high]` and decrement `high`.
+
+3. Continue until `mid` exceeds `high`.
+
+This algorithm sorts the array in-place with a single pass.
+
+Let's implement this solution in PHP: **[75. Sort Colors](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/000075-sort-colors/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+1. **Initialization:**
+ - `low` starts at the beginning (`0`).
+ - `mid` starts at the beginning (`0`).
+ - `high` starts at the end (`n-1`).
+
+2. **Loop Through Array:**
+ - If `nums[mid]` is `0`, it swaps with `nums[low]` because `0` should be at the front. Both pointers `low` and `mid` are then incremented.
+ - If `nums[mid]` is `1`, it is already in the correct position, so only `mid` is incremented.
+ - If `nums[mid]` is `2`, it swaps with `nums[high]` because `2` should be at the end. Only `high` is decremented, while `mid` stays the same to check the swapped element.
+
+3. **Completion:**
+ - The loop continues until `mid` passes `high`, ensuring that the array is sorted in the order of `0`, `1`, and `2`.
+
+This approach is optimal with O(n) time complexity and O(1) space complexity.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
\ No newline at end of file
diff --git a/algorithms/000075-sort-colors/solution.php b/algorithms/000075-sort-colors/solution.php
index 4852aff5a..ec2a50242 100644
--- a/algorithms/000075-sort-colors/solution.php
+++ b/algorithms/000075-sort-colors/solution.php
@@ -6,22 +6,29 @@ class Solution {
* @param Integer[] $nums
* @return NULL
*/
- function sortColors(array &$nums) {
- $l = 0; // The next 0 should be placed in l.
- $r = count($nums) - 1; // The next 2 should be placed in r.
+ function sortColors(&$nums) {
+ $low = 0;
+ $mid = 0;
+ $high = count($nums) - 1;
- for ($i = 0; $i <= $r;) {
- if ($nums[$i] == 0) {
- list($nums[$i], $nums[$l]) = array($nums[$l], $nums[$i]);
- $i++;
- $l++;
- } elseif ($nums[$i] == 1) {
- $i++;
- } else {
- // We may swap a 0 to index i, but we're still not sure whether this 0
- // is placed in the correct index, so we can't move pointer i.
- list($nums[$i], $nums[$r]) = array($nums[$r], $nums[$i]);
- $r--;
+ while ($mid <= $high) {
+ if ($nums[$mid] == 0) {
+ // Swap nums[low] and nums[mid]
+ $temp = $nums[$low];
+ $nums[$low] = $nums[$mid];
+ $nums[$mid] = $temp;
+
+ $low++;
+ $mid++;
+ } elseif ($nums[$mid] == 1) {
+ $mid++;
+ } else { // nums[$mid] == 2
+ // Swap nums[mid] and nums[high]
+ $temp = $nums[$high];
+ $nums[$high] = $nums[$mid];
+ $nums[$mid] = $temp;
+
+ $high--;
}
}
}
diff --git a/algorithms/000078-subsets/README.md b/algorithms/000078-subsets/README.md
index 6ce5755bf..e2f7a7a61 100644
--- a/algorithms/000078-subsets/README.md
+++ b/algorithms/000078-subsets/README.md
@@ -1,6 +1,8 @@
78\. Subsets
-Medium
+**Difficulty:** Medium
+
+**Topics:** `Array`, `Backtracking`, `Bit Manipulation`
Given an integer array `nums` of **unique** elements, return _all possible subsets[^1] (the power set)._
@@ -23,4 +25,82 @@ The solution set **must not** contain duplicate subsets. Return the solution in
- -10 <= nums[i] <= 10
- All the numbers of `nums` are **unique**.
-[^1]: **Subset** A **subset** of an array is a selection of elements (possibly none) of the array.
\ No newline at end of file
+[^1]: **Subset** A **subset** of an array is a selection of elements (possibly none) of the array.
+
+**Solution:**
+
+
+To solve this problem, we can follow these steps:
+
+Let's implement this solution in PHP: **[78. Subsets](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/000078-subsets/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+1. **Function Signature and Input**:
+ - The function `subsets()` takes an array of integers `nums` as input and returns an array of arrays, where each inner array represents a subset of `nums`.
+ - The return type is `Integer[][]`, meaning an array of arrays of integers.
+
+2. **Initialization**:
+ - `$ans = [[]];`: Initialize the result array `$ans` with an empty subset (`[]`). This is the base case where the subset is empty.
+
+3. **Iterating Over Each Number**:
+ - `foreach($nums as $num)`: Loop through each number in the input array `nums`. For each number `num`, you'll generate new subsets by adding `num` to each of the existing subsets.
+
+4. **Generating New Subsets**:
+ - `$ans_size = count($ans);`: Before modifying the `$ans` array, store its current size in `$ans_size`. This is necessary to prevent an infinite loop as new subsets are added.
+ - `for($i = 0; $i < $ans_size; $i++)`: Iterate over the current subsets stored in `$ans` up to `$ans_size`.
+ - `$cur = $ans[$i];`: Take the current subset.
+ - `$cur[] = $num;`: Append the current number `num` to this subset.
+ - `$ans[] = $cur;`: Add this new subset back into `$ans`.
+
+5. **Return the Result**:
+ - `return $ans;`: After processing all numbers in `nums`, return the final array of all possible subsets.
+
+### Example Execution:
+
+Let's go through an example to see how it works.
+
+- **Input**: `$nums = [1, 2]`
+- **Initial State**: `$ans = [[]]`
+
+**Iteration 1 (`num = 1`)**:
+- `$ans_size = 1`: (Because there's only one subset, `[]`)
+- For `i = 0`:
+ - `$cur = []`: Take the empty subset.
+ - `$cur[] = 1`: Append `1` to it, resulting in `[1]`.
+ - `$ans = [[], [1]]`: Add `[1]` to `$ans`.
+
+**Iteration 2 (`num = 2`)**:
+- `$ans_size = 2`: (Now there are two subsets: `[]` and `[1]`)
+- For `i = 0`:
+ - `$cur = []`: Take the empty subset.
+ - `$cur[] = 2`: Append `2` to it, resulting in `[2]`.
+ - `$ans = [[], [1], [2]]`: Add `[2]` to `$ans`.
+- For `i = 1`:
+ - `$cur = [1]`: Take the subset `[1]`.
+ - `$cur[] = 2`: Append `2` to it, resulting in `[1, 2]`.
+ - `$ans = [[], [1], [2], [1, 2]]`: Add `[1, 2]` to `$ans`.
+
+**Final Result**:
+- The function returns `[[], [1], [2], [1, 2]]`, which are all possible subsets of `[1, 2]`.
+
+### Summary:
+
+This function uses a dynamic approach to generate all subsets of an array by iteratively adding each element of the array to existing subsets. The time complexity is \(O(2^n)\), where \(n\) is the number of elements in the input array, because each element can either be included or excluded from each subset, resulting in \(2^n\) possible subsets.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
\ No newline at end of file
diff --git a/algorithms/000079-word-search/README.md b/algorithms/000079-word-search/README.md
index 05545cec7..4e477c999 100644
--- a/algorithms/000079-word-search/README.md
+++ b/algorithms/000079-word-search/README.md
@@ -1,6 +1,8 @@
79\. Word Search
-Medium
+**Difficulty:** Medium
+
+**Topics:** `Array`, `String`, `Backtracking`, `Matrix`
Given an `m x n` grid of characters `board` and a string `word`, return `true` _if_ `word` _exists in the grid_.
@@ -8,19 +10,19 @@ The word can be constructed from letters of sequentially adjacent cells, where a
**Example 1:**
-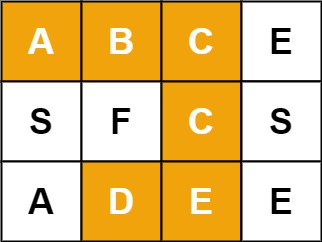
+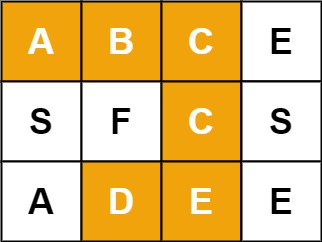
- **Input:** `board = [["A","B","C","E"],["S","F","C","S"],["A","D","E","E"]], word = "ABCCED"`
- **Output:** `true`
**Example 2:**
-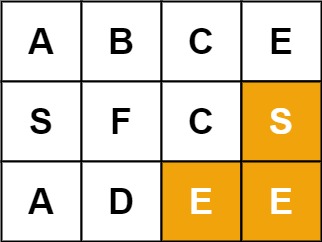
+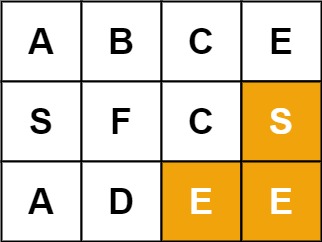
- **Input:** `board = [["A","B","C","E"],["S","F","C","S"],["A","D","E","E"]], word = "SEE"`
- **Output:** `true`
**Example 3:**
-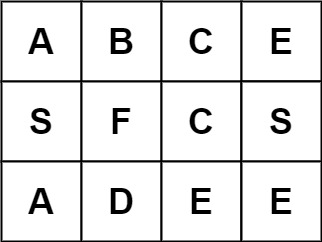
+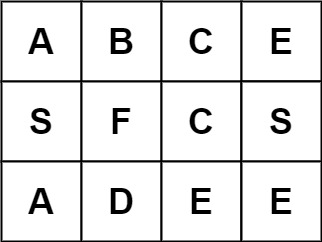
- **Input:** `board = [["A","B","C","E"],["S","F","C","S"],["A","D","E","E"]], word = "ABCB"`
- **Output:** `false`
@@ -32,4 +34,35 @@ The word can be constructed from letters of sequentially adjacent cells, where a
- 1 <= word.length <= 15
- `board` and `word` consists of only lowercase and uppercase English letters.
-**Follow-up:** Could you use search pruning to make your solution faster with a larger `board`?
\ No newline at end of file
+**Follow-up:** Could you use search pruning to make your solution faster with a larger `board`?
+
+
+**Solution:**
+
+
+To solve this problem, we can follow these steps:
+
+Let's implement this solution in PHP: **[79. Word Search](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/000079-word-search/solution.php)**
+
+
+### Explanation:
+
+1. **Initialization:**
+ - The `exist` function iterates through each cell in the grid. It starts a DFS search from each cell to see if the word can be constructed from that starting point.
+
+2. **DFS Search (`dfs` function):**
+ - **Bounds Check:** The DFS function first checks if the current position is out of bounds or if the character at the current position doesn't match the current character in the word.
+ - **Word Completion:** If the current character matches and the end of the word is reached, it returns `true`.
+ - **Marking Cells:** It marks the current cell as visited by setting it to `'*'` to avoid reusing the same cell.
+ - **Recursive Exploration:** It recursively searches in the four possible directions (up, down, left, right).
+ - **Unmarking Cells:** After exploring all directions, it restores the original character in the board.
+
+This approach ensures that each cell is visited at most once per search and uses backtracking to ensure the solution remains efficient.
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
\ No newline at end of file
diff --git a/algorithms/000085-maximal-rectangle/README.md b/algorithms/000085-maximal-rectangle/README.md
index 6284d0c91..70ef74d51 100644
--- a/algorithms/000085-maximal-rectangle/README.md
+++ b/algorithms/000085-maximal-rectangle/README.md
@@ -1,12 +1,14 @@
85\. Maximal Rectangle
-Hard
+**Difficulty:** Hard
+
+**Topics:** `Array`, `Dynamic Programming`, `Stack`, `Matrix`, `Monotonic Stack`
Given a `rows x cols` binary matrix filled with `0`'s and `1`'s, find the largest rectangle containing only `1`'s and return its area.
**Example 1:**
-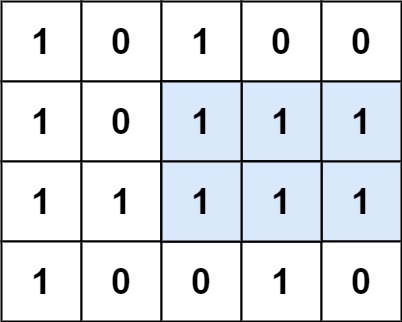
+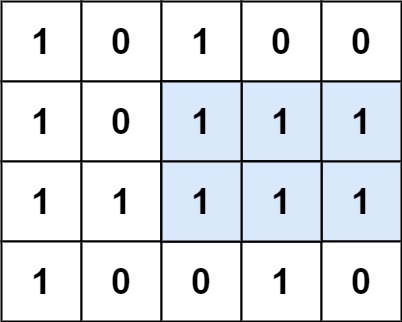
- **Input:** **matrix = [["1","0","1","0","0"],["1","0","1","1","1"],["1","1","1","1","1"],["1","0","0","1","0"]]**
- **Output:** **6**
@@ -27,4 +29,71 @@ Given a `rows x cols` binary matrix filled with `0`'s and `1`'s, find the larges
- `rows == matrix.length`
- `cols == matrix[i].length`
- `1 <= row, cols <= 200`
-- `matrix[i][j]` is `'0'` or `'1'`
.
\ No newline at end of file
+- `matrix[i][j]` is `'0'` or `'1'`
.
+
+
+**Solution:**
+
+
+We can follow a dynamic programming approach. This problem can be effectively tackled by treating each row of the matrix as the base of a histogram, where the height of each column in the histogram represents the number of consecutive '1's up to that row.
+
+1. **Convert each row of the matrix into a histogram**:
+ - Treat each row as the base of a histogram where the height of each bar is the number of consecutive `1`s up to that row.
+2. **Calculate the maximum area of the rectangle in the histogram for each row**:
+ - For each row, use a stack to maintain the indices of the histogram bars and calculate the largest rectangle area
+
+Let's implement this solution in PHP: **[85. Maximal Rectangle](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/000085-maximal-rectangle/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+1. **Matrix Initialization:**
+ - If the matrix is empty or the first row is empty, return `0`.
+
+2. **Height Array:**
+ - Initialize a `height` array that keeps track of the height of '1's for each column.
+
+3. **Updating Heights:**
+ - Traverse each row of the matrix and update the height of each column based on whether the matrix value is '1'.
+
+4. **Histogram Calculation:**
+ - For each updated `height` array, calculate the maximal rectangle area using a stack-based approach. This approach computes the maximum area for a histogram efficiently.
+
+5. **Stack-Based Histogram Calculation:**
+ - Use a stack to keep track of indices of increasing heights.
+ - Calculate area whenever a decrease in height is detected and update the maximum area.
+
+The `calculateMaxHistogramArea` function computes the largest rectangle area in a histogram, which is used to update the maximum area for each row's histogram. This approach ensures that the overall complexity is manageable given the problem constraints.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
\ No newline at end of file
diff --git a/algorithms/000085-maximal-rectangle/solution.php b/algorithms/000085-maximal-rectangle/solution.php
index f457ab848..a9373763f 100644
--- a/algorithms/000085-maximal-rectangle/solution.php
+++ b/algorithms/000085-maximal-rectangle/solution.php
@@ -1,50 +1,62 @@
= 0; $j--) {
- if ($matrix[$i][$j] == '1') {
- $R[$j] = min($R[$j], $right);
- $result = max($result, $H[$j] * ($R[$j] - $L[$j]));
- } else {
- $right = $j;
- }
+ // Calculate the max area for this row's histogram
+ $maxArea = max($maxArea, $this->calculateMaxHistogramArea($height));
+ }
+
+ return $maxArea;
+ }
+
+ /**
+ * @param $heights
+ * @return int|mixed
+ */
+ function calculateMaxHistogramArea($heights) {
+ $stack = array();
+ $maxArea = 0;
+ $index = 0;
+
+ while ($index < count($heights)) {
+ if (empty($stack) || $heights[$index] >= $heights[end($stack)]) {
+ array_push($stack, $index++);
+ } else {
+ $topOfStack = array_pop($stack);
+ $area = $heights[$topOfStack] * (empty($stack) ? $index : $index - end($stack) - 1);
+ $maxArea = max($maxArea, $area);
}
}
- return $result;
+ while (!empty($stack)) {
+ $topOfStack = array_pop($stack);
+ $area = $heights[$topOfStack] * (empty($stack) ? $index : $index - end($stack) - 1);
+ $maxArea = max($maxArea, $area);
+ }
+
+ return $maxArea;
}
+
}
\ No newline at end of file
diff --git a/algorithms/000129-sum-root-to-leaf-numbers/README.md b/algorithms/000129-sum-root-to-leaf-numbers/README.md
index 5119946c9..1a46f7378 100644
--- a/algorithms/000129-sum-root-to-leaf-numbers/README.md
+++ b/algorithms/000129-sum-root-to-leaf-numbers/README.md
@@ -1,6 +1,8 @@
**129\. Sum Root to Leaf Numbers**
-Medium
+**Difficulty:** Medium
+
+**Topics:** `Tree`, `Depth-First Search`, `Binary Tree`
You are given the root of a binary tree containing digits from `0` to `9` only.
@@ -40,4 +42,61 @@ A **leaf** node is a node with no children.
- The number of nodes in the tree is in the range `[1, 1000]`.
- `0 <= Node.val <= 9`
-- The depth of the tree will not exceed `10`.
\ No newline at end of file
+- The depth of the tree will not exceed `10`.
+
+
+**Solution:**
+
+
+We can use a Depth-First Search (DFS) approach. The idea is to traverse the tree, keeping track of the number formed by the path from the root to the current node. When you reach a leaf node, you add the current number to the total sum.
+
+Let's implement this solution in PHP: **[129. Sum Root to Leaf Numbers](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/000129-sum-root-to-leaf-numbers/solution.php)**
+
+```php
+left = new TreeNode(2);
+$root1->right = new TreeNode(3);
+echo sumNumbers($root1); // Output: 25
+
+// Example 2
+$root2 = new TreeNode(4);
+$root2->left = new TreeNode(9);
+$root2->right = new TreeNode(0);
+$root2->left->left = new TreeNode(5);
+$root2->left->right = new TreeNode(1);
+echo sumNumbers($root2); // Output: 1026
+
+?>
+```
+
+### Explanation:
+
+1. **TreeNode Class**: This class represents each node of the binary tree. Each node has a value (`val`), a left child (`left`), and a right child (`right`).
+
+2. **sumNumbers Function**: This is the main function that initiates the DFS traversal by calling the `sumNumbersRecu` function with the root node and an initial `currentSum` of 0.
+
+3. **sumNumbersRecu Function**:
+ - **Base Case**: If the node is `null`, return 0 (this handles the case where there is no child node).
+ - **Update Current Sum**: For each node, update the `currentSum` by multiplying the previous `currentSum` by 10 and adding the node's value.
+ - **Leaf Node Check**: If the node is a leaf (i.e., it has no left or right child), return the `currentSum` as this represents the number formed by the path from the root to this leaf.
+ - **Recursive Calls**: If the node is not a leaf, recursively call `sumNumbersRecu` on both the left and right children and return their sum.
+
+### Edge Cases:
+
+- The function handles cases where the tree has only one node.
+- It correctly computes the sum even for deeper trees, as the depth of the tree will not exceed 10 (as per the problem constraints).
+
+This solution runs efficiently with a time complexity of O(n), where n is the number of nodes in the tree. This is because each node is visited exactly once. The space complexity is O(h), where h is the height of the tree, due to the recursion stack.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
\ No newline at end of file
diff --git a/algorithms/000131-palindrome-partitioning/README.md b/algorithms/000131-palindrome-partitioning/README.md
index 613edf138..0659e350f 100644
--- a/algorithms/000131-palindrome-partitioning/README.md
+++ b/algorithms/000131-palindrome-partitioning/README.md
@@ -1,6 +1,8 @@
131\. Palindrome Partitioning
-Medium
+**Difficulty:** Medium
+
+**Topics:** `String`, `Dynamic Programming`, `Backtracking`
Given a string `s`, partition `s` such that every substring[^1] of the partition is a palindrome[^2]. Return all possible palindrome partitioning of `s`.
@@ -21,4 +23,59 @@ Given a string `s`, partition `s` such that every substring[^1] of the partition
[^1]: **Substring** A **substring** is a contiguous non-empty sequence of characters within a string.
-[^2]: **Palindrome** A **palindrome** is a string that reads the same forward and backward.
\ No newline at end of file
+[^2]: **Palindrome** A **palindrome** is a string that reads the same forward and backward.
+
+
+**Solution:**
+
+
+We can use a combination of backtracking and dynamic programming. The goal is to explore all possible partitions and check if each partition is a palindrome.
+
+### Steps to Solve:
+
+1. **Backtracking**:
+ - We'll explore every possible partitioning of the string. For each possible starting index, we try to partition the string at every possible end index.
+ - If a substring is a palindrome, we recursively partition the remaining string.
+
+2. **Palindrome Check**:
+ - We need a helper function to check if a given substring is a palindrome. This can be done by comparing the substring with its reverse.
+
+3. **Collect Results**:
+ - When we reach the end of the string, we've found a valid partitioning, so we add it to the result list.
+
+
+Let's implement this solution in PHP: **[131. Palindrome Partitioning](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/000131-palindrome-partitioning/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+1. **`partition($s)`**: This function initializes the backtracking process.
+2. **`backtrack($s, $start, &$current, &$result)`**:
+ - It explores each substring starting at index `start`.
+ - If a palindrome is found, it's added to the current partition (`$current`), and the function is called recursively for the next part of the string.
+ - Once all partitions from the current start point are explored, the last substring is removed from `$current` to backtrack and try other possibilities.
+3. **`isPalindrome($s)`**: This helper function checks if a string is a palindrome by comparing characters from the start and end.
+
+### Time Complexity:
+- The time complexity is exponential in the worst case, as we explore all possible partitions (backtracking).
+- The palindrome check is \(O(n)\) for each substring.
+
+This solution works efficiently for the given constraint where the maximum length of the string is 16.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
diff --git a/algorithms/000131-palindrome-partitioning/solution.php b/algorithms/000131-palindrome-partitioning/solution.php
index 7ce2d352b..0ecaa5b20 100644
--- a/algorithms/000131-palindrome-partitioning/solution.php
+++ b/algorithms/000131-palindrome-partitioning/solution.php
@@ -7,56 +7,46 @@ class Solution {
* @return String[][]
*/
function partition($s) {
- $length = strlen($s);
-
- // Create a table to record if the substring s[i..j] is a palindrome.
- $palindromeTable = array_fill(0, $length, array_fill(0, $length, true));
+ $result = [];
+ $current = [];
+ $this->backtrack($s, 0, $current, $result);
+ return $result;
+ }
- // Fill the palindromeTable using dynamic programming.
- for ($i = $length - 1; $i >= 0; $i--) {
- for ($j = $i + 1; $j < $length; $j++) {
- // A substring is a palindrome if its outer characters are equal
- // and if its inner substring is also a palindrome.
- $palindromeTable[$i][$j] = ($s[$i] == $s[$j]) && $palindromeTable[$i + 1][$j - 1];
- }
+ /**
+ * @param $s
+ * @param $start
+ * @param $current
+ * @param $result
+ * @return void
+ */
+ function backtrack($s, $start, &$current, &$result) {
+ if ($start >= strlen($s)) {
+ $result[] = $current;
+ return;
}
- // Create a vector to store all palindrome partitioning results.
- $result = array();
- // Temporary vector to store current partitioning.
- $tempPartition = array();
-
- // Recursively search for palindrome partitions starting from index 0.
- $depthFirstSearch = function($start) use (&$depthFirstSearch, &$result, &$tempPartition, $s, $length, $palindromeTable) {
-
- // If we've reached the end of the string, add the current partitioning to results.
- if ($start == $length) {
- $result[] = $tempPartition;
- return;
+ for ($end = $start; $end < strlen($s); $end++) {
+ if ($this->isPalindrome(substr($s, $start, $end - $start + 1))) {
+ $current[] = substr($s, $start, $end - $start + 1);
+ self::backtrack($s, $end + 1, $current, $result);
+ array_pop($current);
}
+ }
+ }
- // Explore all possible partitioning.
- for ($end = $start; $end < $length; $end++) {
-
- // If the substring starting from 'start' to 'end' is a palindrome
- if ($palindromeTable[$start][$end]) {
-
- // Push the current palindrome substring to the temporary partitioning.
- $tempPartition[] = substr($s, $start, $end - $start + 1);
-
- // Move to the next part of the string.
- $depthFirstSearch($end + 1);
-
- // Backtrack to explore other partitioning possibilities.
- array_pop($tempPartition);
- }
+ /**
+ * @param $s
+ * @return bool
+ */
+ function isPalindrome($s) {
+ $n = strlen($s);
+ for ($i = 0; $i < $n / 2; $i++) {
+ if ($s[$i] !== $s[$n - $i - 1]) {
+ return false;
}
- };
-
- // Start the depth-first search from the first character.
- $depthFirstSearch(0);
-
- // Return all the palindrome partitioning found.
- return $result;
+ }
+ return true;
}
+
}
\ No newline at end of file
diff --git a/algorithms/000140-word-break-ii/README.md b/algorithms/000140-word-break-ii/README.md
index 3288ab633..80e4c06c5 100644
--- a/algorithms/000140-word-break-ii/README.md
+++ b/algorithms/000140-word-break-ii/README.md
@@ -1,6 +1,8 @@
140\. Word Break II
-Hard
+**Difficulty:** Hard
+
+**Topics:** `Array`, `Hash Table`, `String`, `Dynamic Programming`, `Backtracking`, `Trie`, `Memoization`
Given a string `s` and a dictionary of strings `wordDict`, add spaces in `s` to construct a sentence where each word is a valid dictionary word. Return all such possible sentences in **any order**.
@@ -29,4 +31,66 @@ Given a string `s` and a dictionary of strings `wordDict`, add spaces in `s` to
- 1 <= wordDict[i].length <= 10
- `s` and `wordDict[i]` consist of only lowercase English letters.
- All the strings of `wordDict` are **unique**.
-- Input is generated in a way that the length of the answer doesn't exceed 105
.
\ No newline at end of file
+- Input is generated in a way that the length of the answer doesn't exceed 105
.
+
+
+**Solution:**
+
+
+To solve this problem, we can follow these steps:
+
+- **Purpose:** To return all possible sentences that can be constructed from the string `s` using the words in `wordDict`, where each word can be used multiple times.
+
+### Key Components
+
+1. **Memoization Check (`$this->map`):**
+ - The function uses a property `$this->map` to store previously computed results for substrings. This prevents redundant computations and improves efficiency.
+ - If the substring `s` is already present in `$this->map`, the function returns the cached result for that substring.
+
+2. **Base Case:**
+ - If the length of `s` is 0 (i.e., an empty string), it means we have successfully segmented the string up to this point, so we add an empty string to the result array and store this result in `$this->map` for future reference.
+
+3. **Recursive Case:**
+ - The function iterates through each word in `wordDict`. For each word, it checks if the word is a prefix of the string `s` (`strpos($s, $word) === 0`).
+ - If it is a prefix, it recursively calls `wordBreak` on the remaining substring after removing the prefix (`substr($s, strlen($word))`).
+ - For each result from the recursive call (`$subWords`), it concatenates the current word with each result from the recursive call, separating them by a space if necessary.
+
+4. **Storing and Returning Results:**
+ - After processing all possible prefixes and constructing all possible sentences, the results are stored in `$this->map` for the current substring `s`.
+ - Finally, the function returns the result array.
+
+Let's implement this solution in PHP: **[140. Word Break II](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/000140-word-break-ii/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+- **Memoization:** Uses `$this->map` to store results for substrings and avoid redundant calculations.
+- **Recursive Processing:** Tries every word in `wordDict` as a potential prefix and processes the remaining string recursively.
+- **Building Results:** Concatenates words and results from recursive calls to form all possible sentences.
+
+This approach is effective but may not be the most efficient for large inputs due to its exponential nature. The memoization helps mitigate redundant computations, but the overall complexity can still be high.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
diff --git a/algorithms/000145-binary-tree-postorder-traversal/README.md b/algorithms/000145-binary-tree-postorder-traversal/README.md
new file mode 100644
index 000000000..c0387e352
--- /dev/null
+++ b/algorithms/000145-binary-tree-postorder-traversal/README.md
@@ -0,0 +1,107 @@
+145\. Binary Tree Postorder Traversal
+
+**Difficulty:** Easy
+
+**Topics:** `Stack`, `Tree`, `Depth-First Search`, `Binary Tree`
+
+Given the `root` of a binary tree, return _the postorder traversal of its nodes' values_.
+
+**Example 1:**
+
+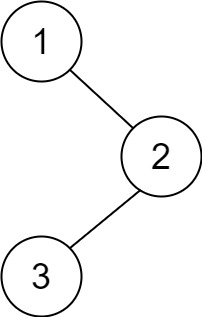
+
+- **Input:** root = [1,null,2,3]
+- **Output:** [3,2,1]
+
+**Example 2:**
+
+- **Input:** root = []
+- **Output:** []
+
+
+**Example 3:**
+
+- **Input:** root = [1]
+- **Output:** [1]
+
+
+
+**Constraints:**
+
+- The number of the nodes in the tree is in the range `[0, 100]`.
+- `-100 <= Node.val <= 100`
+
+
+
+**Solution:**
+
+We can use an iterative approach with a stack. Postorder traversal follows the order: Left, Right, Root.
+
+
+Let's implement this solution in PHP: **[145. Binary Tree Postorder Traversal](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/000145-binary-tree-postorder-traversal/solution.php)**
+
+```php
+val = $val;
+ $this->left = $left;
+ $this->right = $right;
+ }
+}
+/**
+* @param TreeNode $root
+* @return Integer[]
+*/
+function postorderTraversal($root) {
+ ...
+ ...
+ ...
+ /**
+ * go to ./solution.php
+ */
+}
+
+// Example usage:
+
+// Example 1
+$root1 = new TreeNode(1);
+$root1->right = new TreeNode(2);
+$root1->right->left = new TreeNode(3);
+print_r(postorderTraversal($root1)); // Output: [3, 2, 1]
+
+// Example 2
+$root2 = null;
+print_r(postorderTraversal($root2)); // Output: []
+
+// Example 3
+$root3 = new TreeNode(1);
+print_r(postorderTraversal($root3)); // Output: [1]
+?>
+```
+
+### Explanation:
+
+- **TreeNode Class:** The `TreeNode` class defines a node in the binary tree, including its value, left child, and right child.
+
+- **postorderTraversal Function:**
+ - We initialize an empty result array and a stack.
+ - We use a `while` loop that continues as long as either the stack is not empty or the current node is not null.
+ - If the current node is not null, we push it onto the stack and move to its left child.
+ - If the current node is null, we check the top node of the stack. If it has a right child that we haven't visited yet, we move to the right child. Otherwise, we add the node's value to the result array and pop it from the stack.
+
+This iterative approach simulates the recursive postorder traversal without using system recursion, making it more memory-efficient.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
+
diff --git a/algorithms/000145-binary-tree-postorder-traversal/solution.php b/algorithms/000145-binary-tree-postorder-traversal/solution.php
new file mode 100644
index 000000000..3033c3a6e
--- /dev/null
+++ b/algorithms/000145-binary-tree-postorder-traversal/solution.php
@@ -0,0 +1,51 @@
+val = $val;
+ * $this->left = $left;
+ * $this->right = $right;
+ * }
+ * }
+ */
+class Solution {
+
+ /**
+ * @param TreeNode $root
+ * @return Integer[]
+ */
+ function postorderTraversal($root) {
+ $result = [];
+ if ($root === null) {
+ return $result;
+ }
+
+ $stack = [];
+ $lastVisitedNode = null;
+ $current = $root;
+
+ while (!empty($stack) || $current !== null) {
+ if ($current !== null) {
+ array_push($stack, $current);
+ $current = $current->left;
+ } else {
+ $peekNode = end($stack);
+ // if right child exists and traversing node from left child, then move right
+ if ($peekNode->right !== null && $lastVisitedNode !== $peekNode->right) {
+ $current = $peekNode->right;
+ } else {
+ array_push($result, $peekNode->val);
+ $lastVisitedNode = array_pop($stack);
+ }
+ }
+ }
+
+ return $result;
+
+ }
+}
\ No newline at end of file
diff --git a/algorithms/000165-compare-version-numbers/README.md b/algorithms/000165-compare-version-numbers/README.md
index 43eef67da..7062548c7 100644
--- a/algorithms/000165-compare-version-numbers/README.md
+++ b/algorithms/000165-compare-version-numbers/README.md
@@ -1,6 +1,8 @@
165\. Compare Version Numbers
-Medium
+**Difficulty:** Medium
+
+**Topics:** `Two Pointers`, `String`
Given two version numbers, `version1` and `version2`, compare them.
@@ -39,3 +41,51 @@ _Return the following:_
- `version1` and `version2` only contain digits and `'.'`.
- `version1` and `version2` **are valid version numbers**.
- All the given revisions in `version1` and `version2` can be stored in a **32-bit integer**.
+
+**Hint:**
+1. You can use two pointers for each version string to traverse them together while comparing the corresponding segments.
+2. Utilize the substring method to extract each version segment delimited by '.'. Ensure you're extracting the segments correctly by adjusting the start and end indices accordingly.
+
+
+**Solution:**
+
+
+We can use a two-pointer approach to traverse each version number and compare their segments.
+
+1. **Split the Versions**: Use `explode` to split each version number by the dot `'.'` character into arrays of segments.
+2. **Traverse and Compare Segments**: Use two pointers to traverse the segments of each version. Compare corresponding segments as integers, taking care of leading zeros and treating missing segments as `0`.
+
+Let's implement this solution in PHP: **[165. Compare Version Numbers](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/000165-compare-version-numbers/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+1. **Splitting Versions**:
+ - `explode('.', $version1)` and `explode('.', $version2)` convert each version string into arrays of segments based on the dot delimiter.
+
+2. **Traversing Segments**:
+ - `max(count($v1), count($v2))` ensures that the loop runs through the longest of the two version arrays.
+ - `isset($v1[$i]) ? (int)$v1[$i] : 0` gets the segment value or defaults to `0` if the segment does not exist.
+
+3. **Comparing Segments**:
+ - Compare each segment as integers to handle leading zeros.
+ - Return `-1` if the first version is less, `1` if it is greater, and `0` if they are equal after all segments are compared.
+
+This solution efficiently compares version numbers by considering each segment as an integer and handles cases where versions have different lengths by treating missing segments as `0`.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
diff --git a/algorithms/000165-compare-version-numbers/solution.php b/algorithms/000165-compare-version-numbers/solution.php
index 18ce974f2..e529c30c6 100644
--- a/algorithms/000165-compare-version-numbers/solution.php
+++ b/algorithms/000165-compare-version-numbers/solution.php
@@ -7,23 +7,30 @@ class Solution {
* @param String $version2
* @return Integer
*/
- function compareVersion(string $version1, string $version2): int
- {
- $levels1 = explode('.', $version1);
- $levels2 = explode('.', $version2);
- $length = max(count($levels1), count($levels2));
+ function compareVersion($version1, $version2) {
+ // Split the version numbers into arrays of segments
+ $v1 = explode('.', $version1);
+ $v2 = explode('.', $version2);
+ // Get the maximum length to iterate over both arrays
+ $length = max(count($v1), count($v2));
+
+ // Compare each segment
for ($i = 0; $i < $length; $i++) {
- $v1 = isset($levels1[$i]) ? (int)$levels1[$i] : 0;
- $v2 = isset($levels2[$i]) ? (int)$levels2[$i] : 0;
- if ($v1 < $v2) {
+ // Get the current segment from each version, defaulting to "0" if not present
+ $seg1 = isset($v1[$i]) ? (int)$v1[$i] : 0;
+ $seg2 = isset($v2[$i]) ? (int)$v2[$i] : 0;
+
+ // Compare segments
+ if ($seg1 < $seg2) {
return -1;
- }
- if ($v1 > $v2) {
+ } elseif ($seg1 > $seg2) {
return 1;
}
}
+ // All segments are equal
return 0;
+
}
}
\ No newline at end of file
diff --git a/algorithms/000179-largest-number/README.md b/algorithms/000179-largest-number/README.md
new file mode 100644
index 000000000..b5803f14f
--- /dev/null
+++ b/algorithms/000179-largest-number/README.md
@@ -0,0 +1,79 @@
+179\. Largest Number
+
+**Difficulty:** Medium
+
+**Topics:** `Array`, `String`, `Greedy`, `Sorting`
+
+Given a list of non-negative integers `nums`, arrange them such that they form the largest number and return it.
+
+Since the result may be very large, so you need to return a string instead of an integer.
+
+**Example 1:**
+
+- **Input:** nums = [10,2]
+- **Output:** "210"
+
+**Example 2:**
+
+- **Input:** nums = [3,30,34,5,9]
+- **Output:** "9534330"
+
+
+**Constraints:**
+
+- 1 <= nums.length <= 100
+- 0 <= nums[i] <= 109
+
+
+**Solution:**
+
+We need to compare numbers based on their concatenated results. For two numbers `a` and `b`, we compare `ab` (a concatenated with b) and `ba` (b concatenated with a), and decide the order based on which forms a larger number.
+
+### Approach:
+1. **Custom Sorting**: Implement a custom comparator function that sorts the numbers by comparing the concatenated results.
+2. **Edge Case**: If the largest number after sorting is `0`, then the result is `"0"`, as all numbers must be zero.
+3. **Concatenation**: After sorting, concatenate the numbers to form the final result.
+
+Let's implement this solution in PHP: **[179. Largest Number](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/000179-largest-number/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+1. **`usort($nums, $comparator)`**: We sort the array using a custom comparator. For each pair of numbers `a` and `b`, we compare the concatenated strings `a . b` and `b . a`.
+2. **Comparison Logic**: The `strcmp($order2, $order1)` ensures that we get a descending order based on the concatenated strings.
+3. **Edge Case Handling**: If the first character of the resulting concatenated string is `0`, we return `"0"`, which happens when all elements of the array are zeros.
+4. **Time Complexity**: Sorting the numbers takes `O(n log n)`, and concatenating them takes `O(n)`, where `n` is the number of numbers in the input array.
+
+This solution handles the constraints efficiently and returns the largest possible number as a string.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
diff --git a/algorithms/000179-largest-number/solution.php b/algorithms/000179-largest-number/solution.php
new file mode 100644
index 000000000..5784e320f
--- /dev/null
+++ b/algorithms/000179-largest-number/solution.php
@@ -0,0 +1,23 @@
+numIslands($grid1) . "\n"; // Output: 1
+echo "Output for grid2: " . $solution->numIslands($grid2) . "\n"; // Output: 3
+?>
+```
+
+### Explanation:
+
+1. **Class and Method Definitions:**
+ - `class Solution` defines the class that contains the methods for solving the problem.
+ - `numIslands` is the main method to count the number of islands.
+
+2. **`numIslands` Method:**
+ - **Parameters:** Takes a 2D grid (`$grid`) where each cell is either '1' (land) or '0' (water).
+ - **Validation:** Checks if the grid is empty. If so, it returns 0.
+ - **Initialization:** Defines the dimensions of the grid (`$m` for rows and `$n` for columns) and initializes the island count.
+ - **Traversal:** Iterates through each cell in the grid:
+ - **Land Cell Check:** If a cell contains '1', it starts a DFS from that cell and increments the island count.
+ - **DFS Call:** Calls the `dfs` method to mark all connected land cells as visited.
+ - **Return:** Returns the total number of islands found.
+
+3. **`dfs` Method:**
+ - **Parameters:** Takes a reference to the grid (`$grid`), and coordinates (`$x`, `$y`), and the dimensions of the grid (`$m`, `$n`).
+ - **Base Case:** Checks if the current cell is out of bounds or if it is not land ('1'). If so, it returns immediately.
+ - **Marking:** Marks the current land cell as visited by setting it to 'x'.
+ - **Recursive Calls:** Recursively performs DFS in all four possible directions (up, down, left, right) to visit all connected land cells.
+
+### How It Works
+
+- **Initialization:** The `numIslands` method initializes the island count and begins iterating over each cell in the grid.
+- **Island Detection:** Whenever a '1' is encountered, it signifies a new island. The `dfs` method is invoked to explore and mark the entire island, ensuring that all connected land cells are considered part of the same island.
+- **DFS Traversal:** The `dfs` method ensures that each cell in an island is visited exactly once, marking it as visited and preventing it from being counted again in future DFS calls.
+- **Counting Islands:** The main method counts each distinct DFS call initiation as one island and returns the total count.
+
+This approach efficiently counts the number of islands by exploring each land cell and marking all connected cells, ensuring that each island is counted only once.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
diff --git a/algorithms/000205-isomorphic-strings/README.md b/algorithms/000205-isomorphic-strings/README.md
index a8e02d07f..7940665a4 100644
--- a/algorithms/000205-isomorphic-strings/README.md
+++ b/algorithms/000205-isomorphic-strings/README.md
@@ -1,6 +1,8 @@
205\. Isomorphic Strings
-Easy
+**Difficulty:** Easy
+
+**Topics:** `Hash Table`, `String`
Given two strings `s` and `t`, _determine if they are isomorphic_.
@@ -29,4 +31,50 @@ All occurrences of a character must be replaced with another character while pre
- t.length == s.length
- `s` and `t` consist of any valid ascii character.
-**Follow-up:** Can you come up with an algorithm that is less than O(n2)
time complexity?
\ No newline at end of file
+**Follow-up:** Can you come up with an algorithm that is less than O(n2)
time complexity?
+
+
+**Solution:**
+
+
+We can use two hash maps (or associative arrays in PHP) to track the mappings of characters from `s` to `t` and vice versa.
+
+Let's implement this solution in PHP: **[205. Isomorphic Strings](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/000205-isomorphic-strings/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+1. **Length Check:** First, we check if the lengths of the two strings are equal. If not, they cannot be isomorphic.
+
+2. **Mapping Creation:** We use two associative arrays, `$mapST` and `$mapTS`, to track the mappings:
+ - `$mapST` stores the mapping from characters in `s` to characters in `t`.
+ - `$mapTS` stores the mapping from characters in `t` to characters in `s`.
+
+3. **Iteration:**
+ - We iterate through each character in the strings `s` and `t`.
+ - If a mapping already exists, we check if it is consistent. If not, we return `false`.
+ - If no mapping exists, we create a new one.
+
+4. **Return:** If we successfully iterate through the entire string without finding any inconsistencies, we return `true`, indicating that the strings are isomorphic.
+
+This approach runs in O(n) time complexity, where n is the length of the strings, making it efficient for the input constraints.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
+
diff --git a/algorithms/000214-shortest-palindrome/README.md b/algorithms/000214-shortest-palindrome/README.md
new file mode 100644
index 000000000..48d8d73cd
--- /dev/null
+++ b/algorithms/000214-shortest-palindrome/README.md
@@ -0,0 +1,141 @@
+214\. Shortest Palindrome
+
+**Difficulty:** Hard
+
+**Topics:** `String`, `Rolling Hash`, `String Matching`, `Hash Function`
+
+You are given a string `s`. You can convert `s` to a palindrome[^1] by adding characters in front of it.
+
+Return _the shortest palindrome you can find by performing this transformation_.
+
+**Example 1:**
+
+- **Input:** s = "aacecaaa"
+- **Output:** "aaacecaaa"
+
+**Example 2:**
+
+- **Input:** s = "abcd"
+- **Output:** "dcbabcd"
+
+
+**Constraints:**
+
+- 0 <= s.length <= 5 * 104
+- `s` consists of lowercase English letters only.
+
+
+[^1]: **Palindrome** A **palindrome** is a string that reads the same forward and backward.
+
+
+**Solution:**
+
+We need to find the shortest palindrome by adding characters in front of a given string. We can approach this by identifying the longest prefix of the string that is already a palindrome. Once we have that, the remaining part can be reversed and added to the front to make the entire string a palindrome.
+
+### Approach:
+1. **Identify the longest palindromic prefix**: We want to find the longest prefix of the string `s` that is already a palindrome.
+2. **Reverse the non-palindromic suffix**: The part of the string that isn't part of the palindromic prefix is reversed and added to the beginning of the string.
+3. **Concatenate the reversed suffix and the original string** to form the shortest palindrome.
+
+To do this efficiently, we can use string matching techniques such as the **KMP (Knuth-Morris-Pratt) algorithm** to find the longest palindromic prefix.
+
+### Step-by-Step Solution:
+
+1. **Create a new string** by appending the reverse of the string `s` to `s` itself, separated by a special character `'#'` to avoid overlap between the string and its reverse.
+
+ Let the new string be `s + '#' + reverse(s)`.
+
+2. **Use the KMP partial match table** (also known as the LPS array, longest prefix suffix) on this new string to determine the longest prefix of `s` that is also a suffix. This will give us the length of the longest palindromic prefix in `s`.
+
+3. **Construct the shortest palindrome** by adding the necessary characters to the front.
+
+Let's implement this solution in PHP: **[214. Shortest Palindrome](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/000214-shortest-palindrome/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+1. **computeLPS Function**:
+ - This function computes the longest prefix which is also a suffix (LPS) for the given string. This helps to identify how much of the string `s` is already a palindrome.
+
+2. **Forming the Combined String**:
+ - We combine the original string `s` with its reverse and separate them by a special character `#`. The special character ensures that there is no overlap between `s` and `reverse(s)`.
+
+3. **Using the LPS Array**:
+ - The LPS array tells us the longest prefix in `s` which is also a suffix of `s`. This corresponds to the longest part of the string that is already a palindrome.
+
+4. **Constructing the Shortest Palindrome**:
+ - We find the portion of the string that isn't part of the longest palindromic prefix and reverse it. Then, we add the reversed part to the front of the original string `s`.
+
+### Time Complexity:
+- The time complexity of this solution is **O(n)**, where `n` is the length of the string. This is because we are using the KMP algorithm, which runs in linear time.
+
+### Example Walkthrough:
+
+#### Example 1:
+Input: `s = "aacecaaa"`
+
+1. Reverse of `s`: `"aaacecaa"`
+2. Combined string: `"aacecaaa#aaacecaa"`
+3. LPS array for the combined string gives the longest palindromic prefix as `"aacecaaa"`.
+
+Since the whole string is already a palindrome, no characters need to be added. So the output is `"aaacecaaa"`.
+
+#### Example 2:
+Input: `s = "abcd"`
+
+1. Reverse of `s`: `"dcba"`
+2. Combined string: `"abcd#dcba"`
+3. LPS array tells us that the longest palindromic prefix is just `"a"`.
+
+So, we add `"dcb"` (reverse of `"bcd"`) to the front of `s`, resulting in `"dcbabcd"`.
+
+### Conclusion:
+This solution efficiently finds the shortest palindrome by leveraging string matching techniques and the KMP algorithm to identify the longest palindromic prefix. The complexity is linear, making it suitable for large input sizes up to `5 * 10^4`.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
diff --git a/algorithms/000214-shortest-palindrome/solution.php b/algorithms/000214-shortest-palindrome/solution.php
new file mode 100644
index 000000000..ef3de369c
--- /dev/null
+++ b/algorithms/000214-shortest-palindrome/solution.php
@@ -0,0 +1,62 @@
+computeLPS($combined);
+
+ // Length of the longest palindromic prefix in s
+ $longest_palindromic_prefix_len = $lps[strlen($combined) - 1];
+
+ // The characters that need to be added to the front are the remaining part of the string
+ $remaining = substr($s, $longest_palindromic_prefix_len);
+
+ // Add the reverse of the remaining part to the front of s
+ return strrev($remaining) . $s;
+ }
+
+ /**
+ * Helper function to compute the KMP (LPS array) for string matching
+ *
+ * @param $pattern
+ * @return array
+ */
+ function computeLPS($pattern) {
+ $n = strlen($pattern);
+ $lps = array_fill(0, $n, 0);
+ $len = 0;
+ $i = 1;
+
+ while ($i < $n) {
+ if ($pattern[$i] == $pattern[$len]) {
+ $len++;
+ $lps[$i] = $len;
+ $i++;
+ } else {
+ if ($len != 0) {
+ $len = $lps[$len - 1];
+ } else {
+ $lps[$i] = 0;
+ $i++;
+ }
+ }
+ }
+ return $lps;
+ }
+}
\ No newline at end of file
diff --git a/algorithms/000237-delete-node-in-a-linked-list/README.md b/algorithms/000237-delete-node-in-a-linked-list/README.md
index 3ff058d4a..5151f4ee3 100644
--- a/algorithms/000237-delete-node-in-a-linked-list/README.md
+++ b/algorithms/000237-delete-node-in-a-linked-list/README.md
@@ -1,6 +1,8 @@
237\. Delete Node in a Linked List
-Medium
+**Difficulty:** Medium
+
+**Topics:** `Linked List`
There is a singly-linked list `head` and we want to delete a node `node` in it.
@@ -44,4 +46,84 @@ Custom testing:
- The number of the nodes in the given list is in the range `[2, 1000]`.
- `-1000 <= Node.val <= 1000`
- The value of each node in the list is **unique**.
-- The `node` to be deleted is in the list and is **not a tail** node.
\ No newline at end of file
+- The `node` to be deleted is in the list and is **not a tail** node.
+
+
+**Solution:**
+
+
+We need to effectively delete the given node by modifying the list in place. The key idea here is to copy the value from the next node into the current node and then skip over the next node.
+
+### Steps to solve the problem:
+
+1. **Copy the value of the next node** into the given node.
+2. **Update the `next` pointer** of the given node to skip over the next node (effectively removing the next node from the list).
+
+Let's implement this solution in PHP: **[237. Delete Node in a Linked List](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/000237-delete-node-in-a-linked-list/solution.php)**
+
+```php
+val = $val;
+ $this->next = $next;
+ }
+}
+
+function deleteNode($node) {
+ // go to solution.php
+}
+
+// Example usage:
+// Create the linked list: 4 -> 5 -> 1 -> 9
+$head = new ListNode(4);
+$head->next = new ListNode(5);
+$head->next->next = new ListNode(1);
+$head->next->next->next = new ListNode(9);
+
+// Assuming we want to delete node with value 5
+$nodeToDelete = $head->next;
+
+// Call the function to delete the node
+deleteNode($nodeToDelete);
+
+// Output the list to see the result: 4 -> 1 -> 9
+$current = $head;
+while ($current !== null) {
+ echo $current->val . " ";
+ $current = $current->next;
+}
+?>
+```
+
+### Explanation:
+
+- **Step 1: Copy the value from the next node.**
+ - We assign the value of the next node (`$node->next->val`) to the current node (`$node->val`).
+- **Step 2: Update the next pointer.**
+ - We update the `next` pointer of the current node to point to the node after the next one (`$node->next = $node->next->next`), effectively removing the next node from the list.
+
+### Example:
+
+For the input linked list `4 -> 5 -> 1 -> 9` and the node to delete with value `5`:
+- The value `5` in the node is replaced with the value `1` (from the next node).
+- The list is updated to `4 -> 1 -> 9`, effectively deleting the node with the original value `5`.
+
+### Output:
+
+- **Input:** `head = [4,5,1,9]`, `node = 5`
+- **Output:** `4 -> 1 -> 9`
+
+This solution efficiently deletes the node in the linked list without the need to traverse the list from the head, adhering to the problem's constraints.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
diff --git a/algorithms/000237-delete-node-in-a-linked-list/solution.php b/algorithms/000237-delete-node-in-a-linked-list/solution.php
index 88bb45a1b..5c0d81e51 100644
--- a/algorithms/000237-delete-node-in-a-linked-list/solution.php
+++ b/algorithms/000237-delete-node-in-a-linked-list/solution.php
@@ -23,7 +23,10 @@ class Solution
*/
function deleteNode(ListNode $node): void
{
+ // Step 1: Copy the value from the next node into the current node
$node->val = $node->next->val;
+
+ // Step 2: Point the current node's next to the next node's next
$node->next = $node->next->next;
}
}
\ No newline at end of file
diff --git a/algorithms/000241-different-ways-to-add-parentheses/README.md b/algorithms/000241-different-ways-to-add-parentheses/README.md
new file mode 100644
index 000000000..74b7e05fd
--- /dev/null
+++ b/algorithms/000241-different-ways-to-add-parentheses/README.md
@@ -0,0 +1,126 @@
+241\. Different Ways to Add Parentheses
+
+**Difficulty:** Medium
+
+**Topics:** `Math`, `String`, `Dynamic Programming`, `Recursion`, `Memoization`
+
+Given a string `expression` of numbers and operators, return _all possible results from computing all the different possible ways to group numbers and operators_. You may return the answer in **any order**.
+
+The test cases are generated such that the output values fit in a 32-bit integer and the number of different results does not exceed 104
.
+
+**Example 1:**
+
+- **Input:** expression = "2-1-1"
+- **Output:** [0,2]
+- **Explanation:**\
+ ((2-1)-1) = 0\
+ (2-(1-1)) = 2
+
+**Example 2:**
+
+- **Input:** expression = "2*3-4*5"
+- **Output:** [-34,-14,-10,-10,10]
+- **Explanation:**\
+ (2*(3-(4*5))) = -34\
+ ((2*3)-(4*5)) = -14\
+ ((2*(3-4))*5) = -10\
+ (2*((3-4)*5)) = -10\
+ (((2*3)-4)*5) = 10
+
+
+**Constraints:**
+
+- 1 <= expression.length <= 20
+- `expression` consists of digits and the operator `'+'`, `'-'`, and `'*'`.
+- All the integer values in the input expression are in the range `[0, 99]`.
+- The integer values in the input expression do not have a leading `'-'` or `'+'` denoting the sign.
+
+
+**Solution:**
+
+We can use **recursion** combined with **memoization** to store previously computed results for sub-expressions, as this avoids redundant calculations and optimizes the solution.
+
+### Approach:
+1. **Recursion**:
+ - For each operator in the string (`+`, `-`, `*`), we split the expression at that operator.
+ - Recursively compute the left and right parts of the expression.
+ - Combine the results of both parts using the operator.
+
+2. **Memoization**:
+ - Store the results of sub-expressions in an associative array to avoid recalculating the same sub-expression multiple times.
+
+3. **Base Case**:
+ - If the expression contains only a number (i.e., no operators), return that number as the result.
+
+### Example Walkthrough:
+For the input `"2*3-4*5"`:
+- Split at `*` -> `2` and `3-4*5`
+ - Recursively compute `3-4*5` and `2`, then combine.
+- Split at `-` -> `2*3` and `4*5`
+ - Recursively compute each and combine.
+- Similarly for other splits.
+
+Let's implement this solution in PHP: **[241. Different Ways to Add Parentheses](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/000241-different-ways-to-add-parentheses/solution.php)**
+
+```php
+diffWaysToCompute($expression1)); // Output: [0, 2]
+print_r($solution->diffWaysToCompute($expression2)); // Output: [-34, -14, -10, -10, 10]
+?>
+```
+
+### Explanation:
+
+1. **Memoization**: The `$memo` array stores the computed results for each expression to avoid redundant calculations.
+2. **Base Case**: When the expression is numeric, itβs converted to an integer and added to the results.
+3. **Recursive Splitting**: For each operator in the expression, split it into left and right parts, recursively compute the results for both parts, and then combine them based on the operator.
+4. **Example Usage**: The `diffWaysToCompute` function is tested with example expressions to verify the solution.
+
+This approach ensures that you efficiently compute all possible results by leveraging memoization to avoid redundant computations.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
diff --git a/algorithms/000241-different-ways-to-add-parentheses/solution.php b/algorithms/000241-different-ways-to-add-parentheses/solution.php
new file mode 100644
index 000000000..fa0f2215f
--- /dev/null
+++ b/algorithms/000241-different-ways-to-add-parentheses/solution.php
@@ -0,0 +1,69 @@
+compute($expression);
+ }
+
+ /**
+ * @param $expression
+ * @return array|mixed
+ */
+ private function compute($expression) {
+ // Check if the result for this expression is already computed
+ if (isset($this->memo[$expression])) {
+ return $this->memo[$expression];
+ }
+
+ $results = [];
+
+ // Check if the expression is a single number
+ if (is_numeric($expression)) {
+ $results[] = (int)$expression;
+ } else {
+ // Iterate through the expression
+ for ($i = 0; $i < strlen($expression); $i++) {
+ $char = $expression[$i];
+
+ // If the character is an operator
+ if ($char == '+' || $char == '-' || $char == '*') {
+ // Split the expression into two parts
+ $left = substr($expression, 0, $i);
+ $right = substr($expression, $i + 1);
+
+ // Compute all results for left and right parts
+ $leftResults = $this->compute($left);
+ $rightResults = $this->compute($right);
+
+ // Combine the results based on the operator
+ foreach ($leftResults as $leftResult) {
+ foreach ($rightResults as $rightResult) {
+ if ($char == '+') {
+ $results[] = $leftResult + $rightResult;
+ } elseif ($char == '-') {
+ $results[] = $leftResult - $rightResult;
+ } elseif ($char == '*') {
+ $results[] = $leftResult * $rightResult;
+ }
+ }
+ }
+ }
+ }
+ }
+
+ // Memoize the result for the current expression
+ $this->memo[$expression] = $results;
+ return $results;
+ }
+}
\ No newline at end of file
diff --git a/algorithms/000260-single-number-iii/README.md b/algorithms/000260-single-number-iii/README.md
index 94d7934b3..91b1e6df7 100644
--- a/algorithms/000260-single-number-iii/README.md
+++ b/algorithms/000260-single-number-iii/README.md
@@ -1,6 +1,8 @@
260\. Single Number III
-Medium
+**Difficulty:** Medium
+
+**Topics:** `Array`, `Bit Manipulation`
Given an integer array **nums**, in which exactly two elements appear only once and all the other elements appear exactly twice. Find the two elements that appear only once. You can return the answer in **any order**.
@@ -26,4 +28,54 @@ You must write an algorithm that runs in linear runtime complexity and uses only
- 2 <= nums.length <= 3 * 104
- -231 <= nums[i] <= 231 - 1
-- Each integer in **nums** will appear twice, only two integers will appear once.
\ No newline at end of file
+- Each integer in **nums** will appear twice, only two integers will appear once.
+
+
+**Solution:**
+
+
+We can use bit manipulation, specifically the XOR operation. The key idea is that XORing two identical numbers results in 0, and XORing a number with 0 gives the number itself. Using this property, we can find the two unique numbers that appear only once in the array.
+
+### Step-by-Step Solution:
+
+1. **XOR all elements** in the array. The result will be the XOR of the two unique numbers because all other numbers will cancel out (since they appear twice).
+
+2. **Find a set bit** in the XOR result (this bit will be different between the two unique numbers). You can isolate the rightmost set bit using `xor & (-xor)`.
+
+3. **Partition the array** into two groups based on the set bit. XOR all numbers in each group to find the two unique numbers.
+
+Let's implement this solution in PHP: **[260. Single Number III](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/000260-single-number-iii/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+- **Step 1:** The XOR operation eliminates the numbers that appear twice and results in `xor` being the XOR of the two unique numbers.
+- **Step 2:** The rightmost set bit is found, which helps in distinguishing the two unique numbers.
+- **Step 3:** The array is partitioned into two groups: one with the set bit and one without. XORing the numbers in each group gives the two unique numbers.
+
+This solution runs in O(n) time and uses O(1) extra space, meeting the problem's requirements.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
diff --git a/algorithms/000260-single-number-iii/solution.php b/algorithms/000260-single-number-iii/solution.php
index 39a25b0f8..64f32bb33 100644
--- a/algorithms/000260-single-number-iii/solution.php
+++ b/algorithms/000260-single-number-iii/solution.php
@@ -7,20 +7,27 @@ class Solution {
* @return Integer[]
*/
function singleNumber($nums) {
- $xors = array_reduce($nums, function($carry, $item) { return $carry ^ $item; }, 0);
- $lowbit = $xors & -$xors;
- $ans = array_fill(0, 2, 0);
+ // Step 1: XOR all elements
+ $xor = 0;
+ foreach ($nums as $num) {
+ $xor ^= $num;
+ }
+
+ // Step 2: Find a set bit (rightmost set bit in this case)
+ $rightmost_set_bit = $xor & (-$xor);
- // Seperate `nums` into two groups by `lowbit`.
+ // Step 3: Partition the array into two groups and XOR them
+ $num1 = 0;
+ $num2 = 0;
foreach ($nums as $num) {
- if ($num & $lowbit) {
- $ans[0] ^= $num;
+ if (($num & $rightmost_set_bit) == 0) {
+ $num1 ^= $num;
} else {
- $ans[1] ^= $num;
+ $num2 ^= $num;
}
}
- return $ans;
+ return [$num1, $num2];
}
-}
+}
\ No newline at end of file
diff --git a/algorithms/000264-ugly-number-ii/README.md b/algorithms/000264-ugly-number-ii/README.md
new file mode 100644
index 000000000..9086afb2f
--- /dev/null
+++ b/algorithms/000264-ugly-number-ii/README.md
@@ -0,0 +1,68 @@
+264\. Ugly Number II
+
+**Difficulty:** Medium
+
+**Topics:** `Hash Table`, `Math`, `Dynamic Programming`, `Heap (Priority Queue)`
+
+An **ugly number** is a positive integer whose prime factors are limited to `2`, `3`, and `5`.
+
+Given an integer `n`, return _the nth
**ugly number**_.
+
+**Example 1:**
+
+- **Input:** n = 10
+- **Output:** 12
+- **Explanation:** [1, 2, 3, 4, 5, 6, 8, 9, 10, 12] is the sequence of the first 10 ugly numbers.
+
+**Example 2:**
+
+- **Input:** n = 1
+- **Output:** 1
+- **Explanation:** 1 has no prime factors, therefore all of its prime factors are limited to 2, 3, and 5.
+
+**Constraints:**
+
+- 1 <= n <= 1690
+
+**Hint:**
+1. The naive approach is to call `isUgly` for every number until you reach the nth
one. Most numbers are _not_ ugly. Try to focus your effort on generating only the ugly ones.
+2. An ugly number must be multiplied by either 2, 3, or 5 from a smaller ugly number.
+3. The key is how to maintain the order of the ugly numbers. Try a similar approach of merging from three sorted lists: L1, L2, and L3.
+4. Assume you have Uk, the kth ugly number. Then Uk+1 must be Min(L1 * 2, L2 * 3, L3 * 5).
+
+
+**Solution:**
+
+
+We can use a dynamic programming approach. We'll maintain an array to store the ugly numbers and use three pointers to multiply by 2, 3, and 5.
+
+Let's implement this solution in PHP: **[264. Ugly Number II](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/000264-ugly-number-ii/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+- **Dynamic Programming Array:** We maintain an array `uglyNumbers` where each element corresponds to the next ugly number in the sequence.
+- **Pointers (`i2`, `i3`, `i5`):** These pointers track the current position in the sequence for multiplying by 2, 3, and 5, respectively.
+- **Next Multiples (`next2`, `next3`, `next5`):** These variables hold the next potential multiples of 2, 3, and 5.
+- **Main Loop:** We iterate from 1 to `n-1`, and in each iteration, we determine the next ugly number by taking the minimum of `next2`, `next3`, and `next5`. Depending on which value is the minimum, we update the corresponding pointer and calculate the next multiple.
+- **Return:** Finally, we return the nth ugly number.
+
+This solution ensures that we generate the sequence of ugly numbers efficiently, focusing only on valid ugly numbers and skipping non-ugly numbers.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
diff --git a/algorithms/000264-ugly-number-ii/solution.php b/algorithms/000264-ugly-number-ii/solution.php
new file mode 100644
index 000000000..aeb30476c
--- /dev/null
+++ b/algorithms/000264-ugly-number-ii/solution.php
@@ -0,0 +1,39 @@
+ai != bi
- All the pairs (ai, bi)
are distinct.
- The given input is **guaranteed** to be a tree and there will be **no repeated** edges.
+
+**Hint:**
+1. How many MHTs can a graph have at most?
+
+
+
+
+**Solution:**
+
+We can use the concept of graph theory to find nodes that, when chosen as roots, minimize the height of the tree. The basic idea is to perform a two-pass Breadth-First Search (BFS) to find these nodes efficiently.
+
+### Steps to Solve the Problem
+
+1. **Build the Graph**: Represent the tree using an adjacency list. Each node will have a list of its connected nodes.
+
+2. **Find the Leaf Nodes**: Leaf nodes are nodes with only one connection.
+
+3. **Trim the Leaves**: Iteratively remove the leaf nodes and their corresponding edges from the graph until only one or two nodes remain. These remaining nodes are the potential roots for the minimum height trees.
+
+4. **Return the Result**: The remaining nodes after trimming are the roots of minimum height trees.
+
+Let's implement this solution in PHP: **[310. Minimum Height Trees](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/000310-minimum-height-trees/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+1. **Graph Construction**: The adjacency list is constructed to represent the tree.
+
+2. **Initial Leaves Identification**: Nodes with only one neighbor are identified as leaves.
+
+3. **Layer-by-Layer Trimming**: Each layer of leaves is removed, updating the graph, and new leaves are identified until only 1 or 2 nodes are left.
+
+4. **Result**: The remaining nodes are the roots that yield the minimum height trees.
+
+This solution efficiently computes the minimum height trees in O(n) time complexity, which is suitable for large values of `n` up to 20,000.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
+
+
diff --git a/algorithms/000310-minimum-height-trees/solution.php b/algorithms/000310-minimum-height-trees/solution.php
index de2dac081..5235a32c7 100644
--- a/algorithms/000310-minimum-height-trees/solution.php
+++ b/algorithms/000310-minimum-height-trees/solution.php
@@ -7,8 +7,7 @@ class Solution {
* @param Integer[][] $edges
* @return Integer[]
*/
- function findMinHeightTrees(int $n, array $edges): array
- {
+ function findMinHeightTrees($n, $edges) {
if ($n == 1 || empty($edges)) {
return [0];
}
@@ -16,6 +15,7 @@ function findMinHeightTrees(int $n, array $edges): array
$ans = [];
$graph = [];
+ // Build the adjacency list
foreach ($edges as $edge) {
$u = $edge[0];
$v = $edge[1];
@@ -23,18 +23,22 @@ function findMinHeightTrees(int $n, array $edges): array
$graph[$v][] = $u;
}
+ // Initialize leaves
foreach ($graph as $label => $children) {
if (count($children) == 1) {
$ans[] = $label;
}
}
+ // Remove leaves layer by layer
while ($n > 2) {
$n -= count($ans);
$nextLeaves = [];
foreach ($ans as $leaf) {
- $u = current($graph[$leaf]);
+ $u = current($graph[$leaf]); // There is only one neighbor since it's a leaf
+ // Remove the leaf from its neighbor's adjacency list
unset($graph[$u][array_search($leaf, $graph[$u])]);
+ // If neighbor becomes a leaf, add it to newLeaves
if (count($graph[$u]) == 1) {
$nextLeaves[] = $u;
}
diff --git a/algorithms/000330-patching-array/README.md b/algorithms/000330-patching-array/README.md
index ca21393ce..d07fc563a 100644
--- a/algorithms/000330-patching-array/README.md
+++ b/algorithms/000330-patching-array/README.md
@@ -1,6 +1,8 @@
330\. Patching Array
-Hard
+**Difficulty:** Hard
+
+**Topics:** `Array`, `Greedy`
Given a sorted integer array `nums` and an integer `n`, add/patch elements to the array such that any number in the range `[1, n]` inclusive can be formed by the sum of some elements in the array.
@@ -35,4 +37,79 @@ Return _the minimum number of patches required_.
- 1 <= n <= 231 - 1
- **Only one valid answer exists.**
-**Follow-up:** Can you come up with an algorithm that is less than O(n2)
time complexity?
\ No newline at end of file
+
+**Solution:**
+
+We need to ensure that every number in the range `[1, n]` can be formed by the sum of some elements in the given array. We can use a greedy algorithm to determine the minimum number of patches required.
+
+### Solution Explanation:
+
+1. **Key Insight**:
+ - Start with the smallest missing number `miss` which starts at 1.
+ - Iterate through the array `nums`. If the current number in `nums` is less than or equal to `miss`, then `miss` can be covered by adding this number. Otherwise, we need to patch `miss` to the array, effectively doubling the range that can be covered.
+
+2. **Algorithm**:
+ - Initialize `miss = 1` and `patches = 0`.
+ - Loop through the array while `miss <= n`:
+ - If the current number in `nums` can cover `miss`, move to the next number in `nums`.
+ - If the current number is greater than `miss`, patch `miss` and double the range covered by setting `miss = miss * 2`.
+ - Continue until the entire range `[1, n]` is covered.
+
+3. **Complexity**:
+ - The time complexity is O(m + log n), where `m` is the size of the array `nums`.
+
+
+Let's implement this solution in PHP: **[330. Patching Array](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/000330-patching-array/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+- **Initial Setup**: We initialize `miss` as 1, meaning we want to ensure we can generate the number 1.
+- **While Loop**: We loop until `miss` exceeds `n` (the maximum number we need to cover). In each iteration:
+ - If the current number in `nums` can help cover `miss`, we add it to `miss` and move to the next number.
+ - If not, we "patch" the array by effectively adding `miss` to it (without changing the array itself) and then update `miss` to cover the new range.
+- **Output**: The total number of patches required is returned.
+
+This algorithm ensures that we cover the entire range `[1, n]` with the minimum number of patches.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
\ No newline at end of file
diff --git a/algorithms/000330-patching-array/solution.php b/algorithms/000330-patching-array/solution.php
index 06c11780e..4e4026ae4 100644
--- a/algorithms/000330-patching-array/solution.php
+++ b/algorithms/000330-patching-array/solution.php
@@ -8,21 +8,19 @@ class Solution {
* @return Integer
*/
function minPatches($nums, $n) {
- $ans = 0;
- $i = 0; // nums' index
- $miss = 1; // the minimum sum in [1, n] we might miss
-
+ $ans = 0; // Number of patches added
+ $i = 0; // Index in the array nums
+ $miss = 1; // The smallest number that we can't form yet
while ($miss <= $n) {
if ($i < count($nums) && $nums[$i] <= $miss) {
+ // If nums[i] is within the range we need to cover
$miss += $nums[$i++];
} else {
- // Greedily add `miss` itself to increase the range from
- // [1, miss) to [1, 2 * miss).
- $miss += $miss;
+ // If nums[i] is greater than the current miss, we need to patch
+ $miss += $miss; // Add `miss` itself to cover the missing number
++$ans;
}
}
-
return $ans;
}
}
\ No newline at end of file
diff --git a/algorithms/000344-reverse-string/README.md b/algorithms/000344-reverse-string/README.md
index 9a041b89c..1cd3e9b22 100644
--- a/algorithms/000344-reverse-string/README.md
+++ b/algorithms/000344-reverse-string/README.md
@@ -1,6 +1,8 @@
344\. Reverse String
-Easy
+**Difficulty:** Easy
+
+**Topics:** `Two Pointers`, `String`
Write a function that reverses a string. The input string is given as an array of characters `s`.
@@ -19,4 +21,67 @@ You must do this by modifying the input array [in-place](https://en.wikipedia.or
**Constraints:**
- 21 <= s.length <= 105
-- `s[i]` is a [printable ascii character](https://en.wikipedia.org/wiki/ASCII#Printable_characters).
\ No newline at end of file
+- `s[i]` is a [printable ascii character](https://en.wikipedia.org/wiki/ASCII#Printable_characters).
+
+**Hint:**
+1. The entire logic for reversing a string is based on using the opposite directional two-pointer approach!
+
+
+**Solution:**
+
+To solve this problem, we can follow these steps:
+
+Let's implement this solution in PHP: **[344. Reverse String](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/000344-reverse-string/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+1. **Initialization**:
+ - Set two pointers: `left` starting from the beginning (`0`) and `right` from the end (`count($s) - 1`).
+
+2. **Swapping**:
+ - Swap the elements at `left` and `right` positions.
+ - Increment the `left` pointer and decrement the `right` pointer after each swap.
+
+3. **Loop**:
+ - Continue the swapping until `left` is less than `right`.
+
+4. **In-Place Modification**:
+ - The input array `$s` is modified in place, meaning the original array is reversed without using any additional arrays.
+
+This solution has a time complexity of `O(n)` and a space complexity of `O(1)`, as required.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
diff --git a/algorithms/000344-reverse-string/solution.php b/algorithms/000344-reverse-string/solution.php
index 56d8d81e2..6a65e15cb 100644
--- a/algorithms/000344-reverse-string/solution.php
+++ b/algorithms/000344-reverse-string/solution.php
@@ -7,13 +7,18 @@ class Solution {
* @return NULL
*/
function reverseString(&$s) {
- $l = 0;
- $r = count($s) - 1;
+ $left = 0;
+ $right = count($s) - 1;
- while ($l < $r) {
- list($s[$l], $s[$r]) = array($s[$r], $s[$l]);
- $l++;
- $r--;
+ while ($left < $right) {
+ // Swap the characters
+ $temp = $s[$left];
+ $s[$left] = $s[$right];
+ $s[$right] = $temp;
+
+ // Move the pointers
+ $left++;
+ $right--;
}
}
}
\ No newline at end of file
diff --git a/algorithms/000350-intersection-of-two-arrays-ii/README.md b/algorithms/000350-intersection-of-two-arrays-ii/README.md
index 4d55860fd..4c50c5f41 100644
--- a/algorithms/000350-intersection-of-two-arrays-ii/README.md
+++ b/algorithms/000350-intersection-of-two-arrays-ii/README.md
@@ -1,6 +1,8 @@
350\. Intersection of Two Arrays II
-Easy
+**Difficulty:** Easy
+
+**Topics:** `Array`, `Hash Table`, `Two Pointers`, `Binary Search`, `Sorting`
Given two integer arrays `nums1` and `nums2`, return _an array of their intersection_. Each element in the result must appear as many times as it shows in both arrays, and you may return the result in **any order**.
@@ -20,3 +22,75 @@ Given two integer arrays `nums1` and `nums2`, return _an array of their intersec
- 1 <= nums1.length, nums2.length <= 1000
- 0 <= nums1[i], nums2[i] <= 1000
+**Follow up:**
+
+- What if the given array is already sorted? How would you optimize your algorithm?
+- What if nums1's size is small compared to nums2's size? Which algorithm is better?
+- What if elements of nums2 are stored on disk, and the memory is limited such that you cannot load all elements into the memory at once?
+
+
+**Solution:**
+
+
+To solve this problem, we can follow these steps:
+
+Let's implement this solution in PHP: **[350. Intersection of Two Arrays II](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/000350-intersection-of-two-arrays-ii/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+1. **Counting Occurrences**: The solution uses a hash table (`$counts`) to store the count of each number in `nums1`.
+
+2. **Finding the Intersection**: As we iterate through `nums2`, we check if the current number exists in the `$counts` array and has a non-zero count. If so, it's added to the result array, and we decrement the count for that number in `$counts`.
+
+3. **Output**:
+ - For the first example, the output will be `[2, 2]`.
+ - For the second example, the output could be `[4, 9]` or `[9, 4]`, as the order does not matter.
+
+### Follow-Up Considerations:
+1. **Sorted Arrays**: If `nums1` and `nums2` are already sorted, you can use two pointers to traverse both arrays and find the intersection in a single pass.
+
+2. **Small `nums1` Compared to `nums2`**: If `nums1` is smaller, store its elements in a hash table and then iterate through `nums2` to find the intersection. This minimizes the space complexity.
+
+3. **Handling Large `nums2` on Disk**: If `nums2` is too large to fit into memory, you can either:
+ - Sort `nums2` and use a binary search for each element in `nums1`.
+ - Process `nums2` in chunks, updating the hash table for the intersection on the fly.
+
+This approach is efficient for the given problem constraints.
+
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
+
+
diff --git a/algorithms/000386-lexicographical-numbers/README.md b/algorithms/000386-lexicographical-numbers/README.md
new file mode 100644
index 000000000..96e8e3df5
--- /dev/null
+++ b/algorithms/000386-lexicographical-numbers/README.md
@@ -0,0 +1,109 @@
+386\. Lexicographical Numbers
+
+**Difficulty:** Medium
+
+**Topics:** `Depth-First Search`, `Trie`
+
+Given an integer `n`, return _all the numbers in the range `[1, n]` sorted in lexicographical order_.
+
+You must write an algorithm that runs in `O(n)` time and uses `O(1)` extra space.
+
+**Example 1:**
+
+- **Input:** n = 13
+- **Output:** [1,10,11,12,13,2,3,4,5,6,7,8,9]
+
+**Example 2:**
+
+- **Input:** n = 2
+- **Output:** 4
+- **Explanation:** [1,2]
+
+
+
+**Constraints:**
+
+- 1 <= n <= 5 * 104
+
+
+**Solution:**
+
+We can approach it using a Depth-First Search (DFS)-like strategy.
+
+### Key Insights:
+- Lexicographical order is essentially a pre-order traversal over a virtual n-ary tree, where the root node starts at `1`, and each node has up to 9 children, which are formed by appending digits (0 to 9).
+- We can simulate this pre-order traversal by starting with `1` and repeatedly appending numbers, ensuring we don't exceed the given `n`.
+
+### Approach:
+1. Start with the number `1` and attempt to go deeper by multiplying by `10` (i.e., the next lexicographical number with the next digit).
+2. If going deeper (multiplying by 10) is not possible (i.e., exceeds `n`), increment the number, ensuring that it doesnβt introduce an invalid jump across tens (i.e., going from `19` to `20`).
+3. We backtrack when the current number cannot be extended further and move to the next valid number.
+4. Continue until all numbers up to `n` are processed.
+
+Let's implement this solution in PHP: **[386. Lexicographical Numbers](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/000386-lexicographical-numbers/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+- We maintain a current number and try to go as deep as possible by multiplying it by `10` to get the next lexicographical number.
+- When we can't multiply (because it would exceed `n`), we increment the number. We handle cases where the increment leads to numbers like `20, 30, etc.`, by checking for trailing zeros and adjusting the current number accordingly.
+- The loop continues until we've added all numbers up to `n` in lexicographical order.
+
+### Example Walkthrough:
+#### Input: `n = 13`
+1. Start at `1`.
+2. Multiply `1` by `10` -> `10`.
+3. Add `11`, `12`, `13`.
+4. Backtrack to `2` and continue incrementing up to `9`.
+
+#### Output:
+```php
+[1, 10, 11, 12, 13, 2, 3, 4, 5, 6, 7, 8, 9]
+```
+
+#### Input: `n = 2`
+1. Start at `1`.
+2. Move to `2`.
+
+#### Output:
+```php
+[1, 2]
+```
+
+### Time Complexity:
+- `O(n)` since each number from `1` to `n` is processed exactly once.
+
+### Space Complexity:
+- `O(1)` extra space is used (disregarding the space used for the result array).
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
diff --git a/algorithms/000386-lexicographical-numbers/solution.php b/algorithms/000386-lexicographical-numbers/solution.php
new file mode 100644
index 000000000..38caf207d
--- /dev/null
+++ b/algorithms/000386-lexicographical-numbers/solution.php
@@ -0,0 +1,34 @@
+= $n) {
+ $current /= 10;
+ }
+ $current++;
+ while ($current % 10 == 0) {
+ $current /= 10;
+ }
+ }
+ }
+
+ return $result;
+ }
+}
\ No newline at end of file
diff --git a/algorithms/000402-remove-k-digits/README.md b/algorithms/000402-remove-k-digits/README.md
index 3f2d01524..6ea8eff2f 100644
--- a/algorithms/000402-remove-k-digits/README.md
+++ b/algorithms/000402-remove-k-digits/README.md
@@ -1,6 +1,8 @@
402\. Remove K Digits
-Medium
+**Difficulty:** Medium
+
+**Topics:** `String`, `Stack`, `Greedy`, `Monotonic Stack`
Given string num representing a non-negative integer `num`, and an integer `k`, return the smallest possible integer after removing `k` digits from `num`.
@@ -32,3 +34,62 @@ Given string num representing a non-negative integer `num`, and an integer `k`,
- `num` consists of only digits.
- `num` does not have any leading zeros except for the zero itself.
+
+
+**Solution:**
+
+We can use a greedy approach with a stack. The goal is to build the smallest possible number by iteratively removing digits from the given number while maintaining the smallest possible sequence.
+
+### Approach:
+1. **Use a Stack:** Traverse through each digit of the number. Push digits onto a stack while ensuring that the digits in the stack form the smallest possible number.
+2. **Pop from Stack:** If the current digit is smaller than the top of the stack, and we still have `k` digits to remove, pop the stack to remove the larger digits.
+3. **Handle Remaining Digits:** If there are still digits to remove after processing all the digits in `num`, remove them from the end of the stack.
+4. **Build the Result:** Construct the final number from the digits left in the stack. Ensure to strip any leading zeros.
+5. **Edge Cases:** If the result is an empty string after all removals, return "0".
+
+
+Let's implement this solution in PHP: **[402. Remove K Digits](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/000402-remove-k-digits/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+- **Stack-Based Greedy Approach:** We maintain a stack to store the digits of the smallest possible number. As we iterate through the digits in `num`, we compare the current digit with the top of the stack. If the current digit is smaller and we still have digits to remove (`k > 0`), we pop the stack to remove the larger digit.
+- **Post-Processing:** After processing all digits, if we still need to remove more digits (`k > 0`), we pop from the stack until `k` becomes `0`.
+- **Edge Cases:** We handle leading zeros by using `ltrim` to remove them. If the result is an empty string, we return "0".
+
+### Time Complexity:
+The time complexity of this solution is \(O(n)\), where `n` is the length of the input string `num`. This is because each digit is pushed and popped from the stack at most once.
+
+This solution efficiently handles the constraints and provides the correct output for the given examples.
+
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
\ No newline at end of file
diff --git a/algorithms/000402-remove-k-digits/solution.php b/algorithms/000402-remove-k-digits/solution.php
index 8794fc88e..1408bd37b 100644
--- a/algorithms/000402-remove-k-digits/solution.php
+++ b/algorithms/000402-remove-k-digits/solution.php
@@ -1,42 +1,40 @@
0 && !empty($stack) && $stack[count($stack) - 1] > $digit) {
+
+ // Remove digits from the stack if the current digit is smaller and we still have digits to remove
+ while ($k > 0 && !empty($stack) && end($stack) > $digit) {
array_pop($stack);
- $k -= 1;
+ $k--;
}
- array_push($stack, $digit);
+
+ // Push the current digit onto the stack
+ $stack[] = $digit;
}
- for ($j = 0; $j < $k; $j++) {
+ // If we still have digits to remove, remove them from the end
+ while ($k > 0) {
array_pop($stack);
+ $k--;
}
- foreach ($stack as $c) {
- if ($c == '0' && empty($ans)) {
- continue;
- }
- array_push($ans, $c);
- }
+ // Build the final result, removing leading zeros
+ $result = ltrim(implode('', $stack), '0');
+
+ // Return "0" if the result is empty
+ return $result === '' ? '0' : $result;
- return implode($ans) ? implode($ans) : '0';
}
}
\ No newline at end of file
diff --git a/algorithms/000404-sum-of-left-leaves/README.md b/algorithms/000404-sum-of-left-leaves/README.md
index 7b9946bb0..a03b57925 100644
--- a/algorithms/000404-sum-of-left-leaves/README.md
+++ b/algorithms/000404-sum-of-left-leaves/README.md
@@ -1,6 +1,8 @@
404\. Sum of Left Leaves
-Easy
+**Difficulty:** Easy
+
+**Topics:** `Tree`, `Depth-First Search`, `Breadth-First Search`, `Binary Tree`
Given the `root` of a binary tree, return _the sum of all left leaves_.
@@ -8,7 +10,7 @@ A **leaf** is a node with no children. A **left leaf** is a leaf that is the lef
**Example 1:**
-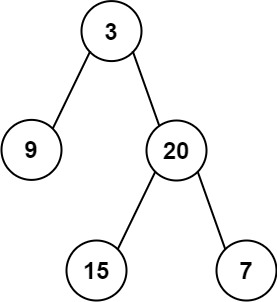
+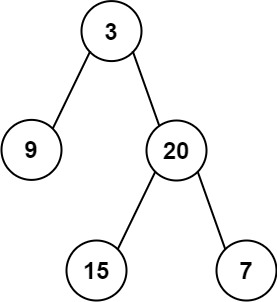
- **Input:** **root = [3,9,20,null,null,15,7]**
- **Output:** **24**
@@ -22,4 +24,86 @@ A **leaf** is a node with no children. A **left leaf** is a leaf that is the lef
**Constraints:**
- The number of nodes in the tree is in the range `[1, 1000]`.
-- `-1000 <= Node.val <= 1000`
\ No newline at end of file
+- `-1000 <= Node.val <= 1000`
+
+
+**Solution:**
+
+We can implement a solution using recursion. Given the constraints and requirements, we will define a function to sum the values of all left leaves in a binary tree.
+
+1. **Define the Binary Tree Node Structure**: Since PHP 5.6 doesnβt support classes with properties as easily, we use arrays to represent the tree nodes.
+
+2. **Recursive Function**: Implement a recursive function to traverse the tree and sum the values of left leaves.
+
+Let's implement this solution in PHP: **[404. Sum of Left Leaves](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/000404-sum-of-left-leaves/solution.php)**
+
+```php
+val = $val;
+ $this->left = $left;
+ $this->right = $right;
+ }
+}
+
+// Define a tree node as an associative array for simplicity
+// ['val' => value, 'left' => left_child, 'right' => right_child]
+
+// Function to compute the sum of left leaves
+function sumOfLeftLeaves($root) {
+ ...
+ ...
+ ...
+ /**
+ * go to ./solution.php
+ */
+}
+
+// Example usage:
+
+// Create the binary tree [3,9,20,null,null,15,7]
+$root = new TreeNode(3);
+$root->left = new TreeNode(9);
+$root->right = new TreeNode(20);
+$root->right->left = new TreeNode(15);
+$root->right->right = new TreeNode(7);
+
+echo sumOfLeftLeaves($root); // Output: 24
+
+// For a single node tree [1]
+$root2 = new TreeNode(1);
+echo sumOfLeftLeaves($root2); // Output: 0
+?>
+```
+
+### Explanation:
+
+1. **Tree Node Definition**:
+ - The `createNode` function creates a node with a value and optional left and right children.
+
+2. **Sum of Left Leaves Function**:
+ - The `sumOfLeftLeaves` function recursively traverses the tree.
+ - It checks if the left child exists and if it's a leaf (i.e., it has no children). If so, it adds the value of this leaf to the sum.
+ - It then recursively processes the right child to account for any left leaves that might be in the right subtree.
+
+3. **Example Usage**:
+ - For the given tree examples, the function calculates the sum of all left leaves correctly.
+
+### Complexity:
+- **Time Complexity**: O(n), where n is the number of nodes in the tree, as each node is visited exactly once.
+- **Space Complexity**: O(h), where h is the height of the tree, due to recursion stack space.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
+
diff --git a/algorithms/000409-longest-palindrome/README.md b/algorithms/000409-longest-palindrome/README.md
index 3f08e4be5..df0fb17cd 100644
--- a/algorithms/000409-longest-palindrome/README.md
+++ b/algorithms/000409-longest-palindrome/README.md
@@ -1,6 +1,8 @@
409\. Longest Palindrome
-Easy
+**Difficulty:** Easy
+
+**Topics:** `Hash Table`, `String`, `Greedy`
Given a string `s` which consists of lowercase or uppercase letters, return the length of the **longest** palindrome[^1]
that can be built with those letters.
@@ -25,3 +27,64 @@ Letters are **case sensitive**, for example, `"Aa"` is not considered a palindro
- `s` consists of lowercase **and/or** uppercase English letters only.
[^1]: **Palindrome** `A palindrome is a string that reads the same forward and backward.`
+
+
+
+**Solution:**
+
+We can use a hash table to count the occurrences of each character. The key insight here is:
+
+1. Characters that appear an even number of times can fully contribute to the palindrome.
+2. Characters that appear an odd number of times can contribute up to the largest even number that is less than or equal to their count. However, you can use one odd character as the center of the palindrome, so we can add 1 to the final length if there's at least one character with an odd count.
+
+
+Let's implement this solution in PHP: **[409. Longest Palindrome](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/000409-longest-palindrome/solution.php)**
+
+```php
+longestPalindrome("abccccdd"); // Output: 7
+echo $solution->longestPalindrome("a"); // Output: 1
+?>
+```
+
+### Explanation:
+
+1. **Character Counting:** We use an associative array `$charCount` to keep track of the number of occurrences of each character in the string.
+
+2. **Calculate the Palindrome Length:**
+ - We iterate through the count of each character:
+ - If the count is even, we add the full count to the palindrome length.
+ - If the count is odd, we add the largest even portion (`$count - 1`) to the palindrome length and mark that we have found an odd count.
+ - If there was at least one character with an odd count, we add `1` to the length to account for the middle character of the palindrome.
+
+3. **Edge Cases:** The solution handles cases with strings of length 1 and mixed case sensitivity.
+
+This approach ensures an optimal solution with a time complexity of O(n), where n is the length of the string `s`.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
+
+
+
+
diff --git a/algorithms/000409-longest-palindrome/solution.php b/algorithms/000409-longest-palindrome/solution.php
index 9c355a67a..c8ca32fdf 100644
--- a/algorithms/000409-longest-palindrome/solution.php
+++ b/algorithms/000409-longest-palindrome/solution.php
@@ -7,25 +7,36 @@ class Solution {
* @return Integer
*/
function longestPalindrome($s) {
- $ans = 0;
- $count = array_fill(0, 128, 0);
+ $charCount = [];
+ $length = 0;
+ $oddCountFound = false;
- foreach(str_split($s) as $c) {
- $count[ord($c)]++;
+ // Count the occurrences of each character
+ for ($i = 0; $i < strlen($s); $i++) {
+ $char = $s[$i];
+ if (!isset($charCount[$char])) {
+ $charCount[$char] = 0;
+ }
+ $charCount[$char]++;
}
- foreach($count as $freq) {
- $ans += $freq % 2 == 0 ? $freq : $freq - 1;
+ // Calculate the length of the longest palindrome
+ foreach ($charCount as $count) {
+ if ($count % 2 == 0) {
+ // Add the entire count if it's even
+ $length += $count;
+ } else {
+ // Add the largest even number less than count
+ $length += $count - 1;
+ $oddCountFound = true;
+ }
}
- $hasOddCount = false;
- foreach($count as $c) {
- if($c % 2 != 0) {
- $hasOddCount = true;
- break;
- }
+ // If there was at least one character with an odd count, we can place one in the middle
+ if ($oddCountFound) {
+ $length += 1;
}
- return $ans + ($hasOddCount ? 1 : 0);
+ return $length;
}
}
\ No newline at end of file
diff --git a/algorithms/000432-all-oone-data-structure/README.md b/algorithms/000432-all-oone-data-structure/README.md
new file mode 100644
index 000000000..d32ee5755
--- /dev/null
+++ b/algorithms/000432-all-oone-data-structure/README.md
@@ -0,0 +1,272 @@
+432\. All O`one Data Structure
+
+**Difficulty:** Hard
+
+**Topics:** `Hash Table`, `Linked List`, `Design`, `Doubly-Linked List`
+
+Design a data structure to store the strings' count with the ability to return the strings with minimum and maximum counts.
+
+Implement the `AllOne` class:
+
+- `AllOne()` Initializes the object of the data structure.
+- `inc(String key)` Increments the count of the string `key` by `1`. If `key` does not exist in the data structure, insert it with count `1`.
+- `dec(String key)` Decrements the count of the string `key` by `1`. If the count of `key` is `0` after the decrement, remove it from the data structure. It is guaranteed that `key` exists in the data structure before the decrement.
+- `getMaxKey()` Returns one of the keys with the maximal count. If no element exists, return an empty string `""`.
+- `getMinKey()` Returns one of the keys with the minimum count. If no element exists, return an empty string `""`.
+
+**Note** that each function must run in `O(1)` average time complexity.
+
+**Example 1:**
+
+- **Input:**\
+ ["AllOne", "inc", "inc", "getMaxKey", "getMinKey", "inc", "getMaxKey", "getMinKey"]\
+ [[], ["hello"], ["hello"], [], [], ["leet"], [], []]
+- **Output:** [null, null, null, "hello", "hello", null, "hello", "leet"]
+- **Explanation:**\
+ AllOne allOne = new AllOne();\
+ allOne.inc("hello");\
+ allOne.inc("hello");\
+ allOne.getMaxKey(); // return "hello"\
+ allOne.getMinKey(); // return "hello"\
+ allOne.inc("leet");\
+ allOne.getMaxKey(); // return "hello"\
+ allOne.getMinKey(); // return "leet"
+
+
+**Constraints:**
+
+- `1 <= key.length <= 10`
+- `key` consists of lowercase English letters.
+- It is guaranteed that for each call to `dec`, `key` is existing in the data structure.
+- At most 5 * 104
calls will be made to `inc`, `dec`, `getMaxKey`, and `getMinKey`.
+
+
+
+**Solution:**
+
+We need to implement a data structure that allows incrementing, decrementing, and retrieving keys with the minimum and maximum counts in constant time (`O(1)`).
+
+### Key Insights:
+1. **Hash Table (for String Count)**:
+ We need a hash table (`counts`) that maps each string (`key`) to its count. This allows for `O(1)` access when incrementing or decrementing the count.
+
+2. **Doubly Linked List (for Counts)**:
+ To keep track of the minimum and maximum counts, we can use a doubly linked list where each node represents a unique count. The node will store all strings with that count in a set. The linked list will help in retrieving the min and max counts in constant time by keeping track of the head (min) and tail (max) nodes.
+
+3. **Two Hash Maps**:
+ - A hash map (`key_to_node`) will map each string (`key`) to the node in the doubly linked list that stores its count. This allows us to move the key from one count node to another in `O(1)` time when we increment or decrement the count.
+ - Another hash map (`counts`) will map each count to its corresponding node in the doubly linked list, ensuring we can locate the node for any count in `O(1)` time.
+
+### Plan:
+- **inc(key)**:
+ - If the key exists, increase its count by 1 and move it to the next node (create a new node if necessary).
+ - If the key does not exist, create a new node with count 1 and insert it.
+
+- **dec(key)**:
+ - Decrease the count of the key by 1.
+ - If the count becomes zero, remove the key from the data structure.
+
+- **getMaxKey()** and **getMinKey()**:
+ - Return the first key from the tail node (max count) or head node (min count) in constant time.
+
+Let's implement this solution in PHP: **[432. All O`one Data Structure](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/000432-all-oone-data-structure/solution.php)**
+
+```php
+inc($key);
+ * $obj->dec($key);
+ * $ret_3 = $obj->getMaxKey();
+ * $ret_4 = $obj->getMinKey();
+ */
+
+// Example usage
+$allOne = new AllOne();
+$allOne->inc("hello");
+$allOne->inc("hello");
+echo $allOne->getMaxKey(); // returns "hello"
+echo $allOne->getMinKey(); // returns "hello"
+$allOne->inc("leet");
+echo $allOne->getMaxKey(); // returns "hello"
+echo $allOne->getMinKey(); // returns "leet"
+?>
+```
+
+### Explanation:
+
+1. **Data Structure**:
+ - `key_to_node`: Maps each key to the corresponding node in the doubly linked list.
+ - `counts`: Maps each count to its corresponding node in the doubly linked list.
+ - `head` and `tail`: Dummy head and tail nodes for easier manipulation of the doubly linked list.
+
+2. **Methods**:
+ - `inc($key)`: If the key exists, it increments its count and moves it to the appropriate node in the list. If not, it inserts it with count 1.
+ - `dec($key)`: Decreases the keyβs count and either removes it or moves it to the appropriate node.
+ - `getMaxKey()`: Returns the key from the node at the tail of the doubly linked list (max count).
+ - `getMinKey()`: Returns the key from the node at the head of the doubly linked list (min count).
+
+### Complexity:
+- All operations are designed to run in `O(1)` average time complexity.
+
+Let me know if you need further clarifications!
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
\ No newline at end of file
diff --git a/algorithms/000432-all-oone-data-structure/solution.php b/algorithms/000432-all-oone-data-structure/solution.php
new file mode 100644
index 000000000..a1f276d40
--- /dev/null
+++ b/algorithms/000432-all-oone-data-structure/solution.php
@@ -0,0 +1,190 @@
+count = $count;
+ $this->keys = [];
+ $this->prev = null;
+ $this->next = null;
+ }
+}
+
+class AllOne {
+ /**
+ * @var array
+ */
+ private $key_to_node;
+ /**
+ * @var array
+ */
+ private $counts;
+ /**
+ * @var Node
+ */
+ private $head;
+ /**
+ * @var Node
+ */
+ private $tail;
+
+ /**
+ */
+ function __construct() {
+ $this->key_to_node = [];
+ $this->counts = [];
+ $this->head = new Node(null); // Dummy head
+ $this->tail = new Node(null); // Dummy tail
+ $this->head->next = $this->tail;
+ $this->tail->prev = $this->head;
+ }
+
+ /**
+ * Insert a new node after a given node
+ *
+ * @param $newNode
+ * @param $prevNode
+ * @return void
+ */
+ private function insertAfter($newNode, $prevNode) {
+ $newNode->next = $prevNode->next;
+ $newNode->prev = $prevNode;
+ $prevNode->next->prev = $newNode;
+ $prevNode->next = $newNode;
+ }
+
+ /**
+ * Remove a node from the linked list
+ *
+ * @param $node
+ * @return void
+ */
+ private function removeNode($node) {
+ $node->prev->next = $node->next;
+ $node->next->prev = $node->prev;
+ unset($this->counts[$node->count]);
+ }
+
+ /**
+ * Increments the count of a key
+ *
+ * @param String $key
+ * @return NULL
+ */
+ function inc($key) {
+ if (!isset($this->key_to_node[$key])) {
+ // Key does not exist, insert with count 1
+ if (!isset($this->counts[1])) {
+ $newNode = new Node(1);
+ $this->insertAfter($newNode, $this->head);
+ $this->counts[1] = $newNode;
+ }
+ $this->counts[1]->keys[$key] = true;
+ $this->key_to_node[$key] = $this->counts[1];
+ } else {
+ // Key exists, increment its count
+ $curNode = $this->key_to_node[$key];
+ $curCount = $curNode->count;
+ $newCount = $curCount + 1;
+
+ // Move the key to the next count node
+ unset($curNode->keys[$key]);
+ if (!isset($this->counts[$newCount])) {
+ $newNode = new Node($newCount);
+ $this->insertAfter($newNode, $curNode);
+ $this->counts[$newCount] = $newNode;
+ }
+ $this->counts[$newCount]->keys[$key] = true;
+ $this->key_to_node[$key] = $this->counts[$newCount];
+
+ // Remove the old node if empty
+ if (empty($curNode->keys)) {
+ $this->removeNode($curNode);
+ }
+ }
+ }
+
+ /**
+ * Decrements the count of a key
+ *
+ * @param String $key
+ * @return NULL
+ */
+ function dec($key) {
+ if (!isset($this->key_to_node[$key])) return;
+
+ $curNode = $this->key_to_node[$key];
+ $curCount = $curNode->count;
+ $newCount = $curCount - 1;
+
+ // Remove the key from the current node
+ unset($curNode->keys[$key]);
+
+ // If the new count is 0, completely remove the key
+ if ($newCount == 0) {
+ unset($this->key_to_node[$key]);
+ } else {
+ // Move the key to the previous count node
+ if (!isset($this->counts[$newCount])) {
+ $newNode = new Node($newCount);
+ $this->insertAfter($newNode, $curNode->prev);
+ $this->counts[$newCount] = $newNode;
+ }
+ $this->counts[$newCount]->keys[$key] = true;
+ $this->key_to_node[$key] = $this->counts[$newCount];
+ }
+
+ // Remove the old node if empty
+ if (empty($curNode->keys)) {
+ $this->removeNode($curNode);
+ }
+ }
+
+ /**
+ * Returns one of the keys with the maximum count
+ *
+ * @return String
+ */
+ function getMaxKey() {
+ if ($this->tail->prev === $this->head) return "";
+ return key($this->tail->prev->keys);
+ }
+
+ /**
+ * Returns one of the keys with the minimum count
+ *
+ * @return String
+ */
+ function getMinKey() {
+ if ($this->head->next === $this->tail) return "";
+ return key($this->head->next->keys);
+ }
+}
+
+/**
+ * Your AllOne object will be instantiated and called as such:
+ * $obj = AllOne();
+ * $obj->inc($key);
+ * $obj->dec($key);
+ * $ret_3 = $obj->getMaxKey();
+ * $ret_4 = $obj->getMinKey();
+ */
diff --git a/algorithms/000440-k-th-smallest-in-lexicographical-order/README.md b/algorithms/000440-k-th-smallest-in-lexicographical-order/README.md
new file mode 100644
index 000000000..7b578f417
--- /dev/null
+++ b/algorithms/000440-k-th-smallest-in-lexicographical-order/README.md
@@ -0,0 +1,109 @@
+440\. K-th Smallest in Lexicographical Order
+
+**Difficulty:** Hard
+
+**Topics:** `Trie`
+
+Given two integers `n` and `k`, return _the kth
lexicographically smallest integer in the range `[1, n]`_.
+
+**Example 1:**
+
+- **Input:** n = 13, k = 2
+- **Output:** 10
+- **Explanation:** The lexicographical order is [1, 10, 11, 12, 13, 2, 3, 4, 5, 6, 7, 8, 9], so the second smallest number is 10.
+
+**Example 2:**
+
+- **Input:** n = 1, k = 1
+- **Output:** 1
+
+**Constraints:**
+
+- 1 <= k <= n <= 109
+
+
+**Solution:**
+
+The idea is to traverse through numbers as they would appear in a lexicographical order, similar to performing a depth-first search (DFS) on a Trie.
+
+### Approach:
+
+1. We can visualize this as a Trie where each node represents a number, and the children of a node `x` are `10 * x + 0`, `10 * x + 1`, ..., `10 * x + 9`.
+2. Starting from `1`, we explore numbers in lexicographical order, counting how many numbers can be placed before we reach the `k`th one.
+3. The key part of the solution is to efficiently compute the number of integers between `start` and `end` in a lexicographical order. This will help us skip some branches when needed.
+
+### Steps:
+
+1. Start from the root number `1`.
+2. Use a helper function to calculate how many numbers exist between `prefix` and the next prefix (`prefix+1`).
+3. Based on this count, decide whether to move deeper in the current prefix (by multiplying it by 10) or move to the next sibling prefix (by incrementing the current prefix).
+
+Let's implement this solution in PHP: **[440. K-th Smallest in Lexicographical Order](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/000440-k-th-smallest-in-lexicographical-order/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+1. **findKthNumber function**:
+ - We start at the root (`current = 1`).
+ - We decrement `k` by 1 because the first number is already considered (i.e., we start with 1).
+ - We then use the `calculateSteps` function to determine how many numbers lie between `current` and `current + 1` in lexicographical order.
+ - If the number of steps is less than or equal to `k`, it means the k-th number is not within the current prefix, so we move to the next prefix (`current++`).
+ - If the k-th number is within the current prefix, we go deeper into the tree (`current *= 10`).
+ - We continue this process until `k` becomes `0`.
+
+2. **calculateSteps function**:
+ - This function calculates how many numbers there are in lexicographical order between `prefix1` and `prefix2` within the range of `n`.
+ - It uses a while loop to compute the steps at each level of the "tree" (i.e., between numbers with the same prefix but different lengths).
+
+### Time Complexity:
+The time complexity is approximately `O(log n)`, where `n` is the input range. This is because in each step, we either go deeper into the current prefix or move to the next prefix, and both operations are logarithmic relative to `n`.
+
+This solution is efficient even for large inputs where `n` can be as large as `10^9`.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
diff --git a/algorithms/000440-k-th-smallest-in-lexicographical-order/solution.php b/algorithms/000440-k-th-smallest-in-lexicographical-order/solution.php
new file mode 100644
index 000000000..fc039fe08
--- /dev/null
+++ b/algorithms/000440-k-th-smallest-in-lexicographical-order/solution.php
@@ -0,0 +1,51 @@
+ 0) {
+ $steps = $this->calculateSteps($n, $current, $current + 1);
+
+ if ($steps <= $k) {
+ // If the k-th number is not in this prefix, move to the next prefix
+ $current++;
+ $k -= $steps; // Decrease k by the number of steps skipped
+ } else {
+ // If the k-th number is in this prefix, move deeper in this branch
+ $current *= 10;
+ $k--; // We are considering the current number itself
+ }
+ }
+
+ return $current;
+ }
+
+ /**
+ * Helper function to calculate the number of steps between two prefixes in the range [1, n]
+ *
+ * @param $n
+ * @param $prefix1
+ * @param $prefix2
+ * @return int|mixed
+ */
+ function calculateSteps($n, $prefix1, $prefix2) {
+ $steps = 0;
+
+ while ($prefix1 <= $n) {
+ $steps += min($n + 1, $prefix2) - $prefix1;
+ $prefix1 *= 10;
+ $prefix2 *= 10;
+ }
+
+ return $steps;
+ }
+
+}
\ No newline at end of file
diff --git a/algorithms/000445-add-two-numbers-ii/README.md b/algorithms/000445-add-two-numbers-ii/README.md
index cf585db29..b60332091 100644
--- a/algorithms/000445-add-two-numbers-ii/README.md
+++ b/algorithms/000445-add-two-numbers-ii/README.md
@@ -1,6 +1,8 @@
445\. Add Two Numbers II
-Medium
+**Difficulty:** Medium
+
+**Topics:** `Linked List`, `Math`, `Stack`
You are given two **non-empty** linked lists representing two non-negative integers. The most significant digit comes first and each of their nodes contains a single digit. Add the two numbers and return the sum as a linked list.
@@ -8,7 +10,7 @@ You may assume the two numbers do not contain any leading zero, except the numbe
**Example 1:**
-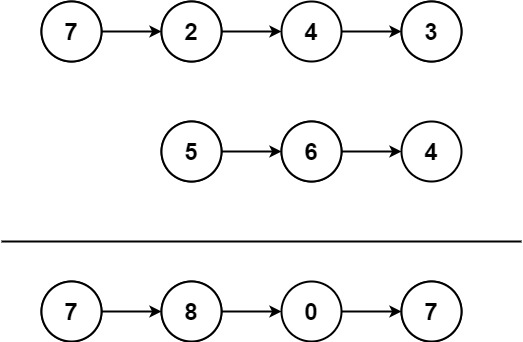
+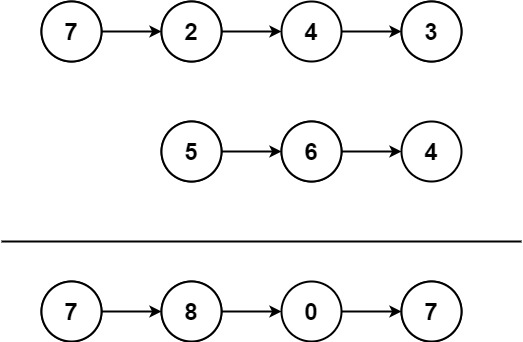
- **Input:** l1 = [7,2,4,3], l2 = [5,6,4]
- **Output:** [7,8,0,7]
@@ -29,4 +31,115 @@ You may assume the two numbers do not contain any leading zero, except the numbe
- 0 <= Node.val <= 9
- It is guaranteed that the list represents a number that does not have leading zeros.
-**Follow-up:** Could you solve it without reversing the input lists?
\ No newline at end of file
+**Follow-up:** Could you solve it without reversing the input lists?
+
+
+
+**Solution:**
+
+We need to add two numbers represented as linked lists. Since the most significant digit comes first, we need to handle the addition from left to right.
+
+### Approach:
+1. **Use Stacks**:
+ - Since we can't traverse the list from the end directly, we can use stacks to store the digits from the linked lists. This allows us to add the numbers starting from the least significant digit (as if we were traversing the list in reverse).
+
+2. **Addition**:
+ - Pop elements from both stacks and add them along with any carry from the previous operation. Create new nodes for the result linked list as we go.
+
+3. **Handle Carry**:
+ - If there's any carry left after all digits have been added, it should be added as a new node at the front of the list.
+
+Let's implement this solution in PHP: **[445. Add Two Numbers II](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/000445-add-two-numbers-ii/solution.php)**
+
+```php
+val = $val;
+ $this->next = $next;
+ }
+}
+
+/**
+ * @param ListNode $l1
+ * @param ListNode $l2
+ * @return ListNode
+ */
+function addTwoNumbers($l1, $l2) {
+ ...
+ ...
+ ...
+ /**
+ * go to ./solution.php
+ */
+}
+
+// Helper function to create a linked list from an array
+/**
+ * @param $arr
+ * @return mixed|null
+ */
+function createLinkedList($arr) {
+ $dummy = new ListNode();
+ $current = $dummy;
+ foreach ($arr as $value) {
+ $current->next = new ListNode($value);
+ $current = $current->next;
+ }
+ return $dummy->next;
+}
+
+// Helper function to print a linked list
+/**
+ * @param $node
+ * @return void
+ */
+function printLinkedList($node) {
+ $output = [];
+ while ($node !== null) {
+ $output[] = $node->val;
+ $node = $node->next;
+ }
+ echo "[" . implode(",", $output) . "]\n";
+}
+
+// Example usage:
+$l1 = createLinkedList([7, 2, 4, 3]);
+$l2 = createLinkedList([5, 6, 4]);
+$result = addTwoNumbers($l1, $l2);
+printLinkedList($result); // Output: [7,8,0,7]
+
+$l1 = createLinkedList([2, 4, 3]);
+$l2 = createLinkedList([5, 6, 4]);
+$result = addTwoNumbers($l1, $l2);
+printLinkedList($result); // Output: [8,0,7]
+
+$l1 = createLinkedList([0]);
+$l2 = createLinkedList([0]);
+$result = addTwoNumbers($l1, $l2);
+printLinkedList($result); // Output: [0]
+?>
+```
+
+### Explanation:
+
+- **Stacks**: The digits are pushed into stacks `stack1` and `stack2` so that the most significant digits are on top.
+- **Addition and Carry**: We pop digits from both stacks, add them with any carry, and create new nodes for the resulting linked list.
+- **Result List**: The result is formed by continuously linking new nodes to the front of the list, ensuring the most significant digits come first.
+- **Edge Cases**: Handles scenarios like different lengths of input lists and carries that need to be added as new nodes.
+
+This solution efficiently handles the addition of two numbers represented by linked lists without reversing the lists, satisfying the problem's constraints.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
diff --git a/algorithms/000445-add-two-numbers-ii/solution.php b/algorithms/000445-add-two-numbers-ii/solution.php
index 427105624..15f144875 100644
--- a/algorithms/000445-add-two-numbers-ii/solution.php
+++ b/algorithms/000445-add-two-numbers-ii/solution.php
@@ -18,38 +18,44 @@ class Solution {
* @param ListNode $l2
* @return ListNode
*/
- function addTwoNumbers(ListNode $l1, ListNode $l2): ListNode
- {
- $s1 = new SplStack();
- $s2 = new SplStack();
- while($l1 != null){
- $s1->push($l1->val);
+ function addTwoNumbers($l1, $l2) {
+ $stack1 = [];
+ $stack2 = [];
+
+ // Push all elements of l1 to stack1
+ while ($l1 !== null) {
+ array_push($stack1, $l1->val);
$l1 = $l1->next;
}
- while($l2 != null){
- $s2->push($l2->val);
+ // Push all elements of l2 to stack2
+ while ($l2 !== null) {
+ array_push($stack2, $l2->val);
$l2 = $l2->next;
}
- $sum = 0;
- $cur = new ListNode();
- while(!$s1->isEmpty() || !$s2->isEmpty()){
- if(!$s1->isEmpty()) $sum += $s1->pop();
- if(!$s2->isEmpty()) $sum += $s2->pop();
-
- $cur->val = $sum%10;
- $head = new ListNode(floor($sum/10));
- $head->next = $cur; // reconstruct
- $cur = $head;// moving on
- $sum /= 10;
- }
- if($cur->val >= 1 ){
- $return_value = 0? $cur->next: $cur;
- }else{
- $return_value = $cur->next;
+ $carry = 0;
+ $head = null;
+
+ // While there are elements in stack1 or stack2 or there's a carry
+ while (!empty($stack1) || !empty($stack2) || $carry > 0) {
+ $sum = $carry;
+
+ if (!empty($stack1)) {
+ $sum += array_pop($stack1);
+ }
+
+ if (!empty($stack2)) {
+ $sum += array_pop($stack2);
+ }
+
+ $carry = intdiv($sum, 10);
+ $newNode = new ListNode($sum % 10);
+ $newNode->next = $head;
+ $head = $newNode;
}
- return ($return_value);
- //return $cur->val == 0? $cur->next: $cur;
+
+ return $head;
+
}
}
\ No newline at end of file
diff --git a/algorithms/000463-island-perimeter/README.md b/algorithms/000463-island-perimeter/README.md
index c990506c2..8887d6ac1 100644
--- a/algorithms/000463-island-perimeter/README.md
+++ b/algorithms/000463-island-perimeter/README.md
@@ -1,6 +1,8 @@
463\. Island Perimeter
-Easy
+**Difficulty:** Easy
+
+**Topics:** `Array`, `Depth-First Search`, `Breadth-First Search`, `Matrix`
You are given `row x col` `grid` representing a map where `grid[i][j] = 1` represents land and `grid[i][j] = 0` represents water.
@@ -10,7 +12,7 @@ The island doesn't have "lakes", meaning the water inside isn't connected to the
**Example 1:**
-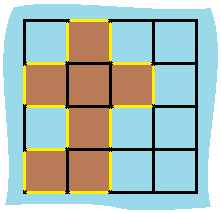
+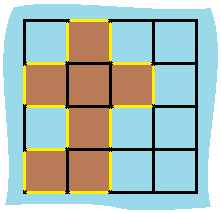
- **Input:** grid = [[0,1,0,0],[1,1,1,0],[0,1,0,0],[1,1,0,0]]
- **Output:** 16
@@ -33,3 +35,78 @@ The island doesn't have "lakes", meaning the water inside isn't connected to the
- `1 <= row, col <= 100`
- `grid[i][j]` is `0` or `1`.
- There is exactly one island in `grid`.
+
+
+**Solution:**
+
+We can iterate through each cell in the grid and apply the following logic:
+
+1. **Count Land Cells**: For each land cell (`grid[i][j] = 1`), assume it contributes `4` to the perimeter (each side of the cell).
+2. **Subtract Shared Edges**: If a land cell has a neighboring land cell either to its right (`grid[i][j + 1] = 1`) or below it (`grid[i + 1][j] = 1`), the perimeter is reduced by `2` for each shared edge, since two adjacent cells share a side.
+
+The overall perimeter can be computed by summing up the individual contributions from each land cell, adjusted for shared edges.
+
+Let's implement this solution in PHP: **[463. Island Perimeter](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/000463-island-perimeter/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+- **Initialization**:
+ - `$rows` and `$cols` store the dimensions of the grid.
+ - `$perimeter` is initialized to `0` and will store the total perimeter.
+
+- **Nested Loops**:
+ - We iterate through each cell in the grid using two nested loops.
+ - If the cell is land (`grid[i][$j] == 1`), we initially add `4` to the perimeter.
+ - We then check for shared edges:
+ - **Right Neighbor**: If there's a land cell to the right, reduce the perimeter by `2` (one edge is shared).
+ - **Bottom Neighbor**: If there's a land cell below, reduce the perimeter by `2`.
+
+- **Result**:
+ - The function returns the calculated perimeter after considering all the land cells and their shared edges.
+
+### Example Output:
+
+For the provided grid:
+
+```php
+$grid = [
+ [0,1,0,0],
+ [1,1,1,0],
+ [0,1,0,0],
+ [1,1,0,0]
+];
+echo islandPerimeter($grid); // Output: 16
+```
+
+The output will be `16`, which matches the expected perimeter for the island in the grid. This approach efficiently computes the perimeter by iterating through the grid once and adjusting the perimeter for shared edges.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
diff --git a/algorithms/000463-island-perimeter/solution.php b/algorithms/000463-island-perimeter/solution.php
index 70d110404..931264330 100644
--- a/algorithms/000463-island-perimeter/solution.php
+++ b/algorithms/000463-island-perimeter/solution.php
@@ -6,28 +6,31 @@ class Solution {
* @param Integer[][] $grid
* @return Integer
*/
- function islandPerimeter(array $grid): float|int
- {
- $m = count($grid);
- $n = count($grid[0]);
+ function islandPerimeter($grid) {
+ $rows = count($grid);
+ $cols = count($grid[0]);
+ $perimeter = 0;
- $islands = 0;
- $neighbors = 0;
-
- for ($i = 0; $i < $m; $i++) {
- for ($j = 0; $j < $n; $j++) {
+ for ($i = 0; $i < $rows; $i++) {
+ for ($j = 0; $j < $cols; $j++) {
if ($grid[$i][$j] == 1) {
- $islands += 1;
- if ($i + 1 < $m && $grid[$i + 1][$j] == 1) {
- $neighbors += 1;
+ // Add 4 for the current land cell
+ $perimeter += 4;
+
+ // Check if the cell has a right neighbor that's also land
+ if ($j < $cols - 1 && $grid[$i][$j + 1] == 1) {
+ $perimeter -= 2; // Subtract 2 for the shared edge with the right neighbor
}
- if ($j + 1 < $n && $grid[$i][$j + 1] == 1) {
- $neighbors += 1;
+
+ // Check if the cell has a bottom neighbor that's also land
+ if ($i < $rows - 1 && $grid[$i + 1][$j] == 1) {
+ $perimeter -= 2; // Subtract 2 for the shared edge with the bottom neighbor
}
}
}
}
- return $islands * 4 - $neighbors * 2;
+ return $perimeter;
+
}
}
\ No newline at end of file
diff --git a/algorithms/000476-number-complement/README.md b/algorithms/000476-number-complement/README.md
new file mode 100644
index 000000000..657bdb19b
--- /dev/null
+++ b/algorithms/000476-number-complement/README.md
@@ -0,0 +1,83 @@
+476\. Number Complement
+
+**Difficulty:** Easy
+
+**Topics:** `Bit Manipulation`
+
+The complement of an integer is the integer you get when you flip all the `0`'s to `1`'s and all the `1`'s to `0`'s in its binary representation.
+
+- For example, The integer `5` is `"101"` in binary and its complement is `"010"` which is the integer `2`.
+
+Given an integer `num`, return _its complement_.
+
+**Example 1:**
+
+- **Input:** num = 5
+- **Output:** 2
+- **Explanation:** The binary representation of 5 is 101 (no leading zero bits), and its complement is 010. So you need to output 2.
+
+**Example 2:**
+
+- **Input:** num = 1
+- **Output:** 0
+- **Explanation:** The binary representation of 1 is 1 (no leading zero bits), and its complement is 0. So you need to output 0.
+
+**Constraints:**
+
+- 1 <= num < 231
+
+**Note:** This question is the same as [1009. Complement of Base 10 Integer](https://leetcode.com/problems/complement-of-base-10-integer/)
+
+
+**Solution:**
+
+We need to flip the bits of the binary representation of a given integer and return the resulting integer.
+
+### Steps to solve the problem:
+
+1. **Convert the number to its binary representation.**
+2. **Flip the bits** (i.e., change `0` to `1` and `1` to `0`).
+3. **Convert the flipped binary string back to an integer**.
+
+Let's implement this solution in PHP: **[476. Number Complement](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/000476-number-complement/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+- **`decbin($num)`**: Converts the given integer to its binary string representation.
+- **Flipping the bits**: We iterate through the binary string and flip each bit by checking if it's a `1` or a `0`.
+- **`bindec($flipped)`**: Converts the flipped binary string back to an integer.
+
+### Example Runs:
+
+1. **Input:** `5`
+ - Binary representation: `"101"`
+ - Flipped binary: `"010"`
+ - Output: `2`
+
+2. **Input:** `1`
+ - Binary representation: `"1"`
+ - Flipped binary: `"0"`
+ - Output: `0`
+
+This solution efficiently calculates the complement by flipping the bits of the binary representation of the given number.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
+
diff --git a/algorithms/000476-number-complement/solution.php b/algorithms/000476-number-complement/solution.php
new file mode 100644
index 000000000..a6d238958
--- /dev/null
+++ b/algorithms/000476-number-complement/solution.php
@@ -0,0 +1,23 @@
+220 combinations when using backtracking.
+
+
+### **Approach**
+We can solve this problem using **Dynamic Programming (Subset Sum Transformation)** or **Backtracking**. Below, we use **Dynamic Programming (DP)** for efficiency.
+
+**Key Observations**:
+1. If we split `nums` into two groups: `P` (positive subset) and `N` (negative subset), then: `target = sum(P) - sum(N)`
+
+ Rearrange terms: `sum(P) + sum(N) = sum(nums)`
+
+ Let S+ be the sum of the positive subset. Then: `S+ = (sum(nums) + target) / 2`
+
+2. If `(sum(nums) + target) % 2 β 0`, return `0` because it's impossible to partition the sums.
+
+
+### **Plan**
+1. Compute the total sum of `nums`.
+2. Check if the target is achievable using the formula for S+
. If invalid, return `0`.
+3. Use a DP approach to find the count of subsets in `nums` whose sum equals S+
.
+
+Let's implement this solution in PHP: **[494. Target Sum](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/000494-target-sum/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+1. **Input Validation**:
+ - We calculate S+ = (sum(nums) + target) / 2
.
+ - If S+
is not an integer, it's impossible to split `nums` into two subsets.
+
+2. **Dynamic Programming Logic**:
+ - `dp[j]` represents the number of ways to form a sum `j` using the given numbers.
+ - Starting with `dp[0] = 1`, for each number in `nums`, we update `dp[j]` by adding the count of ways to form `j - num`.
+
+3. **Result**:
+ - After processing all numbers, `dp[S]` contains the count of ways to achieve the target sum.
+
+
+### **Example Walkthrough**
+**Input**: `nums = [1, 1, 1, 1, 1]`, `target = 3`
+1. `totalSum = 5`, S+ = (5 + 3) / 2 = 4
.
+2. Initialize DP array: `dp = [1, 0, 0, 0, 0]`.
+3. Process each number:
+ - For `1`: Update `dp`: `[1, 1, 0, 0, 0]`.
+ - For `1`: Update `dp`: `[1, 2, 1, 0, 0]`.
+ - For `1`: Update `dp`: `[1, 3, 3, 1, 0]`.
+ - For `1`: Update `dp`: `[1, 4, 6, 4, 1]`.
+ - For `1`: Update `dp`: `[1, 5, 10, 10, 5]`.
+4. Result: `dp[4] = 5`.
+
+
+### **Time Complexity**
+- **Time**: `O(n x S)`, where n is the length of `nums` and S is the target sum.
+- **Space**: `O(S)` for the DP array.
+
+
+### **Output for Example**
+**Input**: `nums = [1,1,1,1,1]`, `target = 3`
+**Output**: `5`
+
+
+This approach efficiently calculates the number of ways to form the target sum using dynamic programming. By reducing the problem to subset sum, we leverage the structure of DP for better performance.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
diff --git a/algorithms/000494-target-sum/solution.php b/algorithms/000494-target-sum/solution.php
new file mode 100644
index 000000000..a63fb0344
--- /dev/null
+++ b/algorithms/000494-target-sum/solution.php
@@ -0,0 +1,34 @@
+= $num; $j--) {
+ $dp[$j] += $dp[$j - $num];
+ }
+ }
+
+ // Step 5: Return the number of ways to achieve the target sum
+ return $dp[$S];
+ }
+}
\ No newline at end of file
diff --git a/algorithms/000502-ipo/README.md b/algorithms/000502-ipo/README.md
index c2f352793..e009a3442 100644
--- a/algorithms/000502-ipo/README.md
+++ b/algorithms/000502-ipo/README.md
@@ -1,6 +1,8 @@
502\. IPO
-Hard
+**Difficulty:** Hard
+
+**Topics:** `Array`, `Greedy`, `Sorting`, `Heap (Priority Queue)`
Suppose LeetCode will start its **IPO** soon. In order to sell a good price of its shares to Venture Capital, LeetCode would like to work on some projects to increase its capital before the **IPO**. Since it has limited resources, it can only finish at most `k` distinct projects before the **IPO**. Help LeetCode design the best way to maximize its total capital after finishing at most `k` distinct projects.
@@ -36,3 +38,74 @@ The answer is guaranteed to fit in a 32-bit signed integer.
- 1 <= n <= 105
- 0 <= profits[i] <= 104
- 0 <= capital[i] <= 109
+
+
+
+**Solution:**
+
+We need to pick the projects that will maximize the final capital after finishing at most `k` projects, given the initial capital `w`.
+
+### Approach
+
+1. **Sort Projects by Capital:**
+ - First, we need to filter the projects based on the available capital. For this, we sort the projects based on the minimum required capital. This allows us to efficiently access the projects that can be started given the current capital.
+
+2. **Use a Max-Heap to Track Profits:**
+ - We maintain a max-heap (priority queue) to keep track of the profits of the projects that can be undertaken with the current capital. This ensures that at each step, we can always choose the project with the highest profit.
+
+3. **Simulate the Process:**
+ - For each project up to `k`, we add all the projects that can be started with the current capital into the max-heap.
+ - Then, we select the project with the highest profit from the max-heap and add its profit to the current capital.
+ - We repeat this process until we have selected `k` projects or there are no more projects that can be started.
+
+Let's implement this solution in PHP: **[502. IPO](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/000502-ipo/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+1. **Initialization:** We start by preparing an array of projects where each project is represented as a tuple `(capital, profit)`. We sort this array by the required capital so that we can efficiently pick projects as the available capital increases.
+
+2. **Max-Heap for Profits:** We then iterate through the sorted projects and add all the projects that can be started with the current capital to a max-heap. The max-heap ensures that we always pick the most profitable project first.
+
+3. **Project Selection:** In each iteration, we extract the maximum profit from the heap and add it to our current capital. This process continues until we have selected `k` projects or no more projects can be started.
+
+This approach efficiently handles the constraints and ensures that we maximize the final capital.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
+
diff --git a/algorithms/000502-ipo/solution.php b/algorithms/000502-ipo/solution.php
index 2932c7123..a7ca7f0a8 100644
--- a/algorithms/000502-ipo/solution.php
+++ b/algorithms/000502-ipo/solution.php
@@ -10,37 +10,40 @@ class Solution {
* @return Integer
*/
function findMaximizedCapital($k, $w, $profits, $capital) {
- $n = count($capital); // Number of projects
+ // Combine the projects into a list of tuples (capital, profit)
+ $projects = [];
+ $n = count($profits);
- // Create a min-heap (priority queue) to keep track of projects based on required capital
- $minCapitalHeap = new SplMinHeap();
-
- // Populate the min-heap with project information - each item is an array where
- // the first element is the capital required and the second element is the profit.
- for ($i = 0; $i < $n; ++$i) {
- $minCapitalHeap->insert([$capital[$i], $profits[$i]]);
+ for ($i = 0; $i < $n; $i++) {
+ $projects[] = [$capital[$i], $profits[$i]];
}
- // Create a max-heap (priority queue) to keep track of profitable projects that we can afford
- $maxProfitHeap = new SplMaxHeap();
+ // Sort projects by the capital needed (ascending)
+ usort($projects, function($a, $b) {
+ return $a[0] - $b[0];
+ });
- // Iterate k times, which represents the maximum number of projects we can select
- while ($k-- > 0) {
+ // A max heap to store the available profits
+ $maxHeap = new SplMaxHeap();
- // Move all the projects that we can afford (w >= required capital) to the max profit heap
- while (!$minCapitalHeap->isEmpty() && $minCapitalHeap->top()[0] <= $w) {
- $maxProfitHeap->insert($minCapitalHeap->extract()[1]);
+ $index = 0;
+ for ($i = 0; $i < $k; $i++) {
+ // Push all projects that can be started with the current capital into the heap
+ while ($index < $n && $projects[$index][0] <= $w) {
+ $maxHeap->insert($projects[$index][1]);
+ $index++;
}
- // If the max profit heap is empty, it means there are no projects we can afford, so we break
- if ($maxProfitHeap->isEmpty()) {
+ // If there are no available projects, break out of the loop
+ if ($maxHeap->isEmpty()) {
break;
}
- // Otherwise, take the most profitable project from the max profit heap and add its profit to our total capital
- $w += $maxProfitHeap->extract();
+ // Pick the project with the maximum profit
+ $w += $maxHeap->extract();
}
- return $w; // Return the maximized capital after picking up to k projects
+ return $w;
+
}
}
\ No newline at end of file
diff --git a/algorithms/000506-relative-ranks/README.md b/algorithms/000506-relative-ranks/README.md
index a8d66a279..cf8744c3a 100644
--- a/algorithms/000506-relative-ranks/README.md
+++ b/algorithms/000506-relative-ranks/README.md
@@ -1,6 +1,8 @@
506\. Relative Ranks
-Easy
+**Difficulty:** Easy
+
+**Topics:** `Array`, `Sorting`, `Heap (Priority Queue)`
You are given an integer array `score` of size `n`, where `score[i]` is the score of the ith
athlete in a competition. All the scores are guaranteed to be **unique**.
@@ -32,3 +34,63 @@ Return an array `answer` of size `n` where `answer[i]` is the rank of the
- 0 <= score[i] <= 1066
- All the values in `score` are **unique**.
+
+
+**Solution:**
+
+We can follow these steps:
+
+1. **Sort the Scores**: We need to know the relative order of each score, so we sort the scores in descending order.
+2. **Assign Ranks**: Once we have the sorted scores, we can map the ranks to the original scores based on their positions.
+3. **Map the Ranks Back to the Original Array**: Using the original indices of the scores, assign the appropriate ranks.
+
+Let's implement this solution in PHP: **[506. Relative Ranks](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/000506-relative-ranks/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+1. **Sorting the Scores**:
+ - We use `arsort()` to sort the scores in descending order while maintaining the original indices. This is important because we need to assign ranks back to the original positions.
+
+2. **Assigning Ranks**:
+ - After sorting, the highest score gets the "Gold Medal", the second-highest gets the "Silver Medal", the third-highest gets the "Bronze Medal", and so on. For the 4th place and beyond, we simply assign the numeric rank as a string.
+
+3. **Mapping Ranks to the Original Array**:
+ - We then loop over the original score array and fill in the result array using the ranks we calculated, maintaining the original order.
+
+### Test Cases:
+
+- `[5, 4, 3, 2, 1]`: The output is `["Gold Medal","Silver Medal","Bronze Medal","4","5"]`, as the scores are already in descending order.
+- `[10, 3, 8, 9, 4]`: The output is `["Gold Medal","5","Bronze Medal","Silver Medal","4"]`, based on the descending order of scores `[10, 9, 8, 4, 3]`.
+
+This solution efficiently handles the problem within the constraints provided.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
+
diff --git a/algorithms/000506-relative-ranks/solution.php b/algorithms/000506-relative-ranks/solution.php
index ce5ce6a86..4d380e8a5 100644
--- a/algorithms/000506-relative-ranks/solution.php
+++ b/algorithms/000506-relative-ranks/solution.php
@@ -6,28 +6,37 @@ class Solution {
* @param Integer[] $score
* @return String[]
*/
- function findRelativeRanks(array $score): array
- {
+ function findRelativeRanks($score) {
$n = count($score);
- $ans = array_fill(0, $n, "");
- $indices = range(0, $n - 1);
+ $ranked = $score;
- usort($indices, function($a, $b) use ($score) {
- return $score[$a] > $score[$b] ? -1 : 1;
- });
+ // Step 1: Sort the scores in descending order while keeping track of original indices
+ arsort($ranked);
- for ($i = 0; $i < $n; $i++) {
- if ($i == 0) {
- $ans[$indices[0]] = "Gold Medal";
- } elseif ($i == 1) {
- $ans[$indices[1]] = "Silver Medal";
- } elseif ($i == 2) {
- $ans[$indices[2]] = "Bronze Medal";
+ // Step 2: Assign ranks
+ $ranks = array();
+ $index = 0;
+
+ foreach ($ranked as $originalIndex => $value) {
+ $index++;
+ if ($index == 1) {
+ $ranks[$originalIndex] = "Gold Medal";
+ } elseif ($index == 2) {
+ $ranks[$originalIndex] = "Silver Medal";
+ } elseif ($index == 3) {
+ $ranks[$originalIndex] = "Bronze Medal";
} else {
- $ans[$indices[$i]] = strval($i + 1);
+ $ranks[$originalIndex] = (string)$index;
}
}
- return $ans;
+ // Step 3: Prepare the result array based on the original scores' indices
+ $result = array();
+ for ($i = 0; $i < $n; $i++) {
+ $result[$i] = $ranks[$i];
+ }
+
+ return $result;
+
}
}
\ No newline at end of file
diff --git a/algorithms/000514-freedom-trail/README.md b/algorithms/000514-freedom-trail/README.md
index 0136269ed..7562d45b5 100644
--- a/algorithms/000514-freedom-trail/README.md
+++ b/algorithms/000514-freedom-trail/README.md
@@ -1,6 +1,8 @@
514\. Freedom Trail
-Hard
+**Difficulty:** Hard
+
+**Topics:** `Array`, `Dynamic Programming`, `Depth-First Search`, `Breadth-First Search`
In the video game Fallout 4, the quest **"Road to Freedom"** requires players to reach a metal dial called the **"Freedom Trail Ring"** and use the dial to spell a specific keyword to open the door.
@@ -37,4 +39,91 @@ So the final output is 4.
- `1 <= ring.length, key.length <= 100`.
- `ring` and `key` consist of only lower case English letters.
-- It is guaranteed that `key` could always be spelled by rotating `ring`.
\ No newline at end of file
+- It is guaranteed that `key` could always be spelled by rotating `ring`.
+
+
+
+**Solution:**
+
+Here's a breakdown of how we approach it:
+
+### Problem Understanding
+We are given two strings:
+- `ring`: the circular ring of characters.
+- `key`: the word we need to spell by rotating the ring.
+
+For each character in `key`, we rotate the `ring` to align the character at the top (index `0`), and this rotation can happen either clockwise or anticlockwise. The goal is to find the minimum number of rotations (steps) required to spell all the characters of `key`.
+
+### Steps for the Solution
+
+1. **Character Positions**:
+ - First, we precompute the positions of each character in `ring`. This allows us to efficiently look up all positions where a given character appears.
+
+2. **Dynamic Programming Table**:
+ - Let `dp[i][j]` represent the minimum number of steps required to spell the first `i` characters of `key`, ending with `key[i-1]` aligned at position `j` in `ring`.
+
+3. **Base Case**:
+ - For the first character of `key`, we initialize the DP table by calculating the steps needed to rotate `ring` to align `key[0]` with index `0`.
+
+4. **State Transition**:
+ - For each character in `key`, we iterate through all positions in `ring` where this character occurs. For each such position, we compute the minimum steps to move from every possible position of the previous character in `key`. The rotation can happen clockwise or anticlockwise, and we take the minimum steps needed for this transition.
+
+5. **Final Answer**:
+ - After processing all characters in `key`, the answer will be the minimum number of steps to spell the last character of `key` from any possible position in `ring`.
+
+Let's implement this solution in PHP: **[514. Freedom Trail](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/000514-freedom-trail/solution.php)**
+
+```php
+findRotateSteps("godding", "gd") . "\n"; // Output: 4
+echo $solution->findRotateSteps("godding", "godding") . "\n"; // Output: 13
+?>
+```
+
+### Explanation:
+
+1. **Preprocessing**:
+ - We create an array `$char_positions` where the key is a character, and the value is an array of all indices in `ring` where this character occurs. This helps in efficiently finding positions of each character when needed.
+
+2. **Dynamic Programming Table Initialization**:
+ - For the first character of `key`, we initialize the DP table by calculating how many steps are required to move the first character of `key` to the top position (`0` index of `ring`).
+
+3. **DP Table Filling**:
+ - For each character in `key`, we compute the minimum number of steps required to move from the previous character's positions to the current character's positions, updating the DP table with the minimum steps.
+
+4. **Result Extraction**:
+ - After processing all characters in `key`, the result is the minimum number of steps found in the last row of the DP table.
+
+### Time Complexity
+- Precomputing the character positions takes O(n), where `n` is the length of `ring`.
+- The DP solution iterates through each character in `key` and for each character, it checks all possible positions in `ring`, resulting in O(k * m^2) complexity, where `k` is the length of `key` and `m` is the number of positions of each character in `ring`.
+
+Thus, the solution efficiently handles the problem's constraints and gives the correct minimum steps to spell the `key`.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
diff --git a/algorithms/000514-freedom-trail/solution.php b/algorithms/000514-freedom-trail/solution.php
index 21621fdd2..d92f15ab1 100644
--- a/algorithms/000514-freedom-trail/solution.php
+++ b/algorithms/000514-freedom-trail/solution.php
@@ -1,57 +1,43 @@
[0, 104]
.
+- -231 <= Node.val <= 231 - 1
+
+
+**Solution:**
+
+The problem **"Find Largest Value in Each Tree Row"** requires identifying the largest value present at each level (row) of a binary tree. Given a binary tree, the goal is to traverse the tree row by row and collect the maximum value from each row. This problem involves fundamental tree traversal techniques such as **Breadth-First Search (BFS)** or **Depth-First Search (DFS)**.
+
+### **Key Points**
+1. **Tree Traversal**: The solution involves traversing all levels of the binary tree to identify the largest value at each level.
+2. **Breadth-First Search (BFS)**: BFS is suitable for level-by-level traversal, which simplifies finding the largest value in each row.
+3. **Constraints**: Handle edge cases like an empty tree and nodes with large or small integer values within the constraint range.
+
+
+### **Approach**
+The most straightforward approach to finding the largest value in each row is using **BFS**:
+- Traverse the tree level by level.
+- For each level, keep track of the largest value.
+
+Alternatively, **DFS** can also be used:
+- Recursively traverse the tree and maintain a record of the maximum value at each depth.
+
+
+### **Plan**
+1. Initialize an array to store the largest values of each row.
+2. Use a queue for BFS traversal:
+ - Start with the root node.
+ - Process all nodes at the current level before moving to the next.
+3. For each level:
+ - Iterate through all nodes and find the maximum value.
+ - Add this value to the result array.
+4. Return the result array after completing the traversal.
+
+
+### **Solution Steps**
+1. **Check Input**: If the root is null, return an empty array.
+2. **Setup BFS**:
+ - Use a queue initialized with the root node.
+ - Initialize an empty result array.
+3. **Traverse Levels**:
+ - For each level, track the maximum value.
+ - Add child nodes to the queue for the next level.
+4. **Update Results**:
+ - Append the maximum value of each level to the result array.
+5. **Return Results**: Return the result array containing the largest values for each row.
+
+Let's implement this solution in PHP: **[515. Find Largest Value in Each Tree Row](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/000515-find-largest-value-in-each-tree-row/solution.php)**
+
+```php
+val = $val;
+ $this->left = $left;
+ $this->right = $right;
+ }
+}
+
+/**
+ * @param TreeNode $root
+ * @return Integer[]
+ */
+function largestValues($root) {
+ ...
+ ...
+ ...
+ /**
+ * go to ./solution.php
+ */
+}
+
+// Example usage:
+$root = new TreeNode(1);
+$root->left = new TreeNode(3);
+$root->right = new TreeNode(2);
+$root->left->left = new TreeNode(5);
+$root->left->right = new TreeNode(3);
+$root->right->right = new TreeNode(9);
+
+print_r(largestValues($root)); // Output: [1, 3, 9]
+?>
+```
+
+### Explanation:
+
+#### Input: `[1,3,2,5,3,null,9]`
+1. **Level 0**: Node values: `[1]` β Maximum: `1`.
+2. **Level 1**: Node values: `[3, 2]` β Maximum: `3`.
+3. **Level 2**: Node values: `[5, 3, 9]` β Maximum: `9`.
+#### Output: `[1, 3, 9]`.
+
+
+### **Time Complexity**
+- **BFS Traversal**: Each node is processed once β **O(n)**.
+- **Finding Maximum**: Done during traversal β **O(1)** per level.
+- **Total**: **O(n)**.
+
+### **Space Complexity**
+- **Queue Storage**: At most, the width of the tree (number of nodes at the largest level) β **O(w)** where `w` is the maximum width of the tree.
+
+
+### **Output for Example**
+Input: `root = [1,3,2,5,3,null,9]`
+Output: `[1, 3, 9]`.
+
+
+This BFS-based solution efficiently computes the largest value in each tree row with linear time complexity. It handles large trees, negative values, and edge cases like empty trees effectively.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
diff --git a/algorithms/000515-find-largest-value-in-each-tree-row/solution.php b/algorithms/000515-find-largest-value-in-each-tree-row/solution.php
new file mode 100644
index 000000000..5312c995d
--- /dev/null
+++ b/algorithms/000515-find-largest-value-in-each-tree-row/solution.php
@@ -0,0 +1,49 @@
+val = $val;
+ * $this->left = $left;
+ * $this->right = $right;
+ * }
+ * }
+ */
+class Solution {
+
+ /**
+ * @param TreeNode $root
+ * @return Integer[]
+ */
+ function largestValues($root) {
+ if ($root === null) return [];
+
+ $queue = [$root];
+ $result = [];
+
+ while (!empty($queue)) {
+ $levelSize = count($queue);
+ $maxValue = PHP_INT_MIN;
+
+ for ($i = 0; $i < $levelSize; $i++) {
+ $currentNode = array_shift($queue);
+ $maxValue = max($maxValue, $currentNode->val);
+
+ if ($currentNode->left !== null) {
+ $queue[] = $currentNode->left;
+ }
+ if ($currentNode->right !== null) {
+ $queue[] = $currentNode->right;
+ }
+ }
+
+ $result[] = $maxValue;
+ }
+
+ return $result;
+ }
+}
\ No newline at end of file
diff --git a/algorithms/000523-continuous-subarray-sum/README.md b/algorithms/000523-continuous-subarray-sum/README.md
index 4418a352f..cac4703a2 100644
--- a/algorithms/000523-continuous-subarray-sum/README.md
+++ b/algorithms/000523-continuous-subarray-sum/README.md
@@ -1,6 +1,8 @@
523\. Continuous Subarray Sum
-Medium
+**Difficulty:** Medium
+
+**Topics:** `Array`, `Hash Table`, `Math`, `Prefix Sum`
Given an integer array nums and an integer k, return `true` _if `nums` has a **good subarray** or false otherwise_.
@@ -39,3 +41,94 @@ Note that:
- 0 <= nums[i] <= 109
- 0 <= sum(nums[i]) <= 231 - 1
- 1 <= k <= 231 - 1
+
+
+
+**Solution:**
+
+We need to check whether there is a subarray of at least two elements whose sum is a multiple of `k`.
+
+### Key Observations:
+
+1. **Modulo Property:**
+ The sum of a subarray can be reduced to the remainder (modulo `k`). Specifically, for any two indices `i` and `j` (where `i < j`), if the prefix sums `prefix_sum[j] % k == prefix_sum[i] % k`, then the sum of the subarray between `i+1` and `j` is a multiple of `k`. This is because the difference between these prefix sums is divisible by `k`.
+
+2. **HashMap for Prefix Modulo:**
+ We'll store the modulo values of prefix sums in a hash table (or associative array). If the same modulo value repeats at a later index, it means the subarray sum between these indices is divisible by `k`.
+
+3. **Handling Edge Cases:**
+ - If `k == 0`, we simply need to check if any subarray has a sum of `0`.
+ - If the subarray length is less than 2, we ignore it.
+
+### Solution Strategy:
+
+1. Initialize a hashmap (associative array) to store the modulo of the prefix sum.
+2. Traverse the array and calculate the cumulative sum. For each element, compute the modulo with `k`.
+3. If the same modulo value has already been seen and the subarray length is at least 2, return `true`.
+4. Store the current modulo and its index in the hashmap if not already present.
+
+Let's implement this solution in PHP: **[523. Continuous Subarray Sum](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/000523-continuous-subarray-sum/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+1. **`mod_map` Initialization:**
+ We initialize the `mod_map` with a key `0` and value `-1`. This is used to handle cases where a subarray from the start of the array is divisible by `k`.
+
+2. **Iterating through `nums`:**
+ We calculate the cumulative sum as we iterate through the array. For each element, we compute the sum modulo `k`.
+
+3. **Modulo Check:**
+ If the current modulo value has already been seen in the `mod_map`, it means there is a subarray whose sum is divisible by `k`. We also ensure the subarray length is greater than or equal to 2 by checking if the difference in indices is more than 1.
+
+4. **Return Result:**
+ - If a valid subarray is found, we return `true`.
+ - If we finish iterating through the array without finding such a subarray, we return `false`.
+
+### Time Complexity:
+
+- **Time Complexity:** O(n), where `n` is the length of the array. We traverse the array once.
+- **Space Complexity:** O(min(n, k)), since we store at most `k` unique remainders in the hashmap.
+
+This solution is efficient and works within the problem's constraints.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
diff --git a/algorithms/000523-continuous-subarray-sum/solution.php b/algorithms/000523-continuous-subarray-sum/solution.php
index 8dfa0a7ea..f74f92191 100644
--- a/algorithms/000523-continuous-subarray-sum/solution.php
+++ b/algorithms/000523-continuous-subarray-sum/solution.php
@@ -8,24 +8,33 @@ class Solution {
* @return Boolean
*/
function checkSubarraySum($nums, $k) {
- $prefixMod = 0;
- $modSeen = array();
- $modSeen[0] = -1;
+ // Initialize a hashmap to store the remainder of prefix sums
+ $mod_map = array(0 => -1); // Initialize with 0 mod value at index -1 for convenience
+ $sum = 0;
+ // Iterate through the array
for ($i = 0; $i < count($nums); $i++) {
- $prefixMod = ($prefixMod + $nums[$i]) % $k;
+ $sum += $nums[$i];
- if (array_key_exists($prefixMod, $modSeen)) {
- // ensures that the size of subarray is atleast 2
- if ($i - $modSeen[$prefixMod] > 1) {
- return 1;
+ // Calculate the remainder of the sum when divided by k
+ if ($k != 0) {
+ $sum %= $k;
+ }
+
+ // If the same remainder has been seen before, we have a subarray sum that is divisible by k
+ if (isset($mod_map[$sum])) {
+ // Ensure the subarray length is at least 2
+ if ($i - $mod_map[$sum] > 1) {
+ return true;
}
} else {
- // mark the value of prefixMod with the current index.
- $modSeen[$prefixMod] = $i;
+ // Store the index of this remainder in the hashmap
+ $mod_map[$sum] = $i;
}
}
- return 0;
+ // No such subarray found
+ return false;
+
}
}
\ No newline at end of file
diff --git a/algorithms/000539-minimum-time-difference/README.md b/algorithms/000539-minimum-time-difference/README.md
new file mode 100644
index 000000000..151a3a877
--- /dev/null
+++ b/algorithms/000539-minimum-time-difference/README.md
@@ -0,0 +1,93 @@
+539\. Minimum Time Difference
+
+**Difficulty:** Medium
+
+**Topics:** `Array`, `Math`, `String`, `Sorting`
+
+Given a list of 24-hour clock time points in **"HH:MM"** format, return _the minimum **minutes** difference between any two time-points in the list_.
+
+**Example 1:**
+
+- **Input:** timePoints = ["23:59","00:00"]
+- **Output:** 1
+
+**Example 2:**
+
+- **Input:** timePoints = ["00:00","23:59","00:00"]
+- **Output:** 0
+
+**Constraints:**
+
+- 2 <= timePoints.length <= 2 * 104
+- `timePoints[i]` is in the format **"HH:MM"**.
+
+
+**Solution:**
+
+The approach can be broken down as follows:
+
+### Approach:
+
+1. **Convert time points to minutes**:
+ Each time point is in `"HH:MM"` format, so we can convert it into the total number of minutes from `00:00`. For example, `"23:59"` would be `23 * 60 + 59 = 1439` minutes.
+
+2. **Sort the time points**:
+ Once all the time points are converted into minutes, sort them to calculate the differences between consecutive time points.
+
+3. **Handle the circular nature of the clock**:
+ The difference between the first and last time points also needs to be considered, because of the circular nature of the clock. This can be handled by adding `1440` (24 hours) to the last time point and comparing it with the first time point.
+
+4. **Find the minimum difference**:
+ After sorting, find the minimum difference between consecutive time points, including the difference between the first and last time points.
+
+
+Let's implement this solution in PHP: **[539. Minimum Time Difference](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/000539-minimum-time-difference/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+1. **`array_map()`**: This function is used to convert each time point from `"HH:MM"` format into the total number of minutes from `00:00`.
+2. **`sort($minutes)`**: After converting the time points into minutes, we sort them to calculate the difference between consecutive time points.
+3. **Calculate consecutive differences**: The difference between consecutive time points is computed and tracked as the minimum difference.
+4. **Circular difference**: The difference between the last and the first time point is calculated as `1440 - last + first`, which ensures that the circular nature of the clock is accounted for.
+
+### Time Complexity:
+- **Sorting the array** takes _**O(n log n)**_, where _**n **_ is the number of time points.
+- **Comparing consecutive time points** takes _**O(n)**_.
+- Overall time complexity: _**O(n log n)**_, which is efficient for _**n**_ up to 20,000 (as given in the constraints).
+
+This solution should work efficiently within the given problem constraints.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
diff --git a/algorithms/000539-minimum-time-difference/solution.php b/algorithms/000539-minimum-time-difference/solution.php
new file mode 100644
index 000000000..a0cec0e0f
--- /dev/null
+++ b/algorithms/000539-minimum-time-difference/solution.php
@@ -0,0 +1,34 @@
+1 <= n <= 109
\ No newline at end of file
+- 1 <= n <= 109
+
+
+
+**Solution:**
+
+We can use a dynamic programming approach. Given the constraints, especially the high value for `n`, an efficient solution is required.
+
+Here's a step-by-step solution approach:
+
+### Solution Approach
+
+1. **Define the State Variables:**
+ We use dynamic programming to keep track of the number of valid records based on:
+ - Number of absences
+ - Number of consecutive late days
+
+ Let:
+ - `dp[i][0][0]` be the number of valid records of length `i` with 0 absences and no late days.
+ - `dp[i][0][1]` be the number of valid records of length `i` with 0 absences and 1 late day.
+ - `dp[i][0][2]` be the number of valid records of length `i` with 0 absences and 2 consecutive late days.
+ - `dp[i][1][0]` be the number of valid records of length `i` with 1 absence and no late days.
+ - `dp[i][1][1]` be the number of valid records of length `i` with 1 absence and 1 late day.
+ - `dp[i][1][2]` be the number of valid records of length `i` with 1 absence and 2 consecutive late days.
+
+2. **Base Case:**
+ - Initialize the DP table for length 1.
+
+3. **State Transition:**
+ - For each record of length `i`, determine how it can be extended to a record of length `i+1`.
+
+4. **Final Calculation:**
+ - Sum up all valid records of length `n` that meet the criteria.
+
+Let's implement this solution in PHP: **[552. Student Attendance Record II](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/000552-student-attendance-record-ii/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+- **Initialization:** We start with records of length 0. For each additional day, we update our counts based on the possible records of the previous day.
+- **Transition:** For each state, we determine how it transitions to the next state based on adding 'P', 'A', or 'L'.
+- **Final Calculation:** We sum up all valid states at the end to get the total number of valid records.
+
+This approach efficiently handles the problem using dynamic programming, considering the constraints and ensuring modular arithmetic to manage large numbers.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
diff --git a/algorithms/000564-find-the-closest-palindrome/README.md b/algorithms/000564-find-the-closest-palindrome/README.md
new file mode 100644
index 000000000..aba2b9dd4
--- /dev/null
+++ b/algorithms/000564-find-the-closest-palindrome/README.md
@@ -0,0 +1,117 @@
+564\. Find the Closest Palindrome
+
+**Difficulty:** Hard
+
+**Topics:** `Math`, `String`
+
+Given a string `n` representing an integer, return _the closest integer (not including itself), which is a palindrome-. If there is a tie, return **the smaller one**.
+
+The closest is defined as the absolute difference minimized between two integers.
+
+**Example 1:**
+
+- **Input:** n = "123"
+- **Output:** "121"
+
+**Example 2:**
+
+- **Input:** n = "1"
+- **Output:** "0"
+- **Explanation:** 0 and 2 are the closest palindromes but we return the smallest which is 0.
+
+**Constraints:**
+
+- `1 <= n.length <= 18`
+- `n` consists of only digits.
+- `n` does not have leading zeros.
+- `n` is representing an integer in the range [1, 1018 - 1]
.
+
+**Hint:**
+1. Will brute force work for this problem? Think of something else.
+2. Take some examples like 1234, 999,1000, etc and check their closest palindromes. How many different cases are possible?
+3. Do we have to consider only left half or right half of the string or both?
+4. Try to find the closest palindrome of these numbers- 12932, 99800, 12120. Did you observe something?
+
+
+
+
+**Solution:**
+
+We'll focus on creating a function that generates potential palindrome candidates and then selects the one closest to the input number.
+
+### Solution Approach:
+
+1. **Identify Palindrome Candidates**:
+ - Mirror the first half of the number to form a palindrome.
+ - Consider edge cases like all digits being `9`, `100...001`, or `99...99`.
+ - Generate palindromes by modifying the middle of the number up or down by 1.
+
+2. **Calculate the Closest Palindrome**:
+ - For each palindrome candidate, compute the absolute difference with the original number.
+ - Return the palindrome with the smallest difference. If there's a tie, return the smaller palindrome.
+
+
+Let's implement this solution in PHP: **[564. Find the Closest Palindrome](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/000564-find-the-closest-palindrome/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+- **generatePalindrome($firstHalf, $isOddLength)**:
+ - This helper function creates a palindrome by mirroring the first half of the number.
+```php
+
+```
+
+- **Edge Cases**:
+ - Palindromes generated from numbers like `100...001` or `99...99` are handled by explicitly checking these cases.
+
+- **Main Logic**:
+ - We calculate possible palindromes and then find the closest one by comparing absolute differences.
+
+This solution efficiently narrows down possible palindrome candidates and picks the closest one by considering only a few options, making it much faster than brute-force approaches.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
+
diff --git a/algorithms/000564-find-the-closest-palindrome/solution.php b/algorithms/000564-find-the-closest-palindrome/solution.php
new file mode 100644
index 000000000..15497d36a
--- /dev/null
+++ b/algorithms/000564-find-the-closest-palindrome/solution.php
@@ -0,0 +1,58 @@
+generatePalindrome($firstHalf, $isOddLength);
+ $candidates[] = $mirroredPalindrome;
+
+ // Adjust the first half up and down by 1
+ $firstHalfPlusOne = strval(intval($firstHalf) + 1);
+ $candidates[] = $this->generatePalindrome($firstHalfPlusOne, $isOddLength);
+
+ $firstHalfMinusOne = strval(intval($firstHalf) - 1);
+ $candidates[] = $this->generatePalindrome($firstHalfMinusOne, $isOddLength);
+
+ // Edge case: 10...01 and 9...9
+ $candidates[] = strval(pow(10, $length - 1) - 1);
+ $candidates[] = strval(pow(10, $length) + 1);
+
+ // Find the closest palindrome
+ $nearestPalindromic = null;
+ $minDifference = PHP_INT_MAX;
+
+ foreach ($candidates as $candidate) {
+ if ($candidate !== $n) {
+ $diff = abs(intval($n) - intval($candidate));
+ if ($diff < $minDifference || ($diff == $minDifference && intval($candidate) < intval($nearestPalindromic))) {
+ $minDifference = $diff;
+ $nearestPalindromic = $candidate;
+ }
+ }
+ }
+
+ return $nearestPalindromic;
+ }
+
+ /**
+ * @param $firstHalf
+ * @param $isOddLength
+ * @return string
+ */
+ private function generatePalindrome($firstHalf, $isOddLength) {
+ $secondHalf = strrev(substr($firstHalf, 0, $isOddLength ? -1 : $firstHalf));
+ return $firstHalf . $secondHalf;
+ }
+
+}
\ No newline at end of file
diff --git a/algorithms/000567-permutation-in-string/README.md b/algorithms/000567-permutation-in-string/README.md
new file mode 100644
index 000000000..fa3523aa3
--- /dev/null
+++ b/algorithms/000567-permutation-in-string/README.md
@@ -0,0 +1,119 @@
+567\. Permutation in String
+
+**Difficulty:** Medium
+
+**Topics:** `Hash Table`, `Two Pointers`, `String`, `Sliding Window`
+
+Given two strings `s1` and `s2`, return _`true` if `s2` contains a permutation[^1] of `s1`, or `false` otherwise_.
+
+In other words, return _`true` if one of `s1`'s permutations is the substring of `s2`_.
+
+**Example 1:**
+
+- **Input:** s1 = "ab", s2 = "eidbaooo"
+- **Output:** true
+- **Explanation:** s2 contains one permutation of s1 ("ba").
+
+**Example 2:**
+
+- **Input:** s1 = "ab", s2 = "eidboaoo"
+- **Output:** false
+
+
+
+**Constraints:**
+
+- 1 <= s1.length, s2.length <= 104
+- `s1` and `s2` consist of lowercase English letters.
+
+
+**Hint:**
+1. Obviously, brute force will result in TLE. Think of something else.
+2. How will you check whether one string is a permutation of another string?
+3. One way is to sort the string and then compare. But, Is there a better way?
+4. If one string is a permutation of another string then they must have one common metric. What is that?
+5. Both strings must have same character frequencies, if one is permutation of another. Which data structure should be used to store frequencies?
+6. What about hash table? An array of size 26?
+
+[^1]: **Permutation** `A permutation is a rearrangement of all the characters of a string.`
+
+
+**Solution:**
+
+We can use the **sliding window** technique with a frequency counter, rather than trying every possible substring permutation (which would be too slow for large inputs).
+
+### Key Idea:
+1. If a permutation of `s1` is a substring of `s2`, both strings must have the same character frequencies for the letters present in `s1`.
+2. We can use a sliding window of length `s1` over `s2` to check if the substring within the window is a permutation of `s1`.
+
+### Steps:
+- Create a frequency counter for `s1` (i.e., count the occurrences of each character).
+- Slide a window over `s2` of size equal to `s1` and check if the frequency of characters in the window matches the frequency of characters in `s1`.
+- If the frequencies match, it means that the substring in the window is a permutation of `s1`.
+
+### Algorithm:
+1. Create a frequency array (`count1`) for `s1`.
+2. Use another frequency array (`count2`) for the current window in `s2`.
+3. Slide the window over `s2` and update the frequency array for the window as you move.
+4. If the frequency arrays match at any point, return `true`.
+5. If you slide through all of `s2` without finding a match, return `false`.
+
+Let's implement this solution in PHP: **[567. Permutation in String](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/000567-permutation-in-string/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+1. **Frequency Arrays**:
+ - We use two arrays (`count1` for `s1` and `count2` for the sliding window in `s2`) of size 26, corresponding to the 26 lowercase letters.
+ - Each array keeps track of how many times each character appears.
+
+2. **Sliding Window**:
+ - We first initialize the frequency of the first window (the first `len1` characters of `s2`) and compare it to `count1`.
+ - Then, we slide the window one character at a time. For each step, we update the frequency array by:
+ - Adding the character that is entering the window.
+ - Removing the character that is leaving the window.
+ - After each slide, we compare the two frequency arrays.
+
+3. **Efficiency**:
+ - Instead of recalculating the frequency for every new window, we efficiently update the frequency array by adjusting just two characters.
+ - This gives an overall time complexity of **O(n)**, where `n` is the length of `s2`.
+
+### Time and Space Complexity:
+- **Time Complexity**: `O(n)` where `n` is the length of `s2`. We iterate over `s2` once, updating the frequency arrays in constant time.
+- **Space Complexity**: `O(1)`, as we are only using fixed-size arrays (size 26) to store the frequency counts of characters.
+
+This approach ensures that the solution is efficient even for larger inputs.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
+
+
+
diff --git a/algorithms/000567-permutation-in-string/solution.php b/algorithms/000567-permutation-in-string/solution.php
new file mode 100644
index 000000000..805dcc830
--- /dev/null
+++ b/algorithms/000567-permutation-in-string/solution.php
@@ -0,0 +1,51 @@
+ $len2) {
+ return false;
+ }
+
+ // Initialize frequency arrays for s1 and the current window of s2
+ $count1 = array_fill(0, 26, 0);
+ $count2 = array_fill(0, 26, 0);
+
+ // Fill the count array for s1 and the first window of s2
+ for ($i = 0; $i < $len1; $i++) {
+ $count1[ord($s1[$i]) - ord('a')]++;
+ $count2[ord($s2[$i]) - ord('a')]++;
+ }
+
+ // Check if the first window matches
+ if ($count1 == $count2) {
+ return true;
+ }
+
+ // Start sliding the window
+ for ($i = $len1; $i < $len2; $i++) {
+ // Add the current character to the window
+ $count2[ord($s2[$i]) - ord('a')]++;
+
+ // Remove the character that is left out of the window
+ $count2[ord($s2[$i - $len1]) - ord('a')]--;
+
+ // Check if the window matches
+ if ($count1 == $count2) {
+ return true;
+ }
+ }
+
+ // If no matching window was found, return false
+ return false;
+ }
+}
\ No newline at end of file
diff --git a/algorithms/000590-n-ary-tree-postorder-traversal/README.md b/algorithms/000590-n-ary-tree-postorder-traversal/README.md
new file mode 100644
index 000000000..ad62b80ff
--- /dev/null
+++ b/algorithms/000590-n-ary-tree-postorder-traversal/README.md
@@ -0,0 +1,144 @@
+590\. N-ary Tree Postorder Traversa
+
+
+**Difficulty:** Easy
+
+**Topics:** `Stack`, `Tree`, `Depth-First Search`
+
+Given the `root` of an n-ary tree, return _the postorder traversal of its nodes' values_.
+
+Nary-Tree input serialization is represented in their level order traversal. Each group of children is separated by the null value (See examples)
+
+**Example 1:**
+
+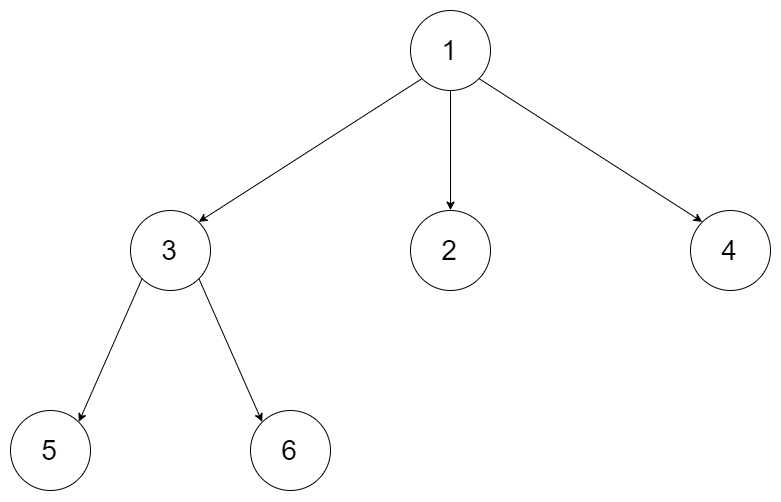
+
+- **Input:** root = [1,null,3,2,4,null,5,6]
+- **Output:** [5,6,3,2,4,1]
+
+**Example 2:**
+
+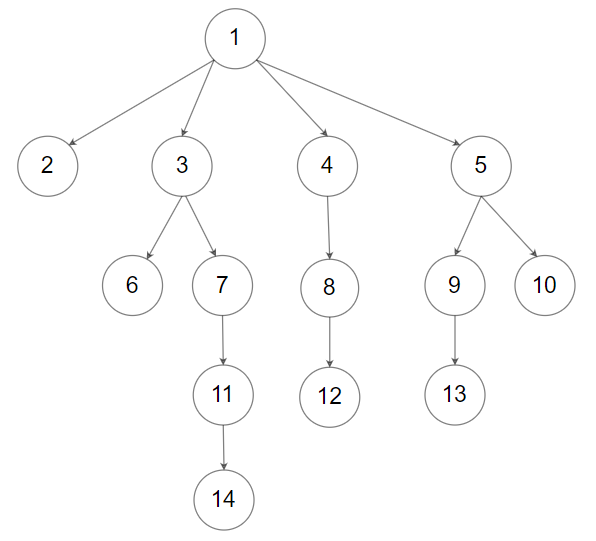
+
+- **Input:** root = [1,null,2,3,4,5,null,null,6,7,null,8,null,9,10,null,null,11,null,12,null,13,null,null,14]
+- **Output:** [2,6,14,11,7,3,12,8,4,13,9,10,5,1]
+
+
+**Constraints:**
+
+- The number of the nodes in the tree is in the range [0, 1004]
.
+- -100 <= Node.val <= 1004
+- The height of the n-ary tree is less than or equal to `1000`.
+
+**Follow up:** Recursive solution is trivial, could you do it iteratively?
+
+
+
+**Solution:**
+
+We can approach it both recursively and iteratively. Since the follow-up asks for an iterative solution, we'll focus on that. Postorder traversal means visiting the children nodes first and then the parent node.
+
+
+Let's implement this solution in PHP: **[590. N-ary Tree Postorder Traversal](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/000590-n-ary-tree-postorder-traversal/solution.php)**
+
+```php
+val = $val;
+ }
+}
+
+/**
+ * @param Node $root
+ * @return integer[]
+ */
+function postorder($root) {
+ ...
+ ...
+ ...
+ /**
+ * go to ./solution.php
+ */
+}
+
+// Example 1:
+$root1 = new Node(1);
+$root1->children = [
+ $node3 = new Node(3),
+ new Node(2),
+ new Node(4)
+];
+$node3->children = [
+ new Node(5),
+ new Node(6)
+];
+
+print_r(postorder($root1)); // Output: [5, 6, 3, 2, 4, 1]
+
+// Example 2:
+$root2 = new Node(1);
+$root2->children = [
+ new Node(2),
+ $node3 = new Node(3),
+ $node4 = new Node(4),
+ $node5 = new Node(5)
+];
+$node3->children = [
+ $node6 = new Node(6),
+ $node7 = new Node(7)
+];
+$node4->children = [
+ $node8 = new Node(8)
+];
+$node5->children = [
+ $node9 = new Node(9),
+ $node10 = new Node(10)
+];
+$node7->children = [
+ new Node(11)
+];
+$node8->children = [
+ new Node(12)
+];
+$node9->children = [
+ new Node(13)
+];
+$node11 = $node7->children[0];
+$node11->children = [
+ new Node(14)
+];
+
+print_r(postorder($root2)); // Output: [2, 6, 14, 11, 7, 3, 12, 8, 4, 13, 9, 10, 5, 1]
+?>
+```
+
+### Explanation:
+
+1. **Initialization**:
+ - Create a stack and push the root node onto it.
+ - Create an empty array `result` to store the final postorder traversal.
+
+2. **Traversal**:
+ - Pop the node from the stack, insert its value at the beginning of the `result` array.
+ - Push all its children onto the stack.
+ - Continue until the stack is empty.
+
+3. **Result**:
+ - The result array will contain the nodes in postorder after the loop finishes.
+
+This iterative approach effectively simulates the postorder traversal by using a stack and reversing the process typically done by recursion.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
+
diff --git a/algorithms/000590-n-ary-tree-postorder-traversal/solution.php b/algorithms/000590-n-ary-tree-postorder-traversal/solution.php
new file mode 100644
index 000000000..7a287280c
--- /dev/null
+++ b/algorithms/000590-n-ary-tree-postorder-traversal/solution.php
@@ -0,0 +1,38 @@
+val = $val;
+ * $this->children = array();
+ * }
+ * }
+ */
+
+class Solution {
+ /**
+ * @param Node $root
+ * @return integer[]
+ */
+ function postorder($root) {
+ if ($root === null) {
+ return [];
+ }
+
+ $result = [];
+ $stack = [$root];
+
+ while (!empty($stack)) {
+ $node = array_pop($stack);
+ array_unshift($result, $node->val);
+ foreach ($node->children as $child) {
+ $stack[] = $child;
+ }
+ }
+
+ return $result;
+ }
+}
\ No newline at end of file
diff --git a/algorithms/000592-fraction-addition-and-subtraction/README.md b/algorithms/000592-fraction-addition-and-subtraction/README.md
new file mode 100644
index 000000000..6d39c991a
--- /dev/null
+++ b/algorithms/000592-fraction-addition-and-subtraction/README.md
@@ -0,0 +1,79 @@
+592\. Fraction Addition and Subtraction
+
+**Difficulty:** Medium
+
+**Topics:** `Math`, `String`, `Simulation`
+
+Given a string `expression` representing an expression of fraction addition and subtraction, return the calculation result in string format.
+
+The final result should be an [irreducible fraction](https://en.wikipedia.org/wiki/Irreducible_fraction). If your final result _is an integer, change it to the format of a fraction that has a denominator `1`_. So in this case, `2` should be converted to `2/1`.
+
+**Example 1:**
+
+- **Input:** expression = "-1/2+1/2"
+- **Output:** "0/1"
+
+**Example 2:**
+
+- **Input:** expression = "-1/2+1/2+1/3"
+- **Output:** "1/3"
+
+**Example 3:**
+
+- **Input:** expression = "1/3-1/2"
+- **Output:** "-1/6"
+
+
+**Constraints:**
+
+
+- The input string only contains `'0'` to `'9'`, `'/'`, `'+'` and `'-'`. So does the output.
+- Each fraction (input and output) has the format `Β±numerator/denominator`. If the first input fraction or the output is positive, then `'+'` will be omitted.
+- The input only contains valid **irreducible fractions**, where the **numerator** and **denominator** of each fraction will always be in the range `[1, 10]`. If the denominator is `1`, it means this fraction is actually an integer in a fraction format defined above.
+- The number of given fractions will be in the range `[1, 10]`.
+- The numerator and denominator of the **final result** are guaranteed to be valid and in the range of **32-bit** int.
+
+
+**Solution:**
+
+We need to carefully parse the input string and perform arithmetic operations on the fractions. The steps are as follows:
+
+1. **Parse the Input Expression**: Extract individual fractions from the expression string.
+2. **Compute the Result**: Add or subtract the fractions step by step.
+3. **Simplify the Result**: Convert the final fraction to its irreducible form.
+
+Let's implement this solution in PHP: **[592. Fraction Addition and Subtraction](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/000592-fraction-addition-and-subtraction/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+- **gcd function**: Computes the greatest common divisor of two numbers, which helps in simplifying the fraction.
+- **addFractions function**: Adds two fractions. It computes the common denominator, adjusts the numerators accordingly, adds them, and then simplifies the resulting fraction.
+- **fractionAddition function**: This is the main function that parses the input expression, uses regular expressions to extract all the fractions, and iteratively adds them up using the `addFractions` function.
+
+### Test Cases:
+- `fractionAddition("-1/2+1/2")` returns `"0/1"`.
+- `fractionAddition("-1/2+1/2+1/3")` returns `"1/3"`.
+- `fractionAddition("1/3-1/2")` returns `"-1/6"`.
+
+This solution handles all the required operations and returns the correct output for each given expression.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
+
diff --git a/algorithms/000592-fraction-addition-and-subtraction/solution.php b/algorithms/000592-fraction-addition-and-subtraction/solution.php
new file mode 100644
index 000000000..f09afab00
--- /dev/null
+++ b/algorithms/000592-fraction-addition-and-subtraction/solution.php
@@ -0,0 +1,62 @@
+addFractions($numerator, $denominator, intval($num), intval($den));
+ }
+
+ // Return the result as a fraction
+ return $numerator . '/' . $denominator;
+
+ }
+
+ /**
+ * @param $a
+ * @param $b
+ * @return float|int
+ */
+ function gcd($a, $b) {
+ while ($b != 0) {
+ $temp = $b;
+ $b = $a % $b;
+ $a = $temp;
+ }
+ return abs($a);
+ }
+
+ /**
+ * @param $numerator1
+ * @param $denominator1
+ * @param $numerator2
+ * @param $denominator2
+ * @return float[]|int[]
+ */
+ function addFractions($numerator1, $denominator1, $numerator2, $denominator2) {
+ // Find the common denominator
+ $commonDenominator = $denominator1 * $denominator2;
+ $newNumerator = $numerator1 * $denominator2 + $numerator2 * $denominator1;
+
+ // Simplify the fraction
+ $commonGCD = $this->gcd($newNumerator, $commonDenominator);
+ $simplifiedNumerator = $newNumerator / $commonGCD;
+ $simplifiedDenominator = $commonDenominator / $commonGCD;
+
+ return [$simplifiedNumerator, $simplifiedDenominator];
+ }
+
+}
\ No newline at end of file
diff --git a/algorithms/000623-add-one-row-to-tree/README.md b/algorithms/000623-add-one-row-to-tree/README.md
index 39fcef45d..7246e6d32 100644
--- a/algorithms/000623-add-one-row-to-tree/README.md
+++ b/algorithms/000623-add-one-row-to-tree/README.md
@@ -1,6 +1,8 @@
623\. Add One Row to Tree
-Medium
+**Difficulty:** Medium
+
+**Topics:** `Tree`, `Depth-First Search`, `Breadth-First Search`, `Binary Tree`
Given the `root` of a binary tree and two integers `val` and `depth`, add a row of nodes with value `val` at the given depth `depth`.
@@ -33,4 +35,128 @@ The adding rule is:
- The depth of the tree is in the range [1, 104]
.
- `-100 <= Node.val <= 100`
- `-105 <= val <= 105`
-- `1 <= depth <= the depth of tree + 1`
\ No newline at end of file
+- `1 <= depth <= the depth of tree + 1`
+
+
+
+**Solution:**
+
+We can use either Breadth-First Search (BFS) or Depth-First Search (DFS) to traverse the tree. The idea is to add the new row at the specified depth.
+
+### Approach:
+1. **Edge Case**: If the `depth` is 1, we need to add the new node as the new root of the tree.
+2. **DFS or BFS**: Traverse the tree to reach nodes at `depth - 1`, then:
+ - Insert new nodes with value `val` as left and right children of each node.
+ - The original left child of the node becomes the left child of the newly inserted left node.
+ - Similarly, the original right child becomes the right child of the newly inserted right node.
+
+### Steps:
+1. If `depth == 1`, create a new root and attach the original tree as the left child of the new root.
+2. Otherwise, traverse the tree until the current depth is `depth - 1`.
+3. At each node, insert new nodes as described.
+
+Let's implement this solution in PHP: **[623. Add One Row to Tree](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/000623-add-one-row-to-tree/solution.php)**
+
+```php
+val = $val;
+ $this->left = $left;
+ $this->right = $right;
+ }
+}
+/**
+ * @param TreeNode $root
+ * @param Integer $val
+ * @param Integer $depth
+ * @return TreeNode
+ */
+function addOneRow($root, $val, $depth) {
+ ...
+ ...
+ ...
+ /**
+ * go to ./solution.php
+ */
+}
+
+// Helper function to print the tree in level order for visualization
+function printTree($root) {
+ $result = [];
+ $queue = [$root];
+ while (!empty($queue)) {
+ $node = array_shift($queue);
+ if ($node !== null) {
+ $result[] = $node->val;
+ $queue[] = $node->left;
+ $queue[] = $node->right;
+ } else {
+ $result[] = null;
+ }
+ }
+ // Remove trailing null values
+ while (end($result) === null) {
+ array_pop($result);
+ }
+ return $result;
+}
+
+// Test case 1
+$root = new TreeNode(4,
+ new TreeNode(2, new TreeNode(3), new TreeNode(1)),
+ new TreeNode(6, new TreeNode(5), null));
+$val = 1;
+$depth = 2;
+$new_tree = addOneRow($root, $val, $depth);
+print_r(printTree($new_tree)); // Output should be [4, 1, 1, 2, null, null, 6, 3, 1, 5]
+
+// Test case 2
+$root = new TreeNode(4,
+ new TreeNode(2, new TreeNode(3), new TreeNode(1)),
+ null);
+$val = 1;
+$depth = 3;
+$new_tree = addOneRow($root, $val, $depth);
+print_r(printTree($new_tree)); // Output should be [4, 2, null, 1, 1, 3, null, null, 1]
+?>
+```
+
+### Explanation:
+
+1. **Edge case (depth == 1)**: We create a new root node with the value `val` and set the original root as the left child of this new root. This is handled right at the start of the function.
+
+2. **BFS traversal**: We traverse the tree level by level using a queue until we reach nodes at depth `depth - 1`. These are the nodes where we will insert the new row.
+
+3. **Inserting new row**: For each node at `depth - 1`, we create two new nodes with value `val`. The original left child of the node becomes the left child of the new left node, and the original right child becomes the right child of the new right node.
+
+### Time Complexity:
+- Traversing the tree requires \(O(n)\) where \(n\) is the number of nodes in the tree.
+- Since we perform BFS until depth `depth - 1` and update each node at that depth, the time complexity is linear in the number of nodes: \(O(n)\).
+
+### Test Cases:
+1. **Example 1**:
+ ```php
+ Input: root = [4,2,6,3,1,5], val = 1, depth = 2
+ Output: [4,1,1,2,null,null,6,3,1,5]
+ ```
+2. **Example 2**:
+ ```php
+ Input: root = [4,2,null,3,1], val = 1, depth = 3
+ Output: [4,2,null,1,1,3,null,null,1]
+ ```
+
+This solution ensures that the tree is modified correctly according to the problem's rules.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
diff --git a/algorithms/000623-add-one-row-to-tree/solution.php b/algorithms/000623-add-one-row-to-tree/solution.php
index a2455770e..f37923c1f 100644
--- a/algorithms/000623-add-one-row-to-tree/solution.php
+++ b/algorithms/000623-add-one-row-to-tree/solution.php
@@ -2,25 +2,18 @@
/**
* Definition for a binary tree node.
+ * class TreeNode {
+ * public $val = null;
+ * public $left = null;
+ * public $right = null;
+ * function __construct($val = 0, $left = null, $right = null) {
+ * $this->val = $val;
+ * $this->left = $left;
+ * $this->right = $right;
+ * }
+ * }
*/
-
-class TreeNode
-{
- public mixed $val = null;
- public mixed $left = null;
- public mixed $right = null;
-
- function __construct($val = 0, $left = null, $right = null)
- {
- $this->val = $val;
- $this->left = $left;
- $this->right = $right;
- }
-}
-
-
-class Solution
-{
+class Solution {
/**
* @param TreeNode $root
@@ -28,41 +21,43 @@ class Solution
* @param Integer $depth
* @return TreeNode
*/
- function addOneRow(TreeNode $root, int $val, int $depth): TreeNode
- {
- return $this->dfs($root, $val, $depth, 1, false);
- }
-
- function dfs($root, $val, $depth, $curr, $isLeft)
- {
- if ($root == null) {
- if ($depth == 1) {
- $root = new TreeNode($val);
- }
- return $root;
+ function addOneRow($root, $val, $depth) {
+ if ($depth == 1) {
+ // Create a new root with value $val, and set the original tree as its left child
+ return new TreeNode($val, $root, null);
}
- if ($depth == 1 && $root != null) {
- $newNode = new TreeNode($val);
- $newNode->left = $root;
- $root = $newNode;
- return $newNode;
+ // Perform BFS to find nodes at depth - 1
+ $queue = [$root];
+ $current_depth = 1;
+
+ // Traverse until we reach depth - 1
+ while (!empty($queue) && $current_depth < $depth - 1) {
+ $size = count($queue);
+ for ($i = 0; $i < $size; $i++) {
+ $node = array_shift($queue);
+ if ($node->left !== null) {
+ $queue[] = $node->left;
+ }
+ if ($node->right !== null) {
+ $queue[] = $node->right;
+ }
+ }
+ $current_depth++;
}
- if ($curr + 1 == $depth) {
- $newNode = new TreeNode($val);
- $newNode->left = $root->left;
- $root->left = $newNode;
+ // At this point, we are at depth - 1. Add the new row.
+ foreach ($queue as $node) {
+ // Save the original left and right children
+ $old_left = $node->left;
+ $old_right = $node->right;
- $newNode1 = new TreeNode($val);
- $newNode1->right = $root->right;
- $root->right = $newNode1;
-
- return $root;
+ // Insert new nodes with value $val
+ $node->left = new TreeNode($val, $old_left, null);
+ $node->right = new TreeNode($val, null, $old_right);
}
- $this->dfs($root->left, $val, $depth, $curr + 1, true);
- $this->dfs($root->right, $val, $depth, $curr + 1, false);
return $root;
+
}
}
\ No newline at end of file
diff --git a/algorithms/000624-maximum-distance-in-arrays/README.md b/algorithms/000624-maximum-distance-in-arrays/README.md
new file mode 100644
index 000000000..005e389ae
--- /dev/null
+++ b/algorithms/000624-maximum-distance-in-arrays/README.md
@@ -0,0 +1,75 @@
+624\. Maximum Distance in Arrays
+
+**Difficulty:** Medium
+
+**Topics:** `Array`, `Greedy`
+
+You are given `m` `arrays`, where each array is sorted in **ascending order**.
+
+You can pick up two integers from two different arrays (each array picks one) and calculate the distance. We define the distance between two integers `a` and `b` to be their absolute difference `|a - b|`.
+
+Return _the maximum distance_.
+
+**Example 1:**
+
+- **Input:** arrays = [[1,2,3],[4,5],[1,2,3]]
+- **Output:** 4
+- **Explanation:** One way to reach the maximum distance 4 is to pick 1 in the first or third array and pick 5 in the second array.
+
+**Example 2:**
+
+- **Input:** arrays = [[1],[1]]
+- **Output:** 0
+
+**Constraints:**
+
+- m == arrays.length
+- 2 <= m <= 105
+- 1 <= arrays[i].length <= 500
+- -104 <= arrays[i][j] <= 104
+- `arrays[i]` is sorted in **ascending order**.
+- There will be at most 105
integers in all the arrays.
+
+
+**Solution:**
+
+We need to calculate the maximum possible distance between two integers, each picked from different arrays. The key observation is that the maximum distance will most likely be between the minimum value of one array and the maximum value of another array.
+
+To solve this problem, we can follow these steps:
+
+1. Track the minimum value and maximum value as you iterate through the arrays.
+2. For each array, compute the potential maximum distance by comparing the current array's minimum with the global maximum and the current array's maximum with the global minimum.
+3. Update the global minimum and maximum as you proceed.
+
+Let's implement this solution in PHP: **[624. Maximum Distance in Arrays](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/000624-maximum-distance-in-arrays/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+- **min_value** and **max_value** are initialized with the first arrayβs minimum and maximum values.
+- As we iterate through each array starting from the second one:
+ - We calculate the distance by comparing the global minimum with the current arrayβs maximum and the global maximum with the current arrayβs minimum.
+ - Update the `max_distance` whenever a larger distance is found.
+ - Update `min_value` and `max_value` to reflect the minimum and maximum values encountered so far.
+- Finally, the function returns the maximum distance found.
+
+This solution runs in O(m) time, where m is the number of arrays, making it efficient given the problem constraints.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
diff --git a/algorithms/000624-maximum-distance-in-arrays/solution.php b/algorithms/000624-maximum-distance-in-arrays/solution.php
new file mode 100644
index 000000000..201a26452
--- /dev/null
+++ b/algorithms/000624-maximum-distance-in-arrays/solution.php
@@ -0,0 +1,31 @@
+-105 <= nums[i][j] <= 105
+- `nums[i]` is sorted in **non-decreasing** order.
+
+
+**Solution:**
+
+We can use a **min-heap** (or priority queue) to track the smallest element from each list while maintaining a sliding window to find the smallest range that includes at least one element from each list.
+
+### Approach
+
+1. **Min-Heap Initialization**: Use a min-heap to store the current elements from each of the `k` lists. Each heap entry will be a tuple containing the value, the index of the list it comes from, and the index of the element in that list.
+2. **Max Value Tracking**: Keep track of the maximum value in the current window. This is important because the range is determined by the difference between the smallest element (from the heap) and the current maximum.
+3. **Iterate Until End of Lists**: For each iteration:
+ - Extract the minimum element from the heap.
+ - Update the range if the current range `[min_value, max_value]` is smaller than the previously recorded smallest range.
+ - Move to the next element in the list from which the minimum element was taken. Update the max value and add the new element to the heap.
+4. **Termination**: The process ends when any list is exhausted.
+
+Let's implement this solution in PHP: **[632. Smallest Range Covering Elements from K Lists](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/000632-smallest-range-covering-elements-from-k-lists/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+1. **Heap Initialization**:
+ - The initial heap contains the first element from each list. We also keep track of the maximum element among the first elements.
+2. **Processing the Heap**:
+ - Extract the minimum element from the heap, and then try to extend the range by adding the next element from the same list (if available).
+ - After adding a new element to the heap, update the `maxValue` if the new element is larger.
+ - Update the smallest range whenever the difference between the `maxValue` and `minValue` is smaller than the previously recorded range.
+3. **Termination**:
+ - The loop stops when any list runs out of elements, as we cannot include all lists in the range anymore.
+
+### Complexity Analysis
+- **Time Complexity**: `O(n * log k)`, where `n` is the total number of elements across all lists, and `k` is the number of lists. The complexity comes from inserting and removing elements from the heap.
+- **Space Complexity**: `O(k)` for storing elements in the heap.
+
+This solution efficiently finds the smallest range that includes at least one number from each of the `k` sorted lists.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
\ No newline at end of file
diff --git a/algorithms/000632-smallest-range-covering-elements-from-k-lists/solution.php b/algorithms/000632-smallest-range-covering-elements-from-k-lists/solution.php
new file mode 100644
index 000000000..a5cb008b2
--- /dev/null
+++ b/algorithms/000632-smallest-range-covering-elements-from-k-lists/solution.php
@@ -0,0 +1,47 @@
+ $list) {
+ $minHeap->insert([$list[0], $rowIndex, 0]);
+ $maxValue = max($maxValue, $list[0]);
+ }
+
+ // Initialize the range with the max possible values
+ $rangeStart = PHP_INT_MIN;
+ $rangeEnd = PHP_INT_MAX;
+
+ // Process the heap until we can no longer include all lists in the window
+ while (!$minHeap->isEmpty()) {
+ list($minValue, $rowIndex, $colIndex) = $minHeap->extract();
+
+ // Update the smallest range
+ if ($maxValue - $minValue < $rangeEnd - $rangeStart) {
+ $rangeStart = $minValue;
+ $rangeEnd = $maxValue;
+ }
+
+ // Move to the next element in the same list (if it exists)
+ if ($colIndex + 1 < count($nums[$rowIndex])) {
+ $nextValue = $nums[$rowIndex][$colIndex + 1];
+ $minHeap->insert([$nextValue, $rowIndex, $colIndex + 1]);
+ $maxValue = max($maxValue, $nextValue);
+ } else {
+ // If any list is exhausted, break the loop
+ break;
+ }
+ }
+
+ return [$rangeStart, $rangeEnd];
+ }
+}
\ No newline at end of file
diff --git a/algorithms/000633-sum-of-square-numbers/README.md b/algorithms/000633-sum-of-square-numbers/README.md
index 3f44eb9a2..af2f20ec9 100644
--- a/algorithms/000633-sum-of-square-numbers/README.md
+++ b/algorithms/000633-sum-of-square-numbers/README.md
@@ -1,6 +1,8 @@
633\. Sum of Square Numbers
-Medium
+**Difficulty:** Medium
+
+**Topics:** `Math`, `Two Pointers`, `Binary Search`
Given a non-negative integer `c`, decide whether there're two integers `a` and `b` such that `a2 + b2 = c`.
@@ -19,3 +21,83 @@ Given a non-negative integer `c`, decide whether there're two integers `a` and `
- 0 <= c <= 231 - 1
+
+
+**Solution:**
+
+We can utilize a two-pointer approach. Here's how we can approach the problem:
+
+### Explanation:
+
+1. **Constraints**:
+ - We're given a non-negative integer `c`.
+ - We need to find two integers `a` and `b` such that `aΒ² + bΒ² = c`.
+
+2. **Two-pointer Approach**:
+ - Start with two pointers: `a` initialized to 0, and `b` initialized to the square root of `c`.
+ - The idea is to check the sum of the squares of `a` and `b`. If `aΒ² + bΒ²` equals `c`, return `true`.
+ - If `aΒ² + bΒ²` is less than `c`, increment `a` to increase the sum.
+ - If `aΒ² + bΒ²` is greater than `c`, decrement `b` to decrease the sum.
+ - Continue this process until `a` is less than or equal to `b`.
+ - If no such pair is found, return `false`.
+
+Let's implement this solution in PHP: **[633. Sum of Square Numbers](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/000633-sum-of-square-numbers/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+1. **Initialization**:
+ - `$a = 0`: The left pointer starts from 0.
+ - `$b = (int) sqrt($c)`: The right pointer starts from the integer value of the square root of `c`, as `bΒ²` cannot exceed `c`.
+
+2. **Loop**:
+ - The loop continues as long as `a <= b`.
+ - If `aΒ² + bΒ²` equals `c`, the function returns `true`.
+ - If `aΒ² + bΒ²` is less than `c`, it means we need a larger sum, so we increment `a`.
+ - If `aΒ² + bΒ²` is greater than `c`, we need a smaller sum, so we decrement `b`.
+
+3. **End Condition**:
+ - If no such integers `a` and `b` are found, return `false`.
+
+### Time Complexity:
+
+- The time complexity is `O(sqrt(c))` because we iterate through the integers from `0` to `sqrt(c)`.
+
+### Example Outputs:
+
+- For `c = 5`, the function will return `true` because `1Β² + 2Β² = 5`.
+- For `c = 3`, the function will return `false` because no integers satisfy the equation `aΒ² + bΒ² = 3`.
+
+This approach efficiently checks for two integers whose squares sum up to `c` using a two-pointer technique.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
diff --git a/algorithms/000633-sum-of-square-numbers/solution.php b/algorithms/000633-sum-of-square-numbers/solution.php
index 2d78c4166..797d0eed5 100644
--- a/algorithms/000633-sum-of-square-numbers/solution.php
+++ b/algorithms/000633-sum-of-square-numbers/solution.php
@@ -7,17 +7,23 @@ class Solution {
* @return Boolean
*/
function judgeSquareSum($c) {
- for ($i = 2; $i * $i <= $c; $i++) {
- $count = 0;
- if ($c % $i == 0) {
- while ($c % $i == 0) {
- $count++;
- $c /= $i;
- }
- if ($i % 4 == 3 && $count % 2 != 0)
- return false;
+ // Initialize two pointers
+ $a = 0;
+ $b = (int) sqrt($c);
+
+ // Iterate while a <= b
+ while ($a <= $b) {
+ $sumOfSquares = $a * $a + $b * $b;
+
+ if ($sumOfSquares == $c) {
+ return true; // Found a pair (a, b)
+ } elseif ($sumOfSquares < $c) {
+ $a++; // Increment a to increase sum
+ } else {
+ $b--; // Decrement b to decrease sum
}
}
- return $c % 4 != 3;
+
+ return false; // No such pair exists
}
-}
+}
\ No newline at end of file
diff --git a/algorithms/000641-design-circular-deque/README.md b/algorithms/000641-design-circular-deque/README.md
new file mode 100644
index 000000000..9d66809f3
--- /dev/null
+++ b/algorithms/000641-design-circular-deque/README.md
@@ -0,0 +1,292 @@
+641\. Design Circular Deque
+
+**Difficulty:** Medium
+
+**Topics:** `Array`, `Linked List`, `Design`, `Queue`
+
+Design your implementation of the circular double-ended queue (deque).
+
+Implement the `MyCircularDeque` class:
+
+- `MyCircularDeque(int k)` Initializes the deque with a maximum size of `k`.
+- `boolean insertFront()` Adds an item at the front of Deque. Returns `true` if the operation is successful, or `false` otherwise.
+- `boolean insertLast()` Adds an item at the rear of Deque. Returns `true` if the operation is successful, or `false` otherwise.
+- `boolean deleteFront()` Deletes an item from the front of Deque. Returns `true` if the operation is successful, or `false` otherwise.
+- `boolean deleteLast()` Deletes an item from the rear of Deque. Returns `true` if the operation is successful, or `false` otherwise.
+- `int getFront()` Returns the front item from the Deque. Returns `-1` if the deque is empty.
+- `int getRear()` Returns the last item from Deque. Returns `-1` if the deque is empty.
+- `boolean isEmpty()` Returns `true` if the deque is empty, or `false` otherwise.
+- `boolean isFull()` Returns `true` if the deque is full, or `false` otherwise.
+
+**Example 1:**
+
+- **Input:**\
+ ["MyCircularDeque", "insertLast", "insertLast", "insertFront", "insertFront", "getRear", "isFull", "deleteLast", "insertFront", "getFront"]\
+ [[3], [1], [2], [3], [4], [], [], [], [4], []]
+- **Output:** [null, true, true, true, false, 2, true, true, true, 4]
+- **Explanation:**\
+ MyCircularDeque myCircularDeque = new MyCircularDeque(3);\
+ myCircularDeque.insertLast(1); // return True\
+ myCircularDeque.insertLast(2); // return True\
+ myCircularDeque.insertFront(3); // return True\
+ myCircularDeque.insertFront(4); // return False, the queue is full.\
+ myCircularDeque.getRear(); // return 2\
+ myCircularDeque.isFull(); // return True\
+ myCircularDeque.deleteLast(); // return True\
+ myCircularDeque.insertFront(4); // return True\
+ myCircularDeque.getFront(); // return 4
+
+
+**Constraints:**
+
+- `1 <= k <= 1000`
+- `0 <= value <= 1000`
+- At most `2000` calls will be made to `insertFront`, `insertLast`, `deleteFront`, `deleteLast`, `getFront`, `getRear`, `isEmpty`, `isFull`.
+
+
+**Solution:**
+
+We can use an array to represent the deque structure. We'll maintain a `head` and `tail` pointer that will help us track the front and rear of the deque, wrapping around when necessary to achieve the circular behavior.
+
+Let's implement this solution in PHP: **[641. Design Circular Deque](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/000641-design-circular-deque/solution.php)**
+
+Here's a step-by-step solution for the `MyCircularDeque` class:
+
+```php
+insertFront($value);
+ * $ret_2 = $obj->insertLast($value);
+ * $ret_3 = $obj->deleteFront();
+ * $ret_4 = $obj->deleteLast();
+ * $ret_5 = $obj->getFront();
+ * $ret_6 = $obj->getRear();
+ * $ret_7 = $obj->isEmpty();
+ * $ret_8 = $obj->isFull();
+ */
+
+?>
+```
+
+### Explanation:
+
+1. **Initialization**:
+ - We initialize the deque using an array of size `k` and set all values to `-1` initially.
+ - The `front` and `rear` pointers are initialized to `0`.
+ - `size` keeps track of the current number of elements in the deque.
+
+2. **insertFront($value)**:
+ - Check if the deque is full using `isFull()`.
+ - If not, decrement the `front` pointer (with circular wrap-around using modulo).
+ - Place the value at the new `front` and increment the `size`.
+
+3. **insertLast($value)**:
+ - Check if the deque is full.
+ - If not, place the value at the `rear` and move the `rear` pointer forward (again using modulo for wrap-around).
+ - Increment the `size`.
+
+4. **deleteFront()**:
+ - Check if the deque is empty using `isEmpty()`.
+ - If not, increment the `front` pointer (circular wrap-around) and decrement the `size`.
+
+5. **deleteLast()**:
+ - Check if the deque is empty.
+ - If not, decrement the `rear` pointer and reduce the `size`.
+
+6. **getFront()**:
+ - If the deque is empty, return `-1`.
+ - Otherwise, return the element at the `front` pointer.
+
+7. **getRear()**:
+ - If the deque is empty, return `-1`.
+ - Otherwise, return the element before the current `rear` pointer (since `rear` points to the next available position).
+
+8. **isEmpty()**:
+ - Returns `true` if the deque is empty (`size` is `0`).
+
+9. **isFull()**:
+ - Returns `true` if the deque is full (`size` equals `maxSize`).
+
+### Example Walkthrough
+
+```php
+$myCircularDeque = new MyCircularDeque(3); // Initialize deque with size 3
+
+$myCircularDeque->insertLast(1); // return true
+$myCircularDeque->insertLast(2); // return true
+$myCircularDeque->insertFront(3); // return true
+$myCircularDeque->insertFront(4); // return false, deque is full
+echo $myCircularDeque->getRear(); // return 2
+echo $myCircularDeque->isFull(); // return true
+$myCircularDeque->deleteLast(); // return true
+$myCircularDeque->insertFront(4); // return true
+echo $myCircularDeque->getFront(); // return 4
+```
+
+### Time Complexity:
+- Each operation (`insertFront`, `insertLast`, `deleteFront`, `deleteLast`, `getFront`, `getRear`, `isEmpty`, `isFull`) runs in **O(1)** time since they all involve constant-time array operations and pointer manipulations.
+
+### Space Complexity:
+- The space complexity is **O(k)**, where `k` is the size of the deque. We allocate space for `k` elements in the deque array.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
\ No newline at end of file
diff --git a/algorithms/000641-design-circular-deque/solution.php b/algorithms/000641-design-circular-deque/solution.php
new file mode 100644
index 000000000..728f3665a
--- /dev/null
+++ b/algorithms/000641-design-circular-deque/solution.php
@@ -0,0 +1,156 @@
+deque = array_fill(0, $k, -1);
+ $this->maxSize = $k;
+ $this->front = 0;
+ $this->rear = 0;
+ $this->size = 0;
+ }
+
+ /**
+ * Adds an item at the front of Deque. Return true if the operation is successful.
+ *
+ * @param Integer $value
+ * @return Boolean
+ */
+ function insertFront($value) {
+ if ($this->isFull()) {
+ return false;
+ }
+ // Move the front pointer backward
+ $this->front = ($this->front - 1 + $this->maxSize) % $this->maxSize;
+ $this->deque[$this->front] = $value;
+ $this->size++;
+ return true;
+ }
+
+ /**
+ * Adds an item at the rear of Deque. Return true if the operation is successful.
+ *
+ * @param Integer $value
+ * @return Boolean
+ */
+ function insertLast($value) {
+ if ($this->isFull()) {
+ return false;
+ }
+ $this->deque[$this->rear] = $value;
+ $this->rear = ($this->rear + 1) % $this->maxSize;
+ $this->size++;
+ return true;
+ }
+
+ /**
+ * Deletes an item from the front of Deque. Return true if the operation is successful.
+ *
+ * @return Boolean
+ */
+ function deleteFront() {
+ if ($this->isEmpty()) {
+ return false;
+ }
+ // Move the front pointer forward
+ $this->front = ($this->front + 1) % $this->maxSize;
+ $this->size--;
+ return true;
+ }
+
+ /**
+ * Deletes an item from the rear of Deque. Return true if the operation is successful.
+ *
+ * @return Boolean
+ */
+ function deleteLast() {
+ if ($this->isEmpty()) {
+ return false;
+ }
+ // Move the rear pointer backward
+ $this->rear = ($this->rear - 1 + $this->maxSize) % $this->maxSize;
+ $this->size--;
+ return true;
+ }
+
+ /**
+ * Get the front item from the deque. If the deque is empty, return -1.
+ *
+ * @return Integer
+ */
+ function getFront() {
+ if ($this->isEmpty()) {
+ return -1;
+ }
+ return $this->deque[$this->front];
+ }
+
+ /**
+ * Get the last item from the deque. If the deque is empty, return -1.
+ *
+ * @return Integer
+ */
+ function getRear() {
+ if ($this->isEmpty()) {
+ return -1;
+ }
+ // Get the element before the current rear position
+ return $this->deque[($this->rear - 1 + $this->maxSize) % $this->maxSize];
+ }
+
+ /**
+ * Checks whether the deque is empty or not.
+ *
+ * @return Boolean
+ */
+ function isEmpty() {
+ return $this->size == 0;
+ }
+
+ /**
+ * Checks whether the deque is full or not.
+ *
+ * @return Boolean
+ */
+ function isFull() {
+ return $this->size == $this->maxSize;
+ }
+}
+
+/**
+ * Your MyCircularDeque object will be instantiated and called as such:
+ * $obj = MyCircularDeque($k);
+ * $ret_1 = $obj->insertFront($value);
+ * $ret_2 = $obj->insertLast($value);
+ * $ret_3 = $obj->deleteFront();
+ * $ret_4 = $obj->deleteLast();
+ * $ret_5 = $obj->getFront();
+ * $ret_6 = $obj->getRear();
+ * $ret_7 = $obj->isEmpty();
+ * $ret_8 = $obj->isFull();
+ */
\ No newline at end of file
diff --git a/algorithms/000648-replace-words/README.md b/algorithms/000648-replace-words/README.md
index 53f2452d4..2f82ca881 100644
--- a/algorithms/000648-replace-words/README.md
+++ b/algorithms/000648-replace-words/README.md
@@ -1,6 +1,8 @@
648\. Replace Words
-Medium
+**Difficulty:** Medium
+
+**Topics:** `Array`, `Hash Table`, `String`, `Trie`
In English, we have a concept called root, which can be followed by some other word to form another longer word - let's call this word **derivative**. For example, when the root `"help"` is followed by the word `"ful"`, we can form a derivative `"helpful"`.
@@ -28,4 +30,176 @@ Return _the `sentence` after the replacement_.
- The number of words in `sentence` is in the range `[1, 1000]`
- The length of each word in `sentence` is in the range `[1, 1000]`
- Every two consecutive words in `sentence` will be separated by exactly one space.
-- `sentence` does not have leading or trailing spaces.
\ No newline at end of file
+- `sentence` does not have leading or trailing spaces.
+
+
+
+**Solution:**
+
+We can approach this using a **Trie** (prefix tree) to store the dictionary of roots. Then, we process each word in the sentence, replacing it with the shortest root if it exists in the Trie.
+
+### Approach:
+1. **Trie Structure**:
+ - We'll build a Trie (prefix tree) from the dictionary of roots. This allows us to efficiently find the shortest root for each word in the sentence.
+
+2. **Processing the Sentence**:
+ - For each word in the sentence, we'll search the Trie to see if there's a root that matches the start of the word. Once we find the first matching root, we replace the word with that root.
+
+3. **Efficiency**:
+ - By using a Trie, searching for the root for each word will be faster than directly comparing the word with each root in the dictionary.
+
+Let's implement this solution in PHP: **[648. Replace Words](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/000648-replace-words/solution.php)**
+
+```php
+root = new TrieNode();
+ }
+
+ /**
+ * Insert a word (root) into the Trie
+ *
+ * @param $word
+ * @return void
+ */
+ public function insert($word) {
+ ...
+ ...
+ ...
+ /**
+ * go to ./solution.php
+ */
+ }
+
+ /**
+ * Find the shortest root for a given word
+ *
+ * @param $word
+ * @return mixed|string
+ */
+ public function findRoot($word) {
+ ...
+ ...
+ ...
+ /**
+ * go to ./solution.php
+ */
+ }
+}
+
+/**
+ * Function to replace words in the sentence
+ *
+ * @param String[] $dictionary
+ * @param String $sentence
+ * @return String
+ */
+function replaceWords($dictionary, $sentence) {
+ ...
+ ...
+ ...
+ /**
+ * go to ./solution.php
+ */
+}
+
+// Example usage:
+
+// Example 1:
+$dictionary = ["cat", "bat", "rat"];
+$sentence = "the cattle was rattled by the battery";
+$result = replaceWords($dictionary, $sentence);
+echo $result . "\n"; // Output: "the cat was rat by the bat"
+
+// Example 2:
+$dictionary2 = ["a", "b", "c"];
+$sentence2 = "aadsfasf absbs bbab cadsfafs";
+$result2 = replaceWords($dictionary2, $sentence2);
+echo $result2 . "\n"; // Output: "a a b c"
+?>
+```
+
+### Explanation:
+
+1. **TrieNode Class**:
+ - This class represents each node of the Trie. It contains:
+ - `$children`: an associative array where each key is a character of a root.
+ - `$isEndOfWord`: a boolean flag indicating if this node marks the end of a root in the Trie.
+
+2. **Trie Class**:
+ - `insert($word)`: Inserts a root word into the Trie character by character.
+ - `findRoot($word)`: Searches for the shortest root for a given word. If found, it returns the root, otherwise, it returns the word itself.
+
+3. **replaceWords Function**:
+ - Builds the Trie using the dictionary of roots.
+ - Processes each word in the sentence, checking for a replacement root using the `findRoot` method.
+ - Reconstructs the modified sentence by joining the processed words.
+
+### Example 1:
+
+**Input**:
+```php
+$dictionary = ["cat", "bat", "rat"];
+$sentence = "the cattle was rattled by the battery";
+```
+
+**Process**:
+- `cattle` is replaced by `cat` (root: "cat").
+- `rattled` is replaced by `rat` (root: "rat").
+- `battery` is replaced by `bat` (root: "bat").
+
+**Output**:
+```
+"the cat was rat by the bat"
+```
+
+### Example 2:
+
+**Input**:
+```php
+$dictionary = ["a", "b", "c"];
+$sentence = "aadsfasf absbs bbab cadsfafs";
+```
+
+**Output**:
+```
+"a a b c"
+```
+
+### Time Complexity:
+- **Building the Trie**: Inserting all roots takes \(O(N \cdot L)\), where \(N\) is the number of roots, and \(L\) is the average length of the roots.
+- **Processing the sentence**: For each word in the sentence, finding the root in the Trie takes \(O(L)\), where \(L\) is the length of the word. So, for \(M\) words in the sentence, the total time complexity is \(O(M \cdot L)\).
+
+Thus, the overall time complexity is \(O(N \cdot L + M \cdot L)\), which is efficient given the constraints.
+
+### Space Complexity:
+The space complexity is \(O(N \cdot L)\) for storing the Trie.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
+
\ No newline at end of file
diff --git a/algorithms/000648-replace-words/solution.php b/algorithms/000648-replace-words/solution.php
index 5db5b1dc8..43161217f 100644
--- a/algorithms/000648-replace-words/solution.php
+++ b/algorithms/000648-replace-words/solution.php
@@ -1,69 +1,94 @@
root = new TrieNode();
- }
-
/**
* @param String[] $dictionary
* @param String $sentence
* @return String
*/
function replaceWords($dictionary, $sentence) {
- foreach ($dictionary as $word) {
- $this->insert($word);
+ // Step 1: Build the Trie with the dictionary of roots
+ $trie = new Trie();
+ foreach ($dictionary as $root) {
+ $trie->insert($root);
}
- $ans = '';
- $iss = explode(' ', $sentence);
+ // Step 2: Split the sentence into words
+ $words = explode(" ", $sentence);
- foreach ($iss as $s) {
- $ans .= $this->search($s) . ' ';
+ // Step 3: For each word, find the root and replace the word if needed
+ for ($i = 0; $i < count($words); $i++) {
+ $words[$i] = $trie->findRoot($words[$i]);
}
- $ans = rtrim($ans);
- return $ans;
+ // Step 4: Join the words back into a sentence
+ return implode(" ", $words);
+
}
+}
- private function insert($word) {
+// Define a Trie Node class
+class TrieNode {
+ /**
+ * @var array
+ */
+ public $children = [];
+ /**
+ * @var bool
+ */
+ public $isEndOfWord = false; // Marks the end of a root word
+}
+
+// Define a Trie class for storing the dictionary of roots
+class Trie {
+ /**
+ * @var TrieNode
+ */
+ private $root;
+
+ public function __construct() {
+ $this->root = new TrieNode();
+ }
+
+ /**
+ * Insert a word (root) into the Trie
+ *
+ * @param $word
+ * @return void
+ */
+ public function insert($word) {
$node = $this->root;
for ($i = 0; $i < strlen($word); $i++) {
- $c = $word[$i];
- $index = ord($c) - ord('a');
- if ($node->children[$index] == null) {
- $node->children[$index] = new TrieNode();
+ $char = $word[$i];
+ if (!isset($node->children[$char])) {
+ $node->children[$char] = new TrieNode();
}
- $node = $node->children[$index];
+ $node = $node->children[$char];
}
- $node->word = $word;
+ $node->isEndOfWord = true; // Mark the end of the root word
}
- private function search($word) {
+ /**
+ * Find the shortest root for a given word
+ *
+ * @param $word
+ * @return mixed|string
+ */
+ public function findRoot($word) {
$node = $this->root;
+ $prefix = "";
+
for ($i = 0; $i < strlen($word); $i++) {
- $c = $word[$i];
- if ($node->word != null) {
- return $node->word;
+ $char = $word[$i];
+ if (!isset($node->children[$char])) {
+ break;
}
- $index = ord($c) - ord('a');
- if ($node->children[$index] == null) {
- return $word;
+ $prefix .= $char;
+ $node = $node->children[$char];
+ if ($node->isEndOfWord) {
+ return $prefix; // Return the root if found
}
- $node = $node->children[$index];
}
- return $word;
+ return $word; // Return the original word if no root is found
}
}
-
-class TrieNode {
- public $children;
- public $word;
-
- public function __construct() {
- $this->children = array_fill(0, 26, null);
- $this->word = null;
- }
-}
\ No newline at end of file
diff --git a/algorithms/000650-2-keys-keyboard/README.md b/algorithms/000650-2-keys-keyboard/README.md
new file mode 100644
index 000000000..ab2e6f37d
--- /dev/null
+++ b/algorithms/000650-2-keys-keyboard/README.md
@@ -0,0 +1,95 @@
+650\. 2 Keys Keyboard
+
+**Difficulty:** Medium
+
+**Topics:** `Math`, `Dynamic Programming`
+
+There is only one character `'A'` on the screen of a notepad. You can perform one of two operations on this notepad for each step:
+
+- Copy All: You can copy all the characters present on the screen (a partial copy is not allowed).
+- Paste: You can paste the characters which are copied last time.
+
+Given an integer `n`, return _the minimum number of operations to get the character `'A'` exactly `n` times on the screen_.
+
+
+
+**Example 1:**
+
+- **Input:** n = 3
+- **Output:** 3
+- **Explanation:** Initially, we have one character 'A'.\
+ In step 1, we use Copy All operation.\
+ In step 2, we use Paste operation to get 'AA'.\
+ In step 3, we use Paste operation to get 'AAA'.
+
+**Example 2:**
+
+- **Input:** n = 1
+- **Output:** 0
+
+**Example 3:**
+
+- **Input:** n = 10
+- **Output:** 7
+
+**Example 2:**
+
+- **Input:** n = 24
+- **Output:** 9
+
+**Constraints:**
+
+- 1 <= n <= 1000
+
+**Hint:**
+1. How many characters may be there in the clipboard at the last step if n = 3? n = 7? n = 10? n = 24?
+
+
+**Solution:**
+
+
+We need to find the minimum number of operations to get exactly `n` characters `'A'` on the screen. We'll use a dynamic programming approach to achieve this.
+
+1. **Understanding the Problem:**
+ - We start with one `'A'` on the screen.
+ - We can either "Copy All" (which copies the current screen content) or "Paste" (which pastes the last copied content).
+ - We need to determine the minimum operations required to have exactly `n` characters `'A'` on the screen.
+
+2. **Dynamic Programming Approach:**
+ - Use a dynamic programming (DP) array `dp` where `dp[i]` represents the minimum number of operations required to get exactly `i` characters on the screen.
+ - Initialize `dp[1] = 0` since it takes 0 operations to have one `'A'` on the screen.
+ - For each number of characters `i` from 2 to `n`, calculate the minimum operations by checking every divisor of `i`. If `i` is divisible by `d`, then:
+ - The number of operations needed to reach `i` is the sum of the operations to reach `d` plus the operations required to multiply `d` to get `i`.
+
+3. **Steps to Solve:**
+ - Initialize a DP array with `INF` (or a large number) for all values except `dp[1]`.
+ - For each `i` from 2 to `n`, iterate through possible divisors of `i` and update `dp[i]` based on the operations needed to reach `i` by copying and pasting.
+
+Let's implement this solution in PHP: **[650. 2 Keys Keyboard](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/000650-2-keys-keyboard/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+- **Initialization:** `dp` is initialized with a large number (`PHP_INT_MAX`) to represent an initially unreachable state.
+- **Divisor Check:** For each number `i`, check all divisors `d`. Update `dp[i]` by considering the operations required to reach `d` and then multiplying to get `i`.
+- **Output:** The result is the value of `dp[n]`, which gives the minimum operations required to get exactly `n` characters on the screen.
+
+This approach ensures we compute the minimum operations efficiently for the given constraints.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
diff --git a/algorithms/000650-2-keys-keyboard/solution.php b/algorithms/000650-2-keys-keyboard/solution.php
new file mode 100644
index 000000000..ccb832757
--- /dev/null
+++ b/algorithms/000650-2-keys-keyboard/solution.php
@@ -0,0 +1,35 @@
+1 <= s.length <= 100
+- `s` consists of lowercase English letters.
+
+
+**Solution:**
+
+We can use dynamic programming. The idea is to minimize the number of turns required to print the string by breaking it down into subproblems.
+
+The problem can be solved using dynamic programming. The idea is to divide the problem into smaller subproblems where you determine the minimum turns required to print every substring of `s`. We can leverage the following observation:
+
+- If two adjacent characters are the same, you can extend a previous operation instead of counting it as a new operation.
+
+### Dynamic Programming Solution
+
+Let `dp[i][j]` be the minimum number of turns required to print the substring `s[i:j+1]`.
+
+1. If `s[i] == s[j]`, then `dp[i][j] = dp[i][j-1]` because the last character `s[j]` can be printed with the previous operation.
+2. Otherwise, `dp[i][j] = min(dp[i][k] + dp[k+1][j])` for all `i <= k < j`.
+
+
+Let's implement this solution in PHP: **[664. Strange Printer](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/000664-strange-printer/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+1. **DP Array:** The 2D array `dp[i][j]` represents the minimum number of turns required to print the substring from index `i` to `j`.
+
+2. **Initialization:** We initialize `dp[i][i] = 1` because a single character can be printed in one turn.
+
+3. **Filling the DP Table:**
+ - If the characters at `i` and `j` are the same (`$s[$i] == $s[$j]`), then printing from `i` to `j` takes the same number of turns as printing from `i` to `j-1` since `$s[$j]` can be printed in the same turn as `$s[$i]`.
+ - If they are different, we try to find the minimum number of turns by dividing the string at different points (`k`).
+
+4. **Result:** The minimum number of turns required to print the entire string is stored in `dp[0][$n - 1]`.
+
+This solution efficiently calculates the minimum number of turns required to print the string by considering all possible ways to split and print the string.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
+
diff --git a/algorithms/000664-strange-printer/solution.php b/algorithms/000664-strange-printer/solution.php
new file mode 100644
index 000000000..3da2acdf6
--- /dev/null
+++ b/algorithms/000664-strange-printer/solution.php
@@ -0,0 +1,28 @@
+= 0; $i--) {
+ $dp[$i][$i] = 1; // Single character needs only one turn
+ for ($j = $i + 1; $j < $n; $j++) {
+ $dp[$i][$j] = $dp[$i][$j - 1] + 1;
+ for ($k = $i; $k < $j; $k++) {
+ if ($s[$k] == $s[$j]) {
+ $dp[$i][$j] = min($dp[$i][$j], $dp[$i][$k] + ($k + 1 <= $j - 1 ? $dp[$k + 1][$j - 1] : 0));
+ }
+ }
+ }
+ }
+
+ return $dp[0][$n - 1];
+
+ }
+}
\ No newline at end of file
diff --git a/algorithms/000670-maximum-swap/README.md b/algorithms/000670-maximum-swap/README.md
new file mode 100644
index 000000000..298661586
--- /dev/null
+++ b/algorithms/000670-maximum-swap/README.md
@@ -0,0 +1,85 @@
+670\. Maximum Swap
+
+**Difficulty:** Medium
+
+**Topics:** `Math`, `Greedy`
+
+You are given an integer `num`. You can swap two digits at most once to get the maximum valued number.
+
+Return _the maximum valued number you can get_.
+
+**Example 1:**
+
+- **Input:** num = 2736
+- **Output:** 7236
+- **Explanation:** Swap the number 2 and the number 7.
+
+**Example 2:**
+
+- **Input:** num = 9973
+- **Output:** 9973
+- **Explanation:** No swap.
+
+
+**Constraints:**
+
+- 0 <= num <= 108
+
+
+**Solution:**
+
+We can follow a greedy approach. Here's a step-by-step explanation and the solution:
+
+### Approach:
+
+1. **Convert the Number to an Array**: Since digits need to be swapped, converting the number into an array of digits makes it easier to access and manipulate individual digits.
+2. **Track the Rightmost Occurrence of Each Digit**: Store the rightmost position of each digit (0-9) in an array.
+3. **Find the Best Swap Opportunity**: Traverse through the number's digits from left to right, and for each digit, check if there is a higher digit that appears later. If so, swap them to maximize the number.
+4. **Perform the Swap and Break**: As soon as the optimal swap is found, perform the swap and break the loop.
+5. **Convert the Array Back to a Number**: After the swap, convert the array of digits back to a number and return it.
+
+Let's implement this solution in PHP: **[670. Maximum Swap](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/000670-maximum-swap/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+- **Step 1:** `strval($num)` converts the integer into a string, and `str_split($numStr)` splits it into an array of digits.
+- **Step 2:** The `last` array keeps track of the rightmost index of each digit from `0` to `9`.
+- **Step 3:** We iterate through each digit and look for a larger digit that can be swapped.
+- **Step 4:** If a suitable larger digit is found (that appears later in the number), the digits are swapped.
+- **Step 5:** The modified array is converted back to a string and then to an integer using `intval()`.
+
+### Complexity:
+- **Time Complexity:** O(n), where `n` is the number of digits in `num`. This is because we make a pass through the number to fill the `last` array and another pass to find the optimal swap.
+- **Space Complexity:** O(1) (ignoring the input size) since the `last` array is fixed with 10 elements.
+
+This solution efficiently finds the maximum value by swapping digits only once, as required.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
diff --git a/algorithms/000670-maximum-swap/solution.php b/algorithms/000670-maximum-swap/solution.php
new file mode 100644
index 000000000..448f2e015
--- /dev/null
+++ b/algorithms/000670-maximum-swap/solution.php
@@ -0,0 +1,42 @@
+ $numArr[$i]; $d--) {
+ if ($last[$d] > $i) {
+ // Swap digits
+ $temp = $numArr[$i];
+ $numArr[$i] = $numArr[$last[$d]];
+ $numArr[$last[$d]] = $temp;
+
+ // Convert back to an integer and return
+ return intval(implode('', $numArr));
+ }
+ }
+ }
+
+ // If no swap was made, return the original number
+ return $num;
+ }
+}
\ No newline at end of file
diff --git a/algorithms/000678-valid-parenthesis-string/README.md b/algorithms/000678-valid-parenthesis-string/README.md
index 451daac7a..ddc7eef3d 100644
--- a/algorithms/000678-valid-parenthesis-string/README.md
+++ b/algorithms/000678-valid-parenthesis-string/README.md
@@ -1,6 +1,8 @@
678\. Valid Parenthesis String
-Medium
+**Difficulty:** Medium
+
+**Topics:** `String`, `Dynamic Programming`, `Stack`, `Greedy`
Given a string `s` containing only three types of characters: `'('`, `')'` and `'*'`, return `true` if `s` is **valid**.
@@ -30,3 +32,81 @@ The following rules define a **valid** string:
* `1 <= s.length <= 100`
* `s[i]` is `'(', ')'` or `'*'`.
+
+
+**Hint:**
+1. Use backtracking to explore all possible combinations of treating '*' as either '(', ')', or an empty string. If any combination leads to a valid string, return true.
+2. DP[i][j] represents whether the substring s[i:j] is valid.
+3. Keep track of the count of open parentheses encountered so far. If you encounter a close parenthesis, it should balance with an open parenthesis. Utilize a stack to handle this effectively.
+4. How about using 2 stacks instead of 1? Think about it.
+
+
+
+**Solution:**
+
+We can use a greedy approach with two counters to track the possible minimum and maximum number of open parentheses `(` that could be valid at any point in the string.
+
+### Key Idea:
+- We maintain two counters: `minOpen` and `maxOpen`.
+ - `minOpen`: Tracks the minimum number of open parentheses that could still be valid.
+ - `maxOpen`: Tracks the maximum number of open parentheses that could still be valid.
+
+- As we iterate through the string:
+ - If the current character is `'('`, we increment both `minOpen` and `maxOpen` since we have one more possible open parenthesis.
+ - If the current character is `')'`, we decrement both `minOpen` and `maxOpen` since we close one open parenthesis.
+ - If the current character is `'*'`, we increment `maxOpen` (treat `*` as `'('`), and we decrement `minOpen` (treat `*` as `')'` or an empty string).
+
+- If at any point `maxOpen` becomes negative, we know it's impossible to balance the parentheses, so the string is invalid.
+- At the end, `minOpen` must be zero for the string to be valid. This ensures that all open parentheses have been closed.
+
+Let's implement this solution in PHP: **[678. Valid Parenthesis String](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/000678-valid-parenthesis-string/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+1. We initialize two counters, `minOpen` and `maxOpen`.
+2. As we traverse the string:
+ - For `'('`, both counters are incremented.
+ - For `')'`, both counters are decremented (with `minOpen` clamped to 0 to avoid negative values).
+ - For `'*'`, we adjust `minOpen` and `maxOpen` based on its flexibility (it can act as `(`, `)`, or `""`).
+3. If `maxOpen` becomes negative at any point, we return `false` because it's impossible to balance.
+4. Finally, we return `true` only if `minOpen` is 0, meaning all open parentheses are properly closed.
+
+### Time Complexity:
+- The time complexity is \(O(n)\), where \(n\) is the length of the string `s`, because we only traverse the string once.
+
+### Space Complexity:
+- The space complexity is \(O(1)\) because we use only two integer variables (`minOpen` and `maxOpen`), regardless of the size of the input.
+
+### Output:
+- For `s = "()"`, the output is `true`.
+- For `s = "(*)"`, the output is `true`.
+- For `s = "(*))"`, the output is `true`.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
\ No newline at end of file
diff --git a/algorithms/000678-valid-parenthesis-string/solution.php b/algorithms/000678-valid-parenthesis-string/solution.php
index a364448ac..635ff3566 100644
--- a/algorithms/000678-valid-parenthesis-string/solution.php
+++ b/algorithms/000678-valid-parenthesis-string/solution.php
@@ -1,22 +1,39 @@
1 <= nums.length <= 2 * 104
+- 1 <= nums[i] < 216
+- `1 <= k <= floor(nums.length / 3)`
+
+
+**Solution:**
+
+We will use a dynamic programming approach. The idea is to break down the problem into smaller subproblems, leveraging the overlap of subarrays to efficiently calculate the maximum sum of three non-overlapping subarrays of length `k`.
+
+### Approach:
+
+1. **Precompute the sums of subarrays of length `k`:**
+ First, we compute the sum of all subarrays of length `k` in the input array `nums`. This can be done efficiently in linear time by using a sliding window technique.
+
+2. **Dynamic Programming (DP):**
+ We create two auxiliary arrays, `left` and `right`, to store the indices of the best subarrays found up to the current position. The `left[i]` will store the index of the best subarray ending before index `i`, and `right[i]` will store the index of the best subarray starting after index `i`.
+
+3. **Iterate and Calculate the Maximum Sum:**
+ For each possible middle subarray starting at index `j`, we calculate the total sum by considering the best left subarray before `j` and the best right subarray after `j`.
+
+4. **Lexicographical Ordering:**
+ If there are multiple valid answers (with the same sum), we return the lexicographically smallest one. This is ensured by the order of iteration.
+
+Let's implement this solution in PHP: **[689. Maximum Sum of 3 Non-Overlapping Subarrays](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/000689-maximum-sum-of-3-non-overlapping-subarrays/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+1. **Subarray Sums Calculation:**
+ - We calculate the sum of all possible subarrays of length `k`. This is done by first calculating the sum of the first `k` elements. Then, for each subsequent position, we subtract the element that is left behind and add the next element in the array, making it an efficient sliding window approach.
+
+2. **Left and Right Arrays:**
+ - `left[i]` holds the index of the subarray with the maximum sum that ends before index `i`.
+ - `right[i]` holds the index of the subarray with the maximum sum that starts after index `i`.
+
+3. **Final Calculation:**
+ - For each middle subarray `j`, we check the combination of the best left subarray and the best right subarray, and update the result if the sum is greater than the current maximum.
+
+4. **Lexicographically Smallest Answer:**
+ - As we iterate from left to right, we ensure the lexicographically smallest solution by naturally choosing the first subarrays that yield the maximum sum.
+
+### Example:
+
+For the input:
+```php
+$nums = [1, 2, 1, 2, 6, 7, 5, 1];
+$k = 2;
+```
+
+The output will be:
+```php
+[0, 3, 5]
+```
+
+This approach ensures that the time complexity remains efficient, with a time complexity of approximately O(n), where `n` is the length of the input array `nums`.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
diff --git a/algorithms/000689-maximum-sum-of-3-non-overlapping-subarrays/solution.php b/algorithms/000689-maximum-sum-of-3-non-overlapping-subarrays/solution.php
new file mode 100644
index 000000000..ed4ff177c
--- /dev/null
+++ b/algorithms/000689-maximum-sum-of-3-non-overlapping-subarrays/solution.php
@@ -0,0 +1,59 @@
+ $sum[$leftMaxSum]) {
+ $leftMaxSum = $i;
+ }
+ $left[$i] = $leftMaxSum;
+ }
+
+ $rightMaxSum = $n - $k;
+ for ($i = $n - $k; $i >= 0; $i--) {
+ if ($sum[$i] >= $sum[$rightMaxSum]) {
+ $rightMaxSum = $i;
+ }
+ $right[$i] = $rightMaxSum;
+ }
+
+ // Step 3: Find the best middle subarray and combine with left and right
+ $maxSum = 0;
+ $result = [];
+ for ($j = $k; $j <= $n - 2 * $k; $j++) {
+ $i = $left[$j - $k];
+ $l = $right[$j + $k];
+ $totalSum = $sum[$i] + $sum[$j] + $sum[$l];
+ if ($totalSum > $maxSum) {
+ $maxSum = $totalSum;
+ $result = [$i, $j, $l];
+ }
+ }
+
+ return $result;
+ }
+}
\ No newline at end of file
diff --git a/algorithms/000703-kth-largest-element-in-a-stream/README.md b/algorithms/000703-kth-largest-element-in-a-stream/README.md
new file mode 100644
index 000000000..48fec4beb
--- /dev/null
+++ b/algorithms/000703-kth-largest-element-in-a-stream/README.md
@@ -0,0 +1,79 @@
+703\. Kth Largest Element in a Stream
+
+**Difficulty:** Easy
+
+**Topics:** `Tree`, `Design`, `Binary Search Tree`, `Heap (Priority Queue)`, `Binary Tree`, `Data Stream`
+
+Design a class to find the kth
largest element in a stream. Note that it is the kth
largest element in the sorted order, not the kth
distinct element.
+
+Implement `KthLargest` class:
+
+- `KthLargest(int k, int[] nums)` Initializes the object with the integer `k` and the stream of integers `nums`.
+- `int add(int val)` Appends the integer `val` to the stream and returns the element representing the kth
largest element in the stream.
+
+
+**Example 1:**
+
+- **Input:**\
+ ["KthLargest", "add", "add", "add", "add", "add"]\
+ [[3, [4, 5, 8, 2]], [3], [5], [10], [9], [4]]
+- **Output:** [null, 4, 5, 5, 8, 8]
+- **Explanation:**\
+ KthLargest kthLargest = new KthLargest(3, [4, 5, 8, 2]);\
+ kthLargest.add(3); // return 4\
+ kthLargest.add(5); // return 5\
+ kthLargest.add(10); // return 5\
+ kthLargest.add(9); // return 8\
+ kthLargest.add(4); // return 8
+
+**Example 2:**
+
+- **Input:**\
+ ["KthLargest","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add","add"]\
+ [[9999,[5917,-7390,8688,8851,4070,1999,143,-4578,-7571,-1336,4778,-7132,2648,3507,9844,-129,7303,-1681,3351,8782,6505,3912,-1577,8845,-3458,5354,-804,-9917,-4504,-1524,3943,-1307,-1931,5847,-1457,4509,-3050,-6123,-7795,-3480,8390,-4121,-6830,7444,-7604,-6608,-8647,-5771,3520,-5580,3721,3693,-5280,-239,6947,6399,-4876,737,-9546,1416,-3314,5411,5851,-7496,-5436,-887,6303,-7848,604,-9441,2903,1090,-1978,9601,-3801,2575,-994,-6180,-2962,1510,360,-9244,242,-4357,-3399,-1691,-4284,-9882,9923,7216,1331,2337,2237,-9894,-2768,1328,-919,-2721,8436,4884,8107,5359,2703,1751,-9986,6479,-2951,-541,137,3338,8152,190,-1623,-9752,-4961,-7182,6367,4380,-2623,6265,-2700,-6791,1999,8505,-56,-1627,-2201,6071,-8489,9201,9042,-7680,-6884,-4369,3500,380,1146,-5186,-340,-9888,9676,-188,-9641,-7082,6049,2744,-8039,1062,6138,-2486,-5884,-462,7584,-8291,4185,3342,-5742,3900,-131,2516,3059,-5450,-3839,4345,5989,-9346,-2814,1465,8235,7044,-3375,-1461,-4704,-9121,7395,-2344,4067,6274,-2498,-4744,-8465,-2239,1985,8718,-6353,-7120,4975,36,3489,-9635,-3046,-8510,1222,7699,-6862,-6447,-8395,6402,6836,-9058,-3154,9107,-5803,-3109,1655,-4116,-6484,-2179,-481,7640,-8780,7990,6050,-9629,1264,-752,9037,4868,-2540,7061,9417,-7086,-9054,991,6086,-1416,4687,2543,-4171,1513,9666,-4342,8722,6390,6907,-4650,-4376,8478,-349,6730,-2246,-1111,3995,8104,-2258,4236,-9690,-6000,560,-176,7757,9654,-7355,6689,811,-7182,3495,-1277,1640,2385,-4900,-7537,-8527,3703,-8340,-1554,985,5782,-5088,-6378,8184,5387,5615,4506,-9440,-7123,695,1365,-1579,-155,290,3803,-5162,-829,1860,8106,6879,-3566,-8309,5938,-2784,-8236,-5678,9387,2036,-6100,1370,-9420,-7644,-4199,-3112,3564,-3390,-6380,8982,7937,7425,-1298,9396,-4522,-3305,-9077,6501,-367,-5327,-761,-535,1518,-9260,7604,-8755,-4785,4340,-3627,-4383,-9770,-6996,-6329,5765,456,-1510,-1494,8434,-3970,-5869,-4837,2457,-5029,3440,-1544,5177,7874,-1233,6581,2285,-7482,-4030,-7203,3284,-1621,1853,4626,-3438,8368,9215,3846,-826,4642,4428,7228,-3316,-707,4151,3098,-7840,-1095,-6909,4755,1806,-626,-5662,-7957,-8210,-8207,-2994,7421,3416,-6087,-5165,5259,7474,6479,3573,-3009,5253,-7240,-7349,161,-8870,5499,-2346,-1154,-6637,9466,8258,-3855,9473,5168,-2172,-1075,-4952,-1783,3207,6165,-5726,-1670,-9535,2726,-4390,7131,-3459,3389,-781,-5300,-1257,2175,-1292,-9970,933,-8177,8187,4336,8995,3825,-1879,258,-6379,-5503,4910,-4620,527,-6417,1349,-6815,-570,-72,3063,9828,4628,-7176,-8145,-2612,-5369,9332,-6919,-5123,443,8168,-7289,-4287,3961,8464,-5237,-3452,-6184,5235,5337,8287,-8451,-6311,-9053,5008,-2953,5422,-2688,-2697,-7834,-3245,544,-1117,-3546,5677,8167,7794,5329,7378,-1162,7094,-3975,1435,2686,858,838,-7292,-4856,696,-2361,7434,5785,-5749,-4911,9250,-1313,4109,-8192,473,-2602,1647,-9284,9960,5445,-2933,1265,6093,-4091,3809,7677,6515,-9519,-1561,8453,5842,-5240,9933,-5001,6782,3813,1083,-3118,-5883,-4972,-9130,-8425,6733,-1149,-7713,9998,-4435,-2321,-1317,-7831,-9012,1371,2086,-9133,-8541,-2602,-9662,4737,5480,1122,-3533,-2650,-7660,-4410,7274,-7321,-9152,-2590,1121,-5509,-7682,9117,7501,438,2548,8002,-9795,-6358,-9011,774,2265,3051,3079,1377,-6951,4279,1420,7445,-4884,-8816,-1562,9643,-295,8865,-9264,-9087,-8237,-1299,-1131,-4776,8945,1113,-1234,9563,9523,9909,8452,5247,-7117,-132,-9064,6966,1671,-6136,4450,6692,2207,2135,6285,-8775,-683,4655,5102,-3439,-3088,-2149,-6219,225,-446,7021,9447,-1322,-718,-5753,-1354,3196,4226,8668,6375,4970,-1491,-8392,-5623,9274,-4477,-3553,6262,-7761,562,-8122,107,-5612,-4742,5792,-4077,-8658,8515,4674,9865,-7795,3621,1491,-8357,-2945,-3060,-8706,-8441,-7131,-2927,-603,-4612,-5842,6376,-7500,3618,1355,-5690,-3647,-680,9765,8956,-7332,4096,-8905,9973,-5985,-4777,3852,4445,8701,-783,-7531,-7422,1763,-5907,2995,-1391,-5556,-7040,501,-4749,1326,-6159,3297,7849,6532,-2295,-6132,9844,3418,8688,2680,-8632,3946,7078,3749,-6638,-9558,1309,-5715,-2564,-4679,-2779,-8312,-9558,2715,-3128,764,-4301,-7144,-7688,-5468,-578,-4580,8557,2315,-8475,4664,8264,359,7122,4405,2344,1523,-3267,-7962,-6434,5761,4629,-145,-4810,1370,-6976,-5587,8104,-4030,-8552,-3482,-2280,-5895,-3661,9481,-2979,1906,-1552,-7196,1614,9696,-9187,-2558,8926,-1750,-5357,-3741,4722,7673,-7738,-456,-3917,5555,7549,5357,-1787,-730,-6993,9644,2448,-3912,-459,305,-4696,5446,-6736,-5988,6592,5169,-7889,2095,-4446,-8509,-6672,-4501,7218,-1525,-6989,-6456,-409,2953,2501,5664,-2319,3511,5269,2840,-6278,1958,-9600,-7101,7500,2775,4424,4895,-1454,-5431,7418,3374,374,3754,-4884,-6977,1558,3696,-3849,6334,9576,-8401,7098,4995,-6392,-5392,-6324,-4780,-6449,6887,-3007,-184,2405,-4557,5416,3527,-5334,2306,-752,-6382,4294,8712,-5228,-670,-5065,6915,8035,59,-2793,-5548,8571,5447,3765,8949,-4819,1450,-5522,-5431,-1743,1534,4704,-593,4536,2782,-9263,-319,-9911,4923,9856,1519,-2554,-3651,-3499,-2032,5476,8286,2897,1327,7519,-1748,-9112,3458,-824,9869,-2521,7824,-2631,1986,381,-1429,1134,-4867,-9410,-8616,-4830,-8784,889,-7421,9585,-3264,-8677,129,-2677,-7301,3252,2376,5289,-3577,-4564,4629,3974,-1315,-5120,-7611,-5126,-4262,3143,4953,8237,-8102,7113,7153,2681,-969,-3050,9877,9100,-5995,4359,-2427,6219,2013,-1339,-8798,-3915,-6593,-4508,-9261,2598,-7528,5339,-1000,6193,-7535,8679,5710,-6223,9126,-702,-3699,1026,-8381,6948,-4207,-6112,-4825,-6744,1091,9354,8811,1147,-6409,9704,-5063,590,8502,3605,-4567,-1626,9574,-2426,-7227,6596,-1802,6529,-5574,6350,-1677,-7193,-5181,8861,8680,7574,-5330,-1056,-215,618,7783,-5318,-3584,2797,7277,-3245,7137,-9776,-4759,-1553,-7667,2126,-6719,-8769,-7600,4171,-5297,-8959,-4309,-2330,6666,6262,-6666,-5249,754,5536,2926,8897,-8291,1981,-6545,-7889,4628,572,-48,-3854,3011,-7646,-2666,5559,-8602,7132,8360,-6273,-3581,-6087,5260,8975,-5344,4469,-5072,8965,-2131,-2968,1121,5761,1248,-9365,-1681,-6257,7420,4405,8412,4735,-4223,5349,7251,582,2110,8840,-6086,-1006,4270,2263,-3632,1820,-1294,-334,8496,-7478,-7066,8460,9628,4897,-4422,70,3201,-8844,-3044,3390,-4094,-5617,-8730,6243,-1546,-8411,5185,7118,8009,4385,254,-3439,-2858,-6897,2868,8643,-3378,9388,1560,584,-1932,-4587,-571,1596,4035,-4483,-2277,-4134,348,-3726,-4450,-9268,-3951,4174,4680,-6624,-3097,7748,7754,6729,2369,-9032,5842,-2126,2760,6603,-5855,-1882,2218,4994,30,6998,-3268,-6157,6102,-7898,5230,7011,-5823,4625,934,288,-1780,9324,-3511,6490,70,2419,-8594,9809,8171,5065,9330,-1376,2244,1389,-6977,-9247,-2497,-4740,3675,-3806,-7164,9424,-8942,-324,7343,2125,3843,-7533,-4563,8480,-2455,-6093,2282,-6999,-3266,6202,8996,6702,-9012,6972,3374,2274,-8310,-376,6885,3567,4759,7508,9053,1247,-2127,-5523,-8537,9687,-5081,1794,6197,-2641,1167,8246,1853,1498,-1814,-5168,1239,9965,4278,1094,3227,-9871,1665,-4206,9309,-6362,-7375,5808,-6840,-9130,-8704,248,7063,7330,-2023,-2099,-3353,-2629,7246,2866,2404,-4260,6795,8313,3280,4753,-8309,-1103,1670,-4779,-6032,-1824,-5315,-3759,7701,5907,-4227,-4166,-5030,-6728,-4935,7439,-2339,4116,3259,-4905,247,-5187,5535,-2302,6924,9126,-942,2422,5913,4369,1261,6158,8426,-9911,-5488,-3513,-9473,-4318,-5059,6554,-4613,7961,914,700,-869,-3530,-3597,-6922,6298,5132,9390,-8567,-364,609,2623,-5762,-1629,3970,-8113,2125,2266,-263,-1975,4464,3928,7627,9856,-9734,2421,-3139,2843,6737,-1694,-7351,1840,-7825,-7375,-7596,-1259,2575,-2485,1521,-8406,935,434,4045,7400,1768,-7141,-9056,-1914,4835,-7123,950,-5569,5793,8432,3442,7108,5527,-3727,-4282,-5078,-5144,9811,1401,-1237,2492,-1323,-9415,4767,-6498,-527,-826,282,9562,6730,-7306,8631,5126,446,-4049,3598,-9211,-6559,378,4604,-9929,3654,5445,-1295,-3839,-8181,6691,1914,-544,4294,3189,4338,3579,663,2193,-3973,-2790,6602,7989,-8439,-2549,-2568,-2920,-6188,4716,6501,-4652,-9617,9079,5952,-8910,-318,-166,958,-1352,-592,-625,-6081,1891,-150,-2925,-8641,8283,-5325,-1619,-3482,-4208,1973,-3430,1761,1821,-6084,1895,7450,9453,-7424,8083,2447,-8972,-5211,9942,1738,-6448,1022,1500,-742,9531,424,1343,7339,7222,-7290,8226,-7737,-2466,-4726,8787,-9603,4477,-1078,1780,-7842,7540,-4114,3925,1151,-2824,-3971,9283,340,-3369,3963,-5189,-2695,4144,-7822,-8383,6886,9887,9624,6254,4661,-7263,-9073,-1934,120,-5369,2861,1857,-7525,-4912,4195,-3431,4656,-6317,-7538,-1422,190,4272,8612,5264,-2986,3682,-8324,-252,-6725,3909,6838,-3231,306,1521,241,-618,-2716,-1071,-5718,8878,926,-7656,-4808,-7947,155,-3981,-9897,-8275,-7018,9625,2031,8011,-1598,-1626,2740,75,-2497,7376,-5122,-6694,528,7003,-9569,7195,9636,-1885,-2344,8211,5387,-7833,-4604,-3260,1256,2322,7645,-757,-649,5352,7782,1471,-6108,9130,3516,2756,-5670,-303,-6438,-4822,-9187,-3362,235,453,-2565,-9174,-2332,-5946,3292,-4261,8144,4257,9868,3055,-8111,-2609,-6339,-3878,-9541,687,5946,1867,-4920,7860,-5228,9282,3528,-2182,8348,-2957,-7259,2533,5620,482,5000,-9963,9165,-6050,1662,-5489,8718,-8270,-7428,-9400,7575,-4374,6414,2558,-2143,9091,2276,8819,2183,-4247,2065,1435,-7361,-9998,6583,894,-791,4423,4700,8303,-7417,361,-9078,-3359,-9995,9990,1551,-9865,9183,-2043,-860,-6842,-3328,1847,9327,3191,1296,-5182,9611,8328,-5981,2369,-4679,-9757,1536,-3544,4276,-703,7800,-8835,-4037,8743,-4791,-609,7200,-9314,-6056,7410,-7189,8137,2806,7029,4177,6283,-4563,-6574,2338,-820,702,8402,-1292,5185,5989,7527,-4846,-396,9089,-5980,1091,4884,-9135,-384,1260,8448,2436,5969,2281,2704,-4089,2062,5554,9199,-2985,-515,7851,-2412,5455,-9017,-4679,5926,1896,-2858,1517,-477,-9751,3863,2784,5454,3076,7802,-4238,8097,3338,4342,-2004,-7064,-3688,-1435,-2673,-5156,9173,9564,8111,-5318,-871,-4874,-8626,2116,-5739,3197,-2226,-2240,-3286,-9574,7313,-2141,-1815,-4498,5573,-9658,8913,-8230,5843,-5463,4525,-9601,330,9219,-888,-7943,2664,-9144,-96,-5387,-1041,-1655,2654,-2663,-8794,443,9618,9770,2544,628,8422,-1833,-5402,1539,8862,-8551,-1679,3287,7594,-433,-6987,6772,3452,2560,-3319,917,-2129,4209,-924,-9509,-5179,-9555,-9931,-6040,7690,-5280,7301,3978,1801,-7318,-1533,3275,-9722,4024,8891,7110,8152,-6540,4625,5429,9438,1655,4401,-3869,-5454,-4326,3757,7019,5347,7708,-1883,3108,-7426,284,9566,-5494,417,5494,2696,1686,1936,6662,4437,-3047,1029,-7413,3410,-8242,1122,-4867,-88,-8210,-2341,5508,-6850,-7946,1881,4117,1477,9342,-2775,-6332,6700,194,8056,5548,8184,-1849,9252,127,3834,-8577,-7911,2376,-1441,5976,6572,-883,5403,4991,3738,4694,-8981,-1798,4487,-8943,-3594,8286,-9189,-6137,4176,-7822,-7682,1782,9641,7997,-3498,-5376,4396,-5924,6510,-874,-4863,-4393,-6814,7336,-3983,-2393,9663,-6338,6984,1508,-6410,1714,-6863,-3719,-8733,-2460,-2788,4238,3875,-272,9494,-2396,-9962,-2254,-8497,-7302,8275,4676,2659,6856,-6453,-894,-4946,-7371,9060,-68,6989,-7440,-4042,-1638,3418,-2788,-9976,-1231,-7242,-4453,7459,8478,4220,1987,-7111,5595,2049,7296,-7765,-6008,-6106,-8285,1632,4803,-6813,-8274,871,940,1803,3355,-9420,5913,-1855,5291,-9993,7436,-3987,6445,-1019,-9331,2850,-3686,-5418,3479,9565,6491,-6121,3995,7517,2816,-4643,3910,-6046,1699,-9781,7798,4043,-2558,6616,5118,-663,-1162,-7952,7224,-6664,-4520,1155,-3577,-5351,7380,6019,-8732,-8398,4680,8794,-4686,7015,-5280,3591,-5885,-5999,-3426,-6095,-9945,7279,498,-6277,9288,6467,-7719,-413,-3192,8484,1280,2267,921,8972,3008,-7094,-1722,6646,3775,586,-6698,-6082,7767,8202,4216,6065,-9104,8650,3960,-8514,-20,-4149,5315,-6824,-2060,9960,5982,3190,4692,-4164,-2818,-8627,-5288,8174,-1393,-8317,-3033,2027,-1475,-136,-2548,2632,-2607,2163,-5243,751,-6159,9990,8515,-4965,1833,7794,-8345,-4587,-914,-2981,8761,-3242,1608,-1785,2713,-5707,7190,-4662,3563,-5153,8341,-9890,-3979,1107,140,7525,5399,1411,-3927,-9378,7619,-4378,-2539,1218,-2865,-3358,-4169,-2795,-8714,-2807,-1401,9688,5066,-2429,2420,-2612,6998,-2428,9504,7508,-2828,6104,2276,-6466,-35,7272,9621,523,-3783,194,9355,-4733,8418,7932,7486,-3526,2743,4916,5551,-1465,8481,-2770,5913,2456,-613,-7389,399,7582,-2302,2604,-9722,-2262,4046,757,6636,6189,-334,-6409,517,4197,4386,5582,1123,-9502,6877,-500,-775,-4989,7370,6009,-8068,9013,-1843,-4099,-396,7477,-3587,-1725,-3764,-5524,7603,-3569,444,-9076,3230,3571,-4861,-8701,-8493,2394,-4277,9908,-7188,-5282,7404,-5698,-6652,-3256,2670,-2366,-9976,-8397,-6894,-3015,9819,-2785,-311,4634,7277,-7980,-4224,9194,-7455,-5677,5160,626,-436,7885,-8835,5353,-1615,-7049,-7514,-7460,9125,-149,9564,601,-8434,2879,-8115,-704,-2061,9370,-7593,-9183,-7150,145,8468,-4243,-614,6755,-6825,9827,-567,6850,-6810,-3850,4361,-6879,-5419,-5096,5227,4226,4769,-8689,8138,-7034,4988,7334,2846,-4212,-2662,4574,-4200,-9805,-8757,5332,9442,9393,7546,-5918,-9190,-8039,-6145,-1333,-9450,-568,2713,-1507,-9932,-6205,-1804,5983,168,-8160,3002,5006,7396,5826,3483,-3793,4468,7143,2767,-5514,7205,-5133,-2832,-2995,-152,-5137,-6316,5207,-4744,3971,-8642,-6987,-9755,-914,7675,-8650,2675,6744,-6049,186,-5458,9710,-6624,5832,-8596,1587,9149,395,5588,-7482,-1377,-3371,-2453,7719,-6555,-1390,4460,-5949,-7421,-8573,1266,-4835,3588,-5696,-222,-4386,-3743,-1795,-4943,5758,-9988,-1722,9374,-6473,-5304,-8377,-8500,-7489,1089,-4214,-1901,8963,3937,7561,-2493,-7560,6930,568,-2870,5662,296,8012,-7981,3462,-505,-9708,-6744,9963,-6968,-4525,-8039,5462,1622,-390,2757,-6409,3991,-1128,6046,-4309,5632,9165,-9585,7207,-5201,-5253,7858,-770,-9523,3202,6613,-4464,-4529,-6529,6119,-7586,-8017,9356,-6699,-7201,9116,-3369,9469,-2673,-4199,-5148,-5921,2577,7292,6762,3081,-8423,7074,-5138,4181,1849,703,-2577,-8391,-9953,3772,9591,-2450,3633,-6711,-8063,-6136,-748,-648,2298,9238,6878,-2536,3113,6362,4161,-3041,-3518,-9741,-1370,1773,1441,9294,3331,-35,-6243,7037,-8470,691,-3701,5659,9511,-5078,3343,7030,618,9293,5105,622,-3576,6770,-1686,9529,-7032,-7678,-3464,4018,8528,8433,-1003,3442,-3936,3264,-4860,5142,-5426,-3064,4262,-2611,5031,-3246,8135,4161,-1638,992,-6042,2466,6082,-6172,-6530,2821,-3212,-4845,-1000,477,8828,-8549,-2120,654,3278,2530,-7633,4468,301,1542,3539,-9180,622,-925,6409,-6407,8362,-5847,-3607,-7378,-4765,-862,4121,-4957,-6557,-4672,4537,2200,3673,1287,2129,-6635,-1286,-5575,8539,1756,354,8565,-9882,1811,-5560,5037,1902,-9065,-5804,7428,-4375,8214,2982,245,-2045,6250,4758,-5494,4077,-7962,-6945,-8283,6733,2879,4662,-8235,-5153,-7781,-2832,-9113,9884,474,7783,-2554,-4993,8801,-6183,3615,7397,9208,-8624,8283,-5201,8338,9727,-3974,9303,-8931,3533,-4444,676,2632,-7164,3947,3854,7575,3012,1346,1972,9707,5480,-3860,5517,5898,5665,4646,1977,4753,9476,9102,1792,2450,5690,-3595,-123,-1780,-8917,487,39,-2775,-65,-8190,6243,-8231,-1724,3874,-4946,-5232,4942,1670,3716,-7728,-9711,-8210,-7956,-430,8703,4381,8298,1238,-2806,6372,6266,-5483,-3393,7050,4528,-1124,-9641,-2347,5681,-2488,-8494,229,2089,-3899,707,-7082,-2383,-5190,5440,-9978,9931,-9100,-1138,2822,4825,4191,-3928,2357,-815,-6411,-6731,-21,-4954,7092,3007,-3569,-2293,1886,-3302,754,1258,9006,-6278,7736,-5744,8350,6705,-9143,1772,4542,-601,303,-9959,8218,6452,-5248,-7032,4398,2864,-8124,-5128,9458,5398,2843,-4490,-346,7394,1845,8334,-8286,2900,3020,-6844,-2367,9230,-5059,2533,998,-660,3617,-4009,617,319,4013,5452,-2962,2006,1852,-5318,2365,-2493,8037,4271,7736,6934,-2001,-8100,7379,5676,2201,1120,-5128,-4011,5785,2050,3487,383,9252,-4246,131,8761,6843,-4685,9306,9625,6076,654,7468,-9404,4617,703,-2825,1485,1150,-4357,9311,9757,-3994,-5018,-3185,5686,-487,-6445,9253,6602,6554,-8580,1924,8267,9731,-9098,92,527,-6433,3292,6495,-7985,-855,-4934,3172,-5470,-9118,-7555,7469,1602,-7213,-9198,3838,4969,9865,-3630,9136,-5987,-7874,-7572,1214,-9660,-3114,9846,9996,534,-7867,-2958,5088,-9777,3534,-7191,-7563,-4095,8641,7047,-6712,-4163,2131,2651,1465,-1413,9000,3293,-8566,-1006,-7524,5973,-6435,4657,-8701,-5183,8645,-9283,3075,-6953,6987,-3225,-5676,-7899,5296,5674,5296,-7062,8910,-1943,-4092,7043,-3145,7614,-2923,-6733,-2314,-3050,-3784,-3148,1145,-2464,2438,-5329,20,7309,-7725,-5980,-2899,-1626,6654,1498,-5273,-5994,8923,-6741,-3796,-4740,-8793,-3448,9441,9328,-765,-9425,-1964,-1514,381,1305,-8948,-5151,9526,-478,3147,-5127,-508,-9381,-5030,7731,5128,8546,9638,6843,-57,-8025,8630,-7648,-8509,-3531,-3165,-1637,7616,9952,754,-8960,4381,702,-8155,-6513,-7163,1276,-3793,-9367,-6548,2600,8655,4989,7649,-9253,-9046,-8592,8867,-4507,-472,3960,3157,7160,3887,-4099,-5852,7717,-4746,1136,-7410,5417,6732,6261,-3092,1495,6989,3957,-5539,9387,-7232,-4812,8213,4201,-1921,-266,2370,-9608,-7155,-1568,-424,9701,-7083,-6451,1872,3987,-4845,-5135,1822,-8011,-6154,-3693,4128,9744,-8479,-4274,5937,3649,-6220,-3857,-7868,5648,-7643,-2157,5248,1576,803,5858,7219,-5043,-7030,-4876,-2064,-391,9978,6370,3498,180,-4752,-3551,-3724,-9012,-8315,-512,3319,-3789,2220,2003,-1903,-4236,2434,-2859,4609,-4897,1353,8411,-7449,-2931,-3097,-7428,-9723,6384,9084,-4836,3353,1602,-1358,-7975,4549,4065,-4128,9485,-6820,2327,2078,-594,4371,4082,6316,2974,6544,9629,-4724,-1494,4340,-2215,-1169,-2216,-6887,-9895,-8454,3258,4750,-5420,-6178,-9740,-1987,4508,-2638,-7211,5492,-9365,5108,-3136,-1587,-4485,-5631,-1395,2260,-9747,7558,6393,364,6537,-1476,-2773,-7633,6909,-8591,4594,7700,9605,9714,-3056,-2731,-54,6813,1613,178,-4119,-9985,3081,2770,149,-9335,-1123,-1394,-4701,6652,1229,558,-6336,8239,6682,-3965,8087,5519,-6234,-4347,6942,4268,5252,9772,2283,-1995,2667,2679,4006,7609,-8550,7654,-9682,8989,7108,-9085,4311,-3830,-1219,3664,-2475,-4239,-4545,-7103,5891,-8884,6770,944,1352,-7658,3598,-7796,2274,-3444,-5023,-4813,-6220,9090,5911,8274,-8354,-7703,6149,5921,1175,-1354,7588,-7012,5384,-9790,-1524,678,-1786,7866,3550,-7973,2956,-1556,-8851,-1474,637,-1833,4797,5833,-5120,9903,7925,8297,7089,-1905,-9520,2184,-850,7870,-4470,-951,1790,9506,8774,-3435,2144,1251,2442,-6901,2984,-6564,-2426,-547,-1779,-5,7237,-9091,8355,-6584,-3671,7091,-2192,-6885,-1124,3853,8081,8014,-6898,1588,8881,9467,-6414,-7921,5142,6325,9080,-8406,-5098,-1147,2078,-8997,-3383,6816,-3345,-8923,9316,2234,-345,-3904,-3891,-7375,8907,-890,1193,9026,-2001,2385,6392,-7836,-2817,-6498,3963,-1432,-2104,532,8316,-6550,2169,2675,-795,-7649,2928,-9981,-8103,1125,-42,-8525,718,6601,1501,-206,-79,2018,1938,-7388,-331,-1663,2951,2775,6459,4909,1868,-1594,1118,2726,7519,264,-5517,-4703,-9621,970,2558,-1307,-6682,9961,-3970,2125,8890,3177,-894,9705,-9389,-6594,-3164,-7665,5568,-8388,8470,-1898,-4781,-6330,-1373,-5647,-6023,-2309,5991,-5677,1793,3994,-7264,5111,-5437,-4724,-2129,-9807,-3758,58,9856,-3461,2499,9141,6029,-276,3289,-1941,5395,-654,6709,7849,-6266,-5226,-3535,-5693,-9574,6025,1568,-4144,-465,-1291,-2061,-6732,5905,-6514,-6191,1744,2901,884,-9198,8296,6629,9773,5894,-2171,2011,-1639,-3932,3206,-2988,-2748,3718,-827,7997,-9068,-3166,-6767,6446,-4138,8281,1893,-8190,2018,8595,7161,-6259,-4203,4351,-7868,-154,-6586,9515,9725,7,8608,-6470,-3059,690,-329,-4621,-1303,-3787,3793,-9184,3634,7965,-8649,1229,-4995,7387,4789,7062,-9776,-3814,-8532,9937,-9874,-6582,-803,-1539,4754,-755,-4410,4353,-8734,5678,-6713,9927,-7851,-9098,-7259,6527,108,-1964,-7724,1936,2783,-6841,4499,4907,-576,-9710,-1173,-8141,5766,1597,9555,-7105,7418,-9799,-220,-1390,3299,-9225,8455,2978,-5646,-2470,-2445,3150,8316,9410,-4126,9566,-7205,-9396,-3375,8212,2722,-5979,-8858,-8669,5513,8280,-3325,-6747,-5578,-3400,-1129,6218,6241,-1982,-1874,-9645,-1257,3999,7169,4992,-5132,-6391,2379,-2279,3448,-1955,-8394,-6521,-7910,6806,-9358,8062,1370,2623,-749,-2692,-324,699,7039,-2942,-1742,8077,1873,5734,-8645,-3666,-375,8092,-2754,9791,7311,-650,-8071,3369,835,-1646,-8655,1658,-1698,7387,9798,-3535,-4952,-4845,-1755,2854,2037,7500,7889,-2830,-8950,6173,634,-6746,-702,-7406,-1598,2937,-1071,-1380,907,7167,6144,2682,-1023,-3133,3060,4241,-5624,2860,6857,2030,1425,6336,4728,-8643,-1309,7981,6493,5054,5830,9035,1630,5093,-1085,5119,-5785,3503,6618,-4520,-9062,7280,4896,-3458,901,9097,-4845,6779,-7252,2519,4680,3647,9030,1731,4555,1574,4814,2957,7869,5643,6514,1894,-2491,7087,-9749,-4159,7606,-1194,5089,4687,755,-5705,7696,-1640,-4286,8261,4378,-8924,-9629,-6828,4712,-6328,4499,-6573,-6871,2073,6896,2490,7566,4079,2675,-8759,2949,-8835,-621,9259,2073,-338,-7840,2338,-5103,7447,-1035,-4459,-6443,-9200,907,5737,2825,2180,4585,9006,-4508,-6600,-2899,4964,-4857,-7346,6385,6545,-2971,-7898,-8717,4054,3396,8821,8886,1323,7749,-1762,1624,-7444,5526,-3932,9300,-4563,-5851,-8085,-2839,9459,-6194,6354,5225,-7242,9093,2138,971,9724,4220,7707,-4806,5630,1592,2369,-1629,-188,-33,9695,-6782,6561,-7995,-6007,-2311,-1746,1767,-3630,7124,7771,-3335,-8371,-7426,8165,-7171,-6168,-1037,8434,7695,4304,-1234,6198,-9671,6840,-3896,4038,4822,7212,-951,6788,-3921,-5537,-8024,-7008,-8722,-1508,-1889,-3006,7002,4899,4173,-6995,-1449,577,5147,-7958,-5309,-9679,-2685,4994,-7635,1305,9829,-4567,-242,3781,3004,4075,-8292,1865,-8434,7632,4381,-6495,1619,-3869,617,-6998,1878,-6685,5057,-5885,5116,9960,2019,-4639,-8636,5020,8517,8699,6104,8620,-9904,4229,-3237,-1791,-6396,7210,-3458,6754,8124,-2709,9179,4248,5265,-9939,3115,4045,-9040,5935,2101,-9115,8099,-6370,-5186,-1553,-292,1245,-141,688,-4569,1546,6597,7177,6199,-8438,7879,6540,9573,9939,9631,2657,1598,5544,-9891,1470,7385,-2165,6660,6035,-5958,-6713,-4951,9298,6533,-2537,7657,7348,-3745,1756,8180,5548,5905,3526,1916,4358,6101,3993,-1191,-491,4926,4278,4626,1757,4298,602,1696,8416,9856,-2691,7095,-4848,7093,-6560,-3507,2239,-9432,-5637,-7482,-322,-125,-5196,-5069,-179,-5832,-6437,4364,3830,9449,538,3399,-4428,5412,9277,-6720,-2319,7299,4834,-1587,-7380,8406,1275,-3238,-6225,679,5269,-973,-8022,-9842,9878,-2016,-231,-1966,7753,1111,4064,-2109,-5344,993,-9017,2386,382,3418,9815,323,2858,-7316,8367,-412,5951,-9664,4468,-7858,-5432,10000,-3130,-7724,-1041,9831,2913,-6267,8954,8635,-4483,-3208,4334,-8241,-3515,7093,2989,6913,3496,-3777,4421,284,-6098,546,3512,583,-5693,-6568,5738,3489,-7797,-4344,-1795,2485,1334,-9137,-8020,-798,2099,-1115,-9420,4060,1344,-7341,1905,4054,4692,-9446,-6228,-7954,9030,5224,-6641,-4802,1723,-5671,-9008,-8799,4988,-5784,4390,4168,6222,659,-668,-9955,-1346,1674,-1319,6779,5476,3170,-815,-9661,-8555,-7494,493,2764,-1237,3315,-867,-5393,-8815,5181,-1562,-2932,2016,-8601,-4103,1727,-778,-7688,9759,-9382,2652,-5386,-7531,-3478,5802,8499,4642,1392,8163,7399,7216,6013,3666,-3668,-2451,-2461,10,6729,696,-2811,-957,9420,9334,7344,-8709,-9369,-7210,3079,5139,8017,5671,5320,5625,-5700,-4511,2263,8436,8540,-7661,-2254,7292,-845,1917,9239,-1327,-861,-9512,-283,-6541,-2718,5950,3406,972,7583,-2015,-789,172,7863,1757,-6880,-3188,-104,1964,1000,4371,8297,-5605,9693,-3531,7164,65,7097,-9623,4404,8649,8540,-6485,6330,-6623,6153,-5556,5879,-1200,6434,-7714,-7802,-8076,5307,-1379,-3939,-2633,-1112,-9454,7229,-3886,-8616,7083,-7221,758,-4512,-8199,-2392,-5665,-5724,-8923,8860,2795,5772,8753,-149,-7784,4126,-5632,8925,132,-2350,8163,-4450,-5369,-2730,-4633,8968,-2999,8806,-7803,-1476,4090,5342,-4917,9577,-3068,-7427,3371,-3663,8203,2865,-9328,-2930,5407,-3831,6233,-3970,72,2224,9433,2808,1580,2793,2772,5914,344,-2897,914,-7829,-2535,7110,-1200,-1598,-9344,-9844,-8732,-9858,1240,-5158,-9194,-3495,-7934,422,-9447,-3850,482,8800,-5382,181,8239,3930,-5198,3429,4551,-9279,1425,7525,-6312,7180,-2421,624,-9880,-1332,1999,-1177,-5004,8050,-3272,2748,-6333,-6597,-1633,-2932,-5966,2086,3096,8881,1373,-5390,7386,1678,1577,-1333,-4964,6333,4568,-6329,1202,8483,-8586,-5440,-6124,-1089,-22,7048,3596,-5475,7162,1804,3877,6067,3999,-3565,-7087,1117,548,-1091,-4024,5058,356,-9616,5478,-9116,4768,-6084,-8608,-5438,4135,2166,9342,-7959,1018,8855,6816,8125,2702,-4182,-9826,-1326,450,-651,-3179,2756,6661,8000,3142,1309,2786,4010,-9304,-9742,1409,2696,-770,-9922,978,-3356,159,-5779,-1600,-8892,-1620,1448,-578,-2660,-4287,7714,-602,-1423,5319,3835,4328,4333,8068,-841,6187,-9764,1936,2893,1124,2733,-2791,-9614,6219,4670,8929,5031,-7512,-4215,-4485,-4759,-5060,329,140,-231,9587,4726,-3321,8503,8516,-6163,6440,291,8195,6248,-7793,-3355,-8029,8959,-4888,-9365,9366,6360,3712,7789,4399,-4153,-1872,-3096,685,-5500,9330,6026,9348,9718,-3675,9948,-5133,9097,1401,8504,1419,3261,-6335,9221,-9514,8738,-4580,2297,-9120,-3695,-7320,-3134,-5337,-8273,-4694,2880,-7328,6974,7275,-6107,-3396,8794,-917,-5018,1770,-8569,-1845,-1506,-5258,5945,-2725,9971,-4717,7443,-516,-93,4000,-1359,7311,4319,-3242,-4597,-412,-1925,-8995,-8788,3216,-2697,414,2378,-7714,8813,3267,9965,-9618,-1207,5308,2633,-359,3554,-3968,1824,6776,-2780,2708,9536,-3179,-4160,2672,-2911,-1053,-2538,404,9571,-9782,-4244,1162,2736,4828,1611,1522,7882,4125,1559,6872,3421,9848,4810,4029,6019,8655,7055,-1929,-1080,61,-7269,1173,-2101,-9402,-1967,6415,6513,2006,4204,2538,8678,-1314,3382,3783,-3952,5538,-9130,-44,8396,6827,4765,-9901,-4291,-8475,8953,3342,1358,1365,6568,-8850,-4494,769,-5021,-4566,-2439,-2952,-690,-1343,-7037,-7719,-6933,1095,6203,-6529,-6552,-1896,-9734,-8642,-6383,3707,-6291,-7017,-6222,-7820,-86,-6920,2851,2902,-3305,-4034,4082,-6960,9234,8221,-7464,5659,-7375,-6226,2864,-4941,8584,5790,-7905,9767,-2348,196,9380,553,-9104,-6991,-2512,-6217,-5616,2565,-1768,-105,5377,7294,-4187,4322,-8007,7947,-3293,-2520,5175,-7777,-8349,525,-1016,6867,428,5989,-5913,-5161,-6693,572,-3608,-3133,2731,8281,-5281,-9533,-7005,5060,4655,-9288,5494,2161,-4235,-9550,4723,3639,-6485,7607,-1847,91,-4009,-4591,7435,-6746,-7678,1135,3259,5488,-1644,-8484,-3590,1388,9795,-3121,-3266,773,1431,9417,1825,-7976,7035,-9946,4308,-8524,9940,-1896,9061,8060,1386,-3104,999,-531,7476,-5234,1952,-1652,2447,7174,27,-1279,3080,-2067,4046,-1492,-6089,470,1341,-7990,2359,6864,8223,-182,-9978,-9488,1503,-8235,2632,184,5380,-8166,4793,4566,-4519,-9736,6188,-256,4678,487,-5550,-1659,5244,5617,-4290,-9812,9009,8144,6425,7706,-6689,-3146,-355,-6085,5757,-1865,1985,3801,-5116,7777,5879,9278,8206,-6165,2785,6427,-9906,6644,-743,2470,-3968,3706,7091,-5394,-8174,-2669,802,-4300,-5696,-2950,4661,-1802,1891,-8922,9238,-3435,-3130,-984,8379,-5567,4249,-6052,1669,-5649,-6212,-1088,7078,-4552,-3442,3517,3866,-7457,8799,-8818,5523,-5816,-4640,-7578,-1640,-167,-4075,-459,-7591,-133,3695,3353,-679,-5767,-581,4818,-3625,9603,-382,-5722,9057,6228,-2577,2740,720,-5609,508,-7096,7779,3741,1079,6756,-8537,-2077,-97,-6446,-4613,-7354,8874,1539,1639,-7159,-1252,-7952,-2354,4103,-4255,7,6021,215,6176,-3377,-2209,-7266,-3711,-9340,-2425,-2546,-5201,1731,-1212,-3653,2740,5969,-7088,-2957,-4670,-9630,-4147,8900,1975,-3146,-3318,-3417,-7380,1366,-3445,-2656,4422,-6718,-90,5082,-9105,-3582,-618,-4266,1344,-6663,-6252,-187,-7570,-8069,-5116,-8251,7681,4183,412,5727,8661,-2326,7862,5699,2747,-1254,-9889,-8286,-8788,3562,-643,6534,-687,5515,2003,-7371,-5619,-7076,3642,-6947,9894,7987,-8820,-7994,1896,9523,7461,-7757,1133,-3709,8873,7525,-9683,-5124,-7971,8606,-6285,-4815,5403,-3188,1944,-269,-195,-9089,-1232,-2126,1497,-9282,-9827,3048,-6443,2605,7804,9047,-4656,-3175,5972,1010,5814,-7372,5544,9863,-2172,-1692,-4491,-2607,6806,-2028,1679,-6228,-8399,-9584,-2282,-5169,7312,6818,-8153,8010,8024,-8088,9248,3727,963,632,-361,4584,4477,6278,2984,-7180,-195,3047,-6741,-4451,8692,8022,3509,9611,5235,-7676,-3438,-164,-6143,-9078,-4825,2417,-3527,-5733,2785,2669,1722,8521,7800,7839,5250,1054,9684,-5794,979,-6952,-3372,1376,438,2732,1104,-5690,7528,5648,-8115,172,-7489,-9553,4023,-3826,-1128,-6415,-2919,7979,-3722,1272,5433,-9028,9942,8265,5482,-7110,4741,5335,-6226,-8187,2846,6599,1188,6430,9916,-6883,2491,122,420,-2189,-4613,4452,1541,-269,-8282,5750,-9659,-3453,9433,-3762,2045,6955,-5412,-5363,-5940,-2953,-1240,3215,2387,-1154,-8589,1697,3184,9544,-1413,-2535,-949,-1615,-2041,-369,-8755,-15,-7985,4295,759,-9743,1630,902,5582,-5698,-4975,-5363,-167,-5970,3236,-146,-2387,825,3338,-2443,-9529,923,-7234,3081,4092,2550,3850,7854,-8731,111,-1830,7180,1237,9258,-8756,-3990,-5575,-8371,-9603,1590,-4053,9544,7479,-589,9494,5445,4099,-1232,1456,5675,-8535,6887,358,-2057,-993,7930,5989,6225,3585,-6924,-5390,-8758,7868,1551,9855,-6348,2166,887,9127,-2484,-1528,-1089,-9930,1105,-7457,-2856,-94,-2489,9205,-8910,6089,72,9403,-8680,2715,-884,-6153,2817,6133,-1165,8664,-6339,5260,-1316,-5561,-4073,34,-1052,4405,-2667,-4288,9799,8241,1502,-6780,8334,-4249,-9324,-419,-4504,2671,6601,-4004,3157,-2508,-7796,-4125,-4113,5365,4045,5717,-5112,1729,-851,9293,5038,5171,7907,-8740,9478,1350,788,-3603,6293,-9123,-2608,-1357,3592,-3287,6950,-1604,-8773,-6480,8059,1262,-3578,3939,7744,-1223,-7887,-3000,-2418,4241,2140,-5676,5074,-3005,-7062,-6428,-8378,-1971,376,-8007,5931,5332,3200,3604,789,-2637,-9524,-4639,-8070,-6086,5096,-950,865,867,-8153,-6560,6956,5044,-2070,5176,-8500,-9008,4208,-9279,-4639,1346,1657,5877,-1643,-6259,7444,-384,-2327,-369,-8966,-3379,-8251,-9153,4879,-1235,3624,1868,-8170,-8835,-2760,-4935,1001,4320,7853,3926,7684,6017,2570,9544,2010,-7465,2429,-9928,-5321,7701,-418,-5518,6789,648,6044,6121,-3309,-9852,-6635,6905,7946,8581,6756,-8101,4212,6686,-6882,7287,1183,9024,7665,-1751,-6107,2001,2822,-1116,453,5759,3571,1,1403,1187,-1354,7864,-9790,-6967,5202,990,2890,-5212,-1495,5043,1036,4107,-1141,-7028,-1214,-1311,4880,2914,162,4425,-452,-9692,2782,5589,3294,-2565,7976,-890,-1408,3709,-7219,-2137,7098,8368,-4781,-2136,-6795,-687,-1026,3586,-5796,1667,-325,-9889,-6520,-2151,1092,2826,-5494,-9325,8825,3592,-5096,-8649,6737,4567,6322,1407,-850,751,9650,-1499,-514,3264,7418,7901,3364,-2993,-3728,1155,-5667,5426,-8288,-2357,-7212,-8761,104,8919,5467,655,9099,-927,-915,3638,-8102,1682,6551,4307,-2973,4322,8666,1592,-9310,8153,-1356,2735,-210,4132,-54,-6273,-4827,163,7901,7153,-1588,-1259,6350,-5532,2880,-7538,-8821,9578,3639,-870,-383,4315,-9526,-9558,2071,-620,-8343,-1149,-3136,-8248,-5376,-6263,882,272,6,-9934,-5673,8013,2607,6675,-6376,8243,-7322,6673,1706,3042,-142,-9175,9987,-6730,-1032,6060,-6107,9470,3496,725,6212,8558,8554,-2344,1750,5486,-7783,-2614,114,-2911,9821,7929,-9537,-2182,2230,-2442,450,6246,7941,3478,-5458,9521,5086,532,6758,5187,-8502,2569,-7553,208,8571,-6834,8265,7539,7059,-272,-9886,-4305,8680,-4928,633,8661,6921,-8151,-691,-247,1977,7414,-8849,-6831,-6962,-1218,-304,7909,-1171,5074,-6022,7296,7776,-6715,3492,-4612,3767,7391,-6484,-1540,4229,-5103,-2058,-7843,-1507,2745,-1491,-3614,3171,-2092,-1366,1682,3472,-9255,-3039,-7253,-5822,-6659,-3509,-1292,-9368,-9595,-5629,5919,3470,4997,-4239,994,8473,-9279,-4311,1266,-1948,5804,3254,3802,-3685,98,-658,5447,7054,-3398,-7909,6436,-3426,7530,-3785,3870,2503,-2394,2225,9195,4,-3644,9505,5484,2814,4858,1069,769,-1276,-5135,3940,8493,-3146,4730,1023,-1309,7664,-5567,5583,2371,5271,-5079,-954,-2465,8212,-5585,-1663,-1532,4305,5257,-3922,-4026,-2878,9595,8317,3348,6445,-2880,6343,8432,-2338,3851,-6980,-8984,5754,-4449,-2929,7828,7044,-4543,-152,-4487,2219,3641,-8436,-7193,-6442,-9263,-9156,8302,1208,7571,-5351,9157,-7079,-6763,2554,-7326,1315,8064,8133,-1987,8274,3469,-1725,-5923,-3841,-7683,274,1958,-5663,-5096,-3429,-6070,3481,-6244,-2835,-7550,-2062,-5231,-1893,6863,-876,120,4805,-5371,-7241,4469,-4915,-5481,5621,8303,-3022,-5833,2329,4437,7808,-9969,-1938,6740,3664,6412,-4277,8559,-9930,-1078,5474,-6315,7565,1094,-2345,-7460,3765,-9978,1730,2996,4856,-8777,3380,-2983,-3421,8799,8118,-8133,801,3951,7366,-1099,-2419,9450,8443,-865,-883,-9804,9203,8865,6962,-2781,8504,-7920,9058,-43,276,-77,-8186,-8169,-8095,2844,8019,2483,3586,5415,662,-2291,354,-390,-2083,-1750,-3142,5703,1557,7681,7206,9952,-6237,1801,4712,15,-4936,-8474,711,8973,385,3717,9471,-2630,-6343,5611,9738,-5952,-4110,4683,-1056,-3134,-5077,1558,-4326,-7580,5314,-9551,-9231,-5873,7917,-3622,-2093,8720,-677,5410,-7196,-921,-3093,8274,-775,4766,-4304,-3951,-6822,9092,152,-3004,-5838,8601,-9231,-8574,-7777,846,-6018,7144,3693,9736,-8923,-2923,6975,3605,-7988,-2461,4357,-585,957,5625,7370,-7466,932,3782,7449,657,658,-962,3253,-6674,-7791,7091,-8832,-5375,-1998,-622,9075,-3600,8234,3461,1331,7677,-8161,2218,-7547,1229,-9753,-1327,5362,-9431,-7189,3430,-477,-6356,6045,-9922,-464,-2876,-8665,-5165,-2822,6493,8738,8744,3684,-9150,-7615,9104,1771,-5289,6334,7712,-8306,-9905,-607,2922,9017,8496,5989,7595,-8461,6110,-9072,-3206,-4227,503,-9887,3850,2181,-2423,7938,5178,8770,-1774,4785,9403,-148,5632,-5731,7245,-9326,7015,-7632,-5090,-9502,-1287,-3417,-2128,2735,-1379,-2392,7511,-7172,-47,-8062,-8733,6381,-4714,-221,3439,3845,-7252,7540,-2101,8921,1529,-4407,7323,-7752,1021,5354,3480,4377,8768,-5842,-264,-6072,-765,-9579,8164,-5228,-1294,-6115,4281,-580,-4460,8636,-4617,-1538,8823,-6514,-3730,4571,3042,4451,-9354,-6449,-5655,-6523,9983,3657,5558,-850,2438,1285,-6460,-8696,3733,-8144,-7512,3158,5087,-8868,-4319,9236,125,6856,3732,3773,-9254,-3970,1818,-3420,9956,2491,8184,9373,163,2395,-2813,3953,-3638,2571,1633,-9588,-1768,-2011,-9154,-93,822,1020,5304,3067,5169,-156,1855,-2249,-1247,4714,3731,2256,6225,3421,9375,1845,-4982,2765,-4348,8783,-8508,-2553,5822,-5617,-5292,8553,2648,-7258,-2371,7280,-5458,-1575,-6984,-9551,8033,-9104,-75,6858,-2590,5557,2906,-9488,3682,-805,7141,114,4580,-80,-3823,-25,2756,1057,-5690,9186,2473,-1048,2104,5923,74,8498,1659,3187,-3878,623,2788,2393,-63,-4683,4800,-7942,8806,-8289,-1273,9900,7905,-4938,7891,-6220,7796,-6595,1751,212,-6628,7833,9528,-3696,-564,-1883,-7964,-2157,2660,7948,-5812,-6583,-4601,5799,4600,-8153,-7674,-9687,-2379,9401,-3946,3859,9809,9354,5914,-4567,-4029,-5284,-122,5172,-5483,4042,-9757,966,-3375,9599,-7992,-2131,-3657,-3240,3029,-52,4966,9472,8062,7445,220,-2984,-2196,6473,1070,-735,7804,-7008,1103,5134,-5884,-4726,-1485,-5525,-2668,8874,7277,6783,-5344,-4179,-2419,-4016,4935,5681,-4186,-4493,895,4655,-6292,5188,-6877,5981,-5634,-8801,-2261,-3326,8657,-133,-255,3695,6334,5256,4100,7616,-8504,-8739,1573,8447,-2598,-3982,6400,439,-8798,7020,3551,-5911,-4512,465,6947,-4037,8206,9498,9371,2402,-3548,-5301,-3106,3096,456,2279,6213,5050,-2995,-4682,8421,-2911,1663,2578,9069,-5191,4843,3214,-1679,-6153,6283,-8547,3989,8632,-4266,4650,-8932,-9206,4693,-6422,655,-371,-730,2888,587,6762,2973,3065,-9679,-3941,-7327,-4474,-1137,-2303,9017,8471,5191,1150,-2595,9629,563,6409,-4923,9728,-7839,-2278,1330,7632,-1919,1848,5325,-7007,-4530,-2785,-4068,-2553,-197,9579,8593,9054,4881,5561,838,-517,5784,2692,-620,-2181,-3196,1902,-2403,-5038,7072,801,-3657,-8722,-4784,-9991,-5205,3805,-5055,-8054,-7193,2886,3168,820,2522,655,-7178,-5731,-9826,-1162,524,-9374,-3186,5465,7881,-6156,-6375,-3685,9358,6189,3181,7586,-2408,-7877,3706,-2863,-2799,2707,2020,-5232,1258,9305,3748,1542,-4512,-1040,-1762,-1868,5534,-4768,-5861,-5033,9915,23,7118,3589,-2484,-546,1616,-2510,-7000,-9421,-116,622,-7226,6371,9974,-8831,3712,-4106,-4325,-3215,-7738,12,-4619,-762,4949,1366,-7209,-2647,9774,1895,-9352,-1433,-5203,-6639,1231,-4436,-7041,-294,2525,8996,5716,7724,-9582,-1677,-3563,-9160,-8596,-9635,-7820,1521,-5040,-5798,-8450,-6176,6861,-4520,3192,6819,5020,8889,3140,2976,-2753,2148,8382,-7084,7115,-2121,-2170,-196,-732,-4238,-3119,1303,-3067,-3608,-6131,-6950,9206,526,6221,786,4602,6707,-7962,-3149,-8868,-6339,-294,-119,-3804,-1803,7108,8302,-8268,3333,8704,7387,441,1771,-9527,-6550,-85,5534,5389,-1788,-5147,-3871,4907,-2572,5804,-4182,9563,1747,-2333,9416,3172,-8941,7507,-294,-7626,3824,6416,-4833,-7930,-1372,-2883,9601,2479,2027,-2741,1272,6732,-7302,2273,-5439,-854,-8110,-1164,-4358,-7331,3206,-3804,401,9606,-316,-7120,-8498,529,-9973,5662,1176,-312,-1923,-2138,1546,3406,6379,8996,1710,-6825,-4314,8411,5287,-1004,-9375,8477,2970,-9543,4852,-5203,-2344,-2003,3079,-5345,-7392,-4703,7036,-9304,8513,-5623,-3331,-6823,-8024,4896,-2879,-8240,5568,-3154,6208,-207,8050,-3603,9576,-5053,3876,-81,-2256,-8036,-7168,6542,-4491,3343,-9982,6770,-4951,-9946,-6530,1421,-237,-3663,-8503,-2089,7461,-3188,1522,-2511,-2109,3898,3009,-8081,1009,-1356,5646,747,6589,-6079,2851,4074,9225,333,7739,7746,-5918,-7240,368,4095,8308,7824,-1980,-4406,1320,1602,5018,1932,-4543,-5913,9594,8885,9285,2203,9875,-671,4061,-5742,-6490,-6485,-3839,1691,2518,5142,1647,-1215,-718,6544,-5152,-1682,-7483,6496,-745,7395,1817,1305,-3883,-5579,742,-422,4911,-264,6686,-2949,7180,1524,6590,-7081,3872,362,-8865,-2963,-3072,-620,2485,-3469,5145,7108,3237,-9167,-7485,2259,8783,2782,5607,-6754,-9667,2043,2097,801,9427,-7771,9146,3901,3324,6888,-9958,-4363,2787,9352,9111,-1088,5512,-52,-1764,3582,5187,9551,-674,-6021,-165,7137,8317,9963,-9987,-3153,2492,-5509,2614,3424,-2998,-8172,5021,660,-5345,3778,-2270,2334,-8302,7675,7601,4014,7640,9933,-8365,2284,6978,-4770,1857,-1559,3175,7566,-4281,-3495,-583,6275,8089,2596,-6351,3447,-9932,-1086,1368,-1061,5777,-7417,-9975,7000,892,-8181,-1693,3500,3586,-5812,-9871,5662,1541,4845,109,-476,9360,-4297,-4508,-7481,-5768,9445,-3058,6477,-8352,51,9841,8209,-1628,7950,2066,-2267,7842,5614,-7593,582,-7059,2964,-3551,-3572,7644,6105,1148,3885,7754,6266,-3031,-6881,9862,-8373,-3234,865,2309,-4247,-6460,7770,383,-120,496,4742,-8984,9539,7794,3520,-8301,-9970,6428,-1075,-7157,-8092,7732,-3924,7396,-174,7072,-6167,-9721,6591,7614,5330,-6064,-6990,6649,4856,-2346,6686,-3093,-2853,-8724,-5697,-5790,8155,-2394,1804,-5001,8774,-6641,-3165,7510,7305,-3394,-5857,-4160,7296,5959,5117,-6196,-3843,-6502,-2677,-4204,-9048,7350,7834,6413,4086,-1411,9516,-5457,-6675,7381,-7340,-4280,-74,2893,-1870,2231,5925,-8287,2454,4814,891,2325,-959,-6588,7019,-3861,-8550,-6198,-7575,-2026,-7683,9280,-354,-274,2869,-8038,-9005,5476,1495,1552,4930,5804,9923,8191,-6825,-844,-2619,-5529,6053,4645,-8813,3885,-6732,-3025,676,3336,-5857,-5045,4471,6503,4593,-3950,3832,9897,-5592,-3911,2702,-4270,1826,-6368,4399,-7449,-2053,-6244,3520,7935,5775,-2995,-8420,-5000,-5896,2324,-328,-8043,7075,-4790,-1820,-8220,-1699,9880,-5301,3913,-2331,-855,4892,479,-4943,330,-9614,4599,9267,2816,-4593,-2812,1646,-6391,5692,4849,9070,-5145,5316,-7503,-6462,4791,2446,-1175,2907,-8101,9527,8766,-6849,1648,-6217,7709,-9554,7897,-1511,5121,772,1607,-2860,2914,587,4171,7732,9214,7644,6532,6828,-1552,1149,-9127,5334,5235,-4401,-8326,-1922,960,-1682,-9892,-8,-9190,-6537,-7410,-2048,-8934,5094,1856,7450,-5067,2693,862,577,5798,-515,1780,-2671,2444,6938,-8160,5675,-3664,-764,-7172,-3553,1158,9749,5696,3767,7204,-8650,-79,7899,-3222,7005,-3449,5416,-4456,-6689,4445,-3314,1612,6640,3064,4821,-4643,-4059,-7782,-2344,6057,7087,6322,5557,8752,-474,9214,-8448,5356,3602,7953,4972,1618,-9802,4238,5328,-4207,-7618,8121,9256,1054,-1163,-7702,8710,4165,212,-6218,4799,2376,-106,-3046,-3087,7737,-3978,2359,-5283,-830,-9572,7339,-6820,-9292,8891,7733,4145,-6048,4900,1649,5378,-6292,-173,6693,-5380,1950,3019,-7208,-5895,6238,-4613,8602,-3916,-4407,1686,8284,281,-9813,-892,-3395,-5363,-5771,-7418,4592,-2641,4345,10,-5305,-4641,-8888,-4438,1190,5467,-6578,-9493,3447,-613,-5052,5970,215,3485,-3051,-9313,3463,-2153,2965,3294,4450,8586,8754,857,-4920,-2175,-3939,7209,2767,4761,-5642,2887,-8739,-8870,4895,2724,-5861,4968,8389,8007,4799,-9408,4602,-8777,4818,-3203,9018,7073,6194,355,-361,7578,-7937,-7719,-3574,-6310,-4808,5071,8008,8729,6006,-8048,-9368,3262,-496,5312,-5057,-1835,1344,3788,3349,7432,2609,-5202,4044,3241,-8130,-4991,-5842,3115,-9663,4810,-3579,-523,-4060,271,-1484,7782,4915,2361,-5531,6337,-2647,744,5371,3249,-4819,1589,6779,-813,-7162,-6641,1452,-4872,8336,6009,-999,-6055,4948,6115,9284,3051,2498,-7968,3967,-9644,5215,-1094,-1707,5901,9781,-2520,2450,1040,4521,-2015,4926,-7153,7226,6080,-1741,-3068,2288,7813,1954,-2288,4727,7271,8481,3243,-5331,-1541,1498,-5026,6061,-8180,-8049,1975,318,3391,6785,3686,-7988,4491,7580,4949,8662,1799,-3208,-9163,6729,7100,-2905,-1510,-535,664,1123,3513,412,-7818,-9254,-5380,1407,5788,-1336,632,-6817,-418,-4615,5962,9082,5010,-532,6953,-8735,4818,7345,117,6736,-8840,-1858,-6385,-4165,8879,6796,-2734,2369,-5541,-5676,-1875,-2583,-1940,-1150,1676,-10,-7825,-449,-7207,265,-9021,9588,-1678,-5580,5987,-6139,-3551,-1497,-2626,5286,9143,-5278,-3289,7835,6579,3354,9639,-5896,1840,-191,-1637,-5687,-1194,5001,7186,-90,4491,-9948,-3771,2765,6861,-6045,-2183,-1560,8820,6278,-9015,-9898,1255,4988,-9251,-1859,-3745,4379,7203,3968,57,-361,1467,-297,9080,9000,8227,5036,3056,-9191,4255,-7303,5385,4822,-2168,3426,9171,-4895,-4470,-2782,-4182,2453,3078,-7753,5948,-238,-9512,9409,-1053,-175,1203,-87,-2015,7915,-6196,-2862,3665,212,4811,2080,7152,5142,-5029,-5111,5278,-5119,-1363,912,-6710,7035,-85,5011,8018,-8609,-5067,8715,-8695,5021,-9570,-2282,-7112,857,-8439,3206,6363,7382,-9405,688,-8326,-2279,-9469,-3233,-5302,9848,4123,-5281,3138,815,-7816,5024,-7130,-6597,9723,9122,2729,-5157,-2785,-4497,6851,-478,8079,1325,1719,5483,-109,7997,1635,-5787,-2317,5694,-1287,4768,1077,-3216,-1871,-1856,5163,7981,7322,-9681,1117,-9266,2859,-2318,5408,-4375,-4152,-2091,6,9524,-946,-6325,577,-5348,814,-9742,-2546,8233,4649,-9535,-2070,1150,1050,-2392,198,-52,-8535,-8695,-6707,5133,1258,-6786,-1654,8789,-7735,6812,-3049,361,5702,68,-4515,-4878,8878,4742,-4757,9198,6877,-3345,9480,-5644,-6529,1934,6711,6678,-5196,9594,-8299,7241,-7221,407,-4357,2241,-715,4882,4602,8682,9393,2547,9143,-5726,-6476,588,8812,2373,-7770,5149,-3784,6029,2034,-5708,-8920,1962,9271,4463,-5666,5014,6921,-7028,-8494,-3810,-7690,-8586,-3203,-5189,8065,5284,-9588,8124,-8645,-3819,-9474,9171,-2984,637,236,4966,1650,-9246,5193,5261,-3510,6627,7400,-8377,-4926,-1043,6500,5165,-8430,-8665,-8460,1752,-7366,4771,8525,-5004,-5294,8645,-5197,-9390,3091,4522,885,4120,5186,-4965,9916,-9838,1999,941,-9060,-7242,9744,-1895,-8670,4316,9488,784,-6794,-3677,-7589,9539,4541,-2490,-647,8582,-3926,-9997,-4473,-2573,6071,-5399,-7581,798,-8653,-7787,8376,5963,-4686,-54,5684,-9385,3943,-6279,-2730,-5449,9606,5533,-4435,-4872,202,-4729,3476,-1923,8855,-8423,4187,-4479,-8028,44,6427,-6554,-7583,-1391,5996,-2984,-480,3007,1322,-1322,6387,1692,8402,603,-7114,6988,4885,4427,3170,-5716,6757,2568,5020,145,-6693,-5830,-4242,3634,-3692,1759,7847,-9370,7876,-9617,-4635,-122,8718,-5031,-1103,-9490,3892,8499,2353,-9079,-9167,3727,-6805,-8449,-9248,-9088,-1698,3497,4336,-9120,-8322,8716,1714,-5248,1911,7591,4318,-4011,282,-5209,3623,4964,-4713,5352,8948,8160,6852,-2245,2292,7146,-42,6613,-6507,-9007,8993,-9725,-8405,7502,-2229,3835,-5064,-4266,-8412,-2194,-9919,1699,-2851,-196,5841,1124,5841,-1647,5778,7152,7827,-7118,3289,-1874,-8351,-7553,-4152,-1317,6509,695,-4515,6818,9180,-122,-4228,3914,4811,-9264,-2638,285,-8024,4137,-5303,5676,2146,-4738,306,-4421,4313,-5930,-8953,-3632,1857,-5177,-1944,540,9115,4618,3476,-5120,-3805,-2277,-6547,-1616,1217,-5011,4630,9886,9282,8397,3795,-4680,-2629,1419,-7814,-5340,5118,3721,-4279,-7239,6815,2675,5880,1819,4747,-5410,733,9811,-7845,4904,6766,-1085,430,-2807,-9499,3647,1351,8185,-8938,1046,-4836,5331,-8637,-4161,8320,641,2359,673,4769,-7579,6194,5158,3139,3158,-7945,1647,-6242,-3899,1035,1959,-8763,2139,-5335,6923,-1252,-67,9615,-2884,-8316,528,-5010,-5432,-4391,-9958,-222,-4420,46,-981,8738,9057,7309,3837,-3631,6683,-959,-9634,1649,-4054,-5547,-8229,-7609,5714,6939,4640,2756,-9392,-5298,-6541,-1494,7194,-3088,6390,-175,-7980,-2152,-644,1015,4699,9266,694,2136,-678,8316,9251,-6879,3176,-5447,1338,4576,-6261,-2303,-5989,6351,1345,-8972,-7901,4509,-9196,6937,1867,-5057,3244,8377,-6317,-163,1146,1950,-2682,2316,-7724,6700,-2754,-8871,1163,8619,9401,-2053,-5747,2745,-4466,-3436,4255,-5889,9902,5335,2386,5335,-4685,-3882,-9448,-3186,1562,5089,-7118,-376,-2227,-3676,2356,4418,-5449,-7926,8099,2438,9114,-2131,7046,-7057,1808,-4941,7369,9444,-9951,-5490,-1480,-7223,9609,1733,-5468,-5645,3254,-8608,-2774,-370,9206,3577,-1405,2077,1422,-2734,1064,-530,-4804,-5357,-7222,9846,1690,6693,3862,6134,3118,3660,-422,-3710,-3546,-4565,-9070,3878,8792,7249,-4166,8143,2048,-1399,1337,8772,9793,-7626,-2638,-3920,6283,4686,-2180,9881,-8622,4457,5745,-4686,9491,-1343,-6754,961,987,-3674,-5956,-1964,-9633,-5564,-3419,-1650,1708,-1217,3006,-7593,1007,-8893,4600,657,8653,-2794,1573,-922,-7266,-4688,6662,9769,-823,158,-2679,-6593,7322,2378,9950,-2980,-8042,253,-5344,1831,2546,1161,2195,6613,-3333,5139,-3762,-3899,-1035,-2858,62,-6622,2016,2751,8211,7932,-3291,6789,2441,695,-2918,-7375,-6329,-353,-7112,9927,8896,-6939,-6107,-3390,6326,-8433,8368,4308,-8427,6491,6427,-4664,-4553,1110,-2223,4697,4459,-6867,3493,-1958,531,-6376,-2990,961,2919,8674,-6953,-2766,-2171,9786,8927,9767,-899,-7860,-9658,9791,5454,8432,575,-7132,344,-2040,2486,491,-3465,-463,7808,-2705,-7849,-2497,6075,1494,-6443,5850,-8772,5681,8865,-2038,2554,6361,2987,-1191,6675,-7878,1925,9461,-6449,1876,228,1249,9673,2507,4674,6581,-824,-9521,-5404,-9615,6090,8946,2145,2593,-125,-826,-2200,7076,566,1633,3510,8545,-4472,-5832,3815,6387,2374,-847,-7186,7052,3234,9634,9765,-8657,5730,4125,8488,4375,6733,9288,-3771,-1264,9271,-7115,-9573,377,-4785,-4378,-8426,-8868,6198,-4820,-9903,-2645,-5306,5959,7895,-5666,-8224,6949,8097,-4,8338,-1525,-9875,9594,-1297,-4931,7658,-1013,9726,2965,-658,3774,2507,-8315,-9690,9741,3053,-7783,6210,3690,4243,-7757,-7154,3846,-401,1879,-2380,-5206,1593,-1753,4587,438,-1133,-4553,907,9757,-7663,-8083,5474,7053,-3056,1433,-9929,-4214,437,110,-8376,-1964,-1427,3773,-8380,-9328,-3219,2338,2550,1158,-6278,8321,5376,9929,9664,902,-8328,-8700,7353,5033,-1727,-18,-8544,1735,-793,-459,588,795,3621,6355,-7454,3777,-5808,432,3412,-578,-5122,-6617,7825,7671,-5137,3986,1278,-6124,-1310,-4698,2186,8423,-4508,-251,-8055,-1517,4876,-9964,-7091,-7615,3907,-4108,-6193,6395,9991,6305,-3212,7057,2010,1588,-3103,617,-1882,-6558,9039,-5715,5409,-9774,6081,-7050,4207,-8228,-4034,1316,1811,-1070,-256,9673,2795,-4648,7120,3362,-8169,2274,8169,-2998,-8218,-8889,2314,3161,3257,6920,4862,-4594,9440,-423,2732,-1405,4353,8886,-2308,6192,6566,-6458,326,9675,4026,-6891,-4256,-5467,5504,7338,1385,1520,260,-3303,-8227,-9219,-6539,-769,1676,-4339,1903,-7695,-8463,1466,623,-6583,1005,-9649,1662,5974,-8844,2965,-3565,5884,3533,-2670,8656,6999,-145,8129,3539,9746,-3423,-2888,647,-7172,1444,-221,-3892,-6440,7836,-6560,-8831,-1454,-5120,-7006,-1191,-9852,-1984,-5424,-7965,1375,-2951,3466,3265,-7356,-7327,-9874,8953,-9952,-1148,-5113,-9170,-3765,199,-6785,5659,8733,4387,-3286,-4198,5858,9140,841,1115,3992,-8667,9161,-3270,2621,8900,402,3410,-8315,1713,395,7367,9366,-7143,6703,-1077,-9691,8986,-2271,4536,5260,-6808,-7003,-7782,475,-5325,660,1193,-4599,2905,7016,-8586,-6506,9290,7262,-6426,-8161,-8810,6592,9780,-4055,7055,4134,176,2601,-5065,8240,-7433,-6192,-7688,-6129,9261,-4073,-7515,-2870,-6866,-5832,5409,6330,-9993,-1781,-5834,-2997,-6680,-3486,8533,4630,-1411,961,-8014,2510,5926,5659,-2242,-9556,7865,4591,-8520,-7009,2230,334,-2057,-4737,-5149,4271,-1854,-6104,4857,-1907,-1519,6592,-2445,-1443,8984,-2519,5693,-9011,-6257,-6163,-4763,3185,1071,-1697,1841,5161,3894,-4690,2854,-4635,-8426,7495,-2467,6440,7083,-3194,1966,-9992,2201,6210,2009,7231,8085,-8636,-8707,7456,-4370,-8162,410,-6913,-229,-6382,-183,-4423,6545,-4798,9993,5415,9372,-315,-7184,-6846,-702,2913,8589,-1135,-6783,8777,-2059,8105,3855,-1822,-956,6736,-3512,1593,-5043,-1074,-8969,-6063,-98,-739,-7445,9554,1006,-8668,2954,2604,-1568,1504,-2463,-7982,-8598,-5585,5617,-8759,-5518,3884,-8058,-8971,-2853,2685,6172,-8999,5766,9512,-9250,5516,8481,7925,-5392,-6838,-5062,4393,-8825,8137,-1240,-4248,-9284,9129,-8637,-5105,6666,5509,6887,-5344,-1410,7534,-1530,-2754,7239,-7869,1789,-1234,6908,4722,-8160,-8205,-5931,-1306,-2194,123,-4763,6249,-8850,3776,-1005,-5790,2309,-6173,505,-3800,-3729,6750,-6206,-3834,-381,-6045,-9722,-4315,1403,-2718,-6413,7836,6867,-7658,-809,2153,-9,-7077,9431,-3257,198,-3003,1895,9968,-2939,-9738,5792,7594,-6682,-3352,9846,-5750,4211,9200,5659,-9798,-1032,-2881,3735,-5839,-9475,-4180,-3343,1656,-6236,2901,2837,-9375,-5447,-7065,4972,-1813,-3720,-7882,1145,3522,6742,-9779,-9172,-2128,-2043,8476,632,4324,-701,3916,3791,9970,-2570,8925,-7944,9801,8750,-158,9615,-5239,-1210,-88,-3450,-2363,-4613,6613,2210,-5014,5326,-7218,-7248,-9640,-8661,-1005,-8359,4113,8654,-2676,7769,-3003,-102,4612,6958,2809,2875,507,-8571,6150,-5782,4060,3395,2007,1914,-2673,-565,-7352,-7076,-5862,-7191,9209,-2355,-6007,6378,-6806,4748,-6190,6334,-7274,-1858,374,5521,-4921,7887,-8562,-3967,6817,-6007,-9665,3624,3658,-5917,-1664,-5875,6726,6068,261,-9738,4180,-694,-9164,990,-5338,7361,2147,6341,9427,-849,6876,-8549,-69,6529,1161,-3062,919,271,8253,8191,49,-336,-8940,7461,6995,2480,-7139,-9923,-3201,8640,9061,-5921,1529,7747,-8665,-6187,1361,1756,-5373,7523,4922,4106,1823,-353,-2480,5874,-3342,-7102,1662,-9152,6347,-9169,-5321,-2035,-7821,8287,3586,-339,904,-3490,-4233,-8540,-7671,-6601,-3847,9229,-238,-6346,-9714,-4693,3891,-2916,8749,-87,-3426,5414,9324,-5266,6676,-2774,-6092,6951,7968,2272,-2465,4888,2988,2108,-7791,794,685,4693,-2323,8896,5884,-1242,-7585,-6696,4272,-199,-4033,3270,-8782,4722,5090,-7724,-1134,8467,8880,-2898,2038,2507,1563,-9642,-2571,-3078,3982,3584,7023,1394,2305,9763,-4863,-3063,-538,4514,-9268,-3276,9025,9113,-9733,-140,7913,-3116,-3634,6376,3068,3005,5769,4079,-3489,6007,-3551,-9834,-525,-953,4237,3468,3659,3855,3841,6276,959,9371,-6684,502,7566,-7170,3982,6715,-3652,-9067,3048,3228,-1266,-2721,-262,5543,4484,5440,7509,-9714,-4996,7041,1995,6198,6579,-7496,-8977,9554,-2698,-5196,1347,5165,-6074,-8362,5984,9791,-8526,1499,1555,4389,-7836,-8845,-3703,-243,-2175,9729,-7466,9217,3089,9169,-2712,7668,8170,3227,-9301,8019,8473,4293,-5845,-3411,-8734,-7299,4137,-9006,2209,7730,-1632,-7307,-7129,7621,8757,-7124,4427,-6580,4235,-3196,44,-7426,3728,6234,115,791,4128,1547,44,338,3468,-9311,1784,-5083,6102,-2828,-830,-6747,8685,-5799,4754,8722,3298,6953,4724,4492,7622,119,-2269,-6025,5615,-3266,520,-4811,8074,-2028,-3002,-877,-1590,-5990,-6802,29,9022,-8104,7713,-4572,-7686,-800,7924,4454,5226,1256,-7889,-4066,-375,-5646,-8938,-6424,8726,6223,-1166,-8369,-7710,3203,855,-910,8040,-657,8726,-7563,-6649,-4555,9204,829,9645,5696,3656,-8541,-5699,9298,1923,1934,6376,5078,-4140,7559,-9710,9404,-5580,3113,7370,7575,-3174,-6673,8967,-8053,-462,-8874,-683,5852,798,2126,7207,8131,1462,-1865,3700,1572,-5974,-8302,4162,9396,2136,-4244,4425,-8655,-80,-6028,-9359,6134,-6497,-7600,4554,2202,5018,3162,-3961,-8781,-2269,9831,9918,8660,-5734,-9187,8569,-73,9227,-2047,2539,-9573,-1845,5502,8355,4884,-877,-4285,4925,-3511,1307,-4091,-8910,-2795,-7027,-6037,-4073,1165,6525,2472,6062,-7717,5012,2271,-7265,-8329,3261,-2431,1219,-1260,6707,-9644,-2605,2683,2754,-483,-709,1898,-1075,5999,5884,3723,8767,5846,3118,7220,3272,7515,-8693,-3190,2280,-236,2477,-4755,7723,8358,-8383,-7806,2273,9090,6413,5855,1268,8222,7652,-8522,446,1516,9635,-7379,-2421,3606,-2185,4626,9810,-6160,-3011,3946,9608,-9975,563,7098,7788,-6251,8081,-5928,-1822,482,-7980,754,2468,2727,6997,4031,7255,-3228,-7378,-4322,-9303,4214,2729,5730,2313,4123,-1724,8916,8954,9340,6718,5920,-647,-1636,3661,5711,-3629,-633,4739,-5544,-5530,-3396,8834,3516,-3771,-2799,-9505,-1036,0,-1191,6847,-7625,8533,8699,4153,-4893,-764,60,8012,6324,6435,7264,-2730,1691,8846,8004,-4528,-5753,-8153,-4792,6589,-8381,129,-1036,7870,-4794,6482,49,1167,-6772,22,2480,-660,2410,-5833,-4885,4678,9282,-1121,-9793,-7553,2222,-642,-7267,-4388,-6131,5374,2380,-9279,528,5295,-5047,-9653,-4393,9124,-3694,4245,95,-1948,-1917,-5053,-8458,-9913,-4139,7892,-3337,-2531,7372,-2674,5439,6865,-386,-6219,8572,-3857,-7187,-7239,466,100,-9076,7076,-3512,-7089,-4438,9476,-5254,4828,5912,-1469,-1677,240,7997,8626,-4815,6344,-7307,136,-8859,1853,4742,-7784,4213,-1193,-8906,-5495,2260,3966,-5403,8377,-642,-5001,4548,8339,6690,-3842,4451,2900,1831,-6299,-5632,-9641,3392,-3609,-4003,-7473,7697,5770,-1142,-1497,7600,-562,-7347,1384,-7271,6500,-9569,9052,6537,-6091,892,3215,-7421,6991,-3562,8480,6433,9805,-4211,3548,-4397,4458,6172,1427,-9971,6002,-9288,-6593,-8338,5807,-3978,-3567,-8363,5870,2224,-9623,5952,-2900,7290,-1764,-4371,-4801,-8640,1184,-9476,3200,-4911,4877,-3266,-8700,4387,-2988,744,872,2680,5227,4057,-1539,-4741,-3196,-1310,-4171,-5262,-9419,8947,3034,-8383,-1718,5884,1387,-3121,3752,3469,-7945,-8593,8084,-9096,-6098,-28,-4003,3154,8045,9671,404,4117,9964,-6396,527,-9540,-4607,3136,-1541,5459,8447,-4582,-3504,9616,-1171,136,1942,5709,-6606,-761,444,-555,-3840,1455,-9746,-3601,-9693,-3175,-8428,-6000,-9495,9330,-1164,1029,-5594,-8042,1678,3690,7070,2839,-5040,9951,-8745,-833,469,5280,-5938,-6711,-9370,1129,-9496,386,-7251,-605,-3584,3020,-9368,2690,-523,5727,-7116,-2122,6579,-136,-6988,-5658,-5671,7927,-7920,-7641,-6670,3386,5310,7384,-9150,8864,-9766,7536,-4564,254,-7180,-516,-4760,3927,-7790,-4193,959,-4099,4298,7241,6923,-7837,-5843,-3018,-4424,-2630,-5756,6136,-3921,-2538,3090,-8326,-8351,-6299,-2796,-4321,8409,3315,7223,1461,-9101,-230,-9090,6080,8943,9601,2014,4346,-1710,5595,5063,-8982,-2665,7031,-2682,-2004,485,311,-1140,1911,-4407,634,-1082,-2588,2559,-6789,6915,9323,-888,-1120,-5628,6886,4437,2928,-2639,708,4786,6838,1254,-113,-8848,-5992,-3037,-9143,-9098,6109,-5568,499,-4457,-6156,-9805,-386,7634,6228,6498,5483,-3393,-3091,-5467,-9662,9283,6215,-8512,-2454,-7445,2103,1958,3576,4627,-1574,1920,1557,157,-498,4381,3458,8802,6922,4602,1775,-9753,-8796,-155,6836,4319,2154,1501,-5126,-5690,182,657,-6074,-7711,4697,-6921,-980,9464,-4056,5067,-1531,-2185,-9372,6547,983,5950,-4399,-3017,6933,-8744,-6148,5128,4016,-7248,5294,9141,-8137,-497,2844,4584,-8866,-5522,-2042,6334,-6470,-839,2555,-4438,4862,9909,3767,9656,-9895,3846,-7770,5551,6021,-1978,9637,5373,-641,-3270,-7403,-5630,-7534,1590,-3976,-8000,-5978,-1469,5162,736,-1191,-9910,3990,6466,-3025,8684,-6433,840,5747,557,-1163,2259,9234,-5853,-7284,-7472,-9328,6187,-6356,-9458,4683,4505,2349,8918,9852,-3341,-5073,3689,-7501,-556,4857,3439,2687,-48,6150,-3290,-5732,4465,3714,-5064,4443,36,-8950,2199,611,-1479,-6682,-8574,8235,3786,9332,5303,-8411,-8815,-8453,6023,2910,5003,-7104,-9813,2511,-8764,3834,-8251,-5790,-7780,3843,7736,-9023,3883,6339,7672,-7576,9873,-2253,-5801,-1866,3563,8066,935,7938,-670,-4156,5471,5034,1398,-8856,-7919,5479,-8937,207,-7174,2254,-9318,9138,4167,-8328,7251,-5751,7643,-8097,-4801,1960,9438,-2939,-1025,-3839,671,453,8933,2411,-7964,-78,1878,-5352,5011,-4191,-119,7483,-9607,8538,6833,-6067,-1857,3954,7469,-8049,-9392,-9872,6098,-6653,3996,2242,-1631,-4585,6540,-5654,4369,8370,1447,3584,-7712,6340,-1690,2524,2313,1358,3714,387,1242,-3736,593,-6529,377,-8326,4198,6627,743,5190,-2131,-4871,-8671,8487,-1502,-9741,-5394,-5331,3406,8521,6292,257,-2518,-2432,5147,996,9101,327,4000,6781,-6706,-9857,9397,-3436,-9268,-111,2212,406,8353,-8355,4414,-8950,-85,-6867,1866,-4414,816,3884,-1667,6187,-7083,3750,-5000,5788,-9538,-505,9177,5648,-8091,-3099,-9762,-2304,211,-7725,7438,6718,-7118,-2707,8365,7709,-2791,1354,-2977,-9360,9634,6284,330,-6729,-9673,6129,-7405,21,240,-9556,-6512,-8633,4046,-5727,-5971,2350,-54,-8775,2643,2136,-6820,6361,-2097,6051,-1476,-2811,2000,-4442,3224,9784,6863,-4372,-6159,-199,3532,2739,8579,2913,-6286,-7404,-6212,5065,2340,7166,6162,-5043,569,360,-7030,-1143,6133,-3985,-2243,7279,6761,1921,9549,5839,638,3445,7718,4280,-4015,-7335,-3209,-1441,-997,262,4256,9365,-668,8390,660,9842,4221,2449,5932,9985,5681]],[8166],[1064],[8508],[6255],[1605],[-2106],[-3534],[4018],[9220],[5121],[613],[-5503],[543],[4440],[9140],[-6495],[9029],[-3536],[-2242],[1393],[7793],[4225],[-1181],[-1161],[-372],[5665],[3407],[-8827],[-1299],[241],[793],[-5001],[4923],[4020],[6537],[-7711],[260],[1301],[-7252],[-3866],[-3865],[9201],[8442],[4662],[2432],[-7055],[-750],[-5089],[-129],[-9148],[-1476],[7098],[3646],[-8668],[-1741],[-6016],[-4131],[-2320],[-4543],[1018],[-7365],[7914],[4915],[7594],[-4997],[-330],[3948],[-6611],[8601],[-7092],[-9964],[4283],[-5156],[9124],[-744],[4596],[-7322],[6075],[3443],[1672],[-2428],[790],[-6368],[-3068],[-6946],[-4902],[1951],[7109],[-6488],[843],[8872],[75],[2448],[8804],[3924],[5859],[6686],[-6585],[-1749],[-9378],[4803],[-258],[-19],[5012],[-8904],[-8905],[2298],[-5292],[7873],[-755],[8218],[-9564],[-5025],[7323],[-4934],[7880],[-3547],[1477],[-6833],[-7526],[-7233],[1175],[-2314],[-2840],[-4516],[1842],[-8536],[4574],[-5905],[-1872],[-7474],[-9153],[4497],[6380],[-6716],[-7831],[-6277],[256],[2515],[6042],[8056],[-9053],[1301],[913],[-8816],[-3162],[9288],[3367],[-59],[-1370],[1985],[1243],[-5848],[-3403],[-5471],[8135],[4518],[-5000],[-5808],[2333],[5385],[1637],[1252],[6699],[-5738],[241],[-7315],[6237],[4459],[-7562],[9918],[2326],[-3652],[555],[-2018],[8096],[6243],[-2376],[1849],[-9843],[9017],[-7834],[-7520],[-7250],[4210],[2436],[-768],[1327],[1070],[-3890],[-1226],[6679],[1300],[1112],[-9816],[-4133],[-8229],[8472],[-6328],[-7276],[9875],[-9531],[9866],[-4845],[-765],[8891],[2258],[2326],[-7246],[1116],[-4068],[9688],[800],[-6422],[-6854],[7135],[3517],[-1806],[5490],[1340],[-4241],[5758],[-6475],[-3809],[-3643],[-6694],[-7610],[-6877],[-3970],[-9229],[7639],[3952],[9931],[-4162],[788],[2948],[1885],[853],[2284],[3741],[4577],[-5905],[4349],[5919],[8437],[-1475],[9651],[6234],[-81],[-1095],[-700],[8102],[2808],[-5954],[4098],[2825],[-3630],[7949],[4121],[9406],[-5426],[-9863],[7103],[7803],[6022],[-115],[9083],[2631],[1739],[-3217],[6258],[7277],[-5664],[-1489],[4551],[-6394],[8594],[-5372],[5841],[542],[-6076],[-5081],[2851],[-2947],[-736],[9841],[7585],[-2342],[-1909],[-5735],[-3343],[7750],[-3670],[2272],[7968],[-3045],[-8229],[-7269],[-8542],[2101],[-2601],[9575],[2148],[3646],[4703],[-3749],[-8888],[8694],[-9693],[19],[-7623],[-4350],[-7128],[-1813],[-613],[-5244],[-5875],[5942],[3876],[8159],[-5977],[-1280],[6357],[-7530],[887],[845],[1232],[-2043],[-4218],[561],[-8373],[-6426],[-4322],[-4061],[-3003],[-8776],[4214],[1693],[-4387],[4453],[-9179],[5983],[-7971],[240],[7355],[-9743],[8354],[9679],[-9763],[3327],[-1294],[-7309],[9921],[-7072],[-973],[-8763],[9495],[-695],[-9228],[-6230],[7884],[-9856],[5666],[-3354],[-6681],[4040],[7426],[-2796],[2781],[8816],[9290],[-8561],[-3620],[-6307],[7215],[-5443],[8116],[-7230],[3313],[-9550],[308],[9080],[-1475],[-3830],[-2672],[3796],[-3654],[-3166],[6412],[-9577],[-2519],[-8868],[249],[8082],[51],[1368],[-3247],[4401],[-5114],[-2049],[482],[457],[-6435],[2347],[-1300],[1864],[-7453],[1727],[-1363],[-2773],[-1091],[-5959],[-703],[-1789],[241],[-4508],[-9657],[9060],[-3307],[-7015],[8418],[-6134],[-1623],[8144],[-6655],[5579],[370],[5979],[4608],[4487],[-6133],[-651],[4715],[6952],[-6809],[-3674],[-1606],[-6883],[4816],[-4825],[7192],[-7405],[-5805],[-9200],[294],[3265],[1333],[6345],[-3235],[-4010],[9129],[558],[331],[-3432],[-3555],[3961],[-1981],[1796],[-3070],[-8093],[-5280],[-5563],[-2742],[-753],[-1521],[-2536],[-9763],[-6425],[2797],[-2733],[8],[8550],[5062],[-8488],[-4118],[4816],[-4762],[-9291],[-7635],[-4277],[2214],[8467],[1395],[7421],[1248],[-5222],[-8928],[-5450],[-5884],[4995],[-7217],[-6036],[2782],[8214],[-5789],[4417],[-5254],[-1379],[-5086],[6580],[8739],[7248],[-8565],[5310],[-2175],[9253],[-3098],[2579],[8427],[-154],[-7202],[4664],[-7500],[1565],[-9142],[6167],[-2641],[5721],[1309],[-5400],[3841],[-6030],[2098],[8033],[-9857],[8288],[1717],[-3841],[8677],[-4140],[-3960],[742],[-380],[-5509],[-1715],[1590],[-5848],[-1394],[6328],[8282],[-5121],[-3489],[-8501],[-2879],[-6782],[-5498],[-6867],[-9597],[-7980],[-7272],[7579],[8340],[1762],[1328],[1533],[2665],[8785],[5056],[9483],[-1788],[8429],[-7175],[-9137],[7462],[-5102],[-6821],[4490],[7645],[-6657],[7581],[3613],[1187],[5047],[7254],[-4122],[-8637],[-7853],[-1428],[-9749],[1755],[8410],[-42],[-2227],[6895],[7049],[7221],[3651],[5292],[3319],[2427],[7625],[-3568],[483],[-9137],[6798],[-7270],[6697],[-4490],[4252],[6606],[-5478],[9799],[5963],[5518],[3652],[-5892],[8674],[4205],[1524],[-5166],[-6105],[-797],[7747],[-8865],[7260],[-5955],[-1823],[-1698],[9345],[-8849],[713],[-694],[-8355],[-7858],[9812],[-7978],[9862],[732],[-769],[8272],[-7086],[-9484],[-4583],[-5485],[-9870],[6963],[7391],[-2095],[-4960],[6966],[-393],[1207],[9607],[-3778],[-618],[-7395],[3474],[1217],[3408],[-9955],[5404],[8142],[2844],[6225],[-5315],[2811],[4041],[3980],[6827],[-8648],[5099],[9165],[-6018],[-7775],[9184],[3889],[9911],[-7412],[4861],[83],[-8313],[-9954],[6172],[2382],[4848],[-6771],[6316],[-6563],[2182],[7454],[3906],[-3083],[8834],[9077],[-4240],[3863],[-5219],[-3547],[9705],[4674],[-502],[3384],[2670],[-1178],[1498],[-7239],[-1593],[9900],[5449],[-8615],[-6288],[-6577],[2014],[-5211],[-9519],[-4369],[7067],[-5301],[-9524],[-4247],[-2498],[6452],[5620],[4059],[993],[-7552],[-5140],[-4917],[2338],[2044],[-5490],[3227],[-4363],[-7731],[7198],[-4133],[3616],[-8107],[-3683],[4178],[9559],[6747],[3911],[5312],[-5044],[6344],[8022],[-9200],[3725],[7175],[7670],[1845],[-8979],[-5715],[-6051],[-8014],[-5292],[-1051],[-1700],[-9327],[6331],[-8413],[-4029],[9086],[6250],[998],[-4386],[3987],[-6460],[8180],[-7824],[-7418],[2887],[5771],[-5241],[-1208],[-6045],[6220],[-6313],[2012],[-9634],[8884],[-4377],[7825],[7118],[-9521],[1050],[-1973],[5884],[-7594],[-4015],[4340],[908],[-1589],[4248],[7635],[1859],[9151],[-3180],[2568],[-1687],[-394],[-6759],[9407],[3568],[-2347],[8188],[-6563],[861],[-3962],[-3399],[3771],[4835],[3672],[4162],[-4373],[-5501],[433],[4131],[-4560],[-2090],[2206],[-5535],[-9099],[2967],[-4288],[-6976],[-4985],[-7939],[6840],[-7294],[8964],[-9605],[-1866],[-8977],[5667],[6968],[-985],[8008],[-9704],[-4907],[9908],[2012],[-1560],[7842],[-362],[-7194],[1131],[3290],[-3245],[-4537],[-7936],[4530],[-1115],[-6907],[-5818],[5332],[3638],[6624],[-4510],[-8669],[-7971],[3118],[-2404],[5066],[-5630],[-7289],[7933],[-7215],[8272],[5017],[-4390],[7460],[8510],[-2352],[-1786],[4799],[5727],[1581],[-605],[-3324],[318],[-5530],[-5764],[-1231],[-9988],[-9832],[-4895],[-2455],[5520],[-3860],[-7626],[-1160],[4462],[2376],[8718],[-3816],[-4123],[1162],[-1802],[6451],[2093],[-2196],[-394],[-3894],[-4067],[5270],[-5481],[5179],[8139],[9178],[1213],[283],[-7479],[3645],[-9771],[3784],[-2327],[1283],[3502],[-2697],[5628],[5492],[7612],[3150],[-2324],[-2058],[-703],[-5316],[-4143],[9503],[-2138],[5008],[6743],[2422],[1194],[650],[-5903],[9092],[2143],[-3316],[5866],[-6299],[4478],[604],[-4859],[8359],[-438],[-6011],[4153],[8062],[7996],[-4021],[-4573],[7654],[-8643],[5676],[32],[-8195],[-181],[3010],[-3372],[9034],[-3654],[8403],[6783],[-3184],[8337],[-2366],[7015],[5301],[1739],[9822],[6875],[-2202],[9342],[8237],[-443],[7124],[952],[7204],[1653],[4249],[-3987],[6898],[-5359],[-5376],[5175],[9366],[5141],[5687],[6730],[2284],[6152],[-2512],[-5261],[4548],[1442],[2452],[3618],[-3008],[-7476],[-185],[1272],[-5897],[-6864],[2283],[-2263],[-6408],[-3812],[-4933],[6096],[-4263],[-1053],[-3454],[8644],[1057],[-6751],[6345],[-6967],[5009],[5536],[3165],[-8958],[-2144],[-5395],[-1639],[4886],[-5501],[1073],[1439],[5315],[-71],[-6262],[6328],[1680],[-8546],[6741],[-699],[402],[8911],[-8825],[-7170],[-6354],[-1088],[-2221],[9286],[-2930],[-7523],[-4379],[-5760],[-2879],[-6957],[-7065],[-3423],[391],[-7377],[-6413],[2898],[4774],[-5454],[9973],[-9586],[-2958],[-2270],[-9844],[8394],[9563],[-81],[9096],[-7734],[-5658],[806],[-2755],[7585],[1571],[-903],[-8261],[8153],[1466],[-4855],[4668],[4956],[3713],[-8592],[8105],[-7873],[-4182],[3233],[7394],[55],[1010],[6376],[3228],[1019],[915],[7486],[3365],[1368],[6713],[8417],[-5191],[3393],[1172],[-7272],[5698],[564],[-4284],[8492],[-5040],[-264],[3432],[6496],[9348],[6524],[-3330],[1702],[-4551],[6128],[-859],[-9916],[591],[2798],[1881],[-9873],[7555],[3053],[4301],[7826],[5484],[1928],[-5235],[-3874],[-7843],[9298],[-9684],[9121],[4301],[4329],[-480],[-636],[-2498],[-1953],[-4667],[-7438],[-5404],[-7183],[-2541],[9426],[3183],[8385],[470],[2200],[7537],[-4753],[2765],[-8618],[-1763],[9100],[-5036],[-924],[-4451],[-9914],[9096],[3839],[-3370],[-6409],[-3138],[-8306],[-9668],[2356],[3303],[9778],[-5658],[8796],[3270],[7657],[9201],[6459],[-7134],[-67],[-6007],[2843],[-4339],[746],[688],[-7257],[8209],[-3183],[-6522],[6204],[-2931],[-1849],[779],[-7112],[-9344],[7153],[3493],[-7792],[-4275],[-1911],[-75],[2856],[6242],[-8249],[2817],[2419],[-7304],[-3651],[-2533],[-3470],[-4361],[3477],[-4538],[7088],[-1372],[-4666],[6587],[3728],[2770],[3761],[-4417],[7529],[7879],[-4467],[6114],[9836],[5096],[6676],[-4477],[-219],[1582],[6433],[7898],[5747],[-8259],[3928],[-5690],[-7339],[-9616],[8217],[-6781],[-5732],[-838],[9515],[-8811],[-7123],[-2929],[-5072],[-1997],[8853],[-4807],[9441],[-36],[2020],[-2236],[-9471],[9062],[-2108],[1743],[-7253],[676],[-3785],[7470],[316],[-3357],[9738],[-4485],[-2326],[8450],[2428],[8944],[-5840],[-5256],[-6836],[-6626],[-2691],[-7954],[-5994],[1592],[-7736],[-5970],[-848],[-9296],[-1446],[3051],[-5501],[7373],[7922],[-5428],[2342],[7594],[5665],[3850],[3068],[9895],[-215],[8535],[6439],[-7207],[2402],[1782],[-5909],[-7064],[-8306],[7627],[-9063],[-8600],[4077],[-4736],[8728],[5480],[1436],[-2114],[6436],[9112],[-255],[3635],[3563],[9646],[5673],[6073],[2714],[-2473],[-5990],[-4136],[-8991],[-7118],[8453],[9144],[-4947],[-2203],[3225],[-9801],[20],[-2783],[-9711],[-8974],[-7100],[-5975],[9107],[8936],[-3323],[6626],[6880],[3443],[-8913],[-4505],[-2148],[3770],[-557],[5074],[-5191],[-4143],[-2827],[-520],[-2250],[-6549],[8060],[8393],[-4200],[4308],[4100],[4463],[-6339],[-8312],[7920],[-9849],[-1732],[933],[-859],[6669],[5830],[-3579],[3163],[8694],[-1646],[8834],[-7349],[-7292],[-6929],[-553],[631],[-1259],[-7289],[7347],[5322],[-7807],[-6585],[3953],[7910],[-3350],[4674],[-2561],[73],[8064],[-543],[7292],[913],[-530],[9021],[-3068],[-9686],[-9381],[2954],[-3328],[-7801],[-6707],[-2661],[-1583],[1815],[3448],[3655],[8683],[1040],[6931],[1161],[8425],[4374],[3632],[1404],[4601],[482],[-3445],[883],[-8667],[-7690],[947],[7488],[5998],[3590],[2438],[-2783],[8879],[-3585],[-6016],[-2162],[9985],[-4778],[-7629],[-6555],[-1055],[-1356],[201],[-3776],[9573],[-1136],[9306],[9866],[-3087],[-2406],[-8242],[-5791],[4566],[-5720],[-6853],[-6063],[4794],[-8695],[3137],[-2370],[-5837],[9820],[8180],[5535],[7744],[-6501],[6867],[-1620],[9194],[4184],[6436],[-7982],[-2208],[4386],[1611],[9621],[8808],[-6881],[-296],[-2834],[-7420],[7430],[405],[-3501],[3537],[-3771],[5384],[-3001],[1517],[-6354],[-8623],[-6812],[-825],[1276],[6174],[-1942],[7705],[7650],[-7832],[7264],[-874],[9595],[-892],[1523],[-6297],[-2094],[8633],[-2101],[807],[-2742],[-5951],[-1254],[-402],[-9886],[2289],[-4596],[-1438],[-1092],[9418],[-9242],[-5444],[3466],[-2099],[-6106],[-495],[3710],[504],[-748],[-7896],[-6674],[-1622],[3449],[1805],[-5234],[-344],[9194],[9766],[1807],[-9087],[-6615],[5373],[2103],[-9104],[3645],[8959],[1214],[-929],[-7218],[-8740],[225],[9872],[-9166],[7430],[5830],[-3611],[5962],[-7775],[4074],[8559],[9353],[1521],[6711],[-1509],[-6548],[-9259],[-2404],[-4524],[-8524],[2942],[6429],[-5184],[54],[1831],[1703],[-2776],[-9460],[7461],[6785],[8834],[8967],[6560],[2180],[8871],[-4243],[-1803],[7757],[4642],[-3394],[3013],[6595],[4750],[8381],[-8109],[1351],[-6796],[-3809],[-7376],[-5186],[8017],[8286],[-1899],[-7555],[-9409],[-8725],[-371],[-2679],[-2670],[-6024],[6877],[8527],[-9431],[-1026],[2145],[-257],[4209],[-8148],[9447],[6693],[-2432],[6496],[3726],[-4539],[1078],[1570],[9456],[-8668],[7569],[-8796],[3976],[-8195],[962],[-9648],[9903],[-5843],[5193],[-2642],[-6754],[7137],[7397],[1012],[6064],[-5107],[-73],[2787],[3760],[2875],[-423],[9645],[6444],[8468],[-6011],[-6817],[-6930],[1243],[-5999],[5787],[7728],[7027],[5802],[7429],[8143],[-9493],[4500],[-4319],[-5217],[-4416],[-1841],[-2612],[-6789],[4010],[-667],[6973],[-265],[622],[-7076],[-1027],[802],[-5338],[-4213],[-4819],[3214],[386],[-7152],[-7622],[-5834],[-2850],[3064],[797],[-5044],[-2535],[-691],[1675],[47],[-3007],[-735],[-1470],[136],[1436],[-8334],[-6568],[7609],[-6987],[-9139],[-1201],[-9129],[-3950],[9907],[6975],[-4850],[4278],[-5906],[7927],[4307],[7972],[-5640],[-8836],[7416],[163],[-3083],[9279],[270],[-9924],[1885],[6023],[3826],[-1399],[-989],[4493],[-2601],[-8166],[2551],[6950],[-3250],[-9300],[5223],[5997],[9799],[-9388],[3650],[896],[8233],[-4889],[7165],[-8192],[-1601],[-8114],[2456],[-2664],[418],[-5211],[-6371],[-106],[-8926],[-3670],[-3773],[2919],[6570],[8328],[-3640],[-633],[-9119],[2435],[1099],[-8059],[5999],[9011],[1155],[-6841],[7039],[9685],[2717],[-2857],[-8248],[1265],[-3855],[8063],[8551],[-6030],[-5037],[5330],[9881],[-3856],[-959],[-986],[3618],[7902],[52],[3594],[3016],[-7366],[-5259],[-5020],[7494],[-1188],[-6695],[-4341],[1125],[-1807],[-7339],[-6735],[-6111],[2830],[5566],[-7467],[-3048],[1992],[9570],[-7527],[-61],[6599],[6305],[2363],[-6867],[-5764],[7318],[2414],[1385],[-2016],[9935],[3062],[4695],[2682],[1038],[-8572],[5428],[-4277],[3486],[-5136],[-5114],[-8453],[6117],[3157],[9606],[-1329],[6405],[8953],[5585],[-3046],[6274],[8384],[75],[4896],[9676],[-5941],[-3603],[-3736],[-6602],[-8802],[-1275],[-5862],[-2328],[-2782],[7633],[-7028],[-9377],[-3244],[928],[8475],[6803],[147],[-3386],[5457],[-2726],[9380],[-474],[-266],[-3431],[-4126],[-6468],[7787],[-4620],[-7092],[-3350],[4006],[8587],[-9289],[614],[1146],[1962],[-8137],[-2466],[-3391],[-1016],[-877],[-4605],[7602],[-4125],[-8434],[-1416],[-8979],[-1529],[-425],[7604],[-620],[-8157],[9593],[-5100],[6555],[-6896],[-1312],[9142],[2390],[6542],[-3202],[1586],[-1338],[-7670],[-30],[-2247],[-7026],[9901],[-5207],[1987],[8821],[-5442],[-917],[806],[-2976],[9430],[5354],[-9313],[6759],[-7934],[2927],[-7482],[3396],[-9698],[-1471],[-1244],[-5555],[-5641],[-5737],[-1910],[5366],[9351],[8334],[-1808],[-6905],[-7261],[4963],[-3095],[-2182],[5958],[-8823],[-5590],[-9033],[-646],[-1617],[8576],[1720],[6581],[6083],[-3221],[5285],[3628],[-5836],[-735],[8717],[9181],[-6408],[7221],[-4517],[620],[2876],[-6106],[3918],[-8395],[-9241],[-9576],[4047],[-5657],[186],[3997],[3618],[4318],[3972],[-7672],[9253],[-4104],[-7112],[3392],[7546],[6915],[-5796],[-8429],[-2082],[-4709],[-2890],[5826],[342],[-9717],[6843],[-7660],[8961],[-6540],[-7698],[-8438],[8941],[4507],[5493],[-3822],[32],[-6586],[-5261],[-4600],[4549],[5189],[5589],[-4326],[8015],[8083],[9385],[1805],[-5287],[-3300],[-7693],[4866],[3291],[-3906],[6317],[-5719],[5073],[1150],[-1863],[7754],[-5245],[9705],[2736],[-5487],[-5511],[1978],[-2983],[-6304],[3646],[-5061],[-4147],[-4649],[543],[4152],[3953],[-6572],[2637],[-7393],[-8790],[3128],[-3743],[4915],[-9802],[-4245],[8124],[1023],[-6918],[-1409],[189],[5667],[4358],[7381],[1245],[2438],[-5135],[4401],[5271],[3671],[4606],[1556],[2566],[5587],[5569],[1332],[-5574],[1751],[-4737],[-8225],[7698],[9632],[-2534],[-3848],[4989],[4210],[2378],[6730],[395],[-9624],[5150],[6135],[-8669],[7560],[6134],[-4102],[324],[-8576],[3076],[8614],[2944],[6285],[4563],[5133],[4323],[-6972],[-4311],[1590],[2983],[-9448],[8296],[-5044],[7524],[-5525],[-6877],[-6307],[2547],[-9833],[-489],[-9347],[-8979],[794],[-2545],[8910],[-7986],[-1042],[-6368],[-6098],[7841],[-1841],[7563],[2316],[1406],[-3473],[-6927],[3741],[-2254],[-2632],[-6644],[3504],[-2141],[3194],[1131],[8911],[1389],[-2105],[6437],[8215],[8380],[3544],[-8468],[1149],[1562],[7880],[6291],[-2722],[4894],[3949],[-8882],[5467],[7174],[-7783],[-5291],[4566],[2337],[-7951],[-1889],[-8298],[3616],[364],[8761],[2180],[-3385],[-9921],[284],[1315],[-5040],[3746],[-9172],[6021],[-2648],[-8853],[1794],[7876],[3696],[-2981],[-1449],[-1783],[6354],[-7316],[1796],[-4968],[-7692],[-1718],[7782],[6308],[3012],[7747],[-897],[-5237],[-4799],[7339],[2242],[-2486],[-7661],[-6197],[-6262],[-820],[4799],[-9062],[-5636],[-9455],[-2731],[6587],[1263],[3287],[-5973],[9461],[6309],[3303],[7854],[295],[3427],[3162],[-1477],[1715],[-5971],[651],[3631],[4324],[-1768],[734],[-8462],[7812],[8912],[-7589],[3965],[-9318],[-5891],[-6640],[4920],[912],[-240],[-7638],[-6180],[-7635],[-5157],[-364],[-6065],[6325],[-8966],[8047],[-4600],[7131],[-7907],[4580],[7084],[26],[-2858],[-7274],[-7949],[6722],[-8929],[-8322],[-5187],[-8741],[7175],[-4932],[1391],[-4274],[-3925],[-8621],[4012],[-8563],[2516],[3586],[-1603],[7025],[-6136],[-3319],[5100],[-2142],[-2128],[707],[2198],[-4386],[-142],[-6506],[-1804],[3700],[-7770],[276],[-8824],[-6108],[-5419],[2169],[546],[3022],[-7634],[6602],[3480],[-3583],[87],[8596],[-8034],[-6856],[-4591],[-5426],[-890],[-6083],[4033],[-837],[-1028],[-9193],[-4447],[5041],[7505],[4556],[424],[4663],[7779],[7473],[364],[3800],[-1230],[-909],[-7484],[4158],[-7394],[5587],[2097],[-2608],[-9889],[-6404],[-5620],[1073],[7509],[9016],[-9856],[7189],[-6033],[-8190],[5849],[4093],[-7701],[2971],[1189],[3648],[4924],[9888],[2863],[4702],[-4258],[-663],[672],[1148],[-5662],[-9338],[-6792],[6386],[-7778],[-1943],[2191],[-7748],[-302],[9278],[4707],[8586],[5030],[2176],[8201],[866],[8249],[109],[-9140],[-6647],[7620],[-6464],[-1210],[-3701],[-3910],[-7289],[-9993],[-766],[4940],[3427],[8137],[2094],[4027],[3523],[7252],[9407],[-5205],[-5205],[-3859],[-2864],[297],[-8286],[918],[181],[8281],[1731],[-3302],[4912],[-6209],[5308],[-5525],[-8626],[5810],[9746],[-486],[-5526],[175],[-1833],[-9114],[2669],[1822],[3736],[4062],[-7155],[5698],[6856],[-24],[-9373],[-1831],[-4065],[-432],[-4548],[-649],[7354],[7451],[-5205],[7882],[-5542],[-9813],[-165],[2732],[6182],[2480],[6450],[7634],[6730],[684],[-8935],[-1013],[-1554],[-5676],[8745],[6954],[-2655],[-3538],[8158],[9896],[8174],[-1968],[-6958],[-9349],[6401],[-2804],[-60],[4001],[-6302],[8586],[-3798],[2670],[-239],[-4354],[-6328],[-7967],[9870],[-3425],[-5824],[-1199],[8276],[9162],[-8656],[3829],[424],[8187],[7301],[-1761],[-7114],[5427],[9979],[5398],[-1793],[-8077],[-3990],[-5748],[5147],[7259],[3764],[4207],[3991],[-9057],[8293],[4465],[8373],[-7383],[3463],[5073],[-403],[415],[9254],[-8525],[4777],[3336],[6370],[7041],[-7707],[-1438],[5214],[845],[-9565],[-7323],[-7836],[8299],[1927],[3234],[2695],[-9567],[9586],[3531],[-7097],[-1169],[-3802],[4721],[5929],[2385],[-8193],[-8189],[-2013],[591],[4175],[7587],[4260],[-8564],[9309],[-9935],[4173],[6415],[9442],[7620],[7783],[-8786],[1862],[8442],[-3273],[4404],[2047],[6420],[6799],[-8883],[2460],[-4910],[1596],[1504],[-8952],[9532],[3914],[-4202],[-7489],[-7336],[-4435],[5977],[3472],[-6119],[2516],[4700],[-7384],[7441],[-1978],[3512],[9762],[-7614],[5230],[-6001],[6443],[365],[109],[7783],[-5296],[6300],[-4501],[7365],[1499],[2683],[-5590],[6439],[1582],[4307],[2035],[2185],[-4399],[-608],[-2789],[-3887],[-5374],[-672],[-554],[9653],[-8955],[-6111],[-4926],[3355],[6234],[2428],[9089],[553],[4238],[6322],[-1036],[3612],[682],[9296],[-5465],[-2661],[8059],[-3382],[6299],[7403],[1684],[-268],[1184],[654],[3777],[8662],[-1301],[-1679],[-7714],[-9449],[-6251],[2774],[-1731],[2338],[-1540],[-6925],[7810],[-3684],[-6612],[786],[389],[4350],[-5410],[818],[-1581],[-9574],[-4582],[-8183],[-8666],[9752],[-9612],[-7992],[9613],[-1966],[-1299],[9931],[1004],[-1433],[7965],[270],[2983],[4004],[7871],[-9383],[6978],[3816],[231],[-6103],[-7108],[3524],[2957],[3352],[3409],[-5884],[3265],[-32],[999],[-6125],[1071],[4002],[3945],[6162],[6605],[-6906],[-843],[-4064],[9857],[-8649],[6998],[9231],[4920],[9243],[700],[5606],[7871],[-3496],[-412],[7518],[6224],[6725],[4860],[6243],[7384],[3034],[2374],[6250],[4284],[-2314],[-2140],[2496],[9254],[-7422],[-4200],[5762],[9132],[1192],[-3817],[-9907],[-9446],[1285],[9610],[9715],[-1901],[-1620],[964],[1603],[-2831],[1774],[7373],[1174],[-2318],[-4807],[-1135],[7238],[4287],[-2703],[1276],[8077],[-3139],[8318],[1775],[-4297],[9369],[-125],[222],[-7930],[9328],[-5078],[4457],[5065],[-9728],[-2974],[5878],[-5723],[-8872],[1528],[9061],[-3773],[-6075],[7119],[8936],[9474],[-3806],[-7958],[7353],[-9316],[7860],[-2675],[-3398],[3409],[2765],[1798],[-3563],[-1745],[2667],[1124],[-6129],[2911],[6602],[-5555],[-9774],[8937],[-32],[6572],[9869],[7332],[-7822],[-355],[-5797],[9215],[3812],[-5382],[6874],[-280],[-6620],[-2672],[-7873],[3860],[7615],[-8256],[-3325],[-8185],[6646],[7518],[6543],[-7348],[-3955],[-1684],[-47],[-6521],[2821],[-9372],[-1243],[4499],[1612],[-7643],[1314],[6402],[9484],[-9253],[7673],[7959],[-7351],[-9530],[1219],[8540],[3471],[-5810],[4130],[9781],[6736],[-9778],[-2031],[3509],[-8120],[6378],[-1043],[-3376],[3705],[-1267],[-9263],[689],[-873],[-4478],[-6293],[1289],[-662],[-7638],[-8867],[-9207],[-9438],[-9841],[6051],[9957],[2189],[-9046],[6224],[3031],[-803],[-2924],[6031],[-4977],[8278],[-7604],[2195],[-944],[-6385],[7849],[-4857],[1046],[9467],[-6026],[-2248],[9278],[6798],[-9994],[-3183],[4745],[-1505],[-1406],[-7159],[-6993],[-5043],[7261],[-6864],[6717],[6801],[-3310],[-5567],[-4330],[4559],[-3958],[8876],[-6346],[9489],[2398],[5659],[4685],[-9307],[2228],[5087],[-7129],[8465],[-7737],[4203],[-719],[811],[5068],[6158],[-4770],[-1604],[-216],[2059],[5103],[3355],[-9925],[2109],[2530],[-6915],[-212],[2539],[513],[-5103],[3720],[-3795],[2128],[4836],[9395],[8526],[8192],[-4831],[-5285],[2604],[1141],[5271],[-1384],[8717],[-6936],[-2115],[-1212],[-4621],[-5448],[8267],[-9709],[7784],[2407],[1238],[-722],[4708],[-988],[-6840],[-4154],[-6148],[4273],[4265],[-2030],[3771],[-6600],[-6662],[-3735],[1935],[6839],[-4319],[-3223],[5867],[-5866],[-9240],[2971],[-2372],[-9079],[-1301],[-6171],[-4935],[9453],[3575],[-3966],[3293],[-7172],[2480],[5859],[2698],[-6394],[-8347],[-8710],[-8384],[-9296],[-7196],[-2111],[5168],[-5356],[-2829],[6317],[-5945],[3863],[-963],[-9809],[-7574],[-2656],[5048],[9622],[-838],[7842],[803],[-4836],[-8996],[-8566],[-6816],[-7850],[-5784],[-2088],[-5808],[-367],[5314],[-3507],[-9389],[-5977],[-9052],[5893],[8719],[-8311],[-9936],[3605],[160],[896],[-787],[122],[3386],[942],[7147],[-7139],[2131],[6461],[-5769],[8108],[3542],[7468],[-6578],[8366],[6608],[8495],[-3086],[5902],[8715],[3964],[1980],[-6715],[-7036],[-9767],[-2110],[540],[7966],[-9431],[-2864],[3049],[-3582],[3949],[1339],[4644],[-8581],[1163],[-8321],[-124],[5861],[6982],[-8563],[1549],[-4480],[199],[-1133],[-4734],[4359],[1255],[9495],[1669],[1541],[9956],[-6004],[7904],[-6188],[7039],[-2274],[1711],[-7577],[8540],[-2374],[3662],[8913],[-6046],[2835],[-7880],[-803],[4709],[8936],[1013],[-7027],[1940],[-9848],[-5823],[8150],[-6548],[6813],[2601],[7882],[-2033],[9975],[-3587],[-1832],[-8870],[-6876],[2361],[9423],[5793],[-1183],[220],[-3046],[2209],[-7303],[-6136],[-8779],[2075],[-8230],[-4561],[-7439],[-6920],[8800],[-1908],[3798],[-206],[-992],[-8088],[3174],[8988],[-655],[-2957],[-1636],[-6884],[8162],[4346],[8478],[-8591],[8967],[4058],[-3693],[6524],[-7987],[-8425],[8466],[-3387],[6347],[6656],[5178],[3613],[-2332],[7589],[5428],[-2963],[-6038],[-909],[5788],[-5264],[-98],[-1968],[9678],[8743],[4529],[-6202],[-7424],[-9374],[-2401],[4026],[6799],[-8642],[3437],[337],[-2874],[-3139],[9709],[6662],[-2264],[760],[5364],[7639],[-4800],[8808],[7971],[5718],[5839],[2677],[-3854],[7949],[8106],[2782],[-3544],[8353],[-8126],[3470],[-5425],[-3348],[-8788],[-1367],[6580],[-7577],[-4345],[-343],[-1589],[373],[618],[5421],[-2864],[9304],[-2796],[-8132],[-2804],[-9947],[1307],[1024],[5175],[3547],[8207],[676],[6220],[-9001],[-3409],[2182],[3621],[1454],[1997],[9927],[-4145],[7265],[-2444],[-5321],[8442],[6832],[982],[-4090],[-4217],[-7868],[-813],[-7258],[-86],[9091],[-7208],[9130],[614],[1092],[2685],[-8632],[-8919],[2367],[6038],[5375],[-375],[-7455],[-9450],[-5948],[6521],[-393],[4931],[-2346],[-1389],[2664],[-2532],[-8312],[4863],[8649],[-479],[-5654],[-4503],[4971],[5044],[-1100],[9182],[-7608],[8981],[-857],[1955],[-3455],[9019],[8152],[1854],[1581],[2322],[-4363],[2485],[9756],[8223],[-9613],[-8346],[-2003],[3432],[5221],[-6120],[3233],[-5289],[8830],[4573],[4496],[6433],[-1308],[9910],[5250],[9541],[-2614],[-4995],[3697],[7936],[6204],[-3059],[-8531],[6426],[-5765],[8482],[-1642],[-3],[6740],[1277],[-1171],[8767],[3310],[-8073],[-9579],[1998],[8362],[9505],[5286],[-3909],[-3196],[-1778],[9157],[1421],[-3923],[-7574],[7196],[5715],[-9060],[9047],[-6847],[-3970],[3236],[-4711],[2947],[843],[-9058],[-519],[-3098],[-9185],[7029],[-765],[578],[-7418],[3047],[-3637],[-6469],[-5255],[6558],[5316],[-2516],[7389],[890],[-5659],[-4878],[-3893],[-3398],[9881],[3955],[-536],[6556],[-3627],[982],[3055],[-4219],[2128],[7115],[149],[-9215],[1079],[7813],[7403],[-6442],[8959],[6770],[-1546],[-8483],[-4881],[286],[8029],[586],[-2816],[3772],[3953],[1377],[1702],[6778],[7327],[3144],[3136],[-8634],[-5981],[-4048],[-3547],[-7108],[-1091],[3585],[-2158],[-1529],[-2282],[2250],[-6881],[9598],[-4006],[-4474],[-8972],[2082],[8043],[-2681],[-934],[7034],[-1279],[692],[7553],[8511],[2548],[9354],[6331],[-6277],[9035],[1183],[-617],[5766],[6539],[5739],[636],[8061],[8424],[1056],[-3068],[-1340],[6103],[1979],[-9999],[-5874],[-6585],[609],[-674],[5643],[-9571],[-6072],[4339],[1439],[7184],[4974],[-3423],[4948],[-6030],[-3525],[-3282],[8813],[-9406],[-3309],[9788],[2318],[-6900],[427],[9205],[-3173],[6627],[4462],[-9277],[8891],[77],[7634],[5395],[7231],[-3822],[-914],[5731],[8045],[616],[2637],[1673],[2415],[3264],[-6945],[3542],[-1718],[-6626],[-3260],[-4300],[9760],[-6674],[-9708],[-2126],[-9659],[-4874],[4056],[-1808],[1365],[-916],[-6587],[-5948],[-1299],[-8006],[750],[-2711],[3687],[-8985],[-8365],[9746],[8986],[6876],[-7833],[8836],[3543],[-9796],[8804],[-6913],[-4409],[-7022],[672],[5662],[3334],[-6021],[-3804],[9628],[-9153],[2929],[-2876],[8857],[-9396],[-6746],[8190],[-2439],[9833],[-4557],[-7706],[-3096],[4226],[1927],[-9986],[-104],[6276],[-9943],[4878],[1711],[-6271],[-8299],[9466],[-8257],[4729],[278],[651],[-931],[-6664],[2853],[9177],[2240],[-9152],[-9251],[-7724],[764],[-6612],[867],[955],[8715],[7725],[-2527],[1022],[-3508],[6995],[6942],[7286],[-8469],[-7520],[-1121],[-6229],[5793],[-1425],[-5145],[-992],[-1373],[8984],[-1087],[8466],[6163],[-7088],[-8053],[5334],[-7300],[4620],[-7993],[3205],[-974],[4403],[-9333],[175],[-3527],[9998],[-2944],[970],[5544],[-9950],[-2662],[547],[7357],[8714],[-6885],[4742],[2197],[1802],[7964],[-33],[-7938],[2312],[-3424],[-4215],[2110],[-8888],[1508],[7323],[-7637],[9341],[-84],[-6129],[-8909],[5097],[831],[4295],[858],[9039],[7656],[-8840],[701],[-6275],[1129],[-5953],[-3077],[-9045],[-9298],[-2015],[-3846],[1467],[2036],[6929],[3031],[-7974],[-118],[2338],[7202],[9528],[6155],[-5004],[-8335],[-8087],[4110],[-4021],[1750],[-5238],[-6770],[-4102],[9626],[-8947],[-2115],[2707],[-6170],[7977],[-2938],[9832],[-5467],[-4740],[5934],[-222],[9150],[-4230],[8601],[-6960],[2644],[472],[-7458],[-3839],[-7344],[-6868],[-1976],[9395],[-1563],[2781],[5411],[-9166],[8312],[-1052],[-8428],[-8629],[-3403],[7104],[-9491],[-7282],[5933],[-9737],[8221],[1877],[-828],[6353],[9781],[8291],[2929],[-441],[902],[-890],[8029],[-1921],[-8271],[2012],[7188],[5286],[8315],[545],[-4886],[9099],[-736],[-100],[6642],[7099],[9476],[6807],[8919],[3256],[3884],[-9277],[5498],[6189],[8501],[-549],[7195],[8557],[-8278],[-3337],[-9413],[-3595],[-963],[-4716],[-4495],[-2297],[8044],[-6081],[-509],[-1015],[-9265],[-2122],[7245],[-1502],[-1100],[-1669],[-4106],[4629],[-8622],[-988],[-9795],[2311],[39],[9980],[5175],[-1298],[-3901],[-2916],[9741],[7791],[9598],[-2603],[514],[-5344],[7402],[2168],[-4320],[-8299],[2399],[6156],[-7184],[-541],[1791],[-8300],[5400],[9220],[6836],[7477],[3330],[2751],[-9923],[4773],[-7150],[796],[2777],[-5985],[-3725],[7022],[-3920],[-7640],[2731],[-8521],[-2003],[-8632],[-9626],[-9531],[-5567],[-8429],[3260],[-8017],[-2545],[7767],[1601],[8112],[-1173],[8284],[-1016],[5441],[-1059],[-2275],[1108],[-6116],[3751],[-5637],[-2795],[-8904],[7962],[-6841],[-2040],[-557],[42],[-5200],[9392],[5621],[-7515],[-1056],[6021],[7750],[9182],[5537],[-9641],[4118],[4648],[-8398],[-8303],[1334],[-6327],[468],[4871],[-1680],[4133],[8062],[-9437],[4166],[3623],[-352],[-3398],[5216],[-3697],[-1511],[2142],[-860],[3649],[619],[1668],[6581],[-4632],[-5716],[5068],[2383],[3513],[-9925],[-5750],[9507],[-3241],[-703],[-74],[8001],[-3294],[-3263],[8614],[-9829],[8101],[2904],[-1842],[-3000],[276],[3496],[-5279],[-1968],[4869],[-1098],[-2908],[1454],[-8390],[3049],[1111],[-1049],[-1157],[3927],[1385],[-3450],[-9989],[5118],[-7389],[-3025],[2295],[3808],[3180],[-5767],[-71],[9866],[4903],[4539],[-6803],[-5354],[-9015],[5593],[-3255],[-4219],[-4560],[5896],[7535],[4524],[-1490],[9132],[-2508],[8555],[8683],[-9444],[-7316],[7569],[-7627],[8676],[-7357],[-1114],[-5394],[-5241],[9959],[980],[-1889],[4495],[5516],[5426],[-2850],[-4733],[8317],[5271],[-8932],[-5300],[-3400],[5706],[-3828],[1135],[4037],[-7567],[816],[-1117],[-4655],[9946],[-3525],[4889],[-2503],[4418],[-1672],[-148],[5162],[6303],[-7599],[-7234],[9948],[-9528],[4205],[-1035],[9664],[4264],[-578],[5245],[-3677],[6273],[7881],[5883],[464],[-2514],[5082],[-4549],[-4780],[7999],[5206],[1305],[6214],[3988],[3345],[-9323],[-5823],[2570],[-3023],[4185],[6993],[-6255],[8810],[-8630],[-229],[5059],[408],[-7970],[-9219],[-5922],[4782],[-6490],[-9063],[7386],[-176],[5398],[-6930],[5095],[3621],[-7590],[-2124],[3045],[2481],[8792],[-7169],[-3088],[-4273],[-4156],[698],[9254],[2798],[7844],[-103],[-621],[-6719],[-5571],[-7484],[-7977],[3969],[-1213],[-7980],[5341],[2701],[-5012],[3817],[-5450],[3828],[-8717],[-2503],[-1203],[1939],[9147],[-8583],[-9350],[3307],[1552],[-7742],[2829],[-2815],[7744],[6228],[4617],[9713],[6122],[-978],[6972],[-3879],[-4606],[-210],[-4344],[-681],[-388],[7958],[-7436],[-9156],[4042],[9421],[1277],[5216],[-5431],[2882],[107],[9638],[4507],[1821],[6606],[674],[-5373],[-1994],[5878],[-4583],[-6078],[-7389],[-4044],[1915],[930],[9939],[-4513],[117],[6103],[6332],[7795],[5326],[2951],[4322],[-7485],[863],[-4688],[1237],[-3943],[-96],[9440],[8204],[9431],[-3330],[-7328],[4907],[-3519],[-7367],[5398],[9436],[-1547],[449],[8073],[8197],[-3439],[8093],[-9890],[4902],[3957],[-3890],[1053],[-934],[4248],[7806],[-4798],[-794],[2565],[7927],[-6290],[2681],[5586],[7169],[-7583],[7092],[1908],[-5279],[-1555],[7089],[1402],[6376],[-9339],[181],[-2028],[-2687],[560],[4058],[4589],[3399],[-2118],[-5437],[-958],[5587],[1755],[3193],[4741],[9103],[-5619],[-4331],[-7737],[1646],[9952],[-5374],[-2061],[-8421],[6131],[2187],[-8766],[-5268],[-7494],[-2288],[6568],[-2687],[9427],[-1299],[-8347],[-9776],[-9258],[6050],[-3678],[6443],[7856],[7375],[8458],[-3437],[-5084],[-5472],[-8727],[-730],[-138],[-8149],[-2956],[1483],[-7801],[7233],[-5042],[-4799],[1059],[3702],[8451],[-6525],[6495],[4659],[-4026],[2662],[-9464],[3501],[7181],[3462],[-903],[2937],[-723],[-3387],[-3932],[-5952],[6580],[5099],[4199],[-5873],[6720],[-3965],[-529],[1634],[-7555],[-2937],[5288],[5384],[-7856],[-1618],[-3724],[9297],[9229],[6164],[8150],[6307],[-1281],[5138],[-9251],[-5213],[-280],[-517],[-722],[-5039],[9253],[-1392],[4162],[-2630],[3023],[8082],[-1427],[8023],[4330],[-4902],[3775],[-7499],[158],[-2554],[5049],[-4625],[1039],[8300],[8598],[-480],[6512],[-7079],[4371],[6365],[-7648],[-7884],[1651],[-3877],[-570],[8886],[-1664],[-8855],[2009],[-8539],[2868],[-6439],[-6906],[-3029],[-7755],[-2636],[5555],[-7315],[-1642],[-1655],[-4150],[3124],[2830],[-6038],[-7510],[-2557],[-4195],[-8522],[-733],[5817],[2685],[1936],[-6775],[-2267],[103],[-9777],[6318],[687],[-4995],[4517],[9744],[-9939],[8290],[-3365],[-9991],[4844],[-8852],[7852],[7330],[-6399],[-7983],[-6225],[-8245],[-1791],[-8358],[4986],[-5051],[6582],[1159],[-3316],[-7982],[-6774],[-4277],[2541],[9109],[9846],[1101],[250],[4834],[-452],[1191],[-1509],[9418],[1609],[-8976],[-5951],[4521],[-2395],[2561],[6778],[218],[8198],[-947],[7188],[2400],[-7147],[182],[9844],[4865],[-7678],[7600],[-7865],[-3665],[-1245],[-2310],[6220],[-3377],[-2702],[-6430],[-2303],[-280],[-1964],[6239],[8952],[-8149],[4502],[-5438],[7644],[2857],[-3996],[1317],[-2290],[-8849],[9745],[4149],[-2333],[3606],[439],[-2194],[-4075],[8539],[9346],[-6820],[-5095],[-137],[7589],[4540],[8785],[-3556],[937],[7435],[-8046],[8296],[8572],[5361],[-8524],[6515],[2309],[-4731],[-3971],[2232],[6931],[-7612],[4064],[-5635],[-3125],[-1449],[761],[2515],[-6100],[7623],[-7612],[-9599],[645],[1638],[-3097],[9228],[-9894],[-4572],[3852],[-3406],[2395],[-5693],[9573],[-4898],[-5676],[6558],[7066],[3651],[4394],[9912],[-3908],[-5682],[7977],[-9828],[-4213],[4179],[-3484],[843],[329],[7771],[-826],[6239],[-4055],[3682],[-4614],[1192],[-7136],[1521],[6358],[-3135],[-3417],[5506],[1890],[-7843],[933],[6104],[-4747],[-569],[9713],[4246],[-9371],[-9931],[-5140],[-9535],[-7649],[-6709],[1041],[8114],[-5193],[-7676],[1468],[7290],[8110],[-6248],[2375],[3528],[9024],[-5261],[-3389],[418],[-2720],[2421],[-2284],[8896],[-9861],[3576],[-2512],[3439],[4340],[-482],[-9156],[1890],[9627],[6967],[4404],[4647],[-8079],[-1327],[7901],[-5150],[3339],[-1954],[-6445],[-5193],[8071],[-7771],[9135],[5185],[2964],[-8568],[-2456],[3032],[3818],[-3465],[-3228],[1885],[-7466],[-691],[-6645],[-2970],[1556],[4615],[-4438],[7041],[4380],[5761],[3630],[-7875],[9878],[-6591],[2242],[4558],[-5193],[-5276],[-4925],[6084],[-3419],[6415],[3008],[9338],[-5717],[635],[-8359],[5487],[4709],[-9022],[-9560],[4278],[8210],[8257],[-3982],[-2586],[-8532],[6604],[-675],[-5608],[-7171],[-1335],[-7964],[6626],[4027],[6671],[1516],[9464],[8138],[-6050],[-8815],[-2827],[249],[-8526],[-7632],[-7104],[3570],[-8165],[4702],[-8521],[-6552],[3129],[-9544],[-1192],[-5046],[-4418],[-1699],[4614],[3339],[4439],[-9068],[-4347],[-2659],[3931],[9645],[4377],[4523],[-7221],[-4045],[9242],[-1520],[-3908],[-320],[-4699],[-200],[-4758],[-1720],[1491],[-2646],[-3718],[-1150],[-308],[4007],[-1869],[-8093],[-8671],[-2321],[3373],[-732],[-7927],[4046],[2403],[-4687],[7324],[3541],[-1402],[-5826],[-3849],[5848],[-110],[9435],[8671],[-8645],[5417],[1038],[-7023],[-6004],[7745],[9243],[-1000],[4311],[-1740],[3290],[-2292],[-9663],[7475],[-5430],[2836],[4220],[-4912],[-6527],[-4131],[-3364],[2813],[-1106],[-9958],[9553],[1535],[9386],[2893],[8167],[9281],[-6387],[-4046],[-349],[-9636],[6359],[8377],[2154],[7820],[127],[892],[-8096],[-2968],[9535],[-5384],[-2054],[-9756],[-678],[-5803],[9647],[-6483],[2224],[1003],[5390],[5114],[-657],[5555],[-8325],[1983],[-9611],[-9653],[4325],[2940],[2860],[-8140],[-6212],[-7113],[-1009],[-611],[5833],[3092],[8367],[9158],[-5964],[-4164],[-4505],[7049],[-2290],[-8724],[-1162],[4240],[4237],[7036],[-3840],[6180],[-2084],[4746],[-3955],[-7956],[-9174],[6762],[5785],[2500],[-6417],[3259],[3200],[2636],[-4952],[-6085],[-28],[-3650],[5519],[4776],[8724],[2921],[-2491],[2744],[-9499],[-2441],[-2528],[3624],[-4322],[5206],[4774],[-8924],[-6778],[-1084],[4099],[8418],[-9091],[-3058],[-1468],[-6744],[4454],[-5974],[-7662],[-6573],[-1867],[3212],[-928],[8116],[4496],[-7297],[4459],[3001],[-3781],[-6797],[-1208],[1581],[2537],[5947],[-2188],[6582],[-6699],[-4249],[9537],[-4958],[1293],[5659],[-1136],[-4330],[5992],[2097],[-3455],[2929],[6128],[4765],[4076],[-7607],[-1936],[-6476],[-7080],[-5262],[8915],[9788],[-7136],[9867],[9840],[8906],[-1556],[-8121],[-4199],[5344],[-9429],[-8936],[1961],[1933],[2925],[8340],[-8392],[-8574],[1512],[6255],[-5735],[-459],[1091],[8277],[-2344],[2180],[-9603],[3165],[-3265],[5835],[-7548],[-4893],[-8987],[4442],[-2947],[8706],[7109],[4033],[9521],[-7661],[271],[-7556],[9541],[-9254],[1822],[3678],[3421],[-6974],[8088],[-2627],[7927],[-896],[1873],[-1609],[1394],[1938],[7610],[2826],[-8554],[3339],[7394],[-6902],[443],[-2863],[5754],[-1959],[1624],[-5420],[3192],[6964],[-1996],[8907],[757],[-7259],[5331],[8617],[8175],[3464],[2891],[-6806],[-9752],[-2359],[-9889],[7562],[-2214],[6691],[-1483],[-1109],[5964],[-8621],[3964],[-9757],[2806],[8856],[7757],[-8427],[-4237],[2362],[-6822],[-3027],[8706],[1189],[4726],[4177],[-6625],[2739],[-7260],[-4265],[9819],[-868],[-6411],[-315],[7481],[-4314],[345],[7103],[1093],[9991],[-7122],[-328],[-6751],[-9482],[-9897],[-2993],[4208],[2340],[-4891],[-4936],[7068],[2462],[-429],[995],[-9468],[4543],[6311],[641],[4149],[-164],[6207],[4252],[-3179],[5229],[8788],[1523],[3937],[-2165],[-3555],[-874],[-1009],[-1027],[443],[-8857],[-1503],[-9849],[4899],[-1547],[-7081],[-6590],[-8955],[-8386],[4004],[-5277],[6222],[-9625],[-3517],[-21],[-7465],[420],[842],[-9035],[1680],[-4106],[2139],[-8937],[-2295],[-3567],[1333],[-1461],[9157],[-8178],[681],[4127],[935],[-5538],[4998],[7769],[-5121],[8411],[2971],[-9562],[5961],[1175],[4474],[1556],[-8898],[8446],[-7935],[-3569],[-9766],[-9689],[2082],[-2989],[-3341],[-1799],[-3953],[-9732],[-8023],[536],[2131],[-7273],[-3655],[9989],[46],[2836],[357],[-9572],[-4079],[3721],[1849],[8066],[1283],[3267],[5186],[-6266],[-6375],[-9082],[-823],[-929],[-5610],[-8347],[6156],[69],[9791],[-8159],[2642],[8055],[3617],[6161],[5515],[-1829],[-523],[3645],[3098],[5318],[2347],[-2397],[754],[-5727],[8863],[4762],[-3709],[4043],[-1541],[6353],[4810],[-7216],[-7627],[-8589],[-9465],[-8871],[-2653],[4869],[957],[8472],[-985],[1440],[-593],[-733],[363],[-6772],[3323],[-7071],[-4178],[6030],[3983],[7767],[-5657],[-4503],[9877],[5131],[3323],[6442],[4965],[-5709],[-6748],[3240],[-6931],[7446],[2553],[982],[-9437],[-315],[4835],[9810],[4830],[4373],[-5127],[1094],[9707],[-1671],[-218],[8260],[-5126],[-9629],[5913],[-8545],[-4282],[7186],[-3905],[6008],[7993],[4566],[4921],[-9814],[5896],[-9553],[-1432],[-9668],[6899],[7666],[5955],[-585],[2938],[-745],[-5953],[-857],[-1565],[9728],[-9179],[6583],[139],[5459],[-3439],[4279],[5596],[-5389],[-2760],[8312],[8986],[7922],[-8671],[6718],[6114],[8450],[-5590],[-209],[5553],[5917],[2633],[105],[8057],[-7135],[-7912],[9297],[6251],[-8860],[3945],[-6410],[-8691],[-8823],[2493],[4563],[-2751],[555],[-2677],[75],[-4454],[-7621],[5149],[-6492],[9619],[-4362],[-5762],[-2460],[9072],[6500],[1426],[-9011],[-3755],[5804],[8139],[-358],[-4650],[-309],[-1667],[5927],[3707],[2585],[736],[6130],[7159],[667],[-8221],[3163],[4676],[-4021],[9360],[406],[-1490],[8071],[-363],[7894],[8584],[6752],[-4616],[7000],[-322],[4108],[-5705],[6648],[-3471],[8394],[-956],[3391],[7700],[-6620],[2787],[-6524],[-2901],[4651],[-8077],[2117],[-8702],[-6129],[-7520],[3737],[-9754],[-9000],[-7461],[5089],[8076],[8492],[-2156],[-2253],[2172],[4271],[4430],[9448],[-2734],[9947],[8294],[412],[-691],[-8205],[504],[-5725],[6348],[-7594],[-4491],[174],[-1464],[8291],[-7164],[7727],[-4007],[3512],[-5345],[6314],[-6335],[3410],[-2710],[4768],[-7199],[-5794],[-1570],[6849],[-7091],[-5617],[9163],[-4312],[3978],[9411],[-1605],[-7783],[-8490],[-7629],[-5929],[-8855],[2580],[-8012],[9362],[-1990],[388],[6870],[-8161],[5401],[-8977],[-3503],[-3934],[-6350],[-6244],[8972],[8525],[8056],[-4227],[6050],[-7011],[8930],[-1397],[4436],[-9318],[-8756],[9667],[2661],[5392],[-1568],[8048],[8041],[-7420],[5588],[-8693],[5778],[7872],[8552],[4747],[3035],[9438],[3979],[-6031],[-8882],[4203],[2159],[-4471],[1189],[8272],[-8908],[-6618],[8615],[1783],[-6086],[2109],[-4672],[8629],[8477],[5982],[-288],[-4641],[722],[4329],[-5457],[-8290],[-9426],[-9874],[8139],[-7517],[6571],[-8187],[-7243],[-585],[5507],[-6577],[-199],[-6991],[-566],[-6015],[-4356],[5999],[-6131],[-62],[-3030],[1623],[8850],[6615],[8328],[-8813],[6678],[-5733],[-5918],[4723],[8960],[-7570],[-6777],[443],[-7184],[2165],[-3652],[7341],[-4426],[6186],[7608],[-8780],[-6696],[3663],[6169],[-4857],[4666],[1859],[-5413],[-9831],[1339],[-846],[-4798],[-6892],[8829],[3768],[9546],[-7895],[-1810],[2646],[-4288],[-1782],[2530],[-3843],[-8868],[-9091],[7371],[-5722],[85],[3814],[-982],[1646],[7575],[3329],[-2598],[-8078],[-7868],[-5573],[-3979],[-5565],[4817],[-5083],[-6185],[9509],[-4240],[7467],[8463],[-884],[-3866],[8263],[2215],[3415],[-6149],[-6674],[-4292],[5735],[-2394],[5889],[8463],[-8330],[3619],[-7259],[5821],[4740],[8284],[4760],[-6759],[-6940],[9396],[190],[1974],[9482],[423],[1507],[-2369],[-4943],[3086],[8765],[-8318],[2985],[-2601],[-4876],[7783],[9498],[-3968],[-1085],[8],[4006],[-5016],[-8489],[-7285],[4231],[2498],[-583],[6784],[-9201],[1387],[1910],[-4475],[6137],[-880],[-7238],[-148],[-8536],[1447],[6524],[9068],[3148],[6155],[-8701],[9032],[4449],[-1883],[-5013],[2124],[-4513],[-5198],[8423],[-9909],[9574],[-5786],[-14],[-4929],[755],[4748],[4180],[-9785],[-9738],[-6942],[159],[-3590],[2479],[-9347],[-1645],[2356],[9101],[-6246],[-9924],[9714],[-3086],[3153],[-1210],[6575],[-4743],[1569],[-2741],[-7307],[-3310],[8952],[-7688],[-7443],[4471],[-7221],[-5089],[-9137],[-8863],[6518],[-1710],[-2413],[8727],[-2409],[-2442],[-1913],[-7255],[7161],[6640],[6303],[6133],[-5034],[-5006],[-5868],[3545],[871],[4588],[1690],[-2153],[1136],[759],[-3496],[7335],[-5259],[3657],[-9278],[5689],[-7673],[-7553],[9805],[309],[-8672],[-1219],[7015],[5238],[2145],[2637],[-7260],[1622],[32],[8044],[2167],[4001],[1598],[-2410],[9123],[-6476],[1247],[-3767],[-8910],[9348],[1858],[-931],[-7109],[2630],[-5720],[942],[4700],[552],[-7795],[-3075],[-3706],[8660],[1379],[-1529],[4195],[63],[-1080],[-5934],[-4473],[-7249],[4003],[5649],[5141],[-2879],[4141],[3628],[-1312],[-2274],[-5648],[-2242],[-102],[88],[-8057],[7608],[-2014],[4154],[7010],[2874],[5622],[5093],[8080],[-836],[2141],[-1031],[-6373],[-9399],[5054],[2389],[-3500],[-9532],[-8477],[-4312],[7388],[-7996],[9852],[-1544],[-4343],[851],[8315],[-259],[3359],[-7397],[6718],[-6716],[9340],[-149],[-2253],[-5397],[-4625],[5375],[-1076],[-7684],[-1531],[-9311],[-1232],[3580],[-865],[-1287],[799],[5442],[2161],[-3091],[435],[-3793],[-7370],[6717],[-9569],[1847],[1898],[1086],[-1837],[-1777],[913],[5381],[1551],[-5764],[7965],[4935],[-2566],[-6695],[3857],[168],[6265],[8383],[8673],[-1910],[-7345],[7582],[-9661],[7984],[-8875],[6875],[-9337],[6354],[4886],[-7088],[-2396],[-7625],[-1583],[3260],[7107],[745],[3700],[9339],[860],[2724],[-5803],[-4124],[3974],[-2922],[-3175],[5891],[7876],[4004],[391],[5281],[6267],[1676],[2959],[-4087],[1171],[-5021],[8923],[5054],[4597],[-3039],[5955],[9855],[-8303],[-3290],[9056],[1002],[6814],[-5685],[-8162],[1357],[831],[3924],[3524],[7278],[-7846],[-6135],[7671],[-3358],[4846],[9513],[-4834],[-4020],[-632],[9085],[-1350],[5216],[9957],[-8938],[-8067],[3033],[1808],[-97],[-2855],[-3816],[-5336],[-2509],[-1654],[1973],[-8754],[118],[-2726],[9086],[-9405],[-2714],[-9092],[958],[4848],[6],[4777],[9177],[-3309],[-5744],[-7228],[-7829],[-4843],[442],[640],[7887],[9735],[5375],[-2195],[-2356],[-6581],[2292],[-1078],[3739],[-3763],[647],[9976],[-2099],[3068],[6726],[-3744],[2216],[-9640],[69],[-9622],[3341],[-5161],[-177],[-4141],[9872],[-6887],[-1557],[9434],[-531],[-7659],[-1356],[8020],[5387],[8573],[-1219],[-7929],[-7930],[-3932],[9759],[1540],[-1305],[-2536],[4660],[3545],[6469],[-5407],[-27],[-4722],[6552],[-8990],[8390],[-1089],[-5757],[-7085],[6369],[1759],[-2684],[-8820],[-2698],[3687],[-2312],[-9149],[5862],[-3230],[7967],[9797],[8550],[7756],[-1118],[-8709],[4243],[4414],[-3573],[-9762],[8682],[-738],[3611],[-4591],[-2694],[4706],[-9688],[-292],[-2396],[-7003],[6240],[-3608],[-2204],[9128],[7193],[-161],[-440],[-8592],[2567],[-3601],[7914],[2088],[3192],[1319],[-7955],[1038],[4098],[4609],[2865],[-7366],[-6574],[-9417],[703],[6850],[258],[-3718],[7018],[-1000],[6165],[-5923],[3062],[2413],[-2915],[4677],[7197],[9912],[-8206],[3862],[7561],[-4986],[-6353],[-405],[8889],[1996],[-8154],[-2103],[-5816],[8292],[9598],[-513],[-9447],[6665],[7445],[6344],[5590],[-6385],[-9890],[-4735],[4328],[5567],[-6693],[5639],[-2007],[7158],[-6035],[-3710],[-768],[6165],[8616],[-2948],[-2518],[-3363],[-3757],[3903],[-3680],[4346],[-8041],[2506],[3165],[-3237],[-8891],[-8509],[6719],[8652],[-7702],[-9995],[499],[-529],[2213],[-7283],[-4957],[4998],[-2429],[-5782],[-6593],[-1421],[-7114],[-2971],[-750],[-3937],[-9439],[-4744],[4046],[6134],[-9306],[7945],[4264],[5777],[955],[-7485],[-8507],[1990],[185],[-6248],[-7195],[4752],[-4660],[277],[6844],[3263],[9788],[5341],[-1184],[563],[5209],[5835],[-5864],[-5131],[-7689],[103],[4445],[8338],[2190],[7684],[-8754],[9639],[7032],[-5629],[3194],[-6421],[-4871],[-6610],[6519],[510],[-8787],[2549],[677],[3823],[9397],[-7866],[-7640],[170],[6971],[-1707],[6821],[-8954],[-324],[877],[6119],[4004],[-3872],[3291],[-358],[8952],[2555],[2237],[3096],[-8032],[5053],[892],[3078],[-637],[-9350],[-3193],[-9186],[7786],[1672],[-5791],[-1128],[-2014],[5088],[1034],[-87],[-3459],[-4928],[1464],[-2834],[2272],[-6158],[-551],[-346],[-944],[865],[4170],[9090],[-9049],[1500],[7137],[-9442],[-6254],[-6643],[9261],[2012],[9151],[1477],[-4614],[9182],[-2680],[8095],[-8211],[3715],[5774],[-2709],[-2622],[-5606],[1745],[-2277],[3363],[-2859],[-7809],[4652],[-2863],[-2573],[-9197],[-8429],[-7287],[-3155],[3654],[3876],[688],[-9423],[8708],[7644],[2767],[-948],[-7824],[4667],[-4430],[-9109],[3450],[552],[8629],[-4856],[-2976],[5444],[-9593],[4002],[-7355],[2922],[-3049],[-9336],[-9558],[-8362],[723],[2228],[6183],[592],[-4273],[6539],[6836],[2197],[598],[-5774],[1465],[-5521],[6891],[2044],[172],[-2989],[-5292],[-1224],[9941],[-4446],[7669],[5206],[-9104],[6331],[-5545],[-7978],[-9722],[-2468],[4808],[-4663],[5518],[-8339],[2452],[-2151],[-7444],[-3683],[-1486],[8696],[3510],[9449],[3995],[5636],[-9873],[751],[-8387],[-4638],[1265],[1559],[6907],[8028],[-5],[-9924],[-9807],[-4335],[9268],[5923],[8758],[1756],[-4516],[7439],[-4268],[6205],[5866],[6447],[562],[-2323],[-6410],[1909],[-7249],[-4282],[5206],[-1882],[-6231],[-2972],[2763],[-4977],[-2672],[9007],[265],[9942],[-3627],[-3729],[3875],[5518],[-9643],[4399],[8231],[-4196],[-9606],[8766],[8954],[1073],[3283],[-6244],[-8078],[-6146],[5925],[-4931],[-179],[4876],[-1708],[8917],[4363],[7262],[-7879],[-5866],[-55],[-8706],[-2269],[-4898],[-6198],[-4537],[-5333],[-919],[81],[-8352],[-6022],[2958],[5374],[-441],[1189],[4871],[-572],[-7755],[-2799],[-4577],[7761],[9724],[-650],[8980],[-4879],[768],[3592],[8346],[-5768],[-1306],[289],[-2572],[7242],[55],[1645],[343],[-893],[-976],[4495],[-7230],[-6841],[-2855],[-8090],[-6492],[-6454],[8892],[-724],[-2688],[616],[-4445],[1503],[-6032],[2250],[-3832],[-2495],[-6322],[-9566],[1349],[-8697],[-1322],[1253],[9454],[-3295],[-4107],[8126],[8592],[-2862],[8895],[2958],[-283],[-2880],[-650],[-5714],[-1256],[-2771],[-2009],[-3977],[-2713],[474],[717],[-8353],[-313],[-3765],[7030],[-6723],[7396],[-1671],[-3912],[6845],[-985],[-5530],[7804],[3514],[-8791],[4167],[-6798],[-4034],[-1286],[8978],[1492],[3813],[9062],[-4958],[-174],[-4742],[1490],[-2987],[7623],[1994],[214],[372],[-7869],[8777],[7732],[-3991],[7212],[-9252],[-2418],[6347],[7996],[9679],[-7677],[-4373],[-5754],[3573],[-5656],[5141],[7908],[-9588],[-6903],[-944],[-317],[5779],[588],[-1748],[3782],[2411],[6384],[236],[660],[-9601],[2442],[3448],[501],[964],[9625],[-5176],[-7799],[6951],[-9878],[-3490],[6313],[4503],[-9303],[-3453],[8176],[9334],[9057],[2497],[6332],[-2193],[7088],[5755],[-5200],[-7198],[703],[-2312],[-5131],[-4834],[7514],[-3999],[8901],[2352],[-2730],[9657],[6914],[7056],[3429],[1045],[9766],[7656],[7674],[-8901],[5724],[3884],[9086],[8639],[-1045],[8433],[-252],[-4557],[-8045],[6234],[-7735],[1210],[5715],[-1239],[4590],[-9635],[109],[1565],[-4392],[5322],[766],[-3566],[5658],[-6884],[-9342],[-5661],[7737],[6702],[779],[-8729],[-7351],[-8464],[663],[-8960],[9800],[-1215],[8623],[-8280],[-6698],[6696],[3668],[-8309],[8288],[-3293],[6617],[-6612],[7742],[-396],[8686],[8104],[-4494],[-5666],[-365],[3825],[9929],[-9729],[5913],[8684],[5023],[8729],[-8364],[5426],[-131],[-7003],[-8890],[1965],[-7134],[-4259],[4532],[7755],[-3309],[5893],[-1695],[9761],[-4185],[-3029],[8360],[-4691],[6233],[2976],[7224],[-1524],[7626],[-259],[-4577],[8697],[-2152],[-2707],[8979],[6428],[7527],[6275],[-579],[6856],[-5282],[2872],[6084],[-4739],[7251],[-9109],[5448],[-3135],[3288],[2852],[8258],[-5009],[-9990],[2279],[-6063],[4881],[-3113],[-6837],[-8283],[-1300],[-3728],[-3525],[-1535],[-1976],[-2088],[9427],[955],[9083],[-7381],[-5108],[-8091],[-4141],[-8610],[1266],[9227],[-9628],[8797],[6241],[-5668],[5561],[-1883],[-7147],[-18],[-6466],[-8390],[5466],[5711],[2148],[-9026],[-5238],[-3609],[1117],[6070],[2059],[-933],[-2380],[-4825],[-7034],[-9819],[5406],[8835],[-1666],[6448],[6009],[-413],[872],[3310],[-1631],[8767],[-2465],[-789],[-9220],[-6503],[-8892],[-2587],[-4953],[6495],[3073],[8402],[-259],[-725],[3461],[-6334],[-6422],[-885],[8701],[9298],[-163],[-7961],[2004],[-5910],[8092],[-6489],[-4147],[-7378],[4713],[1558],[-6335],[-5600],[6081],[586],[437],[9753],[7646],[-9233],[9738],[1206],[-7550],[-8669],[-5122],[-3967],[2168],[3304],[-5422],[-7439],[1331],[7989],[-8410],[9281],[8707],[3706],[-4337],[7827],[-9904],[5685],[-3888],[4138],[2715],[6170],[3275],[-3272],[-4246],[-4736],[3112],[-7617],[1136],[-6565],[-5706],[-730],[-9971],[-8155],[-8882],[-4768],[7044],[-8247],[3654],[3073],[8066],[-4580],[-8282],[-5093],[5201],[9341],[-1151],[-3798],[8595],[-2553],[2065],[8168],[-4676],[5599],[5936],[-783],[-3357],[1184],[5952],[3407],[759],[-2084],[-405],[-386],[-1917],[-1843],[2281],[8878],[-7605],[617],[-3454],[5231],[-9444],[-209],[-8995],[2838],[7433],[-9916],[9103],[-9702],[3195],[-642],[9353],[7963],[2725],[-982],[-615],[-2397],[-7527],[-2414],[152],[1967],[4703],[-2251],[-679],[-6931],[-2112],[-8078],[-9749],[-2197],[6921],[6732],[7818],[4003],[5976],[6339],[9665],[4385],[4317],[2874],[3267],[4620],[5148],[7044],[-5709],[-7206],[-4697],[-3854],[1289],[-2564],[-922],[3214],[-5940],[-2481],[-7922],[4692],[-9340],[-6742],[-872],[-2560],[6807],[1163],[6600],[-2190],[-510],[345],[9777],[-5520],[-9711],[6959],[-4094],[6603],[5921],[1135],[-6782],[5784],[-1388],[9675],[6671],[4878],[2137],[-6997],[8463],[-1923],[1907],[6060],[-1354],[-1469],[6765],[-6302],[-9894],[2170],[-2199],[1497],[4253],[-6639],[-1383],[8],[6988],[-3915],[7148],[20],[-2161],[9503],[9608],[-7816],[8972],[-7733],[1133],[775],[7084],[6404],[-7101],[1301],[-9823],[-3074],[6683],[7652],[3497],[-307],[9132],[3067],[7052],[-8120],[8722],[4708],[-4279],[4000],[-4559],[-1959],[6607],[1831],[-8970],[1714],[2539],[-9732],[-1427],[-1670],[-4902],[7217],[8813],[-493],[7051],[4678],[-9279],[-2535],[6404],[5582],[-7405],[-8608],[9712],[-5969],[8594],[-2853],[820],[8299],[4257],[-5256],[-5665],[-1087],[2312],[901],[-8162],[1812],[1646],[-320],[-9706],[-6743],[3974],[-9789],[7276],[-26],[319],[4045],[8092],[-455],[5956],[-8164],[-818],[6602],[4409],[-6679],[-724],[-1441],[969],[-6280],[6849],[6212],[9499],[-6565],[8240],[8161],[6857],[-8643],[8947],[952],[-7467],[-9193],[218],[9630],[-2606],[2335],[-9523],[-2647],[28],[660],[4438],[3663],[8163],[-593],[7540],[-4823],[8028],[-2615],[2389],[6911],[2411],[8967],[9643],[6775],[-2907],[9302],[-1899],[-7435],[-5792],[-5106],[-8068],[-2843],[-2610],[-1755],[9660],[-3761],[8643],[5247],[-7388],[-1236],[-8742],[-6140],[3205],[-5134],[7139],[5436],[5154],[-2125],[-2822],[-9709],[-5232],[5628],[-9396],[2963],[6946],[-4540],[1369],[9470],[-6239],[5537],[1953],[6484],[2405],[9930],[-6584],[210],[2561],[622],[-9521],[-3911],[-3676],[6996],[-9453],[9650],[-4115],[-2872],[7800],[9433],[1905],[-839],[-6459],[7665],[-3419],[8626],[6125],[7053],[-7913],[-4536],[779],[5890],[-7635],[-2689],[-7049],[-6310],[-4894],[-6615],[-5368],[-9261],[-5638],[-6736],[8626],[6210],[9904],[4819],[-5788],[-5605],[5322],[4107],[4739],[1538],[398],[-8220],[-1212],[4202],[-6584],[1230],[-3077],[9797],[6203],[-6849],[6132],[1589],[-4504],[9175],[9800],[-4024],[-4504],[-9504],[-9694],[-8363],[-6921],[-2692],[-4726],[560],[-7287],[-3327],[5657],[-8358],[-4138],[539],[-1817],[-3517],[2449],[8576],[-1278],[-150],[2217],[-747],[-6470],[1276],[-4573],[-8298],[9632],[-5371],[-9843],[-2181],[4466],[9913],[5867],[-7818],[5688],[5287],[-2769],[1628],[9991],[554],[9450],[-1351],[4150],[-4214],[6401],[-4693],[4952],[5503],[-6833],[1749],[9415],[-9649],[3045],[-7827],[-763],[-223],[8089],[5503],[-2294],[2969],[-1133],[-1698],[-6381],[-6146],[-599],[-5762],[6671],[-394],[-6859],[8237],[8133],[6239],[-7640],[942],[-2964],[-8503],[6761],[-8335],[-5253],[-4761],[7517],[1934],[9898],[-6687],[3121],[-1411],[7948],[-5365],[4585],[-3940],[8591],[6380],[4043],[942],[-9820],[-1827],[7750],[6377],[4638],[-745],[8001],[9212],[3274],[2769],[-4149],[-5586],[1487],[7152],[-5119],[-9508],[-5837],[2126],[9308],[8824],[-4483],[4253],[3206],[4365],[8238],[-6602],[2141],[-6024],[7938],[-3145],[-4321],[-2402],[2307],[5102],[-5840],[3558],[-8256],[-4252],[-5978],[-7376],[-7657],[-4560],[7007],[5066],[6222],[6520],[-151],[3729],[1250],[4383],[2122],[-5103],[5590],[6652],[3724],[1875],[9590],[9093],[8747],[431],[1071],[-4464],[5598],[2341],[-8111],[6622],[-9876],[-1780],[4877],[9798],[1675],[-5172],[8711],[-5007],[-6600],[3163],[-6119],[4737],[-2433],[-2106],[4495],[2320],[-8828],[5066],[-7604],[-401],[-3339],[674],[6747],[-1874],[-2549],[-5643],[7557],[-5718],[4019],[1342],[7795],[-5598],[-4672],[348],[5498],[-1834],[-646],[-4835],[788],[-6509],[-9895],[-1436],[-7773],[-2466],[-6834],[-2877],[-1358],[-1968],[-3590],[6546],[-6316],[3946],[-3249],[6521],[8526],[5525],[5037],[-3752],[-7647],[2459],[-4064],[4590],[1080],[-4698],[1008],[-6949],[9386],[7194],[6983],[7002],[-9226],[2617],[-8872],[873],[-8637],[205],[-824],[-1012],[-6825],[-7239],[-795],[-1751],[9773],[-2386],[-4674],[8971],[-2978],[3723],[6213],[-4493],[-7913],[-1181],[6357],[5529],[13],[-9211],[4215],[1989],[5830],[4317],[5000],[4194],[1056],[3934],[-9502],[-6597],[-6571],[8097],[-8219],[-706],[-3791],[1646],[-1246],[5123],[7949],[-3827],[-7160],[5195],[1484],[-1749],[-9714],[-9708],[-160],[1361],[5340],[3838],[6222],[-6609],[-8876],[-7826],[-8040],[9858],[7099],[-9600],[7909],[-7622],[-168],[1476],[-5625],[5072],[6343],[-4622],[9450],[-7884],[7348],[4818],[-4544],[3566],[-1628],[-7780],[4643],[3338],[-4924],[-4219],[-604],[-5032],[8187],[-5439],[9853],[1922],[-5806],[7460],[-752],[-2146],[8334],[6276],[8995],[-3817],[7127],[5331],[-401],[-1173],[-552],[8304],[6063],[8930],[-7080],[7393],[8817],[2055],[5787],[9182],[-740],[4825],[2157],[-694],[2403],[6214],[3659],[3778],[-9150],[-8477],[6708],[-4113],[2793],[-1824],[-6948],[9208],[1267],[-1998],[-2928],[-8309],[8098],[-8514],[2534],[-2562],[-2163],[3490],[-6589],[8737],[304],[-3798],[-7296],[4568],[-3707],[3609],[-4483],[4556],[-2065],[2098],[-7072],[-7602],[10],[-3036],[7711],[8373],[7470],[-3140],[870],[-5136],[-2202],[6026],[9883],[2448],[-7554],[-8368],[4447],[-8950],[-9354],[6192],[849],[-9463],[-7176],[-6385],[-3523],[-9328],[4985],[-4286],[3527],[-5309],[-3214],[943],[-8877],[-1778],[2401],[7494],[-1147],[-4694],[-7238],[2737],[-3717],[-4722],[-6820],[1696],[-9994],[9542],[3890],[-9710],[-1697],[3017],[-785],[5794],[-1715],[7138],[-4988],[-5252],[-5461],[1160],[-2939],[2774],[688],[8901],[1613],[-988],[-9477],[7688],[9674],[4399],[9669],[6172],[2052],[7469],[2249],[5021],[7079],[163],[-7429],[53],[9621],[7680],[-5032],[8482],[-9718],[2042],[1738],[-2866],[-6506],[-3545],[4895],[1393],[4370],[-9862],[1881],[-331],[6261],[1061],[6169],[-9569],[5829],[-1275],[7659],[4805],[-3535],[-1720],[-4198],[-2266],[-8508],[-5149],[1160],[-6191],[-5071],[-5119],[156],[9974],[5356],[-4564],[3607],[-8014],[-4179],[5729],[2621],[-6131],[6356],[-6726],[-5120],[2714],[4881],[-2014],[4606],[1530],[6474],[8791],[-1251],[-9460],[-7199],[-8903],[8821],[8501],[1463],[734],[1734],[2190],[-404],[-4896],[7878],[4892],[9863],[-6956],[-4817],[-569],[-980],[9734],[9933],[4870],[-318],[-9310],[-1034],[-5563],[595],[470],[-6628],[-4533],[-6951],[-9302],[-6132],[4956],[364],[9760],[-2115],[-1779],[-5891],[152],[-2994],[5002],[-653],[3953],[3045],[-849],[5135],[-3883],[-5708],[-7761],[1478],[-2094],[3961],[-3296],[-5327],[6457],[-3998],[-8746],[1566],[-1988],[-4198],[-6899],[-4810],[7959],[-6070],[-6004],[-1682],[-8032],[-5942],[5060],[-2693],[-9111],[2710],[-2121],[-6219],[-4466],[8751],[4408],[8741],[-5549],[870],[-6922],[-1582],[-3550],[8905],[7308],[1958],[-1011],[-591],[2712],[5755],[688],[4905],[-9272],[-8668],[6888],[5933],[5074],[9704],[5196],[-8148],[-6585],[-1462],[7974],[-3316],[-9339],[3135],[-7529],[9964],[3957],[5610],[-6192],[-5055],[8379],[-764],[7034],[8884],[8021],[-6411],[-723],[-5238],[9410],[7032],[-8807],[-3243],[4723],[7390],[5004],[-1395],[151],[6709],[3995],[-4321],[-4546],[-7078],[3241],[808],[-7693],[-8564],[4827],[-3189],[-3123],[7361],[3606],[280],[3665],[-6455],[-9620],[9948],[8320],[8740],[3635],[8537],[-3485],[2931],[-9225],[1081],[4955],[-6450],[1070],[-9880],[6813],[-6563],[4890],[783],[-8780],[4583],[6031],[-9184],[4011],[-7056],[-629],[-4293],[4057],[-1480],[-9407],[-3497],[2742],[-2804],[361],[-1741],[-5181],[7142],[-3184],[1743],[-3138],[268],[7697],[437],[-1717],[-2009],[6489],[819],[8085],[7876],[4942],[-7358],[3657],[-3980],[-1547],[8833],[1900],[-1456],[3872],[7016],[42],[-9376],[9418],[-3836],[5145],[9803],[-9053],[7466],[-42],[-5165],[-8629],[-1118],[1767],[5572],[5182],[-7121],[3867],[-2084],[5497],[-3739],[202],[9545],[-435],[-2889],[-2816],[2863],[566],[-814],[-1651],[-4079],[3286],[-9912],[-4358],[-8304],[6837],[-802],[-8498],[-9293],[-237],[4475],[-8765],[-9131],[1599],[5488],[-2296],[7467],[4295],[-5099],[-1305],[-9040],[-2325],[-4268],[4310],[6308],[-8236],[-6561],[6718],[2540],[3053],[1002],[2536],[-7160],[4874],[4577],[7785],[-8657],[1956],[-1213],[7745],[-460],[2225],[482],[9276],[-3140],[2718],[-9074],[9261],[-4314],[4554],[-5056],[7905],[2206],[6916],[-6420],[-6170],[-6802],[-1389],[7233],[-2329],[-7531],[9370],[-9178],[8677],[-4756],[3919],[7721],[-2474],[5856],[-3047],[4913],[-6222],[136],[-3515],[-1469],[4984],[-5356],[6861],[-4337],[-3818],[-1937],[-5060],[-134],[-5393],[3089],[3195],[9462],[-3121],[4755],[4589],[9283],[9185],[-6270],[9059],[-5346],[9426],[-4437],[9926],[-5506],[-7425],[1490],[-8294],[9747],[8342],[9662],[7355],[4101],[-1442],[4618],[3074],[3369],[-979],[-8733],[2920],[7238],[-9858],[-5412],[-8930],[2004],[-1057],[-9157],[-8341],[693],[8051],[-3795],[-117],[-6585],[-7970],[-2297],[-7235],[-9374],[3573],[6917],[5347],[-9463],[-8593],[-7458],[5527],[1621],[7024],[2154],[630],[4015],[-1093],[7524],[5668],[4263],[-1827],[9012],[9562],[6027],[-8163],[1330],[468],[-9511],[937],[9119],[-1934],[8760],[-3163],[9151],[-6929],[-2281],[2480],[-9093],[-9158],[-1220],[-8818],[3475],[-6831],[-8567],[-8587],[8071],[4236],[3486],[-5933],[5891],[8962],[6983],[-5831],[5549],[-591],[-9469],[6262],[9645],[4003],[8234],[5348],[-3336],[3936],[6217],[-4383],[-7973],[-8161],[-5525],[8963],[-2769],[6735],[1910],[1712],[-770],[-9231],[-7149],[9584],[-8510],[-1732],[3908],[6573],[-804],[-1950],[819],[2139],[6030],[-6381],[3301],[-3041],[8243],[-2098],[-3379],[-5631],[-5469],[-8081],[8732],[2690],[3099],[9086],[3798],[-3805],[2082],[5722],[-2628],[8061],[7093],[6312],[1218],[1945],[-2082],[66],[7425],[2251],[9895],[3373],[1069],[-3528],[2388],[1973],[-1292],[-6113],[-3127],[-8962],[9138],[-9204],[1920],[-8244],[-7497],[9748],[-883],[-594],[2633],[9584],[6356],[-4961],[9009],[1666],[-4639],[3460],[7828],[-4265],[-3018],[-6454],[-3856],[5857],[-6210],[3775],[-4134],[3360],[1157],[-2721],[3324],[7732],[3671],[-1391],[287],[2797],[-9634],[2713],[-5706],[-9150],[2190],[7275],[621],[-1569],[-9085],[7688],[7159],[-764],[7246],[6305],[6915],[1282],[-7738],[5972],[6436],[6439],[-2878],[6521],[-8689],[-1711],[-1603],[-5509],[-8088],[-8328],[967],[9028],[-2425],[4556],[9031],[-9072],[-2816],[661],[-2797],[1281],[-161],[-1253],[165],[-2079],[-8427],[4630],[5125],[-8623],[1719],[-2548],[-9100],[7754],[-2878],[6963],[2187],[1676],[-1929],[3294],[7453],[6549],[-7512],[4165],[-4114],[-1129],[-310],[6962],[1504],[8888],[5890],[-5939],[8725],[8018],[8988],[-3705],[1834],[-740],[-7315],[-3213],[4507],[8617],[-5494],[5346],[5749],[-7532],[6043],[-7064],[5093],[-8228],[210],[5500],[3017],[-3494],[7226],[923],[3149],[8710],[478],[1329],[-8444],[-5878],[5515],[-5022],[2745],[2568],[-4459],[-7949],[585],[6879],[-8290],[1833],[-1581],[-3586],[-3579],[4901],[6286],[3064],[-9070],[-9851],[2374],[-8395],[-113],[-6339],[1653],[-9049],[4192],[2867],[-1359],[-7109],[980],[-3540],[9000],[7372],[8997],[4752],[7980],[8148],[9488],[-8142],[7757],[-7807],[-3561],[5123],[617],[-6965],[7307],[-8844],[7389],[1184],[-5714],[9312],[-2247],[-7443],[5649],[3624],[8562],[3149],[-3462],[-1527],[6540],[-551],[-9940],[2460],[-7235],[-1602],[-5139],[-6248],[4501],[-7636],[6115],[-137],[-9574],[-7572],[4869],[1673],[-5839],[4650],[4082],[1176],[-606],[-3607],[9654],[-7186],[8102],[6477],[9598],[-8608],[-4519],[4982],[-7333],[-4610],[-3305],[-7004],[-5675],[5646],[6788],[-8736],[732],[-2697],[-7207],[-358],[1627],[1697],[9571],[-1453],[2013],[-8600],[-4472],[566],[-9381],[9899],[7144],[-2473],[-1597],[-940],[9945],[5410],[-7338],[9820],[4368],[7041],[2411],[5210],[-1705],[-915],[-2017],[-1678],[5018],[-2711],[9351],[6050],[1862],[1077],[-4515],[5928],[5006],[-2748],[-332],[728],[-476],[3073],[-9854],[-4038],[-9380],[-5649],[-5120],[-9976],[6500],[-6159],[2420],[4572],[-1633],[7619],[5192],[5406],[8220],[-1087],[-7695],[8253],[-858],[1723],[-7000],[-3249],[4877],[5460],[9061],[-2566],[-1683],[4282],[-3163],[-3515],[-2957],[9406],[-9226],[1207],[3497],[-4302],[-1293],[1961],[6386],[-7889],[-7233],[6617],[-4756],[9793],[2430],[-2405],[-4599],[8194],[599],[2243],[-2657],[-3412],[-374],[3844],[-9445],[-2535],[-4811],[-1836],[4480],[-1283],[-9888],[3940],[-1736],[-9281],[8326],[7213],[-2581],[4509],[4751],[8370],[-5581],[-7427],[-4437],[3833],[-2416],[-2830],[-6890],[-3231],[-3917],[5818],[-7038],[-5238],[-1381],[7549],[9366],[167],[2118],[1980],[-4798],[2636],[-782],[4791],[2026],[-2125],[-3652],[3831],[319],[-9638],[319],[7220],[-3625],[-3962],[6740],[2675],[-9337],[614],[-1672],[7380],[-6398],[267],[-1602],[425],[-7064],[-4481],[8006],[-8470],[2893],[5488],[-556],[-7318],[-3991],[-5953],[-3977],[5177],[4029],[6971],[8395],[-593],[-5844],[-5694],[2193],[714],[4899],[1552],[5112],[2069],[2378],[2412],[-9041],[2599],[5874],[3857],[-9343],[-7623],[5047],[8421],[9756],[-8277],[-7451],[285],[-8904],[931],[-1572],[-8819],[8817],[-7882],[5279],[-7522],[-2219],[954],[1499],[4772],[6931],[7339],[-2069],[4152],[-9300],[9486],[5045],[4690],[-441],[4885],[3178],[-7774],[7809],[591],[-9980],[-3492],[3664],[5546],[4954],[6618],[8931],[-3115],[-3970],[-6778],[-9607],[9184],[76],[-9132],[5522],[9675],[9609],[3008],[6584],[-7317],[-9495],[9016],[-3792],[8232],[-1200],[-1579],[6902],[8358],[-8520],[7216],[-8617],[-332],[9139],[3630],[1019],[1366],[6158],[-269],[-5141],[-4651],[4537],[5225],[-6966],[-9386],[-2581],[9040],[2069],[-8439],[6306],[-3886],[-7194],[-4715],[4579],[696],[6410],[7088],[-8273],[-3035],[5966],[-7155],[-2483],[-7384],[8504],[3035],[-3565],[8524],[-8458],[-62],[6723],[1243],[-2498],[-8003],[173],[3940],[4951],[-303],[2712],[5787],[-7630],[4694],[346],[-7666],[-8180],[-1516],[-2253],[4092],[3193],[1926],[-3928],[5038],[-2994],[-1366],[-437],[-7252],[2721],[109],[2143],[-3927],[-6900],[6650],[-4492],[8670],[-80],[-9095],[6372],[-9865],[-2637],[-3050],[5052],[-1344],[804],[-5573],[7144],[-8625],[376],[-190],[5996],[238],[-112],[3626],[-877],[8092],[828],[8274],[2714],[1229],[5064],[6639],[5437],[-5541],[9177],[-58],[-3231],[-4476],[5377],[-3800],[8031],[7854],[-1065],[4370],[890],[-382],[1452],[-542],[-9156],[-154],[5109],[-7767],[-4373],[-3022],[-6613],[-6152],[6221],[-3096],[-6560],[-9848],[-9015],[-8553],[9337],[-5000],[-5816],[-6659],[-4738],[-7847],[4624],[9568],[-4260],[-3851],[-164],[-8886],[8575],[8772],[5250],[2243],[-8899],[7321],[-9850],[1989],[-224],[-1103],[5128],[705],[-7842],[-2666],[317],[3001],[9202],[8974],[-6314],[-7975],[-7908],[-2516],[-301],[-9264],[2836],[-9915],[-347],[4142],[9185],[-1510],[-1460],[1963],[2277],[-9033],[-4032],[2579],[2625],[4179],[9135],[410],[-2168],[-9381],[632],[-2099],[5878],[-441],[-5445],[6158],[1448],[-637],[2498],[5304],[4934],[-8743],[7039],[1947],[7763],[8079],[-5441],[5803],[7744],[-3063],[2716],[-8424],[-9270],[4324],[3764],[520],[-5018],[9413],[-397],[-2143],[6235],[-7788],[1930],[-7894],[5230],[4482],[-2258],[3209],[9602],[1043],[3482],[-2649],[2686],[-942],[1851],[3198],[-7815],[-2289],[-13],[-2525],[-9138],[-3896],[-5460],[8494],[6944],[-2990],[-677],[-8933],[-5995],[5684],[135],[-1091],[6654],[4963],[-5443],[6610],[-9838],[-3784],[8635],[-2363],[-6264],[1468],[106],[-9750],[6805],[-1053],[2672],[8801],[-7422],[2159],[6460],[1539],[-9548],[-1034],[5931],[-2918],[-516],[-6685],[6789],[-1011],[5482],[9324],[8248],[-9411],[-6450],[1013],[8506],[9719],[2771],[-119],[-1506],[-6021],[-242],[5177],[1010],[9920],[-9874],[6357],[3722],[-8314],[7860],[2259],[-3290],[-3208],[-4935],[-7127],[-7064],[-90],[-9239],[735],[8060],[4866],[-636],[6458],[-6375],[2715],[-466],[7402],[9062],[488],[-7269],[2003],[-3200],[-8275],[-8264],[8988],[174],[-4863],[5903],[-115],[2360],[912],[-9835],[1869],[-312],[3089],[2459],[2758],[-2029],[6723],[9766],[6554],[-113],[-6304],[-9817],[-6377],[4830],[-5198],[-6531],[-7213],[-6933],[9245],[3898],[5792],[-6814],[39],[7697],[-7098],[3572],[-2499],[6734],[-9614],[2592],[1725],[1239],[174],[3752],[7863],[9787],[7879],[9684],[-4870],[3025],[9765],[-7054],[-8613],[-3587],[5381],[-3859],[-2677],[8374],[-2728],[1350],[-3061],[-8865],[-9968],[-1268],[-7634],[6849],[-8957],[2146],[4422],[-8350],[3635],[4067],[-621],[508],[-1847],[-4290],[7344],[5912],[7767],[3225],[7988],[8823],[3569],[4621],[-6429],[-2936],[31],[-8429],[1675],[5546],[7182],[1338],[1464],[2796],[9065],[-2602],[9752],[4995],[-8987],[8297],[1157],[-3220],[-8270],[2126],[-4805],[-8115],[-5601],[4282],[-3167],[-106],[-1663],[9518],[8906],[1930],[5236],[6122],[5564],[2786],[-9430],[-5704],[-2244],[190],[-3512],[6117],[724],[8305],[8518],[9588],[6303],[-6513],[6365],[6198],[2790],[2267],[-3590],[-5412],[7977],[-8942],[875],[-322],[9239],[7780],[-8924],[7906],[-6844],[5600],[393],[3944],[-1599],[-2850],[-7169],[8321],[7513],[-87],[7962],[524],[-1052],[1212],[8378],[-4292],[-3879],[-8939],[-8187],[-2406],[-6189],[5606],[3531],[3885],[-3676],[9110],[6191],[-3546],[-8503],[4772],[9487],[1148],[6079],[-1940],[2970],[-2820],[5350],[-9311],[669],[3175],[240],[9310],[3053],[6158],[2462],[-216],[6754],[3799],[2914],[-2564],[-61],[4199],[8715],[-881],[1393],[-1548],[4689],[7957],[-8157],[-9855],[-5717],[-7330],[-6748],[-453],[-7933],[5644],[578],[4430],[5291],[-7713],[3535],[9495],[-769],[633],[-3436],[-954],[6573],[-3000],[8180],[-17],[3808],[-2959],[-1648],[-5735],[-6399],[9344],[2206],[1736],[-1681],[-4128],[-7771],[5369],[5832],[4124],[1097],[-6398],[-1573],[-7651],[5650],[-4302],[5959],[8139],[-1559],[-4822],[5480],[-2713],[-7230],[-7901],[-635],[-2522],[4311],[-6068],[-1872],[7304],[-1597],[-5030],[-253],[3427],[-5444],[-9521],[1404],[-9079],[-4913],[-2980],[-8792],[-4586],[-7193],[-8421],[-7067],[-2166],[-7211],[4074],[-21],[6367],[-7208],[-4196],[-9762],[6933],[1805],[664],[4494],[-9028],[-402],[5656],[-555],[-9534],[1803],[842],[6654],[-6757],[-8856],[-2287],[-8588],[1177],[9326],[3142],[1781],[-9342],[-1098],[-9801],[-1934],[-7545],[3213],[6968],[7896],[-9085],[-5983],[-6425],[-181],[9025],[5971],[4074],[4802],[-2048],[5391],[2258],[-1356],[9007],[8407],[3643],[3228],[-3813],[-9438],[6308],[-341],[-8162],[8302],[3566],[-9851],[5949],[-4497],[-8824],[-3662],[-4844],[9757],[-1619],[9571],[6559],[-520],[-5195],[3336],[-792],[5812],[5395],[-5435],[-325],[8313],[9585],[-7050],[-4006],[2102],[-5690],[801],[-1446],[-7390],[4315],[-5350],[-3467],[-7345],[-6988],[7457],[-5709],[-3604],[-3600],[-5960],[-3132],[6640],[-7677],[1316],[8544],[8999],[-8127],[-1207],[5270],[-8400],[-8894],[-50],[-9858],[2632],[-9498],[-7230],[9034],[-8455],[2683],[7982],[-6788],[347],[7764],[3443],[3129],[873],[7753],[-3737],[-3381],[-4745],[8932],[3679],[953],[5429],[745],[2264],[1938],[3876],[9860],[-9958],[9508],[3944],[202],[933],[-939],[7928],[1101],[-871],[5264],[6004],[-6502],[-3136],[-8805],[-5035],[7177],[9176],[-2683],[-1722],[1946],[7321],[4869],[-9003],[-5815],[4374],[3632],[-8001],[8366],[7162],[6973],[3167],[-5403],[-3194],[-8295],[5559],[-7614],[-1270],[4908],[-3063],[-3355],[-9395],[-9129],[-8176],[1647],[9778],[-7593],[6860],[-3238],[8771],[3902],[-6037],[9839],[-8184],[7769],[-8814],[1171],[-5428],[2244],[-1638],[-1178],[6640],[-4072],[-7040],[1898],[-4215],[7270],[-4961],[6647],[-4892],[8928],[5840],[3053],[-6496],[5658],[-8398],[5255],[-3298],[-8250],[-6296],[-4497],[8778],[3719],[-7064],[4479],[-1873],[-4212],[-1510],[-4567],[2503],[-4287],[840],[6409],[4052],[-1964],[7727],[-690],[-5085],[6302],[8803],[-2389],[2928],[-9385],[-3662],[-3362],[-2487],[-9649],[-1349],[-114],[-818],[-7302],[9654],[-8526],[1030],[-1606],[-3915],[2307],[-2514],[3066],[5909],[9825],[-5825],[7188],[-2227],[5165],[5166],[-541],[4299],[-6969],[7019],[5220],[9023],[-3960],[-3416],[-7703],[5576],[-9702],[926],[-8492],[-6739],[-656],[-5115],[-5904],[2152],[5832],[-6477],[7509],[6949],[4285],[-1245],[-5083],[206],[-2397],[2560],[-2207],[3252],[-9344],[-4313],[-7638],[-6487],[4546],[3621],[5642],[-6769],[-8445],[1578],[-3540],[3841],[3391],[7509],[6893],[-3938],[5821],[9714],[-5964],[2614],[-8334],[-6550],[-7380],[7629],[3793],[-6820],[-7628],[8646],[7376],[-7249],[-5487],[2719],[-22],[-2105],[9173],[-2664],[5331],[825],[583],[-3691],[-6651],[5301],[-5130],[-609],[-298],[9034],[-852],[7946],[-4859],[-4897],[-6563],[-5402],[7227],[7947],[8315],[-870],[-5137],[-2980],[-5204],[3624],[-5101],[-6679],[-4698],[-8112],[-8927],[2468],[2571],[310],[9588],[1390],[9179],[8340],[1707],[3820],[-9663],[-3967],[5166],[9948],[-7987],[4720],[-8395],[-1691],[-3054],[-6911],[1054],[4685],[-680],[5753],[-8583],[-3437],[8609],[5956],[-6216],[-8391],[699],[-7476],[9792],[-2736],[6971],[495],[6841],[360],[-668],[260],[1505],[9262],[26],[6691],[-1720],[8846],[791],[-3912],[6367],[5311],[6502],[-6243],[2528],[-4999],[5369],[-4520],[8621],[-2075],[-24],[-4041],[-2588],[-454],[8882],[-6487],[9481],[-9125],[-9320],[9727],[8501],[-9228],[5319],[2909],[9621],[-9973],[-8560],[-6674],[2113],[-4316],[6468],[7390],[7462],[5174],[1863],[-5190],[6837],[6622],[-3447],[8265],[-7221],[-27],[-7398],[-2271],[3278],[-5073],[6305],[-7654],[9575],[-9767],[-427],[-8602],[-3734],[-8943],[-3177],[-2545],[4049],[-8911],[-8703],[4816],[9329],[308],[-9254],[-7820],[-7174],[-4529],[-482],[9182],[-2528],[1495],[3660],[-8854],[-7337],[-7496],[-5283],[-5652],[51],[-2700],[-3584],[1143],[-4836],[-7161],[-3866],[7223],[-9227],[5817],[-8780],[-5908],[87],[6880],[5021],[8212],[-8062],[-5572],[-7675],[7358],[7169],[2331],[7315],[5236],[6381],[-1591],[-4300],[-8301],[-8167],[-1289],[6258],[4178],[-5082],[95],[7648],[7952],[-7919],[1317],[-8549],[2813],[211],[3984],[1683],[-8464],[-7049],[-5123],[-7577],[-3144],[7082],[-4985],[3529],[-8167],[-1255],[4161],[-8781],[8660],[-2242],[-1008],[985],[2286],[-2091],[-536],[-7284],[6528],[9146],[8221],[-3955],[6527],[-2866],[4133],[955],[-464],[586],[7595],[6888],[-3932],[571],[-3494],[2832],[8292],[-7403],[-3151],[4400],[3288],[-3763],[7616],[-9733],[8894],[8020],[4472],[6476],[7768],[-2415],[8517],[-2862],[-8344],[-1688],[5006],[-779],[3158],[3888],[-1400],[1717],[5159],[-1493],[6229],[628],[4648],[5285],[-9113],[9836],[-3357],[7948],[-3858],[-2245],[6331],[-4432],[-8739],[-9026],[3929],[768],[7516],[-5785],[-6309],[7742],[-9664],[-5207],[8853],[-3904],[-9383],[-2238],[-2309],[2531],[3624],[-6636],[-4366],[-9597],[-8946],[542],[-3262],[4916],[-2664],[1809],[-2271],[-4864],[-394],[-459],[-6967],[490],[1518],[-1428],[5087],[-1746],[9740],[2654],[1298],[-4189],[-4542],[3527],[8748],[-2590],[-7948],[-714],[4069],[-4445],[5607],[2466],[-6577],[5819],[-1012],[865],[6097],[5738],[5532],[-8688],[2436],[-1596],[2362],[-8921],[-8127],[424],[-4901],[-8742],[8994],[2862],[-8762],[-4596],[3616],[583],[1961],[7207],[-3825],[-1971],[-7453],[6783],[6065],[-2895],[553],[-8213],[5904],[878],[2212],[-8974],[5393],[-4790],[6242],[9388],[7771],[2783],[658],[-7558],[4790],[9557],[-526],[-1922],[3886],[-9584],[8990],[-5817],[-5182],[-3536],[8674],[-790],[-1157],[-4169],[2598],[-3366],[-2015],[4936],[-7549],[8474],[-9664],[2735],[-341],[-103],[3898],[-1654],[-6744],[-3653],[6938],[5359],[-1070],[4830],[8814],[-3788],[8871],[6947],[2228],[2355],[-4941],[2803],[4530],[3581],[-2500],[1102],[-9068],[-5708],[-1799],[-7730],[4747],[6400],[4739],[2048],[-4910],[2795],[-1364],[-7715],[2510],[-7201],[-2420],[5598],[-7472],[1312],[7493],[-4579],[-2760],[4217],[4395],[-8599],[9725],[3228],[5921],[5667],[-7548],[-3545],[970],[800],[1987],[-5244],[-9034],[-8233],[-1321],[537],[4724],[9186],[1207],[-5143],[5296],[9986],[4303],[3557],[-7968],[-1116],[6782],[-4600],[7626],[5610],[-4441],[3689],[236],[4591],[-8157],[-3704],[9958],[8778],[5005],[1451],[5181],[7573],[1805],[-2403],[3375],[2442],[7062],[198],[-1837],[-9176],[-3057],[-6512],[68],[-9698],[8000],[-6846],[4452],[6922],[-7304],[840],[6193],[-8023],[-1140],[1280],[1690],[3872],[-3453],[8736],[-2372],[2904],[-2560],[3520],[6703],[-7009],[-913],[-3110],[7108],[8490],[-2684],[-3754],[1659],[-2989],[9458],[5933],[4830],[3439],[-5427],[-821],[-6746],[-1508],[4736],[-364],[21],[-3599],[-2211],[-7742],[4018],[4105],[4463],[5368],[3907],[5839],[3436],[-6811],[-2771],[7918],[497],[-1543],[-46],[5937],[-8448],[-9191],[4980],[2060],[4973],[9328],[9428],[-7635],[-2764],[9705],[-1287],[4798],[-737],[7579],[8290],[3152],[-6772],[7835],[6377],[343],[-1908],[3336],[-7052],[-448],[-732],[-5660],[-4745],[7464],[4847],[-8097],[348],[4035],[-5390],[-9696],[3998],[-9737],[2637],[-1229],[-9359],[218],[-7113],[-1283],[2193],[9803],[-607],[-1980],[3985],[-1218],[8744],[-6432],[-3087],[-1979],[9247],[-4231],[-80],[-8600],[-8684],[-3691],[1504],[9741],[8888],[-2502],[-9232],[-7369],[2722],[8971],[-4198],[-2000],[-1089],[2345],[2300],[-1213],[9178],[2980],[-6607],[-2535],[7676]]
+- **Output:** [null,-9995,-9993,-9993,-9992,-9991,-9988,-9987,-9986,-9985,-9982,-9981,-9978,-9978,-9978,-9976,-9976,-9975,-9975,-9973,-9971,-9970,-9970,-9969,-9964,-9963,-9962,-9959,-9958,-9958,-9955,-9953,-9952,-9951,-9948,-9946,-9946,-9945,-9939,-9934,-9932,-9932,-9931,-9930,-9930,-9929,-9929,-9928,-9923,-9922,-9922,-9919,-9917,-9913,-9911,-9911,-9910,-9906,-9905,-9904,-9903,-9901,-9898,-9897,-9895,-9895,-9894,-9892,-9891,-9890,-9889,-9889,-9889,-9888,-9887,-9886,-9882,-9882,-9880,-9875,-9874,-9874,-9872,-9871,-9871,-9865,-9858,-9857,-9852,-9852,-9844,-9842,-9838,-9834,-9827,-9826,-9826,-9813,-9813,-9812,-9807,-9805,-9805,-9804,-9802,-9799,-9798,-9795,-9793,-9790,-9790,-9782,-9781,-9779,-9777,-9776,-9776,-9774,-9770,-9766,-9764,-9762,-9757,-9757,-9755,-9753,-9753,-9752,-9751,-9749,-9747,-9746,-9743,-9742,-9742,-9741,-9741,-9740,-9738,-9738,-9736,-9734,-9734,-9733,-9725,-9723,-9722,-9722,-9722,-9721,-9714,-9714,-9711,-9710,-9710,-9708,-9693,-9692,-9691,-9690,-9690,-9687,-9683,-9682,-9681,-9679,-9679,-9673,-9671,-9667,-9665,-9664,-9663,-9662,-9662,-9661,-9660,-9659,-9658,-9658,-9658,-9653,-9649,-9645,-9644,-9644,-9642,-9641,-9641,-9641,-9640,-9635,-9635,-9634,-9633,-9633,-9630,-9629,-9629,-9623,-9623,-9621,-9618,-9617,-9617,-9616,-9615,-9614,-9614,-9608,-9607,-9603,-9603,-9601,-9600,-9595,-9588,-9588,-9585,-9584,-9582,-9579,-9574,-9574,-9573,-9573,-9572,-9570,-9569,-9569,-9564,-9558,-9558,-9558,-9556,-9556,-9555,-9554,-9553,-9551,-9551,-9550,-9546,-9543,-9541,-9540,-9538,-9537,-9535,-9535,-9533,-9531,-9529,-9527,-9526,-9524,-9523,-9521,-9520,-9519,-9514,-9512,-9512,-9512,-9509,-9505,-9502,-9502,-9499,-9496,-9495,-9493,-9490,-9488,-9488,-9476,-9475,-9474,-9473,-9469,-9458,-9454,-9450,-9448,-9447,-9446,-9441,-9440,-9432,-9431,-9425,-9421,-9420,-9420,-9420,-9419,-9415,-9410,-9408,-9405,-9404,-9402,-9400,-9396,-9392,-9392,-9390,-9389,-9385,-9385,-9382,-9381,-9378,-9378,-9375,-9375,-9374,-9372,-9370,-9370,-9369,-9368,-9368,-9368,-9367,-9365,-9365,-9365,-9360,-9359,-9358,-9354,-9352,-9346,-9344,-9340,-9335,-9331,-9328,-9328,-9328,-9326,-9325,-9324,-9318,-9314,-9314,-9313,-9311,-9311,-9310,-9304,-9304,-9303,-9301,-9292,-9288,-9288,-9284,-9284,-9283,-9282,-9282,-9279,-9279,-9279,-9279,-9268,-9268,-9268,-9266,-9264,-9264,-9263,-9263,-9261,-9260,-9255,-9254,-9254,-9254,-9253,-9251,-9250,-9248,-9247,-9246,-9244,-9231,-9231,-9231,-9229,-9228,-9225,-9219,-9211,-9206,-9200,-9198,-9198,-9196,-9194,-9191,-9190,-9190,-9189,-9187,-9187,-9187,-9184,-9183,-9180,-9179,-9175,-9174,-9172,-9170,-9170,-9169,-9167,-9167,-9164,-9163,-9160,-9156,-9154,-9153,-9153,-9152,-9152,-9150,-9150,-9148,-9144,-9143,-9143,-9137,-9135,-9133,-9130,-9130,-9130,-9127,-9123,-9123,-9121,-9120,-9120,-9118,-9116,-9115,-9113,-9112,-9105,-9104,-9104,-9104,-9101,-9100,-9098,-9098,-9098,-9096,-9091,-9090,-9089,-9088,-9088,-9087,-9085,-9079,-9078,-9078,-9077,-9076,-9076,-9073,-9072,-9072,-9070,-9068,-9067,-9065,-9064,-9062,-9060,-9058,-9056,-9054,-9053,-9053,-9048,-9046,-9040,-9032,-9028,-9023,-9021,-9017,-9017,-9015,-9012,-9012,-9012,-9011,-9011,-9008,-9008,-9007,-9006,-9005,-8999,-8997,-8995,-8984,-8984,-8984,-8982,-8981,-8977,-8972,-8972,-8971,-8969,-8966,-8966,-8960,-8959,-8953,-8950,-8950,-8950,-8948,-8943,-8942,-8941,-8940,-8938,-8938,-8937,-8934,-8932,-8931,-8928,-8924,-8923,-8923,-8923,-8923,-8922,-8920,-8917,-8910,-8910,-8910,-8906,-8905,-8905,-8904,-8893,-8892,-8889,-8888,-8888,-8888,-8884,-8874,-8871,-8870,-8870,-8868,-8868,-8868,-8868,-8866,-8865,-8859,-8858,-8856,-8856,-8851,-8850,-8850,-8849,-8848,-8845,-8844,-8844,-8840,-8835,-8835,-8835,-8835,-8832,-8832,-8831,-8831,-8827,-8825,-8821,-8820,-8818,-8816,-8816,-8815,-8815,-8813,-8810,-8801,-8799,-8798,-8798,-8796,-8794,-8794,-8793,-8788,-8788,-8784,-8782,-8782,-8781,-8780,-8777,-8777,-8776,-8775,-8775,-8773,-8772,-8769,-8764,-8764,-8763,-8763,-8763,-8761,-8759,-8759,-8758,-8757,-8756,-8755,-8755,-8745,-8744,-8740,-8739,-8739,-8735,-8735,-8734,-8734,-8733,-8733,-8732,-8732,-8731,-8730,-8724,-8722,-8722,-8717,-8714,-8709,-8707,-8706,-8704,-8701,-8701,-8700,-8700,-8700,-8696,-8695,-8695,-8693,-8689,-8680,-8677,-8671,-8670,-8669,-8668,-8668,-8667,-8665,-8665,-8665,-8661,-8658,-8657,-8655,-8655,-8653,-8650,-8650,-8649,-8649,-8648,-8647,-8645,-8645,-8643,-8642,-8642,-8642,-8641,-8640,-8640,-8637,-8637,-8637,-8636,-8636,-8633,-8632,-8627,-8626,-8624,-8622,-8616,-8616,-8615,-8609,-8608,-8608,-8602,-8601,-8598,-8596,-8596,-8594,-8593,-8592,-8591,-8589,-8586,-8586,-8586,-8586,-8580,-8577,-8577,-8574,-8574,-8573,-8571,-8569,-8567,-8567,-8566,-8565,-8562,-8561,-8555,-8552,-8551,-8550,-8550,-8549,-8549,-8547,-8544,-8542,-8541,-8541,-8540,-8537,-8537,-8536,-8536,-8535,-8535,-8532,-8527,-8527,-8526,-8525,-8524,-8522,-8520,-8514,-8512,-8510,-8509,-8509,-8508,-8504,-8503,-8502,-8501,-8500,-8500,-8498,-8497,-8494,-8494,-8493,-8489,-8488,-8484,-8479,-8475,-8475,-8474,-8470,-8465,-8463,-8461,-8460,-8458,-8454,-8453,-8453,-8451,-8450,-8449,-8448,-8441,-8439,-8439,-8438,-8438,-8436,-8436,-8434,-8434,-8433,-8430,-8430,-8428,-8427,-8426,-8426,-8425,-8423,-8423,-8420,-8413,-8412,-8411,-8411,-8406,-8406,-8405,-8401,-8399,-8398,-8397,-8395,-8395,-8394,-8392,-8391,-8388,-8383,-8383,-8383,-8381,-8381,-8380,-8378,-8377,-8377,-8376,-8373,-8373,-8371,-8371,-8369,-8365,-8363,-8362,-8359,-8357,-8357,-8357,-8355,-8355,-8354,-8352,-8351,-8351,-8349,-8345,-8343,-8340,-8338,-8329,-8328,-8328,-8326,-8326,-8326,-8326,-8324,-8322,-8317,-8316,-8315,-8315,-8315,-8313,-8312,-8310,-8310,-8309,-8309,-8306,-8302,-8302,-8301,-8299,-8292,-8291,-8291,-8289,-8288,-8287,-8286,-8286,-8285,-8283,-8282,-8275,-8274,-8273,-8270,-8268,-8251,-8251,-8251,-8248,-8242,-8241,-8240,-8237,-8236,-8235,-8235,-8231,-8230,-8229,-8229,-8229,-8229,-8228,-8227,-8224,-8220,-8218,-8210,-8210,-8210,-8207,-8205,-8199,-8195,-8192,-8190,-8190,-8187,-8186,-8181,-8181,-8180,-8177,-8174,-8172,-8170,-8169,-8169,-8166,-8162,-8161,-8161,-8160,-8160,-8160,-8155,-8153,-8153,-8153,-8153,-8151,-8145,-8144,-8141,-8137,-8133,-8130,-8124,-8122,-8115,-8115,-8113,-8111,-8110,-8107,-8104,-8103,-8102,-8102,-8101,-8101,-8100,-8097,-8095,-8093,-8092,-8091,-8088,-8085,-8085,-8083,-8081,-8076,-8071,-8070,-8069,-8068,-8063,-8062,-8058,-8055,-8054,-8054,-8053,-8049,-8049,-8048,-8048,-8043,-8042,-8042,-8039,-8039,-8039,-8038,-8036,-8029,-8028,-8025,-8024,-8024,-8024,-8022,-8020,-8017,-8014,-8014,-8011,-8011,-8007,-8007,-8007,-8000,-7995,-7994,-7992,-7990,-7988,-7988,-7985,-7985,-7982,-7981,-7981,-7980,-7980,-7980,-7980,-7978,-7976,-7976,-7975,-7973,-7971,-7971,-7971,-7968,-7965,-7964,-7964,-7962,-7962,-7962,-7959,-7958,-7957,-7956,-7954,-7952,-7952,-7947,-7946,-7945,-7945,-7944,-7943,-7942,-7939,-7937,-7936,-7934,-7930,-7926,-7921,-7920,-7920,-7920,-7919,-7911,-7910,-7910,-7909,-7905,-7901,-7899,-7898,-7898,-7889,-7889,-7889,-7887,-7887,-7882,-7878,-7877,-7874,-7873,-7869,-7868,-7868,-7867,-7860,-7858,-7858,-7853,-7851,-7849,-7848,-7845,-7843,-7843,-7842,-7842,-7840,-7840,-7839,-7837,-7836,-7836,-7836,-7834,-7834,-7833,-7831,-7831,-7831,-7831,-7829,-7825,-7825,-7824,-7822,-7822,-7821,-7820,-7820,-7818,-7816,-7814,-7806,-7803,-7802,-7797,-7796,-7796,-7795,-7795,-7793,-7791,-7791,-7790,-7790,-7787,-7784,-7784,-7784,-7783,-7783,-7782,-7782,-7782,-7781,-7780,-7777,-7777,-7775,-7771,-7770,-7770,-7765,-7761,-7757,-7757,-7753,-7752,-7738,-7738,-7737,-7735,-7734,-7731,-7728,-7725,-7725,-7724,-7724,-7724,-7724,-7719,-7719,-7719,-7719,-7717,-7714,-7714,-7714,-7713,-7712,-7711,-7711,-7710,-7710,-7703,-7702,-7695,-7690,-7688,-7688,-7688,-7686,-7683,-7683,-7683,-7682,-7682,-7680,-7678,-7678,-7676,-7674,-7671,-7667,-7665,-7663,-7661,-7660,-7658,-7658,-7656,-7649,-7648,-7646,-7644,-7644,-7643,-7641,-7641,-7635,-7635,-7635,-7633,-7633,-7632,-7626,-7626,-7626,-7625,-7623,-7618,-7615,-7615,-7611,-7610,-7609,-7604,-7600,-7600,-7596,-7594,-7593,-7593,-7593,-7593,-7593,-7593,-7591,-7589,-7586,-7585,-7583,-7581,-7580,-7579,-7578,-7576,-7575,-7572,-7571,-7570,-7563,-7563,-7562,-7562,-7560,-7555,-7553,-7553,-7553,-7552,-7552,-7550,-7547,-7547,-7547,-7538,-7538,-7537,-7535,-7534,-7533,-7531,-7531,-7531,-7530,-7528,-7526,-7525,-7524,-7523,-7520,-7515,-7514,-7512,-7512,-7503,-7501,-7500,-7500,-7496,-7496,-7494,-7494,-7489,-7489,-7489,-7485,-7483,-7482,-7482,-7482,-7481,-7479,-7478,-7476,-7474,-7473,-7472,-7466,-7466,-7465,-7464,-7460,-7460,-7460,-7457,-7457,-7455,-7454,-7453,-7449,-7449,-7445,-7445,-7444,-7440,-7438,-7433,-7428,-7428,-7428,-7428,-7427,-7427,-7426,-7426,-7426,-7424,-7422,-7421,-7421,-7421,-7418,-7418,-7417,-7417,-7413,-7412,-7410,-7410,-7406,-7405,-7405,-7405,-7405,-7404,-7403,-7395,-7392,-7390,-7389,-7388,-7380,-7380,-7379,-7378,-7378,-7378,-7377,-7375,-7375,-7375,-7375,-7375,-7372,-7371,-7371,-7366,-7366,-7365,-7361,-7356,-7355,-7354,-7352,-7352,-7351,-7349,-7349,-7347,-7346,-7341,-7340,-7339,-7335,-7332,-7331,-7328,-7327,-7327,-7327,-7326,-7322,-7322,-7321,-7320,-7318,-7316,-7316,-7315,-7309,-7307,-7307,-7306,-7304,-7303,-7302,-7302,-7302,-7301,-7299,-7294,-7292,-7292,-7290,-7289,-7289,-7289,-7289,-7284,-7276,-7274,-7272,-7272,-7271,-7270,-7269,-7269,-7267,-7266,-7266,-7266,-7265,-7264,-7263,-7259,-7259,-7259,-7258,-7257,-7253,-7253,-7252,-7252,-7252,-7251,-7251,-7250,-7248,-7248,-7246,-7242,-7242,-7242,-7241,-7240,-7240,-7240,-7239,-7239,-7239,-7239,-7234,-7233,-7232,-7230,-7230,-7227,-7226,-7226,-7223,-7222,-7221,-7221,-7221,-7219,-7218,-7218,-7217,-7215,-7213,-7212,-7212,-7211,-7210,-7210,-7209,-7208,-7207,-7207,-7205,-7203,-7202,-7202,-7201,-7196,-7196,-7194,-7193,-7193,-7193,-7191,-7191,-7189,-7189,-7188,-7187,-7186,-7184,-7183,-7183,-7182,-7182,-7180,-7180,-7180,-7178,-7176,-7175,-7175,-7175,-7175,-7174,-7172,-7172,-7172,-7171,-7170,-7170,-7170,-7168,-7164,-7164,-7164,-7163,-7162,-7159,-7157,-7155,-7154,-7153,-7150,-7144,-7144,-7143,-7143,-7141,-7141,-7139,-7139,-7134,-7132,-7132,-7131,-7130,-7129,-7128,-7124,-7123,-7123,-7123,-7120,-7120,-7118,-7118,-7118,-7118,-7117,-7116,-7115,-7114,-7112,-7112,-7112,-7111,-7110,-7105,-7104,-7103,-7103,-7102,-7101,-7100,-7096,-7094,-7092,-7091,-7089,-7088,-7087,-7086,-7086,-7084,-7083,-7083,-7082,-7082,-7081,-7079,-7077,-7077,-7077,-7076,-7076,-7076,-7072,-7066,-7065,-7065,-7064,-7064,-7062,-7062,-7059,-7057,-7055,-7055,-7050,-7049,-7041,-7041,-7040,-7040,-7037,-7034,-7032,-7032,-7030,-7030,-7028,-7028,-7027,-7018,-7018,-7017,-7015,-7012,-7009,-7008,-7008,-7008,-7007,-7006,-7005,-7003,-7000,-6999,-6999,-6998,-6996,-6995,-6995,-6993,-6991,-6990,-6990,-6989,-6988,-6987,-6987,-6987,-6987,-6984,-6984,-6980,-6977,-6977,-6976,-6976,-6968,-6968,-6967,-6967,-6962,-6960,-6957,-6953,-6953,-6953,-6952,-6951,-6951,-6950,-6947,-6946,-6945,-6939,-6933,-6930,-6929,-6929,-6924,-6922,-6921,-6920,-6919,-6913,-6909,-6907,-6901,-6898,-6897,-6894,-6891,-6887,-6885,-6884,-6884,-6883,-6883,-6882,-6881,-6881,-6880,-6879,-6879,-6879,-6877,-6877,-6871,-6867,-6867,-6867,-6867,-6866,-6866,-6864,-6863,-6862,-6854,-6854,-6853,-6850,-6849,-6846,-6844,-6842,-6841,-6841,-6840,-6838,-6838,-6836,-6834,-6833,-6831,-6830,-6830,-6828,-6825,-6825,-6825,-6824,-6823,-6822,-6821,-6820,-6820,-6820,-6817,-6817,-6815,-6814,-6813,-6812,-6812,-6810,-6809,-6808,-6806,-6805,-6805,-6805,-6802,-6796,-6795,-6794,-6791,-6789,-6789,-6786,-6785,-6783,-6782,-6782,-6781,-6780,-6772,-6771,-6771,-6767,-6763,-6759,-6759,-6754,-6754,-6754,-6754,-6751,-6747,-6747,-6746,-6746,-6744,-6744,-6744,-6741,-6741,-6741,-6736,-6735,-6733,-6733,-6732,-6732,-6731,-6731,-6730,-6729,-6728,-6725,-6720,-6719,-6718,-6718,-6716,-6715,-6715,-6713,-6713,-6712,-6711,-6711,-6710,-6707,-6707,-6706,-6699,-6699,-6698,-6698,-6696,-6696,-6695,-6695,-6694,-6694,-6693,-6693,-6689,-6689,-6685,-6684,-6682,-6682,-6682,-6682,-6682,-6681,-6680,-6675,-6675,-6674,-6674,-6674,-6673,-6672,-6670,-6666,-6664,-6663,-6659,-6657,-6655,-6653,-6652,-6649,-6641,-6641,-6641,-6639,-6638,-6637,-6635,-6635,-6635,-6635,-6635,-6628,-6626,-6624,-6624,-6623,-6622,-6622,-6617,-6615,-6615,-6611,-6608,-6606,-6602,-6602,-6601,-6600,-6597,-6597,-6595,-6595,-6594,-6594,-6593,-6593,-6593,-6593,-6593,-6588,-6586,-6585,-6585,-6585,-6584,-6583,-6583,-6582,-6580,-6578,-6577,-6574,-6573,-6568,-6568,-6564,-6564,-6563,-6563,-6560,-6560,-6560,-6559,-6558,-6557,-6555,-6555,-6554,-6552,-6550,-6550,-6549,-6548,-6548,-6547,-6545,-6541,-6541,-6540,-6540,-6539,-6539,-6537,-6537,-6537,-6530,-6530,-6529,-6529,-6529,-6529,-6529,-6529,-6523,-6522,-6521,-6520,-6514,-6514,-6513,-6512,-6507,-6506,-6502,-6501,-6498,-6498,-6497,-6495,-6495,-6490,-6488,-6485,-6485,-6485,-6485,-6484,-6484,-6480,-6476,-6475,-6473,-6470,-6470,-6470,-6468,-6468,-6466,-6462,-6460,-6460,-6460,-6460,-6458,-6456,-6453,-6451,-6449,-6449,-6449,-6449,-6448,-6447,-6447,-6446,-6445,-6443,-6443,-6443,-6443,-6442,-6442,-6440,-6440,-6440,-6438,-6437,-6435,-6435,-6435,-6434,-6433,-6433,-6428,-6426,-6426,-6425,-6424,-6424,-6422,-6422,-6417,-6417,-6415,-6414,-6413,-6413,-6411,-6410,-6409,-6409,-6409,-6409,-6408,-6408,-6408,-6407,-6396,-6396,-6394,-6392,-6391,-6391,-6391,-6385,-6385,-6383,-6382,-6382,-6382,-6380,-6380,-6379,-6378,-6376,-6376,-6375,-6375,-6371,-6370,-6368,-6368,-6368,-6368,-6362,-6362,-6358,-6356,-6356,-6354,-6354,-6353,-6351,-6351,-6348,-6346,-6346,-6343,-6339,-6339,-6339,-6339,-6338,-6336,-6335,-6333,-6332,-6330,-6330,-6329,-6329,-6329,-6328,-6328,-6328,-6328,-6325,-6324,-6317,-6317,-6316,-6315,-6313,-6312,-6311,-6310,-6307,-6307,-6304,-6299,-6299,-6299,-6297,-6292,-6292,-6291,-6291,-6288,-6286,-6286,-6285,-6285,-6279,-6279,-6278,-6278,-6278,-6278,-6277,-6277,-6277,-6273,-6273,-6267,-6267,-6266,-6263,-6262,-6262,-6262,-6261,-6259,-6259,-6259,-6259,-6257,-6257,-6257,-6257,-6257,-6252,-6251,-6244,-6244,-6243,-6243,-6242,-6242,-6237,-6236,-6234,-6230,-6228,-6228,-6226,-6226,-6225,-6223,-6222,-6220,-6220,-6220,-6220,-6219,-6219,-6219,-6219,-6218,-6217,-6217,-6212,-6212,-6212,-6206,-6205,-6198,-6197,-6196,-6196,-6196,-6196,-6194,-6193,-6192,-6191,-6190,-6188,-6188,-6187,-6184,-6183,-6180,-6180,-6178,-6176,-6173,-6172,-6168,-6167,-6165,-6165,-6163,-6163,-6163,-6160,-6159,-6159,-6159,-6159,-6159,-6157,-6156,-6156,-6156,-6154,-6154,-6153,-6153,-6153,-6148,-6145,-6143,-6139,-6137,-6136,-6136,-6136,-6134,-6133,-6132,-6131,-6131,-6131,-6131,-6131,-6129,-6124,-6124,-6124,-6123,-6121,-6115,-6112,-6111,-6108,-6108,-6107,-6107,-6107,-6107,-6107,-6107,-6107,-6106,-6106,-6106,-6106,-6106,-6105,-6104,-6100,-6098,-6098,-6098,-6095,-6093,-6092,-6091,-6089,-6087,-6087,-6087,-6086,-6086,-6085,-6084,-6084,-6083,-6083,-6082,-6081,-6081,-6079,-6076,-6074,-6074,-6072,-6070,-6070,-6067,-6065,-6064,-6063,-6063,-6063,-6056,-6055,-6055,-6052,-6051,-6050,-6049,-6048,-6046,-6045,-6045,-6045,-6042,-6042,-6040,-6037,-6036,-6033,-6032,-6030,-6030,-6028,-6028,-6025,-6024,-6023,-6022,-6021,-6018,-6018,-6016,-6016,-6011,-6011,-6011,-6011,-6008,-6007,-6007,-6007,-6007,-6007,-6000,-6000,-5999,-5999,-5999,-5999,-5995,-5994,-5994,-5992,-5990,-5990,-5990,-5989,-5988,-5987,-5985,-5981,-5981,-5980,-5980,-5979,-5978,-5978,-5977,-5975,-5974,-5973,-5971,-5971,-5970,-5970,-5970,-5966,-5959,-5959,-5958,-5956,-5955,-5954,-5952,-5952,-5951,-5949,-5946,-5941,-5941,-5940,-5938,-5931,-5931,-5931,-5931,-5930,-5928,-5924,-5923,-5923,-5921,-5921,-5921,-5918,-5918,-5917,-5913,-5913,-5913,-5913,-5911,-5909,-5907,-5906,-5905,-5905,-5905,-5905,-5903,-5897,-5896,-5896,-5895,-5895,-5895,-5892,-5891,-5889,-5885,-5885,-5884,-5884,-5884,-5884,-5883,-5875,-5875,-5875,-5873,-5869,-5869,-5869,-5862,-5862,-5861,-5861,-5861,-5857,-5857,-5857,-5855,-5853,-5852,-5852,-5851,-5851,-5848,-5848,-5847,-5845,-5843,-5843,-5842,-5842,-5842,-5840,-5839,-5838,-5837,-5836,-5834,-5834,-5833,-5833,-5832,-5832,-5832,-5830,-5824,-5823,-5823,-5823,-5822,-5818,-5816,-5812,-5812,-5808,-5808,-5805,-5804,-5803,-5801,-5799,-5798,-5796,-5796,-5794,-5791,-5790,-5790,-5790,-5789,-5787,-5785,-5784,-5782,-5779,-5779,-5779,-5779,-5771,-5771,-5768,-5767,-5767,-5764,-5764,-5764,-5762,-5760,-5756,-5753,-5753,-5751,-5751,-5750,-5750,-5750,-5749,-5749,-5749,-5748,-5747,-5744,-5742,-5742,-5739,-5738,-5737,-5735,-5734,-5733,-5733,-5732,-5732,-5731,-5731,-5731,-5731,-5727,-5726,-5726,-5726,-5724,-5722,-5720,-5720,-5719,-5718,-5716,-5715,-5715,-5715,-5715,-5708,-5707,-5707,-5705,-5700,-5699,-5698,-5698,-5697,-5696,-5696,-5693,-5693,-5690,-5690,-5690,-5690,-5690,-5687,-5678,-5677,-5677,-5676,-5676,-5676,-5676,-5676,-5673,-5671,-5671,-5670,-5667,-5667,-5667,-5666,-5666,-5665,-5664,-5663,-5662,-5662,-5658,-5658,-5658,-5657,-5655,-5654,-5649,-5647,-5646,-5646,-5645,-5644,-5642,-5641,-5640,-5637,-5636,-5634,-5632,-5632,-5632,-5631,-5630,-5630,-5630,-5629,-5628,-5628,-5628,-5624,-5623,-5623,-5623,-5620,-5619,-5617,-5617,-5617,-5616,-5616,-5612,-5609,-5605,-5594,-5592,-5590,-5590,-5587,-5585,-5585,-5585,-5580,-5580,-5580,-5580,-5579,-5578,-5575,-5575,-5574,-5574,-5574,-5574,-5569,-5568,-5567,-5567,-5564,-5564,-5563,-5563,-5561,-5560,-5560,-5556,-5556,-5556,-5555,-5555,-5555,-5550,-5548,-5547,-5547,-5544,-5544,-5542,-5541,-5539,-5539,-5537,-5535,-5532,-5532,-5531,-5530,-5530,-5530,-5530,-5529,-5526,-5526,-5525,-5525,-5525,-5525,-5524,-5523,-5523,-5522,-5522,-5518,-5518,-5517,-5517,-5514,-5511,-5509,-5509,-5509,-5509,-5509,-5509,-5509,-5509,-5509,-5503,-5503,-5501,-5501,-5501,-5501,-5500,-5498,-5495,-5494,-5494,-5494,-5494,-5490,-5490,-5490,-5489,-5488,-5487,-5487,-5485,-5483,-5483,-5483,-5481,-5481,-5478,-5475,-5475,-5475,-5471,-5470,-5470,-5468,-5468,-5467,-5467,-5467,-5465,-5463,-5458,-5458,-5458,-5458,-5457,-5454,-5454,-5454,-5450,-5450,-5450,-5450,-5449,-5449,-5447,-5447,-5444,-5443,-5442,-5440,-5439,-5438,-5437,-5437,-5436,-5432,-5432,-5432,-5431,-5431,-5428,-5426,-5426,-5426,-5424,-5420,-5419,-5419,-5418,-5412,-5410,-5410,-5404,-5404,-5403,-5403,-5402,-5400,-5399,-5399,-5395,-5395,-5394,-5394,-5393,-5392,-5392,-5390,-5390,-5390,-5390,-5387,-5386,-5382,-5382,-5382,-5382,-5380,-5380,-5376,-5376,-5376,-5375,-5375,-5375,-5374,-5373,-5373,-5372,-5372,-5371,-5369,-5369,-5369,-5363,-5363,-5363,-5363,-5359,-5359,-5359,-5359,-5359,-5359,-5359,-5357,-5357,-5356,-5352,-5351,-5351,-5351,-5348,-5348,-5348,-5345,-5345,-5344,-5344,-5344,-5344,-5344,-5344,-5344,-5344,-5344,-5344,-5340,-5340,-5338,-5338,-5337,-5337,-5337,-5337,-5335,-5334,-5334,-5334,-5331,-5331,-5330,-5329,-5327,-5325,-5325,-5321,-5321,-5321,-5318,-5318,-5318,-5318,-5316,-5316,-5315,-5315,-5309,-5306,-5305,-5304,-5303,-5302,-5302,-5302,-5302,-5301,-5301,-5301,-5301,-5300,-5298,-5297,-5296,-5294,-5292,-5292,-5292,-5292,-5292,-5291,-5289,-5289,-5288,-5287,-5285,-5284,-5283,-5282,-5281,-5281,-5280,-5280,-5280,-5280,-5280,-5280,-5278,-5273,-5266,-5266,-5262,-5261,-5261,-5259,-5259,-5258,-5258,-5256,-5254,-5254,-5253,-5253,-5249,-5249,-5249,-5248,-5248,-5248,-5245,-5244,-5243,-5241,-5240,-5239,-5239,-5239,-5237,-5237,-5235,-5234,-5234,-5232,-5232,-5232,-5232,-5232,-5231,-5231,-5228,-5228,-5228,-5228,-5228,-5226,-5222,-5219,-5219,-5217,-5212,-5211,-5211,-5211,-5211,-5209,-5207,-5206,-5206,-5205,-5205,-5205,-5205,-5205,-5205,-5203,-5203,-5202,-5201,-5201,-5201,-5198,-5197,-5196,-5196,-5196,-5196,-5191,-5191,-5191,-5191,-5190,-5189,-5189,-5189,-5189,-5189,-5187,-5187,-5186,-5186,-5186,-5186,-5184,-5183,-5182,-5181,-5179,-5177,-5169,-5168,-5166,-5165,-5165,-5162,-5161,-5158,-5157,-5157,-5156,-5156,-5153,-5153,-5153,-5152,-5152,-5151,-5151,-5149,-5148,-5148,-5147,-5145,-5144,-5140,-5138,-5137,-5137,-5136,-5135,-5135,-5135,-5135,-5135,-5133,-5133,-5132,-5128,-5128,-5127,-5127,-5126,-5126,-5124,-5123,-5122,-5122,-5121,-5120,-5120,-5120,-5120,-5120,-5119,-5116,-5116,-5116,-5114,-5114,-5114,-5113,-5113,-5112,-5111,-5107,-5105,-5105,-5105,-5103,-5103,-5103,-5102,-5102,-5102,-5102,-5100,-5098,-5096,-5096,-5096,-5090,-5089,-5089,-5088,-5086,-5083,-5083,-5081,-5081,-5079,-5078,-5078,-5078,-5078,-5077,-5073,-5072,-5072,-5069,-5067,-5067,-5065,-5065,-5064,-5064,-5063,-5063,-5063,-5062,-5061,-5060,-5060,-5059,-5059,-5059,-5057,-5057,-5055,-5053,-5053,-5052,-5047,-5045,-5044,-5044,-5044,-5043,-5043,-5043,-5043,-5043,-5043,-5040,-5040,-5040,-5040,-5038,-5037,-5036,-5036,-5036,-5033,-5031,-5030,-5030,-5029,-5029,-5026,-5025,-5023,-5021,-5021,-5020,-5018,-5018,-5018,-5018,-5014,-5011,-5010,-5004,-5004,-5004,-5001,-5001,-5001,-5001,-5001,-5000,-5000,-5000,-5000,-5000,-5000,-4997,-4996,-4995,-4995,-4993,-4993,-4991,-4989,-4985,-4982,-4977,-4975,-4972,-4968,-4965,-4965,-4964,-4961,-4960,-4957,-4957,-4954,-4952,-4952,-4952,-4951,-4951,-4947,-4947,-4946,-4946,-4943,-4943,-4941,-4941,-4938,-4936,-4935,-4935,-4935,-4934,-4934,-4934,-4934,-4933,-4932,-4932,-4931,-4928,-4926,-4926,-4923,-4921,-4921,-4920,-4920,-4917,-4917,-4917,-4915,-4912,-4911,-4911,-4910,-4907,-4905,-4902,-4900,-4897,-4895,-4895,-4895,-4893,-4889,-4888,-4885,-4884,-4884,-4881,-4878,-4878,-4876,-4876,-4874,-4872,-4872,-4872,-4872,-4872,-4871,-4867,-4867,-4867,-4867,-4863,-4863,-4861,-4860,-4859,-4859,-4857,-4857,-4856,-4856,-4855,-4850,-4848,-4848,-4846,-4845,-4845,-4845,-4845,-4845,-4845,-4837,-4836,-4836,-4836,-4835,-4833,-4831,-4830,-4827,-4825,-4825,-4825,-4822,-4822,-4820,-4819,-4819,-4819,-4819,-4815,-4815,-4815,-4815,-4815,-4815,-4813,-4812,-4811,-4810,-4810,-4810,-4808,-4808,-4808,-4807,-4807,-4807,-4807,-4806,-4804,-4802,-4802,-4801,-4801,-4801,-4800,-4800,-4799,-4799,-4798,-4794,-4792,-4792,-4791,-4790,-4790,-4785,-4785,-4784,-4784,-4784,-4781,-4781,-4780,-4779,-4779,-4778,-4777,-4776,-4776,-4770,-4770,-4770,-4768,-4765,-4765,-4765,-4763,-4763,-4763,-4762,-4760,-4759,-4759,-4759,-4757,-4755,-4755,-4755,-4755,-4753,-4753,-4752,-4749,-4746,-4744,-4744,-4742,-4741,-4740,-4740,-4738,-4738,-4738,-4737,-4737,-4737,-4736,-4736,-4734,-4733,-4729,-4726,-4726,-4724,-4724,-4724,-4724,-4724,-4717,-4717,-4714,-4713,-4711,-4711,-4709,-4704,-4703,-4703,-4701,-4698,-4698,-4696,-4694,-4693,-4690,-4690,-4688,-4686,-4686,-4686,-4685,-4685,-4685,-4683,-4682,-4680,-4680,-4679,-4679,-4679,-4679,-4672,-4672,-4672,-4670,-4667,-4666,-4664,-4662,-4656,-4656,-4652,-4652,-4650,-4650,-4649,-4649,-4649,-4648,-4643,-4643,-4641,-4640,-4639,-4639,-4639,-4639,-4635,-4635,-4633,-4633,-4633,-4633,-4621,-4621,-4620,-4620,-4620,-4620,-4619,-4619,-4617,-4615,-4615,-4613,-4613,-4613,-4613,-4613,-4613,-4613,-4612,-4612,-4607,-4607,-4605,-4604,-4604,-4601,-4601,-4601,-4600,-4600,-4599,-4597,-4596,-4596,-4594,-4593,-4593,-4593,-4591,-4591,-4591,-4591,-4587,-4587,-4587,-4585,-4583,-4582,-4582,-4580,-4580,-4578,-4573,-4572,-4569,-4567,-4567,-4567,-4567,-4566,-4565,-4564,-4564,-4564,-4563,-4563,-4563,-4561,-4560,-4557,-4557,-4555,-4553,-4553,-4553,-4552,-4551,-4548,-4545,-4543,-4543,-4543,-4543,-4543,-4539,-4539,-4538,-4537,-4530,-4530,-4529,-4528,-4528,-4525,-4524,-4522,-4520,-4520,-4520,-4519,-4519,-4517,-4517,-4516,-4515,-4515,-4512,-4512,-4512,-4511,-4510,-4508,-4508,-4508,-4508,-4508,-4508,-4507,-4505,-4505,-4504,-4504,-4504,-4503,-4501,-4501,-4501,-4498,-4497,-4495,-4494,-4493,-4493,-4491,-4491,-4491,-4490,-4490,-4490,-4487,-4485,-4485,-4485,-4485,-4485,-4485,-4485,-4485,-4485,-4485,-4483,-4483,-4483,-4480,-4479,-4478,-4477,-4477,-4474,-4474,-4473,-4472,-4470,-4470,-4470,-4470,-4467,-4467,-4466,-4466,-4464,-4460,-4459,-4459,-4457,-4456,-4456,-4453,-4451,-4451,-4450,-4450,-4450,-4449,-4447,-4447,-4447,-4446,-4446,-4444,-4442,-4438,-4438,-4438,-4438,-4436,-4435,-4435,-4435,-4428,-4424,-4423,-4422,-4421,-4420,-4417,-4416,-4414,-4414,-4414,-4410,-4410,-4409,-4409,-4409,-4407,-4407,-4407,-4406,-4401,-4399,-4399,-4397,-4397,-4393,-4393,-4391,-4390,-4390,-4388,-4388,-4387,-4386,-4386,-4386,-4383,-4383,-4379,-4378,-4378,-4377,-4376,-4375,-4375,-4375,-4374,-4374,-4373,-4372,-4371,-4370,-4370,-4369,-4369,-4363,-4363,-4363,-4363,-4363,-4363,-4361,-4358,-4358,-4357,-4357,-4357,-4354,-4350,-4348,-4347,-4345,-4345,-4345,-4344,-4344,-4342,-4342,-4341,-4341,-4341,-4339,-4339,-4330,-4326,-4326,-4326,-4325,-4325,-4322,-4322,-4322,-4322,-4321,-4320,-4319,-4319,-4319,-4319,-4318,-4315,-4315,-4314,-4311,-4311,-4309,-4309,-4305,-4304,-4301,-4300,-4300,-4300,-4300,-4300,-4297,-4297,-4291,-4290,-4288,-4288,-4287,-4287,-4286,-4285,-4284,-4284,-4282,-4282,-4282,-4281,-4280,-4279,-4277,-4277,-4277,-4277,-4277,-4277,-4275,-4274,-4274,-4274,-4270,-4270,-4266,-4266,-4266,-4266,-4266,-4266,-4263,-4263,-4263,-4262,-4261,-4260,-4260,-4258,-4256,-4256,-4255,-4249,-4248,-4247,-4247,-4247,-4247,-4247,-4246,-4245,-4244,-4244,-4243,-4243,-4243,-4243,-4243,-4243,-4242,-4241,-4241,-4240,-4239,-4239,-4239,-4239,-4238,-4238,-4238,-4236,-4235,-4233,-4233,-4233,-4230,-4228,-4228,-4227,-4227,-4224,-4223,-4219,-4219,-4218,-4217,-4215,-4215,-4215,-4214,-4214,-4214,-4213,-4212,-4212,-4212,-4211,-4208,-4207,-4207,-4207,-4206,-4204,-4203,-4202,-4200,-4200,-4200,-4200,-4199,-4199,-4199,-4199,-4199,-4198,-4193,-4191,-4187,-4187,-4186,-4182,-4182,-4182,-4182,-4180,-4179,-4179,-4171,-4171,-4171,-4169,-4166,-4166,-4165,-4164,-4163,-4163,-4162,-4161,-4161,-4160,-4160,-4159,-4156,-4156,-4154,-4153,-4152,-4152,-4152,-4149,-4147,-4147,-4145,-4144,-4143,-4143,-4143,-4140,-4140,-4140,-4139,-4138,-4136,-4136,-4134,-4133,-4133,-4133,-4131,-4128,-4126,-4126,-4126,-4125,-4125,-4123,-4122,-4121,-4119,-4118,-4118,-4116,-4114,-4113,-4110,-4108,-4106,-4106,-4106,-4106,-4106,-4104,-4104,-4103,-4103,-4102,-4102,-4102,-4102,-4102,-4099,-4099,-4099,-4095,-4094,-4094,-4094,-4094,-4092,-4091,-4091,-4090,-4089,-4077,-4075,-4075,-4075,-4075,-4073,-4073,-4073,-4073,-4068,-4068,-4068,-4068,-4068,-4067,-4066,-4065,-4065,-4064,-4061,-4060,-4059,-4059,-4056,-4055,-4054,-4053,-4049,-4048,-4044,-4042,-4042,-4037,-4037,-4034,-4034,-4034,-4033,-4030,-4030,-4030,-4029,-4029,-4026,-4026,-4026,-4024,-4021,-4021,-4016,-4015,-4015,-4011,-4011,-4011,-4011,-4010,-4009,-4009,-4009,-4006,-4004,-4003,-4003,-3994,-3990,-3990,-3987,-3987,-3987,-3985,-3985,-3983,-3982,-3981,-3981,-3979,-3978,-3978,-3976,-3975,-3975,-3974,-3973,-3973,-3973,-3971,-3970,-3970,-3970,-3970,-3970,-3970,-3970,-3970,-3970,-3970,-3968,-3968,-3968,-3967,-3967,-3966,-3965,-3965,-3965,-3962,-3962,-3962,-3961,-3961,-3961,-3960,-3958,-3955,-3952,-3952,-3951,-3951,-3951,-3950,-3950,-3950,-3946,-3943,-3943,-3941,-3939,-3939,-3939,-3939,-3936,-3932,-3932,-3932,-3932,-3932,-3932,-3932,-3932,-3932,-3928,-3927,-3926,-3926,-3926,-3926,-3925,-3924,-3923,-3922,-3921,-3921,-3920,-3920,-3917,-3916,-3915,-3915,-3915,-3912,-3911,-3910,-3909,-3906,-3904,-3901,-3899,-3899,-3899,-3899,-3896,-3894,-3894,-3893,-3893,-3892,-3891,-3890,-3890,-3887,-3886,-3886,-3883,-3882,-3879,-3878,-3878,-3878,-3877,-3877,-3874,-3871,-3871,-3869,-3869,-3869,-3866,-3865,-3861,-3860,-3860,-3859,-3859,-3857,-3857,-3857,-3857,-3856,-3855,-3855,-3854,-3854,-3850,-3850,-3850,-3849,-3848,-3847,-3847,-3846,-3843,-3843,-3843,-3842,-3841,-3841,-3841,-3841,-3840,-3839,-3839,-3839,-3839,-3839,-3839,-3839,-3839,-3834,-3831,-3830,-3830,-3830,-3830,-3828,-3826,-3826,-3823,-3823,-3823,-3822,-3822,-3819,-3817,-3816,-3816,-3816,-3814,-3814,-3814,-3812,-3810,-3809,-3809,-3806,-3806,-3805,-3805,-3804,-3804,-3804,-3804,-3804,-3802,-3801,-3800,-3798,-3796,-3796,-3795,-3793,-3793,-3793,-3789,-3787,-3787,-3787,-3787,-3787,-3787,-3787,-3785,-3785,-3785,-3785,-3784,-3784,-3783,-3783,-3778,-3777,-3776,-3776,-3773,-3773,-3771,-3771,-3771,-3771,-3771,-3765,-3764,-3762,-3762,-3759,-3759,-3758,-3749,-3745,-3745,-3743,-3743,-3743,-3741,-3741,-3736,-3736,-3736,-3736,-3735,-3735,-3730,-3729,-3728,-3728,-3727,-3726,-3725,-3724,-3724,-3722,-3722,-3720,-3720,-3719,-3711,-3710,-3710,-3709,-3703,-3701,-3701,-3701,-3699,-3699,-3697,-3696,-3696,-3696,-3696,-3695,-3694,-3693,-3693,-3692,-3692,-3688,-3688,-3686,-3685,-3685,-3685,-3685,-3684,-3683,-3683,-3678,-3678,-3677,-3677,-3677,-3677,-3676,-3676,-3675,-3674,-3674,-3671,-3670,-3670,-3670,-3670,-3668,-3666,-3666,-3666,-3666,-3665,-3665,-3664,-3664,-3664,-3663,-3663,-3663,-3663,-3663,-3661,-3657,-3657,-3654,-3654,-3654,-3654,-3653,-3652,-3652,-3651,-3651,-3651,-3651,-3647,-3647,-3644,-3644,-3643,-3643,-3640,-3638,-3637,-3637,-3634,-3632,-3632,-3631,-3631,-3631,-3630,-3630,-3630,-3630,-3629,-3627,-3627,-3627,-3625,-3622,-3622,-3622,-3620,-3614,-3611,-3609,-3609,-3608,-3608,-3608,-3608,-3607,-3603,-3603,-3603,-3601,-3600,-3597,-3597,-3595,-3595,-3595,-3594,-3594,-3594,-3594,-3590,-3587,-3587,-3587,-3585,-3584,-3584,-3583,-3582,-3582,-3582,-3582,-3581,-3581,-3579,-3579,-3578,-3577,-3577,-3576,-3576,-3574,-3572,-3572,-3569,-3569,-3569,-3569,-3568,-3568,-3567,-3566,-3565,-3565,-3563,-3563,-3563,-3562,-3562,-3562,-3556,-3555,-3553,-3553,-3553,-3553,-3553,-3551,-3551,-3551,-3551,-3548,-3548,-3548,-3548,-3547,-3547,-3547,-3547,-3546,-3546,-3544,-3544,-3544,-3538,-3536,-3536,-3536,-3536,-3535,-3535,-3534,-3534,-3533,-3531,-3531,-3531,-3531,-3530,-3530,-3527,-3527,-3526,-3525,-3525,-3519,-3519,-3518,-3515,-3513,-3513,-3512,-3512,-3512,-3512,-3511,-3511,-3510,-3510,-3509,-3508,-3508,-3507,-3507,-3507,-3507,-3507,-3504,-3501,-3499,-3498,-3498,-3496,-3495,-3495,-3495,-3495,-3490,-3489,-3489,-3486,-3484,-3484,-3484,-3482,-3482,-3482,-3480,-3478,-3478,-3473,-3470,-3469,-3465,-3465,-3464,-3461,-3461,-3459,-3459,-3459,-3459,-3458,-3458,-3458,-3458,-3455,-3455,-3454,-3454,-3454,-3453,-3453,-3453,-3452,-3450,-3450,-3449,-3449,-3449,-3448,-3445,-3445,-3445,-3444,-3442,-3439,-3439,-3439,-3439,-3438,-3438,-3438,-3438,-3438,-3437,-3436,-3436,-3435,-3435,-3432,-3432,-3431,-3431,-3431,-3431,-3430,-3429,-3426,-3426,-3426,-3426,-3425,-3424,-3423,-3423,-3423,-3421,-3420,-3419,-3419,-3419,-3417,-3417,-3417,-3417,-3411,-3409,-3406,-3406,-3403,-3403,-3400,-3400,-3399,-3399,-3399,-3398,-3398,-3398,-3398,-3398,-3398,-3396,-3396,-3396,-3395,-3394,-3394,-3393,-3393,-3393,-3391,-3391,-3390,-3390,-3389,-3389,-3389,-3387,-3387,-3387,-3386,-3385,-3383,-3382,-3382,-3379,-3379,-3379,-3378,-3377,-3377,-3377,-3376,-3376,-3375,-3375,-3375,-3372,-3372,-3372,-3372,-3372,-3372,-3371,-3370,-3369,-3369,-3369,-3369,-3365,-3364,-3362,-3362,-3359,-3358,-3357,-3356,-3355,-3354,-3353,-3352,-3350,-3350,-3348,-3345,-3345,-3345,-3343,-3343,-3342,-3341,-3341,-3337,-3337,-3337,-3335,-3335,-3335,-3335,-3335,-3333,-3333,-3331,-3331,-3331,-3330,-3330,-3330,-3328,-3328,-3328,-3328,-3326,-3326,-3325,-3325,-3325,-3324,-3323,-3323,-3321,-3319,-3319,-3319,-3318,-3316,-3316,-3316,-3316,-3316,-3314,-3314,-3310,-3309,-3309,-3309,-3309,-3309,-3309,-3309,-3307,-3305,-3305,-3305,-3305,-3305,-3305,-3303,-3302,-3302,-3302,-3302,-3300,-3294,-3293,-3293,-3293,-3291,-3290,-3289,-3287,-3286,-3286,-3286,-3286,-3286,-3282,-3276,-3276,-3276,-3273,-3272,-3270,-3270,-3270,-3268,-3267,-3266,-3266,-3266,-3266,-3265,-3264,-3263,-3260,-3260,-3257,-3257,-3256,-3255,-3255,-3250,-3247,-3246,-3245,-3245,-3245,-3245,-3245,-3245,-3245,-3245,-3244,-3242,-3242,-3241,-3240,-3238,-3237,-3237,-3235,-3235,-3235,-3234,-3233,-3231,-3231,-3231,-3228,-3228,-3225,-3223,-3222,-3222,-3222,-3221,-3221,-3219,-3217,-3216,-3216,-3215,-3212,-3212,-3209,-3208,-3208,-3208,-3206,-3203,-3203,-3202,-3202,-3202,-3201,-3201,-3201,-3196,-3196,-3196,-3196,-3196,-3194,-3194,-3192,-3192,-3190,-3190,-3188,-3188,-3188,-3186,-3186,-3185,-3185,-3184,-3183,-3183,-3183,-3180,-3179,-3179,-3179,-3179,-3175,-3175,-3175,-3174,-3173,-3166,-3166,-3166,-3165,-3165,-3165,-3164,-3162,-3154,-3154,-3153,-3149,-3149,-3149,-3148,-3146,-3146,-3146,-3146,-3146,-3146,-3146,-3145,-3142,-3139,-3139,-3139,-3139,-3139,-3138,-3138,-3136,-3136,-3136,-3136,-3135,-3134,-3134,-3134,-3134,-3133,-3133,-3130,-3130,-3130,-3128,-3125,-3121,-3121,-3119,-3118,-3116,-3114,-3114,-3112,-3109,-3109,-3106,-3104,-3103,-3099,-3098,-3098,-3097,-3097,-3097,-3097,-3096,-3096,-3096,-3095,-3095,-3093,-3093,-3092,-3091,-3091,-3088,-3088,-3088,-3088,-3088,-3087,-3087,-3087,-3087,-3087,-3087,-3087,-3087,-3086,-3083,-3083,-3078,-3077,-3072,-3070,-3068,-3068,-3068,-3068,-3068,-3067,-3064,-3064,-3063,-3063,-3062,-3062,-3062,-3060,-3059,-3059,-3059,-3058,-3058,-3058,-3058,-3056,-3056,-3056,-3051,-3050,-3050,-3050,-3050,-3050,-3050,-3050,-3049,-3049,-3048,-3047,-3046,-3046,-3046,-3046,-3046,-3046,-3046,-3046,-3046,-3046,-3045,-3044,-3044,-3041,-3041,-3041,-3041,-3041,-3041,-3039,-3037,-3033,-3033,-3031,-3031,-3029,-3027,-3025,-3025,-3025,-3025,-3025,-3023,-3022,-3018,-3017,-3017,-3015,-3015,-3011,-3009,-3008,-3007,-3007,-3006,-3005,-3005,-3005,-3004,-3003,-3003,-3003,-3003,-3003,-3003,-3002,-3001,-3001,-3000,-3000,-3000,-2999,-2998,-2998,-2998,-2997,-2995,-2995,-2995,-2995,-2995,-2995,-2995,-2995,-2995,-2995,-2994,-2993,-2993,-2993,-2993,-2990,-2990,-2990,-2989,-2989,-2988,-2988,-2988,-2986,-2985,-2984,-2984,-2984,-2984,-2984,-2984,-2983,-2983,-2983,-2983,-2983,-2981,-2981,-2981,-2981,-2980,-2979,-2979,-2979,-2977,-2976,-2976,-2974,-2973,-2973,-2973,-2971,-2970,-2970,-2970,-2968,-2968,-2963,-2963,-2963,-2962,-2962,-2962,-2958,-2958,-2958,-2958,-2958,-2958,-2957,-2957,-2957,-2956,-2953,-2953,-2952,-2952,-2952,-2952,-2952,-2952,-2951,-2951,-2951,-2951,-2951,-2950,-2949,-2947,-2947,-2947,-2945,-2944,-2944,-2944,-2944,-2942,-2939,-2939,-2938,-2938,-2937,-2937,-2933,-2932,-2932,-2931,-2931,-2931,-2931,-2930,-2930,-2929,-2929,-2927,-2925,-2925,-2924,-2923,-2923,-2923,-2920,-2920,-2919,-2918,-2918,-2916,-2916,-2911,-2911,-2911,-2911,-2911,-2911,-2908,-2905,-2905,-2901,-2900,-2900,-2899,-2899,-2899,-2898,-2898,-2897,-2890,-2888,-2884,-2883,-2883,-2881,-2880,-2879,-2879,-2879,-2879,-2879,-2879,-2879,-2878,-2878,-2876,-2876,-2876,-2874,-2870,-2870,-2870,-2870,-2870,-2870,-2865,-2865,-2864,-2864,-2864,-2864,-2864,-2863,-2863,-2863,-2862,-2860,-2860,-2859,-2858,-2858,-2858,-2858,-2858,-2858,-2858,-2858,-2858,-2858,-2858,-2858,-2857,-2856,-2853,-2853,-2851,-2850,-2850,-2850,-2840,-2839,-2835,-2835,-2835,-2835,-2834,-2832,-2832,-2831,-2830,-2829,-2828,-2828,-2828,-2828,-2827,-2827,-2827,-2827,-2827,-2825,-2824,-2824,-2822,-2818,-2817,-2816,-2815,-2815,-2814,-2813,-2812,-2811,-2811,-2807,-2807,-2806,-2806,-2804,-2804,-2804,-2804,-2799,-2799,-2799,-2796,-2796,-2796,-2796,-2795,-2795,-2795,-2795,-2795,-2795,-2794,-2793,-2791,-2791,-2791,-2791,-2791,-2790,-2789,-2788,-2788,-2788,-2785,-2785,-2785,-2785,-2784,-2783,-2783,-2783,-2782,-2782,-2781,-2780,-2779,-2776,-2775,-2775,-2774,-2774,-2773,-2773,-2773,-2773,-2770,-2770,-2770,-2770,-2770,-2768,-2768,-2766,-2760,-2760,-2760,-2755,-2754,-2754,-2754,-2754,-2754,-2753,-2751,-2748,-2748,-2748,-2742,-2742,-2742,-2741,-2741,-2741,-2734,-2734,-2734,-2733,-2731,-2731,-2731,-2730,-2730,-2730,-2730,-2730,-2730,-2726,-2725,-2722,-2721,-2721,-2720,-2718,-2718,-2718,-2716,-2712,-2712,-2711,-2710,-2709,-2707,-2705,-2703,-2703,-2702,-2702,-2700,-2700,-2698,-2698,-2697,-2697,-2697,-2697,-2697,-2695,-2692,-2691,-2691,-2688,-2687,-2687,-2685,-2685,-2685,-2682,-2682,-2682,-2682,-2681,-2679,-2679,-2677,-2677,-2677,-2676,-2676,-2675,-2675,-2674,-2673,-2673,-2673,-2673,-2672,-2672,-2672,-2672,-2671,-2670,-2670,-2670,-2670,-2669,-2668,-2667,-2666,-2666,-2666,-2665,-2665,-2664,-2663,-2663,-2663,-2662,-2662,-2661,-2661,-2660,-2660,-2660,-2659,-2656,-2656,-2656,-2656,-2656,-2655,-2653,-2650,-2650,-2648,-2648,-2647,-2647,-2647,-2647,-2647,-2646,-2645,-2642,-2641,-2641,-2641,-2641,-2641,-2641,-2641,-2641,-2639,-2638,-2638,-2638,-2637,-2637,-2636,-2633,-2632,-2632,-2631,-2630,-2630,-2630,-2629,-2629,-2629,-2629,-2627,-2627,-2626,-2626,-2623,-2623,-2619,-2619,-2614,-2614,-2612,-2612,-2612,-2612,-2611,-2609,-2608,-2608,-2608,-2608,-2608,-2607,-2607,-2605,-2603,-2602,-2602,-2602,-2601,-2601,-2601,-2601,-2601,-2598,-2598,-2598,-2598,-2595,-2595,-2595,-2595,-2590,-2590,-2590,-2588,-2588,-2586,-2583,-2577,-2577,-2573,-2573,-2572,-2571,-2571,-2571,-2570,-2568,-2566,-2566,-2566,-2565,-2565,-2565,-2564,-2564,-2561,-2561,-2558,-2558,-2557,-2554,-2554,-2554,-2554,-2554,-2553,-2553,-2549,-2548,-2548,-2546,-2546,-2545,-2545,-2545,-2545,-2545,-2541,-2540,-2539,-2539,-2538,-2538,-2537,-2537,-2536,-2536,-2536,-2536,-2535,-2535,-2535,-2535,-2534,-2534,-2534,-2533,-2532,-2531,-2531,-2528,-2528,-2527,-2521,-2520,-2520,-2520,-2519,-2519,-2518,-2518,-2518,-2518,-2516,-2514,-2514,-2512,-2512,-2512,-2512,-2512,-2511,-2510,-2509,-2509,-2509,-2509,-2509,-2508,-2508,-2508,-2508,-2503,-2503,-2503,-2503,-2503,-2498,-2498,-2498,-2498,-2498,-2497,-2497,-2497,-2497,-2497,-2493,-2493,-2493,-2493,-2493,-2493,-2491,-2491,-2491,-2491,-2491,-2490,-2490,-2489,-2488,-2486,-2486,-2486,-2486,-2485,-2484,-2484,-2484,-2484,-2484,-2480,-2475,-2473,-2470,-2467,-2466,-2466,-2465,-2465,-2465,-2465,-2465,-2464,-2463,-2461,-2461,-2460,-2460,-2460,-2456,-2456,-2455,-2455,-2455,-2455,-2455,-2454,-2454,-2453,-2451,-2450,-2445,-2445,-2445,-2445,-2444,-2443,-2442,-2442,-2442,-2441,-2439,-2439,-2439,-2432,-2432,-2431,-2429,-2429,-2428,-2428,-2428,-2427,-2426,-2426,-2426,-2426,-2426,-2425,-2423,-2423,-2421,-2421,-2419,-2419,-2418,-2418,-2418,-2413,-2413,-2412,-2412,-2410,-2409,-2408,-2406,-2404,-2404,-2404,-2403,-2401,-2401,-2401,-2401,-2397,-2396,-2396,-2396,-2396,-2395,-2395,-2394,-2394,-2394,-2394,-2394,-2394,-2394,-2393,-2392,-2392,-2392,-2392,-2392,-2392,-2392,-2392,-2392,-2392,-2392,-2387,-2383,-2380,-2380,-2379,-2376,-2374,-2372,-2372,-2371,-2371,-2371,-2370,-2369,-2367,-2367,-2367,-2366,-2366,-2366,-2366,-2363,-2363,-2363,-2363,-2363,-2361,-2359,-2357,-2356,-2356,-2355,-2354,-2352,-2350,-2350,-2348,-2348,-2347,-2347,-2346,-2346,-2346,-2346,-2346,-2346,-2345,-2344,-2344,-2344,-2344,-2344,-2344,-2344,-2344,-2344,-2344,-2344,-2344,-2344,-2344,-2344,-2342,-2341,-2339,-2338,-2333,-2333,-2333,-2332,-2332,-2332,-2332,-2331,-2330,-2328,-2327,-2327,-2327,-2327,-2327,-2326,-2326,-2324,-2324,-2323,-2323,-2321,-2321,-2320,-2319,-2319,-2319,-2319,-2319,-2319,-2318,-2318,-2318,-2318,-2317,-2317,-2317,-2314,-2314,-2314,-2314,-2314,-2312,-2311,-2311,-2310,-2309,-2309,-2309,-2308,-2304,-2303,-2303,-2303,-2303,-2303,-2303,-2303,-2302,-2302,-2297,-2295,-2295,-2293,-2293,-2293,-2292,-2292,-2291,-2291,-2291,-2291,-2291,-2290,-2290,-2290,-2290,-2288,-2288,-2284,-2282,-2282,-2282,-2282,-2282,-2282,-2280,-2279,-2279,-2278,-2278,-2277,-2277,-2277,-2277,-2275,-2274,-2274,-2274,-2271,-2270,-2270,-2269,-2269,-2269,-2269,-2269,-2269,-2269,-2269,-2269,-2267,-2267,-2267,-2264,-2264,-2263,-2263,-2262,-2262,-2262,-2262,-2262,-2261,-2261,-2258,-2256,-2254,-2254,-2254,-2254,-2254,-2254,-2253,-2253,-2253,-2253,-2250,-2250,-2249,-2249,-2248,-2248,-2248,-2247,-2246,-2246,-2245,-2245,-2243,-2243,-2242,-2242,-2242,-2242,-2240,-2240,-2239,-2236,-2236,-2229,-2229,-2229,-2227,-2227,-2226,-2223,-2221,-2221,-2216,-2216,-2215,-2215,-2214,-2209,-2208,-2204,-2204,-2203,-2202,-2202,-2201,-2201,-2201,-2200,-2196,-2196,-2196,-2196,-2196,-2195,-2195,-2194,-2194,-2194,-2194,-2194,-2192,-2189,-2188,-2185,-2185,-2183,-2182,-2182,-2182,-2182,-2181,-2180,-2179,-2175,-2175,-2175,-2175,-2175,-2175,-2175,-2172,-2172,-2172,-2172,-2171,-2171,-2170,-2168,-2165,-2165,-2165,-2162,-2162,-2162,-2158,-2158,-2158,-2158,-2157,-2157,-2157,-2156,-2156,-2153,-2153,-2152,-2151,-2151,-2149,-2148,-2144,-2144,-2143,-2142,-2141,-2141,-2140,-2138,-2138,-2138,-2138,-2137,-2137,-2136,-2131,-2131,-2131,-2131,-2131,-2129,-2129,-2129,-2128,-2128,-2128,-2128,-2128,-2128,-2128,-2127,-2126,-2126,-2126,-2126,-2126,-2126,-2126,-2124,-2122,-2122,-2122,-2122,-2121,-2121,-2120,-2120,-2118,-2118,-2115,-2115,-2114,-2111,-2111,-2111,-2110,-2109,-2109,-2109,-2108,-2106,-2105,-2104,-2104,-2103,-2101,-2101,-2101,-2101,-2101,-2101,-2101,-2099,-2099,-2099,-2099,-2097,-2097,-2097,-2095,-2095,-2094,-2093,-2092,-2091,-2090,-2089,-2089,-2088,-2088,-2088,-2084,-2083,-2082,-2077,-2070,-2070,-2070,-2067,-2064,-2064,-2062,-2062,-2061,-2061,-2061,-2061,-2060,-2060,-2060,-2059,-2059,-2058,-2058,-2058,-2058,-2058,-2058,-2058,-2057,-2057,-2057,-2054,-2053,-2053,-2053,-2053,-2053,-2053,-2053,-2049,-2048,-2048,-2047,-2045,-2045,-2043,-2043,-2043,-2043,-2043,-2043,-2042,-2041,-2040,-2040,-2040,-2040,-2040,-2038,-2035,-2033,-2033,-2033,-2033,-2033,-2032,-2031,-2030,-2028,-2028,-2028,-2026,-2023,-2018,-2016,-2016,-2016,-2016,-2016,-2016,-2016,-2016,-2015,-2015,-2015,-2015,-2014,-2014,-2014,-2014,-2013,-2011,-2009,-2007,-2007,-2004,-2004,-2004,-2004,-2004,-2004,-2003,-2003,-2003,-2003,-2003,-2001,-2001,-1998,-1997,-1997,-1996,-1995,-1995,-1995,-1995,-1995,-1994,-1990,-1990,-1990,-1987,-1987,-1987,-1984,-1982,-1981,-1981,-1980,-1980,-1978,-1978,-1978,-1978,-1976,-1976,-1976,-1976,-1976,-1975,-1975,-1973,-1973,-1973,-1971,-1971,-1971,-1971,-1971,-1968,-1968,-1968,-1968,-1967,-1967,-1967,-1967,-1966,-1966,-1964,-1964,-1964,-1964,-1964,-1964,-1964,-1964,-1959,-1958,-1958,-1955,-1954,-1953,-1948,-1948,-1948,-1944,-1943,-1943,-1942,-1941,-1941,-1940,-1940,-1938,-1938,-1936,-1936,-1934,-1932,-1932,-1931,-1931,-1929,-1925,-1923,-1923,-1922,-1921,-1921,-1921,-1921,-1921,-1919,-1917,-1917,-1917,-1914,-1914,-1914,-1914,-1914,-1914,-1913,-1911,-1910,-1910,-1909,-1908,-1907,-1905,-1903,-1901,-1901,-1899,-1898,-1896,-1896,-1896,-1896,-1896,-1896,-1896,-1895,-1893,-1893,-1893,-1893,-1889,-1889,-1889,-1889,-1889,-1889,-1885,-1883,-1883,-1883,-1883,-1883,-1883,-1883,-1882,-1882,-1882,-1882,-1879,-1879,-1875,-1874,-1874,-1872,-1872,-1871,-1871,-1870,-1870,-1869,-1868,-1867,-1866,-1866,-1866,-1866,-1865,-1865,-1865,-1863,-1863,-1859,-1858,-1858,-1858,-1857,-1856,-1856,-1855,-1854,-1854,-1849,-1849,-1849,-1847,-1845,-1845,-1845,-1843,-1843,-1843,-1843,-1842,-1841,-1841,-1837,-1835,-1833,-1833,-1833,-1833,-1833,-1832,-1832,-1832,-1831,-1830,-1830,-1829,-1824,-1824,-1823,-1822,-1822,-1822,-1820,-1815,-1814,-1813,-1813,-1813,-1813,-1810,-1810,-1810,-1808,-1808,-1808,-1808,-1807,-1806,-1804,-1804,-1804,-1804,-1803,-1803,-1803,-1802,-1802,-1802,-1802,-1802,-1799,-1799,-1798,-1795,-1795,-1793,-1791,-1791,-1789,-1789,-1788,-1788,-1787,-1787,-1786,-1786,-1785,-1785,-1783,-1783,-1782,-1782,-1781,-1780,-1780,-1780,-1779,-1778,-1778,-1778,-1777,-1774,-1774,-1768,-1768,-1768,-1768,-1768,-1764,-1764,-1763,-1762,-1762,-1762,-1761,-1761,-1755,-1753,-1751,-1750,-1750,-1749,-1749,-1748,-1748,-1748,-1748,-1748,-1748,-1748,-1748,-1748,-1748,-1748,-1746,-1745,-1745,-1743,-1743,-1743,-1742,-1742,-1741,-1741,-1740,-1740,-1740,-1740,-1740,-1732,-1732,-1731,-1727,-1727,-1725,-1725,-1725,-1724,-1724,-1722,-1722,-1720,-1720,-1718,-1718,-1718,-1718,-1718,-1718,-1715,-1715,-1710,-1710,-1710,-1710,-1708,-1707,-1707,-1707,-1700,-1700,-1699,-1699,-1698,-1698,-1698,-1698,-1698,-1698,-1698,-1698,-1698,-1698,-1698,-1698,-1698,-1698,-1698,-1697,-1695,-1694,-1693,-1693,-1693,-1692,-1691,-1690,-1687,-1686,-1686,-1684,-1682,-1682,-1682,-1682,-1681,-1681,-1681,-1680,-1679,-1679,-1679,-1679,-1679,-1679,-1679,-1679,-1679,-1679,-1679,-1679,-1678,-1678,-1678,-1677,-1677,-1677,-1677,-1677,-1677,-1677,-1672,-1671,-1671,-1670,-1670,-1670,-1669,-1669,-1669,-1667,-1667,-1667,-1667,-1667,-1666,-1664,-1664,-1664,-1663,-1663,-1663,-1659,-1655,-1655,-1654,-1654,-1654,-1652,-1652,-1650,-1647,-1647,-1646,-1646,-1646,-1645,-1645,-1644,-1643,-1642,-1642,-1642,-1640,-1640,-1640,-1640,-1640,-1639,-1639,-1639,-1638,-1638,-1638,-1637,-1637,-1637,-1636,-1636,-1636,-1636,-1636,-1636,-1636,-1633,-1632,-1631,-1631,-1631,-1629,-1629,-1629,-1628,-1628,-1627,-1626,-1626,-1626,-1626,-1626,-1623,-1623,-1622,-1621,-1620,-1620,-1620,-1619,-1619,-1619,-1618,-1617,-1617,-1617,-1617,-1616,-1615,-1615,-1615,-1609,-1606,-1605,-1604,-1604,-1604,-1604,-1603,-1603,-1603,-1603,-1601,-1600,-1600,-1598,-1598,-1598,-1598,-1598,-1598,-1598,-1598,-1598,-1594,-1593,-1590,-1589,-1589,-1588,-1587,-1587,-1587,-1583,-1583,-1581,-1579,-1577,-1575,-1574,-1570,-1570,-1568,-1568,-1568,-1568,-1568,-1568,-1565,-1563,-1562,-1562,-1562,-1562,-1562,-1561,-1561,-1560,-1560,-1560,-1560,-1559,-1559,-1557,-1557,-1556,-1556,-1556,-1555,-1555,-1555,-1555,-1554,-1554,-1554,-1553,-1553,-1553,-1553,-1552,-1552,-1552,-1547,-1547,-1547,-1547,-1547,-1546,-1546,-1546,-1546,-1546,-1546,-1546,-1546,-1544,-1544,-1544,-1544,-1541,-1541,-1541,-1540,-1540,-1540,-1540,-1540,-1539,-1539,-1539,-1538,-1538,-1535,-1533,-1532,-1531,-1531,-1531,-1531,-1530,-1530,-1529,-1529,-1529,-1529,-1529,-1528,-1528,-1525,-1525,-1525,-1525,-1525,-1524,-1524,-1524,-1524,-1524,-1521,-1520,-1519,-1519,-1517,-1514,-1511,-1511,-1510,-1510,-1509,-1509,-1509,-1509,-1509,-1508,-1508,-1507,-1507,-1507,-1506,-1505,-1503,-1503,-1503,-1502,-1502,-1502,-1502,-1502,-1499,-1497,-1497,-1495,-1494,-1494,-1494,-1494,-1494,-1494,-1494,-1494,-1492,-1492,-1491,-1491,-1491,-1490,-1490,-1490,-1489,-1489,-1486,-1485,-1485,-1484,-1484,-1484,-1483,-1480,-1480,-1480,-1479,-1479,-1477,-1477,-1476,-1476,-1476,-1476,-1476,-1476,-1475,-1475,-1475,-1475,-1474,-1471,-1470,-1469,-1469,-1469,-1468,-1465,-1465,-1464,-1461,-1461,-1457,-1454,-1454,-1449,-1449,-1449,-1446,-1443,-1441,-1441,-1441,-1441,-1441,-1441,-1438,-1438,-1438,-1438,-1436,-1436,-1436,-1436,-1435,-1435,-1433,-1433,-1433,-1433,-1433,-1432,-1432,-1432,-1432,-1429,-1429,-1428,-1428,-1427,-1427,-1427,-1427,-1427,-1427,-1427,-1425,-1423,-1422,-1422,-1421,-1421,-1421,-1416,-1416,-1413,-1413,-1413,-1413,-1413,-1413,-1411,-1411,-1411,-1411,-1411,-1411,-1411,-1411,-1411,-1410,-1410,-1410,-1409,-1409,-1409,-1408,-1406,-1405,-1405,-1405,-1405,-1405,-1405,-1405,-1402,-1402,-1401,-1399,-1399,-1399,-1399,-1397,-1395,-1395,-1394,-1394,-1394,-1394,-1394,-1394,-1393,-1392,-1391,-1391,-1390,-1390,-1390,-1389,-1388,-1384,-1383,-1380,-1379,-1379,-1379,-1377,-1376,-1376,-1373,-1373,-1372,-1372,-1372,-1372,-1370,-1370,-1370,-1370,-1370,-1367,-1366,-1363,-1363,-1363,-1359,-1358,-1358,-1357,-1357,-1356,-1356,-1356,-1356,-1356,-1356,-1356,-1356,-1356,-1356,-1354,-1354,-1354,-1354,-1354,-1354,-1354,-1354,-1352,-1352,-1352,-1351,-1350,-1350,-1346,-1346,-1346,-1343,-1343,-1343,-1340,-1339,-1338,-1336,-1336,-1336,-1336,-1336,-1335,-1333,-1333,-1332,-1329,-1327,-1327,-1327,-1327,-1326,-1323,-1323,-1323,-1322,-1322,-1322,-1319,-1317,-1317,-1317,-1316,-1316,-1315,-1314,-1314,-1314,-1314,-1314,-1314,-1313,-1312,-1312,-1312,-1312,-1312,-1311,-1311,-1310,-1310,-1309,-1309,-1308,-1307,-1307,-1307,-1307,-1307,-1307,-1306,-1306,-1306,-1306,-1306,-1306,-1305,-1305,-1305,-1305,-1305,-1303,-1303,-1303,-1303,-1303,-1303,-1301,-1301,-1301,-1301,-1301,-1301,-1301,-1300,-1300,-1299,-1299,-1299,-1299,-1299,-1299,-1299,-1299,-1299,-1298,-1298,-1297,-1295,-1294,-1294,-1294,-1294,-1294,-1292,-1292,-1292,-1291,-1291,-1291,-1291,-1287,-1287,-1287,-1287,-1287,-1287,-1286,-1286,-1286,-1286,-1281,-1280,-1279,-1279,-1278,-1278,-1277,-1277,-1276,-1275,-1275,-1275,-1275,-1273,-1267,-1267,-1266,-1264,-1260,-1260,-1260,-1260,-1259,-1259,-1259,-1259,-1259,-1259,-1259,-1257,-1257,-1256,-1254,-1254,-1254,-1254,-1252,-1252,-1251,-1247,-1247,-1246,-1246,-1245,-1244,-1244,-1243,-1243,-1242,-1242,-1240,-1240,-1240,-1239,-1237,-1237,-1237,-1237,-1236,-1236,-1235,-1235,-1235,-1235,-1234,-1234,-1234,-1234,-1234,-1234,-1234,-1233,-1233,-1232,-1232,-1232,-1232,-1232,-1231,-1231,-1230,-1226,-1224,-1224,-1223,-1223,-1223,-1219,-1219,-1219,-1219,-1218,-1217,-1217,-1215,-1215,-1215,-1214,-1214,-1213,-1212,-1212,-1212,-1212,-1212,-1210,-1210,-1210,-1210,-1210,-1208,-1208,-1208,-1207,-1203,-1203,-1203,-1201,-1200,-1200,-1200,-1200,-1199,-1199,-1199,-1199,-1194,-1194,-1194,-1194,-1193,-1192,-1192,-1192,-1191,-1191,-1191,-1191,-1191,-1191,-1191,-1191,-1191,-1191,-1191,-1188,-1188,-1188,-1184,-1183,-1181,-1181,-1178,-1178,-1177,-1175,-1173,-1173,-1173,-1173,-1173,-1171,-1171,-1171,-1169,-1169,-1169,-1169,-1166,-1166,-1165,-1165,-1164,-1164,-1163,-1163,-1163,-1163,-1163,-1163,-1163,-1163,-1162,-1162,-1162,-1162,-1162,-1162,-1162,-1161,-1161,-1160,-1160,-1157,-1157,-1157,-1154,-1154,-1154,-1154,-1154,-1154,-1154,-1151,-1151,-1150,-1150,-1149,-1149,-1149,-1148,-1147,-1147,-1147,-1143,-1143,-1142,-1142,-1141,-1141,-1141,-1140,-1140,-1138,-1137,-1136,-1136,-1135,-1135,-1135,-1134,-1133,-1133,-1133,-1133,-1131,-1131,-1131,-1131,-1129,-1128,-1128,-1128,-1128,-1128,-1128,-1124,-1124,-1124,-1124,-1124,-1124,-1124,-1123,-1121,-1121,-1121,-1121,-1121,-1120,-1118,-1118,-1117,-1117,-1116,-1115,-1115,-1114,-1114,-1112,-1111,-1109,-1109,-1106,-1103,-1103,-1103,-1100,-1100,-1100,-1100,-1099,-1099,-1099,-1098,-1098,-1098,-1098,-1098,-1095,-1095,-1095,-1095,-1095,-1094,-1093,-1093,-1092,-1091,-1091,-1091,-1091,-1089,-1089,-1089,-1089,-1088,-1088,-1088,-1088,-1087,-1087,-1087,-1087,-1087,-1087,-1086,-1086,-1085,-1085,-1085,-1084,-1084,-1084,-1082,-1082,-1082,-1080,-1080,-1078,-1078,-1078,-1078,-1077,-1077,-1076,-1076,-1075,-1075,-1075,-1075,-1075,-1075,-1075,-1075,-1074,-1071,-1071,-1071,-1070,-1061,-1061,-1059,-1057,-1056,-1056,-1056,-1056,-1055,-1053,-1053,-1053,-1052,-1052,-1052,-1051,-1049,-1049,-1049,-1049,-1049,-1048,-1048,-1045,-1045,-1045,-1043,-1043,-1042,-1041,-1041,-1040,-1040,-1037,-1036,-1036,-1036,-1036,-1036,-1036,-1036,-1036,-1035,-1035,-1035,-1035,-1035,-1034,-1034,-1032,-1032,-1031,-1031,-1028,-1027,-1027,-1027,-1027,-1027,-1026,-1026,-1025,-1025,-1025,-1023,-1019,-1016,-1016,-1016,-1015,-1013,-1013,-1013,-1012,-1011,-1011,-1009,-1009,-1009,-1009,-1009,-1009,-1009,-1009,-1006,-1006,-1006,-1005,-1005,-1005,-1005,-1005,-1004,-1003,-1003,-1000,-1000,-1000,-1000,-1000,-1000,-1000,-1000,-1000,-999,-999,-997,-994,-993,-993,-992,-992,-989,-989,-988,-988,-988,-988,-988,-986,-985,-985,-985,-985,-984,-982,-982,-982,-981,-981,-981,-980,-980,-980,-979,-978,-978,-976,-976,-974,-974,-973,-973,-969,-969,-963,-963,-962,-959,-959,-959,-959,-959,-958,-958,-957,-956,-956,-956,-956,-954,-954,-953,-953,-953,-953,-951,-951,-950,-950,-950,-949,-949,-948,-948,-947,-947,-946,-944,-944,-944,-944,-944,-944,-942,-934,-934,-933,-931,-931,-931,-929,-929,-929,-929,-928,-928,-927,-927,-925,-924,-924,-924,-924,-924,-922,-922,-921,-919,-919,-919,-919,-917,-917,-917,-917,-917,-917,-917,-916,-916,-915,-914,-914,-914,-914,-914,-914,-910,-909,-909,-903,-903,-903,-903,-899,-897,-896,-896,-896,-894,-894,-894,-894,-894,-894,-894,-893,-893,-892,-892,-892,-892,-890,-890,-890,-890,-890,-890,-890,-888,-888,-888,-887,-887,-887,-887,-885,-884,-884,-884,-883,-883,-883,-880,-880,-880,-880,-880,-877,-877,-877,-877,-876,-874,-874,-874,-874,-874,-873,-872,-871,-870,-870,-870,-870,-870,-870,-870,-869,-869,-868,-867,-867,-865,-865,-862,-861,-861,-861,-860,-860,-859,-859,-859,-859,-858,-857,-857,-857,-857,-857,-857,-857,-855,-855,-855,-854,-854,-854,-851,-850,-850,-850,-850,-850,-850,-849,-849,-849,-849,-848,-847,-847,-847,-846,-845,-845,-845,-845,-845,-844,-844,-844,-843,-843,-843,-841,-839,-839,-839,-838,-838,-838,-838,-838,-837,-837,-837,-837,-837,-837,-836,-836,-836,-836,-833,-830,-830,-829,-828,-828,-827,-826,-826,-826,-826,-826,-826,-825,-825,-824,-824,-824,-824,-824,-823,-823,-823,-823,-820,-820,-820,-820,-818,-818,-818,-815,-815,-815,-814,-813,-813,-813,-813,-813,-813,-809,-805,-804,-804,-804,-804,-803,-803,-803,-802,-800,-798,-797,-795,-795,-795,-794,-793,-793,-793,-791,-789,-789,-789,-789,-787,-787,-785,-785,-785,-783,-783,-783,-783,-783,-782,-781,-778,-775,-775,-775,-770,-770,-770,-770,-769,-769,-768,-768,-768,-766,-765,-765,-765,-765,-765,-765,-764,-764,-764,-764,-764,-764,-764,-764,-764,-763,-762,-761,-761,-757,-757,-757,-755,-755,-755,-755,-755,-753,-752,-752,-752,-752,-752,-750,-750,-749,-748,-748,-747,-747,-747,-745,-745,-745,-745,-745,-745,-744,-744,-743,-743,-743,-743,-742,-740,-740,-739,-739,-739,-738,-738,-738,-738,-736,-736,-736,-735,-735,-735,-735,-733,-733,-733,-733,-732,-732,-730,-730,-730,-730,-730,-725,-725,-725,-725,-725,-724,-724,-723,-723,-723,-723,-723,-722,-722,-722,-719,-718,-718,-718,-718,-718,-715,-709,-709,-707,-707,-707,-707,-706,-706,-704,-704,-703,-703,-703,-703,-703,-702,-702,-702,-702,-701,-700,-699,-695,-694,-694,-694,-691,-691,-691,-691,-691,-691,-691,-691,-690,-687,-687,-687,-683,-683,-681,-680,-680,-679,-679,-679,-679,-679,-679,-679,-678,-678,-678,-678,-678,-678,-678,-678,-678,-678,-678,-678,-677,-675,-675,-675,-674,-674,-674,-672,-671,-670,-670,-670,-670,-668,-668,-668,-667,-663,-663,-663,-663,-662,-660,-660,-660,-660,-660,-660,-658,-658,-658,-658,-657,-657,-655,-655,-655,-654,-653,-653,-653,-651,-651,-650,-650,-650,-650,-650,-649,-649,-649,-648,-648,-647,-647,-646,-646,-644,-643,-643,-642,-642,-642,-641,-641,-637,-637,-637,-636,-636,-636,-633,-633,-632,-632,-629,-626,-626,-625,-625,-622,-622,-621,-621,-621,-620,-620,-620,-620,-620,-618,-618,-618,-618,-618,-618,-617,-617,-617,-617,-617,-615,-614,-614,-614,-614,-614,-613,-613,-613,-613,-611,-611,-609,-609,-609,-608,-608,-608,-607,-606,-606,-605,-605,-605,-604,-604,-603,-602,-601,-601,-601,-599,-599,-594,-594,-594,-594,-593,-593,-593,-593,-593,-593,-592,-591,-591,-589,-589,-589,-585,-585,-585,-583,-583,-583,-581,-581,-580,-579,-579,-579,-579,-579,-579,-578,-578,-578,-578,-578,-578,-576,-576,-572,-571,-570,-570,-569,-569,-569,-569,-569,-569,-568,-567,-567,-566,-565,-564,-562,-562,-557,-557,-556,-556,-555,-555,-554,-553,-552,-551,-551,-551,-551,-551,-551,-551,-551,-551,-549,-547,-546,-546,-544,-543,-543,-542,-542,-541,-541,-541,-538,-536,-535,-535,-532,-531,-531,-530,-530,-530,-529,-529,-529,-529,-529,-529,-529,-527,-527,-525,-525,-525,-525,-525,-525,-523,-523,-523,-523,-523,-520,-519,-519,-517,-517,-517,-517,-516,-516,-516,-515,-515,-514,-513,-513,-513,-512,-512,-510,-509,-508,-505,-505,-502,-500,-500,-498,-497,-497,-496,-495,-495,-495,-493,-493,-493,-493,-491,-491,-489,-489,-487,-486,-483,-482,-481,-480,-480,-480,-480,-480,-480,-480,-479,-478,-478,-477,-477,-476,-476,-476,-474,-474,-472,-472,-472,-466,-466,-465,-464,-463,-462,-462,-462,-462,-460,-459,-459,-459,-459,-459,-459,-459,-456,-455,-452,-452,-452,-449,-449,-449,-449,-449,-449,-449,-446,-443,-441,-441,-441,-441,-441,-441,-441,-440,-438,-437,-437,-436,-436,-435,-435,-433,-432,-430,-429,-425,-424,-423,-423,-422,-422,-419,-419,-418,-418,-413,-413,-413,-413,-412,-412,-412,-412,-412,-412,-412,-412,-412,-412,-409,-405,-405,-405,-404,-403,-403,-402,-402,-402,-401,-401,-401,-401,-397,-397,-397,-397,-397,-396,-396,-396,-396,-396,-396,-394,-394,-394,-393,-393,-393,-393,-393,-393,-391,-390,-390,-390,-390,-390,-390,-390,-390,-390,-388,-388,-388,-386,-386,-386,-386,-386,-386,-386,-384,-384,-384,-384,-384,-384,-384,-384,-384,-384,-384,-384,-383,-382,-382,-382,-382,-382,-381,-380,-376,-376,-376,-376,-376,-376,-375,-375,-375,-375,-375,-375,-375,-374,-372,-371,-371,-371,-371,-371,-371,-371,-370,-369,-369,-369,-369,-369,-367,-367,-365,-364,-364,-364,-363,-362,-362,-361,-361,-361,-359,-359,-359,-358,-358,-358,-358,-355,-355,-355,-355,-355,-355,-355,-354,-354,-353,-353,-353,-353,-352,-352,-349,-349,-349,-347,-346,-346,-346,-346,-345,-345,-344,-344,-344,-343,-343,-343,-343,-343,-341,-341,-341,-341,-341,-341,-340,-340,-339,-338,-336,-336,-336,-334,-334,-334,-334,-334,-332,-332,-332,-332,-332,-331,-331,-331,-330,-329,-328,-328,-325,-325,-325,-325,-325,-324,-324,-324,-322,-322,-322,-320,-320,-319,-319,-318,-318,-317,-316,-316,-315,-315,-315,-315,-313,-313,-313,-313,-313,-312,-312,-312,-312,-311,-310,-309,-309,-309,-308,-307,-307,-304,-303,-303,-302,-302,-302,-302,-301,-301,-301,-297,-297,-297,-297,-297,-297,-296,-295,-295,-294,-294,-294,-294,-294,-292,-292,-292,-292,-288,-288,-283,-283,-283,-283,-283,-283,-280,-280,-280,-280,-280,-280,-276,-274,-272,-272,-272,-272,-269,-269,-269,-269,-269,-269,-269,-269,-268,-268,-268,-268,-268,-266,-266,-266,-265,-264,-264,-264,-264,-264,-264,-263,-263,-262,-262,-262,-262,-262,-262,-262,-259,-259,-259,-259,-259,-259,-259,-259,-258,-258,-257,-256,-256,-256,-255,-255,-255,-253,-253,-252,-252,-252,-251,-247,-247,-247,-247,-243,-243,-242,-242,-242,-242,-242,-242,-242,-240,-240,-239,-239,-238,-238,-238,-238,-238,-237,-237,-237,-237,-237,-237,-237,-236,-231,-231,-231,-231,-230,-230,-229,-229,-224,-223,-223,-222,-222,-222,-222,-222,-222,-222,-221,-221,-221,-221,-220,-219,-219,-219,-218,-216,-216,-216,-216,-215,-215,-212,-212,-212,-210,-210,-210,-210,-210,-210,-209,-209,-209,-209,-209,-209,-207,-206,-206,-206,-206,-206,-206,-206,-206,-206,-206,-206,-200,-199,-199,-199,-197,-196,-196,-195,-195,-195,-195,-191,-190,-190,-188,-188,-188,-188,-188,-188,-187,-187,-185,-185,-185,-184,-183,-183,-183,-182,-182,-181,-181,-181,-179,-179,-177,-177,-176,-176,-175,-175,-174,-174,-174,-173,-173,-168,-167,-167,-167,-166,-166,-165,-165,-165,-165,-164,-164,-164,-164,-164,-164,-164,-164,-164,-163,-163,-163,-161,-161,-160,-160,-160,-160,-158,-158,-156,-155,-155,-154,-154,-154,-154,-152,-152,-152,-152,-151,-151,-151,-150,-150,-150,-150,-149,-149,-149,-149,-149,-149,-149,-149,-149,-149,-149,-149,-148,-148,-148,-148,-148,-148,-148,-148,-148,-146,-145,-145,-145,-145,-145,-145,-145,-145,-145,-142,-142,-142,-142,-142,-142,-141,-141,-141,-140,-138,-137,-137,-137,-137,-137,-136,-136,-134,-133,-133,-132,-132,-132,-132,-132,-132,-131,-131,-131,-129,-129,-125,-125,-125,-125,-125,-124,-123,-122,-122,-122,-122,-122,-122,-122,-122,-122,-122,-122,-120,-120,-119,-119,-119,-119,-119,-119,-119,-119,-118,-117,-116,-116,-115,-115,-115,-114,-114,-113,-113,-113,-113,-112,-112,-111,-110,-110,-110,-109,-106,-106,-106,-106,-106,-105,-104,-104,-103,-103,-102,-102,-102,-102,-102,-102,-100,-98,-98,-98,-97,-97,-97,-96,-96,-94,-94,-93,-93,-93,-93,-93,-90,-90,-90,-90,-90,-90,-88,-88,-88,-88,-88,-88,-87,-87,-87,-87,-87,-87,-87,-87,-87,-87,-87,-87,-87,-86,-86,-86,-86,-86,-86,-86,-86,-85,-85,-85,-85,-85,-84,-81,-81,-81,-81,-81,-80,-80,-80,-80,-80,-80,-80,-79,-79,-79,-79,-78,-77,-75,-75,-75,-74,-74,-74,-74,-74,-73,-73,-73,-73,-72,-72,-72,-71,-71,-69,-68,-68,-68,-68,-67,-67,-67,-65,-65,-63,-62,-62,-62,-61,-61,-61,-60,-59,-58,-57,-57,-56,-55,-55,-55,-54,-54,-54,-54,-54,-54,-54,-54,-54,-54,-54,-54,-54,-52,-52,-52,-52,-52,-52,-52,-50,-50,-50,-50,-48,-48,-48,-47,-47,-47,-44,-43,-42,-42,-42,-42,-42,-36,-36,-35,-35,-35,-35,-35,-35,-33,-33,-32,-32,-32,-32,-32,-30,-30,-30,-28,-28,-28,-27,-27,-27,-27,-26,-26,-25,-24,-24,-22,-22,-22,-22,-21,-21,-21,-21,-21,-21,-21,-20,-19,-18,-18,-18,-17,-15,-14,-14,-14,-13,-13,-10,-9,-9,-8,-5,-5,-5,-5,-4,-3,0,1,4,6,6,6,6,7,7,8,8,8,8,8,8,8,8,8,10,10,10,10,12,12,12,13,15,19,19,20,20,20,20,20,21,21,21,21,22,23,23,23,26,26,26,27,28,29,29,29,29,29,30,30,30,30,30,30,31,32,32,32,34,36,36,36,36,39,39,39,39,39,39,39,42,42,44,44,44,44,44,46,46,46,46,47,49,49,49,51,51,51,51,52,52,52,52,52,52,53,54,54,55,55,55,55,57,57,58,58,58,59,59,59,60,61,61,61,62,62,63,63,63,63,65,65,65,65,65,65,66,68,68,68,68,68,69,69,69,69,69,70,70,70,72,72,72,72,73]
+
+
+**Constraints:**
+
+- 1 <= k <= 104
+- 0 <= nums.length <= 104
+- -104 <= nums[i] <= 104
+- -104 <= val <= 104
+- At most 104
calls will be made to `add`.
+- It is guaranteed that there will be at least `k` elements in the array when you search for the kth
element.
+
+**Solution:**
+
+We can use a min-heap data structure. Hereβs a step-by-step guide to implementing the `KthLargest` class using PHP:
+
+1. **Min-Heap Data Structure**: We will maintain a min-heap (priority queue) of size `k`. The root of this heap will always give us the k-th largest element.
+
+2. **Initialization**: During initialization, we will add the first `k` elements of the stream to the min-heap. For any additional elements, we will compare each new element with the smallest element in the heap (the root). If the new element is larger, we replace the smallest element with this new element and adjust the heap to maintain the k size.
+
+3. **Adding Elements**: Each time an element is added to the stream, we use the same process of comparing and possibly replacing elements in the heap.
+
+Hereβs the PHP code to implement the `KthLargest` class: **[703. Kth Largest Element in a Stream](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/000703-kth-largest-element-in-a-stream/solution.php)**
+
+### Explanation:
+
+1. **Class Constructor**:
+ - Initializes a min-heap to keep track of the top k elements.
+ - Inserts each element from the initial `nums` array into the heap using the `add` method.
+
+2. **`add` Method**:
+ - If the heap contains fewer than `k` elements, the new value is simply inserted.
+ - If the heap already contains `k` elements, compare the new value with the smallest value (root) in the heap:
+ - If the new value is greater, remove the smallest value and insert the new value.
+ - Finally, return the smallest value in the heap, which is the k-th largest element.
+
+This approach ensures that we efficiently keep track of the k-th largest element with each insertion into the stream, maintaining an O(log k) complexity for insertion operations due to heap operations.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
diff --git a/algorithms/000703-kth-largest-element-in-a-stream/solution.php b/algorithms/000703-kth-largest-element-in-a-stream/solution.php
new file mode 100644
index 000000000..f0588b7da
--- /dev/null
+++ b/algorithms/000703-kth-largest-element-in-a-stream/solution.php
@@ -0,0 +1,39 @@
+k = $k;
+ $this->minHeap = new SplMinHeap();
+
+ foreach ($nums as $num) {
+ $this->add($num);
+ }
+ }
+
+ /**
+ * @param Integer $val
+ * @return Integer
+ */
+ function add($val) {
+ if ($this->minHeap->count() < $this->k) {
+ $this->minHeap->insert($val);
+ } elseif ($val > $this->minHeap->top()) {
+ $this->minHeap->extract();
+ $this->minHeap->insert($val);
+ }
+ return $this->minHeap->top();
+ }
+}
+
+/**
+ * Your KthLargest object will be instantiated and called as such:
+ * $obj = KthLargest($k, $nums);
+ * $ret_1 = $obj->add($val);
+ */
\ No newline at end of file
diff --git a/algorithms/000719-find-k-th-smallest-pair-distance/README.md b/algorithms/000719-find-k-th-smallest-pair-distance/README.md
new file mode 100644
index 000000000..8664f36f0
--- /dev/null
+++ b/algorithms/000719-find-k-th-smallest-pair-distance/README.md
@@ -0,0 +1,85 @@
+719\. Find K-th Smallest Pair Distance
+
+**Difficulty:** Hard
+
+**Topics:** `Array`, `Two Pointers`, `Binary Search`, `Sorting`
+
+The **distance of a pair** of integers `a` and `b` is defined as the absolute difference between `a` and `b`.
+
+Given an integer array `nums` and an integer `k`, return _the kth
smallest **distance among all the pairs** `nums[i]` and `nums[j]` where `0 <= i < j < nums.length`_.
+
+**Example 1:**
+
+- **Input:** nums = [1,3,1], k = 1
+- **Output:** 0
+- **Explanation:** Here are all the pairs:\
+ (1,3) -> 2\
+ (1,1) -> 0\
+ (3,1) -> 2\
+ Then the 1st smallest distance pair is (1,1), and its distance is 0.
+
+**Example 2:**
+
+- **Input:** nums = [1,1,1], k = 2
+- **Output:** 0
+
+**Example 3:**
+
+- **Input:** nums = [1,6,1], k = 3
+- **Output:** 5
+
+**Constraints:**
+
+- n == nums.length
+- 2 <= n <= 104
+- 0 <= nums[i] <= 106
+- 1 <= k <= n * (n - 1) / 2
+
+**Hint:**
+1. Binary search for the answer. How can you check how many pairs have distance <= X?
+
+
+**Solution:**
+
+
+We can use a combination of binary search and two-pointer technique. Here's a step-by-step approach to solving this problem:
+
+### Approach:
+
+1. **Sort the Array**: First, sort the array `nums`. Sorting helps in efficiently calculating the number of pairs with a distance less than or equal to a given value.
+
+2. **Binary Search on Distance**: Use binary search to find the `k`-th smallest distance. The search space for the distances ranges from `0` (the smallest possible distance) to `max(nums) - min(nums)` (the largest possible distance).
+
+3. **Count Pairs with Distance β€ Mid**: For each mid value in the binary search, count the number of pairs with a distance less than or equal to `mid`. This can be done efficiently using a two-pointer approach.
+
+4. **Adjust Binary Search Range**: Depending on the number of pairs with distance less than or equal to `mid`, adjust the binary search range. If the count is less than `k`, increase the lower bound; otherwise, decrease the upper bound.
+
+
+Let's implement this solution in PHP: **[719. Find K-th Smallest Pair Distance](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/000719-find-k-th-smallest-pair-distance/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+- **countPairsWithDistanceLessThanOrEqualTo**: This function counts how many pairs have a distance less than or equal to `mid`. It uses two pointers, where `right` is the current element, and `left` is adjusted until the difference between `nums[right]` and `nums[left]` is less than or equal to `mid`.
+
+- **smallestDistancePair**: This function uses binary search to find the `k`-th smallest distance. The `low` and `high` values define the current search range for the distances. The `mid` value is checked using the `countPairsWithDistanceLessThanOrEqualTo` function. Depending on the result, the search space is adjusted.
+
+This algorithm efficiently finds the `k`-th smallest pair distance with a time complexity of `O(n log(max(nums) - min(nums)) + n log n)`.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
diff --git a/algorithms/000719-find-k-th-smallest-pair-distance/solution.php b/algorithms/000719-find-k-th-smallest-pair-distance/solution.php
new file mode 100644
index 000000000..cd648cc52
--- /dev/null
+++ b/algorithms/000719-find-k-th-smallest-pair-distance/solution.php
@@ -0,0 +1,48 @@
+countPairsWithDistanceLessThanOrEqualTo($nums, $mid) < $k) {
+ $low = $mid + 1;
+ } else {
+ $high = $mid;
+ }
+ }
+
+ return $low;
+
+ }
+
+ /**
+ * @param $nums
+ * @param $mid
+ * @return int
+ */
+ function countPairsWithDistanceLessThanOrEqualTo($nums, $mid) {
+ $count = 0;
+ $left = 0;
+
+ for ($right = 1; $right < count($nums); $right++) {
+ while ($nums[$right] - $nums[$left] > $mid) {
+ $left++;
+ }
+ $count += $right - $left;
+ }
+
+ return $count;
+ }
+
+}
\ No newline at end of file
diff --git a/algorithms/000725-split-linked-list-in-parts/README.md b/algorithms/000725-split-linked-list-in-parts/README.md
new file mode 100644
index 000000000..f6a464d23
--- /dev/null
+++ b/algorithms/000725-split-linked-list-in-parts/README.md
@@ -0,0 +1,169 @@
+725\. Split Linked List in Parts
+
+**Difficulty:** Medium
+
+**Topics:** `Linked List`
+
+Given the `head` of a singly linked list and an integer `k`, split the linked list into k consecutive linked list parts.
+
+The length of each part should be as equal as possible: no two parts should have a size differing by more than one. This may lead to some parts being null.
+
+The parts should be in the order of occurrence in the input list, and parts occurring earlier should always have a size greater than or equal to parts occurring later.
+
+Return _an array of the `k` parts_.
+
+**Example 1:**
+
+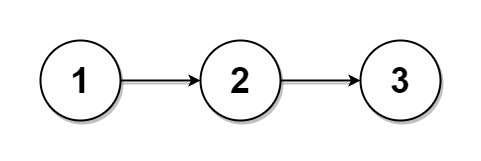
+
+- **Input:** head = [1,2,3], k = 5
+- **Output:** [[1],[2],[3],[],[]]
+- **Explanation:**\
+ The first element output[0] has output[0].val = 1, output[0].next = null.\
+ The last element output[4] is null, but its string representation as a ListNode is [].
+
+**Example 2:**
+
+
+
+- **Input:** head = [1,2,3,4,5,6,7,8,9,10], k = 3
+- **Output:** [[1,2,3,4],[5,6,7],[8,9,10]]
+- **Explanation:**\
+ The input has been split into consecutive parts with size difference at most 1, and earlier parts are a larger size than the later parts.
+
+
+**Constraints:**
+
+- The number of nodes in the list is in the range `[0, 1000]`.
+- `0 <= Node.val <= 1000`
+- `1 <= k <= 50`
+
+
+**Hint:**
+1. If there are N nodes in the list, and k parts, then every part has N/k elements, except the first N%k parts have an extra one.
+
+
+
+**Solution:**
+
+The key observation is that the number of nodes in each part should not differ by more than 1. This means:
+
+1. Calculate the length of the linked list.
+2. Determine the minimum size of each part (`part_size = length // k`).
+3. Distribute the extra nodes evenly across the first few parts (`extra_nodes = length % k`). The first `extra_nodes` parts should have one extra node each.
+
+### Approach
+
+1. **Calculate the length**: Traverse the linked list to find the total number of nodes.
+2. **Determine the size of each part**:
+ - Each part should have at least `length // k` nodes.
+ - The first `length % k` parts should have one extra node.
+3. **Split the list**: Use a loop to split the linked list into `k` parts. For each part:
+ - If it should have extra nodes, assign `part_size + 1` nodes.
+ - If not, assign `part_size` nodes.
+4. **Null parts**: If the list is shorter than `k`, some parts will be empty (null).
+
+
+Let's implement this solution in PHP: **[725. Split Linked List in Parts](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/000725-split-linked-list-in-parts/solution.php)**
+
+```php
+val = $val;
+ $this->next = $next;
+ }
+}
+ /**
+ * @param ListNode $head
+ * @param Integer $k
+ * @return ListNode[]
+ */
+function splitListToParts($head, $k) {
+ ...
+ ...
+ ...
+ /**
+ * go to ./solution.php
+ */
+}
+
+// Helper function to create a linked list from an array
+function createLinkedList($arr) {
+ $head = new ListNode($arr[0]);
+ $current = $head;
+ for ($i = 1; $i < count($arr); $i++) {
+ $current->next = new ListNode($arr[$i]);
+ $current = $current->next;
+ }
+ return $head;
+}
+
+// Helper function to print a linked list
+function printList($head) {
+ $result = [];
+ while ($head !== null) {
+ $result[] = $head->val;
+ $head = $head->next;
+ }
+ return $result;
+}
+
+// Test case 1
+$head = createLinkedList([1, 2, 3]);
+$k = 5;
+$result = splitListToParts($head, $k);
+foreach ($result as $part) {
+ print_r(printList($part));
+}
+
+// Test case 2
+$head = createLinkedList([1, 2, 3, 4, 5, 6, 7, 8, 9, 10]);
+$k = 3;
+$result = splitListToParts($head, $k);
+foreach ($result as $part) {
+ print_r(printList($part));
+}
+?>
+```
+
+### Explanation:
+
+1. **Calculate Length**: We first traverse the linked list to find its length.
+
+2. **Determine Parts**:
+ - We calculate `part_size` as `length // k`, which gives the minimum size each part should have.
+ - We calculate `extra_nodes` as `length % k`, which gives the number of parts that should have one extra node.
+
+3. **Split the List**: We loop through `k` parts and split the list:
+ - For each part, assign `part_size + 1` nodes if it should have an extra node, otherwise just `part_size`.
+ - At the end of each part, we break the link to the rest of the list.
+
+4. **Handle Null Parts**: If there are fewer nodes than `k`, the remaining parts will be `null` (empty).
+
+### Test Cases
+
+- **Example 1**:
+ ```php
+ $head = [1,2,3]; $k = 5;
+ Output: [[1],[2],[3],[],[]]
+ ```
+- **Example 2**:
+ ```php
+ $head = [1,2,3,4,5,6,7,8,9,10]; $k = 3;
+ Output: [[1,2,3,4],[5,6,7],[8,9,10]]
+ ```
+
+This solution efficiently splits the linked list into `k` parts with a time complexity of \(O(n + k)\), where `n` is the length of the list.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
diff --git a/algorithms/000725-split-linked-list-in-parts/solution.php b/algorithms/000725-split-linked-list-in-parts/solution.php
new file mode 100644
index 000000000..eef8528c7
--- /dev/null
+++ b/algorithms/000725-split-linked-list-in-parts/solution.php
@@ -0,0 +1,57 @@
+val = $val;
+ * $this->next = $next;
+ * }
+ * }
+ */
+class Solution {
+
+ /**
+ * @param ListNode $head
+ * @param Integer $k
+ * @return ListNode[]
+ */
+ function splitListToParts($head, $k) {
+ $length = 0;
+ $current = $head;
+
+ // Calculate the total length of the linked list
+ while ($current !== null) {
+ $length++;
+ $current = $current->next;
+ }
+
+ // Determine the size of each part
+ $part_size = intval($length / $k);
+ $extra_nodes = $length % $k;
+
+ // Prepare result array
+ $result = array_fill(0, $k, null);
+
+ $current = $head;
+ for ($i = 0; $i < $k && $current !== null; $i++) {
+ $result[$i] = $current; // Start of the ith part
+ $current_size = $part_size + ($extra_nodes > 0 ? 1 : 0);
+ $extra_nodes--;
+
+ // Move to the end of the current part
+ for ($j = 1; $j < $current_size; $j++) {
+ $current = $current->next;
+ }
+
+ // Break the current part from the rest of the list
+ $next = $current->next;
+ $current->next = null;
+ $current = $next;
+ }
+
+ return $result;
+ }
+}
\ No newline at end of file
diff --git a/algorithms/000729-my-calendar-i/README.md b/algorithms/000729-my-calendar-i/README.md
new file mode 100644
index 000000000..3da64de9b
--- /dev/null
+++ b/algorithms/000729-my-calendar-i/README.md
@@ -0,0 +1,142 @@
+729\. My Calendar I
+
+**Difficulty:** Medium
+
+**Topics:** `Array`, `Binary Search`, `Design`, `Segment Tree`, `Ordered Set`
+
+You are implementing a program to use as your calendar. We can add a new event if adding the event will not cause a **double booking**.
+
+A **double booking** happens when two events have some non-empty intersection (i.e., some moment is common to both events.).
+
+The event can be represented as a pair of integers `start` and `end` that represents a booking on the half-open interval `[start, end)`, the range of real numbers `x` such that `start <= x < end`.
+
+Implement the `MyCalendar` class:
+
+- `MyCalendar()` Initializes the calendar object.
+- `boolean book(int start, int end)` Returns _`true` if the event can be added to the calendar successfully without causing a **double booking**_. Otherwise, return _`false` and do not add the event to the calendar_.
+
+
+**Example 1:**
+
+- **Input:**\
+ ["MyCalendar", "book", "book", "book"]\
+ [[], [10, 20], [15, 25], [20, 30]]
+- **Output:** [null, true, false, true]
+- **Explanation:**\
+ MyCalendar myCalendar = new MyCalendar();\
+ myCalendar.book(10, 20); // return True\
+ myCalendar.book(15, 25); // return False, It can not be booked because time 15 is already booked by another event.\
+ myCalendar.book(20, 30); // return True, The event can be booked, as the first event takes every time less than 20, but not including 20.\
+
+
+**Constraints:**
+
+- 0 <= start < end <= 109
+- At most `1000` calls will be made to `book`.
+
+
+**Hint:**
+1. Store the events as a sorted list of intervals. If none of the events conflict, then the new event can be added.
+
+
+
+**Solution:**
+
+We need to store each event and check if the new event conflicts with any of the existing events before booking it. Since at most 1000 calls to `book` are allowed, we can store the events in a list and iterate through them to check for overlaps when booking new events.
+
+### Plan:
+1. **Storing Events**: We'll maintain a list where each entry is a pair `[start, end]` representing the booked time intervals.
+2. **Check for Conflicts**: Before adding a new event, we'll iterate through the list of booked events and check if the new event conflicts with any existing event. An overlap occurs if the new event's start time is less than the end time of an existing event and the new event's end time is greater than the start time of an existing event.
+3. **Book Event**: If no conflicts are found, we add the new event to our list of bookings.
+
+Let's implement this solution in PHP: **[729. My Calendar I](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/000729-my-calendar-i/solution.php)**
+
+```php
+book($start, $end);
+ */
+
+// Example Usage:
+$myCalendar = new MyCalendar();
+var_dump($myCalendar->book(10, 20)); // true, no conflicts, booking added
+var_dump($myCalendar->book(15, 25)); // false, conflict with [10, 20]
+var_dump($myCalendar->book(20, 30)); // true, no conflicts, booking added
+?>
+```
+
+### Explanation:
+
+1. **Constructor (`__construct`)**: Initializes an empty array `$events` to keep track of all booked events.
+
+2. **Booking Function (`book`)**:
+ - It takes the start and end of a new event.
+ - It iterates through the list of previously booked events and checks for any overlap:
+ - An overlap happens if the new event starts before an existing event ends (`$start < $bookedEnd`) **and** ends after an existing event starts (`$end > $bookedStart`).
+ - If any overlap is found, the function returns `false`, meaning the event cannot be booked.
+ - If no conflicts are found, the event is added to the `$events` array, and the function returns `true` to indicate successful booking.
+
+### Time Complexity:
+- **Booking an Event**: Each call to `book` involves checking the new event against all previously booked events. This leads to a time complexity of `O(n)` for each booking operation, where `n` is the number of previously booked events.
+- **Space Complexity**: The space complexity is `O(n)` because we store up to `n` events in the array.
+
+### Example Walkthrough:
+
+1. **First Booking (`book(10, 20)`)**:
+ - No previous events, so the event `[10, 20]` is successfully booked.
+ - Output: `true`
+
+2. **Second Booking (`book(15, 25)`)**:
+ - The new event `[15, 25]` conflicts with the previously booked event `[10, 20]` because there is an overlap in the time interval (15 is between 10 and 20).
+ - Output: `false`
+
+3. **Third Booking (`book(20, 30)`)**:
+ - The new event `[20, 30]` does not overlap with `[10, 20]` because the start time of the new event is exactly when the first event ends (no overlap since it's a half-open interval).
+ - Output: `true`
+
+This simple approach efficiently handles up to 1000 events while maintaining clarity and correctness.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
diff --git a/algorithms/000729-my-calendar-i/solution.php b/algorithms/000729-my-calendar-i/solution.php
new file mode 100644
index 000000000..8bb00a872
--- /dev/null
+++ b/algorithms/000729-my-calendar-i/solution.php
@@ -0,0 +1,44 @@
+events = [];
+ }
+
+ /**
+ * Books an event if it does not cause a double booking
+ *
+ * @param Integer $start
+ * @param Integer $end
+ * @return Boolean
+ */
+ function book($start, $end) {
+ // Check for any conflicts with existing events
+ foreach ($this->events as $event) {
+ $bookedStart = $event[0];
+ $bookedEnd = $event[1];
+ // Overlap occurs if the new event's start is before the end of the current event
+ // and the new event's end is after the start of the current event
+ if ($start < $bookedEnd && $end > $bookedStart) {
+ return false; // Conflict found, booking not possible
+ }
+ }
+ // No conflicts found, add the new event
+ $this->events[] = [$start, $end];
+ return true;
+ }
+}
+
+/**
+ * Your MyCalendar object will be instantiated and called as such:
+ * $obj = MyCalendar();
+ * $ret_1 = $obj->book($start, $end);
+ */
\ No newline at end of file
diff --git a/algorithms/000731-my-calendar-ii/README.md b/algorithms/000731-my-calendar-ii/README.md
new file mode 100644
index 000000000..aa475a335
--- /dev/null
+++ b/algorithms/000731-my-calendar-ii/README.md
@@ -0,0 +1,146 @@
+731\. My Calendar II
+
+**Difficulty:** Medium
+
+**Topics:** `Array`, `Binary Search`, `Design`, `Segment Tree`, `Prefix Sum`, `Ordered Set`
+
+You are implementing a program to use as your calendar. We can add a new event if adding the event will not cause a **triple booking**.
+
+A **triple booking** happens when three events have some non-empty intersection (i.e., some moment is common to all the three events.).
+
+The event can be represented as a pair of integers `start` and `end` that represents a booking on the half-open interval `[start, end)`, the range of real numbers `x` such that `start <= x < end`.
+
+Implement the `MyCalendarTwo` class:
+
+- `MyCalendarTwo()` Initializes the calendar object.
+- `boolean book(int start, int end)` Returns _`true` if the event can be added to the calendar successfully without causing a **triple booking**_. Otherwise, _`return` false and do not add the event to the calendar_.
+
+
+**Example 1:**
+
+- **Input:**\
+ ["MyCalendarTwo", "book", "book", "book", "book", "book", "book"]\
+ [[], [10, 20], [50, 60], [10, 40], [5, 15], [5, 10], [25, 55]]
+- **Output:** [null, true, true, true, false, true, true]
+- **Explanation:**\
+ MyCalendarTwo myCalendarTwo = new MyCalendarTwo();\
+ myCalendarTwo.book(10, 20); // return True, The event can be booked.\
+ myCalendarTwo.book(50, 60); // return True, The event can be booked.\
+ myCalendarTwo.book(10, 40); // return True, The event can be double booked.\
+ myCalendarTwo.book(5, 15); // return False, The event cannot be booked, because it would result in a triple booking.\
+ myCalendarTwo.book(5, 10); // return True, The event can be booked, as it does not use time 10 which is already double booked.\
+ myCalendarTwo.book(25, 55); // return True, The event can be booked, as the time in [25, 40) will be double booked with the third event, the time [40, 50) will be single booked, and the time [50, 55) will be double booked with the second event.
+
+
+**Constraints:**
+
+- 0 <= start < end <= 109
+- At most `1000` calls will be made to `book`.
+
+
+**Hint:**
+1. Store two sorted lists of intervals: one list will be all times that are at least single booked, and another list will be all times that are definitely double booked. If none of the double bookings conflict, then the booking will succeed, and you should update your single and double bookings accordingly.
+
+
+
+**Solution:**
+
+We need to maintain two lists of bookings:
+
+1. **Single Bookings**: A list that keeps track of all events that are booked once.
+2. **Double Bookings**: A list that keeps track of all events that are double booked.
+
+When a new event is requested, we need to check if it will cause a **triple booking**. To do that:
+
+- We first check if the new event overlaps with any of the intervals in the **double bookings** list. If it does, we cannot add the event because it would lead to a triple booking.
+- If there is no overlap with the **double bookings**, we then check the **single bookings** list and add the overlap of the new event with existing events to the **double bookings** list.
+
+Finally, if the event passes both checks, we add it to the **single bookings** list.
+
+Let's implement this solution in PHP: **[731. My Calendar II](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/000731-my-calendar-ii/solution.php)**
+
+```php
+book($start, $end);
+ */
+
+
+// Example Usage
+$calendar = new MyCalendarTwo();
+echo $calendar->book(10, 20) ? 'true' : 'false'; // true
+echo "\n";
+echo $calendar->book(50, 60) ? 'true' : 'false'; // true
+echo "\n";
+echo $calendar->book(10, 40) ? 'true' : 'false'; // true
+echo "\n";
+echo $calendar->book(5, 15) ? 'true' : 'false'; // false
+echo "\n";
+echo $calendar->book(5, 10) ? 'true' : 'false'; // true
+echo "\n";
+echo $calendar->book(25, 55) ? 'true' : 'false'; // true
+echo "\n";
+?>
+```
+
+### Explanation:
+
+1. **Constructor (`__construct`)**: Initializes the calendar object with two empty arrays to store the single bookings and double bookings.
+
+2. **Book Function (`book`)**:
+ - The function takes the start and end of an event.
+ - It first checks if the event overlaps with any interval in the `doubleBookings` list. If it does, the function returns `false` because it would result in a triple booking.
+ - If there's no triple booking, it checks the overlap with events in the `singleBookings` list. Any overlap found is added to the `doubleBookings` list, as it now represents a double booking.
+ - Finally, the event is added to the `singleBookings` list and the function returns `true`.
+
+### Time Complexity:
+- The time complexity is approximately **O(n)** for each booking operation, where `n` is the number of bookings made so far. This is because for each booking, we may need to check all previous bookings in both the `singleBookings` and `doubleBookings` lists.
+
+This solution efficiently handles up to 1000 calls to the `book` function as required by the problem constraints.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
\ No newline at end of file
diff --git a/algorithms/000731-my-calendar-ii/solution.php b/algorithms/000731-my-calendar-ii/solution.php
new file mode 100644
index 000000000..10df3e471
--- /dev/null
+++ b/algorithms/000731-my-calendar-ii/solution.php
@@ -0,0 +1,56 @@
+singleBookings = [];
+ $this->doubleBookings = [];
+ }
+
+ /**
+ * @param Integer $start
+ * @param Integer $end
+ * @return Boolean
+ */
+ function book($start, $end) {
+ // Check for overlap with double bookings (would result in triple booking)
+ foreach ($this->doubleBookings as $booking) {
+ $overlapStart = max($start, $booking[0]);
+ $overlapEnd = min($end, $booking[1]);
+ if ($overlapStart < $overlapEnd) {
+ // Overlap with a double booking, hence a triple booking will occur
+ return false;
+ }
+ }
+
+ // Check for overlap with single bookings and add overlap to double bookings
+ foreach ($this->singleBookings as $booking) {
+ $overlapStart = max($start, $booking[0]);
+ $overlapEnd = min($end, $booking[1]);
+ if ($overlapStart < $overlapEnd) {
+ // There is overlap, so add this overlap to double bookings
+ $this->doubleBookings[] = [$overlapStart, $overlapEnd];
+ }
+ }
+
+ // If no triple booking, add the event to single bookings
+ $this->singleBookings[] = [$start, $end];
+ return true;
+ }
+}
+
+/**
+ * Your MyCalendarTwo object will be instantiated and called as such:
+ * $obj = MyCalendarTwo();
+ * $ret_1 = $obj->book($start, $end);
+ */
\ No newline at end of file
diff --git a/algorithms/000752-open-the-lock/README.md b/algorithms/000752-open-the-lock/README.md
index 042aa1215..f46f099fd 100644
--- a/algorithms/000752-open-the-lock/README.md
+++ b/algorithms/000752-open-the-lock/README.md
@@ -1,6 +1,8 @@
752\. Open the Lock
-Medium
+**Difficulty:** Medium
+
+**Topics:** `Array`, `Hash Table`, `String`, `Breadth-First Search`
You have a lock in front of you with 4 circular wheels. Each wheel has 10 slots: `'0', '1', '2', '3', '4', '5', '6', '7', '8', '9'`. The wheels can rotate freely and wrap around: for example we can turn `'9'` to be `'0'`, or `'0'` to be `'9'`. Each move consists of turning one wheel one slot.
@@ -41,3 +43,106 @@ Given a `target` representing the value of the wheels that will unlock the lock,
- target.length == 4
- target **will not be** in the list `deadends`.
- `target` and `deadends[i]` consist of digits only.
+
+
+**Hint:**
+1. We can think of this problem as a shortest path problem on a graph: there are `10000` nodes (strings `'0000'` to `'9999'`), and there is an edge between two nodes if they differ in one digit, that digit differs by 1 (wrapping around, so `'0'` and `'9'` differ by 1), and if *both* nodes are not in `deadends`.
+
+
+
+**Solution:**
+
+We can use **Breadth-First Search (BFS)** to find the shortest path from the initial state `'0000'` to the `target` while avoiding the deadends.
+
+### Key Concepts:
+1. **Graph Representation**:
+ - Each lock state (e.g., `'0000'`, `'1234'`) is a node.
+ - Each move consists of changing one wheel either forward (increment by 1) or backward (decrement by 1). This gives a total of 8 possible moves from any given state (2 for each of the 4 wheels).
+
+2. **BFS Approach**:
+ - We start at the initial state `'0000'` and try all possible moves.
+ - If a state matches a deadend or has been visited before, we skip it.
+ - Once we reach the `target`, we return the number of moves (the depth of BFS).
+ - If we explore all possibilities and don't reach the target, we return `-1` (the lock is impossible to open).
+
+### Steps:
+1. Initialize a queue for BFS starting with `'0000'`.
+2. Maintain a set of `deadends` for quick lookup.
+3. Keep track of visited states to avoid revisiting them.
+4. At each step, generate all possible next states by incrementing or decrementing each wheel.
+5. If the `target` is reached, return the number of moves (the BFS level).
+
+Let's implement this solution in PHP: **[752. Open the Lock](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/000752-open-the-lock/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+1. **Deadends Check**:
+ - Before we start the BFS, we check if the initial state `'0000'` is a deadend. If it is, we immediately return `-1` since we can't make any moves.
+
+2. **BFS Algorithm**:
+ - We start with `'0000'` at depth `0` (no moves made yet).
+ - For each state, we generate all possible next states by rotating each wheel either forward or backward (using the helper function `getNextStates()`).
+ - If a state is not in the deadends and hasn't been visited yet, we mark it as visited and add it to the queue with an incremented move count.
+
+3. **Return the Result**:
+ - If we reach the `target` state during the BFS traversal, we return the number of moves taken.
+ - If we exhaust all possibilities and never reach the target, we return `-1`.
+
+### Time Complexity:
+- **Time Complexity**: O(10^4) = O(1), because the total number of possible states is fixed at 10,000 (`0000` to `9999`). BFS explores each state at most once.
+- **Space Complexity**: O(10^4) for storing visited states and the queue.
+
+### Example Walkthrough:
+
+- For input `deadends = ["0201","0101","0102","1212","2002"]` and `target = "0202"`, BFS starts at `'0000'`, and after 6 valid moves, we can reach the target `'0202'`. Thus, the output is `6`.
+
+- For `deadends = ["8888"]` and `target = "0009"`, we can reach the target with just 1 move by rotating the last wheel of `'0000'` backward to `'0009'`. Hence, the output is `1`.
+
+- For `deadends = ["8887","8889","8878","8898","8788","8988","7888","9888"]` and `target = "8888"`, all paths to the target are blocked, so the output is `-1`.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
\ No newline at end of file
diff --git a/algorithms/000752-open-the-lock/solution.php b/algorithms/000752-open-the-lock/solution.php
index fd2a6916c..ba43fb5e6 100644
--- a/algorithms/000752-open-the-lock/solution.php
+++ b/algorithms/000752-open-the-lock/solution.php
@@ -7,48 +7,65 @@ class Solution {
* @param String $target
* @return Integer
*/
- function openLock(array $deadends, string $target): int
- {
- $seen = array_flip($deadends);
- if (isset($seen["0000"])) {
+ function openLock($deadends, $target) {
+ // Convert deadends to a set for O(1) lookup
+ $dead = array_flip($deadends);
+
+ // Check if the initial state '0000' is a deadend
+ if (isset($dead['0000'])) {
return -1;
}
- if ($target == "0000") {
- return 0;
- }
- $ans = 0;
- $q = ["0000"];
-
- while (!empty($q)) {
- $ans++;
- $sz = count($q);
- for ($i = 0; $i < $sz; $i++) {
- $word = array_shift($q);
- for ($j = 0; $j < 4; $j++) {
- $cache = $word[$j];
- $word[$j] = $word[$j] == '9' ? '0' : $word[$j] + 1;
- if ($word == $target) {
- return $ans;
- }
- if (!isset($seen[$word])) {
- $q[] = $word;
- $seen[$word] = true;
- }
- $word[$j] = $cache;
- $word[$j] = $word[$j] == '0' ? '9' : $word[$j] - 1;
- if ($word == $target) {
- return $ans;
- }
- if (!isset($seen[$word])) {
- $q[] = $word;
- $seen[$word] = true;
- }
- $word[$j] = $cache;
+ // Initialize BFS
+ $queue = [['0000', 0]]; // Queue will store the state and the number of moves to reach it
+ $visited = ['0000' => true]; // Mark '0000' as visited
+
+ // BFS loop
+ while (!empty($queue)) {
+ list($state, $moves) = array_shift($queue);
+
+ // If we reach the target, return the number of moves
+ if ($state == $target) {
+ return $moves;
+ }
+
+ // Get all possible next states
+ $nextStates = $this->getNextStates($state);
+ foreach ($nextStates as $next) {
+ // If this state is not a deadend and has not been visited before
+ if (!isset($dead[$next]) && !isset($visited[$next])) {
+ // Mark it as visited and add to the queue
+ $visited[$next] = true;
+ $queue[] = [$next, $moves + 1];
}
}
}
+ // If we exhaust all possibilities and don't reach the target, return -1
return -1;
}
+
+ /**
+ * Helper function to get the next possible states
+ *
+ * @param $current
+ * @return array
+ */
+ private function getNextStates($current) {
+ $result = [];
+ for ($i = 0; $i < 4; $i++) {
+ $digit = $current[$i];
+ // Moving one up
+ $up = $current;
+ $up[$i] = ($digit == '9') ? '0' : chr(ord($digit) + 1);
+ $result[] = $up;
+
+ // Moving one down
+ $down = $current;
+ $down[$i] = ($digit == '0') ? '9' : chr(ord($digit) - 1);
+ $result[] = $down;
+ }
+ return $result;
+ }
+
}
\ No newline at end of file
diff --git a/algorithms/000769-max-chunks-to-make-sorted/README.md b/algorithms/000769-max-chunks-to-make-sorted/README.md
new file mode 100644
index 000000000..25dec2ebd
--- /dev/null
+++ b/algorithms/000769-max-chunks-to-make-sorted/README.md
@@ -0,0 +1,126 @@
+769\. Max Chunks To Make Sorted
+
+**Difficulty:** Medium
+
+**Topics:** `Array`, `Stack`, `Greedy`, `Sorting`, `Monotonic Stack`
+
+You are given an integer array `arr` of length `n` that represents a permutation of the integers in the range `[0, n - 1]`.
+
+We split `arr` into some number of **chunks** (i.e., partitions), and individually sort each chunk. After concatenating them, the result should equal the sorted array.
+
+Return _the largest number of chunks we can make to sort the array._
+
+**Example 1:**
+
+- **Input:** arr = [4,3,2,1,0]
+- **Output:** 1
+- **Explanation:**
+ Splitting into two or more chunks will not return the required result.
+ For example, splitting into [4, 3], [2, 1, 0] will result in [3, 4, 0, 1, 2], which isn't sorted.
+
+**Example 2:**
+
+- **Input:** arr = [1,0,2,3,4]
+- **Output:** 4
+- **Explanation:**
+ We can split into two chunks, such as [1, 0], [2, 3, 4].
+ However, splitting into [1, 0], [2], [3], [4] is the highest number of chunks possible.
+
+
+
+**Constraints:**
+
+- `n == arr.length`
+- `1 <= n <= 10`
+- `0 <= arr[i] < n`
+- All the elements of `arr` are **unique**.
+
+
+**Hint:**
+1. The first chunk can be found as the smallest k for which A[:k+1] == [0, 1, 2, ...k]; then we repeat this process.
+
+
+
+**Solution:**
+
+We need to find the largest number of chunks that can be formed such that each chunk can be sorted individually, and when concatenated, the result is the sorted version of the entire array.
+
+### Approach:
+1. **Key Observation:**
+ - The array is a permutation of integers from `0` to `n-1`. The idea is to traverse the array and find positions where the chunks can be separated.
+ - A chunk can be sorted if, for all indices within the chunk, the maximum element up to that point does not exceed the minimum element after that point in the original sorted array.
+
+2. **Strategy:**
+ - Track the maximum value encountered as you traverse from left to right.
+ - At each index `i`, check if the maximum value up to `i` is less than or equal to `i`. If this condition holds, it means you can split the array at that index because the left part can be sorted independently.
+
+3. **Steps:**
+ - Iterate over the array while maintaining the running maximum.
+ - Whenever the running maximum equals the current index, a chunk can be made.
+ - Count the number of such chunks.
+
+Let's implement this solution in PHP: **[769. Max Chunks To Make Sorted](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/000769-max-chunks-to-make-sorted/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+1. **Initialization:**
+ - We start with `maxSoFar = -1` to ensure that we correctly track the maximum value in the array as we traverse it.
+ - `chunks = 0` keeps track of the number of chunks that can be formed.
+
+2. **Loop:**
+ - We loop through each element in the array.
+ - For each element, we update the `maxSoFar` to be the maximum value between the current element and the previously seen maximum.
+ - If `maxSoFar == i`, it means the array up to index `i` can be independently sorted and this is a valid chunk.
+ - We increment the chunk count each time this condition holds true.
+
+3. **Return:**
+ - Finally, the total number of chunks is returned.
+
+### Time Complexity:
+- **Time Complexity:** _**O(n)**_, where `n` is the length of the array. We only make one pass through the array.
+- **Space Complexity:** _**O(1)**_, as we are only using a few variables to store intermediate results.
+
+### Example Walkthrough:
+
+For `arr = [1, 0, 2, 3, 4]`:
+- At index `0`, the maximum value encountered so far is `1`. We can't split here.
+- At index `1`, the maximum value is `1`, which matches the current index `1`, so we can split into a chunk.
+- At index `2`, the maximum value is `2`, and it matches the index `2`, so we split again.
+- At index `3`, the maximum value is `3`, and it matches the index `3`, so we split again.
+- At index `4`, the maximum value is `4`, and it matches the index `4`, so we split again.
+
+Thus, the output for this case is `4` because we can split it into 4 chunks.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
diff --git a/algorithms/000769-max-chunks-to-make-sorted/solution.php b/algorithms/000769-max-chunks-to-make-sorted/solution.php
new file mode 100644
index 000000000..8019e56a5
--- /dev/null
+++ b/algorithms/000769-max-chunks-to-make-sorted/solution.php
@@ -0,0 +1,28 @@
+
+```
+
+### Explanation:
+
+- **Initial Setup:** We start by converting the 2D board into a 1D string for easier manipulation.
+- **BFS Execution:** We enqueue the initial state of the board along with the move count (starting from 0). In each BFS iteration, we explore the possible moves (based on the `0` tile's position), swap `0` with adjacent tiles, and enqueue the new states.
+- **Visited States:** We use a dictionary to keep track of visited board states to avoid revisiting and looping back to the same states.
+- **Edge Validation:** We check that any move remains within the bounds of the 2x3 grid, especially ensuring no illegal moves that wrap around the grid (such as moving left at the left edge or right at the right edge).
+- **Return Result:** If we reach the target state, we return the number of moves. If BFS completes and we donβt reach the target, we return `-1`.
+
+### Time Complexity:
+- **BFS Complexity:** The time complexity of BFS is `O(N)`, where `N` is the number of unique board states. For this puzzle, there are at most `6!` (720) possible configurations.
+
+### Space Complexity:
+- The space complexity is also `O(N)` due to the storage required for the queue and visited states.
+
+This solution should be efficient enough given the constraints.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
+
+
+
diff --git a/algorithms/000773-sliding-puzzle/solution.php b/algorithms/000773-sliding-puzzle/solution.php
new file mode 100644
index 000000000..e073ad070
--- /dev/null
+++ b/algorithms/000773-sliding-puzzle/solution.php
@@ -0,0 +1,48 @@
+ true]; // Dictionary to track visited states
+
+ // Direction vectors for up, down, left, right movements
+ $directions = [-1, 1, -3, 3]; // Horizontal moves: -1 (left), 1 (right), Vertical moves: -3 (up), 3 (down)
+
+ while (!empty($queue)) {
+ list($current, $moves) = array_shift($queue); // Dequeue the current board state and move count
+
+ if ($current === $target) {
+ return $moves; // If the current state matches the solved state, return the number of moves
+ }
+
+ // Find the position of the empty space (0)
+ $zeroPos = strpos($current, '0');
+
+ foreach ($directions as $direction) {
+ $newPos = $zeroPos + $direction;
+
+ // Ensure the new position is valid and within bounds
+ if ($newPos >= 0 && $newPos < 6 && !($zeroPos % 3 == 0 && $direction == -1) && !($zeroPos % 3 == 2 && $direction == 1)) {
+ // Swap the '0' with the adjacent tile
+ $newState = $current;
+ $newState[$zeroPos] = $newState[$newPos];
+ $newState[$newPos] = '0';
+
+ if (!isset($visited[$newState])) {
+ $visited[$newState] = true;
+ array_push($queue, [$newState, $moves + 1]); // Enqueue the new state with incremented move count
+ }
+ }
+ }
+ }
+
+ return -1; // If BFS completes and we don't find the solution, return -1
+ }
+}
\ No newline at end of file
diff --git a/algorithms/000786-k-th-smallest-prime-fraction/README.md b/algorithms/000786-k-th-smallest-prime-fraction/README.md
index 38aab2b27..3bbba51ae 100644
--- a/algorithms/000786-k-th-smallest-prime-fraction/README.md
+++ b/algorithms/000786-k-th-smallest-prime-fraction/README.md
@@ -1,6 +1,8 @@
786\. K-th Smallest Prime Fraction
-Medium
+**Difficulty:** Medium
+
+**Topics:** `Array`, `Two Pointers`, `Binary Search`, `Sorting`, `Heap (Priority Queue)`
You are given a sorted integer array `arr` containing `1` and **prime** numbers, where all the integers of `arr` are unique. You are also given an integer `k`.
@@ -33,3 +35,84 @@ Return _the kth
smallest fraction considered. Return you
**Follow up:** Can you solve the problem with better than O(n2)
complexity?
+
+
+**Solution:**
+
+Here is a detailed breakdown:
+
+### Approach:
+1. **Binary Search on Fractions**:
+ We perform a binary search over the range of possible fraction values, starting from `0.0` to `1.0`. For each midpoint `m`, we count how many fractions are less than or equal to `m` and track the largest fraction in that range.
+
+2. **Counting Fractions**:
+ Using two pointers, for each prime `arr[i]`, we find the smallest `arr[j]` such that `arr[i] / arr[j]` is greater than the current midpoint `m`. We keep track of the number of valid fractions found and update the fraction closest to `m` but smaller than `m`.
+
+3. **Binary Search Adjustments**:
+ If the number of fractions less than or equal to `m` is exactly `k`, we return the best fraction found so far. If the count is more than `k`, we adjust the right boundary (`r`) of the search. Otherwise, we adjust the left boundary (`l`).
+
+
+Let's implement this solution in PHP: **[786. K-th Smallest Prime Fraction](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/000786-k-th-smallest-prime-fraction/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+1. **Binary Search (`$l` and `$r`)**:
+ We perform a binary search on the possible values of the fractions, starting with `0.0` (the smallest possible value) and `1.0` (the largest possible value). For each midpoint `m`, we check how many fractions are smaller than or equal to `m`.
+
+2. **Counting Valid Fractions**:
+ For each prime `arr[i]`, we use a pointer `j` to find the smallest `arr[j]` such that `arr[i] / arr[j] > m`. This ensures that we only count fractions smaller than `m`.
+
+3. **Tracking the Closest Fraction**:
+ While counting the fractions smaller than or equal to `m`, we also keep track of the largest fraction that is smaller than or equal to `m` using the condition `if ($p * $arr[$j] < $q * $arr[$i])`. This ensures we are tracking the closest fraction to `m` but smaller.
+
+4. **Binary Search Updates**:
+ - If the count of fractions less than or equal to `m` matches `k`, we return the fraction.
+ - If the count is greater than `k`, we shrink the search range (`$r = $m`).
+ - If the count is smaller than `k`, we expand the search range (`$l = $m`).
+
+### Time Complexity:
+- The binary search runs in _**O(log(precision))**_, where the precision refers to the range of fraction values we are considering.
+- For each midpoint, counting the valid fractions and tracking the largest fraction takes _**O(n)**_, as we loop over the array.
+
+Thus, the total time complexity is approximately _**O(n log(precision))**_, where _**n**_ is the length of the array and the precision is determined by how accurately we search for the midpoint. This is better than the brute-force _**O(n2)**_ approach.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
+
+
diff --git a/algorithms/000786-k-th-smallest-prime-fraction/solution.php b/algorithms/000786-k-th-smallest-prime-fraction/solution.php
index d8a0bfb40..4fe821c92 100644
--- a/algorithms/000786-k-th-smallest-prime-fraction/solution.php
+++ b/algorithms/000786-k-th-smallest-prime-fraction/solution.php
@@ -6,40 +6,51 @@ class Solution {
* @param Integer[] $arr
* @param Integer $k
* @return Integer[]
- * @throws Exception
*/
- function kthSmallestPrimeFraction(array $arr, int $k): array
- {
+ function kthSmallestPrimeFraction($arr, $k) {
$n = count($arr);
$l = 0.0;
$r = 1.0;
while ($l < $r) {
- $m = ($l + $r) / 2.0;
- $fractionsNoGreaterThanM = 0;
- $p = 0;
- $q = 1;
+ $m = ($l + $r) / 2.0; // Midpoint in binary search
+ $fractionsNoGreaterThanM = 0; // Count of fractions <= m
+ $p = 0; // Numerator of the closest fraction
+ $q = 1; // Denominator of the closest fraction
- // For each index i, find the first index j s.t. arr[i] / arr[j] <= m,
- // so fractionsNoGreaterThanM for index i will be n - j.
+ // Two pointers for each fraction arr[i] / arr[j]
for ($i = 0, $j = 1; $i < $n; ++$i) {
- while ($j < $n && $arr[$i] > $m * $arr[$j])
+ // Move j while the fraction arr[i] / arr[j] > m
+ while ($j < $n && $arr[$i] > $m * $arr[$j]) {
++$j;
- if ($j == $n)
+ }
+
+ // If we reach the end of the array, break out of the loop
+ if ($j == $n) {
break;
+ }
+
+ // Count fractions with arr[i] / arr[j] <= m
$fractionsNoGreaterThanM += $n - $j;
+
+ // Track the largest fraction <= m
if ($p * $arr[$j] < $q * $arr[$i]) {
$p = $arr[$i];
$q = $arr[$j];
}
}
- if ($fractionsNoGreaterThanM == $k)
- return [$p, $q];
- if ($fractionsNoGreaterThanM > $k)
- $r = $m;
- else
- $l = $m;
+ // Check if the count matches k
+ if ($fractionsNoGreaterThanM == $k) {
+ return [$p, $q]; // Return the k-th smallest fraction
+ }
+
+ // Adjust the binary search bounds
+ if ($fractionsNoGreaterThanM > $k) {
+ $r = $m; // Too many fractions, search left side
+ } else {
+ $l = $m; // Too few fractions, search right side
+ }
}
throw new Exception("Kth smallest prime fraction not found.");
diff --git a/algorithms/000796-rotate-string/README.md b/algorithms/000796-rotate-string/README.md
new file mode 100644
index 000000000..b92e941ef
--- /dev/null
+++ b/algorithms/000796-rotate-string/README.md
@@ -0,0 +1,74 @@
+796\. Rotate String
+
+**Difficulty:** Easy
+
+**Topics:** `String`, `String Matching`
+
+Given two strings `s` and `goal`, return _`true` if and only if `s` can become `goal` after some number of **shifts** on `s`_.
+
+A **shift** on `s` consists of moving the leftmost character of `s` to the rightmost position.
+
+- For example, if `s = "abcde"`, then it will be `"bcdea"` after one shift.
+
+**Example 1:**
+
+- **Input:** s = "abcde", goal = "cdeab"
+- **Output:** true
+
+**Example 2:**
+
+- **Input:** s = "abcde", goal = "abced"
+- **Output:** false
+
+
+**Constraints:**
+
+- `1 <= s.length, goal.length <= 100`
+- `s` and `goal` consist of lowercase English letters.
+
+
+**Solution:**
+
+We can take advantage of the properties of string concatenation. Specifically, if we concatenate the string `s` with itself (i.e., `s + s`), all possible rotations of `s` will appear as substrings within that concatenated string. This allows us to simply check if `goal` is a substring of `s + s`.
+
+Let's implement this solution in PHP: **[796. Rotate String](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/000796-rotate-string/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+1. **Length Check**: We first check if the lengths of `s` and `goal` are the same. If they are not, we immediately return `false`, as it's impossible for `s` to be transformed into `goal`.
+
+2. **Concatenation**: We concatenate the string `s` with itself to create `doubleS`.
+
+3. **Substring Check**: We use the `strpos()` function to check if `goal` exists as a substring in `doubleS`. If it does, we return `true`; otherwise, we return `false`.
+
+### Complexity:
+- **Time Complexity**: O(n), where n is the length of the string, due to the concatenation and substring search.
+- **Space Complexity**: O(n) for the concatenated string.
+
+This solution efficiently determines if one string can become another through rotations.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
diff --git a/algorithms/000796-rotate-string/solution.php b/algorithms/000796-rotate-string/solution.php
new file mode 100644
index 000000000..8d068d3c1
--- /dev/null
+++ b/algorithms/000796-rotate-string/solution.php
@@ -0,0 +1,22 @@
+1 <= n, m <= 104
- 1 <= difficulty[i], profit[i], worker[i] <= 105
+
+
+**Solution:**
+
+We need to assign workers to jobs in such a way that each worker can only do jobs they are capable of based on their ability (which means their ability must be greater than or equal to the job's difficulty). The goal is to maximize the total profit, and one job can be completed multiple times by different workers.
+
+### Plan:
+1. **Sort the jobs by difficulty** so that we can match workers to the most profitable job they can do.
+2. **Sort the workers by their ability**, so that we can easily assign the best job to each worker.
+3. Use a **greedy strategy**: For each worker, find the highest profit they can earn from jobs whose difficulty is less than or equal to their ability.
+
+### Steps:
+1. Combine the difficulty and profit of jobs into a list of tuples and sort them by difficulty. This ensures we process easier jobs first.
+2. Sort the worker array to process workers in increasing order of their ability.
+3. As we iterate over the workers, for each one, assign them the most profitable job they can handle using a two-pointer or binary search approach.
+
+Let's implement this solution in PHP: **[826. Most Profit Assigning Work](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/000826-most-profit-assigning-work/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+1. **Combining jobs**: We create a new array where each job is represented as a tuple `[difficulty, profit]`.
+2. **Sorting jobs**: We sort the jobs by difficulty and, in case of a tie, by profit in descending order. This makes sure we are always trying to assign the most profitable job available that a worker can do.
+3. **Processing workers**: For each worker (sorted by their ability), we move through the sorted job list and assign them the most profitable job they can do.
+4. **Tracking max profit**: The variable `$maxProfit` keeps track of the best profit the worker can earn based on their ability.
+
+### Time Complexity:
+- Sorting jobs and workers both take **O(n log n + m log m)** where `n` is the number of jobs and `m` is the number of workers.
+- The while loop processes each job once, so it is **O(n + m)**.
+- Thus, the overall complexity is **O(n log n + m log m)**.
+
+### Space Complexity:
+- The space complexity is **O(n)** due to the additional array of jobs.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
diff --git a/algorithms/000826-most-profit-assigning-work/solution.php b/algorithms/000826-most-profit-assigning-work/solution.php
index 8316a4caa..d64fd3aed 100644
--- a/algorithms/000826-most-profit-assigning-work/solution.php
+++ b/algorithms/000826-most-profit-assigning-work/solution.php
@@ -9,26 +9,38 @@ class Solution {
* @return Integer
*/
function maxProfitAssignment($difficulty, $profit, $worker) {
- $ans = 0;
- $jobs = array();
-
- for ($i = 0; $i < count($difficulty); ++$i) {
- $jobs[] = array($difficulty[$i], $profit[$i]);
+ // Combine difficulty and profit into a list of jobs
+ $jobs = [];
+ for ($i = 0; $i < count($difficulty); $i++) {
+ $jobs[] = [$difficulty[$i], $profit[$i]];
}
- sort($jobs);
+ // Sort jobs by difficulty (and by profit in case of tie in difficulty)
+ usort($jobs, function($a, $b) {
+ if ($a[0] == $b[0]) {
+ return $b[1] - $a[1]; // sort by profit if difficulties are the same
+ }
+ return $a[0] - $b[0]; // sort by difficulty
+ });
+
+ // Sort the workers by their ability
sort($worker);
- $i = 0;
$maxProfit = 0;
+ $totalProfit = 0;
+ $i = 0; // job index
- foreach ($worker as $w) {
- for (; $i < count($jobs) && $w >= $jobs[$i][0]; ++$i) {
+ // Iterate over each worker
+ foreach ($worker as $ability) {
+ // Assign the best job to the current worker
+ while ($i < count($jobs) && $jobs[$i][0] <= $ability) {
$maxProfit = max($maxProfit, $jobs[$i][1]);
+ $i++;
}
- $ans += $maxProfit;
+ // Add the best profit the worker can achieve
+ $totalProfit += $maxProfit;
}
- return $ans;
+ return $totalProfit;
}
}
\ No newline at end of file
diff --git a/algorithms/000834-sum-of-distances-in-tree/README.md b/algorithms/000834-sum-of-distances-in-tree/README.md
index 2795a904d..59e366260 100644
--- a/algorithms/000834-sum-of-distances-in-tree/README.md
+++ b/algorithms/000834-sum-of-distances-in-tree/README.md
@@ -1,6 +1,8 @@
834\. Sum of Distances in Tree
-Hard
+**Difficulty:** Hard
+
+**Topics:** `Dynamic Programming`, `Tree`, `Depth-First Search`, `Graph`
There is an undirected connected tree with `n` nodes labeled from `0` to `n - 1` and `n - 1` edges.
@@ -10,7 +12,7 @@ Return an array `answer` of length `n` where `answer[i]` is the sum of the dista
**Example 1:**
-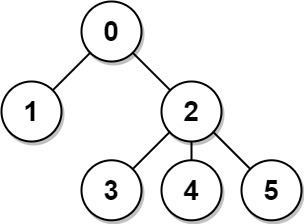
+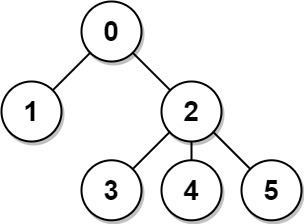
- **Input:** n = 6, edges = [[0,1],[0,2],[2,3],[2,4],[2,5]]
- **Output:** [8,12,6,10,10,10]
@@ -21,14 +23,14 @@ Return an array `answer` of length `n` where `answer[i]` is the sum of the dista
**Example 2:**
-
+
- **Input:** n = 1, edges = []
- **Output:** [0]
**Example 3:**
-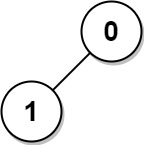
+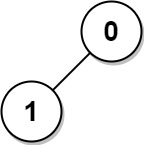
- **Input:** n = 2, edges = [[1,0]]
- **Output:** [1,1]
@@ -41,3 +43,79 @@ Return an array `answer` of length `n` where `answer[i]` is the sum of the dista
- 0 <= ai, bi < n
- ai != bi
- The given input represents a valid tree.
+
+
+
+**Solution:**
+
+We use a combination of Depth-First Search (DFS) and dynamic programming techniques. The goal is to efficiently compute the sum of distances for each node in a tree with `n` nodes and `n-1` edges.
+
+### Approach:
+
+1. **Tree Representation**: Represent the tree using an adjacency list. This helps in efficient traversal using DFS.
+2. **First DFS (`dfs1`)**:
+ - Calculate the size of each subtree.
+ - Compute the sum of distances from the root node to all other nodes.
+3. **Second DFS (`dfs2`)**:
+ - Use the results from the first DFS to compute the sum of distances for all nodes.
+ - Adjust the results based on the parent's result.
+
+### Detailed Steps:
+
+1. **Build the Graph**: Convert the edge list into an adjacency list for efficient traversal.
+2. **First DFS**:
+ - Start from the root node (typically node `0`).
+ - Compute the size of each subtree and the total distance from the root node to all other nodes.
+3. **Second DFS**:
+ - Compute the distance sums for all nodes using the information from the first DFS.
+ - Adjust the distance sum based on the parent nodeβs result.
+
+Let's implement this solution in PHP: **[834. Sum of Distances in Tree](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/000834-sum-of-distances-in-tree/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+1. **Graph Construction**: `array_fill` initializes the adjacency list, `ans`, and `size` arrays.
+2. **`dfs1` Function**: Calculates the total distance from the root and subtree sizes.
+3. **`dfs2` Function**: Adjusts distances for all nodes based on the result from `dfs1`.
+
+This approach efficiently computes the required distances using two DFS traversals, achieving a time complexity of `O(n)`, which is suitable for large trees as specified in the problem constraints.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
diff --git a/algorithms/000834-sum-of-distances-in-tree/solution.php b/algorithms/000834-sum-of-distances-in-tree/solution.php
index 6db8c416a..841971bb6 100644
--- a/algorithms/000834-sum-of-distances-in-tree/solution.php
+++ b/algorithms/000834-sum-of-distances-in-tree/solution.php
@@ -7,22 +7,25 @@ class Solution {
* @param Integer[][] $edges
* @return Integer[]
*/
- function sumOfDistancesInTree(int $n, array $edges): array
- {
+ function sumOfDistancesInTree($n, $edges) {
+ // Build adjacency list
$g = array_fill(0, $n, array());
foreach ($edges as $e) {
$a = $e[0];
$b = $e[1];
- array_push($g[$a], $b);
- array_push($g[$b], $a);
+ $g[$a][] = $b;
+ $g[$b][] = $a;
}
+
+ // Arrays to store answers, subtree sizes, and visit status
$ans = array_fill(0, $n, 0);
$size = array_fill(0, $n, 0);
+ // First DFS to calculate the size of each subtree and the distance for root
$dfs1 = function ($i, $fa, $d) use (&$ans, &$size, &$g, &$dfs1) {
$ans[0] += $d;
$size[$i] = 1;
- foreach ($g[$i] as &$j) {
+ foreach ($g[$i] as $j) {
if ($j != $fa) {
$dfs1($j, $i, $d + 1);
$size[$i] += $size[$j];
@@ -30,17 +33,22 @@ function sumOfDistancesInTree(int $n, array $edges): array
}
};
+ // Second DFS to calculate the distance for all nodes
$dfs2 = function ($i, $fa, $t) use (&$ans, &$size, &$g, &$dfs2, $n) {
$ans[$i] = $t;
- foreach ($g[$i] as &$j) {
+ foreach ($g[$i] as $j) {
if ($j != $fa) {
- $dfs2($j, $i, $t - $size[$j] + $n - $size[$j]);
+ $dfs2($j, $i, $t - $size[$j] + ($n - $size[$j]));
}
}
};
+ // Run the first DFS from node 0
$dfs1(0, -1, 0);
+
+ // Run the second DFS from node 0 with initial total distance
$dfs2(0, -1, $ans[0]);
+
return $ans;
}
}
\ No newline at end of file
diff --git a/algorithms/000840-magic-squares-in-grid/README.md b/algorithms/000840-magic-squares-in-grid/README.md
new file mode 100644
index 000000000..ba72eadad
--- /dev/null
+++ b/algorithms/000840-magic-squares-in-grid/README.md
@@ -0,0 +1,95 @@
+840\. Magic Squares In Grid
+
+Medium
+
+**Topics:** `Array`, `Hash Table`, `Math`, `Matrix`
+
+A `3 x 3` **magic square** is a` 3 x 3` grid filled with distinct numbers **from** `1` **to** `9` such that each row, column, and both diagonals all have the same sum.
+
+Given a `row x col` `grid` of integers, how many `3 x 3` contiguous magic square subgrids are there?
+
+**Note:** while a magic square can only contain numbers from `1` to `9`, `grid` may contain numbers up to `15`.
+
+**Example 1:**
+
+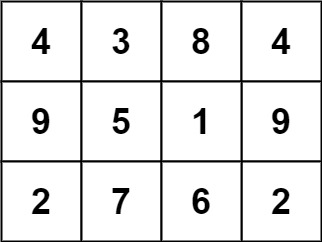
+
+- **Input:** grid = [[4,3,8,4],[9,5,1,9],[2,7,6,2]]
+- **Output:** 1
+- **Explanation:**\
+ The following subgrid is a 3 x 3 magic square:\
+ 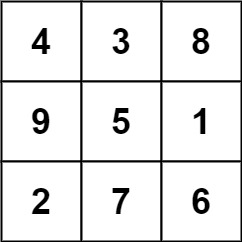\
+ while this one is not:\
+ 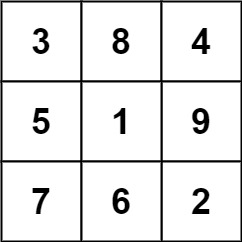\
+ In total, there is only one magic square inside the given grid.
+
+**Example 2:**
+
+- **Input:** grid = [[8]]
+- **Output:** 0
+
+**Constraints:**
+
+- `row == grid.length`.
+- `col == grid[i].length`
+- `1 <= row, col <= 10`
+- `0 <= grid[i][j] <= 15`
+
+
+
+**Solution:**
+
+We need to count how many `3x3` contiguous subgrids in the given grid form a magic square. A magic square is a `3x3` grid where all rows, columns, and both diagonals sum to the same value, and it contains the distinct numbers from `1` to `9`.
+
+To solve this problem, we can follow these steps:
+
+1. **Check if a Subgrid is Magic:**
+ - The subgrid must contain all distinct numbers from `1` to `9`.
+ - The sum of each row, column, and diagonal should be `15`.
+
+2. **Iterate through the Grid:**
+ - Since we need to check `3x3` subgrids, we will iterate from `0` to `row-2` for rows and from `0` to `col-2` for columns.
+ - For each top-left corner of the `3x3` subgrid, extract the subgrid and check if it's a magic square.
+
+Let's implement this solution in PHP: **[840. Magic Squares In Grid](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/000840-magic-squares-in-grid/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+1. **isMagic function:**
+ - Extracts the `3x3` subgrid.
+ - Checks if all numbers are distinct and between `1` to `9`.
+ - Verifies that the sums of the rows, columns, and diagonals are all `15`.
+
+2. **numMagicSquaresInside function:**
+ - Iterates over all possible `3x3` subgrids in the given grid.
+ - Counts how many of those subgrids are magic squares.
+
+This code works efficiently within the constraints, counting all `3x3` magic square subgrids in the given grid.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
diff --git a/algorithms/000840-magic-squares-in-grid/solution.php b/algorithms/000840-magic-squares-in-grid/solution.php
new file mode 100644
index 000000000..f92be1a28
--- /dev/null
+++ b/algorithms/000840-magic-squares-in-grid/solution.php
@@ -0,0 +1,60 @@
+isMagic($grid, $i, $j)) {
+ $count++;
+ }
+ }
+ }
+
+ return $count;
+
+ }
+
+ /**
+ * @param $grid
+ * @param $r
+ * @param $c
+ * @return bool
+ */
+ function isMagic($grid, $r, $c) {
+ // Extract the 3x3 grid
+ $nums = [
+ $grid[$r][$c], $grid[$r][$c+1], $grid[$r][$c+2],
+ $grid[$r+1][$c], $grid[$r+1][$c+1], $grid[$r+1][$c+2],
+ $grid[$r+2][$c], $grid[$r+2][$c+1], $grid[$r+2][$c+2]
+ ];
+
+ // Check for distinct numbers from 1 to 9
+ $set = array_fill(1, 9, 0);
+ foreach ($nums as $num) {
+ if ($num < 1 || $num > 9 || $set[$num] == 1) {
+ return false;
+ }
+ $set[$num] = 1;
+ }
+
+ // Check the sums
+ return ($grid[$r][$c] + $grid[$r][$c+1] + $grid[$r][$c+2] == 15 &&
+ $grid[$r+1][$c] + $grid[$r+1][$c+1] + $grid[$r+1][$c+2] == 15 &&
+ $grid[$r+2][$c] + $grid[$r+2][$c+1] + $grid[$r+2][$c+2] == 15 &&
+ $grid[$r][$c] + $grid[$r+1][$c] + $grid[$r+2][$c] == 15 &&
+ $grid[$r][$c+1] + $grid[$r+1][$c+1] + $grid[$r+2][$c+1] == 15 &&
+ $grid[$r][$c+2] + $grid[$r+1][$c+2] + $grid[$r+2][$c+2] == 15 &&
+ $grid[$r][$c] + $grid[$r+1][$c+1] + $grid[$r+2][$c+2] == 15 &&
+ $grid[$r][$c+2] + $grid[$r+1][$c+1] + $grid[$r+2][$c] == 15);
+ }
+
+}
\ No newline at end of file
diff --git a/algorithms/000846-hand-of-straights/README.md b/algorithms/000846-hand-of-straights/README.md
index 6e201fc3f..9aeee864f 100644
--- a/algorithms/000846-hand-of-straights/README.md
+++ b/algorithms/000846-hand-of-straights/README.md
@@ -1,6 +1,8 @@
846\. Hand of Straights
-Medium
+**Difficulty:** Medium
+
+**Topics:** `Array`, `Hash Table`, `Greedy`, `Sorting`
Alice has some number of cards and she wants to rearrange the cards into groups so that each group is of size groupSize, and consists of `groupSize` consecutive cards.
@@ -30,4 +32,114 @@ Given an integer array `hand` where `hand[i]` is the value written on the
- 0 <= hand[i] <= 109
- 1 <= groupSize <= hand.length
-**Note:** This question is the same as 1296: https://leetcode.com/problems/divide-array-in-sets-of-k-consecutive-numbers/
\ No newline at end of file
+**Note:** This question is the same as 1296: https://leetcode.com/problems/divide-array-in-sets-of-k-consecutive-numbers/
+
+
+
+
+**Solution:**
+
+The goal is to determine whether the array `hand` can be rearranged into groups of consecutive cards where each group has a size of `groupSize`.
+
+### Approach:
+
+1. **Key Idea**:
+ - We can use a greedy approach: first sort the cards, then attempt to form consecutive groups starting from the smallest card.
+ - For each group, reduce the count of each card as they are used to form the group. If any group can't be formed because a card is missing or insufficient in quantity, return `false`.
+
+2. **Frequency Count**:
+ - Use a hash table (associative array in PHP) to count the frequency of each card. This helps track how many of each card is available.
+
+3. **Greedy Strategy**:
+ - Sort the array to ensure that we always start forming groups from the smallest available card.
+ - For each card, try to form a group of `groupSize` consecutive cards.
+ - Decrease the count of each card in the group. If we can't find enough cards to form a valid group, return `false`.
+
+4. **Edge Case**:
+ - If the total number of cards is not divisible by `groupSize`, it's impossible to divide them into valid groups, so we can return `false` early.
+
+Let's implement this solution in PHP: **[846. Hand of Straights](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/000846-hand-of-straights/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+1. **Frequency Count** (`$count`):
+ - We use an associative array to store how many times each card appears in the `hand`.
+
+2. **Sort the Hand**:
+ - We sort the hand so that we always try to form groups starting from the smallest card, which simplifies the process of finding consecutive cards.
+
+3. **Greedy Group Formation**:
+ - For each card in the sorted hand:
+ - If the card has been fully used up in previous groups (i.e., its count is `0`), skip it.
+ - Attempt to form a group of `groupSize` consecutive cards starting from this card.
+ - If we can't find enough consecutive cards to form a group, return `false`.
+ - If we successfully form all the required groups, return `true`.
+
+### Time Complexity:
+- Sorting the array takes _**O(n log n)**_, where _**n**_ is the length of the hand.
+- Forming the groups takes _**O(n x groupSize)**_, but since we visit each card at most once, this is _**O(n)**_.
+- Overall time complexity: _**O(n log n)**_.
+
+### Output:
+
+For `hand = [1,2,3,6,2,3,4,7,8]` and `groupSize = 3`, the output is:
+```
+true
+```
+
+For `hand = [1,2,3,4,5]` and `groupSize = 4`, the output is:
+```
+false
+```
+
+For `hand = [2,1]` and `groupSize = 2`, the output is:
+```
+true
+```
+
+This solution efficiently checks if the hand can be rearranged into consecutive groups.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
+
+
+
diff --git a/algorithms/000846-hand-of-straights/solution.php b/algorithms/000846-hand-of-straights/solution.php
index d539c33c4..225e0f8a4 100644
--- a/algorithms/000846-hand-of-straights/solution.php
+++ b/algorithms/000846-hand-of-straights/solution.php
@@ -8,27 +8,46 @@ class Solution {
* @return Boolean
*/
function isNStraightHand($hand, $groupSize) {
- $cardCounts = array();
+ $n = count($hand);
+
+ // Early return if the number of cards is not divisible by groupSize
+ if ($n % $groupSize != 0) {
+ return false;
+ }
+
+ // Step 1: Count frequency of each card using a hash table
+ $count = [];
foreach ($hand as $card) {
- if (!isset($cardCounts[$card])) {
- $cardCounts[$card] = 1;
+ if (isset($count[$card])) {
+ $count[$card]++;
} else {
- $cardCounts[$card]++;
+ $count[$card] = 1;
}
}
+
+ // Step 2: Sort the hand to ensure we start from the smallest card
sort($hand);
+
+ // Step 3: Try to form groups starting from the smallest card
foreach ($hand as $card) {
- if (isset($cardCounts[$card])) {
- for ($nextCard = $card; $nextCard < $card + $groupSize; $nextCard++) {
- if (!isset($cardCounts[$nextCard])) {
- return false;
- }
- if (--$cardCounts[$nextCard] == 0) {
- unset($cardCounts[$nextCard]);
- }
+ // If this card has already been used up, skip it
+ if ($count[$card] == 0) {
+ continue;
+ }
+
+ // Try to form a group of consecutive cards
+ for ($i = 0; $i < $groupSize; $i++) {
+ $currentCard = $card + $i;
+ // If the card is missing or insufficient in number, return false
+ if (!isset($count[$currentCard]) || $count[$currentCard] == 0) {
+ return false;
}
+ // Decrease the count of the current card
+ $count[$currentCard]--;
}
}
+
+ // If we successfully formed all the groups, return true
return true;
}
}
\ No newline at end of file
diff --git a/algorithms/000857-minimum-cost-to-hire-k-workers/README.md b/algorithms/000857-minimum-cost-to-hire-k-workers/README.md
index ced8dea2a..26fcba60d 100644
--- a/algorithms/000857-minimum-cost-to-hire-k-workers/README.md
+++ b/algorithms/000857-minimum-cost-to-hire-k-workers/README.md
@@ -1,6 +1,8 @@
857\. Minimum Cost to Hire K Workers
-Hard
+**Difficulty:** Hard
+
+**Topics:** `Array`, `Greedy`, `Sorting`, `Heap (Priority Queue)`
There are `n` workers. You are given two integer arrays `quality` and wage where `quality[i]` is the quality of the ith
worker and `wage[i]` is the minimum wage expectation for the ith
worker.
@@ -31,7 +33,109 @@ Given the integer `k`, return _the least amount of money needed to form a paid g
**Constraints:**
-
- n == quality.length == wage.length
- 1 <= k <= n <= 104
- 1 <= quality[i], wage[i] <= 104
+
+
+
+**Solution:**
+
+We need to hire exactly `k` workers while ensuring two conditions:
+1. Each worker is paid at least their minimum wage.
+2. The pay is proportional to the worker's quality.
+
+### Key Insights:
+1. **Ratio of Wage to Quality**: The ratio `wage[i] / quality[i]` defines the minimum acceptable payment rate per unit of quality for worker `i`. If we decide to hire a worker at this ratio, every other worker in the group must be paid according to this ratio.
+2. **Greedy Approach**: To minimize the total cost, we must ensure that the payment is based on the smallest possible wage-to-quality ratio for the group of workers.
+
+### Steps:
+1. **Sort by wage-to-quality ratio**: We first sort the workers by their `wage[i] / quality[i]` ratio.
+2. **Use a Max-Heap for Quality**: As we iterate through the workers in increasing order of the wage-to-quality ratio, we maintain a group of exactly `k` workers. To ensure the total quality is minimized, we use a max-heap to keep track of the largest quality workers. If we encounter a worker with higher quality than one already in the heap, we remove the largest quality worker (since that would increase the total cost).
+3. **Calculate the Total Cost**: For each valid group of `k` workers, we compute the total cost as the sum of the qualities of the `k` workers multiplied by the current wage-to-quality ratio.
+
+Let's implement this solution in PHP: **[857. Minimum Cost to Hire K Workers](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/000857-minimum-cost-to-hire-k-workers/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+1. **Sorting**: First, we calculate the `wage/quality` ratio for each worker and sort them in ascending order. This allows us to consider groups with the smallest wage-to-quality ratio first.
+
+2. **Heap Maintenance**: We maintain a max-heap (using PHP's `SplMaxHeap`) to track the highest quality workers in our group of size `k`. If adding a new worker causes the heap to have more than `k` workers, we remove the one with the highest quality, as it would contribute more to the overall cost.
+
+3. **Cost Calculation**: For each valid group of `k` workers, we calculate the total wage as `qualitySum * wagePerQuality`. The minimum wage is updated with each new group of `k` workers.
+
+### Example Walkthrough:
+
+#### Example 1:
+- Input: `quality = [10, 20, 5]`, `wage = [70, 50, 30]`, `k = 2`
+- Step 1: Calculate the `wage/quality` ratio for each worker:
+ - Worker 0: `70/10 = 7`
+ - Worker 1: `50/20 = 2.5`
+ - Worker 2: `30/5 = 6`
+- Step 2: Sort workers by ratio: `[(2.5, 20), (6, 5), (7, 10)]`
+- Step 3: Use a max-heap to track the top `k=2` workers. The final cost is `105`.
+
+#### Example 2:
+- Input: `quality = [3, 1, 10, 10, 1]`, `wage = [4, 8, 2, 2, 7]`, `k = 3`
+- Step 1: Calculate the `wage/quality` ratio:
+ - Worker 0: `4/3 β 1.33`
+ - Worker 1: `8/1 = 8`
+ - Worker 2: `2/10 = 0.2`
+ - Worker 3: `2/10 = 0.2`
+ - Worker 4: `7/1 = 7`
+- Step 2: Sort workers: `[(0.2, 10), (0.2, 10), (1.33, 3), (7, 1), (8, 1)]`
+- Step 3: The final cost for `k=3` is `30.66667`.
+
+### Time Complexity:
+- Sorting the workers takes **O(n log n)**.
+- Inserting and removing from the heap takes **O(log k)**.
+- The overall complexity is **O(n log n)**, which is efficient given the constraints (`n <= 10^4`).
+
+This solution ensures that the least amount of money is paid while maintaining fairness and proportionality based on worker quality.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
diff --git a/algorithms/000857-minimum-cost-to-hire-k-workers/solution.php b/algorithms/000857-minimum-cost-to-hire-k-workers/solution.php
index 085265285..bac1c94ea 100644
--- a/algorithms/000857-minimum-cost-to-hire-k-workers/solution.php
+++ b/algorithms/000857-minimum-cost-to-hire-k-workers/solution.php
@@ -8,31 +8,40 @@ class Solution {
* @param Integer $k
* @return Float
*/
- function mincostToHireWorkers(array $quality, array $wage, int $k): float
- {
+ function mincostToHireWorkers($quality, $wage, $k) {
+ $n = count($quality);
+ $workers = array();
$ans = PHP_INT_MAX;
$qualitySum = 0;
- // (wagePerQuality, quality) sorted by wagePerQuality
- $workers = array();
- $maxHeap = new SplMaxHeap();
- for ($i = 0; $i < count($quality); ++$i) {
+ // (wagePerQuality, quality) sorted by wagePerQuality
+ for ($i = 0; $i < $n; ++$i) {
$workers[$i] = array((double) $wage[$i] / $quality[$i], $quality[$i]);
}
+ // Sort by wagePerQuality ratio
usort($workers, function($a, $b) {
return $a[0] <=> $b[0];
});
+ // MaxHeap to keep track of largest quality workers
+ $maxHeap = new SplMaxHeap();
+
foreach ($workers as $worker) {
$wagePerQuality = $worker[0];
$q = $worker[1];
- $maxHeap->insert($q);
$qualitySum += $q;
+ $maxHeap->insert($q);
+
+ // Ensure the heap contains at most 'k' workers
if ($maxHeap->count() > $k) {
+ // Remove the worker with the largest quality
$qualitySum -= $maxHeap->extract();
}
+
+ // If we have exactly 'k' workers, calculate the cost
if ($maxHeap->count() == $k) {
+ // Total cost is (total quality of 'k' workers) * (current wagePerQuality)
$ans = min($ans, $qualitySum * $wagePerQuality);
}
}
diff --git a/algorithms/000860-lemonade-change/README.md b/algorithms/000860-lemonade-change/README.md
new file mode 100644
index 000000000..a49cf801c
--- /dev/null
+++ b/algorithms/000860-lemonade-change/README.md
@@ -0,0 +1,79 @@
+860\. Lemonade Change
+
+**Difficulty:** Easy
+
+**Topics:** `Array`, `Greedy`
+
+At a lemonade stand, each lemonade costs `$5`. Customers are standing in a queue to buy from you and order one at a time (in the order specified by bills). Each customer will only buy one lemonade and pay with either a `$5`, `$10`, or `$20` bill. You must provide the correct change to each customer so that the net transaction is that the customer pays `$5`.
+
+**Note** that you do not have any change in hand at first.
+
+Given an integer array `bills` where `bills[i]` is the bill the ith
customer pays, return _`true` if you can provide every customer with the correct change, or `false` otherwise_.
+
+**Example 1:**
+
+- **Input:** bills = [5,5,5,10,20]
+- **Output:** true
+- **Explanation:**\
+ From the first 3 customers, we collect three $5 bills in order.\
+ From the fourth customer, we collect a $10 bill and give back a $5.\
+ From the fifth customer, we give a $10 bill and a $5 bill.\
+ Since all customers got correct change, we output true.
+
+**Example 2:**
+
+- **Input:** bills = [5,5,10,10,20]
+- **Output:** false
+- **Explanation:**\
+ From the first two customers in order, we collect two $5 bills.\
+ For the next two customers in order, we collect a $10 bill and give back a $5 bill.\
+ For the last customer, we can not give the change of $15 back because we only have two $10 bills.\
+ Since not every customer received the correct change, the answer is false.
+
+**Constraints:**
+
+- 5
+- `bills[i]` is either `5`, `10`, or `20`.
+
+
+**Solution:**
+
+
+We need to simulate the process of providing change to customers based on the bills they use to pay. The key is to track the number of $5 and $10 bills you have, as these are needed to provide change for larger bills
+
+Let's implement this solution in PHP: **[860. Lemonade Change](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/000860-lemonade-change/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+1. **Initialization**: We start with `$five` and `$ten` set to 0, representing the count of $5 and $10 bills we have.
+
+2. **Processing Each Bill**:
+ - **If the customer pays with a $5 bill**: We simply increment the count of $5 bills.
+ - **If the customer pays with a $10 bill**: We need to give back one $5 bill as change, so we decrement the count of $5 bills and increment the count of $10 bills. If we don't have any $5 bills, return `false`.
+ - **If the customer pays with a $20 bill**: We prioritize giving one $10 bill and one $5 bill as change. If that's not possible, we try to give three $5 bills. If neither option is available, return `false`.
+
+3. **Final Check**: If we've successfully processed all customers without running out of change, return `true`.
+
+### Edge Cases:
+- The function should handle scenarios where it's impossible to give the correct change, such as when you receive a $10 or $20 bill too early without having the necessary $5 bill(s) on hand.
+- It should efficiently handle large input sizes due to the constraints (up to 100,000 customers). The solution runs in O(n) time complexity, making it optimal for this problem.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
diff --git a/algorithms/000860-lemonade-change/solution.php b/algorithms/000860-lemonade-change/solution.php
new file mode 100644
index 000000000..ad2470f19
--- /dev/null
+++ b/algorithms/000860-lemonade-change/solution.php
@@ -0,0 +1,35 @@
+ 0 && $five > 0) {
+ $ten--;
+ $five--;
+ } elseif ($five >= 3) {
+ $five -= 3;
+ } else {
+ return false;
+ }
+ }
+ }
+ return true;
+ }
+}
\ No newline at end of file
diff --git a/algorithms/000861-score-after-flipping-matrix/README.md b/algorithms/000861-score-after-flipping-matrix/README.md
index ea37b16d3..0bfe91059 100644
--- a/algorithms/000861-score-after-flipping-matrix/README.md
+++ b/algorithms/000861-score-after-flipping-matrix/README.md
@@ -1,6 +1,8 @@
861\. Score After Flipping Matrix
-Medium
+**Difficulty:** Medium
+
+**Topics:** `Array`, `Greedy`, `Bit Manipulation`, `Matrix`
You are given an `m x n` binary matrix `grid`.
@@ -12,7 +14,7 @@ Return _the highest possible **score** after making any number of **moves** (inc
**Example 1:**
-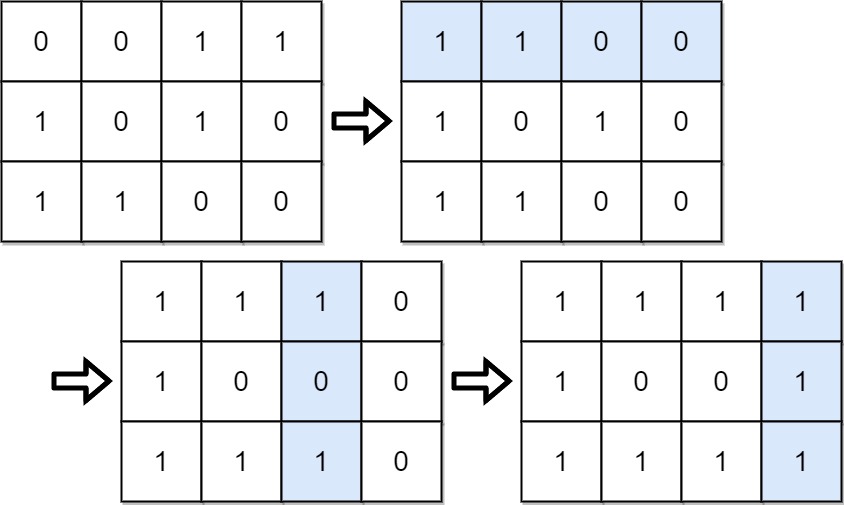
+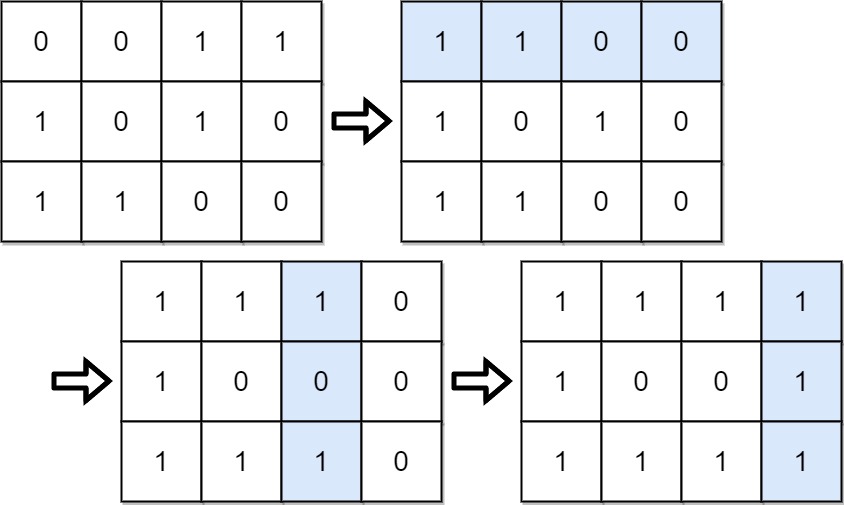
- **Input:** grid = [[0,0,1,1],[1,0,1,0],[1,1,0,0]]
- **Output:** 39
@@ -29,3 +31,97 @@ Return _the highest possible **score** after making any number of **moves** (inc
- `n == grid[i].length`
- `1 <= m, n <= 20`
- `grid[i][j]` is either `0` or `1`.
+
+
+
+**Solution:**
+
+We need to maximize the matrix score by flipping rows or columns. The goal is to interpret each row as a binary number and get the highest possible sum from all the rows.
+
+### Approach:
+
+1. **Initial Observation:**
+ - To maximize the binary number from each row, the most significant bit (leftmost bit) in each row should be `1`. This means we should first flip any row that starts with a `0`.
+ - After ensuring all rows start with `1`, we need to maximize the remaining bits of each row. This can be done by flipping columns to have as many `1`s as possible, especially for higher-bit positions.
+
+2. **Steps:**
+ - Flip any row that doesn't start with `1` to ensure the leftmost bit of every row is `1`.
+ - For each column from the second position onward, check if there are more `0`s than `1`s. If so, flip the column to maximize the number of `1`s in that column.
+ - Calculate the final score by interpreting each row as a binary number.
+
+Let's implement this solution in PHP: **[861. Score After Flipping Matrix](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/000861-score-after-flipping-matrix/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+1. **Row flipping to ensure the leftmost bit is `1`:**
+ - For each row, if the first element (most significant bit) is `0`, we flip the entire row. This guarantees that all rows start with `1`, which maximizes the contribution of the most significant bit.
+
+2. **Column flipping to maximize `1`s:**
+ - For each column starting from the second column, we count how many `1`s there are.
+ - If there are more `0`s than `1`s in a column, we flip the entire column to maximize the number of `1`s in that column.
+
+3. **Score calculation:**
+ - After flipping rows and columns, we compute the score by treating each row as a binary number. Each row is converted from binary to decimal and added to the total score.
+
+### Time Complexity:
+- **Row Flipping:** We iterate over all rows and columns once, so this takes `O(m * n)`.
+- **Column Flipping:** For each column, we count the number of `1`s, which takes `O(m * n)`.
+- **Score Calculation:** Converting rows to binary numbers takes `O(m * n)`.
+
+Thus, the total time complexity is `O(m * n)`, where `m` is the number of rows and `n` is the number of columns. Given the constraints (`m, n <= 20`), this is efficient enough.
+
+### Example Walkthrough:
+
+For the input:
+```php
+$grid = [[0,0,1,1],[1,0,1,0],[1,1,0,0]];
+```
+
+- After ensuring all rows start with `1`:
+ ```
+ [[1,1,0,0],[1,0,1,0],[1,1,0,0]]
+ ```
+
+- After flipping columns to maximize `1`s:
+ ```
+ [[1,1,1,1],[1,0,1,0],[1,1,1,1]]
+ ```
+
+- Final score:
+ - Row 1: `1111` = 15
+ - Row 2: `1001` = 9
+ - Row 3: `1111` = 15
+ - Total score = 15 + 9 + 15 = 39
+
+This gives the correct output of `39`.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
diff --git a/algorithms/000861-score-after-flipping-matrix/solution.php b/algorithms/000861-score-after-flipping-matrix/solution.php
index 76f3c2548..30adcfaa0 100644
--- a/algorithms/000861-score-after-flipping-matrix/solution.php
+++ b/algorithms/000861-score-after-flipping-matrix/solution.php
@@ -4,23 +4,49 @@ class Solution {
/**
* @param Integer[][] $grid
- * @return float|int
+ * @return Integer
*/
- function matrixScore(array $grid): float|int
- {
- $m = count($grid);
- $n = count($grid[0]);
- $ans = $m; // All the cells in the first column are 1.
+ function matrixScore($grid) {
+ $m = count($grid); // Number of rows
+ $n = count($grid[0]); // Number of columns
- for ($j = 1; $j < $n; ++$j) {
+ // Step 1: Ensure all rows start with 1 by flipping rows if necessary
+ for ($i = 0; $i < $m; $i++) {
+ if ($grid[$i][0] == 0) {
+ // Flip the entire row
+ for ($j = 0; $j < $n; $j++) {
+ $grid[$i][$j] = 1 - $grid[$i][$j];
+ }
+ }
+ }
+
+ // Step 2: Maximize the number of 1's in each column from the second column onwards
+ for ($j = 1; $j < $n; $j++) {
$onesCount = 0;
- for ($i = 0; $i < $m; ++$i) {
- // The best strategy is flipping the rows with a leading 0.
- $onesCount += $grid[$i][$j] == $grid[$i][0];
+ for ($i = 0; $i < $m; $i++) {
+ if ($grid[$i][$j] == 1) {
+ $onesCount++;
+ }
+ }
+
+ // If there are more 0's than 1's in this column, flip the column
+ if ($onesCount < $m / 2) {
+ for ($i = 0; $i < $m; $i++) {
+ $grid[$i][$j] = 1 - $grid[$i][$j];
+ }
+ }
+ }
+
+ // Step 3: Calculate the final score by interpreting each row as a binary number
+ $score = 0;
+ for ($i = 0; $i < $m; $i++) {
+ $rowValue = 0;
+ for ($j = 0; $j < $n; $j++) {
+ $rowValue = $rowValue * 2 + $grid[$i][$j];
}
- $ans = $ans * 2 + max($onesCount, $m - $onesCount);
+ $score += $rowValue;
}
- return $ans;
+ return $score;
}
}
\ No newline at end of file
diff --git a/algorithms/000862-shortest-subarray-with-sum-at-least-k/README.md b/algorithms/000862-shortest-subarray-with-sum-at-least-k/README.md
new file mode 100644
index 000000000..e938ff322
--- /dev/null
+++ b/algorithms/000862-shortest-subarray-with-sum-at-least-k/README.md
@@ -0,0 +1,120 @@
+862\. Shortest Subarray with Sum at Least K
+
+**Difficulty:** Hard
+
+**Topics:** `Array`, `Binary Search`, `Queue`, `Sliding Window`, `Heap (Priority Queue)`, `Prefix Sum`, `Monotonic Queue`
+
+Given an integer array `nums` and an integer `k`, return _the length of the shortest non-empty **subarray** of `nums` with a sum of at least `k`. If there is no such **subarray**, return `-1`_.
+
+A **subarray** is a **contiguous** part of an array.
+
+**Example 1:**
+
+- **Input:** nums = [1], k = 1
+- **Output:** 1
+
+**Example 2:**
+
+- **Input:** nums = [1,2], k = 4
+- **Output:** -1
+
+
+**Example 3:**
+
+- **Input:** nums = [2,-1,2], k = 3
+- **Output:** 3
+
+
+
+**Constraints:**
+
+- 1 <= nums.length <= 105
+- -105 <= nums[i] <= 105
+- 1 <= k <= 109
+
+
+**Solution:**
+
+We need to use a sliding window approach combined with prefix sums and a monotonic queue. Here's the step-by-step approach:
+
+### Steps:
+
+1. **Prefix Sum:**
+ - First, calculate the prefix sum array, where each element at index `i` represents the sum of the elements from the start of the array to `i`. The prefix sum allows us to compute the sum of any subarray in constant time.
+
+2. **Monotonic Queue:**
+ - We use a deque (double-ended queue) to maintain the indices of the `prefix_sum` array. The deque will be maintained in an increasing order of prefix sums.
+ - This helps us efficiently find subarrays with the sum greater than or equal to `k` by comparing the current prefix sum with earlier prefix sums.
+
+3. **Sliding Window Logic:**
+ - For each index `i`, check if the difference between the current prefix sum and any previous prefix sum (which is stored in the deque) is greater than or equal to `k`.
+ - If so, compute the length of the subarray and update the minimum length if necessary.
+
+### Algorithm:
+
+1. Initialize `prefix_sum` array with size `n+1` (where `n` is the length of the input array). The first element is `0` because the sum of zero elements is `0`.
+2. Use a deque to store indices of `prefix_sum` values. The deque will help to find the shortest subarray that satisfies the condition in an efficient manner.
+3. For each element in the array, update the `prefix_sum`, and check the deque to find the smallest subarray with sum greater than or equal to `k`.
+
+Let's implement this solution in PHP: **[862. Shortest Subarray with Sum at Least K](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/000862-shortest-subarray-with-sum-at-least-k/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+1. **Prefix Sum Array:**
+ - We compute the cumulative sum of the array in the `prefix_sum` array. This helps in calculating the sum of any subarray `nums[i...j]` by using the formula `prefix_sum[j+1] - prefix_sum[i]`.
+
+2. **Monotonic Queue:**
+ - The deque holds indices of the `prefix_sum` array in increasing order of values. We maintain this order so that we can efficiently find the smallest subarray whose sum is greater than or equal to `k`.
+
+3. **Sliding Window Logic:**
+ - As we traverse through the `prefix_sum` array, we try to find the smallest subarray using the difference between the current `prefix_sum[i]` and previous `prefix_sum[deque[0]]`.
+ - If the difference is greater than or equal to `k`, we calculate the subarray length and update the minimum length found.
+
+4. **Returning Result:**
+ - If no valid subarray is found, return `-1`. Otherwise, return the minimum subarray length.
+
+### Time Complexity:
+- **Time Complexity:** `O(n)`, where `n` is the length of the input array. Each element is processed at most twice (once when added to the deque and once when removed).
+- **Space Complexity:** `O(n)` due to the `prefix_sum` array and the deque used to store indices.
+
+This approach ensures that the solution runs efficiently even for large inputs.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
diff --git a/algorithms/000862-shortest-subarray-with-sum-at-least-k/solution.php b/algorithms/000862-shortest-subarray-with-sum-at-least-k/solution.php
new file mode 100644
index 000000000..00c2372af
--- /dev/null
+++ b/algorithms/000862-shortest-subarray-with-sum-at-least-k/solution.php
@@ -0,0 +1,44 @@
+ 0 && $prefix_sum[$i] - $prefix_sum[$deque[0]] >= $k) {
+ $minLength = min($minLength, $i - $deque[0]);
+ array_shift($deque); // Remove the front of the queue.
+ }
+
+ // Maintain the deque: remove all indices where prefix_sum[i] is less than the current value.
+ while (count($deque) > 0 && $prefix_sum[$i] <= $prefix_sum[$deque[count($deque) - 1]]) {
+ array_pop($deque); // Remove the back of the queue.
+ }
+
+ // Add current index to the deque.
+ array_push($deque, $i);
+ }
+
+ return $minLength == PHP_INT_MAX ? -1 : $minLength;
+ }
+}
\ No newline at end of file
diff --git a/algorithms/000874-walking-robot-simulation/README.md b/algorithms/000874-walking-robot-simulation/README.md
new file mode 100644
index 000000000..474791ef8
--- /dev/null
+++ b/algorithms/000874-walking-robot-simulation/README.md
@@ -0,0 +1,144 @@
+874\. Walking Robot Simulation
+
+**Difficulty:** Medium
+
+**Topics:** `Array`, `Hash Table`, `Simulation`
+
+A robot on an infinite XY-plane starts at point `(0, 0)` facing north. The robot can receive a sequence of these three possible types of `commands`:
+
+- `-2:` Turn left `90` degrees.
+- `-1:` Turn right `90` degrees.
+- `1 <= k <= 9:` Move forward `k` units, one unit at a time.
+
+Some of the grid squares are `obstacles`. The ith
obstacle is at grid point obstacles[i] = (xi, yi)
. If the robot runs into an obstacle, then it will instead stay in its current location and move on to the next command.
+
+Return _the **maximum Euclidean distance** that the robot ever gets from the origin **squared** (i.e. if the distance is `5`, return `25`).
+
+**Note:**
+
+- North means `+Y direction`.
+- East means `+X direction`.
+- South means `-Y direction`.
+- West means `-X direction`.
+- There can be obstacle in `[0,0]`.
+
+
+**Example 1:**
+
+- **Input:** commands = [4,-1,3], obstacles = []
+- **Output:** 25
+- **Explanation:** The robot starts at (0, 0):
+ 1. Move north 4 units to (0, 4).
+ 2. Turn right.
+ 3. Move east 3 units to (3, 4).\
+ The furthest point the robot ever gets from the origin is (3, 4), which squared is 32 + 42 = 25 units away.
+
+**Example 2:**
+
+- **Input:** commands = [4,-1,4,-2,4], obstacles = [[2,4]]
+- **Output:** 65
+- **Explanation:** The robot starts at (0, 0):
+ 1. Move north 4 units to (0, 4).
+ 2. Turn right.
+ 3. Move east 1 unit and get blocked by the obstacle at (2, 4), robot is at (1, 4).
+ 4. Turn left.
+ 5. Move north 4 units to (1, 8).\
+ The furthest point the robot ever gets from the origin is (1, 8), which squared is 12 + 82 = 65 units away.
+
+
+**Example 3:**
+
+- **Input:** commands = [6,-1,-1,6], obstacles = []
+- **Output:** 36
+- Explanation: The robot starts at (0, 0):
+ 1. Move north 6 units to (0, 6).
+ 2. Turn right.
+ 3. Turn right.
+ 4. Move south 6 units to (0, 0).\
+ The furthest point the robot ever gets from the origin is (0, 6), which squared is 62 = 36 units away.
+
+
+
+**Constraints:**
+
+- 1 <= commands.length <= 104
+- `commands[i]` is either `-2`, `-1`, or an integer in the range `[1, 9]`.
+- 0 <= obstacles.length <= 104
+- -3 * 104 <= xi, yi <= 3 * 104
+- The answer is guaranteed to be less than 231
+
+
+**Solution:**
+
+We need to simulate the robot's movement on an infinite 2D grid based on a sequence of commands and avoid obstacles if any. The goal is to determine the maximum Euclidean distance squared that the robot reaches from the origin.
+
+### Approach
+
+1. **Direction Handling**:
+ - The robot can face one of four directions: North, East, South, and West.
+ - We can represent these directions as vectors:
+ - North: `(0, 1)`
+ - East: `(1, 0)`
+ - South: `(0, -1)`
+ - West: `(-1, 0)`
+
+2. **Turning**:
+ - A left turn `(-2)` will shift the direction counterclockwise by 90 degrees.
+ - A right turn `(-1)` will shift the direction clockwise by 90 degrees.
+
+3. **Movement**:
+ - For each move command, the robot will move in its current direction, one unit at a time. If it encounters an obstacle, it stops moving for that command.
+
+4. **Tracking Obstacles**:
+ - Convert the obstacles list into a set of tuples for quick lookup, allowing the robot to quickly determine if it will hit an obstacle.
+
+5. **Distance Calculation**:
+ - Track the maximum distance squared from the origin that the robot reaches during its movements.
+
+Let's implement this solution in PHP: **[874\. Walking Robot Simulation](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/000874-walking-robot-simulation/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+- **Direction Management**: We use a list of vectors to represent the directions, allowing easy calculation of the next position after moving.
+- **Obstacle Detection**: By storing obstacles in a set, we achieve O(1) time complexity for checking if a position is blocked by an obstacle.
+- **Distance Calculation**: We continuously update the maximum squared distance the robot reaches as it moves.
+
+### Test Cases
+- The example test cases provided are used to validate the solution:
+ - `[4,-1,3]` with no obstacles should return `25`.
+ - `[4,-1,4,-2,4]` with obstacles `[[2,4]]` should return `65`.
+ - `[6,-1,-1,6]` with no obstacles should return `36`.
+
+This solution efficiently handles the problem constraints and calculates the maximum distance squared as required.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
diff --git a/algorithms/000874-walking-robot-simulation/solution.php b/algorithms/000874-walking-robot-simulation/solution.php
new file mode 100644
index 000000000..8bb6480a7
--- /dev/null
+++ b/algorithms/000874-walking-robot-simulation/solution.php
@@ -0,0 +1,55 @@
+th person, and an infinite number of boats where each boat can carry a maximum weight of `limit`. Each boat carries at most two people at the same time, provided the sum of the weight of those people is at most `limit`.
@@ -27,4 +29,92 @@ Return _the minimum number of boats to carry every given person._
**Constraints:**
* 1 <= people.length <= 5 * 104
-* 1 <= people[i] <= limit <= 3 * 104
\ No newline at end of file
+* 1 <= people[i] <= limit <= 3 * 104
+
+
+
+**Solution:**
+
+We can use a **two-pointer** greedy strategy combined with sorting. Here's the detailed approach:
+
+1. **Sort the Array**:
+ - First, sort the `people` array. Sorting helps us to easily pair the lightest and heaviest person together in one boat, if possible.
+
+2. **Two Pointer Strategy**:
+ - Use two pointers: one starting from the lightest person (`left`), and the other starting from the heaviest person (`right`).
+ - Try to pair the heaviest person (`right`) with the lightest person (`left`). If the sum of their weights is less than or equal to the limit, they can share the same boat. Move both pointers (`left++` and `right--`).
+ - If they cannot be paired, send the heaviest person alone on a boat and move only the `right` pointer (`right--`).
+
+3. **Count Boats**:
+ - Each time we process a person (or pair), we count it as one boat.
+ - Continue until all people are assigned to boats.
+
+Let's implement this solution in PHP: **[881. Boats to Save People](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/000881-boats-to-save-people/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+1. **Sorting**:
+ - For the example `[3, 2, 2, 1]`, after sorting, it becomes `[1, 2, 2, 3]`.
+
+2. **Two-pointer logic**:
+ - Start with `left = 0` (lightest person, weight 1) and `right = 3` (heaviest person, weight 3).
+ - Check if `people[0] + people[3]` (1 + 3) is less than or equal to the limit (3). It is not, so send the heaviest person alone and decrement `right` to 2.
+ - Now check `people[0] + people[2]` (1 + 2), which fits the limit. Pair them together and move both pointers (`left = 1`, `right = 1`).
+ - The remaining person (weight 2) takes their own boat.
+
+3. **Boat Counting**:
+ - In each iteration, a boat is used whether we pair two people or send one person alone. This guarantees we use the minimum number of boats.
+
+### Time Complexity:
+
+- **Sorting the array**: Sorting takes `O(n log n)` where `n` is the number of people.
+- **Two-pointer traversal**: The two-pointer approach runs in `O(n)` because each pointer moves at most `n` times.
+
+Thus, the overall time complexity is `O(n log n)` due to sorting.
+
+### Space Complexity:
+- The space complexity is `O(1)` because no extra space is used beyond a few pointers and variables.
+
+### Example Walkthrough:
+
+For the input:
+```php
+$people = [3,5,3,4]; $limit = 5;
+```
+
+1. After sorting: `[3, 3, 4, 5]`.
+2. Initial pointers: `left = 0`, `right = 3` (pointing at 5).
+3. Check if `people[0] + people[3]` (3 + 5) β€ 5 β False. The heaviest person (5) goes alone. `right--`.
+4. Check if `people[0] + people[2]` (3 + 4) β€ 5 β False. The next heaviest person (4) goes alone. `right--`.
+5. Check if `people[0] + people[1]` (3 + 3) β€ 5 β False. Each person (3) goes alone.
+
+Thus, the final output is `4` boats.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
diff --git a/algorithms/000881-boats-to-save-people/solution.php b/algorithms/000881-boats-to-save-people/solution.php
index 1e1e16aa8..f32a17c66 100644
--- a/algorithms/000881-boats-to-save-people/solution.php
+++ b/algorithms/000881-boats-to-save-people/solution.php
@@ -7,23 +7,23 @@ class Solution {
* @param Integer $limit
* @return Integer
*/
- function numRescueBoats(array $people, int $limit): int
- {
- $ans = 0;
- $i = 0;
- $j = count($people) - 1;
+ function numRescueBoats($people, $limit) {
+ sort($people); // Step 1: Sort the array
+ $left = 0; // Pointer for the lightest person
+ $right = count($people) - 1; // Pointer for the heaviest person
+ $boats = 0;
- sort($people);
-
- while ($i <= $j) {
- $remain = $limit - $people[$j];
- $j -= 1;
- if ($people[$i] <= $remain) {
- $i += 1;
+ // Step 2: Use two pointers to try and pair people
+ while ($left <= $right) {
+ if ($people[$left] + $people[$right] <= $limit) {
+ // If they can share a boat, move both pointers
+ $left++;
}
- $ans += 1;
+ // Whether they share or not, the heaviest person takes a boat
+ $right--;
+ $boats++; // Count the boat used
}
- return $ans;
+ return $boats; // Return the total number of boats
}
}
\ No newline at end of file
diff --git a/algorithms/000884-uncommon-words-from-two-sentences/README.md b/algorithms/000884-uncommon-words-from-two-sentences/README.md
new file mode 100644
index 000000000..19b31bbd1
--- /dev/null
+++ b/algorithms/000884-uncommon-words-from-two-sentences/README.md
@@ -0,0 +1,108 @@
+884\. Uncommon Words from Two Sentences
+
+**Difficulty:** Easy
+
+**Topics:** `Hash Table`, `String`, `Counting`
+
+A **sentence** is a string of single-space separated words where each word consists only of lowercase letters.
+
+A word is **uncommon** if it appears exactly once in one of the sentences, and **does not appear** in the other sentence.
+
+Given two **sentences** `s1` and `s2`, return a list of all the **uncommon words**. You may return the answer in **any order**.
+
+**Example 1:**
+
+- **Input:** s1 = "this apple is sweet", s2 = "this apple is sour"
+- **Output:** ["sweet","sour"]
+- **Explanation:** The word `"sweet"` appears only in `s1`, while the word `"sour"` appears only in `s2`.
+
+**Example 2:**
+
+- **Input:** s1 = "apple apple", s2 = "banana"
+- **Output:** ["banana"]
+
+
+
+**Constraints:**
+
+- 1 <= s1.length, s2.length <= 200
+- `s1` and `s2` consist of lowercase English letters and spaces.
+- `s1` and `s2` do not have leading or trailing spaces.
+- All the words in `s1` and `s2` are separated by a single space.
+
+
+**Solution:**
+
+We can follow these steps:
+
+1. **Break the sentences into words**: Split both `s1` and `s2` into individual words.
+2. **Count the occurrences of each word**: Use an associative array (hash table) to count how many times each word appears across both sentences.
+3. **Filter uncommon words**: Find words that appear exactly once in the combined set of words from both sentences and do not appear in both sentences.
+
+### Approach:
+- Use the `explode` function to split the sentences into arrays of words.
+- Use an associative array to count the frequency of each word.
+- Return the words that have a count of 1.
+
+Let's implement this solution in PHP: **[884. Uncommon Words from Two Sentences](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/000884-uncommon-words-from-two-sentences/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+1. **Splitting the sentences**: We use `explode(' ', $s1)` and `explode(' ', $s2)` to split the sentences into arrays of words.
+2. **Counting occurrences**: We loop through both arrays of words, incrementing the count of each word in the associative array `$wordCount`.
+3. **Filtering uncommon words**: We then check for words that have a count of exactly 1 in the `$wordCount` array and add them to the result array.
+
+### Example Walkthrough:
+- For `s1 = "this apple is sweet"` and `s2 = "this apple is sour"`, after counting, the associative array `$wordCount` will look like:
+ ```
+ Array (
+ [this] => 2
+ [apple] => 2
+ [is] => 2
+ [sweet] => 1
+ [sour] => 1
+ )
+ ```
+ The words with a count of 1 are `sweet` and `sour`, so the result is `["sweet", "sour"]`.
+
+### Time Complexity:
+- **O(n + m)**, where `n` is the number of words in `s1` and `m` is the number of words in `s2`. This is because we iterate through all the words in both sentences once to count the occurrences.
+
+### Space Complexity:
+- **O(n + m)**, where `n` and `m` are the number of words in `s1` and `s2`. We store all the words in an associative array to track their counts.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
diff --git a/algorithms/000884-uncommon-words-from-two-sentences/solution.php b/algorithms/000884-uncommon-words-from-two-sentences/solution.php
new file mode 100644
index 000000000..16420ec8f
--- /dev/null
+++ b/algorithms/000884-uncommon-words-from-two-sentences/solution.php
@@ -0,0 +1,46 @@
+ $count) {
+ if ($count == 1) {
+ $result[] = $word;
+ }
+ }
+
+ return $result;
+
+ }
+}
\ No newline at end of file
diff --git a/algorithms/000916-word-subsets/README.md b/algorithms/000916-word-subsets/README.md
new file mode 100644
index 000000000..5a9a022a6
--- /dev/null
+++ b/algorithms/000916-word-subsets/README.md
@@ -0,0 +1,105 @@
+916\. Word Subsets
+
+**Difficulty:** Medium
+
+**Topics:** `Array`, `Hash Table`, `String`
+
+You are given two string arrays `words1` and `words2`.
+
+A string `b` is a **subset** of string `a` if every letter in `b` occurs in `a` including multiplicity.
+
+- For example, `"wrr"` is a subset of `"warrior"` but is not a subset of `"world"`.
+
+A string `a` from `words1` is **universal** if for every string `b` in `words2`, `b` is a subset of `a`.
+
+Return _an array of all the **universal** strings in `words1`_. You may return the answer in **any order**.
+
+**Example 1:**
+
+- **Input:** words1 = ["amazon","apple","facebook","google","leetcode"], words2 = ["e","o"]
+- **Output:** ["facebook","google","leetcode"]
+
+**Example 2:**
+
+- **Input:** words1 = ["amazon","apple","facebook","google","leetcode"], words2 = ["l","e"]
+- **Output:** ["apple","google","leetcode"]
+
+
+
+**Constraints:**
+
+- 1 <= words1.length, words2.length <= 104
+- `1 <= words1[i].length, words2[i].length <= 10`
+- `words1[i]` and `words2[i]` consist only of lowercase English letters.
+- All the strings of `words1` are **unique**.
+
+
+
+
+**Solution:**
+
+We need to identify the words in `words1` that are "universal", meaning each string in `words2` is a subset of the word from `words1`.
+
+### Approach:
+
+1. **Count the Frequency of Characters in `words2`:**
+ - First, we need to determine the maximum count for each character across all strings in `words2`. This gives us the required number of occurrences for each character to be a subset.
+
+2. **Check Each Word in `words1`:**
+ - For each word in `words1`, count the frequency of each character.
+ - If the character counts in the word from `words1` meet or exceed the required counts from `words2`, then the word is universal.
+
+3. **Return the Universal Words:**
+ - After checking all words in `words1`, return the ones that are universal.
+
+Let's implement this solution in PHP: **[916. Word Subsets](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/000916-word-subsets/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+1. **Building Frequency Map for `words2`:** We loop through each word in `words2` and calculate the frequency of each character. We keep track of the maximum frequency needed for each character across all words in `words2`.
+
+2. **Checking `words1` Words:** For each word in `words1`, we calculate the frequency of each character and compare it with the required frequency from `words2`. If the word meets the requirements for all characters, it's considered universal.
+
+3. **Result:** We store all the universal words in the result array and return it at the end.
+
+### Time Complexity:
+- **Building the frequency map for `words2`:** O(n * m), where `n` is the length of `words2` and `m` is the average length of words in `words2`.
+- **Checking `words1`:** O(k * m), where `k` is the length of `words1` and `m` is the average length of words in `words1`.
+- The total time complexity is approximately **O(n * m + k * m)**.
+
+This approach ensures that we check each word efficiently and meets the problem's constraints.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
diff --git a/algorithms/000916-word-subsets/solution.php b/algorithms/000916-word-subsets/solution.php
new file mode 100644
index 000000000..a747ebca0
--- /dev/null
+++ b/algorithms/000916-word-subsets/solution.php
@@ -0,0 +1,43 @@
+ $c) {
+ $freqB[$char] = max(isset($freqB[$char]) ? $freqB[$char] : 0, $c);
+ }
+ }
+
+ // Step 2: Check each word in words1
+ $result = [];
+ foreach ($words1 as $word) {
+ $countA = count_chars($word, 1); // get character counts for the word in words1
+ $isValid = true;
+
+ // Step 3: Check if the word in words1 meets the required character frequencies
+ foreach ($freqB as $char => $freq) {
+ if (isset($countA[$char]) && $countA[$char] >= $freq) {
+ continue;
+ }
+ $isValid = false;
+ break;
+ }
+
+ // Step 4: If valid, add the word to the result
+ if ($isValid) {
+ $result[] = $word;
+ }
+ }
+
+ return $result;
+ }
+}
\ No newline at end of file
diff --git a/algorithms/000921-minimum-add-to-make-parentheses-valid/README.md b/algorithms/000921-minimum-add-to-make-parentheses-valid/README.md
new file mode 100644
index 000000000..974b6c2b0
--- /dev/null
+++ b/algorithms/000921-minimum-add-to-make-parentheses-valid/README.md
@@ -0,0 +1,98 @@
+921\. Minimum Add to Make Parentheses Valid
+
+**Difficulty:** Medium
+
+**Topics:** `String`, `Stack`, `Greedy`
+
+A parentheses string is valid if and only if:
+
+- It is the empty string,
+- It can be written as `AB` (`A` concatenated with `B`), where `A` and `B` are valid strings, or
+- It can be written as `(A)`, where `A` is a valid string.
+
+You are given a parentheses string `s`. In one move, you can insert a parenthesis at any position of the string.
+
+- For example, if `s = "()))"`, you can insert an opening parenthesis to be `"(()))"` or a closing parenthesis to be `"())))"`.
+
+Return _the minimum number of moves required to make `s` valid_.
+
+**Example 1:**
+
+- **Input:** s = "())"
+- **Output:** 1
+
+**Example 2:**
+
+- **Input:** s = "((("
+- **Output:** 3
+
+**Constraints:**
+
+- `1 <= s.length <= 1000`
+- `s[i]` is either `'('` or `')'`.
+
+
+
+**Solution:**
+
+We need to determine how many opening or closing parentheses need to be added to make the input string `s` valid. A valid string means that every opening parenthesis `'('` has a corresponding closing parenthesis `')'`.
+
+We can solve this problem using a simple counter approach:
+
+- We use a variable `balance` to keep track of the current balance between opening and closing parentheses.
+- We use another variable `additions` to count the minimum number of parentheses required.
+
+### Approach:
+
+1. Loop through each character of the string `s`.
+2. If the character is `'('`, increment `balance` by 1.
+3. If the character is `')'`, decrement `balance` by 1:
+ - If `balance` becomes negative, it means there are more closing parentheses than opening ones. We need to add an opening parenthesis to balance it, so increment `additions` by 1 and reset `balance` to 0.
+4. At the end of the loop, if `balance` is greater than 0, it indicates there are unmatched opening parentheses, so add `balance` to `additions`.
+
+Let's implement this solution in PHP: **[921. Minimum Add to Make Parentheses Valid](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/000921-minimum-add-to-make-parentheses-valid/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+
+- For the string `s = "())"`:
+ - `balance` becomes negative when the second `')'` is encountered, so `additions` is incremented.
+ - At the end, `balance` is 0, and `additions` is 1, so we need 1 addition to make the string valid.
+- For the string `s = "((("`:
+ - `balance` becomes 3 because there are 3 unmatched `'('` at the end.
+ - The result is `additions + balance`, which is `0 + 3 = 3`.
+
+This solution has a time complexity of _**O(n)**_ where _**n**_ is the length of the string, and a space complexity of _**O(1)**_ since we only use a few variables.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
\ No newline at end of file
diff --git a/algorithms/000921-minimum-add-to-make-parentheses-valid/solution.php b/algorithms/000921-minimum-add-to-make-parentheses-valid/solution.php
new file mode 100644
index 000000000..03f5c2339
--- /dev/null
+++ b/algorithms/000921-minimum-add-to-make-parentheses-valid/solution.php
@@ -0,0 +1,30 @@
+1 <= nums.length <= 105
-- 0 <= nums[i] <= 105
\ No newline at end of file
+- 0 <= nums[i] <= 105
+
+
+**Solution:**
+
+The problem asks us to make every number in a given integer array unique. We can increment any number in the array in one move, and the goal is to minimize the total number of moves required to ensure that each element is distinct.
+
+### Key Points:
+- You are allowed to increment numbers in the array.
+- The task is to find the minimum number of moves necessary to make all numbers unique.
+- You need to handle potentially large arrays, so efficiency is important.
+
+### Approach:
+The strategy involves sorting the array and then iterating over it, ensuring each number is greater than the last (if a number repeats). If a number is smaller than or equal to the previous number (or the minimum available number), we increment it and count the moves.
+
+- **Sorting the array** ensures that we process the numbers in ascending order, making it easy to see if a number is already taken by a previous one.
+- We maintain a variable `minAvailable` which tracks the smallest number that can be assigned to a number (starting from 0).
+- If a number in the array is less than `minAvailable`, we must increment it to `minAvailable` and update the counter for moves.
+
+### Plan:
+1. **Sort the array** to arrange the numbers in increasing order.
+2. Initialize two variables:
+ - `ans`: To store the total number of moves.
+ - `minAvailable`: To track the next available unique number.
+3. Loop through the sorted array:
+ - For each number, if it's less than `minAvailable`, increment it until it's unique.
+ - Update `minAvailable` to be the next available number.
+4. Return the total number of moves.
+
+Let's implement this solution in PHP: **[945. Minimum Increment to Make Array Unique](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/000945-minimum-increment-to-make-array-unique/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+1. **Sorting**: The array is sorted so that we can easily compare each number to the previous one.
+2. **Loop**: For each number, if it's smaller than `minAvailable`, it needs to be incremented. We update `minAvailable` to be one more than the current number after it's incremented.
+3. **Counting moves**: Every time a number is incremented to make it unique, we add to the total moves.
+
+### Example Walkthrough:
+
+#### Example 1:
+- **Input**: nums = [1, 2, 2]
+- **Sorted**: [1, 2, 2]
+- **Initial `minAvailable`**: 0
+ - First number (1): No increment needed. `minAvailable` becomes 2.
+ - Second number (2): No increment needed. `minAvailable` becomes 3.
+ - Third number (2): Since `minAvailable` is 3, we increment 2 to 3. So, the total moves = 1.
+- **Output**: 1
+
+#### Example 2:
+- **Input**: nums = [3, 2, 1, 2, 1, 7]
+- **Sorted**: [1, 1, 2, 2, 3, 7]
+- **Initial `minAvailable`**: 0
+ - First number (1): No increment needed. `minAvailable` becomes 2.
+ - Second number (1): Increment it to 2. Total moves = 1. `minAvailable` becomes 3.
+ - Third number (2): Increment it to 3. Total moves = 2. `minAvailable` becomes 4.
+ - Fourth number (2): Increment it to 4. Total moves = 4. `minAvailable` becomes 5.
+ - Fifth number (3): Increment it to 5. Total moves = 6. `minAvailable` becomes 6.
+ - Sixth number (7): No increment needed. `minAvailable` becomes 8.
+- **Output**: 6
+
+### Time Complexity:
+- **Sorting**: The sorting operation takes _**O(n log n)**_, where _**n**_ is the length of the array.
+- **Iteration**: The iteration through the array is _**O(n)**_.
+ Thus, the overall time complexity is _**O(n log n)**_, dominated by the sorting step.
+
+### Output for Example:
+#### Example 1:
+- **Input**: [1, 2, 2]
+- **Output**: 1
+
+#### Example 2:
+- **Input**: [3, 2, 1, 2, 1, 7]
+- **Output**: 6
+
+The approach efficiently solves the problem by sorting the array and using a greedy strategy to make each element unique. By processing the elements in ascending order, we ensure that we minimize the number of moves required to resolve duplicates. The solution has a time complexity of _**O(n log n)**_, making it feasible even for large inputs (up to _**105**_ elements).
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
\ No newline at end of file
diff --git a/algorithms/000947-most-stones-removed-with-same-row-or-column/README.md b/algorithms/000947-most-stones-removed-with-same-row-or-column/README.md
new file mode 100644
index 000000000..28280b7f7
--- /dev/null
+++ b/algorithms/000947-most-stones-removed-with-same-row-or-column/README.md
@@ -0,0 +1,128 @@
+947\. Most Stones Removed with Same Row or Column
+
+**Difficulty:** Medium
+
+**Topics:** `Hash Table`, `Depth-First Search`, `Union Find`, `Graph`
+
+On a 2D plane, we place `n` stones at some integer coordinate points. Each coordinate point may have at most one stone.
+
+A stone can be removed if it shares either **the same row or the same column** as another stone that has not been removed.
+
+Given an array `stones` of length n where `stones[i] = [xi, yi]` represents the location of the ith
stone, return _the largest possible number of stones that can be removed_.
+
+**Example 1:**
+
+- **Input:** stones = [[0,0],[0,1],[1,0],[1,2],[2,1],[2,2]]
+- **Output:** 5
+- **Explanation:** One way to remove 5 stones is as follows:
+ 1. Remove stone [2,2] because it shares the same row as [2,1].
+ 2. Remove stone [2,1] because it shares the same column as [0,1].
+ 3. Remove stone [1,2] because it shares the same row as [1,0].
+ 4. Remove stone [1,0] because it shares the same column as [0,0].
+ 5. Remove stone [0,1] because it shares the same row as [0,0].
+ 6. Stone [0,0] cannot be removed since it does not share a row/column with another stone still on the plane.
+
+**Example 2:**
+
+- **Input:** stones = [[0,0],[0,2],[1,1],[2,0],[2,2]]
+- **Output:** 3
+- **Explanation:** One way to make 3 moves is as follows:
+ 1. Remove stone [2,2] because it shares the same row as [2,0].
+ 2. Remove stone [2,0] because it shares the same column as [0,0].
+ 3. Remove stone [0,2] because it shares the same row as [0,0].
+ 4. Stones [0,0] and [1,1] cannot be removed since they do not share a row/column with another stone still on the plane.
+
+**Example 3:**
+
+- **Input:** stones = [[0,0]]
+- **Output:** 0
+- **Explanation:** [0,0] is the only stone on the plane, so you cannot remove it.
+
+**Constraints:**
+
+- `1 <= stones.length <= 1000`
+- 0 <= xi, yi <= 104
+- No two stones are at the same coordinate point.
+
+
+**Solution:**
+
+We can implement the solution using a Depth-First Search (DFS) approach. The idea is to consider stones that are connected by rows or columns as part of the same connected component. Once you find all connected components, the maximum number of stones that can be removed is the total number of stones minus the number of connected components.
+
+Let's implement this solution in PHP: **[947. Most Stones Removed with Same Row or Column](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/000947-most-stones-removed-with-same-row-or-column/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+1. **DFS Function**:
+ - The `dfs` function is used to explore all stones that are in the same connected component. If a stone is connected (in the same row or column) to the current stone, we recursively perform DFS on that stone.
+
+2. **Main Function**:
+ - We iterate over all stones, and for each stone that hasn't been visited, we perform a DFS to mark all stones in the same connected component.
+ - We count the number of connected components, and the result is the total number of stones minus the number of connected components (`$n - $numComponents`).
+
+3. **Example Execution**:
+ - For the first example, it correctly finds 5 stones can be removed, leaving 1 stone that cannot be removed.
+
+### Complexity:
+- **Time Complexity**: O(n^2) due to the nested loops and DFS traversal.
+- **Space Complexity**: O(n) for storing visited stones.
+
+This solution should work efficiently within the given constraints.
+
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
+
diff --git a/algorithms/000947-most-stones-removed-with-same-row-or-column/solution.php b/algorithms/000947-most-stones-removed-with-same-row-or-column/solution.php
new file mode 100644
index 000000000..0d648dd34
--- /dev/null
+++ b/algorithms/000947-most-stones-removed-with-same-row-or-column/solution.php
@@ -0,0 +1,40 @@
+dfs($i, $stones, $visited);
+ $numComponents++;
+ }
+ }
+
+ return $n - $numComponents;
+ }
+
+ /**
+ * @param $stoneIndex
+ * @param $stones
+ * @param $visited
+ * @return void
+ */
+ function dfs($stoneIndex, &$stones, &$visited) {
+ $visited[$stoneIndex] = true;
+ for ($i = 0; $i < count($stones); $i++) {
+ if (!isset($visited[$i]) && ($stones[$i][0] == $stones[$stoneIndex][0] || $stones[$i][1] == $stones[$stoneIndex][1])) {
+ self::dfs($i, $stones, $visited);
+ }
+ }
+ }
+
+}
\ No newline at end of file
diff --git a/algorithms/000950-reveal-cards-in-increasing-order/README.md b/algorithms/000950-reveal-cards-in-increasing-order/README.md
index 3297a71c5..16628a259 100644
--- a/algorithms/000950-reveal-cards-in-increasing-order/README.md
+++ b/algorithms/000950-reveal-cards-in-increasing-order/README.md
@@ -1,6 +1,8 @@
950\. Reveal Cards In Increasing Order
-Medium
+**Difficulty:** Medium
+
+**Topics:** `Array`, `Queue`, `Sorting`, `Simulation`
You are given an integer array `deck`. There is a deck of cards where every card has a unique integer. The integer on the ith
card is `deck[i]`.
@@ -12,7 +14,7 @@ You will do the following steps repeatedly until all cards are revealed:
2. If there are still cards in the deck then put the next top card of the deck at the bottom of the deck.
3. If there are still unrevealed cards, go back to step 1. Otherwise, stop.
-Return an ordering of the deck that would reveal the cards in increasing order.
+Return _an ordering of the deck that would reveal the cards in increasing order_.
**Note** that the first entry in the answer is considered to be the top of the deck.
@@ -47,3 +49,110 @@ Return an ordering of the deck that would reveal the cards in increasing orde
- 1 <= deck[i] <= 106
- All the values of `deck` are **unique**.
+
+**Solution:**
+
+The problem "Reveal Cards in Increasing Order" is a simulation-based problem where we are tasked with revealing cards in a specific way such that the revealed sequence is in increasing order. The key challenge lies in finding the initial arrangement of cards to achieve the desired order.
+
+
+### Key Points
+- We can sort the input `deck` array.
+- Using a simulation, we aim to arrange the deck such that following the given process of revealing and moving cards, the result is in ascending order.
+- Data structures like a deque (double-ended queue) can simplify the problem.
+
+
+### Approach
+1. **Sort the Deck**: Begin by sorting the deck in descending order. This simplifies managing the "largest card first" strategy for simulation.
+2. **Simulate the Revealing Process**: Use a deque to simulate the process:
+ - If the deque is not empty, move the card at the bottom of the deque to the front.
+ - Add the current card from the sorted deck to the front of the deque.
+3. **Output the Result**: The deque at the end will represent the required initial arrangement of the deck.
+
+
+### Plan
+1. Sort the input array in descending order.
+2. Initialize an empty deque.
+3. For each card in the sorted deck:
+ - If the deque is not empty, move the last card to the front.
+ - Insert the current card at the front.
+4. Convert the deque to an array and return the result.
+
+Let's implement this solution in PHP: **[950. Reveal Cards In Increasing Order](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/000947-most-stones-removed-with-same-row-or-column/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+The idea is to reverse-simulate the process:
+1. Start with the largest card since it will be revealed last.
+2. Simulate the steps of moving the bottom card to the top and adding the new card to the top.
+3. By sorting the deck in descending order, we construct the correct arrangement backward.
+
+
+### Example Walkthrough
+
+**Input**: `deck = [17, 13, 11, 2, 3, 5, 7]`
+
+1. **Sort the Deck**: `deck = [17, 13, 11, 7, 5, 3, 2]`.
+2. **Initialize Deque**: Start with an empty deque.
+3. **Simulate**:
+ - Add `2`: `deque = [2]`.
+ - Add `3` (move `2` to the back): `deque = [3, 2]`.
+ - Add `5` (move `2` to the back, then `3`): `deque = [5, 2, 3]`.
+ - Add `7` (move `3` to the back, then `2`, then `5`): `deque = [7, 5, 2, 3]`.
+ - Add `11`: Repeat steps, `deque = [11, 7, 5, 2, 3]`.
+ - Add `13`: Repeat steps, `deque = [13, 11, 7, 5, 2, 3]`.
+ - Add `17`: Repeat steps, `deque = [17, 13, 11, 7, 5, 2, 3]`.
+4. Convert deque to array: `[2, 13, 3, 11, 5, 17, 7]`.
+
+**Output**: `[2, 13, 3, 11, 5, 17, 7]`.
+
+
+### Time Complexity
+- **Sorting**: _**O(n log n)**_, where _**n**_ is the number of cards.
+- **Simulation**: _**O(n)**_, as each card is moved at most once.
+- **Overall**: _**O(n log n)**_.
+
+
+### Output for Example
+**Input**: `deck = [17, 13, 11, 2, 3, 5, 7]`
+**Output**: `[2, 13, 3, 11, 5, 17, 7]`.
+
+
+This solution effectively leverages sorting and deque operations to solve the problem in a time-efficient manner. By simulating the process in reverse, we achieve the desired order while maintaining clarity and simplicity.
+
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
diff --git a/algorithms/000951-flip-equivalent-binary-trees/README.md b/algorithms/000951-flip-equivalent-binary-trees/README.md
new file mode 100644
index 000000000..fdf4dac1b
--- /dev/null
+++ b/algorithms/000951-flip-equivalent-binary-trees/README.md
@@ -0,0 +1,120 @@
+951\. Flip Equivalent Binary Trees
+
+**Difficulty:** Medium
+
+**Topics:** `Tree`, `Depth-First Search`, `Binary Tree`
+
+For a binary tree **T**, we can define a **flip operation** as follows: choose any node, and swap the left and right child subtrees.
+
+A binary tree **X** is _flip equivalent_ to a binary tree **Y** if and only if we can make **X** equal to **Y** after some number of flip operations.
+
+Given the roots of two binary trees `root1` and `root2`, return _`true` if the two trees are flip equivalent or `false` otherwise_.
+
+**Example 1:**
+
+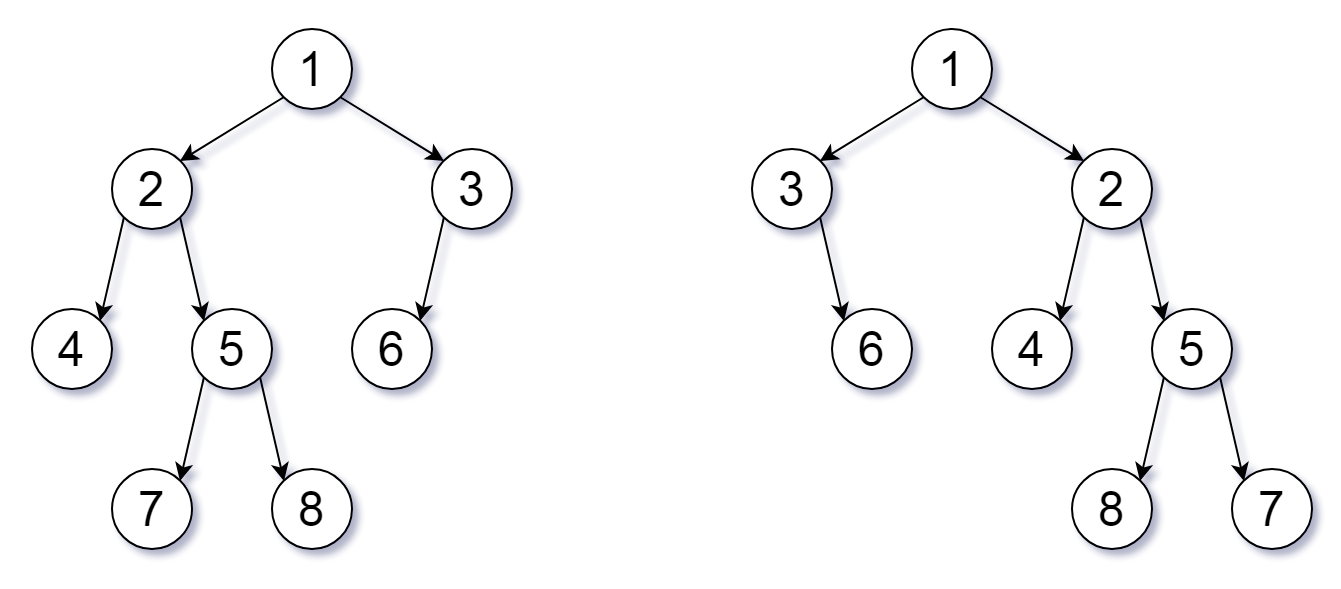
+
+- **Input:** root1 = [1,2,3,4,5,6,null,null,null,7,8], root2 = [1,3,2,null,6,4,5,null,null,null,null,8,7]
+- **Output:** true
+- **Explanation:** We flipped at nodes with values 1, 3, and 5.
+
+**Example 2:**
+
+- **Input:** root1 = [], root2 = []
+- **Output:** true
+
+
+**Example 3:**
+
+- **Input:** root1 = [], root2 = [1]
+- **Output:** false
+
+
+**Constraints:**
+
+- The number of nodes in each tree is in the range `[0, 100]`.
+- Each tree will have unique node values in the range `[0, 99]`.
+
+
+**Solution:**
+
+We can use a recursive depth-first search (DFS). The idea is that two trees are flip equivalent if their root values are the same, and the subtrees are either the same (without any flips) or they become the same after flipping the left and right children at some nodes.
+
+### Plan:
+1. **Base Cases:**
+ - If both `root1` and `root2` are `null`, they are trivially flip equivalent.
+ - If only one of them is `null`, they can't be equivalent.
+ - If the root values of `root1` and `root2` are different, they can't be equivalent.
+
+2. **Recursive Case:**
+ - Recursively check two possibilities:
+ 1. The left subtree of `root1` is flip equivalent to the left subtree of `root2`, and the right subtree of `root1` is flip equivalent to the right subtree of `root2` (i.e., no flip).
+ 2. The left subtree of `root1` is flip equivalent to the right subtree of `root2`, and the right subtree of `root1` is flip equivalent to the left subtree of `root2` (i.e., flip the children).
+
+Let's implement this solution in PHP: **[951. Flip Equivalent Binary Trees](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/000951-flip-equivalent-binary-trees/solution.php)**
+
+```php
+val = $val;
+ $this->left = $left;
+ $this->right = $right;
+ }
+}
+
+/**
+ * @param TreeNode $root1
+ * @param TreeNode $root2
+ * @return Boolean
+ */
+function flipEquiv($root1, $root2) {
+ ...
+ ...
+ ...
+ /**
+ * go to ./solution.php
+ */
+}
+
+// Example usage:
+$root1 = new TreeNode(1,
+ new TreeNode(2, new TreeNode(4), new TreeNode(5, new TreeNode(7), new TreeNode(8))),
+ new TreeNode(3, new TreeNode(6), null)
+);
+
+$root2 = new TreeNode(1,
+ new TreeNode(3, null, new TreeNode(6)),
+ new TreeNode(2, new TreeNode(4), new TreeNode(5, new TreeNode(8), new TreeNode(7)))
+);
+
+var_dump(flipEquiv($root1, $root2)); // Output: bool(true)
+?>
+```
+
+### Explanation:
+
+1. **TreeNode Class**: The `TreeNode` class represents a node in the binary tree, with a constructor to initialize the node's value, left child, and right child.
+
+2. **flipEquiv Function**:
+ - The base cases handle when both nodes are `null`, when one is `null`, or when the values do not match.
+ - The recursive case checks both possibilities (no flip vs. flip), ensuring the subtrees are flip equivalent under either condition.
+
+### Time Complexity:
+- The function checks every node in both trees, and each recursive call processes two subtrees. Therefore, the time complexity is O(N), where N is the number of nodes in the tree.
+
+### Space Complexity:
+- The space complexity is O(H), where H is the height of the tree, because of the recursive stack. In the worst case (for a skewed tree), this could be O(N).
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
diff --git a/algorithms/000951-flip-equivalent-binary-trees/solution.php b/algorithms/000951-flip-equivalent-binary-trees/solution.php
new file mode 100644
index 000000000..e188e36ba
--- /dev/null
+++ b/algorithms/000951-flip-equivalent-binary-trees/solution.php
@@ -0,0 +1,41 @@
+val = $val;
+ * $this->left = $left;
+ * $this->right = $right;
+ * }
+ * }
+ */
+
+class Solution {
+
+ /**
+ * @param TreeNode $root1
+ * @param TreeNode $root2
+ * @return Boolean
+ */
+ function flipEquiv($root1, $root2) {
+ // If both nodes are null, they are equivalent
+ if ($root1 === null && $root2 === null) {
+ return true;
+ }
+
+ // If one of the nodes is null or the values don't match, they are not equivalent
+ if ($root1 === null || $root2 === null || $root1->val !== $root2->val) {
+ return false;
+ }
+
+ // Check both possibilities:
+ // 1. No flip: left with left and right with right
+ // 2. Flip: left with right and right with left
+ return ($this->flipEquiv($root1->left, $root2->left) && $this->flipEquiv($root1->right, $root2->right)) ||
+ ($this->flipEquiv($root1->left, $root2->right) && $this->flipEquiv($root1->right, $root2->left));
+ }
+}
\ No newline at end of file
diff --git a/algorithms/000959-regions-cut-by-slashes/README.md b/algorithms/000959-regions-cut-by-slashes/README.md
new file mode 100644
index 000000000..e94778745
--- /dev/null
+++ b/algorithms/000959-regions-cut-by-slashes/README.md
@@ -0,0 +1,101 @@
+959\. Regions Cut By Slashes
+
+Medium
+
+**Topics:** `Array`, `Hash Table`, `Depth-First Search`, `Breadth-First Search`, `Union Find`, `Matrix`
+
+An `n x n` grid is composed of `1 x 1` squares where each `1 x 1` square consists of a `'/'`, `'\'`, or blank space `' '`. These characters divide the square into contiguous regions.
+
+Given the grid `grid` represented as a string array, return _the number of regions_.
+
+**Note** that backslash characters are escaped, so a `'\'` is represented as `'\\'`.
+
+**Example 1:**
+
+
+
+- **Input:** grid = [" /","/ "]
+- **Output:** 2
+
+**Example 2:**
+
+
+
+- **Input:** grid = [" /"," "]
+- **Output:** 1
+
+**Example 3:**
+
+
+
+- **Input:** grid = ["/\\","\\/"]
+- **Output:** 5
+- **Explanation:** Recall that because \ characters are escaped, "\\/" refers to \/, and "/\\" refers to /\.
+
+**Constraints:**
+
+- `n == grid.length == grid[i].length`
+- `1 <= n <= 30`
+- `grid[i][j]` is either `'/'`, `'\'`, or `' '`.
+
+
+
+**Solution:**
+
+
+We can represent each `1x1` square as 4 triangles, which allows us to apply a Union-Find (Disjoint Set Union, DSU) algorithm to count the distinct regions.
+
+### Step-by-Step Approach:
+
+1. **Grid Representation**:
+ - We treat each `1x1` square as 4 triangles:
+ - Top-left triangle
+ - Top-right triangle
+ - Bottom-left triangle
+ - Bottom-right triangle
+ - Each of these triangles is represented by an index in the Union-Find structure.
+
+2. **Mapping Characters**:
+ - If the square is `' '`, all 4 triangles within it are connected.
+ - If the square is `'/'`, the top-left triangle is connected to the bottom-right, and the top-right triangle is connected to the bottom-left.
+ - If the square is `'\'`, the top-left triangle is connected to the top-right, and the bottom-left triangle is connected to the bottom-right.
+
+3. **Connecting Adjacent Cells**:
+ - We connect the triangles of adjacent cells across the grid's boundaries. This ensures that regions spanning multiple cells are properly connected.
+
+4. **Counting the Regions**:
+ - We count the number of unique sets in the Union-Find structure, which corresponds to the number of regions.
+
+Let's implement this solution in PHP: **[959. Regions Cut By Slashes](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/000959-regions-cut-by-slashes/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+- The `UnionFind` class is used to manage the connected components (regions) in the grid.
+- For each cell in the grid, we apply the union operation based on the character (`'/'`, `'\'`, or `' '`).
+- Finally, the number of unique regions is determined by counting the distinct root parents in the Union-Find structure.
+
+This solution efficiently handles the problem within the given constraints.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
+
+
diff --git a/algorithms/000959-regions-cut-by-slashes/solution.php b/algorithms/000959-regions-cut-by-slashes/solution.php
new file mode 100644
index 000000000..b5f78d50b
--- /dev/null
+++ b/algorithms/000959-regions-cut-by-slashes/solution.php
@@ -0,0 +1,88 @@
+union($root, $root + 1);
+ $uf->union($root + 1, $root + 2);
+ $uf->union($root + 2, $root + 3);
+ } elseif ($char == '/') {
+ $uf->union($root, $root + 3);
+ $uf->union($root + 1, $root + 2);
+ } elseif ($char == '\\') {
+ $uf->union($root, $root + 1);
+ $uf->union($root + 2, $root + 3);
+ }
+
+ // Connect with the right cell
+ if ($j + 1 < $n) {
+ $uf->union($root + 1, 4 * ($i * $n + $j + 1) + 3);
+ }
+ // Connect with the bottom cell
+ if ($i + 1 < $n) {
+ $uf->union($root + 2, 4 * (($i + 1) * $n + $j));
+ }
+ }
+ }
+
+ $regions = 0;
+ for ($i = 0; $i < $n * $n * 4; $i++) {
+ if ($uf->find($i) == $i) {
+ $regions++;
+ }
+ }
+
+ return $regions;
+
+ }
+}
+
+class UnionFind {
+ private $parent;
+
+ /**
+ * @param $n
+ */
+ public function __construct($n) {
+ $this->parent = range(0, $n - 1);
+ }
+
+ /**
+ * @param $x
+ * @return mixed
+ */
+ public function find($x) {
+ if ($this->parent[$x] != $x) {
+ $this->parent[$x] = $this->find($this->parent[$x]);
+ }
+ return $this->parent[$x];
+ }
+
+ /**
+ * @param $x
+ * @param $y
+ * @return void
+ */
+ public function union($x, $y) {
+ $rootX = $this->find($x);
+ $rootY = $this->find($y);
+ if ($rootX != $rootY) {
+ $this->parent[$rootX] = $rootY;
+ }
+ }
+}
+
diff --git a/algorithms/000962-maximum-width-ramp/README.md b/algorithms/000962-maximum-width-ramp/README.md
new file mode 100644
index 000000000..0769509ca
--- /dev/null
+++ b/algorithms/000962-maximum-width-ramp/README.md
@@ -0,0 +1,96 @@
+962\. Maximum Width Ramp
+
+**Difficulty:** Medium
+
+**Topics:** `Array`, `Stack`, `Monotonic Stack`
+
+A **ramp** in an integer array `nums` is a pair `(i, j)` for which `i < j` and `nums[i] <= nums[j]`. The **width** of such a ramp is `j - i`.
+
+Given an integer array `nums`, return _the maximum width of a **ramp** in `nums`_. If there is no **ramp** in `nums`, return `0`.
+
+**Example 1:**
+
+- **Input:** nums = [6,0,8,2,1,5]
+- **Output:** 4
+- **Explanation:** The maximum width ramp is achieved at (i, j) = (1, 5): nums[1] = 0 and nums[5] = 5.
+
+**Example 2:**
+
+- **Input:** nums = [9,8,1,0,1,9,4,0,4,1]
+- **Output:** 7
+- **Explanation:** The maximum width ramp is achieved at (i, j) = (2, 9): nums[2] = 1 and nums[9] = 1.
+
+
+
+**Constraints:**
+
+- 2 <= nums.length <= 5 * 104
+- 0 <= nums[i] <= 5 * 104
+
+
+**Solution:**
+
+We can leverage the concept of a **monotonic stack**. Here's the solution and explanation:
+
+### Approach:
+1. **Monotonic Decreasing Stack**: We create a stack that keeps track of indices of elements in a way such that `nums[stack[i]]` is in a decreasing order. This allows us to later find pairs `(i, j)` where `nums[i] <= nums[j]`.
+2. **Traverse from the End**: After creating the stack, we traverse the array from the end (`j` from `n-1` to `0`) to try and find the farthest `i` for each `j` where `nums[i] <= nums[j]`.
+3. **Update the Maximum Width**: Whenever `nums[i] <= nums[j]` holds for the current top of the stack, calculate the ramp width `j - i` and update the maximum width if it's larger.
+
+Let's implement this solution in PHP: **[962. Maximum Width Ramp](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/000962-maximum-width-ramp/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+1. **Create a Decreasing Stack**:
+ - Iterate through the array and add indices to the stack.
+ - Only add an index if it corresponds to a value that is less than or equal to the value of the last index in the stack. This ensures that values in the stack are in a decreasing order.
+
+2. **Traverse from the End**:
+ - As we go backward through the array, for each `j`, pop indices `i` from the stack as long as `nums[i] <= nums[j]`.
+ - Calculate the width `j - i` and update `maxWidth`.
+
+3. **Why This Works**:
+ - By maintaining a decreasing stack of indices, we ensure that when we encounter a `j` with a larger value, it can give us a larger width `j - i` when `i` is popped from the stack.
+
+4. **Time Complexity**:
+ - Building the stack takes `O(n)` time since each index is pushed once.
+ - The traversal from the end and popping indices also takes `O(n)` since each index is popped at most once.
+ - Overall, the solution runs in `O(n)` time, which is efficient for input size up to `5 * 10^4`.
+
+### Output:
+- For `nums = [6, 0, 8, 2, 1, 5]`, the output is `4`, corresponding to the ramp `(1, 5)`.
+- For `nums = [9, 8, 1, 0, 1, 9, 4, 0, 4, 1]`, the output is `7`, corresponding to the ramp `(2, 9)`.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
diff --git a/algorithms/000962-maximum-width-ramp/solution.php b/algorithms/000962-maximum-width-ramp/solution.php
new file mode 100644
index 000000000..e80f4c1b4
--- /dev/null
+++ b/algorithms/000962-maximum-width-ramp/solution.php
@@ -0,0 +1,34 @@
+ $nums[$i]) {
+ array_push($stack, $i);
+ }
+ }
+
+ // Step 2: Traverse from the end of the array
+ for ($j = $n - 1; $j >= 0; $j--) {
+ // While the stack is not empty and nums[stackTop] <= nums[j]
+ while (!empty($stack) && $nums[$stack[count($stack) - 1]] <= $nums[$j]) {
+ // Calculate the ramp width and update maxWidth
+ $i = array_pop($stack);
+ $maxWidth = max($maxWidth, $j - $i);
+ }
+ }
+
+ return $maxWidth;
+ }
+}
\ No newline at end of file
diff --git a/algorithms/000974-subarray-sums-divisible-by-k/README.md b/algorithms/000974-subarray-sums-divisible-by-k/README.md
index 0b9e904a3..131783b50 100644
--- a/algorithms/000974-subarray-sums-divisible-by-k/README.md
+++ b/algorithms/000974-subarray-sums-divisible-by-k/README.md
@@ -1,6 +1,8 @@
974\. Subarray Sums Divisible by K
-Medium
+**Difficulty:** Medium
+
+**Topics:** `Array`, `Hash Table`, `Prefix Sum`
Given an integer array `nums` and an integer `k`, return _the number of non-empty **subarrays** that have a sum divisible by `k`_.
@@ -25,4 +27,91 @@ A **subarray** is a **contiguous** part of an array.
- 2 <= k <= 104
+**Solution:**
+
+The problem at hand asks us to find the number of non-empty subarrays of an integer array `nums` whose sum is divisible by a given integer `k`. A **subarray** is defined as a contiguous part of the array. The challenge requires an efficient solution given that the length of `nums` can be as large as _**3 \times 10^4**_.
+
+### Key Points
+- **Subarray**: A contiguous section of the array.
+- **Divisibility**: We need to count subarrays where the sum is divisible by `k`.
+- **Prefix Sum**: A technique that can help in reducing the problem to simpler subproblems.
+- **Modulo Operation**: The key insight is using modulo arithmetic to efficiently track sums divisible by `k`.
+
+### Approach
+1. **Prefix Sum Modulo**: The idea is to keep a running sum of elements and compute its modulo with `k`. If the sum of a subarray from index `i` to `j` is divisible by `k`, then the difference between the prefix sums at `j` and `i-1` (inclusive) must be divisible by `k`. Using modulo, this can be tracked.
+
+2. **Using a Hash Map (or Array)**: We maintain an array (`modGroups`) to count how many times each modulo value (from 0 to `k-1`) has occurred for prefix sums. This allows us to determine how many subarrays end at the current index and are divisible by `k`.
+
+3. **Modulo Adjustment**: Modulo values may be negative, so we adjust the modulo result to always be between 0 and `k-1`.
+
+### Plan
+1. Initialize a `prefixMod` to store the current sum's modulo `k`, and a `modGroups` array to count occurrences of different modulo values.
+2. Traverse through the array and update the `prefixMod`. For each number:
+ - Compute the new `prefixMod`.
+ - If `prefixMod` has been seen before, increment the result by the count of such occurrences (because each occurrence represents a valid subarray).
+ - Update the count of the current `prefixMod`.
+3. Return the result after traversing the entire array.
+
+Let's implement this solution in PHP: **[974. Subarray Sums Divisible by K](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/000974-subarray-sums-divisible-by-k/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+- We use the prefix sum technique to keep track of the sum of elements up to the current position.
+- By using modulo `k` at each step, we ensure that we only care about whether the sum of subarrays is divisible by `k`.
+- The `modGroups` array efficiently tracks how many times each modulo value has appeared, which allows us to count subarrays whose sum is divisible by `k`.
+
+### Example Walkthrough
+Consider the input: `nums = [4, 5, 0, -2, -3, 1]` and `k = 5`.
+
+- At the first element (4), the prefix sum modulo 5 is `4`. We haven't seen this before, so we store it.
+- At the second element (5), the prefix sum modulo 5 is `(4 + 5) % 5 = 4 % 5 = 0`. This is divisible by 5, so we have one subarray that ends here (`[5]`).
+- Continue with each element, updating the prefix sum modulo and counting valid subarrays where the sum is divisible by 5.
+
+### Time Complexity
+- **Time Complexity**: _**O(n)**_, where `n` is the length of the `nums` array. We iterate through the array once, performing constant-time operations for each element.
+- **Space Complexity**: _**O(k)**_ due to the `modGroups` array, which stores counts for each possible modulo value (from 0 to `k-1`).
+
+### Output for Example
+
+1. For `nums = [4, 5, 0, -2, -3, 1]` and `k = 5`, the function returns `7`, as there are 7 subarrays whose sum is divisible by 5.
+2. For `nums = [5]` and `k = 9`, the function returns `0`, as there are no subarrays whose sum is divisible by 9.
+
+The problem can be efficiently solved using prefix sums and modulo arithmetic. By tracking the frequency of each modulo value encountered during the traversal of the array, we can determine how many subarrays have sums divisible by `k`. This approach leverages the power of hashing to avoid the need for checking all subarrays explicitly, which would be computationally expensive for large arrays.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
+-
\ No newline at end of file
diff --git a/algorithms/000979-distribute-coins-in-binary-tree/README.md b/algorithms/000979-distribute-coins-in-binary-tree/README.md
index e24e727f0..3b0644e6e 100644
--- a/algorithms/000979-distribute-coins-in-binary-tree/README.md
+++ b/algorithms/000979-distribute-coins-in-binary-tree/README.md
@@ -1,6 +1,8 @@
979\. Distribute Coins in Binary Tree
-Medium
+**Difficulty:** Medium
+
+**Topics:** `Tree`, `Depth-First Search`, `Binary Tree`
You are given the `root` of a binary tree with `n` nodes where each `node` in the tree has `node.val` coins. There are `n` coins in total throughout the whole tree.
@@ -20,7 +22,7 @@ Return _the **minimum** number of moves required to make every node have **exact
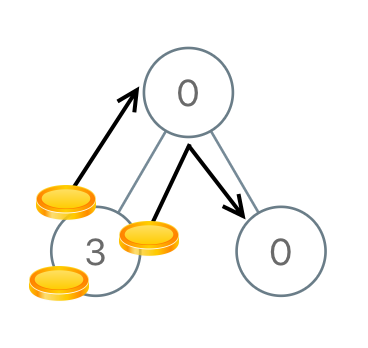
-- **Input:** oot = [0,3,0]
+- **Input:** root = [0,3,0]
- **Output:** 3
- **Explanation:** From the left child of the root, we move two coins to the root `[taking two moves]`. Then, we move one coin from the root of the tree to the right child.
@@ -30,3 +32,120 @@ Return _the **minimum** number of moves required to make every node have **exact
- `1 <= n <= 100`
- `0 <= Node.val <= n`
- The sum of all `Node.val` is `n`.
+
+
+**Solution:**
+
+The problem requires redistributing coins in a binary tree such that each node ends up with exactly one coin. You are given a binary tree with `n` nodes, where each node contains a certain number of coins. The goal is to determine the minimum number of moves needed to ensure every node has exactly one coin. A move consists of transferring a coin between adjacent nodes, either from a parent to a child or vice versa.
+
+### **Key Points**
+1. **Tree structure**: A binary tree is given with `n` nodes.
+2. **Node coins**: Each node may have a number of coins.
+3. **Moves**: A move consists of transferring one coin between adjacent nodes.
+4. **Objective**: Minimize the number of moves to ensure each node has exactly one coin.
+
+### **Approach**
+The key to solving this problem lies in using **Depth First Search (DFS)** to traverse the binary tree and balance the coins. We aim to track the number of excess coins at each node and ensure that excess coins are moved to nodes that have fewer than one coin. Here's how to approach the problem:
+
+1. **DFS Traversal**: Traverse the tree starting from the leaves up to the root. For each node, we calculate how many coins need to be moved.
+2. **Excess Coins**: For each node, if it has more coins than required, it will pass the excess coins to its parent or child nodes. If it has fewer coins, it will receive coins from its child or parent.
+3. **Total Moves**: The total number of moves is the sum of the absolute number of excess coins moved for each node.
+
+### **Plan**
+1. Use DFS to traverse the tree.
+2. For each node, calculate how many moves are required to balance its left and right subtrees.
+3. At each node, count how many coins need to be moved up to the parent or down to the children.
+4. Accumulate the total moves as we traverse the tree.
+5. Return the accumulated total moves.
+
+Let's implement this solution in PHP: **[979. Distribute Coins in Binary Tree](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/000979-distribute-coins-in-binary-tree/solution.php)**
+
+```php
+
+```
+
+1. **DFS Function**: It recursively calculates the excess coins for each node. For each subtree, it moves excess coins up or down to balance the tree.
+2. **Base Case**: If a node is `null`, it returns `0` because there are no coins to redistribute.
+3. **Recursive Case**: The function calculates moves for both left and right children and updates the total move count.
+
+### Explanation:
+
+1. **DFS Function**: This function will return the total number of excess coins at a node and will accumulate the number of moves. The excess coins at each node will be the difference between the node's coin count and 1 (the required number of coins for each node). If the node has excess coins (i.e., more than one), those coins will be passed up or down to other nodes, and the moves are counted.
+
+2. **Returning Excess Coins**: The excess coins for a node are the sum of the excess coins in its left and right subtrees, plus the number of coins in the current node minus one (since each node needs exactly one coin).
+
+3. **Result**: The total number of moves is the sum of absolute values of the excess coins at each node, because every excess coin needs to be moved.
+
+### **Example Walkthrough**
+
+**Example 1**:
+Tree: `[3,0,0]`
+
+```
+ 3
+ / \
+ 0 0
+```
+
+- Root node has 3 coins, and each child node has 0 coins.
+- We need to move 1 coin from the root to its left child, and 1 coin to its right child.
+- Thus, 2 moves are required.
+
+**Example 2**:
+Tree: `[0,3,0]`
+
+```
+ 0
+ / \
+ 3 0
+```
+
+- The left child has 3 coins, which is too many. It moves 2 coins to the root.
+- The root then moves 1 coin to the right child.
+- This requires 3 moves in total.
+
+### **Time Complexity**
+- The algorithm visits each node once during the DFS traversal, so the time complexity is **O(n)**, where `n` is the number of nodes in the tree.
+
+### **Output for Example**
+
+- **Example 1**:
+ Input: `[3,0,0]`
+ Output: `2`
+
+- **Example 2**:
+ Input: `[0,3,0]`
+ Output: `3`
+
+This problem can be efficiently solved using Depth First Search (DFS). By calculating the excess coins for each node and accumulating the required moves, we can determine the minimum number of moves to redistribute the coins such that every node has exactly one. The approach is optimal with a time complexity of O(n), making it suitable for trees with up to 100 nodes.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
\ No newline at end of file
diff --git a/algorithms/000983-minimum-cost-for-tickets/README.md b/algorithms/000983-minimum-cost-for-tickets/README.md
new file mode 100644
index 000000000..0495ce91b
--- /dev/null
+++ b/algorithms/000983-minimum-cost-for-tickets/README.md
@@ -0,0 +1,178 @@
+983\. Minimum Cost For Tickets
+
+**Difficulty:** Medium
+
+**Topics:** `Array`, `Dynamic Programming`
+
+You have planned some train traveling one year in advance. The days of the year in which you will travel are given as an integer array `days`. Each day is an integer from `1` to `365`.
+
+Train tickets are sold in **three different ways**:
+
+- a **1-day** pass is sold for `costs[0]` dollars,
+- a **7-day** pass is sold for `costs[1]` dollars, and
+- a **30-day** pass is sold for `costs[2]` dollars.
+
+The passes allow that many days of consecutive travel.
+
+- For example, if we get a **7-day** pass on day `2`, then we can travel for `7` days: `2`, `3`, `4`, `5`, `6`, `7`, and `8`.
+
+Return _the minimum number of dollars you need to travel every day in the given list of days_.
+
+**Example 1:**
+
+- **Input:** days = [1,4,6,7,8,20], costs = [2,7,15]
+- **Output:** 11
+- **Explanation:** For example, here is one way to buy passes that lets you travel your travel plan:
+ On day 1, you bought a 1-day pass for costs[0] = $2, which covered day 1.
+ On day 3, you bought a 7-day pass for costs[1] = $7, which covered days 3, 4, ..., 9.
+ On day 20, you bought a 1-day pass for costs[0] = $2, which covered day 20.
+ In total, you spent $11 and covered all the days of your travel.
+
+**Example 2:**
+
+- **Input:** days = [1,2,3,4,5,6,7,8,9,10,30,31], costs = [2,7,15]
+- **Output:** 17
+- **Explanation:** For example, here is one way to buy passes that lets you travel your travel plan:
+ On day 1, you bought a 30-day pass for costs[2] = $15 which covered days 1, 2, ..., 30.
+ On day 31, you bought a 1-day pass for costs[0] = $2 which covered day 31.
+ In total, you spent $17 and covered all the days of your travel.
+
+
+
+**Constraints:**
+
+- `1 <= days.length <= 365`
+- `1 <= days[i] <= 365`
+- `days` is in strictly increasing order.
+- `costs.length == 3`
+- `1 <= costs[i] <= 1000`
+
+
+**Solution:**
+
+The problem involves determining the minimum cost to travel on a set of specified days throughout the year. The problem offers three types of travel passes: 1-day, 7-day, and 30-day passes, each with specific costs. The goal is to find the cheapest way to cover all travel days using these passes. The task requires using dynamic programming to efficiently calculate the minimal cost.
+
+### Key Points
+
+- **Dynamic Programming (DP)**: We are using dynamic programming to keep track of the minimum cost for each day.
+- **Travel Days**: The travel days are provided in strictly increasing order, meaning we know exactly which days we need to travel.
+- **Three Types of Passes**: For each day `d` in the `days` array, calculate the minimum cost by considering the cost of buying a pass that covers the current day `d`:
+ - **1-day pass**: The cost would be the cost of the 1-day pass (`costs[0]`) added to the cost of the previous day (`dp[i-1]`).
+ - **7-day pass**: The cost would be the cost of the 7-day pass (`costs[1]`) added to the cost of the most recent day that is within 7 days of `d`.
+ - **30-day pass**: The cost would be the cost of the 30-day pass (`costs[2]`) added to the cost of the most recent day that is within 30 days of `d`.
+- **Base Case**: The minimum cost for a day when no travel is done is 0.
+
+### Approach
+
+1. **DP Array**: We'll use a DP array `dp[]` where `dp[i]` represents the minimum cost to cover all travel days up to day `i`.
+2. **Filling the DP Array**: For each day from 1 to 365:
+ - If the day is a travel day, we calculate the minimum cost by considering:
+ - The cost of using a 1-day pass.
+ - The cost of using a 7-day pass.
+ - The cost of using a 30-day pass.
+ - If the day is not a travel day, the cost for that day will be the same as the previous day (`dp[i] = dp[i-1]`).
+3. **Final Answer**: After filling the DP array, the minimum cost will be stored in `dp[365]`, which covers all possible travel days.
+
+### Plan
+
+1. Initialize an array `dp[]` of size 366 (one extra to handle up to day 365).
+2. Set `dp[0]` to 0, as there is no cost for day 0.
+3. Create a set `travelDays` to quickly check if a particular day is a travel day.
+4. Iterate through each day from 1 to 365:
+ - If it is a travel day, compute the minimum cost by considering each type of pass.
+ - If not, carry over the previous day's cost.
+5. Return the value at `dp[365]`.
+
+Let's implement this solution in PHP: **[983. Minimum Cost For Tickets](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/000983-minimum-cost-for-tickets/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+- The algorithm iterates over each day of the year (365 days).
+- For each travel day, it computes the cost by considering whether it is cheaper to:
+ - Buy a 1-day pass (adds the cost of the 1-day pass to the previous day's cost).
+ - Buy a 7-day pass (adds the cost of the 7-day pass and considers the cost of traveling on the past 7 days).
+ - Buy a 30-day pass (adds the cost of the 30-day pass and considers the cost of traveling over the past 30 days).
+- If it is not a travel day, the cost remains the same as the previous day.
+
+### Example Walkthrough
+
+#### Example 1:
+**Input:**
+```php
+$days = [1, 4, 6, 7, 8, 20];
+$costs = [2, 7, 15];
+```
+- **Day 1**: Buy a 1-day pass for $2.
+- **Day 4**: Buy a 7-day pass for $7 (cover days 4 to 9).
+- **Day 20**: Buy another 1-day pass for $2.
+
+Total cost = $2 + $7 + $2 = **$11**.
+
+#### Example 2:
+**Input:**
+```php
+$days = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 30, 31];
+$costs = [2, 7, 15];
+```
+- **Day 1**: Buy a 30-day pass for $15 (cover days 1 to 30).
+- **Day 31**: Buy a 1-day pass for $2.
+
+Total cost = $15 + $2 = **$17**.
+
+### Time Complexity
+
+The time complexity of the solution is **O(365)**, as we are iterating through all days of the year, and for each day, we perform constant time operations (checking travel days and updating the DP array). Thus, the solution runs in linear time relative to the number of days.
+
+### Output for Example
+
+#### Example 1:
+```php
+$days = [1, 4, 6, 7, 8, 20];
+$costs = [2, 7, 15];
+echo mincostTickets($days, $costs); // Output: 11
+```
+
+#### Example 2:
+```php
+$days = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 30, 31];
+$costs = [2, 7, 15];
+echo mincostTickets($days, $costs); // Output: 17
+```
+
+The solution efficiently calculates the minimum cost of covering the travel days using dynamic programming. By iterating over the days and considering all possible passes (1-day, 7-day, 30-day), the algorithm finds the optimal strategy for purchasing the passes. The time complexity is linear in terms of the number of days, making it suitable for the problem constraints.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
\ No newline at end of file
diff --git a/algorithms/000983-minimum-cost-for-tickets/solution.php b/algorithms/000983-minimum-cost-for-tickets/solution.php
new file mode 100644
index 000000000..2426fc203
--- /dev/null
+++ b/algorithms/000983-minimum-cost-for-tickets/solution.php
@@ -0,0 +1,38 @@
+[1, 8500].
-- `0 <= Node.val <= 25`
\ No newline at end of file
+- `0 <= Node.val <= 25`
+
+
+**Solution:**
+
+This problem is based on finding the lexicographically smallest string that starts at a leaf node and ends at the root in a binary tree. Each node contains a value between 0 and 25, representing the letters 'a' to 'z'. You are required to return the smallest string in lexicographical order formed by the path from any leaf node to the root node.
+
+### **Key Points**
+
+- **Leaf Node**: A node with no children.
+- **Lexicographical Order**: The order in which strings are arranged in a dictionary. Shorter strings come first, and among strings of the same length, the one with the smallest first character comes first.
+- **Depth-First Search (DFS)**: A traversal algorithm to explore all nodes in the tree, which can help solve this problem by checking all leaf nodes.
+- **Backtracking**: Reverting the changes made during the traversal to maintain the correct path.
+
+
+### **Approach**
+
+To solve this problem, we use **Depth-First Search (DFS)** with a backtracking approach:
+
+1. **DFS Traversal**: We start the traversal from the root and go down to all the leaf nodes.
+2. **Path Construction**: As we traverse down the tree, we build a string by appending characters corresponding to the node values.
+3. **String Comparison**: When we reach a leaf node, we compare the string formed by the path with the current lexicographically smallest string and update the result if necessary.
+4. **Backtracking**: After visiting a node, we remove the last character of the current path to backtrack and explore other paths.
+
+
+### **Plan**
+
+1. Start from the root node and use DFS to visit all the nodes.
+2. As we visit each node, append the corresponding character to the current path.
+3. When we reach a leaf node, check if the current path forms a lexicographically smaller string than the previously found smallest string.
+4. If so, update the result.
+5. Backtrack by removing the last character when returning from a node.
+6. Once all leaf nodes are visited, return the lexicographically smallest string.
+
+Let's implement this solution in PHP: **[988. Smallest String Starting From Leaf](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/000988-smallest-string-starting-from-leaf/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+1. **DFS Traversal**: We recursively visit the left and right children of the tree.
+2. **String Building**: For each node, we convert the node's value to a character (`'a'` + `node.val`), and append it to the current path.
+3. **Backtracking**: When backtracking, we undo the change to the path (i.e., remove the last character added).
+4. **Leaf Node Check**: If we are at a leaf (no left or right children), we reverse the string (since we need to form the string starting from the leaf to the root) and compare it with the current smallest string found.
+
+
+### **Example Walkthrough**
+
+#### Example 1:
+Tree structure:
+```
+ 0
+ / \
+ 1 2
+ / \ / \
+ 3 4 3 4
+```
+- Paths: `dba` (leaf 3 β root), `eba`, `dca`, `eca`.
+- Lexicographically smallest: `"dba"`.
+
+#### Example 2:
+Tree structure:
+```
+ 25
+ / \
+ 1 3
+ / \ / \
+ 1 3 0 2
+```
+- Paths: `adz`, `abz`, `acz`, `adz`.
+- Lexicographically smallest: `"adz"`.
+
+
+### **Time Complexity**
+- **Traversal**: Each node is visited once β **O(n)**.
+- **String Operations**: At each leaf, we reverse a string (O(k), where k is the path length). Total β **O(n)**.
+- **Overall**: **O(n)**.
+
+### **Space Complexity**
+- Path storage and recursion stack β **O(h)**, where `h` is the height of the tree.
+
+### **Output for Example**
+
+1. For `root = [0,1,2,3,4,3,4]`, the output is `"dba"`.
+2. For `root = [25,1,3,1,3,0,2]`, the output is `"adz"`.
+3. For `root = [2,2,1,null,1,0,null,0]`, the output is `"abc"`.
+
+
+This approach uses **Depth-First Search (DFS)** with **backtracking** to explore all paths from leaf nodes to the root while maintaining the lexicographically smallest string. The solution efficiently handles the problem within the constraints and ensures that we find the correct smallest string for each given tree structure.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
diff --git a/algorithms/000995-minimum-number-of-k-consecutive-bit-flips/README.md b/algorithms/000995-minimum-number-of-k-consecutive-bit-flips/README.md
index 47345488e..65385d4c4 100644
--- a/algorithms/000995-minimum-number-of-k-consecutive-bit-flips/README.md
+++ b/algorithms/000995-minimum-number-of-k-consecutive-bit-flips/README.md
@@ -1,6 +1,8 @@
995\. Minimum Number of K Consecutive Bit Flips
-Hard
+**Difficulty:** Hard
+
+**Topics:** `Array`, `Bit Manipulation`, `Queue`, `Sliding Window`, `Prefix Sum`
You are given a binary array `nums` and an integer `k`.
@@ -34,4 +36,112 @@ A **subarray** is a **contiguous** part of an array.
**Constraints:**
- 1 <= nums.length <= 105
-- 1 <= k <= nums.length
\ No newline at end of file
+- 1 <= k <= nums.length
+
+
+**Solution:**
+
+We are given a binary array `nums` and an integer `k`. Our goal is to transform all `0`s in the array into `1`s using a minimum number of **k-bit flips**. A k-bit flip involves choosing a contiguous subarray of length `k`, and flipping every bit in that subarray: changing `0` to `1` and `1` to `0`. The task is to find the minimum number of k-bit flips required to make the entire array consist of `1`s. If it's not possible, we should return `-1`.
+
+### **Key Points:**
+- **k-bit flip**: A flip operation on a contiguous subarray of length `k` that reverses the bits (i.e., 0 becomes 1, and 1 becomes 0).
+- The aim is to find the minimum number of flips that convert all `0`s to `1`s.
+- If it's not possible to flip enough bits due to constraints (such as exceeding the array length), the function should return `-1`.
+
+### **Approach:**
+1. **Sliding Window Technique**:
+ - We need to traverse the array and flip the bits when required.
+ - Instead of directly flipping bits, we use a **flip tracking mechanism** to efficiently manage flips over the array.
+ - We utilize a window of size `k` to track valid flips, maintaining a count of flips that affect the current position.
+
+2. **Flip Tracking**:
+ - We use an array `flipped` to keep track of whether a position has been flipped.
+ - A variable `validFlipsFromPastWindow` is used to track the cumulative effect of flips in the current window.
+ - For each position in the array:
+ - If the current bit is incorrect (i.e., it doesn't match the desired `1`), we flip the window starting at that position.
+ - We maintain the effect of flips by updating the flip count and adjusting the valid flips from past windows.
+ - If a flip goes beyond the length of the array, return `-1`.
+
+### **Plan**:
+1. Initialize an array `flipped` to track which positions have been flipped.
+2. Use a variable `validFlipsFromPastWindow` to track the number of flips that affect the current position.
+3. Traverse the array and for each element:
+ - Determine whether a flip is required.
+ - If necessary, flip a subarray of size `k` and update the tracking variables.
+4. At the end of the traversal, return the total number of flips made.
+5. If a flip is not possible (due to bounds constraints), return `-1`.
+
+Let's implement this solution in PHP: **[995. Minimum Number of K Consecutive Bit Flips](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/000995-minimum-number-of-k-consecutive-bit-flips/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+- We traverse the array while keeping track of the cumulative effect of flips in a sliding window of size `k`.
+- Each time we encounter a `0` (or a flipped `0`), we decide whether a flip is required by checking if the flip count up to that point is even or odd.
+- If a flip goes beyond the array length, we return `-1` as it's impossible to flip.
+
+### **Example Walkthrough**:
+Let's walk through **Example 1**:
+
+**Input**: `nums = [0, 1, 0], k = 1`
+
+- Start with `flipped = [false, false, false]` and `validFlipsFromPastWindow = 0`.
+- For `i = 0`:
+ - The bit is `0` and no flip has occurred yet, so we flip the subarray starting at index 0.
+ - The array becomes `[1, 1, 0]`, `flipped = [true, false, false]`, and `flipCount = 1`.
+ - Now, the bit at index 0 is correct.
+- For `i = 2`:
+ - The bit is `0` (after the first flip) and requires another flip.
+ - The array becomes `[1, 1, 1]`, `flipped = [true, false, true]`, and `flipCount = 2`.
+ - Now, the bit at index 2 is correct.
+- The final result is `flipCount = 2`.
+
+### **Time Complexity**:
+- **Time Complexity**: `O(n)` where `n` is the length of the `nums` array. We perform a single pass over the array and use constant-time operations for each element.
+- **Space Complexity**: `O(n)` for the `flipped` array used to track the flip states.
+
+### **Output for Example**:
+- For the input `nums = [0,1,0]` and `k = 1`, the output is `2`.
+
+This approach efficiently solves the problem by using a sliding window technique combined with flip tracking. By ensuring that we only flip the necessary bits and adjusting the flips dynamically, we can minimize the number of flips required. If the problem constraints are such that it's impossible to achieve the desired result, we handle that with an early exit. This method ensures optimal time and space complexity for large inputs.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
diff --git a/algorithms/001002-find-common-characters/README.md b/algorithms/001002-find-common-characters/README.md
index 730adff2d..b7a8adae6 100644
--- a/algorithms/001002-find-common-characters/README.md
+++ b/algorithms/001002-find-common-characters/README.md
@@ -1,6 +1,8 @@
1002\. Find Common Characters
-Easy
+**Difficulty:** Easy
+
+**Topics:** `Array`, `Hash Table`, `String`
Given a string array `words`, return _an array of all characters that show up in all strings within the `words` (including duplicates). You may return the answer in **any order**_.
@@ -21,3 +23,132 @@ Given a string array `words`, return _an array of all characters that show up in
- words[i]
consists of lowercase English letters.
+**Solution:**
+
+The problem asks us to find the common characters that appear in all the strings of a given array. This includes duplicates, meaning if a character appears twice in all strings, it should appear twice in the output. The result can be in any order.
+
+### **Key Points**
+
+1. **Input:** An array of strings `words` with lowercase letters.
+2. **Output:** An array of characters that are common across all strings.
+3. **Constraints:**
+ - Array length and string length are small enough to allow a brute-force approach.
+ - Case sensitivity and duplicates need to be handled.
+
+### **Approach**
+
+The main idea is to:
+1. Maintain a global count of the minimum frequency of each character across all strings.
+2. For each word, count the frequency of characters and update the global count.
+3. Use this global count to construct the final output.
+
+### **Plan**
+
+1. **Initialize a global frequency array:** Create an array of size 26 (for each letter) and set all values to `PHP_INT_MAX` to simulate infinity.
+2. **Iterate through each word:**
+ - Count the frequency of characters in the current word.
+ - Update the global frequency array by taking the minimum count for each letter.
+3. **Build the result:** Iterate through the global frequency array and append characters to the result based on their minimum frequency.
+4. **Return the result array.**
+
+Let's implement this solution in PHP: **[1002. Find Common Characters](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/001002-find-common-characters/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+#### **Step-by-Step Breakdown**
+
+1. **Global Frequency Array:**
+ - Represented as `letterCount` of size 26 (for 'a' to 'z').
+ - Initialized with `PHP_INT_MAX` since we are looking for the minimum frequency.
+
+2. **Iterate Over Words:**
+ - For each word, calculate the frequency of characters in `wordLetterCount`.
+ - Update the global `letterCount` by comparing it with `wordLetterCount` and taking the minimum.
+
+3. **Construct Result:**
+ - For each letter, if its count is greater than 0 in the global array, add it that many times to the result.
+
+4. **Return the Result:**
+ - The result is the final list of common characters.
+
+### **Example Walkthrough**
+
+#### Example 1:
+
+**Input:** `words = ["bella", "label", "roller"]`
+
+1. **Initialization:**
+ - `letterCount` = `[PHP_INT_MAX, PHP_INT_MAX, ..., PHP_INT_MAX]`.
+
+2. **Process Each Word:**
+ - Word "bella":
+ - `wordLetterCount` = `[0, 1, 0, ..., 1, 2]` (frequency of 'b', 'e', 'l', etc.)
+ - Update `letterCount`: `[0, 1, 0, ..., 1, 2]`.
+
+ - Word "label":
+ - `wordLetterCount` = `[1, 1, 0, ..., 2, 1]`.
+ - Update `letterCount`: `[0, 1, 0, ..., 1, 1]`.
+
+ - Word "roller":
+ - `wordLetterCount` = `[0, 0, 0, ..., 1, 2]`.
+ - Update `letterCount`: `[0, 0, 0, ..., 1, 1]`.
+
+3. **Build Result:**
+ - Add 'e', 'l', 'l' based on `letterCount`.
+
+**Output:** `["e", "l", "l"]`.
+
+### **Time Complexity**
+
+1. **Frequency Count per Word:** _**O(N x L)**_, where _**N**_ is the number of words and _**L**_ is the average length of a word.
+2. **Result Construction:** _**O(26)**_.
+
+**Overall Complexity:** _**O(N x L)**_.
+
+### **Output for Example**
+
+#### Example 1:
+**Input:** `["bella", "label", "roller"]`
+**Output:** `["e", "l", "l"]`
+
+#### Example 2:
+**Input:** `["cool", "lock", "cook"]`
+**Output:** `["c", "o"]`
+
+
+This solution efficiently handles the problem within the constraints. It uses the concept of frequency arrays and minimizes space by using constant storage for the alphabet. The logic is simple, and the result respects duplicates.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
+
diff --git a/algorithms/001014-best-sightseeing-pair/README.md b/algorithms/001014-best-sightseeing-pair/README.md
new file mode 100644
index 000000000..4d6fab95d
--- /dev/null
+++ b/algorithms/001014-best-sightseeing-pair/README.md
@@ -0,0 +1,97 @@
+1014\. Best Sightseeing Pair
+
+**Difficulty:** Medium
+
+**Topics:** `Array`, `Dynamic Programming`
+
+You are given an integer array `values` where `values[i]` represents the value of the ith
sightseeing spot. Two sightseeing spots `i` and `j` have a distance `j - i` between them.
+
+The score of a pair (`i < j`) of sightseeing spots is `values[i] + values[j] + i - j`: the sum of the values of the sightseeing spots, minus the distance between them.
+
+Return _the maximum score of a pair of sightseeing spots_.
+
+**Example 1:**
+
+- **Input:** values = [8,1,5,2,6]
+- **Output:** 11
+- **Explanation:** i = 0, j = 2, values[i] + values[j] + i - j = 8 + 5 + 0 - 2 = 11
+
+**Example 2:**
+
+- **Input:** values = [1,2]
+- **Output:** 2
+
+
+
+**Constraints:**
+
+- 2 <= values.length <= 5 * 104
+- `1 <= values[i] <= 1000`
+
+
+**Hint:**
+1. Can you tell the best sightseeing spot in one pass (ie. as you iterate over the input?) What should we store or keep track of as we iterate to do this?
+
+
+
+**Solution:**
+
+We can use a single-pass approach with a linear time complexity _**O(n)**_. The idea is to keep track of the best possible `values[i] + i` as we iterate through the array. This allows us to maximize the score `values[i] + values[j] + i - j` for every valid pair `(i, j)`.
+
+Let's implement this solution in PHP: **[1014. Best Sightseeing Pair](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/001014-best-sightseeing-pair/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+1. **Initialization:**
+ - `maxI` is initialized to `values[0]` because we start evaluating pairs from index `1`.
+ - `maxScore` is initialized to `0` to track the maximum score.
+
+2. **Iterate Over the Array:**
+ - For each index `j` starting from `1`, calculate the score for the pair `(i, j)` using the formula:
+ _**Score = maxI + values[j] - j**_
+ - Update `maxScore` with the maximum value obtained.
+
+3. **Update maxI:**
+ - Update `maxI` to track the maximum possible value of `values[i] + i` for the next iterations.
+
+4. **Return the Maximum Score:**
+ - After iterating through the array, `maxScore` will contain the maximum score for any pair.
+
+### Complexity:
+- **Time Complexity:** _**O(n)**_ because we loop through the array once.
+- **Space Complexity:** _**O(1)**_ as we use a constant amount of space.
+
+This solution efficiently computes the maximum score while adhering to the constraints and is optimized for large inputs.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
diff --git a/algorithms/001014-best-sightseeing-pair/solution.php b/algorithms/001014-best-sightseeing-pair/solution.php
new file mode 100644
index 000000000..dd39142aa
--- /dev/null
+++ b/algorithms/001014-best-sightseeing-pair/solution.php
@@ -0,0 +1,23 @@
+
+```
+
+### Explanation:
+
+- **reverseInorder function**: A helper function performs the reverse inorder traversal. The function will process each node in descending order and accumulate the sum.
+- As we visit each node, we add the `prefix` (sum of all previously visited nodes) to the current nodeβs value and update the `prefix`.
+
+### Example Walkthrough
+
+Consider the input BST:
+```
+ 4
+ / \
+ 1 6
+ / \ / \
+ 0 2 5 7
+ \ \
+ 3 8
+```
+
+- Start at the rightmost node (8). The accumulated sum is 0, so the new value of 8 becomes `8 + 0 = 8`. Update `prefix = 8`.
+- Move to node 7. The accumulated sum is 8, so the new value of 7 becomes `7 + 8 = 15`. Update `prefix = 15`.
+- Continue this process for all nodes in the tree, updating their values and the `prefix` as you move down the tree.
+
+The final Greater Sum Tree looks like this:
+```
+ 30
+ / \
+ 36 21
+ / \ / \
+ 36 35 26 15
+ \ \
+ 33 8
+```
+
+### Time Complexity
+
+- **Time Complexity:** The time complexity is **O(N)**, where **N** is the number of nodes in the tree. This is because we visit each node exactly once during the traversal.
+
+- **Space Complexity:** The space complexity is **O(H)**, where **H** is the height of the tree, due to the recursive stack used during the traversal. In the worst case (a skewed tree), **H = N**, so the space complexity could also be **O(N)**.
+
+### Output for Example
+
+For the input `root = [4,1,6,0,2,5,7,null,null,null,3,null,null,null,8]`, the output will be:
+```
+[30,36,21,36,35,26,15,null,null,null,33,null,null,null,8]
+```
+
+For the input `root = [0,null,1]`, the output will be:
+```
+[1,null,1]
+```
+
+The solution involves a reverse inorder traversal of the BST to accumulate the sum of all greater nodes and modify the current nodeβs value accordingly. This approach ensures an efficient and clean solution, with time complexity proportional to the number of nodes in the tree. The solution is simple, effective, and leverages the properties of a BST to perform the required transformation.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
diff --git a/algorithms/001051-height-checker/README.md b/algorithms/001051-height-checker/README.md
index d9cb2521a..6b8b84e9f 100644
--- a/algorithms/001051-height-checker/README.md
+++ b/algorithms/001051-height-checker/README.md
@@ -1,12 +1,14 @@
1051\. Height Checker
-Easy
+**Difficulty:** Easy
-A school is trying to take an annual photo of all the students. The students are asked to stand in a single file line in non-decreasing order by height. Let this ordering be represented by the integer array expected where expected[i] is the expected height of the ith student in line.
+**Topics:** `Array`, `Sorting`, `Counting Sort`
-You are given an integer array heights representing the current order that the students are standing in. Each heights[i] is the height of the ith student in line (0-indexed).
+A school is trying to take an annual photo of all the students. The students are asked to stand in a single file line in **non-decreasing order** by height. Let this ordering be represented by the integer array `expected` where `expected[i]` is the expected height of the ith
student in line.
-Return the number of indices where heights[i] != expected[i].
+You are given an integer array `heights` representing the **current order** that the students are standing in. Each `heights[i]` is the height of the ith
student in line (**0-indexed**).
+
+Return _the **number of indices** where `heights[i] != expected[i]`_.
**Example 1:**
@@ -41,4 +43,130 @@ Return the number of indices where heights[i] != expected[i].
- 1 <= heights[i] <= 100
+**Hint:**
+1. Build the correct order of heights by sorting another array, then compare the two arrays.
+
+
+**Solution:**
+
+The problem at hand is to calculate the number of indices where the heights of students in a photo do not match the expected order. The students are supposed to be arranged in non-decreasing order by height. We are given the current arrangement and need to compare it with the expected arrangement to count how many students are out of place.
+
+### Key Points:
+- We need to return the number of indices where the current height does not match the expected height.
+- The input is an array representing the current height order.
+- The expected order is simply the sorted version of the input array.
+
+### Approach:
+1. **Sorting the Heights:** First, we need to generate the expected order, which is just the sorted version of the given `heights` array.
+2. **Comparing Arrays:** Once we have the sorted array, we compare each element of the sorted array with the original array. If they are not equal at any index, we increment the counter.
+3. **Return the Result:** The result will be the number of such indices where the heights are not in their expected position.
+
+### Plan:
+1. Create a copy of the `heights` array and sort it to get the `expected` order.
+2. Iterate through both arrays and compare their elements at each index.
+3. Count how many positions do not match between the original array and the sorted array.
+4. Return the count.
+
+Let's implement this solution in PHP: **[1051. Height Checker](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/001051-height-checker/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+The provided code uses a counting sort technique to avoid sorting the array completely. Instead of sorting, it counts the frequency of each height and then checks if the current height matches the expected height by incrementing through the counts.
+
+1. **Counting Heights:** The function first initializes an array `count` of size 101 (since heights range from 1 to 100). It then counts the occurrences of each height in the `heights` array.
+2. **Generating the Expected Heights:** For each height in the `heights` array, it checks the lowest available height using the `currentHeight` pointer. If the current height in the `heights` array doesn't match the expected one, it increments the `ans` counter.
+3. **Decreasing the Count:** After comparing, it decreases the count of the current height.
+4. **Return the Count:** Finally, it returns the number of mismatched positions.
+
+### Example Walkthrough:
+
+**Example 1:**
+
+- **Input:** `heights = [1,1,4,2,1,3]`
+- **Sorted Expected Order:** `expected = [1,1,1,2,3,4]`
+- **Comparison:**
+ - Index 0: 1 == 1 (no change)
+ - Index 1: 1 == 1 (no change)
+ - Index 2: 4 != 1 (mismatch)
+ - Index 3: 2 != 2 (no change)
+ - Index 4: 1 != 3 (mismatch)
+ - Index 5: 3 != 4 (mismatch)
+- **Output:** 3 (3 mismatches at indices 2, 4, and 5)
+
+**Example 2:**
+
+- **Input:** `heights = [5,1,2,3,4]`
+- **Sorted Expected Order:** `expected = [1,2,3,4,5]`
+- **Comparison:**
+ - All positions mismatch.
+- **Output:** 5 (5 mismatches)
+
+**Example 3:**
+
+- **Input:** `heights = [1,2,3,4,5]`
+- **Sorted Expected Order:** `expected = [1,2,3,4,5]`
+- **Comparison:**
+ - All positions match.
+- **Output:** 0 (no mismatches)
+
+### Time Complexity:
+- **Sorting:** The sorting of the array takes O(n log n) time.
+- **Comparing Arrays:** The comparison takes O(n) time since we just iterate through both arrays once.
+- **Overall Complexity:** The time complexity is dominated by the sorting step, so the overall time complexity is **O(n log n)**, where `n` is the length of the `heights` array.
+
+### Output for Example:
+
+1. **Example 1:**
+ - Input: `heights = [1,1,4,2,1,3]`
+ - Output: `3`
+
+2. **Example 2:**
+ - Input: `heights = [5,1,2,3,4]`
+ - Output: `5`
+
+3. **Example 3:**
+ - Input: `heights = [1,2,3,4,5]`
+ - Output: `0`
+
+The `heightChecker` function efficiently calculates the number of indices where the heights of students are out of place compared to the expected order. The approach of using a counting sort allows for a space-efficient solution, and the time complexity is dominated by the sorting step.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
+
+
diff --git a/algorithms/001052-grumpy-bookstore-owner/README.md b/algorithms/001052-grumpy-bookstore-owner/README.md
index e80574147..5344fc157 100644
--- a/algorithms/001052-grumpy-bookstore-owner/README.md
+++ b/algorithms/001052-grumpy-bookstore-owner/README.md
@@ -1,16 +1,18 @@
1052\. Grumpy Bookstore Owner
-Medium
+**Difficulty:** Medium
+
+**Topics:** `Array`, `Sliding Window`
There is a bookstore owner that has a store open for `n` minutes. Every minute, some number of customers enter the store. You are given an integer array `customers` of length n where `customers[i]` is the number of the customer that enters the store at the start of the ith
minute and all those customers leave after the end of that minute.
On some minutes, the bookstore owner is grumpy. You are given a binary array grumpy where `grumpy[i]` is `1` if the bookstore owner is grumpy during the ith
minute, and is `0` otherwise.
-When the bookstore owner is grumpy, the customers of that minute are not satisfied, otherwise, they are satisfied.
+When the bookstore owner is grumpy, the customers of that minute are not **satisfied**, otherwise, they are satisfied.
-The bookstore owner knows a secret technique to keep themselves not grumpy for `minutes` consecutive minutes, but can only use it once.
+The bookstore owner knows a secret technique to keep themselves **not grumpy** for `minutes` consecutive minutes, but can only use it **once**.
-Return _the maximum number of customers that can be satisfied throughout the day_.
+Return _the **maximum** number of customers that can be satisfied throughout the day_.
**Example 1:**
@@ -29,4 +31,139 @@ Return _the maximum number of customers that can be satisfied throughout the day
- n == customers.length == grumpy.length
- 1 <= minutes <= n <= 2 * 104
- 0 <= customers[i] <= 1000
-- `grumpy[i]` is either `0` or `1`.
\ No newline at end of file
+- `grumpy[i]` is either `0` or `1`.
+
+
+**Hint:**
+1. Say the store owner uses their power in minute `1` to `X` and we have some answer `A`. If they instead use their power from minute `2` to `X+1`, we only have to use data from minutes `1`, `2`, `X` and `X+1` to update our answer `A`.
+
+
+**Solution:**
+
+The problem asks us to calculate the maximum number of customers that can be satisfied at a bookstore, given that the bookstore owner can use a special technique to remain non-grumpy for `minutes` consecutive minutes. The goal is to find the maximum number of customers that can be satisfied during the store's opening hours. The satisfaction of customers depends on whether the bookstore owner is grumpy during each minute. If the owner is grumpy, customers entering that minute are not satisfied, but if they are not grumpy, the customers are satisfied.
+
+### **Key Points**
+- The problem involves two arrays: `customers` and `grumpy`.
+ - `customers[i]` represents the number of customers entering at minute `i`.
+ - `grumpy[i]` indicates whether the owner is grumpy at minute `i` (1 if grumpy, 0 if not).
+- The owner has a special technique to remain non-grumpy for a specified number of consecutive minutes, which can be applied only once.
+- The task is to maximize the total number of customers that can be satisfied by choosing a window of `minutes` consecutive minutes during which the owner will remain non-grumpy.
+
+### **Approach**
+To solve this problem, we can use the sliding window technique:
+1. **Initial Satisfaction Calculation**:
+ - Start by calculating the total satisfaction (`satisfied`) when the owner is not grumpy (i.e., when `grumpy[i] == 0`). This is done by iterating through the `grumpy` array and summing the number of customers for each minute where the owner is not grumpy.
+2. **Sliding Window of Grumpy Minutes**:
+ - We want to find the optimal window of `minutes` consecutive minutes where the owner remains non-grumpy to maximize the number of additional satisfied customers.
+ - The sliding window will help us compute this efficiently by keeping track of how many unsatisfied customers could be made happy by using the special technique during the window. As we move the window forward, we subtract the number of customers from the minute that is left behind and add the number of customers from the new minute.
+3. **Calculate Maximum Satisfaction**:
+ - We compute the total satisfied customers as the sum of the initial satisfaction and the best possible satisfaction gained from using the technique.
+
+### **Plan**
+1. Initialize a variable `satisfied` to track the initial satisfied customers.
+2. Use a sliding window of size `minutes` to find the maximum possible additional customers that can be satisfied.
+3. For each minute, if the owner is grumpy, add the number of customers to a temporary variable `windowSatisfied`. This represents the number of customers that can potentially be satisfied if the technique is applied to that window.
+4. As we move the window, update the best possible result (`madeSatisfied`).
+5. Return the total satisfaction, which is the sum of the initially satisfied customers and the best possible window satisfaction.
+
+Let's implement this solution in PHP: **[1052. Grumpy Bookstore Owner](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/001052-grumpy-bookstore-owner/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+1. **Initial Satisfaction Calculation**:
+ - Loop through the `grumpy` array and for each minute where the owner is not grumpy (`grumpy[i] == 0`), add the corresponding number of customers to `satisfied`.
+2. **Sliding Window Calculation**:
+ - Start by summing up the number of customers for the first `minutes` minutes where the owner is grumpy. This sum is stored in `windowSatisfied`.
+ - Slide the window through the array, subtracting the customers from the minute that leaves the window and adding the customers from the minute that enters the window.
+ - Track the maximum value of `windowSatisfied` during this sliding process.
+3. **Final Calculation**:
+ - The total number of satisfied customers is the sum of `satisfied` (the customers who are already satisfied when the owner is not grumpy) and `madeSatisfied` (the maximum number of customers that can be made satisfied using the special technique).
+
+### **Example Walkthrough**
+
+#### Example 1:
+- **Input**:
+ ```php
+ customers = [1, 0, 1, 2, 1, 1, 7, 5]
+ grumpy = [0, 1, 0, 1, 0, 1, 0, 1]
+ minutes = 3
+ ```
+
+- **Initial Satisfaction**:
+ - In the minutes where `grumpy[i] == 0`, the customers are satisfied:
+ - Minute 0: 1 customer (owner not grumpy)
+ - Minute 2: 1 customer (owner not grumpy)
+ - Minute 4: 1 customer (owner not grumpy)
+ - Minute 6: 7 customers (owner not grumpy)
+ - Total satisfied = `1 + 1 + 1 + 7 = 10`
+
+- **Sliding Window Calculation**:
+ - For a window of 3 minutes, calculate how many more customers could be satisfied if the owner stays non-grumpy during those minutes:
+ - Minute 1, 3, 5 (grumpy): 0 + 2 + 1 = 3 potential customers
+ - Minute 2, 4, 6 (grumpy): 1 + 1 + 7 = 9 potential customers
+ - Best possible window = 9 customers
+
+- **Total Satisfaction** = `10 (initial) + 6 (maximized satisfaction) = 16`
+
+- **Output**:
+ ```php
+ 16
+ ```
+
+#### Example 2:
+- **Input**:
+ ```php
+ customers = [1]
+ grumpy = [0]
+ minutes = 1
+ ```
+
+- **Initial Satisfaction**: 1 customer (owner not grumpy)
+- **Sliding Window Calculation**: No grumpy time, so no need for a technique.
+- **Output**: `1`
+
+### **Time Complexity**
+- **Time Complexity**: O(n), where `n` is the length of the `customers` array. This is because we iterate over the array a few times (for initial satisfaction and the sliding window calculation).
+- **Space Complexity**: O(1), since we only use a few variables to track the satisfaction and window.
+
+This problem is efficiently solved using the sliding window technique. By iterating through the array and keeping track of potential satisfied customers, we can optimize the use of the owner's non-grumpy technique. The approach ensures that we calculate the maximum possible satisfaction in linear time, making it feasible even for the upper limits of the problem constraints.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
\ No newline at end of file
diff --git a/algorithms/001072-flip-columns-for-maximum-number-of-equal-rows/README.md b/algorithms/001072-flip-columns-for-maximum-number-of-equal-rows/README.md
new file mode 100644
index 000000000..c27b4a50d
--- /dev/null
+++ b/algorithms/001072-flip-columns-for-maximum-number-of-equal-rows/README.md
@@ -0,0 +1,109 @@
+1072\. Flip Columns For Maximum Number of Equal Rows
+
+**Difficulty:** Medium
+
+**Topics:** `Array`, `Hash Table`, `Matrix`
+
+You are given an `m x n` binary matrix `matrix`.
+
+You can choose any number of columns in the matrix and flip every cell in that column (i.e., Change the value of the cell from `0` to `1` or vice versa).
+
+Return _the maximum number of rows that have all values equal after some number of flips_.
+
+**Example 1:**
+
+- **Input:** matrix = [[0,1],[1,1]]
+- **Output:** 1
+- **Explanation:** After flipping no values, 1 row has all values equal.
+
+**Example 2:**
+
+- **Input:** matrix = [[0,1],[1,0]]
+- **Output:** 2
+- **Explanation:** After flipping values in the first column, both rows have equal values.
+
+
+**Example 3:**
+
+- **Input:** matrix = [[0,0,0],[0,0,1],[1,1,0]]
+- **Output:** 2
+- **Explanation:** After flipping values in the first two columns, the last two rows have equal values.
+
+
+
+**Constraints:**
+
+- `m == matrix.length`
+- `n == matrix[i].length`
+- `1 <= m, n <= 300`
+- `matrix[i][j]` is either `0` or `1`.
+
+
+**Hint:**
+1. Flipping a subset of columns is like doing a bitwise XOR of some number K onto each row. We want rows X with X ^ K = all 0s or all 1s. This is the same as X = X^K ^K = (all 0s or all 1s) ^ K, so we want to count rows that have opposite bits set. For example, if K = 1, then we count rows X = (00000...001, or 1111....110).
+
+
+
+**Solution:**
+
+We can utilize a hash map to group rows that can be made identical by flipping certain columns. Rows that can be made identical have either the same pattern or a complementary pattern (bitwise negation).
+
+Hereβs the step-by-step solution:
+
+### Algorithm:
+1. For each row, calculate its pattern and complementary pattern:
+ - The pattern is the row as it is.
+ - The complementary pattern is the result of flipping all bits in the row.
+2. Use a hash map to count occurrences of patterns and their complements.
+3. The maximum count for any single pattern or its complement gives the result.
+
+Let's implement this solution in PHP: **[1072. Flip Columns For Maximum Number of Equal Rows](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/001072-flip-columns-for-maximum-number-of-equal-rows/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+1. **Pattern and Complement:**
+ - For each row, the `pattern` is the concatenated row (e.g., `010`).
+ - The `complement` flips all bits of the row (e.g., `101`).
+2. **Hash Map:** Count the occurrences of each pattern and its complement. This helps group rows that can be made identical.
+3. **Max Count:** Find the maximum count of a single pattern or its complement to determine how many rows can be made identical.
+
+### Complexity:
+- **Time Complexity:** _**O(m x n)**_, where _**m**_ is the number of rows and _**n**_ is the number of columns.
+- **Space Complexity:** _**O(m x n)**_, for storing patterns in the hash map.
+
+This solution adheres to the constraints and is efficient for the problem size.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
diff --git a/algorithms/001072-flip-columns-for-maximum-number-of-equal-rows/solution.php b/algorithms/001072-flip-columns-for-maximum-number-of-equal-rows/solution.php
new file mode 100644
index 000000000..cf2b6720b
--- /dev/null
+++ b/algorithms/001072-flip-columns-for-maximum-number-of-equal-rows/solution.php
@@ -0,0 +1,42 @@
+'&(subExpr1, subExpr2, ..., subExprn)'
that evaluates to **the logical AND** of the inner expressions subExpr1, subExpr2, ..., subExprn
where `n >= 1`.
+- '|(subExpr1, subExpr2, ..., subExprn)'
that evaluates to **the logical OR** of the inner expressions subExpr1, subExpr2, ..., subExprn
where `n >= 1`.
+
+Given a string `expression` that represents a **boolean expression**, return _the evaluation of that expression_.
+
+It is **guaranteed** that the given expression is valid and follows the given rules.
+
+**Example 1:**
+
+- **Input:** expression = "&(|(f))"
+- **Output:** false
+- **Explanation:**\
+ First, evaluate |(f) --> f. The expression is now "&(f)".\
+ Then, evaluate &(f) --> f. The expression is now "f".\
+ Finally, return false.
+
+**Example 2:**
+
+- **Input:** expression = "|(f,f,f,t)"
+- **Output:** true
+- **Explanation:** The evaluation of (false OR false OR false OR true) is true.
+
+
+**Example 3:**
+
+- **Input:** expression = "!(&(f,t))"
+- **Output:** true
+- **Explanation:**\
+ First, evaluate &(f,t) --> (false AND true) --> false --> f. The expression is now "!(f)".\
+ Then, evaluate !(f) --> NOT false --> true. We return true.
+
+
+
+**Constraints:**
+
+- 1 <= expression.length <= 2 * 104
+- expression[i] is one following characters: `'('`, `')'`, `'&'`, `'|'`, `'!'`, `'t'`, `'f'`, and `','`.
+
+
+**Hint:**
+1. Write a function "parse" which calls helper functions "parse_or", "parse_and", "parse_not".
+
+
+
+**Solution:**
+
+We will break down the solution into smaller functions that handle parsing and evaluating different types of expressions: `parse_or`, `parse_and`, `parse_not`, and a main `parse` function that handles the parsing of the expression recursively. We will use a stack to keep track of nested expressions and evaluate them step-by-step.
+
+### Approach:
+
+1. **Parsing and Recursion**:
+ - Use a stack to keep track of expressions when encountering nested parentheses.
+ - Process characters sequentially and manage the stack for nested evaluations.
+ - When encountering a closing parenthesis `)`, extract the last set of expressions and apply the logical operation (`&`, `|`, or `!`).
+
+2. **Helper Functions**:
+ - `parse_or`: Evaluates `|(expr1, expr2, ..., exprN)` by returning `true` if at least one sub-expression is `true`.
+ - `parse_and`: Evaluates `&(expr1, expr2, ..., exprN)` by returning `true` only if all sub-expressions are `true`.
+ - `parse_not`: Evaluates `!(expr)` by returning the opposite of the sub-expression.
+
+3. **Expression Handling**:
+ - Single characters like `t` and `f` directly translate to `true` and `false`.
+ - When an operation is encountered (`&`, `|`, `!`), the inner expressions are evaluated based on their respective rules.
+
+Let's implement this solution in PHP: **[1106. Parsing A Boolean Expression](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/001106-parsing-a-boolean-expression/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+- **Main Function (`parseBooleanExpression`)**:
+ - Iterates through the expression and pushes characters to a stack.
+ - When encountering a `)`, it collects all the elements inside the parentheses and evaluates them based on the operation (`&`, `|`, `!`).
+ - Converts results to `'t'` (true) or `'f'` (false) and pushes them back to the stack.
+
+- **Helper Functions**:
+ - `parse_and`: Returns `true` if all sub-expressions are `'t'` (true).
+ - `parse_or`: Returns `true` if any sub-expression is `'t'`.
+ - `parse_not`: Reverses the boolean value of a single sub-expression.
+
+### Example Walkthrough:
+
+1. Input: `"&(|(f))"`
+ - Stack processing:
+ - `&`, `(`, `|`, `(`, `f`, `)`, `)` β The inner expression `|(f)` is evaluated to `f`.
+ - Resulting in `&(f)`, which evaluates to `f`.
+ - Output: `false`.
+
+2. Input: `"|(f,f,f,t)"`
+ - Evaluates the `|` operation:
+ - Finds one `'t'`, thus evaluates to `true`.
+ - Output: `true`.
+
+3. Input: `"!(&(f,t))"`
+ - Stack processing:
+ - `!`, `(`, `&`, `(`, `f`, `,`, `t`, `)`, `)` β `&(f,t)` evaluates to `f`.
+ - `!(f)` is then evaluated to `true`.
+ - Output: `true`.
+
+### Complexity:
+
+- **Time Complexity**: O(N), where N is the length of the expression. Each character is processed a limited number of times.
+- **Space Complexity**: O(N), due to the stack used to keep track of nested expressions.
+
+This solution is well-suited for the constraints and should handle the input size effectively.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
diff --git a/algorithms/001106-parsing-a-boolean-expression/solution.php b/algorithms/001106-parsing-a-boolean-expression/solution.php
new file mode 100644
index 000000000..620649e20
--- /dev/null
+++ b/algorithms/001106-parsing-a-boolean-expression/solution.php
@@ -0,0 +1,81 @@
+parse_and($subExpr);
+ } elseif ($operation == '|') {
+ $result = $this->parse_or($subExpr);
+ } elseif ($operation == '!') {
+ $result = $this->parse_not($subExpr);
+ }
+
+ // Push the result back to the stack as 't' or 'f'
+ $stack[] = $result ? 't' : 'f';
+ } elseif ($char != ',') {
+ // Push characters except ',' into the stack
+ $stack[] = $char;
+ }
+ }
+
+ // The result should be the final element in the stack
+ return $stack[0] == 't';
+ }
+
+ /**
+ * @param $subExpr
+ * @return bool
+ */
+ function parse_and($subExpr) {
+ foreach ($subExpr as $expr) {
+ if ($expr == 'f') {
+ return false;
+ }
+ }
+ return true;
+ }
+
+ /**
+ * @param $subExpr
+ * @return bool
+ */
+ function parse_or($subExpr) {
+ foreach ($subExpr as $expr) {
+ if ($expr == 't') {
+ return true;
+ }
+ }
+ return false;
+ }
+
+ /**
+ * @param $subExpr
+ * @return bool
+ */
+ function parse_not($subExpr) {
+ // subExpr contains only one element for NOT operation
+ return $subExpr[0] == 'f';
+ }
+}
\ No newline at end of file
diff --git a/algorithms/001110-delete-nodes-and-return-forest/README.md b/algorithms/001110-delete-nodes-and-return-forest/README.md
index 065f580d1..52ccdb55b 100644
--- a/algorithms/001110-delete-nodes-and-return-forest/README.md
+++ b/algorithms/001110-delete-nodes-and-return-forest/README.md
@@ -1,6 +1,8 @@
1110\. Delete Nodes And Return Forest
-Medium
+**Difficulty:** Medium
+
+**Topics:** `Array`, `Hash Table`, `Tree`, `Depth-First Search`, `Binary Tree`
Given the `root` of a binary tree, each node in the tree has a distinct value.
@@ -42,28 +44,33 @@ Let's implement this solution in PHP: **[1110. Delete Nodes And Return Forest](h
```php
left = new TreeNode($nodes[$i]);
- $queue[] = $current->left;
- }
- $i++;
- if ($i < count($nodes) && $nodes[$i] !== null) {
- $current->right = new TreeNode($nodes[$i]);
- $queue[] = $current->right;
- }
- $i++;
- }
- return $root;
+/**
+ * @param TreeNode $root
+ * @param Integer[] $to_delete
+ * @return TreeNode[]
+ */
+function delNodes($root, $to_delete) {
+ ...
+ ...
+ ...
+ /**
+ * go to ./solution.php
+ */
+}
+
+/**
+ * @param $node
+ * @param $forest
+ * @param $to_delete_set
+ * @return mixed|null
+ */
+function helper($node, &$forest, $to_delete_set) {
+ ...
+ ...
+ ...
+ /**
+ * go to ./solution.php
+ */
}
// Example usage:
@@ -109,4 +116,4 @@ If you found this series helpful, please consider giving the **[repository](http
If you want more helpful content like this, feel free to follow me:
- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
-- **[GitHub](https://github.com/mah-shamim)**
+- **[GitHub](https://github.com/mah-shamim)**
\ No newline at end of file
diff --git a/algorithms/001122-relative-sort-array/README.md b/algorithms/001122-relative-sort-array/README.md
index 50b5d70b3..9dd0ce6fd 100644
--- a/algorithms/001122-relative-sort-array/README.md
+++ b/algorithms/001122-relative-sort-array/README.md
@@ -1,6 +1,8 @@
1122\. Relative Sort Array
-Easy
+**Difficulty:** Easy
+
+**Topics:** `Array`, `Hash Table`, `Sorting`, `Counting Sort`
Given two arrays `arr1` and `arr2`, the elements of `arr2` are distinct, and all elements in `arr2` are also in `arr1`.
@@ -23,3 +25,133 @@ Sort the elements of `arr1` such that the relative ordering of items in `arr1` a
- All the elements of `arr2` are **distinct**.
- Each `arr2[i]` is in `arr1`.
+
+**Hint:**
+1. Using a hashmap, we can map the values of arr2 to their position in arr2.
+2. After, we can use a custom sorting function.
+
+
+
+**Solution:**
+
+The problem requires sorting an array `arr1` such that its elements are rearranged to match the relative order of elements found in another array `arr2`. Elements from `arr2` should appear in the same order in `arr1`, and any remaining elements from `arr1` that are not present in `arr2` should be placed at the end in ascending order.
+
+### **Key Points:**
+- Elements of `arr2` are distinct.
+- All elements in `arr2` are guaranteed to be present in `arr1`.
+- Any element in `arr1` that is not in `arr2` should appear at the end, sorted in ascending order.
+
+### **Approach:**
+
+1. **Use a Hashmap:** The order of `arr2` is essential. By storing the index of each element in `arr2`, we can sort `arr1` based on the relative order of `arr2`. We will use the values in `arr2` as keys to determine the order of elements in `arr1`.
+
+2. **Custom Sorting:** We need to implement a sorting function that first places elements from `arr2` in the correct order, then sorts the remaining elements (not found in `arr2`) in ascending order.
+
+3. **Mark Processed Elements:** To avoid processing the same element multiple times in `arr1`, we can mark elements already accounted for by setting them to a special value (like `-1`).
+
+### **Plan:**
+
+1. **Create a hashmap for the positions of `arr2` elements.** This hashmap will help map the relative order of elements in `arr2` to positions.
+
+2. **Traverse `arr1` and place elements in the `result` array** based on the relative order of `arr2`. If an element in `arr1` matches an element in `arr2`, add it to the `result` and mark it as visited by setting it to `-1`.
+
+3. **Sort the remaining elements in `arr1`** that do not appear in `arr2 in ascending order and append them to the result.
+
+4. **Return the final sorted `arr1`.**
+
+Let's implement this solution in PHP: **[1122. Relative Sort Array](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/001122-relative-sort-array/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+1. We first traverse through `arr2` and check each of its elements in `arr1`. Each time we find a match, we add it to the result array.
+
+2. After we've processed all elements in `arr2`, we then sort the remaining elements in `arr1` (those not found in `arr2`) and append them at the end of the result array.
+
+3. This approach ensures that elements from `arr2` appear in the specified order, while elements not found in `arr2` are placed at the end in ascending order.
+
+### **Example Walkthrough:**
+
+#### Example 1:
+- **Input:** `arr1 = [2,3,1,3,2,4,6,7,9,2,19]`, `arr2 = [2,1,4,3,9,6]`
+
+ **Steps:**
+ 1. Traverse `arr2`:
+ - First, process `2`, add all `2`s from `arr1` to `result` β `result = [2, 2, 2]`.
+ - Then, process `1`, add `1` from `arr1` to `result` β `result = [2, 2, 2, 1]`.
+ - Process `4`, add `4` from `arr1` to `result` β `result = [2, 2, 2, 1, 4]`.
+ - Process `3`, add both `3`s from `arr1` to `result` β `result = [2, 2, 2, 1, 4, 3, 3]`.
+ - Process `9`, add `9` from `arr1` to `result` β `result = [2, 2, 2, 1, 4, 3, 3, 9]`.
+ - Process `6`, add `6` from `arr1` to `result` β `result = [2, 2, 2, 1, 4, 3, 3, 9, 6]`.
+
+ 2. Remaining elements in `arr1` after processing `arr2` are `7` and `19`. Sort them and append β `result = [2, 2, 2, 1, 4, 3, 3, 9, 6, 7, 19]`.
+
+ **Output:** `[2, 2, 2, 1, 4, 3, 3, 9, 6, 7, 19]`.
+
+#### Example 2:
+- **Input:** `arr1 = [28,6,22,8,44,17]`, `arr2 = [22,28,8,6]`
+
+ **Steps:**
+ 1. Traverse `arr2`:
+ - Process `22`, add it from `arr1` β `result = [22]`.
+ - Process `28`, add it from `arr1` β `result = [22, 28]`.
+ - Process `8`, add it from `arr1` β `result = [22, 28, 8]`.
+ - Process `6`, add it from `arr1` β `result = [22, 28, 8, 6]`.
+
+ 2. Remaining elements in `arr1` after processing `arr2` are `44` and `17`. Sort them and append β `result = [22, 28, 8, 6, 17, 44]`.
+
+ **Output:** `[22, 28, 8, 6, 17, 44]`.
+
+### **Time Complexity:**
+
+- **Traversing `arr2` and `arr1`:** O(n * m), where `n` is the length of `arr1` and `m` is the length of `arr2`.
+- **Sorting the remaining elements of `arr1`:** O(n log n).
+- The overall time complexity is O(n * m + n log n), which is efficient given the problem constraints.
+
+### **Output for Example:**
+
+1. **Example 1:**
+ - **Input:** `arr1 = [2,3,1,3,2,4,6,7,9,2,19]`, `arr2 = [2,1,4,3,9,6]`
+ - **Output:** `[2, 2, 2, 1, 4, 3, 3, 9, 6, 7, 19]`
+
+2. **Example 2:**
+ - **Input:** `arr1 = [28,6,22,8,44,17]`, `arr2 = [22,28,8,6]`
+ - **Output:** `[22, 28, 8, 6, 17, 44]`
+
+
+The solution efficiently sorts `arr1` based on the relative order of `arr2` while placing remaining elements in ascending order. By using a hashmap to map the order and a custom sorting strategy, this problem is solved optimally. The algorithm works well within the provided constraints and efficiently handles the sorting process.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
diff --git a/algorithms/001137-n-th-tribonacci-number/README.md b/algorithms/001137-n-th-tribonacci-number/README.md
index 0eabaeb5f..c5bf54596 100644
--- a/algorithms/001137-n-th-tribonacci-number/README.md
+++ b/algorithms/001137-n-th-tribonacci-number/README.md
@@ -1,6 +1,8 @@
1137\. N-th Tribonacci Number
-Easy
+**Difficulty:** Easy
+
+**Topics:** `Math`, `Dynamic Programming`, `Memoization`
The **Tribonacci** sequence Tn is defined as follows:
@@ -27,4 +29,102 @@ Given `n`, return the value of Tn.
**Constraints:**
- 0 <= n <= 37
-- The answer is guaranteed to fit within a 32-bit integer, i.e. answer <= 2^31 - 1
.
+- The answer is guaranteed to fit within a 32-bit integer, i.e. answer <= 231 - 1
.
+
+
+**Hint:**
+1. Make an array F of length 38, and set F[0] = 0, F[1] = F[2] = 1.
+2. Now write a loop where you set F[n+3] = F[n] + F[n+1] + F[n+2], and return F[n].
+
+
+
+**Solution:**
+
+The Tribonacci sequence is a variation of the Fibonacci sequence, where each number is the sum of the previous three numbers. In this problem, you're tasked with calculating the N-th Tribonacci number using the given recurrence relation:
+- _**T0 = 0, T1 = 1, T2 = 1**_
+- _**Tn+3 = Tn + Tn+1 + Tn+2 for n β₯ 0**_
+
+The goal is to compute _**Tn**_ efficiently, considering the constraints _**0 β€ n β€ 37**_.
+
+
+### **Key Points**
+1. **Dynamic Programming Approach**: Since each Tribonacci number depends on the previous three, this is an excellent candidate for dynamic programming.
+2. **Space Optimization**: Instead of maintaining a full array for all intermediate values, we only need to track the last three numbers.
+3. **Constraints**: The value of _**n**_ is relatively small, so both time and space requirements are manageable.
+
+
+### **Approach**
+1. Use a dynamic programming technique to iteratively calculate Tn.
+2. Maintain a rolling window of size 3 (space optimization) to track the last three values.
+3. Initialize the base cases _**T0, T1, T2**_ as _**0**_, _**1**_, and _**1**_, respectively.
+4. Use a loop to calculate _**Tn**_ for _**n β₯ 3**_.
+
+
+### **Plan**
+1. Initialize an array `dp` of size 3 to store the values of _**T0, T1, T2**_.
+2. Iterate from _**3**_ to _**n**_, updating `dp` using the recurrence relation: _**dp[i % 3] = dp[0] + dp[1] + dp[2]**_
+3. Return the value of `dp[n % 3]` as the result.
+
+Let's implement this solution in PHP: **[1137. N-th Tribonacci Number](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/001137-n-th-tribonacci-number/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+- **Initialization**: Start with the first three Tribonacci numbers, _**T0 = 0, T1 = 1, T2 = 1**_.
+- **Loop Logic**: For _**n β₯ 3**_, calculate the next number as the sum of the previous three. Use modulo operations to store the values cyclically in the array.
+- **Return Result**: Use _**dp[n % 3]**_ to get the desired Tribonacci number after the loop.
+
+
+### **Example Walkthrough**
+#### Input: _**n = 4**_
+1. **Initialization**: _**dp = [0, 1, 1]**_ (represents _**T0, T1, T2**_).
+2. **Iteration**:
+ - _**i = 3**_: _**dp[0] = dp[0] + dp[1] + dp[2] = 0 + 1 + 1 = 2**_, _**dp = [2, 1, 1]**_
+ - _**i = 4**_: _**dp[1] = dp[0] + dp[1] + dp[2] = 2 + 1 + 1 = 4**_, _**dp = [2, 4, 1]**_
+3. **Return Result**: _**dp[4 % 3] = dp[1] = 4**_
+
+#### Output: _**4**_
+
+### **Time Complexity**
+- **Computation**: _**O(n)**_ since we iterate from _**3**_ to _**n**_.
+- **Space**: _**O(1)**_ as we only use a fixed-size array of length _**3**_.
+
+### **Output for Example**
+- _**n = 4**_: _**T4 = 4**_
+- _**n = 25**_: _**T25 = 1389537**_
+
+
+The optimized solution efficiently calculates the N-th Tribonacci number using a space-optimized dynamic programming approach. It ensures both correctness and performance, adhering to the constraints provided in the problem.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
\ No newline at end of file
diff --git a/algorithms/001140-stone-game-ii/README.md b/algorithms/001140-stone-game-ii/README.md
new file mode 100644
index 000000000..cd3a6b908
--- /dev/null
+++ b/algorithms/001140-stone-game-ii/README.md
@@ -0,0 +1,83 @@
+1140\. Stone Game II
+
+**Difficulty:** Medium
+
+**Topics:** `Array`, `Math`, `Dynamic Programming`, `Prefix Sum`, `Game Theory`
+
+Alice and Bob continue their games with piles of stones. There are a number of piles **arranged in a row**, and each pile has a positive integer number of stones `piles[i]`. The objective of the game is to end with the most stones.
+
+Alice and Bob take turns, with Alice starting first. Initially,` M = 1`.
+
+On each player's turn, that player can take **all the stones** in the **first** `X` remaining piles, where `1 <= X <= 2M`. Then, we set `M = max(M, X)`.
+
+The game continues until all the stones have been taken.
+
+Assuming Alice and Bob play optimally, return _the maximum number of stones Alice can get_.
+
+**Example 1:**
+
+- **Input:** piles = [2,7,9,4,4]
+- **Output:** 10
+- **Explanation:** If Alice takes one pile at the beginning, Bob takes two piles, then Alice takes 2 piles again. Alice can get 2 + 4 + 4 = 10 piles in total. If Alice takes two piles at the beginning, then Bob can take all three piles left. In this case, Alice get 2 + 7 = 9 piles in total. So we return 10 since it's larger.
+
+**Example 2:**
+
+- **Input:** piles = [1,2,3,4,5,100]
+- **Output:** 104
+
+**Constraints:**
+
+- 1 <= piles.length <= 100
+- 1 <= piles[i] <= 104
+
+**Hint:**
+1. Use dynamic programming: the states are (i, m) for the answer of `piles[i:]` and that given `m`.
+
+
+**Solution:**
+
+
+We need to use dynamic programming to find the maximum number of stones Alice can get if both players play optimally. Here's a step-by-step approach to develop the solution:
+
+1. **Define the State and Transition:**
+ - Define a 2D DP array where `dp[i][m]` represents the maximum stones Alice can collect starting from pile `i` with a maximum pick limit `m`.
+ - Use a prefix sum array to efficiently calculate the sum of stones in subarrays.
+
+2. **Base Case:**
+ - If there are no stones left to pick, the score is 0.
+
+3. **Recursive Case:**
+ - For each pile `i` and maximum allowed pick `m`, calculate the maximum stones Alice can collect by considering all possible moves (taking `1` to `2m` piles).
+
+4. **Transition Function:**
+ - For each possible number of piles Alice can pick, compute the total stones Alice can get and use the results of future states to decide the optimal move.
+
+Let's implement this solution in PHP: **[1140. Stone Game II](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/001140-stone-game-ii/solution.php)**
+
+```php
+stoneGameII($piles1) . "\n"; // Output: 10
+echo $solution->stoneGameII($piles2) . "\n"; // Output: 104
+?>
+```
+
+### Explanation:
+
+1. **Prefix Sum Calculation:** This helps in quickly calculating the sum of stones from any pile `i` to `j`.
+2. **DP Array Initialization:** `dp[i][m]` holds the maximum stones Alice can get starting from pile `i` with a maximum pick limit of `m`.
+3. **Dynamic Programming Transition:** For each pile and `m`, compute the maximum stones Alice can collect by iterating over possible pile counts she can take and updating the DP array accordingly.
+
+This approach ensures that the solution is computed efficiently, taking advantage of dynamic programming to avoid redundant calculations.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
diff --git a/algorithms/001140-stone-game-ii/solution.php b/algorithms/001140-stone-game-ii/solution.php
new file mode 100644
index 000000000..1216e3082
--- /dev/null
+++ b/algorithms/001140-stone-game-ii/solution.php
@@ -0,0 +1,55 @@
+= 0; --$i) {
+ $suffix[$i] += $suffix[$i + 1];
+ }
+
+ return $this->stoneGameIIHelper($suffix, 0, 1, $mem);
+ }
+
+ /**
+ * @param $suffix
+ * @param $i
+ * @param $M
+ * @param $mem
+ * @return mixed
+ */
+ private function stoneGameIIHelper($suffix, $i, $M, &$mem) {
+ $n = count($suffix);
+
+ // Base case: If we can take all remaining piles
+ if ($i + 2 * $M >= $n) {
+ return $suffix[$i];
+ }
+
+ // Check memoization
+ if ($mem[$i][$M] > 0) {
+ return $mem[$i][$M];
+ }
+
+ $opponent = $suffix[$i];
+
+ // Recursive case: Minimize the maximum stones opponent can take
+ for ($X = 1; $X <= 2 * $M; ++$X) {
+ if ($i + $X < $n) {
+ $opponent = min($opponent, $this->stoneGameIIHelper($suffix, $i + $X, max($M, $X), $mem));
+ }
+ }
+
+ // Memoize and return the result
+ $mem[$i][$M] = $suffix[$i] - $opponent;
+ return $mem[$i][$M];
+ }
+}
\ No newline at end of file
diff --git a/algorithms/001190-reverse-substrings-between-each-pair-of-parentheses/README.md b/algorithms/001190-reverse-substrings-between-each-pair-of-parentheses/README.md
index 6fe3c304b..6aebdbfff 100644
--- a/algorithms/001190-reverse-substrings-between-each-pair-of-parentheses/README.md
+++ b/algorithms/001190-reverse-substrings-between-each-pair-of-parentheses/README.md
@@ -1,6 +1,8 @@
1190\. Reverse Substrings Between Each Pair of Parentheses
-Medium
+**Difficulty:** Medium
+
+**Topics:** `String`, `Stack`
You are given a string `s` that consists of lower case English letters and brackets.
@@ -31,6 +33,13 @@ Your result should **not** contain any brackets.
- `s` only contains lower case English characters and parentheses.
- It is guaranteed that all parentheses are balanced.
+
+**Hint:**
+1. Find all brackets in the string.
+2. Does the order of the reverse matter ?
+3. The order does not matter.
+
+
**Solution:**
@@ -46,6 +55,19 @@ Here's the implementation in PHP: **[1190. Reverse Substrings Between Each Pair
```php
+```
+
+### Explanation:
+
+1. **Calculate the Cost for Each Character Pair:**
+ - The cost for each character pair in `s` and `t` is `abs(ord(s[i]) - ord(t[i]))`.
+
+2. **Sliding Window:**
+ - Initialize two pointers, `i` and `j`, representing the window's start and end positions.
+ - Iterate through the string with `i`, and add the corresponding costs to a running total.
+ - If the running total exceeds `maxCost`, increment `j` to reduce the window size and subtract the costs of characters that are no longer in the window.
+ - Track the maximum window size during the iteration.
+
+### **Example Walkthrough:**
+
+**Example 1:**
+```php
+$s = "abcd";
+$t = "bcdf";
+$maxCost = 3;
+```
+- Cost Array: `[1, 1, 1, 3]`
+- Start with `j = 0` and `i = 0`. The total cost starts at 0.
+ - At `i = 0`: Add cost of 1 (`abs(ord('a') - ord('b'))`).
+ - At `i = 1`: Add cost of 1 (`abs(ord('b') - ord('c'))`).
+ - At `i = 2`: Add cost of 1 (`abs(ord('c') - ord('d'))`).
+ - At `i = 3`: Add cost of 3 (`abs(ord('d') - ord('f'))`), total cost = 6, which exceeds `maxCost`.
+ - Shrink window by incrementing `j`, subtract cost of 1 (`abs(ord('a') - ord('b'))`), total cost = 5.
+ - Shrink window by incrementing `j` again, subtract cost of 1, total cost = 4.
+ - Shrink window by incrementing `j` again, subtract cost of 1, total cost = 3.
+- Maximum valid substring length is 3.
+
+**Example 2:**
+```php
+$s = "abcd";
+$t = "cdef";
+$maxCost = 3;
+```
+- Cost Array: `[2, 2, 2, 2]`
+- Start with `j = 0` and `i = 0`.
+ - At `i = 0`: Add cost of 2.
+ - At `i = 1`: Add cost of 2, total cost = 4, which exceeds `maxCost`.
+ - Shrink window by incrementing `j`.
+ - Maximum valid substring length is 1.
+
+### **Time Complexity:**
+- **O(n)**: We process each character once and use the sliding window technique to maintain a valid substring in linear time.
+
+### **Output for Example:**
+
+**Example 1:**
+```php
+$s = "abcd";
+$t = "bcdf";
+$maxCost = 3;
+```
+- Output: `3`
+
+**Example 2:**
+```php
+$s = "abcd";
+$t = "cdef";
+$maxCost = 3;
+```
+- Output: `1`
+
+The sliding window approach efficiently solves the problem by maintaining a valid substring whose transformation cost does not exceed `maxCost`. By leveraging two pointers (`i` and `j`), we can efficiently find the longest valid substring in linear time. This approach handles the problem's constraints effectively, ensuring optimal performance.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
diff --git a/algorithms/001219-path-with-maximum-gold/README.md b/algorithms/001219-path-with-maximum-gold/README.md
index 1dccc8831..ac87c80ce 100644
--- a/algorithms/001219-path-with-maximum-gold/README.md
+++ b/algorithms/001219-path-with-maximum-gold/README.md
@@ -1,6 +1,8 @@
1219\. Path with Maximum Gold
-Medium
+**Difficulty:** Medium
+
+**Topics:** `Array`, `Backtracking`, `Matrix`
In a gold mine `grid` of size `m x n`, each cell in this mine has an integer representing the amount of gold in that cell, `0` if it is empty.
@@ -43,3 +45,144 @@ Return the maximum amount of gold you can collect under the conditions:
- `1 <= m, n <= 15`
- `0 <= grid[i][j] <= 100`
- There are at most **25** cells containing gold.
+
+
+**Hint:**
+1. Use recursion to try all such paths and find the one with the maximum value.
+
+
+
+**Solution:**
+
+The "Path with Maximum Gold" problem is a typical backtracking problem where we need to find the maximum amount of gold we can collect in a grid-based mine. The challenge is to explore different paths under certain conditions like not revisiting any cell and avoiding cells that contain 0 gold. This problem requires recursive exploration of possible paths, which is where the backtracking approach comes in.
+
+### Key Points
+
+1. **Grid Representation**: The grid is a 2D matrix with each cell representing either a gold amount (positive integer) or 0 if it's empty.
+2. **Movement**: we can move up, down, left, or right from any given cell.
+3. **Goal**: Maximize the total gold collected by exploring valid paths.
+4. **Constraints**: we cannot visit the same cell more than once and must avoid cells with 0 gold.
+
+### Approach
+
+1. **Depth-First Search (DFS)**: The idea is to use recursion (DFS) to explore each potential path starting from any cell that contains gold.
+2. **Backtracking**: At each cell, after exploring the four possible directions (up, down, left, right), backtrack by restoring the state of the grid (i.e., marking the cell as unvisited).
+3. **Memoization**: To avoid re-exploring paths, we can mark cells as visited temporarily by setting their value to 0. Once we return from that path, we restore the cell value.
+4. **Track the Maximum**: For each starting cell, run the DFS and track the maximum gold collected by comparing it to the previously found maximum.
+
+### Plan
+
+1. **Initialization**: Get the dimensions of the grid.
+2. **DFS Function**: Implement a helper function that takes the current coordinates and recursively explores all four possible directions.
+3. **Iterate Through Grid**: Start DFS from each cell that contains gold, keeping track of the maximum gold collected.
+
+Let's implement this solution in PHP: **[1219. Path with Maximum Gold](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/001219-path-with-maximum-gold/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+The DFS function works as follows:
+- If the current position is out of bounds or the cell has no gold (`grid[i][j] == 0`), return 0.
+- Temporarily mark the current cell as visited by setting it to 0.
+- Explore all four directions (up, down, left, right), and add the gold from the current cell to the maximum gold collected from the next valid positions.
+- Restore the cell value after exploring.
+- The main function iterates through all cells and keeps track of the maximum gold collected.
+
+### Example Walkthrough
+
+**Example 1:**
+```php
+grid = [
+ [0,6,0],
+ [5,8,7],
+ [0,9,0]
+]
+```
+
+- Start from cell `(1,1)` which has 8 gold.
+- From there, move to `(1,2)` with 7 gold, then to `(2,2)` with 9 gold.
+- Collecting the total gold: `9 + 8 + 7 = 24`.
+
+**Example 2:**
+```php
+grid = [
+ [1,0,7],
+ [2,0,6],
+ [3,4,5],
+ [0,3,0],
+ [9,0,20]
+]
+```
+
+- Start from cell `(4,2)` with 20 gold.
+- Then move to `(2,2)` with 5 gold, then to `(2,1)` with 6 gold, and so on.
+- Collecting the total gold: `1 + 2 + 3 + 4 + 5 + 6 + 7 = 28`.
+
+### Time Complexity
+
+The time complexity is **O(m * n)**, where `m` is the number of rows and `n` is the number of columns in the grid. This is because each cell is visited once during the DFS exploration.
+
+- **DFS Calls**: Each DFS call explores four possible directions.
+- **Worst Case**: In the worst case, we explore all cells, leading to a time complexity of **O(m * n)**.
+
+### Output for Example
+
+For the example inputs:
+
+1. **Input 1:**
+```php
+grid = [
+ [0,6,0],
+ [5,8,7],
+ [0,9,0]
+]
+```
+**Output:** `24`
+
+2. **Input 2:**
+```php
+grid = [
+ [1,0,7],
+ [2,0,6],
+ [3,4,5],
+ [0,3,0],
+ [9,0,20]
+]
+```
+**Output:** `28`
+
+
+The "Path with Maximum Gold" problem is a great exercise in using DFS with backtracking. It requires careful exploration of all possible paths in a grid while ensuring we do not revisit cells. The approach demonstrated here ensures we can find the maximum amount of gold efficiently by using recursion and backtracking. The time complexity of **O(m * n)** makes the solution feasible even for the largest grid sizes, within the given constraints.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
+
diff --git a/algorithms/001233-remove-sub-folders-from-the-filesystem/README.md b/algorithms/001233-remove-sub-folders-from-the-filesystem/README.md
new file mode 100644
index 000000000..1866d40dc
--- /dev/null
+++ b/algorithms/001233-remove-sub-folders-from-the-filesystem/README.md
@@ -0,0 +1,108 @@
+1233\. Remove Sub-Folders from the Filesystem
+
+**Difficulty:** Medium
+
+**Topics:** `Array`, `String`, `Depth-First Search`, `Trie`
+
+Given a list of folders `folder`, return _the folders after removing all **sub-folders** in those folders_. You may return the answer in **any order**.
+
+If a `folder[i]` is located within another `folder[j]`, it is called a **sub-folder** of it. A sub-folder of `folder[j]` must start with `folder[j]`, followed by a `"/"`. For example, `"/a/b"` is a sub-folder of `"/a"`, but `"/b"` is not a sub-folder of `"/a/b/c"`.
+
+The format of a path is one or more concatenated strings of the form: `'/'` followed by one or more lowercase English letters.
+
+- For example, `"/leetcode"` and `"/leetcode/problems"` are valid paths while an empty string and `"/"` are not.
+
+
+**Example 1:**
+
+- **Input:** folder = ["/a","/a/b","/c/d","/c/d/e","/c/f"]
+- **Output:** ["/a","/c/d","/c/f"]
+- **Explanation:** Folders "/a/b" is a subfolder of "/a" and "/c/d/e" is inside of folder "/c/d" in our filesystem.
+
+**Example 2:**
+
+- **Input:** folder = ["/a","/a/b/c","/a/b/d"]
+- **Output:** ["/a"]
+- **Explanation:** Folders "/a/b/c" and "/a/b/d" will be removed because they are subfolders of "/a".
+
+
+**Example 3:**
+
+- **Input:** folder = ["/a/b/c","/a/b/ca","/a/b/d"]
+- **Output:** ["/a/b/c","/a/b/ca","/a/b/d"]
+
+
+
+**Constraints:**
+
+- 1 <= folder.length <= 4 * 104
+- `2 <= folder[i].length <= 100`
+- `folder[i]` contains only lowercase letters and `'/'`.
+- `folder[i]` always starts with the character `'/'`.
+- Each folder name is **unique**.
+
+
+**Hint:**
+1. Sort the folders lexicographically.
+2. Insert the current element in an array and then loop until we get rid of all of their subfolders, repeat this until no element is left.
+
+
+
+**Solution:**
+
+We can utilize a combination of sorting and string comparison. The steps below outline a solution in PHP:
+
+1. **Sort the Folders Lexicographically**: Sorting the folder paths in lexicographical order ensures that any sub-folder will follow its parent folder immediately. For example, `"/a"` will be followed by `"/a/b"` in the sorted list, allowing us to check for sub-folder relationships easily.
+
+2. **Identify and Filter Out Sub-Folders**: We can iterate through the sorted list, checking if the current folder path is a sub-folder of the previously added path. If it is, we skip it. If not, we add it to our result list.
+
+3. **Implement the Solution in PHP**: We keep track of the last folder path added to the result list. If the current folder starts with this last folder and is immediately followed by a `/`, itβs a sub-folder and should be ignored.
+
+Let's implement this solution in PHP: **[1233. Remove Sub-Folders from the Filesystem](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/001233-remove-sub-folders-from-the-filesystem/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+1. **Sorting**: The `sort()` function arranges folders in lexicographical order. This makes it easier to find sub-folder relationships because sub-folders will follow their parent folders directly.
+
+2. **Loop through each folder**:
+ - If `result` is empty (first iteration) or if the current folder path does not start with the last added folder followed by a `/`, it is not a sub-folder and is added to the `result` array.
+ - If it does start with the last folder path and has a `/` immediately following, itβs a sub-folder, and we skip adding it to `result`.
+
+3. **Result**: The function returns `result`, which contains only the root folders, excluding any sub-folders.
+
+This approach is efficient with a time complexity of _**O(n log n)**_ due to the sorting step, and the linear scan has _**O(n)**_, making this a good solution for larger inputs within the problem's constraints.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
diff --git a/algorithms/001233-remove-sub-folders-from-the-filesystem/solution.php b/algorithms/001233-remove-sub-folders-from-the-filesystem/solution.php
new file mode 100644
index 000000000..ce166eb0d
--- /dev/null
+++ b/algorithms/001233-remove-sub-folders-from-the-filesystem/solution.php
@@ -0,0 +1,27 @@
+1 <= nums[i] <= 10^5
- 1 <= k <= nums.length
+
+**Hint:**
+1. After replacing each even by zero and every odd by one can we use prefix sum to find answer ?
+2. Can we use two pointers to count number of sub-arrays ?
+3. Can we store the indices of odd numbers and for each k indices count the number of sub-arrays that contains them ?
+
+
+
+**Solution:**
+
+The problem requires finding the number of subarrays in an integer array `nums` that contain exactly `k` odd numbers. Subarrays are contiguous, and we need to optimize for performance due to constraints on the size of the array.
+
+### Key Points:
+1. **Continuous Subarrays**: The solution must consider only contiguous parts of the array.
+2. **Odd Numbers Identification**: Odd numbers can be identified by checking if `num % 2 != 0` or using bitwise operation `num & 1`.
+3. **Constraints**:
+ - Array size can be up to 50,000, so a brute-force approach (checking all possible subarrays) would be too slow.
+4. **Optimal Solution**:
+ - Using prefix sums or a sliding window approach efficiently counts the valid subarrays.
+
+### Approach:
+The problem can be solved using the **sliding window** technique combined with prefix sums. The main idea is to efficiently track the number of odd numbers within a moving subarray and count the valid subarrays.
+
+### Plan:
+1. **Transform the Array**: Replace odd numbers with `1` and even numbers with `0` to simplify counting.
+2. **Prefix Sum with Sliding Window**:
+ - Maintain a count of subarrays with exactly `k` odd numbers using a prefix sum and a hash table.
+ - Track how many times each prefix sum has occurred to efficiently calculate the number of valid subarrays ending at the current position.
+3. **Two Pointers Sliding Window**:
+ - Track the indices of odd numbers and compute the valid subarrays based on the indices of the first and last `k` odd numbers in the window.
+
+Let's implement this solution in PHP: **[1248. Count Number of Nice Subarrays](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/001248-count-number-of-nice-subarrays/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+We iterate through the array while maintaining the following:
+1. **`r[1]`**: Number of odd numbers in the current window.
+2. **`pre`**: Position of the left boundary of the valid window (updated when `r[1]` equals `k`).
+3. **`cur`**: Tracks the first valid subarray start for the current window.
+4. Count subarrays by determining how many valid windows can be formed.
+
+### Example Walkthrough:
+
+#### Example 1:
+**Input**: `nums = [1, 1, 2, 1, 1], k = 3`
+
+1. Convert the array to `[1, 1, 0, 1, 1]`.
+2. Use a sliding window to count:
+ - Window `[1, 1, 0, 1]`: 3 odd numbers β Count = 1.
+ - Window `[1, 0, 1, 1]`: 3 odd numbers β Count = 1.
+3. **Result**: `2`.
+
+#### Example 2:
+**Input**: `nums = [2, 4, 6], k = 1`
+
+1. Convert the array to `[0, 0, 0]`.
+2. No odd numbers β **Result**: `0`.
+
+#### Example 3:
+**Input**: `nums = [2, 2, 2, 1, 2, 2, 1, 2, 2, 2], k = 2`
+
+1. Convert the array to `[0, 0, 0, 1, 0, 0, 1, 0, 0, 0]`.
+2. Count valid windows:
+ - For each pair of odd numbers (`k = 2`), calculate all subarrays containing them.
+ - **Result**: `16`.
+
+### Time Complexity:
+- **Sliding Window Update**: O(n) β Each element is processed once.
+- **Overall Complexity**: O(n).
+
+### Space Complexity:
+- O(1) β Constant space for tracking counts.
+
+### Output for Examples:
+1. Example 1: Output = 2.
+2. Example 2: Output = 0.
+3. Example 3: Output = 16.
+
+The sliding window with prefix sums efficiently counts the number of "nice" subarrays. This approach is optimal for handling large arrays due to its linear time complexity.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
\ No newline at end of file
diff --git a/algorithms/001249-minimum-remove-to-make-valid-parentheses/README.md b/algorithms/001249-minimum-remove-to-make-valid-parentheses/README.md
index 01742a591..aadec6cd3 100644
--- a/algorithms/001249-minimum-remove-to-make-valid-parentheses/README.md
+++ b/algorithms/001249-minimum-remove-to-make-valid-parentheses/README.md
@@ -1,6 +1,8 @@
1249\. Minimum Remove to Make Valid Parentheses
-Medium
+**Difficulty:** Medium
+
+**Topics:** `String`, `Stack`
Given a string s of `'('` , `')'` and lowercase English characters.
@@ -38,3 +40,125 @@ Formally, a parentheses string is valid if and only if:
* 1 <= s.length <= 105
* `s[i]` is either`'('` , `')'`, or lowercase English letter`.`
+
+**Hint:**
+1. Each prefix of a balanced parentheses has a number of open parentheses greater or equal than closed parentheses, similar idea with each suffix.
+2. Check the array from left to right, remove characters that do not meet the property mentioned above, same idea in backward way.
+
+
+
+**Solution:**
+
+The task is to remove the minimum number of parentheses from a given string to make it a valid parentheses string. A valid parentheses string is defined as:
+- It is empty or contains only lowercase letters.
+- It can be written as `AB`, where `A` and `B` are valid parentheses strings.
+- It can be written as `(A)`, where `A` is a valid parentheses string.
+
+### Key Points
+1. **Valid Parentheses String**: The number of opening and closing parentheses must match. Each opening parenthesis `(` must have a corresponding closing parenthesis `)` in a valid order.
+2. **Strategy**: We need to remove the invalid parentheses while keeping the valid characters (both letters and valid parentheses) in the resulting string.
+3. **Two-pass Approach**: The solution involves two passes through the string:
+ - **First pass**: Remove invalid closing parentheses `)` that don't have a matching opening parenthesis `(`.
+ - **Second pass**: Remove invalid opening parentheses `(` that don't have a matching closing parenthesis `)`.
+
+### Approach
+
+1. **First Pass**: Iterate over the string from left to right. Use a counter (`openCount`) to keep track of the balance between opening and closing parentheses.
+ - If we encounter a `)` and the counter `openCount` is zero, it means there is no preceding `(` to balance it, so skip this `)`.
+ - For each `(`, increase the counter, and for each valid `)`, decrease the counter.
+ - Save all valid characters in a temporary stack.
+
+2. **Second Pass**: After the first pass, iterate over the temporary stack in reverse. Use a counter to track the number of `)` characters.
+ - If we encounter an `(` and the counter for `)` is zero, it means there is no closing parenthesis to balance it, so skip this `(`.
+ - Save all valid characters in the final result list.
+
+3. **Return the Result**: The result list will be in reverse order, so reverse it back and return as a string.
+
+### Plan
+1. **Step 1**: Traverse the string from left to right to filter out unnecessary closing parentheses.
+2. **Step 2**: Traverse the filtered string from right to left to filter out unnecessary opening parentheses.
+3. **Step 3**: Reverse the final list of valid characters and join them into a string.
+
+Let's implement this solution in PHP: **[1249. Minimum Remove to Make Valid Parentheses](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/001249-minimum-remove-to-make-valid-parentheses/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+The algorithm uses two passes to ensure that only valid parentheses are kept:
+- **First pass** ensures that we remove invalid closing parentheses `)` which donβt have matching opening parentheses `(`.
+- **Second pass** ensures that we remove invalid opening parentheses `(` which donβt have matching closing parentheses `)`.
+
+Each pass modifies the string based on the matching parentheses, making sure the final string is valid.
+
+### Example Walkthrough
+
+**Example 1**:
+```text
+Input: "lee(t(c)o)de)"
+First pass (remove invalid closing parentheses):
+Result after first pass: "lee(t(c)o)de"
+Second pass (remove invalid opening parentheses):
+Final result: "lee(t(c)o)de"
+```
+
+**Example 2**:
+```text
+Input: "a)b(c)d"
+First pass (remove invalid closing parentheses):
+Result after first pass: "ab(c)d"
+Second pass (no change needed as all parentheses are valid):
+Final result: "ab(c)d"
+```
+
+**Example 3**:
+```text
+Input: "))(("
+First pass (remove invalid closing parentheses):
+Result after first pass: ""
+Second pass (no change needed as there are no valid parentheses):
+Final result: ""
+```
+
+### Time Complexity
+
+- **Time Complexity**: O(n), where n is the length of the input string.
+ - We traverse the string twice, each traversal taking O(n) time.
+- **Space Complexity**: O(n), where n is the length of the input string.
+ - We use a temporary stack to store intermediate results, which could store up to the length of the input.
+
+This approach ensures that we can efficiently remove invalid parentheses in a two-pass solution. By using a stack and maintaining a balance between opening and closing parentheses, we can guarantee a valid output string with minimal removals. The solution is both time-efficient and space-efficient, handling large input sizes within the problem's constraints.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
diff --git a/algorithms/001255-maximum-score-words-formed-by-letters/README.md b/algorithms/001255-maximum-score-words-formed-by-letters/README.md
index d753ad1a9..92f7cc05f 100644
--- a/algorithms/001255-maximum-score-words-formed-by-letters/README.md
+++ b/algorithms/001255-maximum-score-words-formed-by-letters/README.md
@@ -1,6 +1,8 @@
1255\. Maximum Score Words Formed by Letters
-Hard
+**Difficulty:** Hard
+
+**Topics:** `Array`, `String`, `Dynamic Programming`, `Backtracking`, `Bit Manipulation`, `Bitmask`
Given a list of `words`, list of single `letters` (might be repeating) and `score` of every character.
@@ -41,4 +43,137 @@ It is not necessary to use all characters in `letters` and each letter can only
- letters[i].length == 1
- score.length == 26
- 0 <= score[i] <= 10
-- `words[i]`, `letters[i]` contains only lower case English letters.
\ No newline at end of file
+- `words[i]`, `letters[i]` contains only lower case English letters.
+
+
+**Hint:**
+1. Note that `words.length` is small. This means you can iterate over every subset of words (2N).
+
+
+
+**Solution:**
+
+The problem is about maximizing the score of valid words formed using a given set of letters. The words can only be used once, and each letter in the list can be used at most as many times as it appears in the list. The score for each word is determined by the sum of the scores of its individual characters. We aim to find the maximum score obtainable from any combination of valid words formed.
+
+### Key Points
+
+1. **Words and Letters**: You are given a list of words and a set of available letters.
+2. **Score Mapping**: Each letter has an associated score between 0 and 10 (inclusive). The score of each word is the sum of the scores of its characters.
+3. **Valid Word Formation**: Each word must be formed using the available letters, without exceeding the letter counts in the list.
+4. **Maximizing the Score**: We need to compute the maximum score by selecting any combination of words that can be formed from the given letters.
+
+### Approach
+
+- **Bitmasking**: Since the number of words is small (<=14), we can iterate over all subsets of words using bitmasking. Each subset corresponds to a combination of words. There are _**2^n**_ subsets, where _**n**_ is the number of words.
+
+- **Counting Letters**: For each subset of words, we need to check if we can form those words with the available letters. We can maintain a frequency count of letters in the list and ensure that we don't exceed the available count for any letter.
+
+- **Score Calculation**: For each valid combination of words, calculate the total score by summing the scores of the characters in those words.
+
+### Plan
+
+1. **Count Available Letters**: Create a frequency count for the available letters in the list.
+2. **Iterate Over All Word Subsets**: For each subset of words, calculate the letter frequency required and check if itβs valid.
+3. **Calculate Score**: If the subset is valid, compute the score for the words in that subset.
+4. **Track Maximum Score**: Keep track of the maximum score encountered.
+
+Let's implement this solution in PHP: **[1255. Maximum Score Words Formed by Letters](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/001255-maximum-score-words-formed-by-letters/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+1. **Letter Count**: For each word, we calculate how many times each letter appears. We need to ensure that the combined letter counts for any subset of words don't exceed the available counts from the letter list.
+
+2. **Subset Generation**: We generate subsets of words using bitmasking. For each subset, we check if it's possible to form the words in that subset with the available letters. If it's possible, we calculate the score and update the maximum score if necessary.
+
+3. **Score Calculation**: For each valid subset, the score is calculated by adding the scores of individual letters in the words of that subset.
+
+### Example Walkthrough
+
+**Example 1:**
+- **Input**:
+ ```php
+ words = ["dog","cat","dad","good"]
+ letters = ["a","a","c","d","d","d","g","o","o"]
+ score = [1,0,9,5,0,0,3,0,0,0,0,0,0,0,2,0,0,0,0,0,0,0,0,0,0,0]
+ ```
+
+- **Step 1**: Count the occurrences of letters:
+ ```php
+ letterCounts = [2, 0, 1, 3, 0, 0, 1, 0, 0, 0, 0, 0, 0, 0, 1, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0] // for a to z
+ ```
+
+- **Step 2**: Generate subsets of words and check if they can be formed with the available letters:
+ - For subset `["dad", "good"]`, we calculate the letter counts needed:
+ ```php
+ wordCounts = [2, 0, 1, 3, 0, 0, 1, 0, 0, 0, 0, 0, 0, 0, 1, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0]
+ ```
+ This is valid because the letter counts do not exceed the available letters.
+
+- **Step 3**: Calculate the score for this valid subset:
+ ```php
+ score = 5 + 1 + 5 + 3 + 2 + 2 + 5 = 23
+ ```
+- **Step 4**: Update the maximum score. The final result is `23`.
+
+### Time Complexity
+
+- **Generating subsets**: There are _**2n**_ subsets, where _**n**_ is the number of words.
+- **Checking each subset**: For each subset, we need to calculate the letter counts, which takes _**O(w)**_ time, where _**w**_ is the maximum length of a word.
+- **Total Complexity**: The total time complexity is _**O(2n Γ w)**_, which is feasible given _**n β€ 14**_.
+
+### Output for Example 1
+
+```php
+maxScoreWords(["dog", "cat", "dad", "good"], ["a", "a", "c", "d", "d", "d", "g", "o", "o"], [1,0,9,5,0,0,3,0,0,0,0,0,0,0,2,0,0,0,0,0,0,0,0,0,0,0])
+```
+
+**Output**:
+```
+23
+```
+
+This approach efficiently computes the maximum score of any valid combination of words using bitmasking and dynamic checking of letter counts. The use of subsets ensures that we examine all possible combinations of words, while the letter counting ensures we only consider valid word formations.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
diff --git a/algorithms/001277-count-square-submatrices-with-all-ones/README.md b/algorithms/001277-count-square-submatrices-with-all-ones/README.md
new file mode 100644
index 000000000..e1dfb23f2
--- /dev/null
+++ b/algorithms/001277-count-square-submatrices-with-all-ones/README.md
@@ -0,0 +1,105 @@
+1277\. Count Square Submatrices with All Ones
+
+**Difficulty:** Medium
+
+**Topics:** `Array`, `Dynamic Programming`, `Matrix`
+
+Given a `m * n` matrix of ones and zeros, return _how many **square** submatrices have all ones_.
+
+**Example 1:**
+
+- **Input:** matrix = [[0,1,1,1], [1,1,1,1], [0,1,1,1]]
+- **Output:** 15
+- **Explanation:**
+ - There are **10** squares of side 1.
+ - There are **4** squares of side 2.
+ - There is **1** square of side 3.
+ - Total number of squares = 10 + 4 + 1 = **15**.
+
+**Example 2:**
+
+- **Input:** matrix = [[1,0,1], [1,1,0], [1,1,0]]
+- **Output:** 7
+- **Explanation:**
+ - There are 6 squares of side 1.
+ - There is 1 square of side 2.
+ - Total number of squares = 6 + 1 = 7.
+
+
+**Constraints:**
+
+- `1 <= arr.length <= 300`
+- `1 <= arr[0].length <= 300`
+- `0 <= arr[i][j] <= 1`
+
+
+**Hint:**
+1. Create an additive table that counts the sum of elements of **submatrix** with the superior corner at `(0,0)`.
+2. Loop over all **subsquares** in O(n3)
and check if the sum make the whole array to be ones, if it checks then add `1` to the answer.
+
+
+
+**Solution:**
+
+We can use **Dynamic Programming (DP)** to keep track of the number of square submatrices with all ones that can end at each cell in the matrix. Here's the approach to achieve this:
+
+1. **DP Matrix Definition**:
+ - Define a DP matrix `dp` where `dp[i][j]` represents the size of the largest square submatrix with all ones that has its bottom-right corner at cell `(i, j)`.
+
+2. **Transition Formula**:
+ - For each cell `(i, j)` in the matrix:
+ - If `matrix[i][j]` is 1, the value of `dp[i][j]` depends on the minimum of the squares that can be formed by extending from `(i-1, j)`, `(i, j-1)`, and `(i-1, j-1)`. The transition formula is:
+ ```
+ dp[i][j] = min(dp[i-1][j], dp[i][j-1], dp[i-1][j-1]) + 1
+ ```
+ - If `matrix[i][j]` is 0, `dp[i][j]` will be 0 because a square of ones cannot end at a cell with a zero.
+
+3. **Count All Squares**:
+ - Accumulate the values of `dp[i][j]` for all `(i, j)` to get the total number of squares of all sizes.
+
+4. **Time Complexity**:
+ - The solution works in _**O(m X n)**_, where _**m**_ and _**n**_ are the dimensions of the matrix.
+
+Let's implement this solution in PHP: **[1277. Count Square Submatrices with All Ones](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/001277-count-square-submatrices-with-all-ones/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+1. We initialize a 2D array `dp` to keep track of the size of the largest square submatrix ending at each position `(i, j)`.
+2. For each cell in the matrix:
+ - If the cell has a 1, we compute `dp[i][j]` based on neighboring cells and add its value to `totalSquares`.
+3. Finally, `totalSquares` contains the count of all square submatrices with all ones.
+
+This solution is efficient and meets the constraints provided in the problem.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
diff --git a/algorithms/001277-count-square-submatrices-with-all-ones/solution.php b/algorithms/001277-count-square-submatrices-with-all-ones/solution.php
new file mode 100644
index 000000000..48bb786b6
--- /dev/null
+++ b/algorithms/001277-count-square-submatrices-with-all-ones/solution.php
@@ -0,0 +1,31 @@
+
+```
+
+### Explanation:
+
+- **Row Traversal:** For each row, we calculate the possible falling path sums by adding the element in the current row to the minimum or second minimum sum of the previous row, ensuring no two elements come from the same column.
+- **Optimization:** Instead of recalculating the minimum for each element in the row, we keep track of the two smallest sums in the previous row and use them accordingly.
+- **Efficiency:** This solution only requires a single pass through the matrix, making it efficient for large grids.
+
+### Example Walkthrough:
+Consider the matrix:
+
+```
+grid = [[1, 2, 3],
+ [4, 5, 6],
+ [7, 8, 9]]
+```
+
+- **Step 1 (First row):**
+ - The first row does not have previous rows to compare to, so the minimum path sum for each column is the value itself.
+ - `minFirstPathSum = 1`, `minSecondPathSum = 2` (since 1 is the smallest and 2 is the second smallest in the first row).
+
+- **Step 2 (Second row):**
+ - For the second row, we cannot choose elements from the same column as the first row:
+ - For column 0: The falling sum is `1 (from row 1, column 0) + 4 (from row 2, column 0) = 5`.
+ - For column 1: The falling sum is `2 (from row 1, column 1) + 5 (from row 2, column 1) = 7`.
+ - For column 2: The falling sum is `1 (from row 1, column 0) + 6 (from row 2, column 2) = 7`.
+ - After the second row, `minFirstPathSum = 5` and `minSecondPathSum = 6`.
+
+- **Step 3 (Third row):**
+ - For the third row, we choose the best path that doesn't repeat columns from the second row:
+ - For column 0: The falling sum is `5 (from row 2, column 0) + 7 (from row 3, column 0) = 12`.
+ - For column 1: The falling sum is `5 (from row 2, column 0) + 8 (from row 3, column 1) = 13`.
+ - For column 2: The falling sum is `6 (from row 2, column 1) + 9 (from row 3, column 2) = 15`.
+ - After the third row, `minFirstPathSum = 13` and `minSecondPathSum = 15`.
+
+### Output for Example:
+For the matrix:
+```
+grid = [[1, 2, 3],
+ [4, 5, 6],
+ [7, 8, 9]]
+```
+The minimum falling path sum is `13`, which corresponds to the path `[1, 5, 7]`.
+
+### Time Complexity:
+- **Time Complexity:** O(nΒ²), where `n` is the number of rows (or columns) in the matrix. We process each element of the matrix once.
+- **Space Complexity:** O(n), as we only need a few variables to store the minimum and second minimum sums for each row, and we donβt require a full DP table.
+
+This approach efficiently computes the minimum falling path sum using dynamic programming with optimization through the tracking of the two smallest values from the previous row. This solution scales well for larger matrices and avoids redundant recalculations, providing an optimal solution.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
diff --git a/algorithms/001310-xor-queries-of-a-subarray/README.md b/algorithms/001310-xor-queries-of-a-subarray/README.md
new file mode 100644
index 000000000..9016f11d3
--- /dev/null
+++ b/algorithms/001310-xor-queries-of-a-subarray/README.md
@@ -0,0 +1,124 @@
+1310\. XOR Queries of a Subarray
+
+**Difficulty:** Medium
+
+**Topics:** `Array`, `Bit Manipulation`, `Prefix Sum`
+
+You are given an array `arr` of positive integers. You are also given the array `queries` where queries[i] = [lefti, righti]
.
+
+For each query `i` compute the XOR of elements from lefti
to righti
(that is, arr[lefti] XOR arr[lefti + 1] XOR ... XOR arr[righti]
).
+
+Return _an array `answer` where `answer[i]` is the answer to the ith query_.
+
+**Example 1:**
+
+- **Input:** arr = [1,3,4,8], queries = [[0,1],[1,2],[0,3],[3,3]]
+- **Output:** [2,7,14,8]
+- **Explanation:**\
+ The binary representation of the elements in the array are:\
+ 1 = 0001\
+ 3 = 0011\
+ 4 = 0100\
+ 8 = 1000\
+ The XOR values for queries are:\
+ [0,1] = 1 xor 3 = 2\
+ [1,2] = 3 xor 4 = 7\
+ [0,3] = 1 xor 3 xor 4 xor 8 = 14\
+ [3,3] = 8
+
+**Example 2:**
+
+- **Input:** arr = [4,8,2,10], queries = [[2,3],[1,3],[0,0],[0,3]]
+- **Output:** [8,0,4,4]
+
+
+
+**Constraints:**
+
+- 1 <= arr.length, queries.length <= 3 * 104
+- 1 <= arr[i] <= 109
+- queries[i].length == 2
+- 0 <= lefti <= righti < arr.length
+
+
+**Hint:**
+1. What is the result of x ^ y ^ x ?
+2. Compute the prefix sum for XOR.
+3. Process the queries with the prefix sum values.
+
+
+
+**Solution:**
+
+We can make use of the **prefix XOR** technique. Here's how it works:
+
+### Approach:
+1. **Prefix XOR Array**: We compute a prefix XOR array where `prefix[i]` represents the XOR of all elements from the start of the array up to index `i`. This allows us to compute the XOR of any subarray in constant time.
+
+2. **XOR of a subarray**:
+ - To compute the XOR of elements between indices `left` and `right`:
+ - If `left > 0`, we can compute the XOR from `left` to `right` as `prefix[right] ^ prefix[left - 1]`.
+ - If `left == 0`, then the result is simply `prefix[right]`.
+
+ This allows us to answer each query in constant time after constructing the prefix XOR array.
+
+### Plan:
+1. Build the prefix XOR array.
+2. For each query, use the prefix XOR array to compute the XOR for the range `[left_i, right_i]`.
+
+Let's implement this solution in PHP: **[1310. XOR Queries of a Subarray](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/001310-xor-queries-of-a-subarray/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+1. **Prefix XOR Construction**:
+ - The array `prefix` is built such that `prefix[i]` contains the XOR of all elements from `arr[0]` to `arr[i]`.
+ - For example, given `arr = [1, 3, 4, 8]`, the `prefix` array will be `[1, 1^3, 1^3^4, 1^3^4^8]` or `[1, 2, 6, 14]`.
+
+2. **Answering Queries**:
+ - For each query `[left, right]`, we compute the XOR of the subarray `arr[left]` to `arr[right]` using:
+ - `prefix[right] ^ prefix[left - 1]` (if `left > 0`)
+ - `prefix[right]` (if `left == 0`)
+
+### Time Complexity:
+- **Building the prefix array**: O(n), where `n` is the length of the `arr`.
+- **Processing the queries**: O(q), where `q` is the number of queries.
+- **Overall Time Complexity**: O(n + q), which is efficient for the given constraints.
+
+This approach ensures we can handle up to 30,000 queries on an array of size up to 30,000 efficiently.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
diff --git a/algorithms/001310-xor-queries-of-a-subarray/solution.php b/algorithms/001310-xor-queries-of-a-subarray/solution.php
new file mode 100644
index 000000000..ccf779a14
--- /dev/null
+++ b/algorithms/001310-xor-queries-of-a-subarray/solution.php
@@ -0,0 +1,34 @@
+0 <= arr.length <= 105
+- -109 <= arr[i] <= 109
+
+
+
+**Hint:**
+1. Use a temporary array to copy the array and sort it.
+2. The rank of each element is the number of unique elements smaller than it in the sorted array plus one.
+
+
+
+**Solution:**
+
+We can break it down into the following steps:
+
+1. **Copy and sort the array:** This helps in determining the rank of each unique element.
+2. **Use a hash map to assign ranks to elements:** Since multiple elements can share the same value, a hash map (associative array in PHP) will help map each element to its rank.
+3. **Replace the original elements with their ranks:** Using the hash map, we can replace each element in the original array with its corresponding rank.
+
+Let's implement this solution in PHP: **[1331. Rank Transform of an Array](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/001331-rank-transform-of-an-array/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+1. **Copy and sort the array:**
+ - We create a copy of the input array `$sorted` and sort it. This helps in determining the rank of each unique element.
+
+2. **Assign ranks to elements:**
+ - We iterate through the sorted array and use a hash map `$rank` to store the rank of each unique element.
+ - We use `isset` to check if an element has already been assigned a rank. If not, we assign the current rank and increment it.
+
+3. **Replace elements with their ranks:**
+ - We then iterate through the original array and replace each element with its corresponding rank by looking it up in the `$rank` hash map.
+
+### Time Complexity:
+- Sorting the array takes _**`O(n log n)`**_, where _**`n`**_ is the size of the array.
+- Assigning ranks and replacing values takes _**`O(n`**_).
+- Overall time complexity is _**`O(n log n)`**_.
+
+This solution efficiently handles large arrays while maintaining simplicity.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
diff --git a/algorithms/001331-rank-transform-of-an-array/solution.php b/algorithms/001331-rank-transform-of-an-array/solution.php
new file mode 100644
index 000000000..5ae99336c
--- /dev/null
+++ b/algorithms/001331-rank-transform-of-an-array/solution.php
@@ -0,0 +1,39 @@
+-103 <= arr[i] <= 103
+
+
+**Hint:**
+1. Loop from `i = 0` to `arr.length`, maintaining in a hashTable the array elements from `[0, i - 1]`.
+2. On each step of the loop check if we have seen the element `2 * arr[i]` so far.
+3. Also check if we have seen `arr[i] / 2` in case `arr[i] % 2 == 0`.
+
+
+
+**Solution:**
+
+We can use a hash table (associative array) to track the elements we have already encountered while iterating through the array. The idea is to check for each element `arr[i]` if its double (i.e., `2 * arr[i]`) or half (i.e., `arr[i] / 2` if it's an even number) has already been encountered.
+
+Hereβs a step-by-step solution:
+
+### Plan:
+1. Iterate through the array.
+2. For each element `arr[i]`, check if we have seen `2 * arr[i]` or `arr[i] / 2` (if `arr[i]` is even) in the hash table.
+3. If any condition is satisfied, return `true`.
+4. Otherwise, add `arr[i]` to the hash table and continue to the next element.
+5. If no match is found by the end, return `false`.
+
+Let's implement this solution in PHP: **[1346. Check If N and Its Double Exist](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/001346-check-if-n-and-its-double-exist/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+1. **Hash Table**: We use the `$hashTable` associative array to store the elements we've encountered so far.
+2. **First Condition**: For each element `arr[i]`, we check if `arr[i] * 2` exists in the hash table.
+3. **Second Condition**: If the element is even, we check if `arr[i] / 2` exists in the hash table.
+4. **Adding to Hash Table**: After checking, we add `arr[i]` to the hash table for future reference.
+5. **Return**: If we find a match, we immediately return `true`. If no match is found after the loop, we return `false`.
+
+### Time Complexity:
+- The time complexity is **O(n)**, where `n` is the length of the array. This is because each element is processed once and checking or adding elements in the hash table takes constant time on average.
+
+### Space Complexity:
+- The space complexity is **O(n)** due to the storage required for the hash table.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
diff --git a/algorithms/001346-check-if-n-and-its-double-exist/solution.php b/algorithms/001346-check-if-n-and-its-double-exist/solution.php
new file mode 100644
index 000000000..5d85b6882
--- /dev/null
+++ b/algorithms/001346-check-if-n-and-its-double-exist/solution.php
@@ -0,0 +1,29 @@
+val = $val;
+ $this->next = $next;
+ }
+}
+
+// Definition for a binary tree node.
+class TreeNode {
+ public $val = 0;
+ public $left = null;
+ public $right = null;
+ function __construct($val = 0, $left = null, $right = null) {
+ $this->val = $val;
+ $this->left = $left;
+ $this->right = $right;
+ }
+}
+
+class Solution {
+
+ /**
+ * @param ListNode $head
+ * @param TreeNode $root
+ * @return Boolean
+ */
+ function isSubPath($head, $root) {
+ ...
+ ...
+ ...
+ /**
+ * go to ./solution.php
+ */
+ }
+
+ // Helper function to match the linked list starting from the current tree node.
+ function dfs($head, $root) {
+ ...
+ ...
+ ...
+ /**
+ * go to ./solution.php
+ */
+ }
+}
+
+// Example usage:
+
+// Linked List: 4 -> 2 -> 8
+$head = new ListNode(4);
+$head->next = new ListNode(2);
+$head->next->next = new ListNode(8);
+
+// Binary Tree:
+// 1
+// / \
+// 4 4
+// \ \
+// 2 2
+// / \ / \
+// 1 6 8 8
+$root = new TreeNode(1);
+$root->left = new TreeNode(4);
+$root->right = new TreeNode(4);
+$root->left->right = new TreeNode(2);
+$root->right->left = new TreeNode(2);
+$root->left->right->left = new TreeNode(1);
+$root->left->right->right = new TreeNode(6);
+$root->right->left->right = new TreeNode(8);
+$root->right->left->right = new TreeNode(8);
+
+$solution = new Solution();
+$result = $solution->isSubPath($head, $root);
+echo $result ? "true" : "false"; // Output: true
+?>
+```
+
+### Explanation:
+
+1. **`isSubPath($head, $root)`**:
+ - This function recursively checks whether the linked list starting from `$head` corresponds to any downward path in the tree.
+ - It first checks if the current root node is the start of the list (by calling `dfs`).
+ - If not, it recursively searches the left and right subtrees.
+
+2. **`dfs($head, $root)`**:
+ - This helper function checks if the linked list matches the tree starting at the current tree node.
+ - If the list is fully traversed (`$head === null`), it returns `true`.
+ - If the tree node is `null` or values donβt match, it returns `false`.
+ - Otherwise, it continues to check the left and right children.
+
+### Example Execution:
+
+For input `head = [4,2,8]` and `root = [1,4,4,null,2,2,null,1,null,6,8]`, the algorithm will:
+- Start at the root (node 1), fail to match.
+- Move to the left child (node 4), match 4, then move down and match 2, then match 8, returning `true`.
+
+### Complexity:
+- **Time Complexity**: O(N * min(L, H)), where N is the number of nodes in the binary tree, L is the length of the linked list, and H is the height of the binary tree.
+- **Space Complexity**: O(H) due to the recursion depth of the binary tree.
+
+This solution efficiently checks for the subpath in the binary tree using DFS.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
\ No newline at end of file
diff --git a/algorithms/001367-linked-list-in-binary-tree/solution.php b/algorithms/001367-linked-list-in-binary-tree/solution.php
new file mode 100644
index 000000000..ca18647d4
--- /dev/null
+++ b/algorithms/001367-linked-list-in-binary-tree/solution.php
@@ -0,0 +1,64 @@
+val = $val;
+ * $this->next = $next;
+ * }
+ * }
+ */
+/**
+ * Definition for a binary tree node.
+ * class TreeNode {
+ * public $val = null;
+ * public $left = null;
+ * public $right = null;
+ * function __construct($val = 0, $left = null, $right = null) {
+ * $this->val = $val;
+ * $this->left = $left;
+ * $this->right = $right;
+ * }
+ * }
+ */
+class Solution {
+
+ /**
+ * @param ListNode $head
+ * @param TreeNode $root
+ * @return Boolean
+ */
+ function isSubPath($head, $root) {
+ // Base case: If root is null, no path can exist.
+ if ($root === null) {
+ return false;
+ }
+
+ // Check if the current node is the starting point of the linked list.
+ if ($this->dfs($head, $root)) {
+ return true;
+ }
+
+ // Otherwise, keep searching in the left and right subtrees.
+ return $this->isSubPath($head, $root->left) || $this->isSubPath($head, $root->right);
+ }
+
+ // Helper function to match the linked list starting from the current tree node.
+ function dfs($head, $root) {
+ // If the linked list is fully traversed, return true.
+ if ($head === null) {
+ return true;
+ }
+
+ // If the binary tree node is null or the values don't match, return false.
+ if ($root === null || $head->val !== $root->val) {
+ return false;
+ }
+
+ // Continue the search downwards in both left and right subtrees.
+ return $this->dfs($head->next, $root->left) || $this->dfs($head->next, $root->right);
+ }
+}
\ No newline at end of file
diff --git a/algorithms/001371-find-the-longest-substring-containing-vowels-in-even-counts/README.md b/algorithms/001371-find-the-longest-substring-containing-vowels-in-even-counts/README.md
new file mode 100644
index 000000000..92c8ddd01
--- /dev/null
+++ b/algorithms/001371-find-the-longest-substring-containing-vowels-in-even-counts/README.md
@@ -0,0 +1,124 @@
+1371\. Find the Longest Substring Containing Vowels in Even Counts
+
+**Difficulty:** Medium
+
+**Topics:** `Hash Table`, `String`, `Bit Manipulation`, `Prefix Sum`
+
+Given the string `s`, return _the size of the longest substring containing each vowel an even number of times_. That is, `'a'`, `'e'`, `'i'`, `'o'`, and `'u'` must appear an even number of times.
+
+**Example 1:**
+
+- **Input:** s = "eleetminicoworoep"
+- **Output:** 13
+- **Explanation:** The longest substring is "leetminicowor" which contains two each of the vowels: **e**, **i** and **o** and zero of the vowels: **a** and **u**.
+
+**Example 2:**
+
+- **Input:** s = "leetcodeisgreat"
+- **Output:** 5
+- **Explanation:** The longest substring is "leetc" which contains two **e**'s.
+
+
+**Example 3:**
+
+- **Input:** s = "bcbcbc"
+- **Output:** 6
+- **Explanation:** In this case, the given string "bcbcbc" is the longest because all vowels: **a**, **e**, **i**, **o** and **u** appear zero times.
+
+
+
+**Constraints:**
+
+- `1 <= s.length <= 5 x 10^5`
+- `s` contains only lowercase English letters.
+
+
+**Hint:**
+1. Represent the counts (odd or even) of vowels with a bitmask.
+2. Precompute the prefix xor for the bitmask of vowels and then get the longest valid substring.
+
+
+
+**Solution:**
+
+We can use **bit manipulation** to track the parity (even or odd) of vowels, along with a **hash table** to store prefix states. Here's how it can be approached:
+
+### Steps:
+1. **Bitmask Representation:** Since there are five vowels (`a, e, i, o, u`), we can use a 5-bit integer (bitmask) to represent whether the count of each vowel is odd or even. For each character:
+ - Bit `0` represents whether the count of 'a' is odd.
+ - Bit `1` represents whether the count of 'e' is odd.
+ - Bit `2` represents whether the count of 'i' is odd.
+ - Bit `3` represents whether the count of 'o' is odd.
+ - Bit `4` represents whether the count of 'u' is odd.
+
+ For example, a bitmask `00110` means `i` and `o` appear an odd number of times, while `a`, `e`, and `u` appear an even number of times.
+
+2. **Prefix Sum with Bitmask:** Traverse the string and keep track of the current bitmask state as we encounter each character. If we encounter a bitmask that has been seen before, it means the substring between the previous occurrence of this bitmask and the current index has an even number of vowels for each vowel.
+
+3. **Hash Table:** Store the first occurrence of each bitmask in a hash table (or associative array) to allow quick lookup.
+
+4. **Initialization:** The bitmask starts at 0 (all vowels have been seen an even number of times initially).
+
+5. **Edge Case:** If no vowels appear, the longest substring is the entire string since the count of vowels is trivially even (0 occurrences).
+
+Let's implement this solution in PHP: **[1371. Find the Longest Substring Containing Vowels in Even Counts](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/001371-find-the-longest-substring-containing-vowels-in-even-counts/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+1. **Bitmask Updates:**
+ - For each character in the string, if it's a vowel, its corresponding bit in the bitmask is flipped using XOR.
+
+2. **Hash Table (`$mask_position`):**
+ - This stores the first occurrence of each bitmask.
+ - If the same bitmask appears again, the substring between the two indices has an even count of vowels.
+
+3. **Iterating Through the String:**
+ - For each character, check the current bitmask.
+ - If the bitmask has been seen before, calculate the length of the substring.
+ - If not, store the index of this new bitmask.
+
+### Time Complexity:
+- **Time Complexity:** O(n), where n is the length of the string. We only pass through the string once and perform constant-time operations for each character.
+- **Space Complexity:** O(1) for the bitmask and O(n) for the hash table.
+
+### Example Walkthrough:
+
+For the input `"eleetminicoworoep"`:
+- The longest valid substring is `"leetminicowor"`, which contains `e, i, o` two times each, and `a, u` zero times.
+
+For the input `"leetcodeisgreat"`:
+- The longest valid substring is `"leetc"`, where the vowels `e` appear twice.
+
+For the input `"bcbcbc"`:
+- Since there are no vowels, the entire string is valid. Hence, the output is `6`.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
diff --git a/algorithms/001371-find-the-longest-substring-containing-vowels-in-even-counts/solution.php b/algorithms/001371-find-the-longest-substring-containing-vowels-in-even-counts/solution.php
new file mode 100644
index 000000000..ae1892816
--- /dev/null
+++ b/algorithms/001371-find-the-longest-substring-containing-vowels-in-even-counts/solution.php
@@ -0,0 +1,41 @@
+ 0, 'e' => 1, 'i' => 2, 'o' => 3, 'u' => 4];
+
+ // Initialize the hash table to store the first occurrence of each bitmask
+ // At mask = 0 (start), we have already seen it at index -1 (before any characters)
+ $mask_position = array();
+ $mask_position[0] = -1;
+
+ for ($i = 0; $i < $n; $i++) {
+ $ch = $s[$i];
+
+ // If the character is a vowel, flip its corresponding bit in the bitmask
+ if (isset($vowels[$ch])) {
+ $mask ^= (1 << $vowels[$ch]);
+ }
+
+ // Check if this bitmask has been seen before
+ if (isset($mask_position[$mask])) {
+ // Calculate the length of the substring from the previous occurrence
+ $longest = max($longest, $i - $mask_position[$mask]);
+ } else {
+ // Record the first time this bitmask is encountered
+ $mask_position[$mask] = $i;
+ }
+ }
+
+ return $longest;
+
+ }
+}
\ No newline at end of file
diff --git a/algorithms/001381-design-a-stack-with-increment-operation/README.md b/algorithms/001381-design-a-stack-with-increment-operation/README.md
new file mode 100644
index 000000000..12063abe8
--- /dev/null
+++ b/algorithms/001381-design-a-stack-with-increment-operation/README.md
@@ -0,0 +1,216 @@
+1381\. Design a Stack With Increment Operation
+
+**Difficulty:** Medium
+
+**Topics:** `Array`, `Stack`, `Design`
+
+Design a stack that supports increment operations on its elements.
+
+Implement the `CustomStack` class:
+
+- `CustomStack(int maxSize)` Initializes the object with `maxSize` which is the maximum number of elements in the stack.
+- `void push(int x)` Adds `x` to the top of the stack if the stack has not reached the `maxSize`.
+- `int pop()` Pops and returns _the top of the stack or `-1` if the stack is empty_.
+- `void inc(int k, int val)` Increments the bottom `k` elements of the stack by `val`. If there are less than `k` elements in the stack, increment all the elements in the stack.
+
+
+**Example 1:**
+
+- **Input:**
+```
+ ["CustomStack","push","push","pop","push","push","push","increment","increment","pop","pop","pop","pop"]
+ [[3],[1],[2],[],[2],[3],[4],[5,100],[2,100],[],[],[],[]]
+
+```
+- **Output:**
+```
+ [null,null,null,2,null,null,null,null,null,103,202,201,-1]
+
+```
+- **Explanation:**
+```
+ CustomStack stk = new CustomStack(3); // Stack is Empty []
+ stk.push(1); // stack becomes [1]
+ stk.push(2); // stack becomes [1, 2]
+ stk.pop(); // return 2 --> Return top of the stack 2, stack becomes [1]
+ stk.push(2); // stack becomes [1, 2]
+ stk.push(3); // stack becomes [1, 2, 3]
+ stk.push(4); // stack still [1, 2, 3], Do not add another elements as size is 4
+ stk.increment(5, 100); // stack becomes [101, 102, 103]
+ stk.increment(2, 100); // stack becomes [201, 202, 103]
+ stk.pop(); // return 103 --> Return top of the stack 103, stack becomes [201, 202]
+ stk.pop(); // return 202 --> Return top of the stack 202, stack becomes [201]
+ stk.pop(); // return 201 --> Return top of the stack 201, stack becomes []
+ stk.pop(); // return -1 --> Stack is empty return -1.
+
+```
+
+**Constraints:**
+
+- `1 <= maxSize, x, k <= 1000`
+- `0 <= val <= 100`
+- At most `1000` calls will be made to each method of `increment`, `push` and `pop` each separately.
+
+**Hint:**
+1. Use an array to represent the stack. Push will add new integer to the array. Pop removes the last element in the array and increment will add val to the first k elements of the array.
+2. This solution run in O(1) per push and pop and O(k) per increment.
+
+
+
+**Solution:**
+
+We can follow a typical stack implementation but with an additional method that allows incrementing the bottom `k` elements by a given value. The increment operation will iterate through the first `k` elements of the stack and add the value to each.
+
+We'll implement this stack in PHP 5.6, using an array to represent the stack. The core operations are:
+
+1. **push(x)**: Adds the element `x` to the top of the stack, if the stack has not reached its `maxSize`.
+2. **pop()**: Removes the top element of the stack and returns it. If the stack is empty, return `-1`.
+3. **increment(k, val)**: Adds the value `val` to the first `k` elements in the stack, or to all the elements if the stack contains fewer than `k` elements.
+
+Let's implement this solution in PHP: **[1381. Design a Stack With Increment Operation](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/001381-design-a-stack-with-increment-operation/solution.php)**
+
+```php
+push($x);
+ * $ret_2 = $obj->pop();
+ * $obj->increment($k, $val);
+ */
+
+// Example usage
+$customStack = new CustomStack(3); // Stack is Empty []
+$customStack->push(1); // stack becomes [1]
+$customStack->push(2); // stack becomes [1, 2]
+echo $customStack->pop() . "\n"; // return 2, stack becomes [1]
+$customStack->push(2); // stack becomes [1, 2]
+$customStack->push(3); // stack becomes [1, 2, 3]
+$customStack->push(4); // stack still [1, 2, 3], maxSize is 3
+$customStack->increment(5, 100); // stack becomes [101, 102, 103]
+$customStack->increment(2, 100); // stack becomes [201, 202, 103]
+echo $customStack->pop() . "\n"; // return 103, stack becomes [201, 202]
+echo $customStack->pop() . "\n"; // return 202, stack becomes [201]
+echo $customStack->pop() . "\n"; // return 201, stack becomes []
+echo $customStack->pop() . "\n"; // return -1, stack is empty
+?>
+```
+
+### Explanation:
+
+1. **push($x)**:
+ - We use `array_push` to add elements to the stack. We check if the current size of the stack is less than `maxSize`. If it is, we push the new element.
+
+2. **pop()**:
+ - We check if the stack is empty using `empty($this->stack)`. If it's not empty, we pop the top element using `array_pop` and return it. If it's empty, we return `-1`.
+
+3. **increment($k, $val)**:
+ - We calculate the minimum of `k` and the current stack size to determine how many elements to increment. We then loop over these elements, adding `val` to each.
+
+### Example Execution:
+
+For the input operations:
+
+```php
+["CustomStack","push","push","pop","push","push","push","increment","increment","pop","pop","pop","pop"]
+[[3],[1],[2],[],[2],[3],[4],[5,100],[2,100],[],[],[],[]]
+```
+
+The output will be:
+
+```
+[null, null, null, 2, null, null, null, null, null, 103, 202, 201, -1]
+```
+
+This output is based on the following:
+
+- **push(1)**: Stack becomes `[1]`
+- **push(2)**: Stack becomes `[1, 2]`
+- **pop()**: Returns `2`, stack becomes `[1]`
+- **push(2)**: Stack becomes `[1, 2]`
+- **push(3)**: Stack becomes `[1, 2, 3]`
+- **push(4)**: Stack remains `[1, 2, 3]` (maxSize reached)
+- **increment(5, 100)**: Stack becomes `[101, 102, 103]`
+- **increment(2, 100)**: Stack becomes `[201, 202, 103]`
+- **pop()**: Returns `103`, stack becomes `[201, 202]`
+- **pop()**: Returns `202`, stack becomes `[201]`
+- **pop()**: Returns `201`, stack becomes `[]`
+- **pop()**: Returns `-1` (stack is empty)
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
diff --git a/algorithms/001381-design-a-stack-with-increment-operation/solution.php b/algorithms/001381-design-a-stack-with-increment-operation/solution.php
new file mode 100644
index 000000000..65e926018
--- /dev/null
+++ b/algorithms/001381-design-a-stack-with-increment-operation/solution.php
@@ -0,0 +1,68 @@
+stack = array();
+ $this->maxSize = $maxSize;
+ }
+
+ /**
+ * Push an element to the stack if it has not reached the maxSize
+ *
+ * @param Integer $x
+ * @return NULL
+ */
+ function push($x) {
+ if (count($this->stack) < $this->maxSize) {
+ array_push($this->stack, $x);
+ }
+ }
+
+ /**
+ * Pop the top element from the stack and return it, return -1 if the stack is empty
+ *
+ * @return Integer
+ */
+ function pop() {
+ if (empty($this->stack)) {
+ return -1;
+ }
+ return array_pop($this->stack);
+ }
+
+ /**
+ * Increment the bottom k elements of the stack by val
+ *
+ * @param Integer $k
+ * @param Integer $val
+ * @return NULL
+ */
+ function increment($k, $val) {
+ // We will only iterate through the first `k` elements or the full stack if it's smaller than `k`
+ $limit = min($k, count($this->stack));
+ for ($i = 0; $i < $limit; $i++) {
+ $this->stack[$i] += $val;
+ }
+ }
+}
+
+/**
+ * Your CustomStack object will be instantiated and called as such:
+ * $obj = CustomStack($maxSize);
+ * $obj->push($x);
+ * $ret_2 = $obj->pop();
+ * $obj->increment($k, $val);
+ */
\ No newline at end of file
diff --git a/algorithms/001400-construct-k-palindrome-strings/README.md b/algorithms/001400-construct-k-palindrome-strings/README.md
new file mode 100644
index 000000000..a9ab6fa7e
--- /dev/null
+++ b/algorithms/001400-construct-k-palindrome-strings/README.md
@@ -0,0 +1,111 @@
+1400\. Construct K Palindrome Strings
+
+**Difficulty:** Medium
+
+**Topics:** `Hash Table`, `String`, `Greedy`, `Counting`
+
+Given a string `s` and an integer `k`, return _`true` if you can use all the characters in `s` to construct `k` palindrome strings or `false` otherwise_.
+
+**Example 1:**
+
+- **Input:** s = "annabelle", k = 2
+- **Output:** true
+- **Explanation:** You can construct two palindromes using all characters in s.
+ Some possible constructions "anna" + "elble", "anbna" + "elle", "anellena" + "b"
+
+**Example 2:**
+
+- **Input:** s = "leetcode", k = 3
+- **Output:** false
+- **Explanation:** It is impossible to construct 3 palindromes using all the characters of s.
+
+
+**Example 3:**
+
+- **Input:** s = "true", k = 4
+- **Output:** true
+- **Explanation:** The only possible solution is to put each character in a separate string.
+
+
+
+**Constraints:**
+
+- 1 <= s.length <= 105
+- `s` consists of lowercase English letters.
+- 1 <= k <= 105
+
+
+**Hint:**
+1. If the s.length < k we cannot construct k strings from s and answer is false.
+2. If the number of characters that have odd counts is > k then the minimum number of palindrome strings we can construct is > k and answer is false.
+3. Otherwise you can construct exactly k palindrome strings and answer is true (why ?).
+
+
+
+**Solution:**
+
+We need to consider the following points:
+
+### Key Observations:
+1. **Palindrome Characteristics**:
+ - A palindrome is a string that reads the same forwards and backwards.
+ - For an even-length palindrome, all characters must appear an even number of times.
+ - For an odd-length palindrome, all characters except one must appear an even number of times (the character that appears an odd number of times will be in the center).
+
+2. **Necessary Conditions**:
+ - If the length of `s` is less than `k`, it's impossible to form `k` strings, so return `false`.
+ - The total number of characters that appear an odd number of times must be at most `k` to form `k` palindromes. This is because each palindrome can have at most one character with an odd count (the center character for odd-length palindromes).
+
+### Approach:
+1. Count the frequency of each character in the string.
+2. Count how many characters have an odd frequency.
+3. If the number of odd frequencies exceeds `k`, return `false` (because it's impossible to form `k` palindromes).
+
+Let's implement this solution in PHP: **[1400. Construct K Palindrome Strings](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/001400-construct-k-palindrome-strings/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+1. **Frequency Count**: We use an associative array `$freq` to count the occurrences of each character in the string.
+2. **Odd Count**: We check how many characters have odd occurrences. This will help us determine if we can form palindromes.
+3. **Condition Check**: If the number of characters with odd frequencies is greater than `k`, it's impossible to form `k` palindromes, so we return `false`. Otherwise, we return `true`.
+
+### Time Complexity:
+- Counting the frequencies takes `O(n)`, where `n` is the length of the string.
+- Checking the odd frequencies takes `O(m)`, where `m` is the number of distinct characters (at most 26 for lowercase English letters).
+- The overall time complexity is `O(n + m)`, which simplifies to `O(n)` in this case.
+
+### Edge Cases:
+1. If `k` is greater than the length of `s`, we return `false`.
+2. If all characters have even frequencies, we can always form a palindrome, so the result depends on whether `k` is possible.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
diff --git a/algorithms/001400-construct-k-palindrome-strings/solution.php b/algorithms/001400-construct-k-palindrome-strings/solution.php
new file mode 100644
index 000000000..7863b4b7f
--- /dev/null
+++ b/algorithms/001400-construct-k-palindrome-strings/solution.php
@@ -0,0 +1,43 @@
+ $k) {
+ return false;
+ }
+
+ // Step 5: Otherwise, return true
+ return true;
+ }
+}
\ No newline at end of file
diff --git a/algorithms/001405-longest-happy-string/README.md b/algorithms/001405-longest-happy-string/README.md
new file mode 100644
index 000000000..85b6dd17e
--- /dev/null
+++ b/algorithms/001405-longest-happy-string/README.md
@@ -0,0 +1,119 @@
+1405\. Longest Happy String
+
+**Difficulty:** Medium
+
+**Topics:** `String`, `Greedy`, `Heap (Priority Queue)`
+
+A string `s` is called **happy** if it satisfies the following conditions:
+
+- `s` only contains the letters `'a'`, `'b'`, and `'c'`.
+- `s` does not contain any of `"aaa"`, `"bbb"`, or `"ccc"` as a substring.
+- `s` contains at most `a` occurrences of the letter `'a'`.
+- `s` contains at most `b` occurrences of the letter `'b'`.
+- `s contains at most `c` occurrences of the letter `'c'`.
+
+Given three integers `a`, `b`, and `c`, return _the **longest possible happy** string_. If there are multiple longest happy strings, return _any of them_. If there is no such string, return _the empty string `""`_.
+
+A **substring** is a contiguous sequence of characters within a string.
+
+**Example 1:**
+
+- **Input:** a = 1, b = 1, c = 7
+- **Output:** "ccaccbcc"
+- **Explanation:** "ccbccacc" would also be a correct answer.
+
+**Example 2:**
+
+- **Input:** a = 7, b = 1, c = 0
+- **Output:** "aabaa"
+- **Explanation:** It is the only correct answer in this case.
+
+
+**Constraints:**
+
+- `0 <= a, b, c <= 100`
+- `a + b + c > 0`
+
+
+**Hint:**
+1. Use a greedy approach.
+2. Use the letter with the maximum current limit that can be added without breaking the condition.
+
+
+
+**Solution:**
+
+We can employ a greedy algorithm. The approach is based on the following steps:
+
+1. **Use a Priority Queue (Max Heap)**:
+ - We can use a max heap to keep track of the characters and their remaining counts. This allows us to always choose the character with the highest remaining count while ensuring that we don't exceed two consecutive occurrences of the same character.
+
+2. **Construct the String**:
+ - Continuously extract the character with the highest count from the heap and append it to the result string.
+ - If the last two characters in the result string are the same, we cannot append the same character again. Instead, we will append the next character with the highest count.
+ - If we can append a character, we reduce its count and continue the process.
+
+3. **Return the Result**:
+ - If at any point we can't append a character without breaking the happy string conditions, we stop the process.
+
+Let's implement this solution in PHP: **[1405. Longest Happy String](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/001405-longest-happy-string/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+1. **Max Heap Creation**:
+ - We initialize an array `$maxHeap` with the counts of characters `'a'`, `'b'`, and `'c'`.
+
+2. **Sorting the Heap**:
+ - We use `usort` to sort the heap based on the remaining counts in descending order.
+
+3. **Building the Result String**:
+ - While there are characters in the heap:
+ - Sort the heap to ensure we always access the character with the highest count.
+ - If the last two characters in the result string are the same as the character with the highest count, we need to check if we can add a different character.
+ - If we can add the character, we decrement its count and continue.
+
+4. **Return**:
+ - Finally, we return the constructed happy string.
+
+### Complexity Analysis
+- **Time Complexity**: The solution runs in _**O(n log m)**_ where _**n**_ is the total number of characters we might output (i.e., _**a + b + c**_), and _**m**_ is the number of different characters (which is at most 3).
+- **Space Complexity**: The space complexity is _**O(1)**_ for the counts since we only keep a constant number of characters in the heap.
+
+This solution efficiently constructs the longest happy string while adhering to the constraints specified in the problem.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
\ No newline at end of file
diff --git a/algorithms/001405-longest-happy-string/solution.php b/algorithms/001405-longest-happy-string/solution.php
new file mode 100644
index 000000000..998f8ffea
--- /dev/null
+++ b/algorithms/001405-longest-happy-string/solution.php
@@ -0,0 +1,58 @@
+ 0) $maxHeap[] = ['char' => 'a', 'count' => $a];
+ if ($b > 0) $maxHeap[] = ['char' => 'b', 'count' => $b];
+ if ($c > 0) $maxHeap[] = ['char' => 'c', 'count' => $c];
+
+ // Sort the heap based on count (max heap)
+ usort($maxHeap, function($x, $y) {
+ return $y['count'] - $x['count'];
+ });
+
+ $result = '';
+
+ while (count($maxHeap) > 0) {
+ // Sort the heap to get the character with the highest count
+ usort($maxHeap, function($x, $y) {
+ return $y['count'] - $x['count'];
+ });
+
+ // Get the character with the highest count
+ $first = array_shift($maxHeap); // Most frequent character
+ if (strlen($result) >= 2 && $result[-1] == $first['char'] && $result[-2] == $first['char']) {
+ // We can't add this character because it would create 3 in a row
+ if (count($maxHeap) == 0) break; // No other character to add
+
+ // Get the second character
+ $second = array_shift($maxHeap);
+ $result .= $second['char'];
+ $second['count']--;
+ if ($second['count'] > 0) {
+ $maxHeap[] = $second; // Add it back if there's more left
+ }
+ // Put the first character back
+ $maxHeap[] = $first; // Add the first character back
+ } else {
+ // We can add the first character
+ $result .= $first['char'];
+ $first['count']--;
+ if ($first['count'] > 0) {
+ $maxHeap[] = $first; // Add it back if there's more left
+ }
+ }
+ }
+
+ return $result;
+ }
+}
\ No newline at end of file
diff --git a/algorithms/001408-string-matching-in-an-array/README.md b/algorithms/001408-string-matching-in-an-array/README.md
new file mode 100644
index 000000000..1e57007a4
--- /dev/null
+++ b/algorithms/001408-string-matching-in-an-array/README.md
@@ -0,0 +1,128 @@
+1408\. String Matching in an Array
+
+**Difficulty:** Easy
+
+**Topics:** `Array`, `String`, `String Matching`
+
+Given an array of string `words`, return _all strings in `words` that is a **substring** of another word_. You can return the answer in **any order**.
+
+A **substring** is a contiguous sequence of characters within a string
+
+**Example 1:**
+
+- **Input:** words = ["mass","as","hero","superhero"]
+- **Output:** ["as","hero"]
+- **Explanation:** "as" is substring of "mass" and "hero" is substring of "superhero".
+ ["hero","as"] is also a valid answer.
+
+**Example 2:**
+
+- **Input:** words = ["leetcode","et","code"]
+- **Output:** ["et","code"]
+- **Explanation:** "et", "code" are substring of "leetcode".
+
+
+**Example 3:**
+
+- **Input:** words = ["blue","green","bu"]
+- **Output:** []
+- **Explanation:** No string of words is substring of another string.
+
+
+
+**Constraints:**
+
+- `1 <= words.length <= 100`
+- `1 <= words[i].length <= 30`
+- `words[i]` contains only lowercase English letters.
+- All the strings of `words` are **unique**.
+
+
+**Hint:**
+1. Bruteforce to find if one string is substring of another or use KMP algorithm.
+
+
+
+**Solution:**
+
+We need to find all strings in the `words` array that are substrings of another word in the array, you can use a brute force approach. The approach involves checking each string in the list and verifying if it's a substring of any other string.
+
+Let's implement this solution in PHP: **[1408. String Matching in an Array](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/001408-string-matching-in-an-array/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+1. The function `stringMatching` loops through all the words in the input array.
+2. For each word, it compares it with every other word in the array using a nested loop.
+3. It uses PHP's `strpos()` function to check if one string is a substring of another. The `strpos()` function returns `false` if the substring is not found.
+4. If a substring is found, we add the word to the result array and break out of the inner loop, as we only need to record the word once.
+5. Finally, the function returns the result array containing all the substrings.
+
+### Time Complexity:
+- The time complexity is _**O(n2 x m)**_, where _**n**_ is the number of words and _**m**_ is the maximum length of a word. This is because we are performing a substring search for each word within every other word.
+
+### Example Outputs:
+
+For input `["mass", "as", "hero", "superhero"]`, the output will be:
+```php
+Array
+(
+ [0] => as
+ [1] => hero
+)
+```
+
+For input `["leetcode", "et", "code"]`, the output will be:
+```php
+Array
+(
+ [0] => et
+ [1] => code
+)
+```
+
+For input `["blue", "green", "bu"]`, the output will be:
+```php
+Array
+(
+)
+```
+
+This solution works well for the given problem constraints.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
diff --git a/algorithms/001408-string-matching-in-an-array/solution.php b/algorithms/001408-string-matching-in-an-array/solution.php
new file mode 100644
index 000000000..26c0f9bb8
--- /dev/null
+++ b/algorithms/001408-string-matching-in-an-array/solution.php
@@ -0,0 +1,30 @@
+
+```
+
+### Explanation:
+
+1. **Prefix sum computation**: We compute the prefix sum of ones in the array `$prefixOneCount`, where each index holds the cumulative count of ones up to that point.
+
+2. **Iterating over possible splits**: We start iterating through each index `i` (from 0 to `n-2`), where the string is split into a left part (from 0 to `i`) and a right part (from `i+1` to `n-1`).
+ - For each split, count the zeros in the left substring (`$zeroCountLeft`).
+ - Use the precomputed `$prefixOneCount` to calculate how many ones are in the right substring.
+
+3. **Score calculation**: The score for each split is calculated as the sum of zeros in the left part and ones in the right part. We update the maximum score encountered during this iteration.
+
+4. **Final result**: The function returns the maximum score found during all splits.
+
+### Complexity:
+
+- **Time Complexity**: _**O(n)**_
+ - Precomputing prefix sums and iterating through the string both take _**O(n)**_.
+ - Iterating through the string to compute scores also takes O(n).
+ Thus, the total time complexity is O(n), which is efficient for the given input size (`n β€ 500`).
+
+- **Space Complexity**: _**O(n)**_
+ - The prefix sum array requires _**O(n)**_ additional space.
+
+### Example:
+
+```php
+echo maxScore("011101"); // Output: 5
+echo maxScore("00111"); // Output: 5
+echo maxScore("1111"); // Output: 3
+```
+
+This solution is optimal and handles the problem within the constraints.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
diff --git a/algorithms/001422-maximum-score-after-splitting-a-string/solution.php b/algorithms/001422-maximum-score-after-splitting-a-string/solution.php
new file mode 100644
index 000000000..cb75ed648
--- /dev/null
+++ b/algorithms/001422-maximum-score-after-splitting-a-string/solution.php
@@ -0,0 +1,42 @@
+
+```
+
+### Explanation:
+
+1. **Splitting the Sentence into Words**:
+ We use `explode(" ", $sentence)` to split the sentence into an array of words.
+
+2. **Iterating Over Words**:
+ Use a `foreach` loop to iterate through each word in the sentence. The `$index` variable keeps track of the position of the word (0-indexed).
+
+3. **Checking for Prefix**:
+ Use `strpos($word, $searchWord) === 0` to check if the `searchWord` occurs at the start of the current word.
+
+4. **Returning the Result**:
+ If a match is found, return the 1-based index of the word by adding 1 to `$index`. If no match is found after the loop, return `-1`.
+
+### Example Outputs:
+- For the input `sentence = "i love eating burger"` and `searchWord = "burg"`, the output is `4` because `"burger"` is the 4th word.
+- For the input `sentence = "this problem is an easy problem"` and `searchWord = "pro"`, the output is `2` because `"problem"` is the 2nd word.
+- For the input `sentence = "i am tired"` and `searchWord = "you"`, the output is `-1` because no word starts with `"you"`.
+
+### Time Complexity:
+- Splitting the sentence into words takes O(n), where `n` is the length of the sentence.
+- Checking each word for a prefix takes O(m), where `m` is the length of the `searchWord`.
+- Thus, the overall time complexity is O(n * m), which is efficient for the input size constraints.
+
+This solution satisfies the constraints and is efficient for the given input size.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
diff --git a/algorithms/001455-check-if-a-word-occurs-as-a-prefix-of-any-word-in-a-sentence/solution.php b/algorithms/001455-check-if-a-word-occurs-as-a-prefix-of-any-word-in-a-sentence/solution.php
new file mode 100644
index 000000000..21baca282
--- /dev/null
+++ b/algorithms/001455-check-if-a-word-occurs-as-a-prefix-of-any-word-in-a-sentence/solution.php
@@ -0,0 +1,26 @@
+ $word) {
+ // Check if searchWord is a prefix of the word
+ if (substr($word, 0, strlen($searchWord)) === $searchWord) {
+ // Return the 1-indexed position
+ return $index + 1;
+ }
+ }
+
+ // If no word contains searchWord as a prefix, return -1
+ return -1;
+ }
+}
\ No newline at end of file
diff --git a/algorithms/001475-final-prices-with-a-special-discount-in-a-shop/README.md b/algorithms/001475-final-prices-with-a-special-discount-in-a-shop/README.md
new file mode 100644
index 000000000..68f2f4f57
--- /dev/null
+++ b/algorithms/001475-final-prices-with-a-special-discount-in-a-shop/README.md
@@ -0,0 +1,124 @@
+1475\. Final Prices With a Special Discount in a Shop
+
+**Difficulty:** Easy
+
+**Topics:** `Array`, `Stack`, `Monotonic Stack`
+
+You are given an integer array `prices` where `prices[i]` is the price of the ith
item in a shop.
+
+There is a special discount for items in the shop. If you buy the ith
item, then you will receive a discount equivalent to `prices[j]` where `j` is the minimum index such that `j > i` and `prices[j] <= prices[i]`. Otherwise, you will not receive any discount at all.
+
+Return an integer array `answer` where `answer[i]` is the final price you will pay for the ith
item of the shop, considering the special discount.
+
+**Example 1:**
+
+- **Input:** prices = [8,4,6,2,3]
+- **Output:** [4,2,4,2,3]
+- **Explanation:**
+ - For item 0 with price[0]=8 you will receive a discount equivalent to prices[1]=4, therefore, the final price you will pay is 8 - 4 = 4.
+ - For item 1 with price[1]=4 you will receive a discount equivalent to prices[3]=2, therefore, the final price you will pay is 4 - 2 = 2.
+ - For item 2 with price[2]=6 you will receive a discount equivalent to prices[3]=2, therefore, the final price you will pay is 6 - 2 = 4.
+ - For items 3 and 4 you will not receive any discount at all.
+
+**Example 2:**
+
+- **Input:** prices = [1,2,3,4,5]
+- **Output:** [1,2,3,4,5]
+- **Explanation:** In this case, for all items, you will not receive any discount at all.
+
+
+**Example 3:**
+
+- **Input:** prices = [10,1,1,6]
+- **Output:** [9,0,1,6]
+
+
+
+**Constraints:**
+
+- `1 <= prices.length <= 500`
+- `1 <= prices[i] <= 1000`
+
+
+**Hint:**
+1. Use brute force: For the ith item in the shop with a loop find the first position j satisfying the conditions and apply the discount, otherwise, the discount is 0.
+
+
+
+**Solution:**
+
+We need to apply a special discount based on the condition that there is a later item with a price less than or equal to the current price, we can use a brute-force approach. We'll iterate over the `prices` array and, for each item, look for the first item with a lower or equal price after it. This can be achieved with nested loops. We can utilize a stack to efficiently keep track of the items' prices and apply the special discount.
+
+### Approach:
+1. **Stack Approach**:
+ - We can iterate over the prices array from left to right. For each item, we'll use the stack to keep track of prices that haven't yet found a discount.
+ - For each price, we'll check if it is smaller than or equal to the price at the top of the stack. If so, it means that we can apply a discount.
+ - The stack will store the indices of the items, and for each item, we will check if the current price is greater than the price at the index in the stack, meaning there is no discount. Otherwise, apply the discount by subtracting the corresponding price from the current price.
+
+2. **Edge Case**: If no item has a smaller price later in the array, no discount is applied.
+
+Let's implement this solution in PHP: **[1475. Final Prices With a Special Discount in a Shop](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/001475-final-prices-with-a-special-discount-in-a-shop/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+1. **Initialization**:
+ - Create an array `$result` of the same size as `$prices` and initialize it to `0`.
+
+2. **Outer Loop**:
+ - Loop through each price at index `$i` to calculate its final price after discounts.
+
+3. **Inner Loop**:
+ - For each price `$i`, iterate through the subsequent prices `$j` (where `$j > $i`).
+ - Check if `$prices[$j]` is less than or equal to `$prices[$i]`. If true, set `$discount = $prices[$j]` and exit the inner loop.
+
+4. **Final Price Calculation**:
+ - Subtract the found discount from `$prices[$i]` and store the result in `$result[$i]`.
+
+5. **Return Result**:
+ - After processing all prices, return the final result array.
+
+### Complexity:
+- **Time Complexity**: _**O(nΒ²)**_ (due to the nested loops for each price).
+- **Space Complexity**: _**O(n)**_ (for the result array).
+
+### Example Outputs:
+- For `prices = [8, 4, 6, 2, 3]`, the output is `[4, 2, 4, 2, 3]`.
+- For `prices = [1, 2, 3, 4, 5]`, the output is `[1, 2, 3, 4, 5]`.
+- For `prices = [10, 1, 1, 6]`, the output is `[9, 0, 1, 6]`.
+
+This approach will work within the constraints of the problem (`1 <= prices.length <= 500`), even though it is not the most optimized solution.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
diff --git a/algorithms/001475-final-prices-with-a-special-discount-in-a-shop/solution.php b/algorithms/001475-final-prices-with-a-special-discount-in-a-shop/solution.php
new file mode 100644
index 000000000..87e8c58b9
--- /dev/null
+++ b/algorithms/001475-final-prices-with-a-special-discount-in-a-shop/solution.php
@@ -0,0 +1,25 @@
+= $prices[$i]) {
+ $index = array_pop($stack); // Pop the index from the stack
+ $answer[$index] = $prices[$index] - $prices[$i]; // Apply the discount
+ }
+ array_push($stack, $i); // Push the current index onto the stack
+ }
+
+ return $answer;
+ }
+}
\ No newline at end of file
diff --git a/algorithms/001497-check-if-array-pairs-are-divisible-by-k/README.md b/algorithms/001497-check-if-array-pairs-are-divisible-by-k/README.md
new file mode 100644
index 000000000..ee85a16e7
--- /dev/null
+++ b/algorithms/001497-check-if-array-pairs-are-divisible-by-k/README.md
@@ -0,0 +1,130 @@
+1497\. Check If Array Pairs Are Divisible by k
+
+**Difficulty:** Medium
+
+**Topics:** `Array`, `Hash Table`, `Counting`
+
+Given an array of integers `arr` of even length `n` and an integer `k`.
+
+We want to divide the array into exactly `n / 2` pairs such that the sum of each pair is divisible by `k`.
+
+Return _`true` If you can find a way to do that or `false` otherwise_.
+
+**Example 1:**
+
+- **Input:** arr = [1,2,3,4,5,10,6,7,8,9], k = 5
+- **Output:** true
+- **Explanation:** Pairs are (1,9),(2,8),(3,7),(4,6) and (5,10).
+
+**Example 2:**
+
+- **Input:** arr = [1,2,3,4,5,6], k = 7
+- **Output:** true
+- **Explanation:** Pairs are (1,6),(2,5) and(3,4).
+
+
+**Example 3:**
+
+- **Input:** arr = [1,2,3,4,5,6], k = 10
+- **Output:** false
+- **Explanation:** You can try all possible pairs to see that there is no way to divide arr into 3 pairs each with sum divisible by 10.
+
+**Constraints:**
+
+- `arr.length == n`
+- 1 <= n <= 105
+- `n` is even.
+- -109 <= arr[i] <= 109
+- 1 <= k <= 105
+
+**Hint:**
+1. Keep an array of the frequencies of ((x % k) + k) % k for each x in arr.
+2. for each i in [0, k - 1] we need to check if freq[i] == freq[k - i]
+3. Take care of the case when i == k - i and when i == 0
+
+
+
+**Solution:**
+
+We need to ensure that the array can be divided into pairs where the sum of each pair is divisible by `k`. To do this efficiently, we'll use a frequency count of the remainders of elements when divided by `k`.
+
+### Key Idea:
+
+For two numbers `a` and `b`, their sum is divisible by `k` if: `(a + b) % k == 0`
+
+This is equivalent to: `(a % k + b % k) % k == 0`
+
+This means for each number `x` in the array, if `x % k = r`, we need to pair it with another number `y` such that `y % k = k - r`.
+
+### Steps to solve:
+1. **Compute remainders**: For each element in the array, calculate its remainder when divided by `k`. This will give us the needed pairing information.
+2. **Frequency count of remainders**: Keep track of how many elements have each remainder.
+3. **Check pairing conditions**:
+ - For remainder `0`, the number of such elements must be even because they can only pair with each other.
+ - For each remainder `r`, we need the same number of elements with remainder `k - r` for pairing.
+ - Special case: when `r == k/2`, the number of such elements must also be even because they need to pair among themselves.
+
+Let's implement this solution in PHP: **[1497. Check If Array Pairs Are Divisible by k](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/001497-check-if-array-pairs-are-divisible-by-k/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+1. **Modulo Operation**: To account for negative numbers, we use this formula to always get a positive remainder:
+ ```php
+ ($num % $k + $k) % $k;
+ ```
+ This ensures that even negative numbers are handled properly.
+
+2. **Frequency Array**: We create an array `freq` of size `k` where `freq[i]` counts how many numbers have a remainder `i` when divided by `k`.
+
+3. **Conditions**:
+ - For each remainder `i`, we need to check if the count of numbers with remainder `i` is the same as the count of numbers with remainder `k - i`.
+ - Special cases:
+ - `i = 0`: The number of elements with remainder 0 must be even, as they need to pair with each other.
+ - `i = k/2`: If `k` is even, then elements with remainder `k/2` must also be even, as they can only pair with themselves.
+
+### Time Complexity:
+- The time complexity is O(n) where `n` is the length of the array because we iterate over the array once to calculate remainders and once again to check the pairing conditions.
+
+This solution efficiently checks whether it's possible to divide the array into pairs such that each pair's sum is divisible by `k`.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
diff --git a/algorithms/001497-check-if-array-pairs-are-divisible-by-k/solution.php b/algorithms/001497-check-if-array-pairs-are-divisible-by-k/solution.php
new file mode 100644
index 000000000..c890fdf34
--- /dev/null
+++ b/algorithms/001497-check-if-array-pairs-are-divisible-by-k/solution.php
@@ -0,0 +1,39 @@
+2 <= n <= 10^4
+- 0 <= start, end < n
+- start != end
+- 0 <= a, b < n
+- a != b
+- 0 <= succProb.length == edges.length <= 2*10^4
+- 0 <= succProb[i] <= 1
+- There is at most one edge between every two nodes
+
+
+**Hint:**
+1. Multiplying probabilities will result in precision errors.
+2. Take log probabilities to sum up numbers instead of multiplying them.
+3. Use Dijkstra's algorithm to find the minimum path between the two nodes after negating all costs.
+
+
+**Solution:**
+
+
+We can use a modified version of Dijkstra's algorithm. Instead of finding the shortest path, you'll be maximizing the probability of success.
+
+Let's implement this solution in PHP: **[1514\. Path with Maximum Probability](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/001514-path-with-maximum-probability/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+1. **Graph Representation**: The graph is represented as an adjacency list where each node points to its neighbors along with the success probabilities of the edges connecting them.
+
+2. **Max Probability Array**: An array `maxProb` is used to store the maximum probability to reach each node from the start node.
+
+3. **Priority Queue**: A max heap (`SplPriorityQueue`) is used to explore paths with the highest probability first. This is crucial to ensure that when we reach the destination node, we've found the path with the maximum probability.
+
+4. **Algorithm**:
+ - Initialize the start node's probability as 1 (since the probability of staying at the start is 1).
+ - Use the priority queue to explore nodes, updating the maximum probability to reach each neighbor.
+ - If the destination node is reached, return the probability.
+ - If no path exists, return 0.
+
+### Output:
+For the example provided:
+```php
+$n = 3;
+$edges = [[0,1],[1,2],[0,2]];
+$succProb = [0.5,0.5,0.2];
+$start_node = 0;
+$end_node = 2;
+```
+The output will be `0.25`.
+
+This approach ensures an efficient solution using Dijkstra's algorithm while handling the specifics of probability calculations.
+
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
+
diff --git a/algorithms/001514-path-with-maximum-probability/solution.php b/algorithms/001514-path-with-maximum-probability/solution.php
new file mode 100644
index 000000000..2d59dbc1c
--- /dev/null
+++ b/algorithms/001514-path-with-maximum-probability/solution.php
@@ -0,0 +1,54 @@
+ $edge) {
+ list($a, $b) = $edge;
+ $graph[$a][] = [$b, $succProb[$i]];
+ $graph[$b][] = [$a, $succProb[$i]];
+ }
+
+ // Initialize the maximum probabilities array
+ $maxProb = array_fill(0, $n, 0.0);
+ $maxProb[$start_node] = 1.0;
+
+ // Use a max heap (priority queue) to explore the paths
+ $pq = new SplPriorityQueue();
+ $pq->insert([$start_node, 1.0], 1.0);
+
+ // Dijkstra's algorithm to find the path with maximum probability
+ while (!$pq->isEmpty()) {
+ list($node, $prob) = $pq->extract();
+
+ // If we reached the end node, return the probability
+ if ($node == $end_node) {
+ return $prob;
+ }
+
+ // Explore the neighbors
+ foreach ($graph[$node] as $neighbor) {
+ list($nextNode, $edgeProb) = $neighbor;
+ $newProb = $prob * $edgeProb;
+ if ($newProb > $maxProb[$nextNode]) {
+ $maxProb[$nextNode] = $newProb;
+ $pq->insert([$nextNode, $newProb], $newProb);
+ }
+ }
+ }
+
+ // If no path was found, return 0
+ return 0.0;
+
+ }
+}
\ No newline at end of file
diff --git a/algorithms/001545-find-kth-bit-in-nth-binary-string/README.md b/algorithms/001545-find-kth-bit-in-nth-binary-string/README.md
new file mode 100644
index 000000000..234567a9e
--- /dev/null
+++ b/algorithms/001545-find-kth-bit-in-nth-binary-string/README.md
@@ -0,0 +1,148 @@
+1545\. Find Kth Bit in Nth Binary String
+
+**Difficulty:** Medium
+
+**Topics:** `String`, `Recursion`, `Simulation`
+
+Given two positive integers `n` and `k`, the binary string Sn
is formed as follows:
+
+- S1 = "0"
+- Si = Si - 1 + "1" + reverse(invert(Si - 1))
for `i > 1`
+
+Where `+` denotes the concatenation operation, `reverse(x)` returns the reversed string `x`, and `invert(x)` inverts all the bits in `x` (`0` changes to `1` and `1` changes to `0`).
+
+For example, the first four strings in the above sequence are:
+
+- S1 = "0"
+- S2 = "011"
+- S3 = "0111001"
+- S4 = "011100110110001"
+
+Return _the kth
bit in Sn
_. It is guaranteed that `k` is valid for the given `n`.
+
+**Example 1:**
+
+- **Input:** n = 3, k = 1
+- **Output:** "0"
+- **Explanation:** S3 is "**0**111001".\
+ The 1st bit is "0".
+
+**Example 2:**
+
+- **Input:** n = 4, k = 11
+- **Output:** "1"
+- **Explanation:** S4 is "0111001101**1**0001".
+ The 11th bit is "1".
+
+**Example 3:**
+
+- **Input:** n = 3, k = 2
+- **Output:** "0"
+
+
+
+**Constraints:**
+
+- `1 <= n <= 20`
+- 1 <= k <= 2n - 1
+
+
+**Hint:**
+1. Since n is small, we can simply simulate the process of constructing S1 to Sn.
+
+
+
+**Solution:**
+
+We need to understand the recursive process used to generate each binary string _Sn_
and use this to determine the _`k`_-th bit without constructing the entire string.
+
+### Approach:
+
+1. **Recursive String Construction**:
+ - **S1 = "0"**.
+ - For `i > 1`:
+ - **Si**
is constructed as:
+
+ **Si = Si-1 + "1" + reverse(invert(Si-1))**
+
+ - This means _**Si**_ consists of three parts:
+ - _**Si-1**_ (the original part)
+ - "1" (the middle bit)
+ - **reverse(invert(_Si-1_))** (the transformed part)
+
+2. **Key Observations**:
+ - _**Si**_ has a length of _**2i-1**_.
+ - The middle bit (position _**2i-1**_ of _**Si**_ is always "1".
+ - If _**k**_ lies in the first half, it falls within _**Si-1**_.
+ - If _**k**_ is exactly the middle position, the answer is "1".
+ - If _**k**_ is in the second half, it corresponds to the reverse-inverted part.
+
+3. **Inverting and Reversing**:
+ - To determine the bit in the second half, map _**k**_ to its corresponding position in the first half using:
+
+ _k' = 2i - k_
+
+ - The bit at this position in the original half is inverted, so we need to flip the result.
+
+4. **Recursive Solution**:
+ - Recursively determine the _**k**_-th bit by reducing _**n**_ and mapping _**k**_ until _**n = 1**_.
+
+Let's implement this solution in PHP: **[1545. Find Kth Bit in Nth Binary String](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/001545-find-kth-bit-in-nth-binary-string/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+- **Base Case**: If _**n = 1**_, _**Si**_ is "0", so the only possible value for any _**k**_ is "0".
+- **Recursive Steps**:
+ - Calculate the middle index _**mid**_ which is _**2n-1**_.
+ - If _**k**_ matches the middle index, return "1".
+ - If _**k**_ is less than _**mid**_, the _**k**_-th bit lies in the first half, so we recursively find the _**k**_-th bit in _**Sn-1**_.
+ - If _**k**_ is greater than _**mid**_, we find the corresponding bit in the reverse-inverted second half and flip it.
+
+### Complexity Analysis:
+
+- **Time Complexity**: _**O(n)**_ because each recursive call reduces _**n**_ by 1.
+- **Space Complexity**: _**O(n)**_ for the recursive call stack.
+
+### Example Walkthrough:
+
+1. **Input**: _**n = 3**_ , _**k = 1**_
+ - _**S3**_ = **"0111001"**
+ - _**k = 1**_ falls in the first half, so we look for _**k = 1**_ in _**S2**_.
+ - In _**S2**_ = **"011"** , _**k = 1**_ corresponds to "0".
+
+2. **Input**: _**n = 4**_ , _**k = 11**_
+ - _**S4**_ = **"011100110110001"**
+ - The middle index for _**S4**_ is **8**.
+ - _**k = 11**_ falls in the second half.
+ - Map _**k = 11**_ to the corresponding bit in the first half: _**k'**_ **= 24 - 11 = 5**.
+ - Find _**k = 5**_ in _**S3**_ , which is "0", then flip it to "1".
+
+By leveraging recursion and properties of the string construction, this solution avoids generating the entire string, making it efficient even for larger _**n**_.
+
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
diff --git a/algorithms/001545-find-kth-bit-in-nth-binary-string/solution.php b/algorithms/001545-find-kth-bit-in-nth-binary-string/solution.php
new file mode 100644
index 000000000..c3fa8b9bb
--- /dev/null
+++ b/algorithms/001545-find-kth-bit-in-nth-binary-string/solution.php
@@ -0,0 +1,26 @@
+findKthBit($n - 1, $k);
+ } else {
+ $result = $this->findKthBit($n - 1, $mid * 2 - $k);
+ return $result === "0" ? "1" : "0"; // Flip the bit
+ }
+ }
+}
\ No newline at end of file
diff --git a/algorithms/001568-minimum-number-of-days-to-disconnect-island/README.md b/algorithms/001568-minimum-number-of-days-to-disconnect-island/README.md
new file mode 100644
index 000000000..c225f43c1
--- /dev/null
+++ b/algorithms/001568-minimum-number-of-days-to-disconnect-island/README.md
@@ -0,0 +1,95 @@
+1568\. Minimum Number of Days to Disconnect Island
+
+
+**Difficulty:** Hard
+
+**Topics:** `Array`, `Depth-First Search`, `Breadth-First Search`, `Matrix`, `Strongly Connected Component`
+
+You are given an `m x n` binary grid `grid` where `1` represents land and `0` represents water. An **island** is a maximal **4-directionally** (horizontal or vertical) connected group of `1`'s.
+
+The grid is said to be **connected** if we have **exactly one island**, otherwise is said **disconnected**.
+
+In one day, we are allowed to change a****ny single land cell `(1)` into a water cell `(0)`.
+
+Return _the minimum number of days to disconnect the grid_.
+
+**Example 1:**
+
+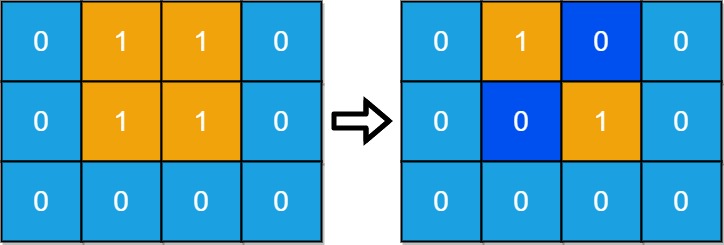
+
+- **Input:** grid = [[0,1,1,0],[0,1,1,0],[0,0,0,0]]
+- **Output:** 2
+- **Explanation:** We need at least 2 days to get a disconnected grid.\
+ Change land `grid[1][1]` and `grid[0][2]` to water and get 2 disconnected island.
+
+**Example 2:**
+
+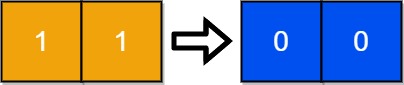
+
+- **Input:** grid = [[1,1]]
+- **Output:** 2
+- **Explanation:** Grid of full water is also disconnected `([[1,1]] -> [[0,0]])`, `0` islands.
+
+**Constraints:**
+
+- `m == grid.length`
+- `n == grid[i].length`
+- `1 <= m, n <= 30`
+- `grid[i][j]` is either `0` or `1`.
+
+**Hint:**
+1. Return 0 if the grid is already disconnected.
+2. Return 1 if changing a single land to water disconnect the island.
+3. Otherwise return 2.
+4. We can disconnect the grid within at most 2 days.
+
+
+**Solution:**
+
+We need to consider the following steps:
+
+### Steps to Solve the Problem:
+1. **Check Initial Connectivity**: First, check if the grid is already disconnected by determining if there is more than one island in the grid. If it's already disconnected, return `0`.
+
+2. **Check If Single Removal Disconnects the Island**: Iterate through each cell of the grid. Temporarily convert the cell from `1` to `0` (if it's `1`) and check if the grid becomes disconnected by counting the number of islands. If converting a single cell disconnects the island, return `1`.
+
+3. **Two Day Disconnection**: If no single cell conversion disconnects the island, then the grid can be disconnected by converting any two adjacent land cells. Therefore, return `2`.
+
+### Key Functions:
+- **DFS (Depth-First Search)** to find and count islands.
+- **isConnected** to check if the grid is connected.
+
+Let's implement this solution in PHP: **[1568. Minimum Number of Days to Disconnect Island](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/001568-minimum-number-of-days-to-disconnect-island/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+- **`minDays()`** function handles the main logic.
+- **`countIslands()`** counts how many islands are present using DFS.
+- **`dfs()`** is the recursive function to explore the grid and mark visited land cells.
+
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
diff --git a/algorithms/001568-minimum-number-of-days-to-disconnect-island/solution.php b/algorithms/001568-minimum-number-of-days-to-disconnect-island/solution.php
new file mode 100644
index 000000000..eba0d6b71
--- /dev/null
+++ b/algorithms/001568-minimum-number-of-days-to-disconnect-island/solution.php
@@ -0,0 +1,81 @@
+countIslands($grid) != 1) {
+ return 0;
+ }
+
+ // Check if a single land removal disconnects the grid
+ for ($i = 0; $i < $m; $i++) {
+ for ($j = 0; $j < $n; $j++) {
+ if ($grid[$i][$j] == 1) {
+ $grid[$i][$j] = 0; // Temporarily convert to water
+ if ($this->countIslands($grid) != 1) {
+ return 1;
+ }
+ $grid[$i][$j] = 1; // Revert back to land
+ }
+ }
+ }
+
+ // Otherwise, it will take 2 days to disconnect the grid
+ return 2;
+ }
+
+ /**
+ * @param $grid
+ * @return int
+ */
+ function countIslands(&$grid) {
+ $m = count($grid);
+ $n = count($grid[0]);
+ $visited = array_fill(0, $m, array_fill(0, $n, false));
+ $islandCount = 0;
+
+ for ($i = 0; $i < $m; $i++) {
+ for ($j = 0; $j < $n; $j++) {
+ if ($grid[$i][$j] == 1 && !$visited[$i][$j]) {
+ $islandCount++;
+ $this->dfs($grid, $i, $j, $visited);
+ }
+ }
+ }
+
+ return $islandCount;
+ }
+
+ /**
+ * @param $grid
+ * @param $i
+ * @param $j
+ * @param $visited
+ * @return void
+ */
+ function dfs(&$grid, $i, $j, &$visited) {
+ $m = count($grid);
+ $n = count($grid[0]);
+ $directions = [[0, 1], [1, 0], [0, -1], [-1, 0]];
+
+ $visited[$i][$j] = true;
+
+ foreach ($directions as $dir) {
+ $ni = $i + $dir[0];
+ $nj = $j + $dir[1];
+
+ if ($ni >= 0 && $nj >= 0 && $ni < $m && $nj < $n && $grid[$ni][$nj] == 1 && !$visited[$ni][$nj]) {
+ self::dfs($grid, $ni, $nj, $visited);
+ }
+ }
+ }
+
+}
\ No newline at end of file
diff --git a/algorithms/001574-shortest-subarray-to-be-removed-to-make-array-sorted/README.md b/algorithms/001574-shortest-subarray-to-be-removed-to-make-array-sorted/README.md
new file mode 100644
index 000000000..dc0bddb73
--- /dev/null
+++ b/algorithms/001574-shortest-subarray-to-be-removed-to-make-array-sorted/README.md
@@ -0,0 +1,121 @@
+1574\. Shortest Subarray to be Removed to Make Array Sorted
+
+**Difficulty:** Medium
+
+**Topics:** `Array`, `Two Pointers`, `Binary Search`, `Stack`, `Monotonic Stack`
+
+Given an integer array `arr`, remove a subarray (can be empty) from `arr` such that the remaining elements in `arr` are **non-decreasing**.
+
+Return _the length of the shortest subarray to remove_.
+
+A **subarray** is a contiguous subsequence of the array.
+
+**Example 1:**
+
+- **Input:** arr = [1,2,3,10,4,2,3,5]
+- **Output:** 3
+- **Explanation:** The shortest subarray we can remove is `[10,4,2]` of length `3`. The remaining elements after that will be `[1,2,3,3,5]` which are sorted.
+ Another correct solution is to remove the subarray `[3,10,4]`.
+
+**Example 2:**
+
+- **Input:** arr = [5,4,3,2,1]
+- **Output:** 4
+- **Explanation:** Since the array is strictly decreasing, we can only keep a single element. Therefore we need to remove a subarray of length `4`, either `[5,4,3,2]` or `[4,3,2,1]`.
+
+
+**Example 3:**
+
+- **Input:** arr = [1,2,3]
+- **Output:** 0
+- **Explanation:** The array is already non-decreasing. We do not need to remove any elements.
+
+
+
+**Constraints:**
+
+- 1 <= arr.length <= 105
+- 0 <= arr[i] <= 109
+
+
+**Hint:**
+1. The key is to find the longest non-decreasing subarray starting with the first element or ending with the last element, respectively.
+2. After removing some subarray, the result is the concatenation of a sorted prefix and a sorted suffix, where the last element of the prefix is smaller than the first element of the suffix.
+
+
+
+**Solution:**
+
+We can use sorting and binary search techniques. Hereβs the plan:
+
+### Approach:
+
+1. **Two Pointers Approach:**
+ - First, identify the longest non-decreasing prefix (`left` pointer).
+ - Then, identify the longest non-decreasing suffix (`right` pointer).
+ - After that, try to combine these two subarrays by considering the middle part of the array and adjusting the subarray to be removed in such a way that the combined array is non-decreasing.
+
+2. **Monotonic Stack:**
+ - Use a monotonic stack to help manage subarray elements in a sorted fashion.
+
+3. **Steps:**
+ - Find the longest non-decreasing prefix (`left`).
+ - Find the longest non-decreasing suffix (`right`).
+ - Try to merge the two subarrays by looking for elements that can form a valid combination.
+
+4. **Optimization:**
+ - Use binary search to optimize the merging step for finding the smallest subarray to remove.
+
+Let's implement this solution in PHP: **[1574. Shortest Subarray to be Removed to Make Array Sorted](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/001574-shortest-subarray-to-be-removed-to-make-array-sorted/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+1. **Longest Non-Decreasing Prefix and Suffix**:
+ - The prefix is determined by traversing the array from the start until elements are in non-decreasing order.
+ - Similarly, the suffix is determined by traversing from the end.
+
+2. **Initial Minimum Removal**:
+ - Calculate the removal length by keeping only the prefix or the suffix.
+
+3. **Merging Prefix and Suffix**:
+ - Use two pointers (`i` for prefix and `j` for suffix) to find the smallest subarray to remove such that the last element of the prefix is less than or equal to the first element of the suffix.
+
+4. **Return Result**:
+ - The result is the minimum length of the subarray to remove, calculated as the smaller of the initial removal or the merging of prefix and suffix.
+
+### Complexity
+- **Time Complexity**: _**O(n)**_, as the array is traversed at most twice.
+- **Space Complexity**: _**O(1)**_, as only a few variables are used.
+
+This solution efficiently finds the shortest subarray to be removed to make the array sorted by using a two-pointer technique, and it handles large arrays up to the constraint of `10^5` elements.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
diff --git a/algorithms/001574-shortest-subarray-to-be-removed-to-make-array-sorted/solution.php b/algorithms/001574-shortest-subarray-to-be-removed-to-make-array-sorted/solution.php
new file mode 100644
index 000000000..4bd1e4e5d
--- /dev/null
+++ b/algorithms/001574-shortest-subarray-to-be-removed-to-make-array-sorted/solution.php
@@ -0,0 +1,46 @@
+ 0 && $arr[$right - 1] <= $arr[$right]) {
+ $right--;
+ }
+
+ // Minimum removal when keeping only the prefix or suffix
+ $minRemove = min($n - $left - 1, $right);
+
+ // Try to merge prefix and suffix
+ $i = 0;
+ $j = $right;
+ while ($i <= $left && $j < $n) {
+ if ($arr[$i] <= $arr[$j]) {
+ $minRemove = min($minRemove, $j - $i - 1);
+ $i++;
+ } else {
+ $j++;
+ }
+ }
+
+ return $minRemove;
+ }
+}
\ No newline at end of file
diff --git a/algorithms/001590-make-sum-divisible-by-p/README.md b/algorithms/001590-make-sum-divisible-by-p/README.md
new file mode 100644
index 000000000..2b26a1e10
--- /dev/null
+++ b/algorithms/001590-make-sum-divisible-by-p/README.md
@@ -0,0 +1,130 @@
+1590\. Make Sum Divisible by P
+
+**Difficulty:** Medium
+
+**Topics:** `Array`, `Hash Table`, `Prefix Sum`
+
+Given an array of positive integers `nums`, remove the **smallest** subarray (possibly **empty**) such that the sum of the remaining elements is divisible by `p`. It is `not` allowed to remove the whole array.
+
+Return _the length of the smallest subarray that you need to remove, or `-1` if it's impossible_.
+
+A **subarray** is defined as a contiguous block of elements in the array.
+
+**Example 1:**
+
+- **Input:** nums = [3,1,4,2], p = 6
+- **Output:** 1
+- **Explanation:** The sum of the elements in nums is 10, which is not divisible by 6. We can remove the subarray [4], and the sum of the remaining elements is 6, which is divisible by 6.
+
+**Example 2:**
+
+- **Input:** nums = [6,3,5,2], p = 9
+- **Output:** 2
+- **Explanation:** We cannot remove a single element to get a sum divisible by 9. The best way is to remove the subarray [5,2], leaving us with [6,3] with sum 9.
+
+
+**Example 3:**
+
+- **Input:** nums = [1,2,3], p = 3
+- **Output:** 0
+- **Explanation:** Here the sum is 6. which is already divisible by 3. Thus we do not need to remove anything.
+
+
+
+**Constraints:**
+
+- 1 <= nums.length <= 105
+- 1 <= nums[i] <= 109
+- 1 <= p <= 109
+
+
+
+**Hint:**
+1. Use prefix sums to calculate the subarray sums.
+2. Suppose you know the remainder for the sum of the entire array. How does removing a subarray affect that remainder? What remainder does the subarray need to have in order to make the rest of the array sum up to be divisible by k?
+3. Use a map to keep track of the rightmost index for every prefix sum % p.
+
+
+
+**Solution:**
+
+We can use a combination of prefix sums and a hash table to efficiently compute the smallest subarray that needs to be removed such that the sum of the remaining elements is divisible by `p`.
+
+### Key Insights:
+1. **Prefix Sum Modulo**: The sum of the entire array modulo `p` gives us the remainder when the array sum is divided by `p`. If this remainder is zero, the sum is already divisible by `p`, and no subarray needs to be removed. Otherwise, the goal is to remove a subarray that brings the sum modulo `p` to zero.
+
+2. **Target Remainder**: If the total sum modulo `p` is `r`, we need to find a subarray whose sum modulo `p` is also `r`. Removing this subarray will result in the remaining sum being divisible by `p`.
+
+3. **Efficient Search Using a Hash Map**: We can use a hash map to store the prefix sum modulo `p` and the index at which that sum occurs. This allows us to quickly find if we can remove a subarray that satisfies the required condition.
+
+### Approach:
+1. Compute the total sum of the array, and find its remainder when divided by `p`. Call this remainder `r`.
+2. Traverse through the array while maintaining the current prefix sum and looking for a previously seen prefix sum that satisfies the condition (i.e., removing the elements between the current index and the previous index will result in a sum divisible by `p`).
+3. Use a hash map to store the last occurrence of each prefix sum modulo `p`.
+
+Let's implement this solution in PHP: **[1590. Make Sum Divisible by P](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/001590-make-sum-divisible-by-p/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+1. **Initialization**:
+ - We calculate the total sum of the array and its remainder when divided by `p`. If the remainder `r` is zero, the array is already divisible by `p`, so we return `0`.
+ - We initialize a hash map (`prefixMap`) to store the last occurrence of each prefix sum modulo `p`. The map starts with `0 => -1` to handle cases where the subarray starts from the beginning.
+
+2. **Prefix Sum Calculation**:
+ - As we iterate through the array, we maintain a running `prefixSum`. At each step, we compute `prefixSum % p`.
+
+3. **Finding the Target**:
+ - We need to check if there exists a previous prefix sum that, when subtracted from the current prefix sum, results in a sum divisible by `p`. This is achieved by calculating the `target = (prefixSum - r + p) % p`.
+
+4. **Subarray Removal**:
+ - If the `target` is found in the `prefixMap`, we compute the length of the subarray that would need to be removed to achieve the desired sum and update the `minLength` accordingly.
+
+5. **Final Result**:
+ - If no valid subarray is found (`minLength` remains `PHP_INT_MAX`), we return `-1`. Otherwise, we return the smallest subarray length found.
+
+### Time Complexity:
+- The solution runs in **O(n)** time, where `n` is the length of the array, since we traverse the array once and each operation (like hash map lookup and insertion) takes constant time.
+
+### Space Complexity:
+- The space complexity is **O(n)** due to the hash map storing at most `n` prefix sums.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
+
diff --git a/algorithms/001590-make-sum-divisible-by-p/solution.php b/algorithms/001590-make-sum-divisible-by-p/solution.php
new file mode 100644
index 000000000..8c38cbebc
--- /dev/null
+++ b/algorithms/001590-make-sum-divisible-by-p/solution.php
@@ -0,0 +1,46 @@
+ -1); // Initialize with 0 => -1 for cases when we find a match early
+
+ for ($i = 0; $i < $n; $i++) {
+ // Calculate the prefix sum
+ $prefixSum = ($prefixSum + $nums[$i]) % $p;
+
+ // We want to find a previous prefix sum that satisfies (prefixSum - r) % p == 0
+ $target = ($prefixSum - $r + $p) % $p;
+
+ if (isset($prefixMap[$target])) {
+ // Calculate the length of the subarray to remove
+ $minLength = min($minLength, $i - $prefixMap[$target]);
+ }
+
+ // Update the map with the current prefix sum % p and its index
+ $prefixMap[$prefixSum] = $i;
+ }
+
+ // If minLength is still INT_MAX, it's impossible to find a valid subarray
+ return $minLength < $n ? $minLength : -1;
+ }
+}
\ No newline at end of file
diff --git a/algorithms/001593-split-a-string-into-the-max-number-of-unique-substrings/README.md b/algorithms/001593-split-a-string-into-the-max-number-of-unique-substrings/README.md
new file mode 100644
index 000000000..d00ac7985
--- /dev/null
+++ b/algorithms/001593-split-a-string-into-the-max-number-of-unique-substrings/README.md
@@ -0,0 +1,126 @@
+1593\. Split a String Into the Max Number of Unique Substrings
+
+**Difficulty:** Medium
+
+**Topics:** `Hash Table`, `String`, `Backtracking`
+
+Given a string `s`, return _the maximum number of unique substrings that the given string can be split into_.
+
+You can split string `s` into any list of **non-empty substrings**, where the concatenation of the substrings forms the original string. However, you must split the substrings such that all of them are **unique**.
+
+A **substring** is a contiguous sequence of characters within a string.
+
+**Example 1:**
+
+- **Input:** s = "ababccc"
+- **Output:** 5
+- **Explanation:** One way to split maximally is ['a', 'b', 'ab', 'c', 'cc']. Splitting like ['a', 'b', 'a', 'b', 'c', 'cc'] is not valid as you have 'a' and 'b' multiple times.
+
+**Example 2:**
+
+- **Input:** s = "aba"
+- **Output:** 2
+- **Explanation:** One way to split maximally is ['a', 'ba'].
+
+
+**Example 3:**
+
+- **Input:** s = "aa"
+- **Output:** 1
+- **Explanation:** It is impossible to split the string any further.
+
+
+
+**Constraints:**
+
+- `1 <= s.length <= 16`
+- `s` contains only lower case English letters.
+
+
+**Hint:**
+1. Use a set to keep track of which substrings have been used already
+2. Try each possible substring at every position and backtrack if a complete split is not possible
+
+
+
+**Solution:**
+
+We can use a backtracking approach. This involves recursively trying to create substrings from the current position in the string and keeping track of the unique substrings we've used so far.
+
+Here's a step-by-step solution:
+
+1. **Recursive Function**: Create a function that will explore all possible substrings starting from the current index of the string.
+2. **Set for Uniqueness**: Use a set (or an array in PHP) to keep track of the unique substrings that have been used in the current recursion path.
+3. **Backtracking**: When a substring is chosen, we can continue to choose the next substring. If we reach a point where no further substrings can be formed without repeating, we backtrack.
+4. **Base Case**: If we reach the end of the string, we count the unique substrings formed.
+
+Let's implement this solution in PHP: **[1593. Split a String Into the Max Number of Unique Substrings](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/001593-split-a-string-into-the-max-number-of-unique-substrings/solution.php)**
+
+```php
+maxUniqueSplit("ababccc"); // Output: 5
+echo "\n";
+echo $solution->maxUniqueSplit("aba"); // Output: 2
+echo "\n";
+echo $solution->maxUniqueSplit("aa"); // Output: 1
+?>
+```
+
+### Explanation:
+
+1. **Function Signature**: The main function is `maxUniqueSplit`, which initializes the backtracking process.
+
+2. **Backtracking**:
+ - The `backtrack` function takes the string, the array of used substrings, and the current starting index.
+ - If the `start` index reaches the end of the string, it returns the count of unique substrings collected.
+ - A loop iterates through possible end indices to create substrings from the `start` index.
+ - If the substring is unique (not already in the `used` array), it's added to `used`, and the function recurses for the next index.
+ - After exploring that path, it removes the substring to backtrack and explore other possibilities.
+
+3. **Output**: The function returns the maximum number of unique substrings for various input strings.
+
+### Complexity
+- The time complexity can be high due to the nature of backtracking, especially for longer strings, but given the constraints (maximum length of 16), this solution is efficient enough for the input limits.
+
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
diff --git a/algorithms/001593-split-a-string-into-the-max-number-of-unique-substrings/solution.php b/algorithms/001593-split-a-string-into-the-max-number-of-unique-substrings/solution.php
new file mode 100644
index 000000000..68c9c6abd
--- /dev/null
+++ b/algorithms/001593-split-a-string-into-the-max-number-of-unique-substrings/solution.php
@@ -0,0 +1,48 @@
+backtrack($s, [], 0);
+ }
+
+ /**
+ * @param $s
+ * @param $used
+ * @param $start
+ * @return int|mixed
+ */
+ private function backtrack($s, $used, $start) {
+ // If we've reached the end of the string, return the size of the used set
+ if ($start === strlen($s)) {
+ return count($used);
+ }
+
+ $maxCount = 0;
+
+ // Try to create substrings from start index to the end of the string
+ for ($end = $start + 1; $end <= strlen($s); $end++) {
+ // Get the current substring
+ $substring = substr($s, $start, $end - $start);
+
+ // Check if the substring is unique (not in the used set)
+ if (!in_array($substring, $used)) {
+ // Add the substring to the used set
+ $used[] = $substring;
+
+ // Recur for the next part of the string
+ $maxCount = max($maxCount, $this->backtrack($s, $used, $end));
+
+ // Backtrack: remove the last added substring
+ array_pop($used);
+ }
+ }
+
+ return $maxCount;
+ }
+}
\ No newline at end of file
diff --git a/algorithms/001595-minimum-cost-to-connect-two-groups-of-points/README.md b/algorithms/001595-minimum-cost-to-connect-two-groups-of-points/README.md
new file mode 100644
index 000000000..3280b9dba
--- /dev/null
+++ b/algorithms/001595-minimum-cost-to-connect-two-groups-of-points/README.md
@@ -0,0 +1,127 @@
+1595\. Minimum Cost to Connect Two Groups of Points
+
+**Difficulty:** Hard
+
+**Topics:** `Array`, `Dynamic Programming`, `Bit Manipulation`, `Matrix`, `Bitmask`
+
+You are given two groups of points where the first group has size1
points, the second group has size2
points, and size1 >= size2
.
+
+The `cost` of the connection between any two points are given in an size1 x size2
matrix where `cost[i][j]` is the cost of connecting point `i` of the first group and point `j` of the second group. The groups are connected if **each point in both groups is connected to one or more points in the opposite group**. In other words, each point in the first group must be connected to at least one point in the second group, and each point in the second group must be connected to at least one point in the first group.
+
+Return _the minimum cost it takes to connect the two groups_.
+
+**Example 1:**
+
+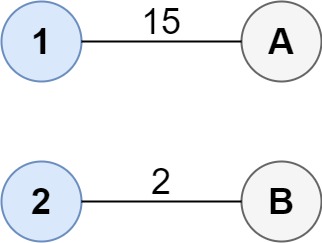
+
+- **Input:** cost = [[15, 96], [36, 2]]
+- **Output:** 17
+- **Explanation:** The optimal way of connecting the groups is:\
+ 1--A\
+ 2--B\
+ This results in a total cost of 17.
+
+**Example 2:**
+
+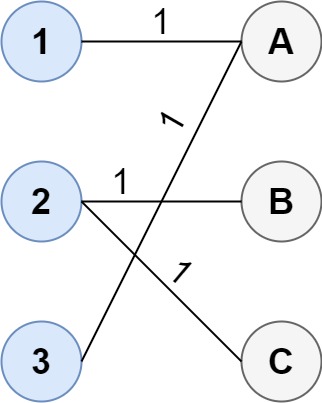
+
+- **Input:** cost = [[1, 3, 5], [4, 1, 1], [1, 5, 3]]
+- **Output:** 4
+- **Explanation:** The optimal way of connecting the groups is:\
+ 1--A\
+ 2--B\
+ 2--C\
+ 3--A\
+ This results in a total cost of 4.\
+ Note that there are multiple points connected to point 2 in the first group and point A in the second group. This does not matter as there is no limit to the number of points that can be connected. We only care about the minimum total cost.
+
+
+**Example 3:**
+
+- **Input:** cost = [[2, 5, 1], [3, 4, 7], [8, 1, 2], [6, 2, 4], [3, 8, 8]]
+- **Output:** 10
+
+
+
+**Constraints:**
+
+- size1 == cost.length
+- size2 == cost[i].length
+- 1 <= size1, size2 <= 12
+- size1 >= size2
+- `0 <= cost[i][j] <= 100`
+
+
+**Hint:**
+1. Each point on the left would either be connected to exactly point already connected to some left node, or a subset of the nodes on the right which are not connected to any node
+2. Use dynamic programming with bitmasking, where the state will be (number of points assigned in first group, bitmask of points assigned in second group).
+
+
+
+**Solution:**
+
+We can leverage dynamic programming with bitmasking. The idea is to minimize the cost by considering each point in the first group and trying to connect it to all points in the second group.
+
+### Dynamic Programming (DP) Approach with Bitmasking
+
+#### Steps:
+1. **State Representation**:
+ - Use a DP table `dp[i][mask]` where:
+ - `i` is the index in the first group (ranging from `0` to `size1-1`).
+ - `mask` is a bitmask representing which points in the second group have been connected.
+
+2. **State Transition**:
+ - For each point in the first group, try connecting it to every point in the second group, updating the DP table accordingly.
+ - If a new point in the second group is connected, update the corresponding bit in the mask.
+
+3. **Base Case**:
+ - Start with `dp[0][0] = 0` (no connections initially).
+
+4. **Goal**:
+ - Compute the minimum cost for `dp[size1][(1 << size2) - 1]`, which represents connecting all points from both groups.
+
+Let's implement this solution in PHP: **[1595. Minimum Cost to Connect Two Groups of Points](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/001595-minimum-cost-to-connect-two-groups-of-points/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+- The DP array `dp[i][mask]` stores the minimum cost to connect the first `i` points from group 1 with the points in group 2 as indicated by `mask`.
+- The nested loops iterate over each combination of `i` and `mask`, trying to find the optimal cost by considering all possible connections.
+- In the end, the solution calculates the minimum cost considering the scenarios where some points in the second group may still be unconnected, ensuring all points are connected.
+
+This approach efficiently handles the problem's constraints and ensures the minimum cost for connecting the two groups.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
+
diff --git a/algorithms/001595-minimum-cost-to-connect-two-groups-of-points/solution.php b/algorithms/001595-minimum-cost-to-connect-two-groups-of-points/solution.php
new file mode 100644
index 000000000..00154459b
--- /dev/null
+++ b/algorithms/001595-minimum-cost-to-connect-two-groups-of-points/solution.php
@@ -0,0 +1,49 @@
+ith character (**0-indexed**) of `target`, you can choose the kth
character of the jth
string in words if `target[i] = words[j][k]`.
+- Once you use the kth
character of the jth
string of `words`, you **can no longer** use the xth
character of any string in `words` where `x <= k`. In other words, all characters to the left of or at index `k` become unusuable for every string.
+- Repeat the process until you form the string `target`.
+
+**Notice** that you can use **multiple characters** from the **same string** in `words` provided the conditions above are met.
+
+Return _the number of ways to form `target` from `words`_. Since the answer may be too large, return it modulo 109 + 7
.
+
+**Example 1:**
+
+- **Input:** words = ["acca","bbbb","caca"], target = "aba"
+- **Output:** 6
+- **Explanation:** There are 6 ways to form target.
+ "aba" -> index 0 ("acca"), index 1 ("bbbb"), index 3 ("caca")
+ "aba" -> index 0 ("acca"), index 2 ("bbbb"), index 3 ("caca")
+ "aba" -> index 0 ("acca"), index 1 ("bbbb"), index 3 ("acca")
+ "aba" -> index 0 ("acca"), index 2 ("bbbb"), index 3 ("acca")
+ "aba" -> index 1 ("caca"), index 2 ("bbbb"), index 3 ("acca")
+ "aba" -> index 1 ("caca"), index 2 ("bbbb"), index 3 ("caca")
+
+**Example 2:**
+
+- **Input:** words = ["abba","baab"], target = "bab"
+- **Output:** 4
+- **Explanation:** There are 4 ways to form target.
+ "bab" -> index 0 ("baab"), index 1 ("baab"), index 2 ("abba")
+ "bab" -> index 0 ("baab"), index 1 ("baab"), index 3 ("baab")
+ "bab" -> index 0 ("baab"), index 2 ("baab"), index 3 ("baab")
+ "bab" -> index 1 ("abba"), index 2 ("baab"), index 3 ("baab")
+
+
+
+**Constraints:**
+
+
+- `1 <= words.length <= 1000`
+- `1 <= words[i].length <= 1000`
+- All strings in `words` have the same length.
+- `1 <= target.length <= 1000`
+- `words[i]` and `target` contain only lowercase English letters.
+
+
+
+**Hint:**
+1. For each index i, store the frequency of each character in the ith
row.
+2. Use dynamic programing to calculate the number of ways to get the target string using the frequency array.
+
+
+
+**Solution:**
+
+The problem requires finding the number of ways to form a `target` string from a dictionary of `words`, following specific rules about character usage. This is a combinatorial problem that can be solved efficiently using **Dynamic Programming (DP)** and preprocessing character frequencies.
+
+
+### **Key Points**
+1. **Constraints**:
+ - Words are of the same length.
+ - Characters in words can only be used in a left-to-right manner.
+2. **Challenges**:
+ - Efficiently counting ways to form `target` due to large constraints.
+ - Avoid recomputation with memoization.
+3. **Modulo**:
+ - Since the result can be large, all calculations are done modulo 109 + 7
.
+
+
+### **Approach**
+The solution uses:
+1. **Preprocessing**:
+ - Count the frequency of each character at every position across all words.
+2. **Dynamic Programming**:
+ - Use a 2D DP table to calculate the number of ways to form the `target` string.
+
+**Steps**:
+1. Preprocess `words` into a frequency array (`counts`).
+2. Define a DP function to recursively find the number of ways to form `target` using the preprocessed counts.
+
+
+### **Plan**
+1. **Input Parsing**:
+ - Accept `words` and `target`.
+2. **Preprocess**:
+ - Create a `counts` array where `counts[j][char]` represents the frequency of `char` at position `j` in all words.
+3. **Dynamic Programming**:
+ - Use a recursive function with memoization to compute the number of ways to form `target` using characters from valid positions in `words`.
+4. **Return the Result**:
+ - Return the total count modulo 109 + 7
.
+
+Let's implement this solution in PHP: **[1639. Number of Ways to Form a Target String Given a Dictionary](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/001639-number-of-ways-to-form-a-target-string-given-a-dictionary/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+1. **Preprocessing Step**:
+ - Build a `counts` array:
+ - For each column in `words`, count the occurrences of each character.
+ - Example: If `words = ["acca", "bbbb", "caca"]`, for the first column, `counts[0] = {'a': 1, 'b': 1, 'c': 1}`.
+
+2. **Recursive DP**:
+ - Define `dp(i, j)`:
+ - `i` is the current index in `target`.
+ - `j` is the current position in `words`.
+ - Base Cases:
+ - If `i == len(target)`: Entire target formed β return 1.
+ - If `j == len(words[0])`: No more columns to use β return 0.
+ - Recurrence:
+ - Option 1: Use `counts[j][target[i]]` characters from position `j`.
+ - Option 2: Skip position `j`.
+
+3. **Optimization**:
+ - Use a memoization table to store results of overlapping subproblems.
+
+
+### **Example Walkthrough**
+**Input**:
+`words = ["acca", "bbbb", "caca"], target = "aba"`
+
+1. **Preprocessing**:
+ - `counts[0] = {'a': 2, 'b': 0, 'c': 1}`
+ - `counts[1] = {'a': 0, 'b': 3, 'c': 0}`
+ - `counts[2] = {'a': 1, 'b': 0, 'c': 2}`
+ - `counts[3] = {'a': 2, 'b': 0, 'c': 1}`
+
+2. **DP Calculation**:
+ - `dp(0, 0)`: How many ways to form `"aba"` starting from column 0.
+ - Recursively calculate, combining the use of `counts` and skipping columns.
+
+**Output**: `6`
+
+
+### **Time Complexity**
+1. **Preprocessing**: _**O(n x m)**_, where `n` is the number of words and `m` is their length.
+2. **Dynamic Programming**: _**O(l x m)**_, where `l` is the length of the target.
+3. **Total**: _**O(n x m + l x m)**_.
+
+
+### **Output for Example**
+- **Input**:
+ `words = ["acca", "bbbb", "caca"], target = "aba"`
+- **Output**: `6`
+
+
+This problem is a great example of combining preprocessing and dynamic programming to solve a combinatorial challenge efficiently.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
diff --git a/algorithms/001639-number-of-ways-to-form-a-target-string-given-a-dictionary/solution.php b/algorithms/001639-number-of-ways-to-form-a-target-string-given-a-dictionary/solution.php
new file mode 100644
index 000000000..e006a8fe4
--- /dev/null
+++ b/algorithms/001639-number-of-ways-to-form-a-target-string-given-a-dictionary/solution.php
@@ -0,0 +1,53 @@
+dp(0, 0, $counts, $target, $memo);
+ }
+
+ /**
+ * Helper function for DP
+ *
+ * @param $i
+ * @param $j
+ * @param $counts
+ * @param $target
+ * @param $memo
+ * @return float|int|mixed
+ */
+ private function dp($i, $j, $counts, $target, &$memo) {
+ if ($i == strlen($target)) return 1; // Entire target formed
+ if ($j == count($counts)) return 0; // Out of bounds
+ if ($memo[$i][$j] != -1) return $memo[$i][$j];
+
+ $ways = $this->dp($i, $j + 1, $counts, $target, $memo); // Skip this column
+ $charIndex = ord($target[$i]) - ord('a');
+
+ if ($counts[$j][$charIndex] > 0) {
+ $ways += $this->dp($i + 1, $j + 1, $counts, $target, $memo) * $counts[$j][$charIndex];
+ $ways %= Solution::MOD;
+ }
+
+ return $memo[$i][$j] = $ways;
+ }
+}
\ No newline at end of file
diff --git a/algorithms/001652-defuse-the-bomb/README.md b/algorithms/001652-defuse-the-bomb/README.md
new file mode 100644
index 000000000..7354c577e
--- /dev/null
+++ b/algorithms/001652-defuse-the-bomb/README.md
@@ -0,0 +1,128 @@
+1652\. Defuse the Bomb
+
+**Difficulty:** Easy
+
+**Topics:** `Array`, `Sliding Window`
+
+You have a bomb to defuse, and your time is running out! Your informer will provide you with a **circular** array `code` of length of `n` and a key `k`.
+
+To decrypt the code, you must replace every number. All the numbers are replaced **simultaneously**.
+
+- If `k > 0`, replace the ith
number with the sum of the **next** `k` numbers.
+- If `k < 0`, replace the ith
number with the sum of the **previous** `k` numbers.
+- If `k == 0`, replace the ith
number with `0`.
+
+As `code` is circular, the next element of `code[n-1]` is `code[0]`, and the previous element of `code[0]` is `code[n-1]`.
+
+Given the **circular** array `code` and an integer key `k`, return _the decrypted code to defuse the bomb_!
+
+**Example 1:**
+
+- **Input:** code = [5,7,1,4], k = 3
+- **Output:** [12,10,16,13]
+- **Explanation:** Each number is replaced by the sum of the next 3 numbers. The decrypted code is [7+1+4, 1+4+5, 4+5+7, 5+7+1]. Notice that the numbers wrap around.
+
+**Example 2:**
+
+- **Input:** code = [1,2,3,4], k = 0
+- **Output:** [0,0,0,0]
+- **Explanation:** When k is zero, the numbers are replaced by 0.
+
+
+**Example 3:**
+
+- **Input:** code = [2,4,9,3], k = -2
+- **Output:** [12,5,6,13]
+- **Explanation:** The decrypted code is [3+9, 2+3, 4+2, 9+4]. Notice that the numbers wrap around again. If k is negative, the sum is of the previous numbers.
+
+
+**Constraints:**
+
+- `n == code.length`
+- `1 <= n <= 100`
+- `1 <= code[i] <= 100`
+- `-(n - 1) <= k <= n - 1`
+
+
+**Hint:**
+1. As the array is circular, use modulo to find the correct index.
+2. The constraints are low enough for a brute-force solution.
+
+
+
+**Solution:**
+
+We can implement a function that iterates over the `code` array and computes the sum of the appropriate numbers based on the value of `k`.
+
+The general approach will be as follows:
+1. If `k == 0`, replace all elements with 0.
+2. If `k > 0`, replace each element with the sum of the next `k` elements in the circular array.
+3. If `k < 0`, replace each element with the sum of the previous `k` elements in the circular array.
+
+The circular nature of the array means that for indices that exceed the bounds of the array, you can use modulo (`%`) to "wrap around" the array.
+
+Let's implement this solution in PHP: **[1652. Defuse the Bomb](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/001652-defuse-the-bomb/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+1. **Initialization**:
+ - We create a result array initialized with zeros using `array_fill`.
+
+2. **Handling `k == 0`**:
+ - If `k` is zero, the output array is simply filled with zeros, as required by the problem.
+
+3. **Iterating Through the Array**:
+ - For each index `i` in the array:
+ - If `k > 0`, sum the next `k` elements using modulo arithmetic to wrap around.
+ - If `k < 0`, sum the previous `|k|` elements using modulo arithmetic with an offset to handle negative indices.
+
+4. **Modulo Arithmetic**:
+ - We use `($i + $j) % $n` to wrap around to the beginning of the array when accessing indices greater than `n - 1`.
+ - Similarly, `($i - $j + $n) % $n` handles backward wrapping for negative indices.
+
+5. **Complexity**:
+ - Time Complexity: _**O(n . |k|)**_, where _**n**_ is the size of the array and _**|k|**_ is the absolute value of _**k**_.
+ - Space Complexity: _**O(n)**_ for the result array.
+
+### Outputs:
+The provided examples match the expected results. Let me know if you need further explanation or optimizations!
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
diff --git a/algorithms/001652-defuse-the-bomb/solution.php b/algorithms/001652-defuse-the-bomb/solution.php
new file mode 100644
index 000000000..d5c409c86
--- /dev/null
+++ b/algorithms/001652-defuse-the-bomb/solution.php
@@ -0,0 +1,37 @@
+ 0) {
+ for ($j = 1; $j <= $k; $j++) {
+ $sum += $code[($i + $j) % $n]; // Wrap around using modulo
+ }
+ } else { // k < 0
+ for ($j = 1; $j <= abs($k); $j++) {
+ $sum += $code[($i - $j + $n) % $n]; // Wrap around backward using modulo
+ }
+ }
+
+ $result[$i] = $sum;
+ }
+
+ return $result;
+ }
+}
\ No newline at end of file
diff --git a/algorithms/001671-minimum-number-of-removals-to-make-mountain-array/README.md b/algorithms/001671-minimum-number-of-removals-to-make-mountain-array/README.md
new file mode 100644
index 000000000..89b661378
--- /dev/null
+++ b/algorithms/001671-minimum-number-of-removals-to-make-mountain-array/README.md
@@ -0,0 +1,115 @@
+1671\. Minimum Number of Removals to Make Mountain Array
+
+**Difficulty:** Hard
+
+**Topics:** `Array`, `Binary Search`, `Dynamic Programming`, `Greedy`
+
+You may recall that an array `arr` is a **mountain array** if and only if:
+
+- `arr.length >= 3`
+- There exists some index `i` (**0-indexed**) with `0 < i < arr.length - 1` such that:
+ - `arr[0] < arr[1] < ... < arr[i - 1] < arr[i]`
+ - `arr[i] > arr[i + 1] > ... > arr[arr.length - 1]`
+
+Given an integer array `nums`, return _the **minimum** number of elements to remove to make `nums` a **mountain array**_.
+
+**Example 1:**
+
+- **Input:** nums = [1,3,1]
+- **Output:** 0
+- **Explanation:** The array itself is a mountain array so we do not need to remove any elements.
+
+**Example 2:**
+
+- **Input:** nums = [2,1,1,5,6,2,3,1]
+- **Output:** 3
+- **Explanation:** One solution is to remove the elements at indices 0, 1, and 5, making the array nums = [1,5,6,3,1].
+
+
+
+**Constraints:**
+
+- `3 <= nums.length <= 1000`
+- 1 <= nums[i] <= 109
+- `It is guaranteed that you can make a mountain array out of nums.`
+
+
+**Hint:**
+1. Think the opposite direction instead of minimum elements to remove the maximum mountain subsequence
+2. Think of LIS it's kind of close
+
+
+
+**Solution:**
+
+We can use a dynamic programming approach with the idea of finding the **maximum mountain subsequence** rather than directly counting elements to remove. This approach is based on finding two **Longest Increasing Subsequences (LIS)** for each position in the array: one going **left-to-right** and the other going **right-to-left**. Once we have the longest possible mountain subsequence, the difference between the original array length and this subsequence length will give us the minimum elements to remove.
+
+### Solution Outline
+
+1. **Identify increasing subsequence lengths**:
+ - Compute the `leftLIS` array, where `leftLIS[i]` represents the length of the longest increasing subsequence ending at `i` (going left to right).
+
+2. **Identify decreasing subsequence lengths**:
+ - Compute the `rightLIS` array, where `rightLIS[i]` represents the length of the longest decreasing subsequence starting at `i` (going right to left).
+
+3. **Calculate maximum mountain length**:
+ - For each index `i` where `0 < i < n - 1`, check if there exists a valid peak (i.e., `leftLIS[i] > 1` and `rightLIS[i] > 1`). Calculate the mountain length at `i` as `leftLIS[i] + rightLIS[i] - 1`.
+
+4. **Get the minimum removals**:
+ - The minimum elements to remove will be the original array length minus the longest mountain length found.
+
+Let's implement this solution in PHP: **[1671. Minimum Number of Removals to Make Mountain Array](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/001671-minimum-number-of-removals-to-make-mountain-array/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+1. **Left LIS Calculation**:
+ - `leftLIS[i]` stores the maximum length of an increasing subsequence ending at `i`. We iterate through each `i`, and for each `i`, iterate from `0` to `i-1` to find the longest subsequence ending at `i`.
+
+2. **Right LIS Calculation**:
+ - `rightLIS[i]` stores the maximum length of a decreasing subsequence starting at `i`. We iterate backward from `n-2` to `0`, and for each `i`, iterate from `n-1` down to `i+1` to find the longest subsequence starting at `i`.
+
+3. **Mountain Calculation**:
+ - A valid peak at index `i` must have `leftLIS[i] > 1` and `rightLIS[i] > 1`. The length of a mountain with peak at `i` is `leftLIS[i] + rightLIS[i] - 1`.
+
+4. **Final Calculation**:
+ - The minimum removals needed is the difference between the original array length and the maximum mountain length found.
+
+### Complexity Analysis
+
+- **Time Complexity**: _**O(n2)**_, due to the double loop in the LIS calculations.
+- **Space Complexity**: _**O(n)**_, for storing the `leftLIS` and `rightLIS` arrays.
+
+This solution ensures that we find the maximum mountain subsequence and compute the minimum removals required to achieve a mountain array.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
diff --git a/algorithms/001671-minimum-number-of-removals-to-make-mountain-array/solution.php b/algorithms/001671-minimum-number-of-removals-to-make-mountain-array/solution.php
new file mode 100644
index 000000000..c11d32cdc
--- /dev/null
+++ b/algorithms/001671-minimum-number-of-removals-to-make-mountain-array/solution.php
@@ -0,0 +1,43 @@
+= 0; $i--) {
+ for ($j = $n - 1; $j > $i; $j--) {
+ if ($nums[$j] < $nums[$i]) {
+ $rightLIS[$i] = max($rightLIS[$i], $rightLIS[$j] + 1);
+ }
+ }
+ }
+
+ // Step 3: Find the maximum length of a mountain sequence
+ $maxMountainLength = 0;
+ for ($i = 1; $i < $n - 1; $i++) {
+ if ($leftLIS[$i] > 1 && $rightLIS[$i] > 1) { // Valid peak
+ $maxMountainLength = max($maxMountainLength, $leftLIS[$i] + $rightLIS[$i] - 1);
+ }
+ }
+
+ // Step 4: Calculate minimum removals
+ return $n - $maxMountainLength;
+ }
+}
\ No newline at end of file
diff --git a/algorithms/001684-count-the-number-of-consistent-strings/README.md b/algorithms/001684-count-the-number-of-consistent-strings/README.md
new file mode 100644
index 000000000..22e5390b7
--- /dev/null
+++ b/algorithms/001684-count-the-number-of-consistent-strings/README.md
@@ -0,0 +1,149 @@
+1684\. Count the Number of Consistent Strings
+
+**Difficulty:** Easy
+
+**Topics:** `Array`, `Hash Table`, `String`, `Bit Manipulation`, `Counting`
+
+You are given a string `allowed` consisting of distinct characters and an array of strings `words`. A string is **consistent** if all characters in the string appear in the string `allowed`.
+
+Return _the number of **consistent** strings in the array `words`_.
+
+**Example 1:**
+
+- **Input:** allowed = "ab", words = ["ad","bd","aaab","baa","badab"]
+- **Output:** 2
+- **Explanation:** Strings "aaab" and "baa" are consistent since they only contain characters 'a' and 'b'.
+
+**Example 2:**
+
+- **Input:** allowed = "abc", words = ["a","b","c","ab","ac","bc","abc"]
+- **Output:** 7
+- **Explanation:** All strings are consistent.
+
+
+**Example 3:**
+
+- **Input:** allowed = "cad", words = ["cc","acd","b","ba","bac","bad","ac","d"]
+- **Output:** 4
+- **Explanation:** Strings "cc", "acd", "ac", and "d" are consistent.
+
+
+
+**Constraints:**
+
+- 1 <= words.length <= 104
+- 1 <= allowed.length <= 26
+- 1 <= words[i].length <= 10
+- The characters in `allowed` are **distinct**.
+- `words[i]` and `allowed` contain only lowercase English letters.
+
+
+**Hint:**
+1. A string is incorrect if it contains a character that is not allowed
+2. Constraints are small enough for brute force
+
+
+
+**Solution:**
+
+The idea is to check if each word in the `words` array is **consistent** with the characters in the `allowed` string. A word is **consistent** if all its characters are present in the `allowed` string.
+
+### Plan
+
+1. **Allowed Characters Set**:
+ - We can convert the `allowed` string into a set of characters to efficiently check if each character in the word exists in the set.
+
+2. **Word Consistency Check**:
+ - For each word in the `words` array, check if all its characters exist in the `allowed` set.
+
+3. **Count Consistent Words**:
+ - Initialize a counter. For each word that is consistent, increment the counter.
+
+4. **Return the Count**:
+ - Once all words are processed, return the count of consistent words.
+
+Let's implement this solution in PHP: **[1684. Count the Number of Consistent Strings](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/001684-count-the-number-of-consistent-strings/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+1. **Allowed Set**:
+ - We create an associative array `$allowedSet` where each key is a character from the `allowed` string. This allows for fast lookups.
+
+2. **Word Consistency**:
+ - For each word in the `words` array, we loop through its characters and check if they are in `$allowedSet`. If we find any character that isn't in the set, the word is marked as inconsistent, and we move on to the next word.
+
+3. **Counting**:
+ - Every time we find a consistent word, we increment the counter `$consistentCount`.
+
+4. **Return the Result**:
+ - After processing all words, the counter holds the number of consistent strings, which we return.
+
+### Time Complexity:
+
+- **Time Complexity**: O(n * m), where `n` is the number of words and `m` is the average length of the words. We are iterating through all the words and their characters.
+
+### Example Walkthrough:
+
+For the input:
+```php
+$allowed = "ab";
+$words = ["ad", "bd", "aaab", "baa", "badab"];
+```
+- We create a set: `allowedSet = ['a' => true, 'b' => true]`.
+- Checking each word:
+ - "ad" is inconsistent (contains 'd').
+ - "bd" is inconsistent (contains 'd').
+ - "aaab" is consistent (only contains 'a' and 'b').
+ - "baa" is consistent (only contains 'a' and 'b').
+ - "badab" is inconsistent (contains 'd').
+
+Thus, the function returns `2`.
+
+### Constraints Handling:
+
+- Since `allowed` can only have up to 26 distinct characters, and `words` has at most 10,000 entries, this brute-force solution is efficient enough given the constraints. Each word can have a maximum length of 10, making it feasible to iterate over all characters.
+
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
diff --git a/algorithms/001684-count-the-number-of-consistent-strings/solution.php b/algorithms/001684-count-the-number-of-consistent-strings/solution.php
new file mode 100644
index 000000000..222f7ef4c
--- /dev/null
+++ b/algorithms/001684-count-the-number-of-consistent-strings/solution.php
@@ -0,0 +1,39 @@
+ith bag contains `nums[i]` balls. You are also given an integer `maxOperations`.
+
+You can perform the following operation at most `maxOperations` times:
+
+- Take any bag of balls and divide it into two new bags with a **positive** number of balls.
+ - For example, a bag of `5` balls can become two new bags of `1` and `4` balls, or two new bags of `2` and `3` balls.
+
+Your penalty is the **maximum** number of balls in a bag. You want to minimize your penalty after the operations.
+
+Return _the minimum possible penalty after performing the operations_.
+
+**Example 1:**
+
+- **Input:** nums = [9], maxOperations = 2
+- **Output:** 3
+- **Explanation:**
+ - Divide the bag with 9 balls into two bags of sizes 6 and 3. [9] -> [6,3].
+ - Divide the bag with 6 balls into two bags of sizes 3 and 3. [6,3] -> [3,3,3].
+ The bag with the most number of balls has 3 balls, so your penalty is 3 and you should return 3.
+
+**Example 2:**
+
+- **Input:** nums = [2,4,8,2], maxOperations = 4
+- **Output:** 2
+- **Explanation:**
+ - Divide the bag with 8 balls into two bags of sizes 4 and 4. [2,4,8,2] -> [2,4,4,4,2].
+ - Divide the bag with 4 balls into two bags of sizes 2 and 2. [2,4,4,4,2] -> [2,2,2,4,4,2].
+ - Divide the bag with 4 balls into two bags of sizes 2 and 2. [2,2,2,4,4,2] -> [2,2,2,2,2,4,2].
+ - Divide the bag with 4 balls into two bags of sizes 2 and 2. [2,2,2,2,2,4,2] -> [2,2,2,2,2,2,2,2].
+ The bag with the most number of balls has 2 balls, so your penalty is 2, and you should return 2.
+
+
+
+**Constraints:**
+
+- 1 <= nums.length <= 105
+- 1 <= maxOperations, nums[i] <= 109
+
+
+**Hint:**
+1. Let's change the question if we know the maximum size of a bag what is the minimum number of bags you can make
+2. note that as the maximum size increases the minimum number of bags decreases so we can binary search the maximum size
+
+
+
+**Solution:**
+
+We can use **binary search** to find the minimum possible penalty. The key insight is that if we can determine whether a given penalty is achievable, we can narrow down the search range using binary search.
+
+### Steps to Solve:
+1. **Binary Search Setup**:
+ - The minimum penalty is `1` (all balls are split into single-ball bags).
+ - The maximum penalty is the largest number in the `nums` array.
+
+2. **Feasibility Check**:
+ - For a given penalty `mid`, check if it's possible to achieve it with at most `maxOperations` splits.
+ - To do this, for each bag size in `nums`, calculate the number of splits required to make all bags have `mid` balls or fewer. If the total splits exceed `maxOperations`, the penalty `mid` is not feasible.
+
+3. **Iterate**:
+ - Use binary search to adjust the range `[low, high]` based on whether a penalty `mid` is feasible.
+
+Let's implement this solution in PHP: **[1760. Minimum Limit of Balls in a Bag](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/001760-minimum-limit-of-balls-in-a-bag/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+1. **Binary Search**:
+ - The search space is between `1` and the maximum number in the `nums` array.
+ - The midpoint `mid` represents the current penalty we are testing.
+
+2. **Feasibility Check (`canAchievePenalty`)**:
+ - For each bag, calculate the required splits to ensure all bags have `mid` balls or fewer:
+ - `ceil(balls / mid) - 1` gives the number of splits needed.
+ - If the total splits exceed `maxOperations`, the penalty is not feasible.
+
+3. **Adjust Search Space**:
+ - If the penalty is feasible, reduce the upper bound (`high = mid`).
+ - If not, increase the lower bound (`low = mid + 1`).
+
+4. **Result**:
+ - When the loop exits, `low` contains the smallest feasible penalty.
+
+### Complexity:
+- **Time Complexity**: _**O(n . log(max(nums)))**_
+ - Binary search runs in _**O(log(max(nums)))**_, and the feasibility check for each midpoint takes _**O(n)**_.
+- **Space Complexity**: _**O(1)**_, as we only use constant extra space.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
diff --git a/algorithms/001760-minimum-limit-of-balls-in-a-bag/solution.php b/algorithms/001760-minimum-limit-of-balls-in-a-bag/solution.php
new file mode 100644
index 000000000..76edf3f66
--- /dev/null
+++ b/algorithms/001760-minimum-limit-of-balls-in-a-bag/solution.php
@@ -0,0 +1,48 @@
+canAchievePenalty($nums, $maxOperations, $mid)) {
+ $high = $mid; // Try smaller penalties
+ } else {
+ $low = $mid + 1; // Increase penalty
+ }
+ }
+
+ return $low; // Minimum penalty that satisfies the condition
+ }
+
+ /**
+ * Helper function to check if a penalty is feasible
+ *
+ * @param $nums
+ * @param $maxOperations
+ * @param $penalty
+ * @return bool
+ */
+ function canAchievePenalty($nums, $maxOperations, $penalty) {
+ $operations = 0;
+ foreach ($nums as $balls) {
+ if ($balls > $penalty) {
+ // Calculate the number of splits needed
+ $operations += ceil($balls / $penalty) - 1;
+ if ($operations > $maxOperations) {
+ return false;
+ }
+ }
+ }
+ return true;
+ }
+}
\ No newline at end of file
diff --git a/algorithms/001769-minimum-number-of-operations-to-move-all-balls-to-each-box/README.md b/algorithms/001769-minimum-number-of-operations-to-move-all-balls-to-each-box/README.md
new file mode 100644
index 000000000..ed2902915
--- /dev/null
+++ b/algorithms/001769-minimum-number-of-operations-to-move-all-balls-to-each-box/README.md
@@ -0,0 +1,143 @@
+1769\. Minimum Number of Operations to Move All Balls to Each Box
+
+**Difficulty:** Medium
+
+**Topics:** `Array`, `String`, `Prefix Sum`
+
+You have `n` boxes. You are given a binary string `boxes` of length `n`, where `boxes[i]` is `'0'` if the ith
box is **empty**, and `'1'` if it contains **one** ball.
+
+In one operation, you can move **one** ball from a box to an adjacent box. Box `i` is adjacent to box `j` if `abs(i - j) == 1`. Note that after doing so, there may be more than one ball in some boxes.
+
+Return _an array `answer` of size `n`, where `answer[i]` is the **minimum** number of operations needed to move all the balls to the ith
box_.
+
+Each `answer[i]` is calculated considering the initial state of the boxes.
+
+**Example 1:**
+
+- **Input:** boxes = "110"
+- **Output:** [1,1,3]
+- **Explanation:** The answer for each box is as follows:
+ 1) First box: you will have to move one ball from the second box to the first box in one operation.
+ 2) Second box: you will have to move one ball from the first box to the second box in one operation.
+ 3) Third box: you will have to move one ball from the first box to the third box in two operations, and move one ball from the second box to the third box in one operation.
+
+**Example 2:**
+
+- **Input:** boxes = "001011"
+- **Output:** [11,8,5,4,3,4]
+
+
+
+**Constraints:**
+
+- `n == boxes.length`
+- `1 <= n <= 2000`
+- `boxes[i]` is either `'0'` or `'1'`.
+
+
+**Hint:**
+1. If you want to move a ball from box `i` to box `j`, you'll need `abs(i-j)` moves.
+2. To move all balls to some box, you can move them one by one.
+3. For each box `i`, iterate on each ball in a box `j`, and add `abs(i-j)` to `answers[i]`.
+
+
+
+**Solution:**
+
+We can use a **prefix sum** approach that allows us to calculate the minimum number of operations needed to move all balls to each box without explicitly simulating each operation.
+
+### Key Observations:
+1. The number of moves required to move a ball from box `i` to box `j` is simply `abs(i - j)`.
+2. We can calculate the total number of moves to move all balls to a particular box by leveraging the positions of the balls and a running total of operations.
+3. By calculating moves from left to right and right to left, we can determine the result in two passes.
+
+### Approach:
+1. **Left-to-Right Pass**: In this pass, calculate the number of moves to bring all balls to the current box starting from the left.
+2. **Right-to-Left Pass**: In this pass, calculate the number of moves to bring all balls to the current box starting from the right.
+3. Combine the results of both passes to get the final result for each box.
+
+### Solution Steps:
+1. Start by iterating through the `boxes` string and counting how many balls are to the left and to the right of each box.
+2. During the iteration, calculate the number of moves required to bring all balls to the current box using both the left and right information.
+
+Let's implement this solution in PHP: **[1769. Minimum Number of Operations to Move All Balls to Each Box](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/001769-minimum-number-of-operations-to-move-all-balls-to-each-box/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+1. **Left-to-right pass**: We calculate the total number of operations required to bring all balls from the left side to the current box. For each ball found (`'1'`), we update the total number of moves.
+2. **Right-to-left pass**: Similar to the left-to-right pass, but we calculate the number of operations to move balls from the right side to the current box.
+3. The total number of operations for each box is the sum of moves from the left and right passes.
+
+### Example Walkthrough:
+
+#### Example 1:
+```php
+$boxes = "110";
+print_r(minOperations($boxes));
+```
+**Output**:
+```
+Array
+(
+ [0] => 1
+ [1] => 1
+ [2] => 3
+)
+```
+
+#### Example 2:
+```php
+$boxes = "001011";
+print_r(minOperations($boxes));
+```
+**Output**:
+```
+Array
+(
+ [0] => 11
+ [1] => 8
+ [2] => 5
+ [3] => 4
+ [4] => 3
+ [5] => 4
+)
+```
+
+### Time Complexity:
+- The solution runs in `O(n)` time because we iterate over the `boxes` string twice (once for the left-to-right pass and once for the right-to-left pass).
+- The space complexity is `O(n)` because we store the `answer` array to hold the results.
+
+This solution efficiently computes the minimum number of operations for each box using the prefix sum technique.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
diff --git a/algorithms/001769-minimum-number-of-operations-to-move-all-balls-to-each-box/solution.php b/algorithms/001769-minimum-number-of-operations-to-move-all-balls-to-each-box/solution.php
new file mode 100644
index 000000000..368b2e754
--- /dev/null
+++ b/algorithms/001769-minimum-number-of-operations-to-move-all-balls-to-each-box/solution.php
@@ -0,0 +1,37 @@
+= 0; $i--) {
+ $answer[$i] += $rightMoves;
+ if ($boxes[$i] == '1') {
+ $rightCount++;
+ }
+ $rightMoves += $rightCount;
+ }
+
+ return $answer;
+ }
+}
\ No newline at end of file
diff --git a/algorithms/001792-maximum-average-pass-ratio/README.md b/algorithms/001792-maximum-average-pass-ratio/README.md
new file mode 100644
index 000000000..79adedfeb
--- /dev/null
+++ b/algorithms/001792-maximum-average-pass-ratio/README.md
@@ -0,0 +1,149 @@
+1792\. Maximum Average Pass Ratio
+
+**Difficulty:** Medium
+
+**Topics:** `Array`, `Greedy`, `Heap (Priority Queue)`
+
+There is a school that has classes of students and each class will be having a final exam. You are given a 2D integer array `classes`, where classes[i] = [passi, totali]
. You know beforehand that in the ith
class, there are totali
total students, but only passi
number of students will pass the exam.
+
+You are also given an integer `extraStudents`. There are another `extraStudents` brilliant students that are **guaranteed** to pass the exam of any class they are assigned to. You want to assign each of the `extraStudents` students to a class in a way that **maximizes** the **average** pass ratio across **all** the classes.
+
+The **pass ratio** of a class is equal to the number of students of the class that will pass the exam divided by the total number of students of the class. The **average pass ratio** is the sum of pass ratios of all the classes divided by the number of the classes.
+
+Return the **maximum** possible average pass ratio after assigning the `extraStudents` students. Answers within 10-5
of the actual answer will be accepted.
+
+**Example 1:**
+
+- **Input:** classes = [[1,2],[3,5],[2,2]], extraStudents = 2
+- **Output:** 0.78333
+- **Explanation:** You can assign the two extra students to the first class. The average pass ratio will be equal to (3/4 + 3/5 + 2/2) / 3 = 0.78333.
+
+**Example 2:**
+
+- **Input:** classes = [[2,4],[3,9],[4,5],[2,10]], extraStudents = 4
+- **Output:** 4
+- **Explanation:** 0.53485
+
+
+
+**Constraints:**
+
+- 1 <= classes.length <= 105
+- `classes[i].length == 2`
+- 1 <= passi <= totali <= 105
+- 1 <= extraStudents <= 105
+
+
+**Hint:**
+1. Pay attention to how much the pass ratio changes when you add a student to the class. If you keep adding students, what happens to the change in pass ratio? The more students you add to a class, the smaller the change in pass ratio becomes.
+2. Since the change in the pass ratio is always decreasing with the more students you add, then the very first student you add to each class is the one that makes the biggest change in the pass ratio.
+3. Because each class's pass ratio is weighted equally, it's always optimal to put the student in the class that makes the biggest change among all the other classes.
+4. Keep a max heap of the current class sizes and order them by the change in pass ratio. For each extra student, take the top of the heap, update the class size, and put it back in the heap.
+
+
+**Solution:**
+
+We can use a **max-heap (priority queue)**. This is because we need to efficiently find the class that benefits the most (maximizes the change in pass ratio) when adding an extra student.
+
+### Approach:
+
+1. **Understand the Gain Calculation**:
+ - When adding one student to a class, the change in the pass ratio can be calculated as:
+ `gain = (pass + 1)/(total + 1) - pass/total`
+ - The task is to maximize the sum of pass ratios across all classes by distributing `extraStudents` optimally.
+
+2. **Use a Max-Heap**:
+ - For each class, calculate the initial gain and insert it into a max-heap along with the class details.
+ - Each heap element is a tuple: `[negative gain, pass, total]`. (We use a negative gain because PHP's `SplPriorityQueue` is a **min-heap** by default.)
+
+3. **Iteratively Distribute Extra Students**:
+ - Pop the class with the maximum gain from the heap.
+ - Add one student to that class, recalculate the gain, and push it back into the heap.
+ - Repeat until all `extraStudents` are distributed.
+
+4. **Calculate the Final Average**:
+ - Once all extra students are assigned, calculate the average pass ratio of all classes.
+
+Let's implement this solution in PHP: **[1792. Maximum Average Pass Ratio](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/001792-maximum-average-pass-ratio/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+
+1. **Heap Setup**:
+ - We use a max-heap (priority queue) to prioritize classes based on their potential improvement in pass ratio when an extra student is added.
+ - In PHP, `SplPriorityQueue` is used for the heap. The higher the priority value, the earlier the class is processed.
+
+2. **Distributing Extra Students**:
+ - For each extra student, we extract the class with the highest potential improvement from the heap.
+ - After adding one student to that class, we recalculate its potential improvement and reinsert it into the heap.
+
+3. **Final Average Calculation**:
+ - After distributing all extra students, we calculate the total pass ratio for all classes and return the average.
+
+4. **Precision**:
+ - The calculations are performed using floating-point arithmetic, which ensures answers are accurate to `10^-5` as required.
+
+### Complexity:
+
+- **Time Complexity**:
+ - Heap insertion and extraction take _**O(log N)**_, where _**N**_ is the number of classes.
+ - For _**extraStudents**_ iterations, the complexity is _**O(extraStudents x log N)**_.
+ - The final pass ratio summation is _**O(N)**_.
+
+- **Space Complexity**:
+ - The heap stores _**N**_ elements, so the space complexity is _**O(N)**_.
+
+This implementation efficiently distributes the extra students and computes the maximum average pass ratio.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
diff --git a/algorithms/001792-maximum-average-pass-ratio/solution.php b/algorithms/001792-maximum-average-pass-ratio/solution.php
new file mode 100644
index 000000000..9e596ef21
--- /dev/null
+++ b/algorithms/001792-maximum-average-pass-ratio/solution.php
@@ -0,0 +1,53 @@
+extraPassRatio($pass, $total);
+ $maxHeap->insert([$pass, $total], $extraRatio);
+ }
+
+ // Distribute the extra students
+ for ($i = 0; $i < $extraStudents; ++$i) {
+ list($pass, $total) = $maxHeap->extract();
+ $pass += 1;
+ $total += 1;
+ $extraRatio = $this->extraPassRatio($pass, $total);
+ $maxHeap->insert([$pass, $total], $extraRatio);
+ }
+
+ // Calculate the average pass ratio
+ $totalRatio = 0;
+ $count = count($classes);
+
+ while (!$maxHeap->isEmpty()) {
+ list($pass, $total) = $maxHeap->extract();
+ $totalRatio += $pass / $total;
+ }
+
+ return $totalRatio / $count;
+ }
+
+ /**
+ * Calculate the extra pass ratio when a student is added to the class
+ *
+ * @param $pass
+ * @param $total
+ * @return float|int
+ */
+ private function extraPassRatio($pass, $total)
+ {
+ return ($pass + 1) / ($total + 1) - $pass / $total;
+ }
+}
diff --git a/algorithms/001813-sentence-similarity-iii/README.md b/algorithms/001813-sentence-similarity-iii/README.md
new file mode 100644
index 000000000..7188f14be
--- /dev/null
+++ b/algorithms/001813-sentence-similarity-iii/README.md
@@ -0,0 +1,115 @@
+1813\. Sentence Similarity III
+
+**Difficulty:** Medium
+
+**Topics:** `Array`, `Two Pointers`, `String`
+
+You are given two strings `sentence1` and `sentence2`, each representing a **sentence** composed of words. A sentence is a list of **words** that are separated by a **single** space with no leading or trailing spaces. Each word consists of only uppercase and lowercase English characters.
+
+Two sentences `s1` and `s2` are considered **similar** if it is possible to insert an arbitrary sentence (_possibly empty_) inside one of these sentences such that the two sentences become equal. **Note** that the inserted sentence must be separated from existing words by spaces.
+
+For example,
+
+- `s1 = "Hello Jane"` and `s2 = "Hello my name is Jane"` can be made equal by inserting `"my name is"` between `"Hello"` and `"Jane"` in s1.
+- `s1 = "Frog cool"` and `s2 = "Frogs are cool"` are not similar, since although there is a sentence `"s are"` inserted into `s1`, it is not separated from `"Frog"` by a space.
+
+Given two sentences `sentence1` and `sentence2`, return **true** if `sentence1` and `sentence2` are **similar**. Otherwise, return **false**.
+
+**Example 1:**
+
+- **Input:** sentence1 = "My name is Haley", sentence2 = "My Haley"
+- **Output:** true
+- **Explanation:** `sentence2` can be turned to `sentence1` by inserting "name is" between "My" and "Haley".
+
+**Example 2:**
+
+- **Input:** sentence1 = "of", sentence2 = "A lot of words"
+- **Output:** false
+- **Explanation:** No single sentence can be inserted inside one of the sentences to make it equal to the other.
+
+
+**Example 3:**
+
+- **Input:** sentence1 = "Eating right now", sentence2 = "Eating"
+- **Output:** true
+- **Explanation:** `sentence2` can be turned to `sentence1` by inserting "right now" at the end of the sentence.
+
+
+
+**Constraints:**
+
+- `1 <= sentence1.length, sentence2.length <= 100`
+- `sentence1` and `sentence2` consist of lowercase and uppercase English letters and spaces.
+- The words in `sentence1` and `sentence2` are separated by a single space.
+
+
+**Hint:**
+1. One way to look at it is to find one sentence as a concatenation of a prefix and suffix from the other sentence.
+2. Get the longest common prefix between them and the longest common suffix.
+
+
+
+**Solution:**
+
+We can approach it by comparing the longest common prefix and suffix of both sentences. If the words in the remaining part of one sentence are completely contained within the other sentence (possibly empty), then the sentences can be considered similar.
+
+### Steps:
+
+1. Split both sentences into arrays of words.
+2. Use two pointers to compare the longest common prefix from the start of both arrays.
+3. Use another two pointers to compare the longest common suffix from the end of both arrays.
+4. After comparing the common prefix and suffix, if the remaining words in one of the sentences form an empty array (meaning they have all been matched), then the sentences are considered similar.
+
+Let's implement this solution in PHP: **[1813. Sentence Similarity III](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/001813-sentence-similarity-iii/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+1. **Splitting sentences into words:** We use `explode()` to split the sentence strings into arrays of words.
+2. **Common prefix comparison:** We iterate over both arrays from the start and compare corresponding words. The loop continues as long as the words match.
+3. **Common suffix comparison:** We compare the words from the end of both arrays, again using a loop to check for matching words.
+4. **Final check:** After processing the common prefix and suffix, we check if the number of words that have been matched (prefix + suffix) covers all the words in the shorter sentence. If so, the sentences can be considered similar.
+
+### Time Complexity:
+- The time complexity is **O(n + m)**, where `n` and `m` are the lengths of `sentence1` and `sentence2` respectively. This is because we process the words in both sentences only once while checking the common prefix and suffix.
+
+This solution should work efficiently given the constraints.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
diff --git a/algorithms/001813-sentence-similarity-iii/solution.php b/algorithms/001813-sentence-similarity-iii/solution.php
new file mode 100644
index 000000000..d274ca88d
--- /dev/null
+++ b/algorithms/001813-sentence-similarity-iii/solution.php
@@ -0,0 +1,34 @@
+= min($n1, $n2);
+ }
+}
\ No newline at end of file
diff --git a/algorithms/001829-maximum-xor-for-each-query/README.md b/algorithms/001829-maximum-xor-for-each-query/README.md
new file mode 100644
index 000000000..690128765
--- /dev/null
+++ b/algorithms/001829-maximum-xor-for-each-query/README.md
@@ -0,0 +1,133 @@
+1829\. Maximum XOR for Each Query
+
+**Difficulty:** Medium
+
+**Topics:** `Array`, `Bit Manipulation`, `Prefix Sum`
+
+You are given a **sorted** array `nums` of `n` non-negative integers and an integer `maximumBit`. You want to perform the following query `n` **times**:
+
+- Find a non-negative integer k < 2maximumBit
such that `nums[0] XOR nums[1] XOR ... XOR nums[nums.length-1] XOR k` is **maximized**. `k` is the answer to the ith
query.
+- Remove the **last** element from the current array `nums`.
+
+Return _an array `answer`, where `answer[i]` is the answer to the ith
query_.
+
+**Example 1:**
+
+- **Input:** nums = [0,1,1,3], maximumBit = 2
+- **Output:** [0,3,2,3]
+- **Explanation:** The queries are answered as follows:
+ 1stst query: nums = [0,1,1,3], k = 0 since 0 XOR 1 XOR 1 XOR 3 XOR 0 = 3.
+ 2nd query: nums = [0,1,1], k = 3 since 0 XOR 1 XOR 1 XOR 3 = 3.
+ 3rd query: nums = [0,1], k = 2 since 0 XOR 1 XOR 2 = 3.
+ 4th query: nums = [0], k = 3 since 0 XOR 3 = 3.
+
+**Example 2:**
+
+- **Input:** nums = [2,3,4,7], maximumBit = 3
+- **Output:** [5,2,6,5]
+- **Explanation:** The queries are answered as follows:
+ 1stst query: nums = [2,3,4,7], k = 5 since 2 XOR 3 XOR 4 XOR 7 XOR 5 = 7.
+ 2nd query: nums = [2,3,4], k = 2 since 2 XOR 3 XOR 4 XOR 2 = 7.
+ 3rd query: nums = [2,3], k = 6 since 2 XOR 3 XOR 6 = 7.
+ 4th query: nums = [2], k = 5 since 2 XOR 5 = 7.
+
+
+**Example 3:**
+
+- **Input:** nums = [0,1,2,2,5,7], maximumBit = 3
+- **Output:** [4,3,6,4,6,7]
+
+
+
+**Constraints:**
+
+- `nums.length == n`
+- 1 <= n <= 105
+- `1 <= maximumBit <= 20`
+- 0 <= nums[i] < 2maximumBit
+- `numsβββ` is sorted in **ascending** order.
+
+
+**Hint:**
+1. Note that the maximum possible XOR result is always 2(maximumBit) - 1
+2. So the answer for a prefix is the XOR of that prefix XORed with 2(maximumBit)-1
+
+
+
+**Solution:**
+
+We need to efficiently calculate the XOR of elements in the array and maximize the result using a value `k` such that `k` is less than `2^maximumBit`. Here's the approach for solving this problem:
+
+### Observations and Approach
+
+1. **Maximizing XOR**:
+ The maximum number we can XOR with any prefix sum for `maximumBit` bits is \( 2^{\text{maximumBit}} - 1 \). This is because XORing with a number of all `1`s (i.e., `111...1` in binary) will always maximize the result.
+
+2. **Prefix XOR Calculation**:
+ Instead of recalculating the XOR for each query, we can maintain a cumulative XOR for the entire array. Since XOR has the property that `A XOR B XOR B = A`, removing the last element from the array can be achieved by XORing out that element from the cumulative XOR.
+
+3. **Algorithm**:
+ - Compute the XOR of all elements in `nums` initially. Let's call this `currentXOR`.
+ - For each query (from last to first):
+ - Calculate the optimal value of `k` for that query by XORing `currentXOR` with `maxNum` where `maxNum = 2^maximumBit - 1`.
+ - Append `k` to the result list.
+ - Remove the last element from `nums` by XORing it out of `currentXOR`.
+ - The result list will contain the answers in reverse order, so reverse it at the end.
+
+Let's implement this solution in PHP: **[1829. Maximum XOR for Each Query](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/001829-maximum-xor-for-each-query/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+1. **Calculate `maxNum`**:
+ - `maxNum` is calculated as `2^maximumBit - 1`, which is the number with all `1`s in binary for the specified bit length.
+
+2. **Initial XOR Calculation**:
+ - We XOR all elements in `nums` to get the cumulative XOR (`currentXOR`), representing the XOR of all numbers in the array.
+
+3. **Iterate Backwards**:
+ - We start from the last element in `nums` and calculate the maximum XOR for each step:
+ - `currentXOR ^ maxNum` gives the maximum `k` for the current state.
+ - Append `k` to `answer`.
+ - We then XOR the last element of `nums` with `currentXOR` to "remove" it from the XOR sum for the next iteration.
+
+4. **Return the Answer**:
+ - Since we processed the list in reverse, `answer` will contain the values in reverse order, so the final list is already arranged correctly for our requirements.
+
+### Complexity Analysis
+
+- **Time Complexity**: _**O(n)**_, since we compute the initial XOR in _**O(n)**_ and each query is processed in constant time.
+- **Space Complexity**: _**O(n)**_, for storing the `answer`.
+
+This code is efficient and should handle the upper limits of the constraints well.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
diff --git a/algorithms/001829-maximum-xor-for-each-query/solution.php b/algorithms/001829-maximum-xor-for-each-query/solution.php
new file mode 100644
index 000000000..be2f9879a
--- /dev/null
+++ b/algorithms/001829-maximum-xor-for-each-query/solution.php
@@ -0,0 +1,32 @@
+= 0; $i--) {
+ // Find k that maximizes the XOR result
+ $answer[] = $currentXOR ^ $maxNum;
+
+ // Remove the last element from currentXOR by XORing it out
+ $currentXOR ^= $nums[$i];
+ }
+
+ return $answer; // We populated answer in reverse order
+ }
+}
\ No newline at end of file
diff --git a/algorithms/001861-rotating-the-box/README.md b/algorithms/001861-rotating-the-box/README.md
new file mode 100644
index 000000000..470e2fd18
--- /dev/null
+++ b/algorithms/001861-rotating-the-box/README.md
@@ -0,0 +1,175 @@
+1861\. Rotating the Box
+
+**Difficulty:** Medium
+
+**Topics:** `Array`, `Two Pointers`, `Matrix`
+
+You are given an `m x n` matrix of characters `box` representing a side-view of a box. Each cell of the box is one of the following:
+
+- A stone `'#'`
+- A stationary obstacle `'*'`
+- Empty `'.'`
+
+The box is rotated **90 degrees clockwise**, causing some of the stones to fall due to gravity. Each stone falls down until it lands on an obstacle, another stone, or the bottom of the box. Gravity **does not** affect the obstacles' positions, and the inertia from the box's rotation **does not** affect the stones' horizontal positions.
+
+It is **guaranteed** that each stone in `box` rests on an obstacle, another stone, or the bottom of the box.
+
+Return _an `n x m` matrix representing the box after the rotation described above_.
+
+
+**Example 1:**
+
+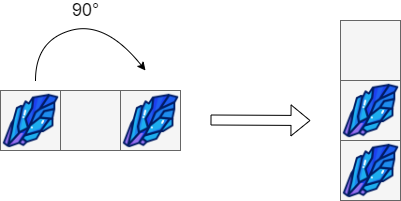
+
+- **Input:** box = [["#",".","#"]]
+- **Output:** [["."], ["#"], ["#"]]
+
+**Example 2:**
+
+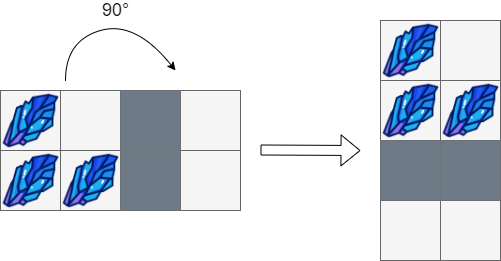
+
+- **Input:** box = [["#",".","*","."], ["#","#","*","."]]
+- **Output:** [["#","."], ["#","#"], ["*","*"], [".","."]]
+
+
+**Example 3:**
+
+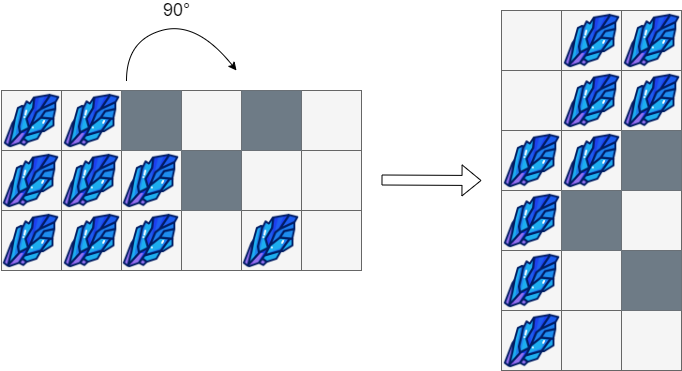
+
+- **Input:** box = [["#","#","*",".","*","."], ["#","#","#","*",".","."], ["#","#","#",".","#","."]]
+- **Output:** [[".","#","#"], [".","#","#"], ["#","#","*"], ["#","*","."], ["#",".","*"], ["#",".","."]]
+
+
+
+**Constraints:**
+
+- `m == box.length`
+- `n == box[i].length`
+- `1 <= m, n <= 500`
+- `box[i][j]` is either `'#'`, `'*'`, or `'.'`.
+
+
+**Hint:**
+1. Rotate the box using the relation `rotatedBox[i][j] = box[m - 1 - j][i]`.
+2. Start iterating from the bottom of the box and for each empty cell check if there is any stone above it with no obstacles between them.
+
+
+
+**Solution:**
+
+We need to follow a few distinct steps:
+
+1. **Rotate the box**: We first rotate the matrix 90 degrees clockwise. The rotated matrix will have `n` rows and `m` columns, where `n` is the number of columns in the original box, and `m` is the number of rows.
+
+2. **Gravity effect**: After rotating, we need to simulate the effect of gravity. This means that all stones (`'#'`) should "fall" to the bottom of their new column, stopping only when they encounter an obstacle (`'*'`) or another stone (`'#'`).
+
+### Approach:
+
+1. **Rotation**: After the rotation, the element at position `[i][j]` in the original matrix will be placed at position `[j][m-1-i]` in the rotated matrix.
+
+2. **Gravity simulation**: We need to process each column from bottom to top. If there is a stone (`'#'`), it will fall down until it reaches an obstacle or the bottom. If the cell is empty (`'.'`), it can hold a stone.
+
+### Step-by-step explanation:
+
+1. **Create a new matrix for the rotated box**.
+2. **Iterate through each column of the rotated matrix (after rotation)**.
+3. **Simulate gravity for each column** by starting from the bottom and moving upwards. Place stones (`'#'`) as far down as possible, leaving obstacles (`'*'`) in place.
+4. **Return the final rotated matrix**.
+
+Let's implement this solution in PHP: **[1861. Rotating the Box](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/001861-rotating-the-box/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+1. **Simulate Gravity**:
+ - Traverse each row from right to left. Use a pointer (`emptySlot`) to track where the next stone should fall.
+ - If a stone (`#`) is encountered, move it to the `emptySlot`, and then decrement `emptySlot`.
+ - If an obstacle (`*`) is encountered, reset `emptySlot` to the position just before the obstacle.
+
+2. **Rotate the Matrix**:
+ - Create a new matrix where the element at `[i][j]` in the rotated box is taken from `[m - 1 - j][i]` of the original box.
+
+### Example Output
+
+#### Input:
+```php
+$box = [
+ ["#", ".", "#"],
+];
+```
+#### Output:
+```php
+[
+ [".",],
+ ["#",],
+ ["#",],
+]
+```
+
+#### Input:
+```php
+$box = [
+ ["#", ".", "*", "."],
+ ["#", "#", "*", "."],
+];
+```
+#### Output:
+```php
+[
+ ["#", "."],
+ ["#", "#"],
+ ["*", "*"],
+ [".", "."],
+]
+```
+
+### Time Complexity
+1. Gravity simulation: _**O(m x n)**_, as we iterate through each element in the matrix.
+2. Rotation: _**O(m x n)**_, as we create the rotated matrix.
+
+**Total**: _**O(m x n)**_.
+
+### Space Complexity
+- _**O(m x n)**_ for the rotated matrix.
+
+This solution is efficient and adheres to the constraints of the problem.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
\ No newline at end of file
diff --git a/algorithms/001861-rotating-the-box/solution.php b/algorithms/001861-rotating-the-box/solution.php
new file mode 100644
index 000000000..a825013b3
--- /dev/null
+++ b/algorithms/001861-rotating-the-box/solution.php
@@ -0,0 +1,41 @@
+= 0; $j--) {
+ if ($box[$i][$j] === '#') {
+ // Move stone to the lowest possible position
+ $box[$i][$j] = '.';
+ $box[$i][$emptySlot] = '#';
+ $emptySlot--;
+ } elseif ($box[$i][$j] === '*') {
+ // Reset emptySlot to just above the obstacle
+ $emptySlot = $j - 1;
+ }
+ }
+ }
+
+ // Step 2: Rotate the box 90 degrees clockwise
+ $rotatedBox = [];
+ for ($j = 0; $j < $n; $j++) {
+ $newRow = [];
+ for ($i = $m - 1; $i >= 0; $i--) {
+ $newRow[] = $box[$i][$j];
+ }
+ $rotatedBox[] = $newRow;
+ }
+
+ return $rotatedBox;
+ }
+}
\ No newline at end of file
diff --git a/algorithms/001894-find-the-student-that-will-replace-the-chalk/README.md b/algorithms/001894-find-the-student-that-will-replace-the-chalk/README.md
new file mode 100644
index 000000000..69b104c55
--- /dev/null
+++ b/algorithms/001894-find-the-student-that-will-replace-the-chalk/README.md
@@ -0,0 +1,143 @@
+1894\. Find the Student that Will Replace the Chalk
+
+**Difficulty:** Medium
+
+**Topics:** `Array`, `Binary Search`, `Simulation`, `Prefix Sum`
+
+There are `n` students in a class numbered from `0` to `n - 1`. The teacher will give each student a problem starting with the student number `0`, then the student number `1`, and so on until the teacher reaches the student number `n - 1`. After that, the teacher will restart the process, starting with the student number `0` again.
+
+You are given a **0-indexed** integer array `chalk` and an integer `k`. There are initially `k` pieces of chalk. When the student number `i` is given a problem to solve, they will use `chalk[i]` pieces of chalk to solve that problem. However, if the current number of chalk pieces is **strictly less** than `chalk[i]`, then the student number `i` will be asked to **replace** the chalk.
+
+Return _the **index** of the student that will **replace** the chalk pieces_.
+
+**Example 1:**
+
+- **Input:** chalk = [5,1,5], k = 22
+- **Output:** 0
+- **Explanation:** The students go in turns as follows:
+ - Student number 0 uses 5 chalk, so k = 17.
+ - Student number 1 uses 1 chalk, so k = 16.
+ - Student number 2 uses 5 chalk, so k = 11.
+ - Student number 0 uses 5 chalk, so k = 6.
+ - Student number 1 uses 1 chalk, so k = 5.
+ - Student number 2 uses 5 chalk, so k = 0.
+ - Student number 0 does not have enough chalk, so they will have to replace it.
+
+**Example 2:**
+
+- **Input:** chalk = [3,4,1,2], k = 25
+- **Output:** 1
+- **Explanation:** The students go in turns as follows:
+ - Student number 0 uses 3 chalk so k = 22.
+ - Student number 1 uses 4 chalk so k = 18.
+ - Student number 2 uses 1 chalk so k = 17.
+ - Student number 3 uses 2 chalk so k = 15.
+ - Student number 0 uses 3 chalk so k = 12.
+ - Student number 1 uses 4 chalk so k = 8.
+ - Student number 2 uses 1 chalk so k = 7.
+ - Student number 3 uses 2 chalk so k = 5.
+ - Student number 0 uses 3 chalk so k = 2.
+ - Student number 1 does not have enough chalk, so they will have to replace it.
+
+**Constraints:**
+
+- chalk.length == n
+- 1 <= n <= 105
+- 1 <= chalk[i] <= 105
+- 1 <= k <= 109
+
+
+**Hint:**
+1. Subtract the sum of chalk from k until k is less than the sum of chalk.
+2. Now iterate over the array. If chalk[i] is less than k, this is the answer. Otherwise, subtract chalk[i] from k and continue.
+
+
+
+**Solution:**
+
+Let's break down the problem step by step:
+
+### Approach:
+
+1. **Total Chalk Consumption:**
+ First, calculate the total amount of chalk needed for one complete round (from student `0` to student `n-1`). This will help us reduce the value of `k` by taking into account how many complete rounds can be covered by `k` pieces of chalk.
+
+2. **Reduce `k` by Modulo:**
+ If `k` is larger than the total chalk required for one complete round, we can simplify the problem by taking `k % total_chalk`. This operation will give us the remaining chalk after as many full rounds as possible, leaving us with a smaller problem to solve.
+
+3. **Find the Student Who Runs Out of Chalk:**
+ Iterate through each student's chalk consumption, subtracting it from `k` until `k` becomes less than the current student's chalk requirement. The index of this student is our answer.
+
+### Example Walkthrough:
+
+Let's take an example `chalk = [3, 4, 1, 2]` and `k = 25`:
+
+1. **Total Chalk Consumption:**
+ ```
+ text{total_chalk} = 3 + 4 + 1 + 2 = 10
+ ```
+
+2. **Reduce `k`:**
+ ```
+ k % 10 = 25 % 10 = 5
+ ```
+ Now we have `k = 5` after subtracting as many full rounds as possible.
+
+3. **Find the Student:**
+ - Student `0` uses `3` chalk, so `k = 5 - 3 = 2`.
+ - Student `1` requires `4` chalk, but `k = 2`, which is less than `4`.
+ - Therefore, student `1` will be the one who needs to replace the chalk.
+
+Let's implement this solution in PHP: **[1894. Find the Student that Will Replace the Chalk](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/001894-find-the-student-that-will-replace-the-chalk/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+1. **Total Chalk Sum:** We sum up all the chalk requirements to get the total for one complete round.
+2. **Modulo Operation:** Using modulo with `k`, we get the effective number of chalks to distribute after full rounds.
+3. **Find the Student:** We then iterate through the students, checking if the remaining chalk is sufficient. The first time it's insufficient, that student's index is the answer.
+
+### Complexity:
+- **Time Complexity:** O(n) β we sum the array and then iterate through it once.
+- **Space Complexity:** O(1) β only a few variables are used, independent of the input size.
+
+This approach ensures that the problem is solved efficiently even for large inputs.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
+
diff --git a/algorithms/001894-find-the-student-that-will-replace-the-chalk/solution.php b/algorithms/001894-find-the-student-that-will-replace-the-chalk/solution.php
new file mode 100644
index 000000000..6157f4e06
--- /dev/null
+++ b/algorithms/001894-find-the-student-that-will-replace-the-chalk/solution.php
@@ -0,0 +1,27 @@
+= $m || $j >= $n || $grid2[$i][$j] == 0) {
+ return true;
+ }
+
+ $grid2[$i][$j] = 0; // Mark the cell as visited in grid2
+ $isSubIsland = $grid1[$i][$j] == 1; // Check if it's a sub-island
+
+ // Explore all 4 directions
+ $isSubIsland &= dfs($grid1, $grid2, $i + 1, $j, $m, $n);
+ $isSubIsland &= dfs($grid1, $grid2, $i - 1, $j, $m, $n);
+ $isSubIsland &= dfs($grid1, $grid2, $i, $j + 1, $m, $n);
+ $isSubIsland &= dfs($grid1, $grid2, $i, $j - 1, $m, $n);
+
+ return $isSubIsland;
+}
+
+// Example usage:
+
+// Example 1
+$grid1 = [
+ [1,1,1,0,0],
+ [0,1,1,1,1],
+ [0,0,0,0,0],
+ [1,0,0,0,0],
+ [1,1,0,1,1]
+];
+
+$grid2 = [
+ [1,1,1,0,0],
+ [0,0,1,1,1],
+ [0,1,0,0,0],
+ [1,0,1,1,0],
+ [0,1,0,1,0]
+];
+
+echo countSubIslands($grid1, $grid2); // Output: 3
+
+// Example 2
+$grid1 = [
+ [1,0,1,0,1],
+ [1,1,1,1,1],
+ [0,0,0,0,0],
+ [1,1,1,1,1],
+ [1,0,1,0,1]
+];
+
+$grid2 = [
+ [0,0,0,0,0],
+ [1,1,1,1,1],
+ [0,1,0,1,0],
+ [0,1,0,1,0],
+ [1,0,0,0,1]
+];
+
+echo countSubIslands($grid1, $grid2); // Output: 2
+?>
+```
+
+### Explanation:
+
+- **DFS Function:** The `dfs` function explores the island in `grid2` and checks if the corresponding cells in `grid1` are all land cells. If any cell in `grid2` is land but the corresponding cell in `grid1` is water, the island in `grid2` is not a sub-island.
+- **Mark Visited:** As we traverse `grid2`, we mark cells as visited by setting them to `0`.
+- **Main Loop:** We iterate through all cells in `grid2`. Whenever we find a land cell that hasn't been visited, we initiate a DFS to check if it's part of a sub-island.
+
+### Time Complexity:
+The time complexity is \(O(m \times n)\) where `m` is the number of rows and `n` is the number of columns. This is because we potentially visit every cell once.
+
+This solution should work efficiently within the given constraints.
+
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
diff --git a/algorithms/001905-count-sub-islands/solution.php b/algorithms/001905-count-sub-islands/solution.php
new file mode 100644
index 000000000..55e678fa7
--- /dev/null
+++ b/algorithms/001905-count-sub-islands/solution.php
@@ -0,0 +1,52 @@
+dfs($grid1, $grid2, $i, $j, $m, $n)) {
+ $count++;
+ }
+ }
+ }
+
+ return $count;
+ }
+
+ /**
+ * @param $grid1
+ * @param $grid2
+ * @param $i
+ * @param $j
+ * @param $m
+ * @param $n
+ * @return int|true
+ */
+ function dfs(&$grid1, &$grid2, $i, $j, $m, $n) {
+ if ($i < 0 || $j < 0 || $i >= $m || $j >= $n || $grid2[$i][$j] == 0) {
+ return true;
+ }
+
+ $grid2[$i][$j] = 0; // Mark the cell as visited in grid2
+ $isSubIsland = $grid1[$i][$j] == 1; // Check if it's a sub-island
+
+ // Explore all 4 directions
+ $isSubIsland &= self::dfs($grid1, $grid2, $i + 1, $j, $m, $n);
+ $isSubIsland &= self::dfs($grid1, $grid2, $i - 1, $j, $m, $n);
+ $isSubIsland &= self::dfs($grid1, $grid2, $i, $j + 1, $m, $n);
+ $isSubIsland &= self::dfs($grid1, $grid2, $i, $j - 1, $m, $n);
+
+ return $isSubIsland;
+ }
+
+}
\ No newline at end of file
diff --git a/algorithms/001930-unique-length-3-palindromic-subsequences/README.md b/algorithms/001930-unique-length-3-palindromic-subsequences/README.md
new file mode 100644
index 000000000..5cde2a3ab
--- /dev/null
+++ b/algorithms/001930-unique-length-3-palindromic-subsequences/README.md
@@ -0,0 +1,138 @@
+1930\. Unique Length-3 Palindromic Subsequences
+
+**Difficulty:** Medium
+
+**Topics:** `Hash Table`, `String`, `Bit Manipulation`, `Prefix Sum`
+
+Given a string `s`, return the number of **unique palindromes of length three** that are a **subsequence** of `s`.
+
+**Note** that even if there are multiple ways to obtain the same subsequence, it is still only counted once.
+
+A **palindrome** is a string that reads the same forwards and backwards.
+
+A **subsequence** of a string is a new string generated from the original string with some characters (can be none) deleted without changing the relative order of the remaining characters.
+
+- For example, `"ace"` is a subsequence of `"abcde"`.
+
+
+**Example 1:**
+
+- **Input:** s = "aabca"
+- **Output:** 3
+- **Explanation:** The 3 palindromic subsequences of length 3 are:
+ - "aba" (subsequence of "aabca")
+ - "aaa" (subsequence of "aabca")
+ - "aca" (subsequence of "aabca")
+
+**Example 2:**
+
+- **Input:** s = "adc"
+- **Output:** 0
+- **Explanation:** There are no palindromic subsequences of length 3 in "adc".
+
+
+**Example 3:**
+
+- **Input:** s = "bbcbaba"
+- **Output:** 4
+- **Explanation:** The 4 palindromic subsequences of length 3 are:
+ - "bbb" (subsequence of "bbcbaba")
+ - "bcb" (subsequence of "bbcbaba")
+ - "bab" (subsequence of "bbcbaba")
+ - "aba" (subsequence of "bbcbaba")
+
+
+
+**Constraints:**
+
+- 3 <= s.length <= 105
+- `s` consists of only lowercase English letters.
+
+
+**Hint:**
+1. What is the maximum number of length-3 palindromic strings?
+2. How can we keep track of the characters that appeared to the left of a given position?
+
+
+
+**Solution:**
+
+We can use an efficient algorithm that leverages prefix and suffix character tracking to count all valid palindromic subsequences.
+
+### Approach
+
+1. **Track Prefix Characters**:
+ Use an array to store the set of characters encountered to the left of each position in the string. This will help in efficiently checking if a character can form the first part of a palindromic subsequence.
+
+2. **Track Suffix Characters**:
+ Use another array to store the set of characters encountered to the right of each position in the string. This will help in efficiently checking if a character can form the third part of a palindromic subsequence.
+
+3. **Count Palindromic Subsequences**:
+ For each character in the string, consider it as the middle character of a length-3 palindrome. Check for all valid combinations of prefix and suffix characters to determine unique palindromes.
+
+4. **Store Results**:
+ Use a hash set to store unique palindromic subsequences, ensuring no duplicates.
+
+Let's implement this solution in PHP: **[1930. Unique Length-3 Palindromic Subsequences](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/001930-unique-length-3-palindromic-subsequences/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+1. **Prefix Array**:
+ - For each character at position `i`, `prefix[i]` stores all distinct characters encountered before index `i`.
+
+2. **Suffix Array**:
+ - For each character at position `i`, `suffix[i]` stores all distinct characters encountered after index `i`.
+
+3. **Middle Character**:
+ - Consider each character as the middle of the palindrome. For each combination of prefix and suffix characters that matches the middle character, form a length-3 palindrome.
+
+4. **Hash Map**:
+ - Use an associative array (`$uniquePalindromes`) to store unique palindromes, ensuring duplicates are not counted.
+
+### Complexity
+
+- **Time Complexity**: _**O(n)**_
+ - Traversing the string twice to compute the prefix and suffix arrays.
+ - A third traversal checks valid palindromic subsequences.
+
+- **Space Complexity**: _**O(n)**_
+ - For prefix and suffix arrays.
+
+### Output
+
+The code produces the correct results for the given examples:
+
+- **Input**: `"aabca"` β **Output**: `3`
+- **Input**: `"adc"` β **Output**: `0`
+- **Input**: `"bbcbaba"` β **Output**: `4`
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
diff --git a/algorithms/001930-unique-length-3-palindromic-subsequences/solution.php b/algorithms/001930-unique-length-3-palindromic-subsequences/solution.php
new file mode 100644
index 000000000..94dbc143e
--- /dev/null
+++ b/algorithms/001930-unique-length-3-palindromic-subsequences/solution.php
@@ -0,0 +1,44 @@
+= 0; $i--) {
+ $suffix[$i] = $seen;
+ $seen[$s[$i]] = true;
+ }
+
+ // Track unique palindromic subsequences
+ $uniquePalindromes = [];
+
+ // Check for each character as the middle character
+ for ($i = 1; $i < $n - 1; $i++) {
+ foreach ($prefix[$i] as $char1 => $true) {
+ if (isset($suffix[$i][$char1])) {
+ $palindrome = $char1 . $s[$i] . $char1;
+ $uniquePalindromes[$palindrome] = true;
+ }
+ }
+ }
+
+ // Return the count of unique palindromic subsequences
+ return count($uniquePalindromes);
+ }
+}
\ No newline at end of file
diff --git a/algorithms/001937-maximum-number-of-points-with-cost/README.md b/algorithms/001937-maximum-number-of-points-with-cost/README.md
new file mode 100644
index 000000000..3196102db
--- /dev/null
+++ b/algorithms/001937-maximum-number-of-points-with-cost/README.md
@@ -0,0 +1,114 @@
+1937\. Maximum Number of Points with Cost
+
+**Difficulty:** Medium
+
+**Topics:** `Array`, `Dynamic Programming`
+
+You are given an `m x n` integer matrix `points` (**0-indexed**). Starting with `0` points, you want to **maximize** the number of points you can get from the matrix.
+
+To gain points, you must pick one cell in **each row**. Picking the cell at coordinates `(r, c)` will **add** `points[r][c]` to your score.
+
+However, you will lose points if you pick a cell too far from the cell that you picked in the previous row. For every two adjacent rows `r` and `r + 1` (where `0 <= r < m - 1`), picking cells at coordinates (r, c1)
and (r + 1, c2)
will **subtract** abs(c1 - c2)
from your score.
+
+Return _the **maximum** number of points you can achieve_.
+
+`abs(x)` is defined as:
+
+- `x` for `x >= 0`.
+- `-x` for `x < 0`.
+
+
+**Example 1:**
+
+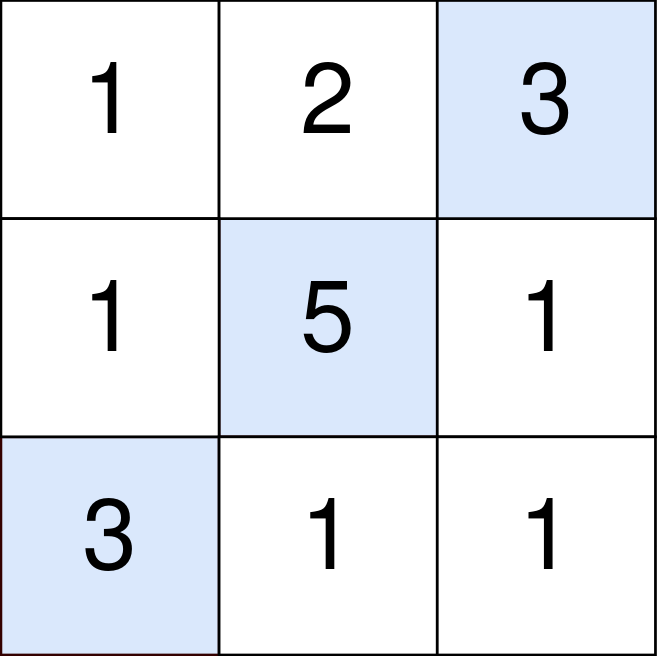
+
+- **Input:** l1 = [2,4,3], l2 = [5,6,4]
+- **Output:** 9
+- **Explanation:**\
+ The blue cells denote the optimal cells to pick, which have coordinates (0, 2), (1, 1), and (2, 0).\
+ You add 3 + 5 + 3 = 11 to your score.\
+ However, you must subtract abs(2 - 1) + abs(1 - 0) = 2 from your score.\
+ Your final score is 11 - 2 = 9.
+
+**Example 2:**
+
+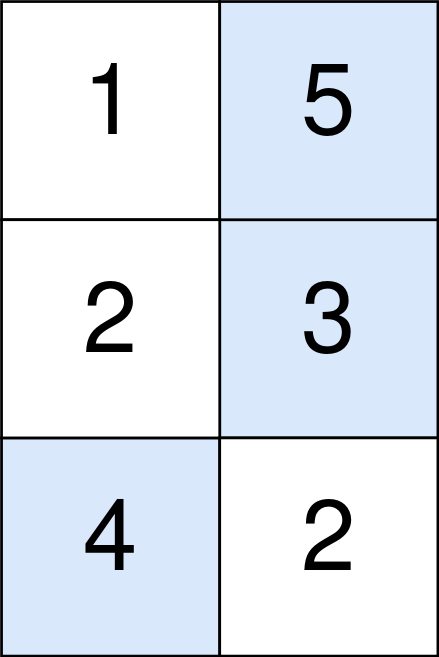
+
+- **Input:** points = [[1,5],[2,3],[4,2]]
+- **Output:** 11
+- **Explanation:**\
+ The blue cells denote the optimal cells to pick, which have coordinates (0, 1), (1, 1), and (2, 0).\
+ You add 5 + 3 + 4 = 12 to your score.\
+ However, you must subtract abs(1 - 1) + abs(1 - 0) = 1 from your score.\
+ Your final score is 12 - 1 = 11.
+
+**Constraints:**
+
+- `m == points.length`
+- `n == points[r].length`
+- 1 <= m, n <= 105
+- 1 <= m * n <= 105
+- 0 <= points[r][c] <= 105
+
+**Hint:**
+1. Try using dynamic programming.
+2. dp[i][j] is the maximum number of points you can have if points[i][j] is the most recent cell you picked.
+
+
+
+
+
+**Solution:**
+
+
+We can break down the solution into several steps:
+
+### Step 1: Define the DP Array
+We will use a 2D array `dp` where `dp[i][j]` represents the maximum points we can achieve by selecting the cell at row `i` and column `j`.
+
+### Step 2: Initialize the DP Array
+Initialize the first row of `dp` to be the same as the first row of `points` since there are no previous rows to subtract the cost.
+
+### Step 3: Calculate DP Values for Each Row
+For each subsequent row, we calculate the maximum possible points for each column considering the costs of switching from the previous row.
+
+To efficiently calculate the transition from row `i-1` to row `i`, we can use two auxiliary arrays `left` and `right`:
+
+- `left[j]` will store the maximum value we can achieve for the `j`-th column considering only transitions from the left.
+- `right[j]` will store the maximum value we can achieve for the `j`-th column considering only transitions from the right.
+
+### Step 4: Update DP for Each Row
+For each column `j` in row `i`:
+- Update `dp[i][j]` using the maximum of either `left[j]` or `right[j]` plus `points[i][j]`.
+
+### Step 5: Return the Maximum Value from the Last Row
+The result will be the maximum value in the last row of the `dp` array.
+
+
+Let's implement this solution in PHP: **[1937. Maximum Number of Points with Cost](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/001937-maximum-number-of-points-with-cost/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+- **`left` and `right` arrays:** These help us compute the maximum points we can gain for each cell by considering the previous row's values, efficiently accounting for the penalty from moving across columns.
+- **Dynamic programming approach:** This method ensures that each row is calculated based on the previous row, making the solution scalable for large matrices.
+
+This approach has a time complexity of \(O(m \times n)\), which is efficient given the constraints.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
diff --git a/algorithms/001937-maximum-number-of-points-with-cost/solution.php b/algorithms/001937-maximum-number-of-points-with-cost/solution.php
new file mode 100644
index 000000000..e269917a4
--- /dev/null
+++ b/algorithms/001937-maximum-number-of-points-with-cost/solution.php
@@ -0,0 +1,53 @@
+val = $val;
+ * $this->next = $next;
+ * }
+ * }
+ */
+class Solution
+{
+
+ /**
+ * @param ListNode $l1
+ * @param ListNode $l2
+ * @return ListNode
+ */
+ function addTwoNumbers(ListNode $l1, ListNode $l2): ListNode
+ {
+ $dummyHead = new ListNode(0);
+ $p = $l1;
+ $q = $l2;
+ $curr = $dummyHead;
+ $carry = 0;
+
+ while ($p !== null || $q !== null) {
+ $x = ($p !== null) ? $p->val : 0;
+ $y = ($q !== null) ? $q->val : 0;
+ $sum = $carry + $x + $y;
+ $carry = (int)($sum / 10);
+
+ $curr->next = new ListNode($sum % 10);
+ $curr = $curr->next;
+
+ if ($p !== null) {
+ $p = $p->next;
+ }
+ if ($q !== null) {
+ $q = $q->next;
+ }
+ }
+
+ if ($carry > 0) {
+ $curr->next = new ListNode($carry);
+ }
+
+ return $dummyHead->next;
+ }
+}
\ No newline at end of file
diff --git a/algorithms/001942-the-number-of-the-smallest-unoccupied-chair/README.md b/algorithms/001942-the-number-of-the-smallest-unoccupied-chair/README.md
new file mode 100644
index 000000000..59cb5c5fe
--- /dev/null
+++ b/algorithms/001942-the-number-of-the-smallest-unoccupied-chair/README.md
@@ -0,0 +1,198 @@
+1942\. The Number of the Smallest Unoccupied Chair
+
+**Difficulty:** Medium
+
+**Topics:** `Array`, `Hash Table`, `Heap (Priority Queue)`
+
+There is a party where `n` friends numbered from `0` to `n - 1` are attending. There is an **infinite** number of chairs in this party that are numbered from `0` to `infinity`. When a friend arrives at the party, they sit on the unoccupied chair with the `smallest number`.
+
+- For example, if chairs `0`, `1`, and `5` are occupied when a friend comes, they will sit on chair number `2`.
+
+When a friend leaves the party, their chair becomes unoccupied at the moment they leave. If another friend arrives at that same moment, they can sit in that chair.
+
+You are given a **0-indexed** 2D integer array `times` where times[i] = [arrivali, leavingi]
, indicating the arrival and leaving times of the ith
friend respectively, and an integer `targetFriend`. All arrival times are **distinct**.
+
+Return _the **chair number** that the friend numbered `targetFriend` will sit on_.
+
+**Example 1:**
+
+- **Input:** times = [[1,4],[2,3],[4,6]], targetFriend = 1
+- **Output:** 1
+- **Explanation:**
+ - Friend 0 arrives at time 1 and sits on chair 0.
+ - Friend 1 arrives at time 2 and sits on chair 1.
+ - Friend 1 leaves at time 3 and chair 1 becomes empty.
+ - Friend 0 leaves at time 4 and chair 0 becomes empty.
+ - Friend 2 arrives at time 4 and sits on chair 0.\
+ Since friend 1 sat on chair 1, we return 1.
+
+**Example 2:**
+
+- **Input:** times = [[3,10],[1,5],[2,6]], targetFriend = 0
+- **Output:** 2
+- **Explanation:**
+ - Friend 1 arrives at time 1 and sits on chair 0.
+ - Friend 2 arrives at time 2 and sits on chair 1.
+ - Friend 0 arrives at time 3 and sits on chair 2.
+ - Friend 1 leaves at time 5 and chair 0 becomes empty.
+ - Friend 2 leaves at time 6 and chair 1 becomes empty.
+ - Friend 0 leaves at time 10 and chair 2 becomes empty.
+ Since friend 0 sat on chair 2, we return 2.
+
+**Example 3:**
+
+- **Input:**
+ ```
+ times = [[33889,98676],[80071,89737],[44118,52565],[52992,84310],[78492,88209],[21695,67063],[84622,95452],[98048,98856],[98411,99433],[55333,56548],[65375,88566],[55011,62821],[48548,48656],[87396,94825],[55273,81868],[75629,91467]],
+ targetFriend = 6
+ ```
+- **Output:** 2
+
+**Example 4:**
+
+- **Input:**
+ ```
+ times = [[4435,4436],[2958,2959],[743,744],[3439,3440],[5872,5873],[2507,2508],[3809,3810],[5084,5085],[6509,6510],[7740,7741],[1189,1190],[1277,1278],[7655,7656],[3229,3230],[4527,4528],[2242,2243],[1933,1934],[3348,3349],[6063,6064],[5344,5345],[5708,5709],[4439,4440],[4596,4597],[7572,7573],[330,331],[5153,5154],[2777,2778],[2127,2128],[7024,7025],[6983,6984],[2313,2314],[5961,5962],[7141,7142],[6150,6151],[1011,1012],[2146,2147],[1367,1368],[6741,6742],[64,65],[841,842],[6973,6974],[5002,5003],[2029,2030],[6131,6132],[947,948],[81,82],[2331,2332],[3740,3741],[1486,1487],[6176,6177],[3000,3001],[2805,2806],[6278,6279],[5273,5274],[660,661],[4833,4834],[8293,8294],[7490,7491],[2628,2629],[4032,4033],[5403,5404],[8062,8063],[5043,5044],[6038,6039],[3977,3978],[7045,7046],[1161,1162],[63,64],[2013,2014],[2400,2401],[5947,5948],[4563,4564],[6593,6594],[114,115],[2566,2567],[5297,5298],[8069,8070],[1675,1676],[1746,1747],[7713,7714],[4814,4815],[7651,7652],[6304,6305],[5638,5639],[5881,5882],[1761,1762],[3250,3251],[4391,4392],[1800,1801],[1136,1137],[6489,6490],[556,557],[3729,3730],[7901,7902],[7866,7867],[8312,8313],[1785,1786],[6200,6201],[4469,4470],[5619,5620],[4732,4733],[8039,8040],[4670,4671],[4558,4559],[8076,8077],[3743,3744],[4839,4840],[418,419],[3764,3765],[1118,1119],[3442,3443],[4605,4606],[6806,6807],[179,180],[7753,7754],[1321,1322],[6133,6134],[2456,2457],[6385,6386],[1584,1585],[6111,6112],[5156,5157],[2930,2931],[927,928],[710,711],[501,502],[2308,2309],[1971,1972],[5560,5561],[6216,6217],[5779,5780],[7486,7487],[5750,5751],[6462,6463],[2796,2797],[1543,1544],[6411,6412],[831,832],[5540,5541],[5981,5982],[3925,3926],[199,200],[8332,8333],[7338,7339],[5528,5529],[4962,4963],[3102,3103],[2063,2064],[255,256],[4317,4318],[2207,2208],[1996,1997],[1409,1410],[5095,5096],[7212,7213],[674,675],[2321,2322],[2571,2572],[4678,4679],[5542,5543],[6126,6127],[5270,5271],[6373,6374],[5856,5857],[353,354],[4295,4296],[8216,8217],[6102,6103],[7374,7375],[1578,1579],[3779,3780],[1015,1016],[5278,5279],[2320,2321],[6854,6855],[1722,1723],[1706,1707],[6310,6311],[3080,3081],[3520,3521],[6321,6322],[4505,4506],[4145,4146],[3026,3027],[5105,5106],[3284,3285],[1817,1818],[4374,4375],[4891,4892],[1640,1641],[5057,5058],[1152,1153],[7393,7394],[745,746],[8204,8205],[8321,8322],[7775,7776],[5796,5797],[2626,2627],[3991,3992],[3565,3566],[3671,3672],[6487,6488],[1592,1593],[1144,1145],[5966,5967],[1443,1444],[8224,8225],[1803,1804],[2046,2047],[3586,3587],[1162,1163],[3201,3202],[3783,3784],[6890,6891],[1695,1696],[155,156],[7390,7391],[8173,8174],[5515,5516],[3704,3705],[5422,5423],[4458,4459],[6899,6900],[2169,2170],[1555,1556],[1370,1371],[6224,6225],[7695,7696],[2369,2370],[1560,1561],[1445,1446],[2987,2988],[8114,8115],[673,674],[111,112],[5395,5396],[7122,7123],[1178,1179],[6485,6486],[5101,5102],[62,63],[1345,1346],[3386,3387],[2851,2852],[4294,4295],[5702,5703],[256,257],[7196,7197],[2587,2588],[4850,4851],[2293,2294],[7654,7655],[3251,3252],[5506,5507],[3583,3584],[7467,7468],[6560,6561],[6747,6748],[1240,1241],[7933,7934],[3237,3238],[7539,7540],[4903,4904],[409,410],[2261,2262],[6335,6336],[5310,5311],[2938,2939],[2565,2566],[7909,7910],[3418,3419],[278,279],[3253,3254],[6954,6955],[1582,1583],[898,899],[7749,7750],[4865,4866],[2713,2714],[3320,3321],[1737,1738],[4504,4505],[5339,5340],[2002,2003],[7453,7454],[8145,8146],[2200,2201],[3889,3890],[4155,4156],[513,514],[2335,2336],[874,875],[3055,3056],[7335,7336],[790,791],[7653,7654],[4806,4807],[2526,2527],[5168,5169],[1190,1191],[870,871],[2082,2083],[2756,2757],[4408,4409],[6135,6136],[5137,5138],[7000,7001],[1513,1514],[5991,5992],[291,292],[8218,8219],[8297,8298],[3582,3583],[3578,3579],[4497,4498],[731,732],[6307,6308],[3272,3273],[8197,8198],[77,78],[3389,3390],[2586,2587],[8155,8156],[8067,8068],[3658,3659],[1885,1886],[3631,3632],[1449,1450],[5850,5851],[306,307],[4711,4712],[3195,3196],[6623,6624],[6154,6155],[497,498],[3717,3718],[918,919],[3493,3494],[4436,4437],[2114,2115],[5575,5576],[6764,6765],[5600,5601],[1710,1711],[432,433],[143,144],[7482,7483],[1622,1623],[627,628],[7316,7317],[5421,5422],[6229,6230],[2136,2137],[7038,7039],[2306,2307],[3379,3380],[6253,6254],[2989,2990],[689,690],[6189,6190],[3533,3534],[476,477],[4241,4242],[5534,5535],[6768,6769],[3661,3662],[7,8],[785,786],[5756,5757],[7310,7311],[1472,1473],[7442,7443],[2715,2716],[20,21],[123,124],[1446,1447],[818,819],[6563,6564],[4941,4942],[1320,1321],[506,507],[346,347],[2011,2012],[2366,2367],[3241,3242],[104,105],[5454,5455],[130,131],[1601,1602],[1275,1276],[5520,5521],[4323,4324],[6612,6613],[3265,3266],[5312,5313],[4680,4681],[8286,8287],[1906,1907],[6341,6342],[2020,2021],[6916,6917],[3673,3674],[5025,5026],[6756,6757],[4320,4321],[7215,7216],[4047,4048],[580,581],[7121,7122],[6690,6691],[3183,3184],[863,864],[2411,2412],[7776,7777],[4429,4430],[5510,5511],[7689,7690],[3984,3985],[4425,4426],[8162,8163],[4682,4683],[4006,4007],[6069,6070],[3275,3276],[4106,4107],[5818,5819],[855,856],[1123,1124],[5176,5177],[3281,3282],[7729,7730],[5924,5925],[5020,5021],[5141,5142],[7988,7989],[970,971],[1260,1261],[6599,6600],[6244,6245],[3247,3248],[6919,6920],[1751,1752],[1142,1143],[5561,5562],[1339,1340],[1292,1293],[1232,1233],[4773,4774],[6116,6117],[3535,3536],[7598,7599],[2358,2359],[1012,1013],[5992,5993],[717,718],[6348,6349],[4210,4211],[5836,5837],[3986,3987],[5098,5099],[1413,1414],[4815,4816],[4597,4598],[7850,7851],[7188,7189],[5530,5531],[4390,4391],[783,784],[6757,6758],[1718,1719],[5196,5197],[7662,7663],[4963,4964],[633,634],[413,414],[1399,1400],[6932,6933],[3011,3012],[1101,1102],[719,720],[9,10],[8277,8278],[6290,6291],[4623,4624],[7326,7327],[361,362],[7864,7865],[2880,2881],[2923,2924],[2124,2125],[4878,4879],[968,969],[2709,2710],[2664,2665],[3855,3856],[5617,5618],[7847,7848],[6521,6522],[4799,4800],[843,844],[5568,5569],[7551,7552],[3215,3216],[1072,1073],[2118,2119],[4725,4726],[2834,2835],[410,411],[1014,1015],[125,126],[4401,4402],[3848,3849],[6711,6712],[1958,1959],[8030,8031],[184,185],[374,375],[4532,4533],[6955,6956],[725,726],[3434,3435],[5816,5817],[1875,1876],[2698,2699],[378,379],[1317,1318],[3856,3857],[4480,4481],[3613,3614],[7542,7543],[3629,3630],[6544,6545],[2159,2160],[1732,1733],[6312,6313],[6846,6847],[3518,3519],[3538,3539],[6723,6724],[6671,6672],[8185,8186],[7946,7947],[4433,4434],[1007,1008],[6783,6784],[6338,6339],[6717,6718],[2153,2154],[7488,7489],[3052,3053],[1078,1079],[4045,4046],[2007,2008],[1823,1824],[5669,5670],[5833,5834],[4380,4381],[4041,4042],[3650,3651],[1520,1521],[2532,2533],[5114,5115],[3841,3842],[8166,8167],[7036,7037],[4994,4995],[7937,7938],[5301,5302],[5423,5424],[7106,7107],[2885,2886],[1048,1049],[3790,3791],[2912,2913],[6980,6981],[1266,1267],[1075,1076],[7856,7857],[5437,5438],[2418,2419],[4838,4839],[7283,7284],[4102,4103],[8,9],[2314,2315],[1639,1640],[4119,4120],[3245,3246],[2143,2144],[907,908],[953,954],[1540,1541],[3306,3307],[215,216],[2106,2107],[6877,6878],[4063,4064],[82,83],[4305,4306],[2986,2987],[4110,4111],[2741,2742],[6553,6554],[2257,2258],[1460,1461],[5891,5892],[576,577],[4561,4562],[7211,7212],[4675,4676],[552,553],[480,481],[3679,3680],[4688,4689],[1316,1317],[910,911],[5109,5110],[6904,6905],[195,196],[5723,5724],[224,225],[5407,5408],[7688,7689],[4453,4454],[6231,6232],[7345,7346],[236,237],[630,631],[4250,4251],[6481,6482],[4954,4955],[5771,5772],[6207,6208],[7330,7331],[6161,6162],[1427,1428],[1172,1173],[5394,5395],[539,540],[1576,1577],[4357,4358],[4577,4578],[5752,5753],[6947,6948],[2404,2405],[1299,1300],[8326,8327],[5269,5270],[1174,1175],[7770,7771],[7712,7713],[812,813],[4249,4250],[5527,5528],[2530,2531],[1965,1966],[2964,2965],[164,165],[2228,2229],[7194,7195],[709,710],[5106,5107],[1074,1075],[357,358],[6110,6111],[4150,4151],[2233,2234],[738,739],[7447,7448],[6617,6618],[4259,4260],[2260,2261],[3453,3454],[2600,2601],[2141,2142],[5763,5764],[5994,5995],[7588,7589],[107,108],[2567,2568],[5935,5936],[7767,7768],[3056,3057],[3716,3717],[1238,1239],[4159,4160],[7099,7100],[6048,6049],[6271,6272],[657,658],[6308,6309],[5319,5320],[7541,7542],[6039,6040],[6942,6943],[4092,4093],[5661,5662],[7199,7200],[7465,7466],[5843,5844],[1792,1793],[2345,2346],[1649,1650],[2993,2994],[7484,7485],[6300,6301],[1742,1743],[7833,7834],[4866,4867],[1115,1116],[4028,4029],[574,575],[3014,3015],[157,158],[6641,6642],[3171,3172],[3980,3981],[180,181],[7975,7976],[1636,1637],[7119,7120],[5489,5490],[4351,4352],[7242,7243],[2517,2518],[3777,3778],[3638,3639],[3754,3755],[4293,4294],[3415,3416],[2778,2779],[4463,4464],[915,916],[817,818],[5920,5921],[7600,7601],[4043,4044],[4649,4650],[7914,7915],[2773,2774],[1454,1455],[4635,4636],[1546,1547],[8066,8067],[2499,2500],[5921,5922],[5870,5871],[3798,3799],[5052,5053],[1532,1533],[2919,2920],[3329,3330],[4519,4520],[2902,2903],[4370,4371],[2968,2969],[5681,5682],[7532,7533],[502,503],[2700,2701],[6658,6659],[7238,7239],[4058,4059],[6604,6605],[7249,7250],[2339,2340],[2219,2220],[3115,3116],[5897,5898],[7589,7590],[6162,6163],[7105,7106],[1924,1925],[7171,7172],[2189,2190],[1898,1899],[5949,5950],[3343,3344],[1756,1757],[7409,7410],[5898,5899],[522,523],[7748,7749],[6086,6087],[3993,3994],[5776,5777],[4378,4379],[1786,1787],[1690,1691],[4601,4602],[1164,1165],[6497,6498],[5627,5628],[113,114],[7144,7145],[5819,5820],[2183,2184],[3659,3660],[3737,3738],[6314,6315],[3861,3862],[507,508],[463,464],[5998,5999],[4014,4015],[4882,4883],[3866,3867],[6104,6105],[3385,3386],[1635,1636],[7926,7927],[6332,6333],[992,993],[5291,5292],[96,97],[5236,5237],[7366,7367],[7780,7781],[97,98],[6345,6346],[3916,3917],[4000,4001],[1815,1816],[3197,3198],[6456,6457],[2446,2447],[4658,4659],[4103,4104],[383,384],[7967,7968],[3006,3007],[4184,4185],[3875,3876],[1585,1586],[2623,2624],[6527,6528],[5217,5218],[5805,5806],[4686,4687],[2092,2093],[53,54],[2370,2371],[1533,1534],[4857,4858],[4353,4354],[3931,3932],[1102,1103],[6122,6123],[4420,4421],[7223,7224],[6120,6121],[5033,5034],[3732,3733],[7387,7388],[7659,7660],[3944,3945],[5148,5149],[1553,1554],[1826,1827],[1126,1127],[163,164],[2453,2454],[735,736],[6677,6678],[7229,7230],[2385,2386],[1296,1297],[7159,7160],[1766,1767],[6259,6260],[3921,3922],[5882,5883],[6186,6187],[4191,4192],[6921,6922],[1297,1298],[7621,7622],[4549,4550],[1291,1292],[6698,6699],[6033,6034],[7011,7012],[1633,1634],[4445,4446],[2691,2692],[3719,3720],[6530,6531],[1000,1001],[6158,6159],[2723,2724],[7520,7521],[5231,5232],[6467,6468],[1602,1603],[102,103],[7419,7420],[978,979],[4590,4591],[1250,1251],[3258,3259],[4263,4264],[3269,3270],[2426,2427],[3120,3121],[3791,3792],[7294,7295],[527,528],[4760,4761],[7720,7721],[8169,8170],[1458,1459],[4300,4301],[877,878],[8256,8257],[3231,3232],[5448,5449],[6501,6502],[526,527],[3651,3652],[1708,1709],[4625,4626],[4188,4189],[3928,3929],[7034,7035],[4910,4911],[6459,6460],[1921,1922],[6567,6568],[2755,2756],[5144,5145],[3882,3883],[607,608],[1877,1878],[7339,7340],[1175,1176],[6922,6923],[4931,4932],[6785,6786],[6676,6677],[7100,7101],[3285,3286],[3561,3562],[6305,6306],[5736,5737],[4204,4205],[3159,3160],[6404,6405],[1180,1181],[240,241],[2374,2375],[5480,5481],[6249,6250],[6,7],[5011,5012],[4767,4768],[2232,2233],[2871,2872],[5809,5810],[1953,1954],[1834,1835],[7785,7786],[7049,7050],[4170,4171],[4957,4958],[7677,7678],[7950,7951],[5874,5875],[2872,2873],[6790,6791],[8079,8080],[5739,5740],[4765,4766],[5076,5077],[8268,8269],[3452,3453],[7176,7177],[1334,1335],[6888,6889],[7672,7673],[1644,1645],[5810,5811],[1418,1419],[7280,7281],[7372,7373],[7054,7055],[1054,1055],[7508,7509],[5172,5173],[5769,5770],[7645,7646],[7496,7497],[5077,5078],[1041,1042],[516,517],[8278,8279],[6653,6654],[1821,1822],[642,643],[3429,3430],[6288,6289],[7630,7631],[7771,7772],[1069,1070],[6668,6669],[3592,3593],[6596,6597],[3966,3967],[8320,8321],[76,77],[4143,4144],[288,289],[1891,1892],[7356,7357],[4203,4204],[2914,2915],[3362,3363],[5626,5627],[1515,1516],[6852,6853],[6772,6773],[550,551],[540,541],[4970,4971],[8344,8345],[7222,7223],[8097,8098],[3699,3700],[2751,2752],[7992,7993],[3325,3326],[6127,6128],[1647,1648],[2855,2856],[3214,3215],[1569,1570],[5476,5477],[7322,7323],[8038,8039],[4539,4540],[3728,3729],[5,6],[1030,1031],[2858,2859],[1026,1027],[7078,7079],[2414,2415],[4395,4396],[5240,5241],[4706,4707],[2380,2381],[3584,3585],[5065,5066],[7068,7069],[688,689],[801,802],[4276,4277],[615,616],[208,209],[6896,6897],[585,586],[644,645],[4151,4152],[5046,5047],[773,774],[4603,4604],[8289,8290],[1311,1312],[3869,3870],[7423,7424],[3610,3611],[4953,4954],[5846,5847],[1518,1519],[3331,3332],[3745,3746],[1471,1472],[7883,7884],[7944,7945],[88,89],[2205,2206],[2714,2715],[7535,7536],[7235,7236],[6661,6662],[4926,4927],[3242,3243],[7174,7175],[1999,2000],[8341,8342],[2270,2271],[4518,4519],[3297,3298],[5853,5854],[7644,7645],[198,199],[8088,8089],[1382,1383],[5410,5411],[2867,2868],[1680,1681],[4672,4673],[6907,6908],[1326,1327],[3372,3373],[962,963],[1243,1244],[3065,3066],[6892,6893],[7020,7021],[7110,7111],[815,816],[6929,6930],[1977,1978],[5572,5573],[3191,3192],[2445,2446],[6129,6130],[7930,7931],[2386,2387],[1985,1986],[4316,4317],[5482,5483],[2016,2017],[5383,5384],[148,149],[8298,8299],[8109,8110],[25,26],[587,588],[1396,1397],[3691,3692],[1773,1774],[5169,5170],[2150,2151],[2981,2982],[2827,2828],[2954,2955],[3653,3654],[5939,5940],[3347,3348],[4326,4327],[6323,6324],[3260,3261],[2574,2575],[7571,7572],[6643,6644],[2010,2011],[4826,4827],[6956,6957],[845,846],[1156,1157],[4314,4315],[5893,5894],[4163,4164],[4455,4456],[5793,5794],[7727,7728],[6561,6562],[6654,6655],[3,4],[3063,3064],[1880,1881],[4144,4145],[4713,4714],[7757,7758],[4409,4410],[5313,5314],[5791,5792],[7919,7920],[1528,1529],[6434,6435],[3697,3698],[6860,6861],[3639,3640],[7329,7330],[4146,4147],[3833,3834],[2504,2505],[7940,7941],[8209,8210],[2926,2927],[6425,6426],[2210,2211],[4626,4627],[2531,2532],[4734,4735],[6173,6174],[7789,7790],[86,87],[4321,4322],[1289,1290],[367,368],[2781,2782],[7463,7464],[7074,7075],[4,5],[8010,8011],[3300,3301],[8221,8222],[2006,2007],[811,812],[4449,4450],[3505,3506],[5724,5725],[3829,3830],[2821,2822],[406,407],[7996,7997],[1968,1969],[7410,7411],[5373,5374],[3287,3288],[7201,7202],[2728,2729],[6083,6084],[8288,8289],[7884,7885],[8130,8131],[131,132],[3536,3537],[798,799],[631,632],[989,990],[8003,8004],[5519,5520],[4413,4414],[1027,1028],[3474,3475],[1579,1580],[4131,4132],[1036,1037],[15,16],[5099,5100],[6339,6340],[7458,7459],[2767,2768],[6008,6009],[2273,2274],[4702,4703],[99,100],[3222,3223],[6329,6330],[1046,1047],[3744,3745],[4695,4696],[2457,2458],[4418,4419],[617,618],[5490,5491],[891,892],[4126,4127],[5621,5622],[8179,8180],[2706,2707],[5715,5716],[5429,5430],[1559,1560],[4371,4372],[3264,3265],[7285,7286],[1479,1480],[2081,2082],[7671,7672],[4567,4568],[3782,3783],[5829,5830],[2988,2989],[6834,6835],[509,510],[1595,1596],[149,150],[2166,2167],[5671,5672],[6715,6716],[4368,4369],[7936,7937],[6971,6972],[3476,3477],[8070,8071],[4334,4335],[7665,7666],[1500,1501],[3244,3245],[4976,4977],[4787,4788],[2381,2382],[5808,5809],[5770,5771],[4461,4462],[4657,4658],[2263,2264],[6696,6697],[2908,2909],[7056,7057],[3401,3402],[370,371],[3054,3055],[7592,7593],[7722,7723],[973,974],[4347,4348],[6183,6184],[4117,4118],[7398,7399],[5590,5591],[2309,2310],[7371,7372],[7803,7804],[7120,7121],[5342,5343],[4964,4965],[1485,1486],[5473,5474],[3302,3303],[6672,6673],[4101,4102],[5192,5193],[443,444],[1657,1658],[2060,2061],[4071,4072],[1903,1904],[5923,5924],[481,482],[1671,1672],[6268,6269],[2680,2681],[328,329],[3093,3094],[5871,5872],[8226,8227],[917,918],[5573,5574],[7165,7166],[1090,1091],[2963,2964],[6477,6478],[3464,3465],[5096,5097],[4871,4872],[4034,4035],[4557,4558],[1778,1779],[7133,7134],[833,834],[2238,2239],[3252,3253],[4035,4036],[8217,8218],[1612,1613],[2961,2962],[6364,6365],[2186,2187],[8138,8139],[1298,1299],[3705,3706],[6457,6458],[3315,3316],[4002,4003],[4337,4338],[5027,5028],[1276,1277],[2634,2635],[2096,2097],[4462,4463],[429,430],[7516,7517],[2271,2272],[2528,2529],[4082,4083],[4046,4047],[4790,4791],[5353,5354],[6993,6994],[6078,6079],[4939,4940],[1448,1449],[5797,5798],[7192,7193],[6579,6580],[6491,6492],[1806,1807],[2496,2497],[6722,6723],[281,282],[6649,6650],[5073,5074],[2589,2590],[2697,2698],[7714,7715],[1476,1477],[900,901],[7885,7886],[1990,1991],[809,810],[7013,7014],[5664,5665],[4307,4308],[5441,5442],[225,226],[7911,7912],[5133,5134],[651,652],[5327,5328],[2129,2130],[2731,2732],[718,719],[6556,6557],[354,355],[74,75],[5977,5978],[1309,1310],[2585,2586],[7375,7376],[1917,1918],[553,554],[3796,3797],[2874,2875],[3309,3310],[7587,7588],[8339,8340],[5139,5140],[5548,5549],[2137,2138],[5499,5500],[5469,5470],[6610,6611],[767,768],[3066,3067],[2396,2397],[8000,8001],[2591,2592],[2035,2036],[3298,3299],[4057,4058],[6297,6298],[4642,4643],[8040,8041],[7027,7028],[6591,6592],[6044,6045],[1475,1476],[5582,5583],[4697,4698],[2372,2373],[2911,2912],[5195,5196],[2844,2845],[1642,1643],[7898,7899],[2681,2682],[8208,8209],[6869,6870],[8231,8232],[1743,1744],[600,601],[7565,7566],[3788,3789],[412,413],[7317,7318],[3595,3596],[4087,4088],[2036,2037],[2925,2926],[6713,6714],[2156,2157],[1587,1588],[8002,8003],[5016,5017],[7502,7503],[5464,5465],[5177,5178],[799,800],[7042,7043],[4036,4037],[1691,1692],[188,189],[1197,1198],[2429,2430],[6175,6176],[568,569],[8156,8157],[7434,7435],[1589,1590],[247,248],[4880,4881],[564,565],[6155,6156],[979,980],[212,213],[536,537],[6885,6886],[4574,4575],[4448,4449],[792,793],[3064,3065],[7403,7404],[4733,4734],[490,491],[4313,4314],[4487,4488],[6095,6096],[4712,4713],[1783,1784],[4777,4778],[2107,2108],[6618,6619],[646,647],[2779,2780],[2224,2225],[6695,6696],[4632,4633],[3946,3947],[4129,4130],[7796,7797],[2482,2483],[430,431],[2970,2971],[563,564],[7231,7232],[6667,6668],[4786,4787],[4887,4888],[5579,5580],[8202,8203],[4412,4413],[1489,1490],[5404,5405],[7844,7845],[2227,2228],[7431,7432],[6522,6523],[7957,7958],[3248,3249],[7604,7605],[6972,6973],[2155,2156],[7319,7320],[2049,2050],[8033,8034],[4068,4069],[5700,5701],[866,867],[7823,7824],[844,845],[310,311],[7987,7988],[159,160],[6600,6601],[2045,2046],[2383,2384],[2213,2214],[270,271],[1287,1288],[2760,2761],[1348,1349],[4819,4820],[2443,2444],[1637,1638],[1241,1242],[8254,8255],[2497,2498],[3002,3003],[2378,2379],[4437,4438],[8118,8119],[3073,3074],[1135,1136],[2768,2769],[1099,1100],[7985,7986],[434,435],[2621,2622],[294,295],[4691,4692],[6306,6307],[3475,3476],[2632,2633],[5493,5494],[3806,3807],[2318,2319],[7286,7287],[5131,5132],[8276,8277],[5869,5870],[1005,1006],[2299,2300],[6367,6368],[7646,7647],[2079,2080],[453,454],[4175,4176],[3438,3439],[1547,1548],[4914,4915],[1480,1481],[4937,4938],[8177,8178],[1253,1254],[3181,3182],[5503,5504],[5933,5934],[7531,7532],[1349,1350],[1682,1683],[3623,3624],[3029,3030],[6451,6452],[205,206],[7155,7156],[7751,7752],[4576,4577],[2447,2448],[7474,7475],[57,58],[5143,5144],[6529,6530],[8022,8023],[6866,6867],[6143,6144],[1564,1565],[538,539],[411,412],[2933,2934],[3317,3318],[448,449],[4620,4621],[5629,5630],[1150,1151],[1211,1212],[2329,2330],[7699,7700],[6510,6511],[5071,5072],[2171,2172],[1660,1661],[3694,3695],[3077,3078],[5958,5959],[510,511],[5280,5281],[515,516],[4512,4513],[3990,3991],[5390,5391],[4242,4243],[3076,3077],[5425,5426],[624,625],[4096,4097],[2764,2765],[1842,1843],[1717,1718],[6027,6028],[3713,3714],[664,665],[6837,6838],[4979,4980],[470,471],[1870,1871],[705,706],[116,117],[1926,1927],[5814,5815],[3033,3034],[7533,7534],[1897,1898],[287,288],[4949,4950],[1703,1704],[1258,1259],[7893,7894],[355,356],[5409,5410],[830,831],[5868,5869],[6498,6499],[7554,7555],[788,789],[2388,2389],[1598,1599],[5175,5176],[3228,3229],[6097,6098],[8270,8271],[4386,4387],[297,298],[2762,2763],[417,418],[8180,8181],[8035,8036],[2703,2704],[2752,2753],[5876,5877],[5592,5593],[1307,1308],[6388,6389],[1265,1266],[1794,1795],[8164,8165],[2037,2038],[1851,1852],[4349,4350],[5491,5492],[498,499],[4795,4796],[6255,6256],[746,747],[1580,1581],[6531,6532],[7869,7870],[6242,6243],[7505,7506],[6901,6902],[1182,1183],[3419,3420],[2888,2889],[730,731],[6725,6726],[7359,7360],[6730,6731],[8253,8254],[6673,6674],[5363,5364],[4687,4688],[8353,8354],[7473,7474],[5980,5981],[4919,4920],[3447,3448],[6909,6910],[3392,3393],[4302,4303],[8243,8244],[2894,2895],[6858,6859],[1236,1237],[6632,6633],[4737,4738],[2179,2180],[1213,1214],[2441,2442],[7578,7579],[5368,5369],[2448,2449],[8005,8006],[4415,4416],[3239,3240],[5953,5954],[5332,5333],[7006,7007],[1411,1412],[771,772],[7846,7847],[2201,2202],[4535,4536],[1573,1574],[161,162],[4715,4716],[4763,4764],[5351,5352],[7787,7788],[8337,8338],[4992,4993],[2062,2063],[5037,5038],[3622,3623],[238,239],[5997,5998],[2303,2304],[318,319],[4547,4548],[7301,7302],[2690,2691],[5315,5316],[3564,3565],[712,713],[5302,5303],[7451,7452],[4973,4974],[4818,4819],[5017,5018],[1645,1646],[7414,7415],[750,751],[8322,8323],[7849,7850],[2415,2416],[450,451],[8083,8084],[4951,4952],[3117,3118],[3122,3123],[3599,3600],[8098,8099],[6236,6237],[3960,3961],[704,705],[4874,4875],[3123,3124],[5377,5378],[6958,6959],[2379,2380],[4524,4525],[7661,7662],[5252,5253],[4111,4112],[6191,6192],[3625,3626],[6930,6931],[7290,7291],[7679,7680],[7733,7734],[1873,1874],[1820,1821],[6020,6021],[1979,1980],[5800,5801],[3563,3564],[3205,3206],[2099,2100],[3887,3888],[7545,7546],[8049,8050],[2552,2553],[5379,5380],[4484,4485],[7395,7396],[7287,7288],[1634,1635],[4253,4254],[4424,4425],[1937,1938],[5322,5323],[7250,7251],[6508,6509],[7164,7165],[5968,5969],[6707,6708],[3098,3099],[876,877],[5385,5386],[769,770],[7246,7247],[4746,4747],[12,13],[2898,2899],[2949,2950],[3230,3231],[6863,6864],[7900,7901],[7402,7403],[7019,7020],[7990,7991],[8006,8007],[136,137],[5232,5233],[634,635],[707,708],[2180,2181],[6867,6868],[1394,1395],[7927,7928],[7454,7455],[3963,3964],[2491,2492],[2212,2213],[3642,3643],[3289,3290],[4513,4514],[4287,4288],[4098,4099],[3108,3109],[5452,5453],[3663,3664],[2870,2871],[2485,2486],[6390,6391],[5036,5037],[6025,6026],[7807,7808],[5063,5064],[85,86],[260,261],[6709,6710],[4005,4006],[6006,6007],[6986,6987],[2950,2951],[5329,5330],[3053,3054],[3213,3214],[2972,2973],[729,730],[4027,4028],[7128,7129],[5759,5760],[3690,3691],[1022,1023],[1170,1171],[7032,7033],[1081,1082],[3725,3726],[6898,6899],[7851,7852],[1244,1245],[4244,4245],[6928,6929],[4764,4765],[2959,2960],[3182,3183],[6480,6481],[151,152],[7652,7653],[1523,1524],[4756,4757],[7354,7355],[339,340],[4485,4486],[1709,1710],[2362,2363],[1481,1482],[7439,7440],[4738,4739],[4859,4860],[73,74],[8318,8319],[6831,6832],[761,762],[2250,2251],[2985,2986],[5040,5041],[625,626],[5889,5890],[8225,8226],[6999,7000],[1884,1885],[4001,4002],[7127,7128],[937,938],[5728,5729],[1426,1427],[4118,4119],[1025,1026],[6751,6752],[2877,2878],[1002,1003],[6444,6445],[622,623],[5609,5610],[4350,4351],[5502,5503],[8008,8009],[5298,5299],[6801,6802],[5518,5519],[7530,7531],[4467,4468],[7158,7159],[2975,2976],[3542,3543],[8074,8075],[636,637],[2102,2103],[5202,5203],[2747,2748],[3180,3181],[4867,4868],[1796,1797],[6966,6967],[4475,4476],[775,776],[39,40],[1053,1054],[3318,3319],[7092,7093],[2094,2095],[5481,5482],[4160,4161],[2344,2345],[7764,7765],[5479,5480],[3945,3946],[48,49],[3645,3646],[5474,5475],[994,995],[6562,6563],[680,681],[6356,6357],[1662,1663],[6003,6004],[7958,7959],[653,654],[1216,1217],[3487,3488],[5397,5398],[7170,7171],[945,946],[602,603],[3962,3963],[3001,3002],[4716,4717],[5288,5289],[3709,3710],[7182,7183],[1863,1864],[8213,8214],[6714,6715],[7037,7038],[7890,7891],[3547,3548],[7248,7249],[5415,5416],[7698,7699],[4073,4074],[5408,5409],[2611,2612],[7728,7729],[3763,3764],[4132,4133],[2927,2928],[3459,3460],[2122,2123],[2819,2820],[220,221],[1400,1401],[4905,4906],[6455,6456],[2886,2887],[4556,4557],[6766,6767],[7415,7416],[4091,4092],[1501,1502],[2646,2647],[6461,6462],[524,525],[3424,3425],[4901,4902],[7313,7314],[7369,7370],[6765,6766],[1440,1441],[7125,7126],[883,884],[1952,1953],[3929,3930],[2235,2236],[2070,2071],[1905,1906],[2519,2520],[141,142],[373,374],[5537,5538],[2579,2580],[3430,3431],[1086,1087],[4438,4439],[5999,6000],[2598,2599],[5227,5228],[5622,5623],[1858,1859],[4822,4823],[6383,6384],[8031,8032],[1019,1020],[4222,4223],[5563,5564],[6453,6454],[5792,5793],[2932,2933],[3539,3540],[3959,3960],[6546,6547],[2674,2675],[4148,4149],[6045,6046],[2039,2040],[3692,3693],[6402,6403],[4178,4179],[7149,7150],[3448,3449],[3815,3816],[4285,4286],[1545,1546],[7175,7176],[1138,1139],[13,14],[5401,5402],[5307,5308],[6586,6587],[4559,4560],[2040,2041],[1227,1228],[6250,6251],[231,232],[7477,7478],[1357,1358],[259,260],[5637,5638],[6327,6328],[5913,5914],[6287,6288],[892,893],[1650,1651],[1827,1828],[2790,2791],[2840,2841],[3086,3087],[598,599],[5211,5212],[8336,8337],[3038,3039],[2746,2747],[2148,2149],[2994,2995],[7701,7702],[6532,6533],[11,12],[2087,2088],[2466,2467],[6042,6043],[436,437],[5783,5784],[4509,4510],[1016,1017],[7407,7408],[2229,2230],[6547,6548],[1883,1884],[1328,1329],[8184,8185],[8133,8134],[5956,5957],[5190,5191],[3537,3538],[1611,1612],[2520,2521],[8189,8190],[1941,1942],[1604,1605],[1098,1099],[89,90],[7905,7906],[368,369],[3162,3163],[3780,3781],[7559,7560],[5251,5252],[5134,5135],[8161,8162],[7903,7904],[5214,5215],[3772,3773],[8199,8200],[468,469],[7426,7427],[4562,4563],[6728,6729],[6004,6005],[7678,7679],[5498,5499],[7098,7099],[3483,3484],[6381,6382],[304,305],[8139,8140],[3227,3228],[6350,6351],[8239,8240],[3057,3058],[2518,2519],[2080,2081],[4104,4105],[5424,5425],[8052,8053],[7440,7441],[474,475],[2502,2503],[4710,4711],[6284,6285],[4196,4197],[494,495],[5015,5016],[5706,5707],[5749,5750],[4594,4595],[5337,5338],[2347,2348],[3427,3428],[5888,5889],[4020,4021],[6740,6741],[4481,4482],[7640,7641],[6352,6353],[3823,3824],[3634,3635],[5194,5195],[4654,4655],[2275,2276],[1942,1943],[2843,2844],[5555,5556],[6818,6819],[2392,2393],[7813,7814],[2367,2368],[232,233],[7834,7835],[1107,1108],[732,733],[4933,4934],[6105,6106],[3703,3704],[3262,3263],[5289,5290],[2522,2523],[7906,7907],[5607,5608],[6857,6858],[964,965],[338,339],[5501,5502],[5405,5406],[3457,3458],[201,202],[5272,5273],[2483,2484],[257,258],[6995,6996],[1057,1058],[117,118],[4431,4432],[8017,8018],[1735,1736],[5937,5938],[7108,7109],[7999,8000],[2022,2023],[5009,5010],[2602,2603],[2897,2898],[3879,3880],[4816,4817],[1754,1755],[388,389],[1664,1665],[2725,2726],[6893,6894],[239,240],[5380,5381],[6422,6423],[611,612],[2940,2941],[3859,3860],[983,984],[1969,1970],[4064,4065],[6359,6360],[1181,1182],[1149,1150],[560,561],[2053,2054],[3138,3139],[5812,5813],[5374,5375],[3723,3724],[8211,8212],[6142,6143],[1752,1753],[6841,6842],[8072,8073],[1281,1282],[4397,4398],[2548,2549],[128,129],[8064,8065],[147,148],[4381,4382],[7180,7181],[4297,4298],[5008,5009],[3410,3411],[846,847],[4460,4461],[1702,1703],[5598,5599],[7421,7422],[5837,5838],[7685,7686],[5418,5419],[7576,7577],[3096,3097],[5722,5723],[3132,3133],[7777,7778],[1672,1673],[5284,5285],[5366,5367],[2826,2827],[3160,3161],[6128,6129],[2241,2242],[922,923],[5083,5084],[4343,4344],[2173,2174],[2555,2556],[5526,5527],[7887,7888],[5283,5284],[2663,2664],[6755,6756],[3508,3509],[1097,1098],[6488,6489],[7260,7261],[246,247],[6151,6152],[7758,7759],[2588,2589],[7522,7523],[3206,3207],[1087,1088],[7779,7780],[3570,3571],[2515,2516],[4832,4833],[391,392],[5878,5879],[7843,7844],[3270,3271],[5178,5179],[274,275],[3407,3408],[2569,2570],[1,2],[2823,2824],[5517,5518],[5229,5230],[213,214],[8012,8013],[3208,3209],[6914,6915],[2078,2079],[823,824],[2047,2048],[938,939],[2286,2287],[7560,7561],[2688,2689],[4376,4377],[7269,7270],[3068,3069],[4474,4475],[144,145],[7116,7117],[6905,6906],[4008,4009],[2617,2618],[649,650],[4209,4210],[360,361],[3344,3345],[6443,6444],[7435,7436],[100,101],[7102,7103],[3445,3446],[3517,3518],[5104,5105],[1819,1820],[1908,1909],[933,934],[6788,6789],[2631,2632],[650,651],[4945,4946],[6657,6658],[4010,4011],[8259,8260],[5687,5688],[4372,4373],[2832,2833],[3044,3045],[7617,7618],[5445,5446],[5275,5276],[60,61],[4506,4507],[5896,5897],[6961,6962],[5406,5407],[16,17],[2247,2248],[4968,4969],[1247,1248],[7190,7191],[6258,6259],[5660,5661],[581,582],[3219,3220],[6868,6869],[4048,4049],[7706,7707],[3968,3969],[8343,8344],[7835,7836],[2862,2863],[4701,4702],[6912,6913],[7293,7294],[1495,1496],[755,756],[282,283],[4095,4096],[3647,3648],[2761,2762],[2753,2754],[3507,3508],[2355,2356],[5259,5260],[84,85],[5417,5418],[8192,8193],[6340,6341],[5460,5461],[4496,4497],[252,253],[1896,1897],[1329,1330],[6800,6801],[3696,3697],[4810,4811],[5370,5371],[7441,7442],[982,983],[4346,4347],[3808,3809],[8131,8132],[3165,3166],[7219,7220],[3371,3372],[6160,6161],[1127,1128],[2892,2893],[1461,1462],[4472,4473],[5761,5762],[4618,4619],[6822,6823],[632,633],[5838,5839],[2484,2485],[3255,3256],[2277,2278],[3822,3823],[6850,6851],[2500,2501],[6794,6795],[4827,4828],[925,926],[2401,2402],[1375,1376],[1668,1669],[6503,6504],[8246,8247],[6987,6988],[2018,2019],[5428,5429],[2085,2086],[7923,7924],[2622,2623],[7872,7873],[6777,6778],[4820,4821],[4848,4849],[6415,6416],[6771,6772],[826,827],[7117,7118],[4990,4991],[828,829],[6569,6570],[4122,4123],[7394,7395],[6015,6016],[7029,7030],[6810,6811],[6471,6472],[17,18],[5054,5055],[6931,6932],[1565,1566],[6903,6904],[4136,4137],[2480,2481],[6590,6591],[878,879],[3209,3210],[8111,8112],[5860,5861],[1610,1611],[7443,7444],[7750,7751],[3465,3466],[8105,8106],[3667,3668],[31,32],[5338,5339],[6927,6928],[5663,5664],[2015,2016],[1679,1680],[1340,1341],[261,262],[7384,7385],[5541,5542],[2966,2967],[2423,2424],[6237,6238],[6760,6761],[4803,4804],[2349,2350],[2839,2840],[3246,3247],[5261,5262],[2815,2816],[5326,5327],[7033,7034],[1139,1140],[2849,2850],[6559,6560],[2123,2124],[1961,1962],[3784,3785],[3019,3020],[7432,7433],[4236,4237],[3437,3438],[2613,2614],[4663,4664],[923,924],[5667,5668],[4231,4232],[285,286],[7660,7661],[7534,7535],[1643,1644],[6442,6443],[5601,5602],[6628,6629],[5824,5825],[6447,6448],[3981,3982],[7364,7365],[7746,7747],[4566,4567],[5901,5902],[55,56],[7311,7312],[1964,1965],[2641,2642],[2645,2646],[8190,8191],[3094,3095],[5056,5057],[7079,7080],[3541,3542],[4053,4054],[1335,1336],[7801,7802],[5640,5641],[33,34],[1226,1227],[7334,7335],[7510,7511],[4516,4517],[6203,6204],[5972,5973],[1433,1434],[6002,6003],[4135,4136],[2135,2136],[7003,7004],[4798,4799],[6315,6316],[3394,3395],[920,921],[6000,6001],[4906,4907],[1954,1955],[1039,1040],[5936,5937],[2161,2162],[8124,8125],[6720,6721],[545,546],[1767,1768],[7224,7225],[7639,7640],[2800,2801],[5584,5585],[6276,6277],[3956,3957],[1009,1010],[737,738],[333,334],[3421,3422],[4836,4837],[2837,2838],[4234,4235],[4239,4240],[3467,3468],[1893,1894],[4369,4370],[5292,5293],[4167,4168],[264,265],[6597,6598],[7071,7072],[1550,1551],[3005,3006],[203,204],[6542,6543],[1066,1067],[5650,5651],[3701,3702],[7622,7623],[1609,1610],[7126,7127],[5127,5128],[7302,7303],[5570,5571],[4306,4307],[1153,1154],[6091,6092],[2104,2105],[1430,1431],[6409,6410],[2533,2534],[397,398],[3286,3287],[6906,6907],[7265,7266],[45,46],[4088,4089],[4260,4261],[8053,8054],[302,303],[5849,5850],[708,709],[793,794],[7016,7017],[776,777],[4075,4076],[3605,3606],[3232,3233],[7044,7045],[2177,2178],[5447,5448],[4589,4590],[4024,4025],[4660,4661],[2967,2968],[1890,1891],[3644,3645],[4274,4275],[1037,1038],[6181,6182],[4748,4749],[7035,7036],[8068,8069],[5108,5109],[1511,1512],[5213,5214],[3431,3432],[8187,8188],[3874,3875],[4523,4524],[1453,1454],[6620,6621],[7647,7648],[6820,6821],[1536,1537],[6861,6862],[7299,7300],[1179,1180],[2134,2135],[3127,3128],[7557,7558],[5265,5266],[2775,2776],[5439,5440],[6832,6833],[5174,5175],[2794,2795],[2559,2560],[1788,1789],[2098,2099],[6286,6287],[2283,2284],[946,947],[7870,7871],[6871,6872],[2671,2672],[1855,1856],[105,106],[1813,1814],[4792,4793],[4502,4503],[3768,3769],[2220,2221],[1534,1535],[3388,3389],[424,425],[1744,1745],[1740,1741],[4640,4641],[7997,7998],[1600,1601],[4079,4080],[3839,3840],[4837,4838],[1839,1840],[5082,5083],[4884,4885],[3675,3676],[8200,8201],[4478,4479],[6659,6660],[913,914],[112,113],[2976,2977],[8025,8026],[3702,3703],[960,961],[5180,5181],[1608,1609],[1035,1036],[7642,7643],[939,940],[4830,4831],[485,486],[3446,3447],[3588,3589],[7244,7245],[4432,4433],[6811,6812],[2488,2489],[7303,7304],[3710,3711],[5138,5139],[3370,3371],[8271,8272],[4581,4582],[1829,1830],[6274,6275],[6041,6042],[4643,4644],[7514,7515],[5376,5377],[5746,5747],[4332,4333],[5504,5505],[3157,3158],[784,785],[6441,6442],[1199,1200],[7633,7634],[4730,4731],[6180,6181],[670,671],[2801,2802],[5184,5185],[3061,3062],[2957,2958],[2181,2182],[7438,7439],[1729,1730],[4328,4329],[2884,2885],[1769,1770],[7581,7582],[3828,3829],[4659,4660],[1147,1148],[7109,7110],[7240,7241],[4553,4554],[903,904],[850,851],[7378,7379],[121,122],[2364,2365],[3454,3455],[1414,1415],[3511,3512],[4456,4457],[7091,7092],[1169,1170],[6164,6165],[4769,4770],[3748,3749],[1222,1223],[6870,6871],[6665,6666],[728,729],[5183,5184],[7615,7616],[1116,1117],[7763,7764],[914,915],[4958,4959],[1930,1931],[3095,3096],[4985,4986],[8262,8263],[5525,5526],[6171,6172],[2960,2961],[5187,5188],[7821,7822],[3200,3201],[4403,4404],[365,366],[2479,2480],[5780,5781],[7529,7530],[106,107],[4639,4640],[271,272],[6802,6803],[6815,6816],[3280,3281],[1185,1186],[6205,6206],[6247,6248],[7998,7999],[2357,2358],[3646,3647],[6829,6830],[5883,5884],[6269,6270],[1255,1256],[825,826],[6072,6073],[3930,3931],[2614,2615],[6052,6053],[6022,6023],[835,836],[8272,8273],[2743,2744],[3351,3352],[7632,7633],[881,882],[5928,5929],[1847,1848],[3807,3808],[5386,5387],[5794,5795],[1468,1469],[3224,3225],[5735,5736],[5090,5091],[3516,3517],[772,773],[4752,4753],[4157,4158],[7347,7348],[4569,4570],[3071,3072],[2749,2750],[301,302],[4042,4043],[8212,8213],[7342,7343],[5026,5027],[851,852],[4479,4480],[3357,3358],[2654,2655],[3943,3944],[7575,7576],[7028,7029],[7737,7738],[7569,7570],[8194,8195],[439,440],[1723,1724],[7550,7551],[4269,4270],[6688,6689],[3603,3604],[7259,7260],[3151,3152],[6611,6612],[2465,2466],[6874,6875],[7358,7359],[6051,6052],[475,476],[5066,5067],[963,964],[1674,1675],[2582,2583],[6084,6085],[976,977],[806,807],[4646,4647],[4303,4304],[5857,5858],[3973,3974],[8163,8164],[300,301],[1301,1302],[4220,4221],[6377,6378],[4121,4122],[7608,7609],[848,849],[4385,4386],[3909,3910],[537,538],[4797,4798],[2167,2168],[965,966],[2417,2418],[5633,5634],[8296,8297],[7585,7586],[613,614],[3271,3272],[1853,1854],[7809,7810],[1931,1932],[8117,8118],[3324,3325],[6085,6086],[5097,5098],[1318,1319],[4974,4975],[5699,5700],[32,33],[7986,7987],[2945,2946],[559,560],[1483,1484],[4662,4663],[3398,3399],[8047,8048],[8201,8202],[2771,2772],[1421,1422],[6689,6690],[3715,3716],[5803,5804],[1474,1475],[5628,5629],[7323,7324],[7307,7308],[1095,1096],[7237,7238],[824,825],[7341,7342],[4726,4727],[7972,7973],[8129,8130],[3128,3129],[2149,2150],[3967,3968],[7994,7995],[5577,5578],[2175,2176],[1571,1572],[548,549],[3463,3464],[5695,5696],[530,531],[7568,7569],[4375,4376],[4038,4039],[8282,8283],[4459,4460],[996,997],[4033,4034],[2341,2342],[4685,4686],[4614,4615],[1567,1568],[4315,4316],[5456,5457],[4932,4933],[2296,2297],[2055,2056],[8292,8293],[6141,6142],[1808,1809],[2508,2509],[5399,5400],[5884,5885],[2643,2644],[3039,3040],[3373,3374],[7891,7892],[1760,1761],[3648,3649],[4224,4225],[5758,5759],[4219,4220],[4402,4403],[6835,6836],[7075,7076],[5107,5108],[6248,6249],[1995,1996],[4428,4429],[5155,5156],[3412,3413],[3551,3552],[2100,2101],[3279,3280],[4544,4545],[1374,1375],[3491,3492],[6187,6188],[1230,1231],[3082,3083],[5075,5076],[5929,5930],[7080,7081],[5102,5103],[5523,5524],[1980,1981],[5983,5984],[671,672],[640,641],[5400,5401],[6029,6030],[1109,1110],[6256,6257],[5446,5447],[1302,1303],[5324,5325],[6985,6986],[713,714],[6318,6319],[6570,6571],[54,55],[2066,2067],[6322,6323],[4115,4116],[2866,2867],[4515,4516],[3878,3879],[7005,7006],[2956,2957],[6168,6169],[2103,2104],[6336,6337],[6421,6422],[4703,4704],[7261,7262],[2878,2879],[4078,4079],[3085,3086],[3718,3719],[3393,3394],[5060,5061],[7218,7219],[2883,2884],[1616,1617],[4872,4873],[5182,5183],[2580,2581],[3765,3766],[852,853],[305,306],[1522,1523],[3637,3638],[5414,5415],[6992,6993],[2132,2133],[3674,3675],[8087,8088],[5954,5955],[782,783],[7761,7762],[7549,7550],[4331,4332],[8280,8281],[2539,2540],[4950,4951],[4182,4183],[8242,8243],[266,267],[5569,5570],[1618,1619],[3342,3343],[1323,1324],[3020,3021],[93,94],[5245,5246],[414,415],[1155,1156],[525,526],[283,284],[7252,7253],[2249,2250],[6337,6338],[4739,4740],[3382,3383],[6799,6800],[3148,3149],[2763,2764],[4189,4190],[6264,6265],[3521,3522],[6564,6565],[6950,6951],[222,223],[381,382],[4583,4584],[1122,1123],[934,935],[1721,1722],[8233,8234],[1687,1688],[3291,3292],[936,937],[566,567],[262,263],[7430,7431],[5343,5344],[4503,4504],[4262,4263],[2258,2259],[2230,2231],[2430,2431],[2402,2403],[7289,7290],[4152,4153],[5946,5947],[6798,6799],[4348,4349],[8054,8055],[496,497],[376,377],[592,593],[1669,1670],[2992,2993],[5926,5927],[5927,5928],[2115,2116],[8007,8008],[329,330],[2285,2286],[5436,5437],[3069,3070],[1802,1803],[6843,6844],[1774,1775],[1242,1243],[7363,7364],[1925,1926],[8257,8258],[2467,2468],[2676,2677],[375,376],[4329,4330],[6897,6898],[2812,2813],[2570,2571],[3934,3935],[5922,5923],[4440,4441],[3514,3515],[1809,1810],[4049,4050],[6392,6393],[6780,6781],[6031,6032],[5198,5199],[764,765],[7160,7161],[2558,2559],[618,619],[3458,3459],[5688,5689],[3846,3847],[4012,4013],[5632,5633],[5166,5167],[6759,6760],[5317,5318],[7959,7960],[6139,6140],[3106,3107],[187,188],[4938,4939],[3501,3502],[514,515],[1970,1971],[5186,5187],[1405,1406],[1419,1420],[4185,4186],[8167,8168],[2584,2585],[520,521],[5785,5786],[7138,7139],[1733,1734],[6941,6942],[6174,6175],[1052,1053],[1912,1913],[7910,7911],[2248,2249],[2001,2002],[7812,7813],[6774,6775],[1810,1811],[449,450],[957,958],[766,767],[4736,4737],[6943,6944],[4543,4544],[4920,4921],[4211,4212],[4854,4855],[2267,2268],[7717,7718],[3762,3763],[6473,6474],[6437,6438],[3131,3132],[1231,1232],[4257,4258],[7605,7606],[2084,2085],[5412,5413],[59,60],[4900,4901],[7519,7520],[6089,6090],[6450,6451],[4907,4908],[5391,5392],[7225,7226],[2121,2122],[5772,5773],[420,421],[3179,3180],[5173,5174],[2387,2388],[6587,6588],[8149,8150],[1843,1844],[2278,2279],[1295,1296],[6574,6575],[4192,4193],[2422,2423],[5727,5728],[6393,6394],[2929,2930],[5753,5754],[4023,4024],[3294,3295],[6302,6303],[5495,5496],[3978,3979],[245,246],[3756,3757],[2237,2238],[4120,4121],[2110,2111],[2109,2110],[5614,5615],[7234,7235],[2900,2901],[4809,4810],[4379,4380],[5580,5581],[137,138],[5450,5451],[3617,3618],[7912,7913],[4450,4451],[4205,4206],[1369,1370],[3074,3075],[6552,6553],[7017,7018],[1038,1039],[4609,4610],[277,278],[998,999],[4690,4691],[83,84],[5647,5648],[6232,6233],[1787,1788],[325,326],[2670,2671],[4327,4328],[693,694],[6401,6402],[7526,7527],[7499,7500],[6691,6692],[1119,1120],[5539,5540],[6735,6736],[8304,8305],[1467,1468],[4585,4586],[1654,1655],[3858,3859],[1267,1268],[599,600],[4896,4897],[7594,7595],[3217,3218],[1092,1093],[6282,6283],[3003,3004],[8045,8046],[3504,3505],[1916,1917],[6417,6418],[5471,5472],[6093,6094],[2685,2686],[1271,1272],[4500,4501],[5890,5891],[5644,5645],[2603,2604],[744,745],[4354,4355],[3781,3782],[178,179],[1902,1903],[2660,2661],[758,759],[757,758],[7184,7185],[1623,1624],[7471,7472],[2899,2900],[171,172],[1062,1063],[1860,1861],[2937,2938],[3643,3644],[1288,1289],[3414,3415],[1234,1235],[2328,2329],[396,397],[1407,1408],[7536,7537],[706,707],[1452,1453],[1765,1766],[8152,8153],[8338,8339],[7638,7639],[2202,2203],[7517,7518],[3633,3634],[7169,7170],[5738,5739],[1590,1591],[3109,3110],[2615,2616],[1157,1158],[5538,5539],[8071,8072],[4171,4172],[3104,3105],[6750,6751],[4928,4929],[7947,7948],[6934,6935],[4613,4614],[6046,6047],[1363,1364],[4022,4023],[7697,7698],[3677,3678],[7616,7617],[6146,6147],[6358,6359],[928,929],[4406,4407],[1724,1725],[857,858],[433,434],[4310,4311],[3312,3313],[6301,6302],[3888,3889],[6926,6927],[7876,7877],[3397,3398],[5092,5093],[1940,1941],[4696,4697],[331,332],[7183,7184],[3022,3023],[3581,3582],[4966,4967],[7831,7832],[385,386],[1013,1014],[6087,6088],[1753,1754],[1538,1539],[5308,5309],[1619,1620],[3296,3297],[659,660],[7173,7174],[4622,4623],[7675,7676],[160,161],[1849,1850],[2633,2634],[2222,2223],[5352,5353],[6275,6276],[3972,3973],[7093,7094],[3641,3642],[6088,6089],[1286,1287],[7096,7097],[6839,6840],[5516,5517],[2024,2025],[555,556],[7657,7658],[4309,4310],[2397,2398],[162,163],[3422,3423],[7725,7726],[6372,6373],[4074,4075],[387,388],[1516,1517],[2609,2610],[2199,2200],[759,760],[7504,7505],[4789,4790],[4858,4859],[4967,4968],[4173,4174],[4834,4835],[348,349],[3908,3909],[4758,4759],[1790,1791],[7521,7522],[7862,7863],[4270,4271],[3527,3528],[191,192],[1103,1104],[645,646],[3549,3550],[6844,6845],[4768,4769],[1209,1210],[6900,6901],[1992,1993],[2147,2148],[2455,2456],[6951,6952],[4235,4236],[4778,4779],[2266,2267],[7507,7508],[3589,3590],[431,432],[8191,8192],[1080,1081],[3666,3667],[7228,7229],[6355,6356],[6965,6966],[5372,5373],[312,313],[3482,3483],[7583,7584],[7791,7792],[3083,3084],[2026,2027],[1624,1625],[4870,4871],[7739,7740],[7373,7374],[2474,2475],[1111,1112],[6009,6010],[3773,3774],[4434,4435],[2873,2874],[7015,7016],[153,154],[3226,3227],[202,203],[5263,5264],[1824,1825],[7816,7817],[7247,7248],[122,123],[797,798],[3770,3771],[5072,5073],[8205,8206],[2489,2490],[1499,1500],[7104,7105],[7267,7268],[2425,2426],[466,467],[8330,8331],[24,25],[6634,6635],[321,322],[6856,6857],[2551,2552],[3207,3208],[666,667],[8248,8249],[2469,2470],[2803,2804],[295,296],[5643,5644],[2846,2847],[6782,6783],[6625,6626],[6266,6267],[5676,5677],[4508,4509],[2359,2360],[7081,7082],[2406,2407],[8020,8021],[1699,1700],[8063,8064],[984,985],[6362,6363],[4062,4063],[1270,1271],[6736,6737],[3721,3722],[6303,6304],[8016,8017],[1548,1549],[2935,2936],[7694,7695],[272,273],[3847,3848],[7275,7276],[5747,5748],[2048,2049],[4153,4154],[6387,6388],[5455,5456],[437,438],[2065,2066],[3891,3892],[6721,6722],[2946,2947],[5725,5726],[250,251],[7498,7499],[5788,5789],[6218,6219],[733,734],[3817,3818],[4491,4492],[6879,6880],[327,328],[2977,2978],[7538,7539],[4638,4639],[7416,7417],[3075,3076],[4653,4654],[639,640],[6554,6555],[5113,5114],[8150,8151],[6887,6888],[6663,6664],[7711,7712],[1712,1713],[993,994],[8018,8019],[109,110],[7161,7162],[2140,2141],[1223,1224],[534,535],[4197,4198],[4318,4319],[3685,3686],[3546,3547],[4498,4499],[7308,7309],[399,400],[3175,3176],[5656,5657],[7475,7476],[454,455],[5305,5306],[2252,2253],[7839,7840],[4404,4405],[2550,2551],[6167,6168],[5004,5005],[4517,4518],[3935,3936],[1994,1995],[3969,3970],[1950,1951],[6646,6647],[643,644],[146,147],[4466,4467],[7506,7507],[5578,5579],[4665,4666],[4617,4618],[4216,4217],[4093,4094],[1572,1573],[3918,3919],[30,31],[4666,4667],[3042,3043],[872,873],[5255,5256],[4927,4928],[5285,5286],[2470,2471],[2537,2538],[3840,3841],[1020,1021],[4800,4801],[3092,3093],[1697,1698],[7058,7059],[5911,5912],[5558,5559],[4407,4408],[1076,1077],[5226,5227],[6808,6809],[3795,3796],[1366,1367],[7828,7829],[5453,5454],[1204,1205],[3913,3914],[6979,6980],[4336,4337],[5918,5919],[7152,7153],[1685,1686],[4007,4008],[1713,1714],[8232,8233],[2105,2106],[1945,1946],[7501,7502],[2176,2177],[521,522],[3428,3429],[1151,1152],[7597,7598],[2635,2636],[7977,7978],[546,547],[3787,3788],[5257,5258],[3125,3126],[6194,6195],[4995,4996],[7351,7352],[8307,8308],[921,922],[2316,2317],[1272,1273],[3573,3574],[2025,2026],[6010,6011],[243,244],[5692,5693],[1684,1685],[5438,5439],[5223,5224],[175,176],[7072,7073],[655,656],[2487,2488],[6525,6526],[5145,5146],[4065,4066],[3597,3598],[1922,1923],[623,624],[110,111],[3805,3806],[7555,7556],[6295,6296],[6524,6525],[7908,7909],[5866,5867],[5649,5650],[7643,7644],[8089,8090],[3045,3046],[8228,8229],[1689,1690],[8140,8141],[8349,8350],[8300,8301],[1519,1520],[7266,7267],[2965,2966],[1051,1052],[1146,1147],[2076,2077],[4011,4012],[3837,3838],[1163,1164],[3751,3752],[5623,5624],[827,828],[8284,8285],[1462,1463],[1128,1129],[3425,3426],[5085,5086],[1539,1540],[5362,5363],[6998,6999],[6113,6114],[1269,1270],[6642,6643],[3964,3965],[5111,5112],[4766,4767],[8252,8253],[2274,2275],[1525,1526],[5595,5596],[398,399],[1450,1451],[6136,6137],[2326,2327],[2052,2053],[214,215],[7723,7724],[2984,2985],[1094,1095],[3116,3117],[6776,6777],[2050,2051],[2225,2226],[6615,6616],[5444,5445],[7755,7756],[4775,4776],[2325,2326],[2742,2743],[5915,5916],[6476,6477],[7580,7581],[8175,8176],[1129,1130],[3836,3837],[7411,7412],[1237,1238],[1293,1294],[999,1000],[4673,4674],[7562,7563],[5887,5888],[3668,3669],[4802,4803],[4965,4966],[6376,6377],[7189,7190],[4991,4992],[7918,7919],[4648,4649],[1626,1627],[6511,6512],[3105,3106],[7760,7761],[3794,3795],[3299,3300],[1444,1445],[7612,7613],[133,134],[5851,5852],[3686,3687],[6882,6883],[3665,3666],[1728,1729],[3470,3471],[5103,5104],[5620,5621],[7254,7255],[6540,6541],[4030,4031],[4916,4917],[4168,4169],[1651,1652],[303,304],[8126,8127],[402,403],[4245,4246],[8028,8029],[6639,6640],[4771,4772],[4793,4794],[8351,8352],[3657,3658],[5378,5379],[1508,1509],[1696,1697],[3404,3405],[5253,5254],[2196,2197],[5235,5236],[6687,6688],[3193,3194],[6096,6097],[6163,6164],[1073,1074],[2472,2473],[2279,2280],[3282,3283],[2377,2378],[8090,8091],[2647,2648],[5834,5835],[1653,1654],[3292,3293],[1040,1041],[7255,7256],[3851,3852],[6484,6485],[3024,3025],[7103,7104],[858,859],[1981,1982],[5912,5913],[2765,2766],[377,378],[8301,8302],[7086,7087],[2221,2222],[3142,3143],[1355,1356],[787,788],[7838,7839],[3477,3478],[5817,5818],[2419,2420],[2921,2922],[4055,4056],[1324,1325],[1325,1326],[3495,3496],[5668,5669],[5731,5732],[7241,7242],[2750,2751],[5463,5464],[6865,6866],[3051,3052],[6702,6703],[279,280],[1498,1499],[3268,3269],[2376,2377],[362,363],[6021,6022],[7346,7347],[4212,4213],[6395,6396],[5006,5007],[3523,3524],[4217,4218],[6805,6806],[1378,1379],[3091,3092],[6534,6535],[6016,6017],[1895,1896],[5179,5180],[807,808],[1755,1756],[6819,6820],[5125,5126],[204,205],[2463,2464],[7941,7942],[2943,2944],[3506,3507],[569,570],[2095,2096],[3326,3327],[505,506],[3730,3731],[5088,5089],[7774,7775],[3156,3157],[6438,6439],[7112,7113],[8348,8349],[1273,1274],[6581,6582],[3497,3498],[7389,7390],[5051,5052],[5744,5745],[4550,4551],[2577,2578],[5904,5905],[87,88],[51,52],[3218,3219],[3655,3656],[1736,1737],[2845,2846],[2978,2979],[6426,6427],[2712,2713],[1360,1361],[652,653],[1568,1569],[6918,6919],[5678,5679],[6416,6417],[4684,4685],[3681,3682],[1327,1328],[7002,7003],[1698,1699],[6439,6440],[1390,1391],[904,905],[4986,4987],[570,571],[1510,1511],[4141,4142],[5058,5059],[1434,1435],[4885,4886],[5059,5060],[2041,2042],[7790,7791],[5170,5171],[5962,5963],[7304,7305],[5767,5768],[2757,2758],[786,787],[408,409],[5611,5612],[614,615],[6291,6292],[694,695],[7436,7437],[3820,3821],[7970,7971],[6013,6014],[1280,1281],[5821,5822],[7778,7779],[6034,6035],[3652,3653],[6157,6158],[7981,7982],[7788,7789],[95,96],[2516,2517],[6913,6914],[7938,7939],[6065,6066],[736,737],[5909,5910],[1003,1004],[309,310],[2310,2311],[588,589],[6423,6424],[4304,4305],[2444,2445],[2907,2908],[912,913],[1346,1347],[3099,3100],[8294,8295],[3097,3098],[7305,7306],[3678,3679],[2701,2702],[6732,6733],[2538,2539],[2412,2413],[1306,1307],[4873,4874],[7934,7935],[7897,7898],[8001,8002],[6875,6876],[3432,3433],[1218,1219],[5873,5874],[4843,4844],[2307,2308],[5333,5334],[8147,8148],[3130,3131],[8046,8047],[2657,2658],[467,468],[5535,5536],[2644,2645],[351,352],[7858,7859],[2130,2131],[2744,2745],[6334,6335],[1811,1812],[7047,7048],[3158,3159],[6028,6029],[1832,1833],[2187,2188],[677,678],[6077,6078],[6684,6685],[7227,7228],[720,721],[139,140],[8036,8037],[4360,4361],[473,474],[4542,4543],[2254,2255],[345,346],[1383,1384],[3277,3278],[2901,2902],[7649,7650],[5242,5243],[5264,5265],[1581,1582],[7827,7828],[3021,3022],[4860,4861],[3555,3556],[8151,8152],[638,639],[2042,2043],[3897,3898],[3842,3843],[8051,8052],[3436,3437],[3890,3891],[3509,3510],[5773,5774],[4606,4607],[2853,2854],[6445,6446],[4468,4469],[2719,2720],[4446,4447],[3894,3895],[1006,1007],[8319,8320],[2245,2246],[2391,2392],[1341,1342],[2616,2617],[5940,5941],[6694,6695],[6130,6131],[5865,5866],[4384,4385],[226,227],[4514,4515],[4842,4843],[4142,4143],[2324,2325],[1986,1987],[5892,5893],[716,717],[5041,5042],[349,350],[8222,8223],[3402,3403],[6970,6971],[5030,5031],[2903,2904],[5733,5734],[5413,5414],[5847,5848],[389,390],[1408,1409],[1056,1057],[234,235],[7984,7985],[2510,2511],[4025,4026],[1521,1522],[558,559],[5225,5226],[94,95],[5574,5575],[8159,8160],[5171,5172],[2547,2548],[1514,1515],[2375,2376],[3203,3204],[237,238],[1976,1977],[4925,4926],[22,23],[343,344],[1388,1389],[216,217],[2668,2669],[2288,2289],[1024,1025],[7696,7697],[2215,2216],[683,684],[7007,7008],[7137,7138],[3786,3787],[4946,4947],[2553,2554],[1932,1933],[7574,7575],[8207,8208],[6589,6590],[5430,5431],[2192,2193],[132,133],[1159,1160],[3950,3951],[5839,5840],[7385,7386],[854,855],[1158,1159],[7825,7826],[2399,2400],[2290,2291],[2514,2515],[8141,8142],[6170,6171],[7462,7463],[5443,5444],[6515,6516],[290,291],[6399,6400],[2434,2435],[186,187],[1901,1902],[7040,7041],[7586,7587],[7602,7603],[8101,8102],[5323,5324],[7860,7861],[229,230],[2068,2069],[6374,6375],[230,231],[7198,7199],[3433,3434],[2638,2639],[6311,6312],[1936,1937],[1284,1285],[5478,5479],[969,970],[2164,2165],[805,806],[7829,7830],[1562,1563],[4745,4746],[7547,7548],[1463,1464],[3017,3018],[5487,5488],[2217,2218],[3988,3989],[7511,7512],[5485,5486],[691,692],[7626,7627],[5978,5979],[6614,6615],[3804,3805],[2218,2219],[2384,2385],[2648,2649],[2718,2719],[5486,5487],[2776,2777],[8260,8261],[2810,2811],[2861,2862],[5511,5512],[1865,1866],[3789,3790],[5221,5222],[2352,2353],[5704,5705],[2545,2546],[3755,3756],[8340,8341],[1336,1337],[2170,2171],[954,955],[2408,2409],[5641,5642],[7943,7944],[3336,3337],[1872,1873],[2231,2232],[1630,1631],[6686,6687],[3023,3024],[6184,6185],[1678,1679],[3548,3549],[6974,6975],[6410,6411],[5844,5845],[3682,3683],[194,195],[8137,8138],[3030,3031],[1617,1618],[26,27],[5234,5235],[1625,1626],[629,630],[7929,7930],[658,659],[7452,7453],[4165,4166],[2350,2351],[7118,7119],[2918,2919],[3884,3885],[551,552],[626,627],[1835,1836],[4206,4207],[5973,5974],[2142,2143],[6053,6054],[3985,3986],[4586,4587],[6656,6657],[6482,6483],[7648,7649],[3761,3762],[4571,4572],[8354,8355],[5331,5332],[3954,3955],[4883,4884],[1749,1750],[2629,2630],[210,211],[6889,6890],[6380,6381],[168,169],[129,130],[3405,3406],[2008,2009],[4223,4224],[2312,2313],[5585,5586],[2772,2773],[6609,6610],[1720,1721],[6880,6881],[2259,2260],[7089,7090],[5188,5189],[7853,7854],[1929,1930],[4114,4115],[3304,3305],[5044,5045],[942,943],[8274,8275],[6433,6434],[1670,1671],[7048,7049],[6035,6036],[1563,1564],[3137,3138],[4714,4715],[1745,1746],[7018,7019],[6370,6371],[2009,2010],[3303,3304],[4757,4758],[4721,4722],[5712,5713],[4911,4912],[6523,6524],[6674,6675],[8095,8096],[3149,3150],[493,494],[4492,4493],[3136,3137],[1401,1402],[532,533],[3920,3921],[5721,5722],[7456,7457],[8178,8179],[8299,8300],[3750,3751],[5241,5242],[1768,1769],[3534,3535],[379,380],[2824,2825],[2527,2528],[3510,3511],[3167,3168],[3577,3578],[5552,5553],[3635,3636],[6475,6476],[6762,6763],[358,359],[2605,2606],[1428,1429],[2612,2613],[6062,6063],[2825,2826],[6640,6641],[6886,6887],[7449,7450],[1252,1253],[108,109],[2424,2425],[2194,2195],[401,402],[7197,7198],[3971,3972],[2294,2295],[71,72],[2145,2146],[4845,4846],[4551,4552],[4340,4341],[3443,3444],[1063,1064],[6849,6850],[1059,1060],[6864,6865],[7130,7131],[6602,6603],[4470,4471],[6354,6355],[3683,3684],[4069,4070],[3254,3255],[3354,3355],[6030,6031],[882,883],[4564,4565],[2593,2594],[3640,3641],[6386,6387],[5945,5946],[814,815],[6754,6755],[3906,3907],[1188,1189],[6019,6020],[5416,5417],[603,604],[1117,1118],[5513,5514],[4668,4669],[1201,1202],[6616,6617],[5243,5244],[3899,3900],[4754,4755],[6281,6282],[4113,4114],[5392,5393],[2936,2937],[6940,6941],[4382,4383],[7552,7553],[4742,4743],[1143,1144],[3792,3793],[5554,5555],[5117,5118],[3845,3846],[5951,5952],[4177,4178],[6195,6196],[1168,1169],[3031,3032],[7782,7783],[3857,3858],[573,574],[3140,3141],[3621,3622],[8314,8315],[2413,2414],[4040,4041],[2291,2292],[5877,5878],[4377,4378],[1160,1161],[1935,1936],[5625,5626],[4944,4945],[3335,3336],[8238,8239],[7324,7325],[2808,2809],[6603,6604],[3526,3527],[7544,7545],[6005,6006],[181,182],[7258,7259],[1967,1968],[7963,7964],[4322,4323],[2620,2621],[5442,5443],[4174,4175],[6630,6631],[6558,6559],[5553,5554],[3800,3801],[5670,5671],[7146,7147],[1141,1142],[5467,5468],[323,324],[8308,8309],[4366,4367],[5986,5987],[3380,3381],[1987,1988],[5328,5329],[3118,3119],[4128,4129],[5475,5476],[6693,6694],[3444,3445],[2305,2306],[1308,1309],[1121,1122],[3361,3362],[3333,3334],[974,975],[7613,7614],[6838,6839],[135,136],[3872,3873],[6960,6961],[2730,2731],[5347,5348],[4719,4720],[4166,4167],[3995,3996],[2726,2727],[7397,7398],[2251,2252],[5079,5080],[451,452],[7976,7977],[8355,8356],[4482,4483],[8311,8312],[4308,4309],[4116,4117],[839,840],[5396,5397],[4971,4972],[1686,1687],[6624,6625],[4054,4055],[2658,2659],[3989,3990],[103,104],[6074,6075],[28,29],[499,500],[472,473],[5781,5782],[4772,4773],[4999,5000],[7073,7074],[1782,1783],[2543,2544],[748,749],[7692,7693],[3519,3520],[249,250],[7732,7733],[2473,2474],[7066,7067],[206,207],[7556,7557],[7848,7849],[2887,2888],[8250,8251],[308,309],[165,166],[2075,2076],[3628,3629],[803,804],[1552,1553],[7810,7811],[5618,5619],[3188,3189],[5393,5394],[6770,6771],[4627,4628],[5659,5660],[3844,3845],[7446,7447],[6758,6759],[5653,5654],[3958,3959],[601,602],[5069,5070],[4881,4882],[7857,7858],[8056,8057],[4796,4797],[2361,2362],[2708,2709],[2597,2598],[2281,2282],[6140,6141],[7292,7293],[3707,3708],[518,519],[977,978],[5205,5206],[941,942],[3466,3467],[4671,4672],[5146,5147],[3390,3391],[317,318],[1764,1765],[6100,6101],[452,453],[6032,6033],[4607,4608],[3070,3071],[3900,3901],[3391,3392],[1646,1647],[7991,7992],[2814,2815],[1021,1022],[1478,1479],[6572,6573],[7243,7244],[5367,5368],[1214,1215],[2298,2299],[1966,1967],[7867,7868],[6538,6539],[7811,7812],[2952,2953],[7230,7231],[4279,4280],[503,504],[8310,8311],[4977,4978],[6855,6856],[7563,7564],[6937,6938],[661,662],[1701,1702],[8168,8169],[5014,5015],[4808,4809],[3830,3831],[3223,3224],[47,48],[5707,5708],[6984,6985],[4248,4249],[4587,4588],[2393,2394],[5790,5791],[7479,7480],[3383,3384],[7343,7344],[7336,7337],[5419,5420],[7216,7217],[3616,3617],[1797,1798],[1248,1249],[4890,4891],[4689,4690],[894,895],[7808,7809],[7039,7040],[6537,6538],[3711,3712],[4811,4812],[5551,5552],[4233,4234],[5152,5153],[2627,2628],[6320,6321],[5371,5372],[185,186],[5900,5901],[253,254],[5748,5749],[5993,5994],[352,353],[4186,4187],[951,952],[1217,1218],[7797,7798],[3524,3525],[512,513],[7824,7825],[1962,1963],[68,69],[8115,8116],[7263,7264],[7842,7843],[4753,4754],[2074,2075],[6192,6193],[1386,1387],[1807,1808],[5303,5304],[1361,1362],[6548,6549],[1859,1860],[4709,4710],[4647,4648],[3173,3174],[2208,2209],[1134,1135],[1294,1295],[4862,4863],[4533,4534],[322,323],[3835,3836],[3072,3073],[1524,1525],[1023,1024],[8014,8015],[5755,5756],[5028,5029],[7123,7124],[1431,1432],[6040,6041],[4731,4732],[6066,6067],[8013,8014],[3472,3473],[46,47],[3834,3835],[3832,3833],[5203,5204],[1300,1301],[6745,6746],[366,367],[2498,2499],[1132,1133],[5811,5812],[7001,7002],[4108,4109],[1529,1530],[7634,7635],[8004,8005],[1923,1924],[7370,7371],[906,907],[8061,8062],[7855,7856],[3473,3474],[3134,3135],[7650,7651],[3330,3331],[5729,5730],[7961,7962],[486,487],[6267,6268],[5694,5695],[1028,1029],[1659,1660],[810,811],[2138,2139],[5087,5088],[5703,5704],[3596,3597],[2292,2293],[4362,4363],[889,890],[98,99],[8121,8122],[2436,2437],[1469,1470],[2955,2956],[464,465],[2452,2453],[5932,5933],[3400,3401],[6201,6202],[3801,3802],[4261,4262],[1913,1914],[1455,1456],[6165,6166],[4026,4027],[7603,7604],[5544,5545],[2439,2440],[8024,8025],[5745,5746],[1814,1815],[7863,7864],[742,743],[8172,8173],[8198,8199],[3166,3167],[4194,4195],[2323,2324],[7177,7178],[5880,5881],[4292,4293],[3656,3657],[795,796],[5021,5022],[6813,6814],[6057,6058],[1205,1206],[2209,2210],[7095,7096],[4134,4135],[4009,4010],[7025,7026],[6506,6507],[6795,6796],[7719,7720],[6261,6262],[5855,5856],[8037,8038],[6121,6122],[3880,3881],[5864,5865],[5220,5221],[3580,3581],[5053,5054],[7607,7608],[6209,6210],[2333,2334],[3932,3933],[1948,1949],[390,391],[5677,5678],[6470,6471],[8333,8334],[685,686],[4823,4824],[5206,5207],[7055,7056],[1997,1998],[2683,2684],[2353,2354],[3558,3559],[880,881],[2655,2656],[8244,8245],[6279,6280],[4161,4162],[3860,3861],[4846,4847],[1919,1920],[3752,3753],[8059,8060],[531,532],[6215,6216],[2311,2312],[8171,8172],[886,887],[5151,5152],[6080,6081],[8329,8330],[4915,4916],[6418,6419],[2529,2530],[837,838],[3178,3179],[5345,5346],[79,80],[6627,6628],[5902,5903],[4893,4894],[5279,5280],[6319,6320],[7663,7664],[3938,3939],[4267,4268],[3560,3561],[1993,1994],[7916,7917],[8073,8074],[562,563],[4443,4444],[668,669],[6638,6639],[8103,8104],[5536,5537],[1507,1508],[3957,3958],[884,885],[4652,4653],[2088,2089],[5885,5886],[5023,5024],[3088,3089],[961,962],[5334,5335],[681,682],[6068,6069],[6239,6240],[1120,1121],[7208,7209],[3500,3501],[669,670],[3016,3017],[6208,6209],[6056,6057],[5823,5824],[6148,6149],[5248,5249],[320,321],[6054,6055],[4510,4511],[4476,4477],[6147,6148],[1730,1731],[5266,5267],[6507,6508],[847,848],[5655,5656],[5589,5590],[6662,6663],[6894,6895],[5420,5421],[975,976],[7886,7887],[336,337],[2211,2212],[7448,7449],[5799,5800],[1047,1048],[8085,8086],[1747,1748],[995,996],[7769,7770],[5543,5544],[4454,4455],[7315,7316],[751,752],[1285,1286],[3515,3516],[5045,5046],[7512,7513],[6125,6126],[7730,7731],[4844,4845],[1716,1717],[1319,1320],[6257,6258],[7069,7070],[2021,2022],[4301,4302],[2190,2191],[3363,3364],[8032,8033],[299,300],[7420,7421],[4358,4359],[2818,2819],[4373,4374],[4555,4556],[3170,3171],[7325,7326],[4536,4537],[52,53],[2607,2608],[5613,5614],[2319,2320],[4017,4018],[4602,4603],[6440,6441],[5765,5766],[6431,6432],[2440,2441],[4228,4229],[6734,6735],[8009,8010],[3263,3264],[1064,1065],[7083,7084],[1380,1381],[6991,6992],[5835,5836],[7107,7108],[2032,2033],[6692,6693],[1001,1002],[7193,7194],[248,249],[40,41],[3852,3853],[1194,1195],[7023,7024],[1305,1306],[6613,6614],[6413,6414],[6520,6521],[5698,5699],[4955,4956],[4888,4889],[6407,6408],[4283,4284],[6729,6730],[3204,3205],[5529,5530],[6178,6179],[4744,4745],[7010,7011],[1371,1372],[5806,5807],[3636,3637],[1166,1167],[4392,4393],[8015,8016],[3396,3397],[1558,1559],[1982,1983],[7057,7058],[2442,2443],[5599,5600],[2816,2817],[7880,7881],[6683,6684],[3975,3976],[7684,7685],[929,930],[6299,6300],[3119,3120],[7896,7897],[6948,6949],[7683,7684],[5246,5247],[2476,2477],[7279,7280],[1631,1632],[7599,7600],[3240,3241],[3669,3670],[4056,4057],[3010,3011],[5123,5124],[6555,6556],[5193,5194],[2806,2807],[1356,1357],[724,725],[836,837],[4133,4134],[7840,7841],[1384,1385],[4442,4443],[7388,7389],[1114,1115],[1402,1403],[7245,7246],[5019,5020],[4681,4682],[7904,7905],[5743,5744],[3174,3175],[3479,3480],[1989,1990],[5287,5288],[3618,3619],[6828,6829],[5916,5917],[1391,1392],[3850,3851],[6465,6466],[4394,4395],[7418,7419],[1131,1132],[5124,5125],[1470,1471],[1588,1589],[1892,1893],[5361,5362],[1574,1575],[1861,1862],[5917,5918],[7806,7807],[2072,2073],[6812,6813],[2795,2796],[3189,3190],[7942,7943],[4791,4792],[5496,5497],[3352,3353],[3532,3533],[350,351],[2822,2823],[7845,7846],[3037,3038],[4940,4941],[7167,7168],[1956,1957],[5335,5336],[1759,1760],[6982,6983],[834,835],[8223,8224],[4608,4609],[2817,2818],[5740,5741],[6635,6636],[2289,2290],[6518,6519],[6761,6762],[5907,5908],[5346,5347],[6043,6044],[6454,6455],[3417,3418],[2058,2059],[7278,7279],[1504,1505],[4661,4662],[1435,1436],[5685,5686],[5389,5390],[7515,7516],[4312,4313],[3046,3047],[1034,1035],[2460,2461],[4677,4678],[6298,6299],[1008,1009],[7386,7387],[5201,5202],[5159,5160],[8235,8236],[1403,1404],[7865,7866],[3350,3351],[8041,8042],[5402,5403],[7964,7965],[8263,8264],[3746,3747],[1484,1485],[1627,1628],[2083,2084],[5719,5720],[5237,5238],[2464,2465],[723,724],[4983,4984],[4243,4244],[3111,3112],[6079,6080],[2493,2494],[610,611],[4615,4616],[4341,4342],[4784,4785],[7913,7914],[4959,4960],[820,821],[5658,5659],[8240,8241],[4266,4267],[6495,6496],[1750,1751],[6049,6050],[4169,4170],[1531,1532],[5276,5277],[7591,7592],[1447,1448],[5126,5127],[5035,5036],[5610,5611],[5160,5161],[3550,3551],[4621,4622],[608,609],[4138,4139],[2809,2810],[3776,3777],[4692,4693],[4423,4424],[4727,4728],[6012,6013],[6156,6157],[8206,8207],[2253,2254],[3714,3715],[6408,6409],[3553,3554],[7450,7451],[1259,1260],[70,71],[4085,4086],[8247,8248],[3619,3620],[4264,4265],[3322,3323],[7899,7900],[3378,3379],[4099,4100],[7349,7350],[7700,7701],[944,945],[3150,3151],[867,868],[2451,2452],[5642,5643],[7282,7283],[3090,3091],[332,333],[8255,8256],[3824,3825],[4698,4699],[2999,3000],[6533,6534],[7383,7384],[4664,4665],[2920,2921],[7960,7961],[1261,1262],[3155,3156],[223,224],[2563,2564],[4704,4705],[2524,2525],[393,394],[4282,4283],[4029,4030],[2073,2074],[2486,2487],[5431,5432],[7951,7952],[118,119],[2356,2357],[6575,6576],[7667,7668],[2860,2861],[6769,6770],[7273,7274],[2334,2335],[2512,2513],[5509,5510],[2732,2733],[3110,3111],[8261,8262],[4490,4491],[822,823],[4943,4944],[5985,5986],[3488,3489],[404,405],[7151,7152],[2916,2917],[1544,1545],[3915,3916],[6017,6018],[6047,6048],[6331,6332],[1089,1090],[7596,7597],[6724,6725],[7708,7709],[7053,7054],[3901,3902],[145,146],[2120,2121],[2711,2712],[6517,6518],[1779,1780],[8285,8286],[6945,6946],[6872,6873],[4499,4500],[8120,8121],[6957,6958],[5984,5985],[4683,4684],[4624,4625],[7437,7438],[3903,3904],[7573,7574],[426,427],[7741,7742],[3381,3382],[4947,4948],[5375,5376],[3979,3980],[3818,3819],[5586,5587],[5934,5935],[4724,4725],[4717,4718],[421,422],[2852,2853],[966,967],[6648,6649],[5766,5767],[5250,5251],[2830,2831],[1303,1304],[4344,4345],[4335,4336],[5867,5868],[887,888],[7226,7227],[29,30],[3135,3136],[7920,7921],[7966,7967],[6435,6436],[6814,6815],[3571,3572],[3129,3130],[3199,3200],[2523,2524],[6405,6406],[6196,6197],[4729,4730],[3184,3185],[292,293],[2144,2145],[3409,3410],[3365,3366],[1184,1185],[458,459],[741,742],[6536,6537],[2915,2916],[489,490],[6037,6038],[4588,4589],[3924,3925],[1667,1668],[4960,4961],[8195,8196],[3712,3713],[3600,3601],[4628,4629],[6124,6125],[2304,2305],[8219,8220],[7979,7980],[3449,3450],[2856,2857],[5210,5211],[6403,6404],[4419,4420],[7253,7254],[156,157],[200,201],[1071,1072],[7492,7493],[3862,3863],[6234,6235],[3612,3613],[7050,7051],[2461,2462],[7318,7319],[6543,6544],[5925,5926],[8100,8101],[2284,2285],[5795,5796],[5545,5546],[1975,1976],[2540,2541],[6007,6008],[7094,7095],[2640,2641],[6719,6720],[2525,2526],[3575,3576],[4876,4877],[3025,3026],[8346,8347],[5355,5356],[1229,1230],[4611,4612],[4572,4573],[3316,3317],[447,448],[4616,4617],[2991,2992],[6935,6936],[8048,8049],[3048,3049],[2669,2670],[7766,7767],[1031,1032],[6608,6609],[2174,2175],[7687,7688],[604,605],[6082,6083],[7360,7361],[8313,8314],[6949,6950],[862,863],[7874,7875],[7558,7559],[3540,3541],[1065,1066],[5247,5248],[2178,2179],[6448,6449],[1176,1177],[3905,3906],[7931,7932],[3970,3971],[5512,5513],[5147,5148],[4592,4593],[5556,5557],[902,903],[930,931],[2064,2065],[4534,4535],[4246,4247],[5299,5300],[7627,7628],[7469,7470],[5726,5727],[7669,7670],[5531,5532],[8044,8045],[7067,7068],[6565,6566],[7956,7957],[4059,4060],[5200,5201],[8058,8059],[371,372],[950,951],[7491,7492],[4207,4208],[4399,4400],[3994,3995],[3257,3258],[4595,4596],[6347,6348],[808,809],[6446,6447],[7792,7793],[1597,1598],[749,750],[2394,2395],[5290,5291],[4338,4339],[6351,6352],[5730,5731],[3462,3463],[5737,5738],[7691,7692],[567,568],[7721,7722],[1666,1667],[4718,4719],[1591,1592],[1352,1353],[3490,3491],[3883,3884],[6884,6885],[7124,7125],[1424,1425],[5778,5779],[4700,4701],[5316,5317],[7396,7397],[5154,5155],[1193,1194],[7973,7974],[2673,2674],[4123,4124],[1338,1339],[2666,2667],[273,274],[8266,8267],[943,944],[7077,7078],[1410,1411],[8042,8043],[7062,7063],[7637,7638],[967,968],[6428,6429],[5507,5508],[5672,5673],[7204,7205],[2371,2372],[5784,5785],[4723,4724],[7819,7820],[2924,2925],[5830,5831],[7527,7528],[6349,6350],[4856,4857],[6241,6242],[50,51],[8123,8124],[856,857],[1133,1134],[2475,2476],[1900,1901],[6977,6978],[1077,1078],[1137,1138],[7668,7669],[5971,5972],[2707,2708],[4019,4020],[871,872],[1310,1311],[6172,6173],[5969,5970],[4804,4805],[3559,3560],[1692,1693],[4817,4818],[3738,3739],[1677,1678],[8236,8237],[2004,2005],[6067,6068],[5047,5048],[6545,6546],[5258,5259],[315,316],[2904,2905],[5774,5775],[6767,6768],[6978,6979],[8092,8093],[508,509],[7401,7402],[6824,6825],[5686,5687],[4493,4494],[5990,5991],[6601,6602],[7852,7853],[2405,2406],[3601,3602],[2509,2510],[6791,6792],[3868,3869],[1497,1498],[8080,8081],[6539,6540],[154,155],[6225,6226],[2766,2767],[4050,4051],[1212,1213],[2909,2910],[19,20],[5831,5832],[869,870],[4526,4527],[5863,5864],[58,59],[6664,6665],[1274,1275],[3531,3532],[3060,3061],[6071,6072],[2788,2789],[5314,5315],[6499,6500],[1436,1437],[7817,7818],[5185,5186],[6594,6595],[1412,1413],[1049,1050],[6469,6470],[5963,5964],[721,722],[3041,3042],[1731,1732],[8230,8231],[3256,3257],[842,843],[8119,8120],[3590,3591],[2403,2404],[5564,5565],[5222,5223],[7030,7031],[1256,1257],[4788,4789],[4525,4526],[6911,6912],[4511,4512],[2702,2703],[5001,5002],[2368,2369],[5757,5758],[4289,4290],[2769,2770],[6204,6205],[6500,6501],[8220,8221],[7781,7782],[1613,1614],[5842,5843],[7408,7409],[18,19],[6169,6170],[7090,7091],[2511,2512],[4291,4292],[740,741],[6273,6274],[4537,4538],[2854,2855],[3028,3029],[3081,3082],[873,874],[6519,6520],[265,266],[5427,5428],[2337,2338],[2694,2695],[5350,5351],[2223,2224],[2995,2996],[4193,4194],[703,704],[3499,3500],[36,37],[800,801],[8291,8292],[3377,3378],[4176,4177],[6149,6150],[2133,2134],[384,385],[4904,4905],[7284,7285],[899,900],[7868,7869],[7577,7578],[3936,3937],[4840,4841],[78,79],[1614,1615],[342,343],[3736,3737],[1688,1689],[1358,1359],[5742,5743],[7153,7154],[3202,3203],[7935,7936],[7232,7233],[578,579],[7747,7748],[1195,1196],[1220,1221],[8146,8147],[5631,5632],[4339,4340],[1416,1417],[2157,2158],[7773,7774],[7969,7970],[2044,2045],[1856,1857],[6836,6837],[5068,5069],[6583,6584],[4400,4401],[7300,7301],[142,143],[2630,2631],[575,576],[1957,1958],[3395,3396],[115,116],[3727,3728],[4359,4360],[3233,3234],[6375,6376],[2759,2760],[2471,2472],[1793,1794],[4015,4016],[1365,1366],[6655,6656],[1725,1726],[2112,2113],[4319,4320],[702,703],[2363,2364],[8227,8228],[4051,4052],[4457,4458],[8135,8136],[7664,7665],[1058,1059],[2758,2759],[167,168],[5318,5319],[1055,1056],[6429,6430],[5061,5062],[2061,2062],[3133,3134],[5150,5151],[838,839],[2642,2643],[6925,6926],[1876,1877],[5384,5385],[6496,6497],[6953,6954],[441,442],[1914,1915],[7459,7460],[3486,3487],[2097,2098],[3598,3599],[1125,1126],[3177,3178],[6652,6653],[774,775],[6206,6207],[4179,4180],[2716,2717],[2997,2998],[911,912],[6466,6467],[8183,8184],[3368,3369],[6826,6827],[4584,4585],[5903,5904],[1140,1141],[879,880],[4067,4068],[1415,1416],[3569,3570],[6001,6002],[5048,5049],[4705,4706],[3976,3977],[2297,2298],[6706,6707],[2454,2455],[7328,7329],[193,194],[7306,7307],[2573,2574],[1509,1510],[3885,3886],[4201,4202],[4237,4238],[5462,5463],[2850,2851],[6202,6203],[7494,7495],[4355,4356],[5142,5143],[1315,1316],[3359,3360],[5567,5568],[2462,2463],[6464,6465],[3803,3804],[2160,2161],[3766,3767],[7026,7027],[2863,2864],[7186,7187],[4256,4257],[2043,2044],[7922,7923],[7765,7766],[5357,5358],[3403,3404],[7553,7554],[1628,1629],[3814,3815],[1245,1246],[6708,6709],[235,236],[1096,1097],[8055,8056],[1045,1046],[6743,6744],[1972,1973],[3450,3451],[2572,2573],[140,141],[3687,3688],[6412,6413],[1228,1229],[2101,2102],[2416,2417],[3877,3878],[8350,8351],[6571,6572],[5281,5282],[2998,2999],[2360,2361],[6807,6808],[1658,1659],[4735,4736],[2780,2781],[4272,4273],[6786,6787],[7348,7349],[3562,3563],[8143,8144],[5852,5853],[6845,6846],[2934,2935],[4630,4631],[5321,5322],[3926,3927],[5191,5192],[4414,4415],[7043,7044],[7187,7188],[3480,3481],[7277,7278],[1551,1552],[675,676],[4507,4508],[6514,6515],[3799,3800],[4365,4366],[2689,2690],[7902,7903],[3948,3949],[3469,3470],[7115,7116],[1874,1875],[4722,4723],[5197,5198],[4651,4652],[5787,5788],[4984,4985],[2802,2803],[23,24],[2625,2626],[5720,5721],[596,597],[461,462],[6763,6764],[7131,7132],[4877,4878],[1246,1247],[7220,7221],[4441,4442],[4060,4061],[3126,3127],[296,297],[7731,7732],[7582,7583],[4565,4566],[254,255],[2268,2269],[3478,3479],[4909,4910],[5209,5210],[6217,6218],[1620,1621],[7296,7297],[2395,2396],[777,778],[1208,1209],[1343,1344],[6737,6738],[1828,1829],[4447,4448],[4240,4241],[6211,6212],[1233,1234],[3288,3289],[1781,1782],[1836,1837],[3187,3188],[3387,3388],[5679,5680],[7064,7065],[7156,7157],[8158,8159],[8091,8092],[5012,5013],[7350,7351],[589,590],[3513,3514],[2969,2970],[2338,2339],[3210,3211],[4835,4836],[4982,4983],[3983,3984],[2131,2132],[2675,2676],[4779,4780],[7214,7215],[3579,3580],[2111,2112],[457,458],[6588,6589],[1776,1777],[6118,6119],[5959,5960],[7921,7922],[8153,8154],[4488,4489],[6666,6667],[3689,3690],[4841,4842],[2941,2942],[1693,1694],[637,638],[6024,6025],[8060,8061],[2158,2159],[5238,5239],[7635,7636],[7546,7547],[1570,1571],[6626,6627],[3778,3779],[3009,3010],[7609,7610],[6685,6686],[217,218],[3567,3568],[6430,6431],[4580,4581],[1949,1950],[2797,2798],[3358,3359],[711,712],[6679,6680],[1442,1443],[7772,7773],[1586,1587],[4149,4150],[663,664],[5018,5019],[3451,3452],[8181,8182],[6073,6074],[2754,2755],[1804,1805],[5330,5331],[3615,3616],[8273,8274],[8182,8183],[7953,7954],[1279,1280],[7528,7529],[3543,3544],[2302,2303],[690,691],[5615,5616],[1963,1964],[5820,5821],[1561,1562],[407,408],[1882,1883],[7353,7354],[5576,5577],[2705,2706],[4471,4472],[8057,8058],[5356,5357],[4750,4751],[1429,1430],[591,592],[3865,3866],[4528,4529],[5500,5501],[8210,8211],[6577,6578],[6092,6093],[7620,7621],[6644,6645],[7680,7681],[7674,7675],[4952,4953],[27,28],[3008,3009],[5549,5550],[1871,1872],[2835,2836],[2226,2227],[4770,4771],[3498,3499],[196,197],[6784,6785],[1505,1506],[1607,1608],[4286,4287],[3440,3441],[6132,6133],[6468,6469],[280,281],[6847,6848],[684,685],[5547,5548],[5979,5980],[3733,3734],[372,373],[49,50],[2295,2296],[3624,3625],[1862,1863],[5494,5495],[7427,7428],[1594,1595],[1530,1531],[7705,7706],[2541,2542],[8305,8306],[8144,8145],[1998,1999],[5158,5159],[382,383],[6238,6239],[1354,1355],[6391,6392],[1437,1438],[6109,6110],[561,562],[6309,6310],[5930,5931],[4238,4239],[7379,7380],[3328,3329],[819,820],[6452,6453],[6748,6749],[119,120],[1192,1193],[7818,7819],[1599,1600],[6245,6246],[7205,7206],[2365,2366],[5603,5604],[6619,6620],[3468,3469],[4451,4452],[386,387],[1439,1440],[6718,6719],[956,957],[1186,1187],[7716,7717],[400,401],[4181,4182],[5648,5649],[5701,5702],[6939,6940],[6739,6740],[7257,7258],[3058,3059],[1368,1369],[4699,4700],[286,287],[1154,1155],[1988,1989],[6738,6739],[6833,6834],[4066,4067],[949,950],[804,805],[337,338],[7836,7837],[3238,3239],[2686,2687],[6036,6037],[4997,4998],[1477,1478],[7917,7918],[4345,4346],[727,728],[4886,4887],[4861,4862],[5675,5676],[1029,1030],[6997,6998],[2590,2591],[6055,6056],[6883,6884],[3062,3063],[6198,6199],[935,936],[5388,5389],[4918,4919],[289,290],[5136,5137],[2116,2117],[6479,6480],[3367,3368],[1944,1945],[8099,8100],[7272,7273],[2729,2730],[6346,6347],[4501,4502],[1881,1882],[3460,3461],[4281,4282],[3585,3586],[6137,6138],[2027,2028],[422,423],[3114,3115],[4198,4199],[6607,6608],[3998,3999],[5112,5113],[542,543],[5354,5355],[1780,1781],[1206,1207],[4669,4670],[4898,4899],[8110,8111],[209,210],[2264,2265],[2996,2997],[1379,1380],[619,620],[4486,4487],[8203,8204],[4633,4634],[4921,4922],[4912,4913],[4634,4635],[5565,5566],[6219,6220],[4529,4530],[511,512],[3007,3008],[1886,1887],[7928,7929],[8188,8189],[1043,1044],[4061,4062],[445,446],[7925,7926],[6830,6831],[6551,6552],[2682,2683],[6424,6425],[8325,8326],[7422,7423],[1683,1684],[4080,4081],[2677,2678],[1566,1567],[1165,1166],[5652,5653],[2193,2194],[5732,5733],[5710,5711],[3349,3350],[4578,4579],[5348,5349],[6449,6450],[1676,1677],[3812,3813],[6317,6318],[3739,3740],[8265,8266],[2813,2814],[698,699],[5813,5814],[3907,3908],[6920,6921],[1656,1657],[3826,3827],[3374,3375],[908,909],[919,920],[477,478],[1681,1682],[3688,3689],[5260,5261],[5514,5515],[189,190],[6952,6953],[251,252],[2005,2006],[8122,8123],[8237,8238],[6153,6154],[2077,2078],[1938,1939],[959,960],[1868,1869],[4579,4580],[5716,5717],[1984,1985],[4548,4549],[5665,5666],[4582,4583],[1177,1178],[3572,3573],[3141,3142],[6605,6606],[2346,2347],[6221,6222],[356,357],[4255,4256],[6134,6135],[3018,3019],[4290,4291],[1845,1846],[5905,5906],[765,766],[4280,4281],[2407,2408],[621,622],[4086,4087],[7500,7501],[4720,4721],[2398,2399],[1526,1527],[4897,4898],[7031,7032],[5157,5158],[3947,3948],[3949,3950],[7203,7204],[2003,2004],[1911,1912],[4895,4896],[1714,1715],[5120,5121],[152,153],[3898,3899],[5594,5595],[6792,6793],[667,668],[7114,7115],[4545,4546],[7391,7392],[789,790],[1044,1045],[319,320],[7794,7795],[924,925],[7297,7298],[2542,2543],[752,753],[5955,5956],[2216,2217],[6463,6464],[7826,7827],[6296,6297],[7380,7381],[2023,2024],[5484,5485],[3775,3776],[1351,1352],[5524,5525],[7221,7222],[5140,5141],[7195,7196],[4125,4126],[5325,5326],[5807,5808],[6796,6797],[7889,7890],[4037,4038],[6781,6782],[2618,2619],[7406,7407],[1494,1495],[6816,6817],[6492,6493],[2495,2496],[3078,3079],[7670,7671],[2478,2479],[4929,4930],[2740,2741],[7344,7345],[1493,1494],[4127,4128],[1960,1961],[1978,1979],[5212,5213],[997,998],[5359,5360],[363,364],[5682,5683],[699,700],[6478,6479],[544,545],[4333,4334],[6650,6651],[7841,7842],[2922,2923],[6252,6253],[5616,5617],[3185,3186],[1934,1935],[8327,8328],[988,989],[1554,1555],[586,587],[4988,4989],[7059,7060],[605,606],[4388,4389],[4674,4675],[4610,4611],[425,426],[3892,3893],[5358,5359],[4641,4642],[2185,2186],[1482,1483],[2459,2460],[5488,5489],[3124,3125],[7702,7703],[3346,3347],[7425,7426],[4538,4539],[7433,7434],[2770,2771],[5562,5563],[8281,8282],[8102,8103],[3295,3296],[6859,6860],[1878,1879],[6272,6273],[7993,7994],[4031,4032],[3461,3462],[3951,3952],[3319,3320],[8342,8343],[2373,2374],[7830,7831],[2433,2434],[7210,7211],[6566,6567],[6152,6153],[5080,5081],[2198,2199],[726,727],[7873,7874],[1262,1263],[35,36],[7686,7687],[3881,3882],[4208,4209],[1517,1518],[8352,8353],[5875,5876],[4899,4900],[2745,2746],[2168,2169],[4251,4252],[7262,7263],[6397,6398],[3489,3490],[8160,8161],[5764,5765],[1983,1984],[6578,6579],[2067,2068],[1663,1664],[1638,1639],[4483,4484],[3015,3016],[7191,7192],[7172,7173],[3853,3854],[2437,2438],[6550,6551],[987,988],[2090,2091],[4978,4979],[1490,1491],[2431,2432],[7636,7637],[307,308],[7154,7155],[3147,3148],[7800,7801],[3999,4000],[5861,5862],[7762,7763],[2895,2896],[5483,5484],[5841,5842],[754,755],[6573,6574],[2917,2918],[3530,3531],[5910,5911],[662,663],[4855,4856],[5233,5234],[3067,3068],[1130,1131],[760,761],[1417,1418],[5605,5606],[1593,1594],[5709,5710],[6369,6370],[4761,4762],[6166,6167],[1032,1033],[5996,5997],[2255,2256],[1506,1507],[6325,6326],[1337,1338],[4828,4829],[7879,7880],[1726,1727],[4330,4331],[1549,1550],[4039,4040],[3886,3887],[316,317],[8107,8108],[1603,1604],[7579,7580],[3145,3146],[1110,1111],[124,125],[2748,2749],[3566,3567],[8229,8230],[1789,1790],[7288,7289],[7690,7691],[1904,1905],[3321,3322],[6333,6334],[756,757],[7656,7657],[6400,6401],[1456,1457],[3802,3803],[3375,3376],[364,365],[2351,2352],[7601,7602],[5662,5663],[7413,7414],[2990,2991],[695,696],[3927,3928],[3758,3759],[7333,7334],[5826,5827],[7022,7023],[2031,2032],[2287,2288],[7143,7144],[1173,1174],[2330,2331],[7786,7787],[1844,1845],[5751,5752],[347,348],[177,178],[8094,8095],[1771,1772],[7101,7102],[4190,4191],[268,269],[8279,8280],[392,393],[2594,2595],[2662,2663],[5081,5082],[3708,3709],[7051,7052],[1727,1728],[3937,3938],[6512,6513],[2503,2504],[1200,1201],[1221,1222],[2564,2565],[7424,7425],[5858,5859],[2494,2495],[4575,4576],[2973,2974],[8287,8288],[1105,1106],[715,716],[2246,2247],[7768,7769],[5458,5459],[6959,6960],[2191,2192],[7417,7418],[4801,4802],[972,973],[5952,5953],[5802,5803],[8315,8316],[8136,8137],[5988,5989],[5470,5471],[3545,3546],[2857,2858],[5434,5435],[4829,4830],[190,191],[3089,3090],[7485,7486],[2239,2240],[4363,4364],[3278,3279],[2842,2843],[1010,1011],[3176,3177],[3013,3014],[5862,5863],[6398,6399],[1079,1080],[4139,4140],[4398,4399],[7206,7207],[3587,3588],[1512,1513],[2091,2092],[7063,7064],[6289,6290],[7745,7746],[6752,6753],[7162,7163],[183,184],[687,688],[1425,1426],[7784,7785],[7724,7725],[6580,6581],[6263,6264],[519,520],[5550,5551],[5588,5589],[4311,4312],[66,67],[6060,6061],[590,591],[1615,1616],[5691,5692],[2820,2821],[44,45],[1707,1708],[816,817],[6061,6062],[3471,3472],[2269,2270],[4942,4943],[2720,2721],[5606,5607],[8196,8197],[609,610],[7381,7382],[6108,6109],[2578,2579],[4930,4931],[6915,6916],[8084,8085],[5521,5522],[986,987],[1918,1919],[1278,1279],[182,183],[6313,6314],[1362,1363],[7444,7445],[6384,6385],[3153,3154],[2659,2660],[7087,7088],[469,470],[6254,6255],[2240,2241],[6701,6702],[1313,1314],[8214,8215],[2910,2911],[7703,7704],[7584,7585],[4831,4832],[1864,1865],[3706,3707],[3273,3274],[1991,1992],[3035,3036],[864,865],[3413,3414],[4554,4555],[4004,4005],[821,822],[5121,5122],[7145,7146],[4100,4101],[2876,2877],[2592,2593],[2649,2650],[37,38],[5162,5163],[3364,3365],[7355,7356],[3940,3941],[403,404],[38,39],[1088,1089],[7564,7565],[340,341],[3406,3407],[3216,3217],[2798,2799],[3496,3497],[2562,2563],[7673,7674],[6584,6585],[5440,5441],[6371,6372],[6458,6459],[6981,6982],[2951,2952],[6988,6989],[1974,1975],[2974,2975],[5477,5478],[6414,6415],[6251,6252],[2896,2897],[6675,6676],[791,792],[2093,2094],[7142,7143],[5976,5977],[5840,5841],[6964,6965],[4265,4266],[3769,3770],[2942,2943],[6817,6818],[6226,6227],[6535,6536],[7606,7607],[5591,5592],[1171,1172],[1392,1393],[565,566],[6526,6527],[4987,4988],[5657,5658],[541,542],[4807,4808],[5228,5229],[7150,7151],[7014,7015],[6989,6990],[8345,8346],[3735,3736],[2672,2673],[5468,5469],[1333,1334],[6975,6976],[5398,5399],[2163,2164],[868,869],[1420,1421],[7483,7484],[3933,3934],[3720,3721],[1542,1543],[1451,1452],[5944,5945],[7974,7975],[2282,2283],[8258,8259],[2983,2984],[6793,6794],[7548,7549],[8065,8066],[4296,4297],[176,177],[5432,5433],[276,277],[7321,7322],[4667,4668],[948,949],[5754,5755],[5571,5572],[7624,7625],[2652,2653],[1457,1458],[4894,4895],[6681,6682],[1084,1085],[7982,7983],[813,814],[3441,3442],[3749,3750],[7756,7757],[5557,5558],[1715,1716],[7693,7694],[6944,6945],[6432,6433],[6486,6487],[7744,7745],[5039,5040],[3139,3140],[895,896],[4278,4279],[4473,4474],[3220,3221],[4776,4777],[1894,1895],[794,795],[6651,6652],[5559,5560],[7445,7446],[2056,2057],[3554,3555],[6220,6221],[2868,2869],[7365,7366],[2188,2189],[991,992],[4980,4981],[2595,2596],[5974,5975],[4879,4880],[7798,7799],[120,121],[8011,8012],[1017,1018],[335,336],[3502,3503],[5128,5129],[1762,1763],[7168,7169],[5382,5383],[958,959],[7134,7135],[3902,3903],[2409,2410],[7352,7353],[2438,2439],[1738,1739],[1050,1051],[6326,6327],[896,897],[8295,8296],[8241,8242],[5957,5958],[3609,3610],[4989,4990],[6502,6503],[1629,1630],[7493,7494],[4853,4854],[3154,3155],[4077,4078],[5845,5846],[5032,5033],[5064,5065],[7147,7148],[3339,3340],[7854,7855],[6541,6542],[859,860],[7281,7282],[69,70],[4922,4923],[6697,6698],[6342,6343],[5804,5805],[7802,7803],[2831,2832],[5734,5735],[2033,2034],[747,748],[3742,3743],[2881,2882],[1818,1819],[483,484],[3849,3850],[3420,3421],[7618,7619],[7357,7358],[3797,3798],[7008,7009],[4530,4531],[369,370],[5161,5162],[150,151],[3360,3361],[2879,2880],[2490,2491],[6240,6241],[3146,3147],[7793,7794],[2704,2705],[8269,8270],[4821,4822],[1840,1841],[7495,7496],[1108,1109],[579,580],[6472,6473],[2727,2728],[7795,7796],[701,702],[1830,1831],[3827,3828],[6011,6012],[7487,7488],[7610,7611],[832,833],[648,649],[6344,6345],[7327,7328],[4728,4729],[6114,6115],[2601,2602],[7429,7430],[3274,3275],[6394,6395],[8234,8235],[3813,3814],[5762,5763],[7314,7315],[1831,1832],[778,779],[8104,8105],[1254,1255],[5859,5860],[2089,2090],[6933,6934],[3107,3108],[6018,6019],[2300,2301],[6726,6727],[3338,3339],[3249,3250],[438,439],[4452,4453],[5948,5949],[5943,5944],[4598,4599],[1372,1373],[7971,7972],[2696,2697],[533,534],[2982,2983],[8331,8332],[2182,2183],[7274,7275],[5697,5698],[5426,5427],[3266,3267],[2869,2870],[4495,4496],[2152,2153],[7754,7755],[1577,1578],[4383,4384],[594,595],[4072,4073],[197,198],[1888,1889],[1959,1960],[2492,2493],[1187,1188],[2576,2577],[5336,5337],[5457,5458],[65,66],[5566,5567],[1741,1742],[4522,4523],[7932,7933],[3863,3864],[7804,7805],[1215,1216],[1825,1826],[6637,6638],[5042,5043],[1290,1291],[1491,1492],[4762,4763],[3494,3495],[4427,4428],[8275,8276],[5007,5008],[2535,2536],[5654,5655],[3825,3826],[7955,7956],[1632,1633],[1398,1399],[802,803],[5274,5275],[1033,1034],[2,3],[2450,2451],[582,583],[7567,7568],[4969,4970],[2581,2582],[2693,2694],[6516,6517],[4180,4181],[6504,6505],[7412,7413],[2841,2842],[4254,4255],[4352,4353],[5942,5943],[233,234],[72,73],[1748,1749],[3917,3918],[6483,6484],[6967,6968],[549,550],[597,598],[1502,1503],[4277,4278],[4089,4090],[1332,1333],[5848,5849],[7271,7272],[2939,2940],[5522,5523],[5038,5039],[5219,5220],[931,932],[221,222],[6081,6082],[7480,7481],[1104,1105],[7113,7114],[471,472],[4464,4465],[21,22],[6712,6713],[8249,8250],[1203,1204],[926,927],[4232,4233],[2799,2800],[2953,2954],[7065,7066],[2262,2263],[990,991],[5360,5361],[8186,8187],[8023,8024],[1264,1265],[2971,2972],[4147,4148],[5340,5341],[5583,5584],[169,170],[861,862],[7392,7393],[34,35],[4869,4870],[1145,1146],[665,666],[6825,6826],[3594,3595],[2828,2829],[4094,4095],[3423,3424],[3693,3694],[890,891],[5199,5200],[6190,6191],[8347,8348],[535,536],[1207,1208],[1432,1433],[7213,7214],[6878,6879],[2905,2906],[7570,7571],[219,220],[8075,8076],[3087,3088],[2734,2735],[5639,5640],[6710,6711],[5165,5166],[5311,5312],[875,876],[341,342],[6379,6380],[4656,4657],[7097,7098],[1061,1062],[5760,5761],[6853,6854],[7832,7833],[5593,5594],[2948,2949],[6576,6577],[5832,5833],[7619,7620],[4183,4184],[3334,3335],[500,501],[6749,6750],[5029,5030],[6243,6244],[3221,3222],[5683,5684],[1422,1423],[2382,2383],[7822,7823],[2784,2785],[554,555],[3369,3370],[4644,4645],[4774,4775],[6293,6294],[5465,5466],[5115,5116],[7540,7541],[6353,6354],[4570,4571],[672,673],[2236,2237],[6223,6224],[3522,3523],[6647,6648],[5714,5715],[8112,8113],[4044,4045],[5268,5269],[2787,2788],[442,443],[3591,3592],[7340,7341],[1225,1226],[4956,4957],[6936,6937],[4743,4744],[6963,6964],[7518,7519],[3939,3940],[8245,8246],[6270,6271],[7200,7201],[5309,5310],[1784,1785],[2549,2550],[6277,6278],[6557,6558],[3103,3104],[5546,5547],[4908,4909],[7376,7377],[2637,2638],[5505,5506],[5282,5283],[3529,3530],[5798,5799],[7148,7149],[679,680],[2315,2316],[6075,6076],[4864,4865],[4288,4289],[7298,7299],[7468,7469],[3121,3122],[4070,4071],[7738,7739],[620,621],[2012,2013],[940,941],[6585,6586],[1791,1792],[7658,7659],[1850,1851],[885,886],[6014,6015],[6094,6095],[8077,8078],[7476,7477],[4221,4222],[6366,6367],[8157,8158],[3212,3213],[5055,5056],[628,629],[7497,7498],[3211,3212],[380,381],[3955,3956],[5630,5631],[504,505],[2786,2787],[3332,3333],[7704,7705],[3161,3162],[5365,5366],[1848,1849],[1758,1759],[5249,5250],[7264,7265],[6292,6293],[2651,2652],[678,679],[780,781],[3607,3608],[4521,4522],[2791,2792],[2276,2277],[2875,2876],[1124,1125],[91,92],[5003,5004],[2546,2547],[2568,2569],[1347,1348],[2477,2478],[5508,5509],[8132,8133],[2301,2302],[6787,6788],[8334,8335],[7861,7862],[3785,3786],[495,496],[5964,5965],[6962,6963],[7337,7338],[971,972],[1704,1705],[3757,3758],[6117,6118],[482,483],[2108,2109],[7629,7630],[4805,4806],[7209,7210],[4489,4490],[158,159],[7276,7277],[4852,4853],[5300,5301],[5349,5350],[3384,3385],[3049,3050],[2733,2734],[5604,5605],[3355,3356],[4247,4248],[3225,3226],[4140,4141],[2057,2058],[7888,7889],[7945,7946],[43,44],[5293,5294],[7157,7158],[7320,7321],[7163,7164],[4465,4466],[654,655],[7472,7473],[7076,7077],[7989,7990],[8154,8155],[6144,6145],[5718,5719],[488,489],[2449,2450],[3952,3953],[2947,2948],[7736,7737],[4081,4082],[3503,3504],[1915,1916],[5306,5307],[4229,4230],[1191,1192],[2838,2839],[2028,2029],[5459,5460],[4875,4876],[3741,3742],[2836,2837],[2636,2637],[2544,2545],[4013,4014],[4637,4638],[1330,1331],[5674,5675],[484,485],[1757,1758],[3996,3997],[6419,6420],[3327,3328],[3376,3377],[1304,1305],[8116,8117],[7012,7013],[2327,2328],[2792,2793],[4741,4742],[2667,2668],[4785,4786],[4130,4131],[41,42],[6260,6261],[3112,3113],[2695,2696],[901,902],[7046,7047],[2624,2625],[4619,4620],[267,268],[7233,7234],[7614,7615],[3724,3725],[5967,5968],[4052,4053],[6328,6329],[4284,4285],[6123,6124],[7752,7753],[1312,1313],[3854,3855],[4783,4784],[897,898],[1085,1086],[3767,3768],[686,687],[853,854],[2128,2129],[2789,2790],[6622,6623],[6210,6211],[6213,6214],[4740,4741],[3308,3309],[4426,4427],[5181,5182],[8302,8303],[2054,2055],[3050,3051],[5129,5130],[6680,6681],[6778,6779],[7135,7136],[2071,2072],[5341,5342],[1805,1806],[172,173],[4851,4852],[1846,1847],[4531,4532],[7962,7963],[2864,2865],[3528,3529],[1879,1880],[7561,7562],[3722,3723],[4299,4300],[8081,8082],[3680,3681],[1438,1439],[1798,1799],[4868,4869],[1775,1776],[2256,2257],[7681,7682],[2710,2711],[1235,1236],[258,259],[1068,1069],[7666,7667],[2354,2355],[3941,3942],[2785,2786],[1841,1842],[5608,5609],[5801,5802],[4367,4368],[4252,4253],[1770,1771],[5118,5119],[5645,5646],[1342,1343],[75,76],[4560,4561],[5908,5909],[985,986],[2980,2981],[2722,2723],[5466,5467],[6742,6743],[4444,4445],[166,167],[4389,4390],[2204,2205],[3163,3164],[1113,1114],[5286,5287],[14,15],[6343,6344],[779,780],[7004,7005],[5673,5674],[2717,2718],[134,135],[446,447],[697,698],[1763,1764],[571,572],[7085,7086],[4825,4826],[7461,7462],[1734,1735],[5914,5915],[3426,3427],[6098,6099],[6731,6732],[1350,1351],[7595,7596],[2410,2411],[6917,6918],[7181,7182],[3340,3341],[4935,4936],[6076,6077],[2557,2558],[5684,5685],[1239,1240],[6197,6198],[7140,7141],[6946,6947],[2427,2428],[4421,4422],[8034,8035],[7368,7369],[192,193],[7859,7860],[5369,5370],[6895,6896],[1947,1948],[5711,5712],[7481,7482],[6106,6107],[6230,6231],[5451,5452],[42,43],[2030,2031],[3831,3832],[6222,6223],[4781,4782],[7759,7760],[2343,2344],[2653,2654],[1541,1542],[5587,5588],[6699,6700],[8335,8336],[893,894],[722,723],[6357,6358],[4934,4935],[5031,5032],[6803,6804],[5666,5667],[6938,6939],[5782,5783],[6099,6100],[1283,1284],[1801,1802],[4158,4159],[3695,3696],[80,81],[4629,4630],[5070,5071],[269,270],[5277,5278],[3895,3896],[5239,5240],[6070,6071],[8108,8109],[2619,2620],[5089,5090],[7623,7624],[8251,8252],[2034,2035],[4258,4259],[2735,2736],[6389,6390],[616,617],[5919,5920],[932,933],[1535,1536],[3997,3998],[2272,2273],[3620,3621],[6923,6924],[5215,5216],[6474,6475],[2317,2318],[462,463],[2811,2812],[4430,4431],[829,830],[2051,2052],[583,584],[7503,7504],[6378,6379],[2481,2482],[5294,5295],[419,420],[491,492],[5533,5534],[2435,2436],[311,312],[7251,7252],[1920,1921],[2656,2657],[435,436],[1393,1394],[2737,2738],[5825,5826],[2684,2685],[3525,3526],[5960,5961],[6705,6706],[7082,7083],[3411,3412],[6592,6593],[7820,7821],[6490,6491],[7875,7876],[4325,4326],[4631,4632],[1910,1911],[6145,6146],[5646,5647],[905,906],[4364,4365],[3283,3284],[7948,7949],[2280,2281],[1322,1323],[3873,3874],[6549,6550],[7743,7744],[577,578],[2197,2198],[3168,3169],[2332,2333],[7331,7332],[2556,2557],[1268,1269],[696,697],[2014,2015],[492,493],[7455,7456],[3310,3311],[5024,5025],[3484,3485],[5091,5092],[6733,6734],[6294,6295],[1812,1813],[3698,3699],[1596,1597],[7543,7544],[4230,4231],[1795,1796],[6363,6364],[3101,3102],[6842,6843],[3556,3557],[2575,2576],[557,558],[4199,4200],[7641,7642],[3904,3905],[3172,3173],[2243,2244],[4902,4903],[7980,7981],[2038,2039],[5163,5164],[1093,1094],[8021,8022],[1083,1084],[4981,4982],[3953,3954],[5262,5263],[7915,7916],[427,428],[5789,5790],[7041,7042],[6910,6911],[3700,3701],[3684,3685],[5135,5136],[5775,5776],[6582,6583],[7734,7735],[1196,1197],[5941,5942],[7377,7378],[4361,4362],[7631,7632],[5651,5652],[3614,3615],[4097,4098],[4708,4709],[860,861],[1711,1712],[572,573],[3604,3605],[6285,6286],[3307,3308],[1251,1252],[1816,1817],[3608,3609],[1772,1773],[5970,5971],[3731,3732],[6026,6027],[1282,1283],[3356,3357],[1376,1377],[6436,6437],[5093,5094],[3819,3820],[298,299],[3793,3794],[5899,5900],[7805,7806],[1496,1497],[3627,3628],[1939,1940],[4195,4196],[3568,3569],[2560,2561],[4214,4215],[7478,7479],[3079,3080],[3323,3324],[6809,6810],[1973,1974],[4416,4417],[3574,3575],[1167,1168],[170,171],[1359,1360],[6233,6234],[3992,3993],[6873,6874],[7060,7061],[547,548],[6406,6407],[5581,5582],[3234,3235],[2931,2932],[2154,2155],[3987,3988],[3004,3005],[4676,4677],[3027,3028],[3726,3727],[7217,7218],[5717,5718],[1219,1220],[6716,6717],[5230,5231],[6361,6362],[4356,4357],[1946,1947],[2774,2775],[126,127],[8323,8324],[753,754],[4215,4216],[478,479],[4993,4994],[7628,7629],[3676,3677],[7061,7062],[5078,5079],[7707,7708],[7309,7310],[4612,4613],[2962,2963],[3034,3035],[4812,4813],[1257,1258],[7709,7710],[395,396],[4917,4918],[3923,3924],[3914,3915],[5827,5828],[1854,1855],[7837,7838],[2390,2391],[1648,1649],[3912,3913],[7525,7526],[1395,1396],[7088,7089],[6138,6139],[244,245],[676,677],[7939,7940],[5938,5939],[5013,5014],[6182,6183],[2583,2584],[2172,2173],[1852,1853],[2944,2945],[4650,4651],[6235,6236],[4546,4547],[529,530],[543,544],[3771,3772],[2506,2507],[5130,5131],[8113,8114],[3305,3306],[2265,2266],[4707,4708],[1719,1720],[423,424],[5050,5051],[6773,6774],[7566,7567],[4342,4343],[4213,4214],[428,429],[3774,3775],[6064,6065],[3753,3754],[2859,2860],[7404,7405],[2661,2662],[6678,6679],[7682,7683],[2739,2740],[770,771],[3047,3048],[5094,5095],[4076,4077],[6101,6102],[6629,6630],[1951,1952],[3485,3486],[865,866],[2244,2245],[416,417],[3243,3244],[6779,6780],[1655,1656],[4998,4999],[7084,7085],[6427,6428],[6365,6366],[4227,4228],[2521,2522],[7185,7186],[606,607],[1857,1858],[5164,5165],[6990,6991],[2721,2722],[3662,3663],[3435,3436],[5256,5257],[5254,5255],[3810,3811],[5786,5787],[3632,3633],[2913,2914],[3190,3191],[3032,3033],[2165,2166],[487,488],[641,642],[3353,3354],[2639,2640],[3192,3193],[5705,5706],[275,276],[5304,5305],[1837,1838],[6324,6325],[4226,4227],[1673,1674],[3084,3085],[67,68],[6908,6909],[7202,7203],[2804,2805],[1397,1398],[4393,4394],[8026,8027],[4794,4795],[3235,3236],[2119,2120],[7611,7612],[584,585],[734,735],[2608,2609],[4112,4113],[1060,1061],[714,715],[7513,7514],[5713,5714],[6513,6514],[3896,3897],[4593,4594],[2234,2235],[6902,6903],[3481,3482],[6746,6747],[7523,7524],[4422,4423],[7460,7461],[523,524],[888,889],[4218,4219],[5906,5907],[7268,7269],[7166,7167],[5216,5217],[227,228],[3399,3400],[2536,2537],[7995,7996],[138,139],[1385,1386],[7179,7180],[394,395],[5449,5450],[6682,6683],[456,457],[3919,3920],[5122,5123],[3911,3912],[241,242],[1889,1890],[4411,4412],[7590,7591],[8093,8094],[4202,4203],[1869,1870],[5989,5990],[1822,1823],[4162,4163],[7236,7237],[6703,6704],[656,657],[768,769],[6396,6397],[4889,4890],[3811,3812],[2793,2794],[2889,2890],[5267,5268],[763,764],[2678,2679],[1473,1474],[8148,8149],[5931,5932],[7270,7271],[5116,5117],[2059,2060],[4520,4521],[2882,2883],[7070,7071],[3626,3627],[7881,7882],[2979,2980],[2336,2337],[2807,2808],[5067,5068],[4693,4694],[6420,6421],[5208,5209],[6840,6841],[3576,3577],[5680,5681],[5886,5887],[7362,7363],[4679,4680],[5207,5208],[6700,6701],[359,360],[3747,3748],[593,594],[7895,7896],[700,701],[4645,4646],[7742,7743],[8127,8128],[8106,8107],[6193,6194],[1464,1465],[7949,7950],[2679,2680],[4780,4781],[4105,4106],[1224,1225],[1082,1083],[4396,4397],[7136,7137],[173,174],[2214,2215],[127,128],[5074,5075],[2599,2600],[6848,6849],[8174,8175],[4172,4173],[4655,4656],[6775,6776],[4124,4125],[2000,2001],[460,461],[1091,1092],[1202,1203],[8309,8310],[3606,3607],[5224,5225],[6789,6790],[6595,6596],[6316,6317],[4387,4388],[2340,2341],[7207,7208],[4016,4017],[2513,2514],[5879,5880],[314,315],[1106,1107],[4552,4553],[8128,8129],[7715,7716],[5689,5690],[3593,3594],[8027,8028],[284,285],[4913,4914],[8193,8194],[174,175],[7405,7406],[5828,5829],[3293,3294],[7291,7292],[5433,5434],[3965,3966],[6185,6186],[6660,6661],[595,596],[7489,7490],[1364,1365],[7718,7719],[7399,7400],[7139,7140],[1739,1740],[8316,8317],[4636,4637],[4541,4542],[3871,3872],[4599,4600],[8082,8083],[1556,1557],[2195,2196],[5461,5462],[3876,3877],[517,518],[344,345],[6023,6024],[7400,7401],[1665,1666],[90,91],[3455,3456],[5854,5855],[739,740],[2893,2894],[8078,8079],[8142,8143],[7009,7010],[4164,4165],[1866,1867],[4694,4695],[4324,4325],[6797,6798],[4156,4157],[6493,6494],[7466,7467],[1112,1113],[1700,1701],[6823,6824],[7111,7112],[3143,3144],[5022,5023],[5411,5412],[3276,3277],[6103,6104],[4003,4004],[3366,3367],[7710,7711],[5049,5050],[4107,4108],[5987,5988],[3672,3673],[3870,3871],[5010,5011],[4782,4783],[2126,2127],[3670,3671],[7983,7984],[6669,6670],[2890,2891],[2019,2020],[4961,4962],[5596,5597],[2604,2605],[8170,8171],[3012,3013],[955,956],[2206,2207],[2610,2611],[6199,6200],[8303,8304],[7676,7677],[1100,1101],[4494,4495],[5435,5436],[4747,4748],[218,219],[6704,6705],[8283,8284],[8086,8087],[2650,2651],[3843,3844],[3821,3822],[6996,6997],[5296,5297],[3552,3553],[1314,1315],[4200,4201],[1042,1043],[263,264],[5005,5006],[5086,5087],[981,982],[7625,7626],[2782,2783],[6821,6822],[4972,4973],[4600,4601],[2184,2185],[2348,2349],[3059,3060],[1799,1800],[5034,5035],[2783,2784],[3337,3338],[1943,1944],[1537,1538],[5381,5382],[3290,3291],[1928,1929],[2458,2459],[6227,6228],[4540,4541],[334,335],[4755,4756],[1381,1382],[6368,6369],[1263,1264],[3301,3302],[7978,7979],[5634,5635],[1899,1900],[1955,1956],[2432,2433],[6494,6495],[1557,1558],[6505,6506],[1466,1467],[6744,6745],[3734,3735],[2113,2114],[4298,4299],[4591,4592],[2848,2849],[528,529],[8328,8329],[4273,4274],[8317,8318],[1503,1504],[5167,5168],[6621,6622],[1661,1662],[5218,5219],[952,953],[6115,6116],[4477,4478],[5741,5742],[5597,5598],[61,62],[4083,4084],[6727,6728],[692,693],[6460,6461],[2736,2737],[3611,3612],[1641,1642],[2428,2429],[455,456],[3198,3199],[4187,4188],[781,782],[5624,5625],[7537,7538],[1344,1345],[5894,5895],[6631,6632],[7332,7333],[2322,2323],[459,460],[2928,2929],[7132,7133],[3152,3153],[1887,1888],[6994,6995],[5950,5951],[3236,3237],[6214,6215],[1838,1839],[5497,5498],[5320,5321],[3982,3983],[3816,3817],[8215,8216],[6188,6189],[211,212],[6090,6091],[6112,6113],[1377,1378],[4573,4574],[4021,4022],[1423,1424],[4923,4924],[1907,1908],[2421,2422],[444,445],[3557,3558],[3961,3962],[3043,3044],[5295,5296],[1070,1071],[5149,5150],[3267,3268],[7361,7362],[1148,1149],[7965,7966],[6968,6969],[4849,4850],[242,243],[6862,6863],[228,229],[1389,1390],[4892,4893],[465,466],[6382,6383],[2125,2126],[682,683],[4863,4864],[2069,2070],[6360,6361],[2468,2469],[3164,3165],[1441,1442],[7021,7022],[647,648],[7312,7313],[6050,6051],[293,294],[5119,5120],[207,208],[3759,3760],[6246,6247],[2596,2597],[3649,3650],[1527,1528],[92,93],[1606,1607],[4090,4091],[313,314],[3341,3342],[3259,3260],[2203,2204],[7954,7955],[7509,7510],[2342,2343],[6212,6213],[3760,3761],[6876,6877],[5602,5603],[2139,2140],[5982,5983],[1833,1834],[4417,4418],[4084,4085],[3036,3037],[6107,6108],[1387,1388],[6280,6281],[7052,7053],[6881,6882],[1331,1332],[8165,8166],[6528,6529],[5387,5388],[5364,5365],[3630,3631],[7129,7130],[1575,1576],[7894,7895],[7726,7727],[7814,7815],[3311,3312],[6059,6060],[7428,7429],[762,763],[2561,2562],[3922,3923],[5204,5205],[635,636],[6969,6970],[2865,2866],[8096,8097],[8324,8325],[2017,2018],[7367,7368],[5975,5976],[4275,4276],[8134,8135],[2086,2087],[8264,8265],[2117,2118],[5693,5694],[4924,4925],[4936,4937],[3416,3417],[3664,3665],[2692,2693],[7815,7816],[2606,2607],[2833,2834],[6330,6331],[6645,6646],[8176,8177],[1198,1199],[479,480],[7239,7240],[415,416],[3100,3101],[3867,3868],[2151,2152],[8125,8126],[5472,5473],[3144,3145],[5271,5272],[4759,4760],[6891,6892],[4996,4997],[4975,4976],[405,406],[1459,1460],[3838,3839],[1465,1466],[4568,4569],[3345,3346],[6753,6754],[6633,6634],[8019,8020],[7593,7594],[6179,6180],[6827,6828],[3942,3943],[4751,4752],[7892,7893],[2906,2907],[5110,5111],[5636,5637],[5777,5778],[1487,1488],[4813,4814],[5995,5996],[1018,1019],[1004,1005],[440,441],[6670,6671],[5244,5245],[3186,3187],[980,981],[5000,5001],[4410,4411],[5690,5691],[3314,3315],[3654,3655],[3602,3603],[7470,7471],[1927,1928],[3113,3114],[3194,3195],[7871,7872],[1705,1706],[1210,1211],[1404,1405],[6851,6852],[2687,2688],[2738,2739],[7256,7257],[56,57],[7524,7525],[4109,4110],[6159,6160],[6177,6178],[3196,3197],[8290,8291],[2534,2535],[7952,7953],[2162,2163],[6058,6059],[1488,1489],[5696,5697],[4405,4406],[5100,5101],[326,327],[5062,5063],[7882,7883],[2665,2666],[3974,3975],[7799,7800],[2420,2421],[7295,7296],[1406,1407],[3040,3041],[5768,5769],[6606,6607],[2554,2555],[3893,3894],[4824,4825],[5132,5133],[2724,2725],[101,102],[7878,7879],[4225,4226],[10,11],[1583,1584],[3169,3170],[7907,7908],[849,850],[1621,1622],[5965,5966],[6262,6263],[1353,1354],[916,917],[7877,7878],[1652,1653],[7924,7925],[4018,4019],[6804,6805],[3910,3911],[2505,2506],[3864,3865],[4847,4848],[5822,5823],[4604,4605],[3408,3409],[4154,4155],[3492,3493],[7382,7383],[2501,2502],[1694,1695],[612,613],[6568,6569],[5532,5533],[7178,7179],[1605,1606],[1909,1910],[6976,6977],[5815,5816],[8043,8044],[4948,4949],[5189,5190],[2389,2390],[1867,1868],[1492,1493],[3456,3457],[4137,4138],[3512,3513],[1249,1250],[7783,7784],[840,841],[7457,7458],[5635,5636],[6598,6599],[8267,8268],[6265,6266],[8050,8051],[3261,3262],[909,910],[796,797],[1067,1068],[2829,2830],[4271,4272],[1183,1184],[4749,4750],[1777,1778],[3660,3661],[6228,6229],[1373,1374],[7464,7465],[3544,3545],[2891,2892],[6119,6120],[5612,5613],[3313,3314],[5895,5896],[7968,7969],[324,325],[6924,6925],[2699,2700],[2847,2848],[6636,6637],[6283,6284],[8029,8030],[5492,5493],[7735,7736],[8306,8307],[4268,4269]],
+ targetFriend = 4519
+ ```
+- **Output:** 0
+
+**Example 5:**
+
+- **Input:**
+ ```
+ times = [[4,5],[12,13],[5,6],[1,2],[8,9],[9,10],[6,7],[3,4],[7,8],[13,14],[15,16],[14,15],[10,11],[11,12],[2,3],[16,17]],
+ targetFriend = 15
+ ```
+- **Output:** 0
+
+**Example 6:**
+
+- **Input:**
+ ```
+ times = [[48210,85285],[46524,48493],[69982,84568],[68053,76419],[93591,97693],[10826,92490],[19366,83205],[90379,92055],[83953,88146],[6466,6795],[96743,98347],[29871,31619],[22949,83080],[58306,91003],[63487,81278],[33256,49208],[60964,98563],[17086,25143],[53332,97203],[18856,26826],[12517,27880],[49357,67393],[96514,97453],[43732,82311],[51936,65126],[17825,77373],[7190,77681],[38818,87041],[60711,84473],[3754,66051],[99983,99984],[5814,55830],[3833,39153],[71632,91461],[99252,99712],[15002,35534],[65097,77671],[6286,87838],[7891,8509],[40505,43365],[54073,74776],[89699,91243],[95158,97485],[31149,42770],[63519,87434],[61935,78221],[2380,28991],[79881,81382],[40190,94494],[41862,73438],[74482,76848],[63016,79828],[12951,13848],[92131,95646],[36030,88066],[86638,92405],[97928,99197],[97679,99457],[69204,95987],[95934,99864],[71117,95167],[1698,50492],[10030,82025],[40893,59120],[13482,73281],[12018,66449],[63542,73061],[98886,99681],[54333,57707],[30903,62762],[10177,27610],[32048,80510],[10389,89810],[62864,81389],[81477,82859],[84637,90371],[15071,27615],[42528,93941],[9344,92289],[54720,88699],[92290,95965],[20387,98404],[17024,86124],[47573,66022],[85502,86949],[52486,61448],[82220,87356],[32475,32637],[58452,59429],[30109,56426],[93029,93594],[51255,59828],[60958,88823],[63538,83957],[276,72672],[28666,54076],[29968,86864],[97360,98067],[1117,34572],[14894,42110],[92657,94778],[5953,72908],[46901,59410],[51178,69717],[1653,79730],[16366,68014],[77911,82792],[81177,82314],[71569,72574],[61956,78298],[75833,88337],[35502,65000],[24047,73173],[9286,24350],[68679,76990],[42891,85895],[65128,83149],[95757,99264],[53956,90056],[75742,77288],[45770,64643],[77996,81439],[21454,59154],[47681,75505],[94171,96038],[36963,96891],[86335,99925],[28351,84927],[90370,91656],[32595,81002],[38745,85563],[82472,99551],[99587,99822],[91156,98592],[8267,86739],[10890,86010],[10411,49492],[10720,74250],[15126,40651],[36192,57999],[69060,98034],[41147,85448],[56870,88507],[58418,77267],[95891,99661],[11388,73213],[40708,50499],[69455,80483],[89904,93067],[5648,27531],[91406,92397],[74965,78521],[48515,87121],[32058,60312],[56742,96316],[44835,54573],[94510,95744],[3323,32938],[6014,53489],[87328,88036],[81596,95508],[8626,99463],[64969,91642],[97634,98280],[84575,99213],[59643,92364],[27550,49499],[28523,30075],[18272,62477],[32870,34885],[93990,99711],[79664,82479],[79885,81238],[43488,96059],[62227,69236],[86015,99363],[52258,87524],[84395,94009],[260,61980],[94639,96103],[81610,93673],[8375,73739],[92648,98303],[43573,80190],[97479,99688],[12786,62429],[85068,99354],[65625,73789],[39586,80043],[60278,99931],[69644,77411],[87222,92178],[52067,89116],[32663,66802],[32590,43620],[11531,27051],[164,63564],[98119,99886],[60542,86235],[65210,80094],[82852,97232],[66702,71196],[39672,50854],[97270,98306],[4351,52670],[93525,95535],[15402,50714],[42024,77025],[46532,59101],[8418,96138],[77412,91249],[4775,11015],[22526,76789],[23669,73864],[64077,92284],[86509,99576],[23456,41142],[45007,65172],[4577,9088],[96650,98345],[35929,70561],[47528,72447],[8645,66485],[18387,31301],[15698,31613],[8899,74243],[94207,98592],[67673,81051],[48106,69025],[39626,94628],[9983,72729],[67724,68340],[29765,59531],[89752,90556],[21787,54950],[5324,34185],[64786,87911],[97565,97961],[81401,85960],[81947,91400],[55608,85874],[30719,95522],[39371,84566],[92294,97705],[96165,99620],[57736,74149],[13000,81640],[52622,95627],[48409,59629],[27390,51248],[63747,69043],[65597,81709],[10095,19419],[15234,47659],[34309,44757],[33863,48810],[91084,92091],[67817,77971],[72221,82727],[16295,25043],[64353,98511],[93077,96137],[16133,30596],[75551,89906],[60231,96814],[41132,88940],[84501,90563],[47674,68829],[55742,92010],[79179,90956],[55505,60411],[7914,76864],[98625,98825],[145,92328],[90200,95685],[70600,82915],[43649,91009],[78906,93751],[97838,98151],[83318,89717],[98298,99752],[73204,77019],[7553,30523],[79612,98738],[72655,80248],[68949,81988],[49684,66571],[40856,45815],[83263,84874],[66452,98833],[9840,86578],[68947,82875],[45036,74480],[8597,45642],[16608,65906],[62817,75808],[45101,93029],[69711,89393],[30250,33181],[73993,88229],[68561,97074],[10416,28408],[18613,91871],[43356,97486],[16478,80062],[97635,98588],[62841,65681],[39104,94753],[89839,96542],[90255,92840],[34444,59080],[36182,69681],[84572,91103],[29941,79497],[36183,68570],[73978,99514],[64217,79142],[98422,99473],[70793,97469],[93645,95936],[8226,18858],[28505,90716],[51201,83463],[46106,55693],[20409,87755],[62465,69983],[42227,91273],[69358,89552],[87758,94452],[50585,61421],[9715,11012],[32184,56961],[80236,85023],[49359,78848],[33379,55761],[64301,68225],[58143,59732],[57764,61277],[13392,20123],[35847,72600],[6320,17506],[37938,58714],[6931,55733],[42995,54295],[26446,55508],[60320,95890],[51491,98384],[53667,94853],[64411,73445],[35472,98590],[90014,95044],[81137,96203],[20188,54722],[97982,99708],[53844,62015],[29479,61845],[55780,64875],[7649,62885],[40659,72525],[37547,91079],[50357,81305],[17270,68219],[88440,97576],[55488,74175],[48510,98601],[64930,73430],[72043,88705],[35274,79794],[39563,48976],[14685,61638],[63394,87152],[41116,82629],[71637,79436],[67602,91570],[20474,28582],[31092,33856],[68892,99866],[46289,92097],[52586,73310],[37503,72921],[41804,79456],[59437,88679],[77483,78221],[60103,97591],[36679,95310],[40213,81817],[65240,89639],[79269,83549],[55601,82016],[21360,59862],[24548,80994],[16246,68866],[56580,89848],[39130,58524],[50841,64263],[39907,87578],[40662,45741],[67283,92731],[74322,81972],[36835,63122],[12750,76952],[28508,68179],[5664,8240],[46241,65294],[16314,80961],[69337,85186],[95253,98281],[63798,74794],[29283,64252],[41219,59705],[11976,98446],[13495,77758],[66383,97354],[92064,95491],[44046,77018],[45792,68508],[13863,65851],[4707,63866],[18769,84274],[54407,56300],[64538,98723],[97459,98943],[43487,77679],[80462,92336],[10716,37297],[78005,88552],[52181,53694],[65038,95780],[22464,28390],[61157,98227],[30002,70151],[31466,38774],[95516,98857],[33250,86754],[13818,98814],[13124,98697],[80682,95382],[1526,40615],[8427,57750],[45034,46575],[63384,64377],[75878,95619],[9667,82706],[99597,99937],[96449,97467],[2412,48396],[13833,68490],[27165,59186],[11604,13092],[81303,84643],[3496,11933],[10654,19830],[32195,75070],[10535,52659],[99987,100000],[16324,18264],[88230,93898],[55245,80709],[82361,91053],[40132,74643],[74356,96249],[91360,93421],[3868,47732],[23225,78524],[62860,82767],[72079,99180],[52137,94053],[23101,38335],[53124,78262],[80546,91353],[19556,25035],[91259,94000],[72254,93370],[94506,98622],[4074,29807],[99544,99645],[98893,99355],[73965,95892],[5109,20976],[47369,95079],[97951,98659],[42884,78641],[53823,95984],[77230,98797],[98477,98821],[4389,81523],[29068,49881],[55998,75245],[96770,99322],[3369,97714],[14218,75162],[45574,53838],[84577,98854],[89165,97666],[13854,21255],[33104,92578],[45552,47366],[21600,46408],[95147,99135],[13860,50758],[36418,41403],[25938,49904],[5608,46172],[20444,44686],[46648,51413],[17017,71283],[66708,91855],[59259,67795],[41291,88456],[96999,97786],[29214,56233],[92649,97263],[71254,89927],[89712,93161],[23421,57702],[93778,95583],[73130,88303],[5653,62472],[8087,79345],[70801,97857],[54849,83841],[74007,97419],[18434,56061],[22347,46597],[59916,91645],[98786,99702],[22513,54495],[53265,60995],[90292,92288],[92706,94755],[94596,97074],[54772,82630],[8980,49105],[50212,91179],[32509,46489],[83034,85872],[45915,81854],[63047,68760],[91668,93759],[36426,86423],[37004,91364],[69121,83246],[16247,99532],[91849,96199],[61292,97317],[23915,80238],[15928,34240],[50592,95344],[89105,97813],[10472,72695],[12574,16102],[47749,94090],[50653,61501],[30353,59078],[8604,88636],[24336,63652],[51674,99847],[15461,80223],[47568,54776],[444,44785],[43382,78665],[76892,90366],[99415,99945],[45607,55499],[53215,74525],[4523,51689],[17756,47861],[86,46919],[11334,45549],[95531,97527],[27123,62928],[90997,92660],[79666,84069],[68435,88215],[85779,95119],[94753,99796],[82952,84089],[72104,97291],[90986,97682],[82577,86709],[2478,70898],[28262,63326],[26326,39170],[6918,22915],[73633,91502],[47576,81375],[442,99140],[74713,99498],[67403,76105],[35034,54968],[29140,49864],[46352,87193],[20775,56580],[64762,73615],[69071,90592],[46624,56506],[20142,62220],[78789,79311],[89301,96406],[38055,72285],[7096,52485],[49788,69958],[85885,92793],[62559,65447],[51238,56336],[97584,99242],[93481,99790],[64610,87315],[70413,92288],[51964,81232],[70330,71367],[22611,89012],[7341,40615],[81726,89963],[59441,95279],[90043,99457],[17421,41402],[47304,75365],[38154,98965],[38104,96314],[88567,93845],[44673,74898],[16833,99027],[9879,27828],[43140,95822],[65590,69653],[68840,86138],[46713,99588],[16056,99894],[20004,62106],[75974,89820],[40209,81898],[90144,96902],[25708,66590],[31366,95083],[50469,68530],[48165,95137],[71326,73156],[30609,86246],[17685,50103],[89813,99401],[58923,83851],[1430,33408],[46147,85073],[67134,98851],[88503,98020],[52442,85340],[36713,68269],[50787,60769],[5249,21010],[22455,45057],[37326,83138],[82727,96928],[76025,93980],[26763,58264],[76652,88913],[42447,75594],[34132,90890],[52147,86057],[21043,90152],[86389,94972],[14690,93128],[93462,95508],[39363,47331],[63078,74492],[21033,77827],[75083,79875],[94685,97544],[61995,78536],[55179,97701],[20654,60942],[39083,61408],[46038,55154],[66114,69702],[15571,80475],[98552,99413],[47921,91593],[8973,46745],[12256,51041],[34294,88880],[20184,69205],[12081,39221],[6828,28556],[18609,36512],[29401,73251],[83803,91740],[54406,75100],[14199,16482],[20078,59313],[63870,97642],[56058,95510],[51064,59469],[52888,60562],[11973,50238],[78911,87630],[32726,46593],[51751,98892],[58942,72591],[65536,72614],[9700,66647],[25336,43094],[32243,55015],[68219,73776],[17494,73567],[73898,79023],[8850,73509],[13853,41565],[65504,67474],[19343,90371],[51633,65672],[24427,29013],[44362,44776],[55850,67270],[76523,90896],[40325,73331],[39769,62908],[87180,88158],[74875,81702],[51591,59957],[86992,88784],[92033,95878],[72178,91046],[96004,98754],[49578,90639],[18122,87877],[36759,78342],[39705,81703],[82712,83227],[87771,96051],[2668,97081],[39317,39711],[88235,97497],[85765,90283],[33312,54495],[72784,92690],[64680,99532],[47954,84324],[51760,66820],[97296,98087],[59497,88038],[47798,91303],[30453,79324],[89672,93174],[63912,82326],[67765,86319],[79027,98815],[16774,57231],[86136,89235],[96028,96532],[79099,96188],[54756,62665],[66001,70416],[57603,59502],[27212,84318],[30423,95980],[63280,90768],[40316,75899],[51808,99722],[10375,62260],[21499,76088],[93863,98002],[73878,99992],[89833,99220],[37149,66043],[96359,97622],[17401,89277],[10251,87933],[53090,77748],[23693,80401],[84254,89281],[81182,94585],[16043,62025],[33760,76918],[47715,53333],[20471,91148],[29420,80649],[38255,60090],[96122,96366],[73788,93193],[90800,94234],[12304,96880],[21103,21437],[12459,56164],[64811,65433],[90608,98802],[57376,94033],[21953,86887],[33833,66774],[62250,78163],[72959,90180],[13079,59745],[85737,89575],[25761,51323],[95351,98232],[90474,94952],[96153,96702],[91620,94572],[36724,87300],[76411,93477],[20600,65200],[28352,87128],[47150,52628],[73497,96617],[41614,76347],[57303,95959],[34767,97928],[69080,82501],[25962,64415],[8053,63071],[84793,91080],[46479,84710],[4826,35140],[23715,62792],[15948,55459],[59231,65504],[69300,86235],[27509,38385],[55025,82974],[64175,71114],[24981,80695],[8377,27941],[1877,20509],[68221,88176],[84512,88861],[16644,57629],[53543,94932],[66115,78781],[74511,86229],[22436,23290],[79123,84938],[44410,58132],[27696,92888],[48944,80038],[21442,89383],[11760,20347],[46181,57330],[9015,64308],[65669,93015],[56875,86158],[55035,72697],[1095,74501],[72377,94332],[90368,98870],[61598,70678],[94203,96801],[32178,75618],[43622,58049],[44782,63971],[40552,82141],[48835,56479],[60327,93264],[95410,96027],[42209,90843],[85984,90639],[17773,43718],[4394,16119],[99313,99693],[45627,71870],[14012,69931],[66568,66850],[24500,57008],[31046,60636],[50649,67242],[92234,98383],[64595,90395],[30379,79612],[19905,74066],[91304,92988],[88583,97151],[83979,84951],[31373,38657],[55058,88452],[45760,60591],[27577,33741],[82681,97188],[84244,95178],[56021,87427],[84219,87115],[65649,98363],[9555,38518],[89579,93588],[32944,59650],[83138,95140],[6773,94431],[74441,94855],[23685,81170],[44404,47462],[57513,93140],[3136,63111],[6799,52393],[55438,71626],[50172,85969],[85964,97342],[13957,53504],[74466,93491],[64673,89435],[78675,96450],[60735,94338],[70511,92808],[82256,99054],[25832,84678],[59601,75689],[31991,72096],[69353,97715],[39831,59935],[52353,66839],[11321,57695],[82585,96918],[70130,70685],[17131,72367],[57561,84885],[11888,43932],[41903,59762],[31661,98755],[31159,36016],[58683,81174],[45965,48869],[21556,42388],[15077,19012],[78776,79316],[65708,86694],[57890,95924],[89510,90942],[22563,78498],[74268,81879],[94709,96037],[31266,58471],[9998,27036],[17363,95848],[92599,94747],[21054,45623],[41832,58147],[7351,53982],[33775,83302],[64040,77914],[69346,86423],[13993,51858],[45425,49714],[96776,97521],[7207,9407],[6274,30393],[93241,94067],[73071,75561],[3172,80950],[32393,63232],[28447,64011],[8451,22056],[41675,47885],[45674,78160],[12684,81275],[81029,93555],[97449,99287],[53286,67982],[66246,76885],[92792,95511],[35116,68333],[49662,67355],[47914,81538],[7692,19693],[48718,59947],[75476,98993],[65744,80535],[50717,74879],[68231,84755],[19316,61340],[58648,85183],[70012,85809],[59366,98677],[34359,44405],[55215,97312],[68754,74010],[64590,81100],[50239,62420],[33401,93142],[19997,31337],[65613,81089],[79441,80286],[78512,97851],[58262,98237],[43441,53014],[51803,67020],[61012,95683],[19840,21343],[20699,49473],[77084,85909],[54085,77728],[75711,85695],[28752,91410],[5815,36717],[75672,93819],[33222,66757],[80515,86623],[32765,34467],[24769,66730],[24149,99001],[97903,98304],[77765,85311],[9537,84591],[53643,81083],[24516,26918],[1209,20941],[78081,87762],[55056,98309],[90662,96373],[8497,46084],[13584,74075],[99075,99162],[24176,77147],[49999,73290],[67566,75260],[98053,98796],[85588,92251],[80891,90648],[96159,96996],[46518,48324],[38429,46752],[58959,67635],[66170,75023],[95366,97476],[43480,74600],[14425,34961],[71134,83192],[30921,90420],[46990,49728],[69631,94007],[73047,81116],[21021,73737],[54122,71887],[26268,95891],[87547,95928],[3922,85323],[55846,57723],[91365,96835],[76646,95472],[90664,98872],[4232,27563],[73515,82497],[46108,47248],[70642,97110],[59008,76030],[11429,98945],[65009,79888],[16973,41058],[15775,69914],[23406,59497],[32857,81566],[15819,98590],[33129,59733],[30385,62223],[89623,96011],[2213,44786],[23641,60813],[38723,56484],[4630,62247],[48167,57424],[30564,39740],[20946,80392],[1373,55609],[77221,96184],[88337,93782],[77499,97762],[27241,40024],[1410,66672],[60234,89876],[67342,72100],[33046,63146],[62072,80046],[59448,88054],[66666,77911],[30360,43504],[93562,97777],[59558,91896],[65682,97182],[45077,94510],[28328,37656],[23612,41544],[52116,83035],[77653,98527],[51577,53664],[78817,93831],[73499,98329],[91917,97975],[37262,41910],[76060,82430],[25888,50349],[44413,53700],[2335,56512],[25058,78900],[76132,80500],[57786,74383],[26806,94067],[29729,64294],[31279,38142],[77690,94340],[52619,66733],[20642,88106],[78729,99860],[52699,83419],[38815,70010],[98488,99602],[8756,54289],[39064,62551],[64748,69153],[52340,56558],[24647,55133],[28348,72544],[7798,37728],[19435,76820],[41235,70073],[12746,92502],[67764,81354],[7130,83515],[86323,94274],[53690,57660],[71739,99392],[90027,92872],[59166,62272],[77928,81889],[50708,59127],[82489,96045],[5964,37108],[69311,77251],[58136,79621],[4942,53215],[37525,41146],[86981,94481],[24290,24586],[59396,95184],[83439,88202],[10622,37960],[10310,12001],[69688,93967],[29591,72300],[32638,67166],[92551,98523],[39018,82200],[34121,73415],[82350,91535],[54473,79619],[36201,56141],[99824,99930],[71925,80259],[42003,80785],[75398,79911],[80789,84951],[53853,80767],[74059,90244],[25198,96976],[983,35996],[25833,73780],[19117,38696],[62908,81461],[32994,59095],[63609,96939],[80973,97448],[96404,97284],[38998,86918],[6518,59383],[35999,57992],[31080,68100],[93408,93774],[95847,97641],[62398,79385],[62184,88069],[35990,79179],[2187,85829],[57610,76445],[44052,48558],[4966,69554],[28075,73463],[15109,17410],[92554,99444],[54630,92713],[62867,88053],[23431,94786],[50602,97613],[6614,33560],[71894,83706],[47826,97056],[76387,99138],[17391,80996],[28700,87970],[73582,90842],[19870,68107],[15281,65013],[85480,98035],[77160,85652],[59229,91174],[33764,94318],[26460,54240],[78990,85748],[41861,48865],[2090,66404],[30802,53668],[96774,99002],[71071,80520],[74794,95150],[15513,58844],[88391,89662],[90128,91654],[14360,55843],[1731,81288],[68774,69956],[73257,77067],[91109,97655],[52192,71942],[93530,99470],[59036,96520],[49709,84688],[52771,96747],[67433,68665],[66362,88355],[70964,92790],[87409,90978],[59995,66620],[31890,58379],[33959,39191],[92341,98390],[10333,73279],[47001,47988],[5406,23122],[70501,91323],[62580,87851],[49511,55630],[47322,89361],[1548,55372],[76587,83039],[820,2294],[42101,91653],[64807,66221],[4609,68672],[22868,33680],[15586,47794],[9067,16754],[16020,30443],[43985,47399],[6819,85595],[98278,98898],[53742,98916],[22566,87830],[47634,57132],[87921,92944],[38710,82027],[49398,83419],[99486,99821],[86589,98397],[3982,31100],[62590,93970],[54783,69110],[93215,98677],[91123,93441],[94648,99439],[87193,95278],[97627,97775],[51692,60180],[34880,90391],[10010,40077],[81696,95027],[12713,99211],[65037,92940],[93138,99689],[43513,93770],[7161,47936],[50794,94290],[67628,99580],[22103,95899],[45984,51204],[11994,26573],[46233,92169],[42977,75263],[72980,84780],[49465,75211],[77175,87997],[89362,92706],[31119,65107],[2223,29769],[11811,63200],[9288,46801],[38884,93423],[32702,58460],[57528,70524],[24646,97936],[6200,56476],[91264,97510],[49281,66239],[7110,57043],[49532,54347],[7405,32817],[92022,93992],[32187,84331],[18596,39229],[11259,20387],[89947,92633],[14801,40571],[27790,56983],[50889,60612],[18085,18779],[7652,25291],[66063,95926],[40431,84904],[42626,58649],[28157,91963],[47738,75537],[17890,27133],[52315,78563],[11211,90143],[26400,29231],[63673,96478],[14046,27213],[83311,88197],[94044,95968],[9951,78794],[9209,66436],[51516,52946],[60015,86468],[62263,77608],[87977,90615],[80416,95897],[20301,28123],[94123,96641],[15132,37292],[32686,37075],[41556,67464],[58373,67030],[59384,98648],[2955,78972],[9688,47361],[97993,98493],[72795,78328],[51586,72193],[10049,52152],[84906,87813],[93790,94411],[17307,20222],[85974,91138],[88117,88305],[8347,84681],[36918,59761],[21836,59305],[93528,93913],[83094,84840],[82956,96337],[17364,94565],[25299,55966],[30286,90053],[79344,97876],[29922,50541],[11929,40946],[29641,90948],[40457,82725],[13978,84379],[61671,94661],[96744,97791],[92936,93174],[37611,49456],[57086,81430],[12321,75375],[53187,61956],[74656,92123],[36021,99320],[45491,49264],[16886,79216],[47531,80755],[59550,78471],[6549,70575],[30482,41647],[53755,92090],[69692,93405],[18625,64164],[6734,72904],[9934,44680],[41992,79046],[83429,91575],[64711,89187],[21605,44358],[67932,69075],[2918,23220],[99670,99706],[80768,98812],[73198,95745],[26162,60989],[51722,92237],[92398,93282],[33191,89532],[52391,94016],[5905,82529],[29338,99525],[67191,77209],[42023,81399],[85474,88135],[40276,82932],[51973,89344],[2023,65620],[36175,51104],[82639,99677],[76546,81319],[36072,86381],[90440,95297],[84433,98278],[53584,72564],[5181,86113],[50410,90416],[26258,38660],[86330,90594],[44375,81513],[60455,96756],[99358,99859],[38307,55687],[10443,13757],[14980,28416],[82324,98125],[34856,54381],[91875,95706],[29531,86084],[26988,29840],[75967,99939],[49654,89516],[63238,94993],[62042,86079],[93406,95957],[17293,24546],[34897,36731],[4996,99868],[8442,72646],[33350,37819],[82847,82852],[45018,75349],[55805,99621],[53435,73616],[93482,99314],[12976,71895],[12592,97815],[75875,77575],[3292,47907],[23144,99173],[45225,64548],[21440,68804],[88997,93286],[37210,48190],[83520,95215],[89501,95592],[30018,97885],[91499,99041],[12129,89363],[7346,29319],[43571,80880],[99919,99922],[99818,99878],[6416,32413],[23390,97256],[39285,48642],[17628,58612],[31758,87831],[82797,84925],[10210,27759],[29121,85291],[2608,11147],[68585,75876],[14135,79274],[74345,74410],[57822,81792],[92670,98418],[66699,71667],[42603,88523],[9142,46802],[47226,70718],[64607,83773],[24698,27245],[1100,17476],[83593,90838],[80679,86027],[78772,83136],[32714,51844],[1996,28548],[63887,75145],[46600,82987],[53663,71028],[64127,86496],[68350,78799],[57937,76935],[65927,79876],[70706,99246],[53421,62721],[30992,45989],[48954,72154],[91969,98277],[30633,83023],[88758,89042],[50285,98381],[99014,99106],[91496,97456],[83725,86605],[69331,86874],[9685,40655],[46891,98521],[74749,77515],[73567,99832],[15632,47014],[9158,41598],[65538,73533],[61799,97360],[56895,79131],[92995,93037],[62886,77620],[37968,86423],[4248,97070],[66521,86308],[39422,44007],[98372,98859],[26746,97115],[95803,97942],[72302,78381],[68164,98752],[20056,82959],[99142,99493],[54748,65632],[66618,79566],[83687,99602],[83483,94195],[8412,50178],[7689,68197],[39957,94477],[27927,46058],[52993,62720],[5212,79788],[23224,62098],[24120,49584],[27686,88050],[85240,93970],[13503,19484],[61831,94464],[59200,91060],[87176,87489],[74510,85737],[87720,98936],[1972,97164],[98638,99977],[40105,47796],[85054,87349],[26184,53949],[1322,36688],[92955,98858],[18884,41975],[52868,61277],[65241,69298],[13521,41795],[48749,74552],[36973,41044],[15564,87837],[3089,23541],[35108,54314],[16474,67390],[64987,65997],[85673,87247],[20086,90062],[89287,91016],[78157,79821],[14689,57497],[57678,72035],[42552,95212],[31629,33909],[1265,91842],[65347,69487],[32061,32537],[78792,84132],[73021,82218],[37796,91375],[24368,56812],[47695,98286],[49338,74597],[82247,93373],[91812,96847],[49667,85662],[78251,91136],[73103,95609],[93999,96360],[46176,78290],[87496,89926],[19140,23688],[65112,92858],[37317,37918],[81845,94357],[38174,75417],[66506,94816],[25032,70593],[12916,61097],[24145,98394],[74449,75895],[68733,71254],[40269,84231],[94151,95221],[14651,50381],[86179,91657],[9364,86149],[79826,99335],[93825,93907],[75856,97371],[89328,91537],[63693,81832],[62338,95700],[41425,99911],[62058,87187],[90058,96519],[97874,99732],[55083,91864],[6589,89853],[17945,46493],[11586,47321],[25662,88171],[89790,98992],[5794,95420],[60163,67736],[47203,95272],[49383,84413],[1459,82001],[32779,83934],[47301,50440],[62803,68431],[28358,99050],[66415,99980],[46349,65556],[23057,46742],[51092,76112],[8526,83734],[6857,69217],[14603,91967],[33188,85019],[81165,96399],[64920,96723],[68601,86767],[28591,86683],[84715,98630],[10031,81993],[31562,90188],[1838,13714],[3016,88096],[50023,63268],[9660,35813],[11706,25423],[98901,99987],[70967,86547],[23894,95060],[48095,69667],[32953,46732],[54065,70805],[33873,85691],[13349,71476],[99288,99525],[40591,41196],[10409,66795],[79820,88188],[19270,92932],[15517,22911],[15568,36484],[5137,48043],[64257,92191],[99410,99517],[94205,99280],[27351,76479],[48237,49358],[10775,87665],[65480,80488],[75184,98898],[33689,58050],[61611,95259],[59821,93808],[28988,63410],[58242,72164],[91303,95227],[44270,55371],[31287,37105],[77243,96887],[11953,95971],[89725,93986],[33947,73144],[95112,99513],[65961,74221],[18207,70526],[90706,99504],[34208,45214],[86078,90748],[14231,32723],[73404,75174],[98941,99962],[55194,59906],[16305,46411],[70306,73299],[48268,62539],[30161,87973],[21030,29662],[94485,98546],[34415,67692],[19240,88057],[91534,99938],[11317,37715],[98707,98793],[9259,11694],[70363,88398],[51055,52840],[34394,95920],[87703,97797],[92160,95864],[4530,47931],[41797,63798],[66631,87182],[29221,62360],[98820,99321],[89562,93059],[46334,72995],[21109,41626],[48066,61831],[52760,82626],[97799,98993],[16881,64680],[97200,97285],[20398,80453],[67381,92303],[57173,74155],[96136,99485],[65425,94307],[19104,66208],[25233,79980],[24636,70151],[17783,35848],[51940,99843],[37851,79463],[45091,62811],[65145,67527],[22419,96264],[27177,83507],[22910,30849],[6061,46619],[6470,22756],[45089,68052],[797,74125],[91970,92972],[87518,88240],[33734,71800],[61763,77103],[42610,91905],[20046,47209],[37939,58811],[59147,80738],[17271,81638],[18883,96757],[84329,90693],[13499,90904],[92700,96097],[10292,51348],[77308,95044],[15644,47280],[86684,87256],[50933,78748],[11635,22982],[69319,78580],[50738,78099],[62438,82317],[43294,84656],[34929,60998],[40895,49133],[46026,88676],[94726,97601],[41637,52881],[65768,99906],[68335,96776],[92850,93804],[10723,35311],[62962,95815],[30490,63740],[61077,86766],[63853,70516],[81209,83289],[70896,89327],[27838,58886],[25547,45102],[54988,80815],[15965,74355],[70445,77235],[93605,99124],[87252,97382],[92130,96911],[76154,76188],[1945,95431],[51444,98273],[62134,92861],[71346,81081],[78381,91256],[56278,97270],[98572,98885],[5876,80230],[45720,61992],[42468,63732],[5025,44041],[73661,88099],[97936,99767],[90827,96438],[77333,86817],[77504,97773],[83625,84587],[37269,85412],[35106,64712],[59282,86993],[36636,98792],[18823,65356],[47481,58682],[82938,99821],[22058,97487],[75407,77049],[53886,74947],[30569,99728],[95927,99216],[40716,64912],[18874,66221],[51747,66661],[35939,85108],[9561,15134],[60257,62534],[59086,83627],[20407,22672],[66758,77316],[35042,36798],[4608,26344],[71555,73944],[35982,76451],[75728,77551],[9695,63939],[66363,91185],[63758,75260],[33230,45572],[80502,98807],[31633,68043],[22991,84225],[65117,90567],[82074,91270],[17353,33817],[15681,22907],[75552,75871],[6205,19329],[90336,92279],[31855,57386],[69630,75624],[39604,93991],[72315,82154],[78006,96967],[85370,97459],[65013,92596],[2256,48062],[76453,87216],[15569,40348],[25539,81587],[97886,99924],[81436,90669],[5620,8934],[9722,10494],[76828,92470],[49552,89398],[53888,96392],[12751,77438],[9475,44264],[93321,95441],[94200,98844],[7434,45512],[85250,87781],[38201,74425],[69850,92511],[18100,68290],[10353,79840],[91374,97446],[16723,66751],[26841,89225],[73344,86121],[28260,89365],[24620,51631],[22637,54502],[21393,46903],[80022,92281],[79316,98183],[82432,92896],[22209,32145],[62940,68637],[29332,38010],[44875,71901],[46286,76637],[31256,60772],[25730,54470],[58023,76874],[98864,99593],[99215,99464],[89680,99545],[93392,95342],[91598,94636],[54266,67044],[30162,46783],[76356,89369],[40356,71003],[96240,96259],[973,57492],[66791,95980],[12307,84950],[39776,71140],[50513,79847],[39777,54853],[28990,88729],[48193,81632],[58646,70863],[60761,98004],[1352,61950],[12198,25046],[9405,58468],[58041,78649],[81201,87798],[5809,94285],[32282,35286],[12652,56034],[9590,38160],[51805,93649],[24249,77790],[45106,71598],[23104,24151],[69338,96947],[19747,30219],[17120,63234],[70318,99787],[41944,61422],[66630,77233],[53235,91814],[6782,10457],[77386,92965],[81470,93823],[60029,87331],[14788,65630],[83886,98953],[26431,36627],[5524,98960],[67789,92818],[90095,92577],[40582,54426],[80979,87066],[85544,91562],[70382,91572],[27724,45838],[78561,80354],[65086,99107],[50246,71738],[39613,49194],[18080,77755],[2611,61024],[36682,58225],[46010,72318],[63200,95989],[37532,98104],[20002,90986],[14084,88512],[12306,88667],[26396,47417],[59597,88907],[79287,86449],[43053,65012],[44368,58152],[74664,92413],[33310,73398],[9848,43721],[64951,89437],[61143,89538],[7487,60245],[84670,92377],[97498,98688],[97008,98398],[17462,64940],[64767,69925],[5002,53884],[2051,18759],[63513,91739],[37273,69678],[58254,63106],[24143,99183],[16753,95813],[77204,83050],[75548,87221],[8557,40114],[38474,65494],[71885,80155],[16838,87610],[8820,55654],[58922,66160],[78336,97022],[31457,82610],[90101,94140],[26034,30452],[92759,95795],[3363,92113],[49832,91907],[57211,85036],[43763,68013],[70954,84238],[80142,90371],[261,8194],[95386,97439],[47751,92198],[992,68399],[9554,16248],[44820,92765],[99349,99966],[13087,35168],[53164,89361],[61490,80378],[62970,98611],[80948,86498],[15351,62373],[55622,92421],[70280,73745],[8145,12457],[56394,93395],[31246,75196],[23898,99887],[88984,99937],[17444,84395],[77641,92634],[72856,95713],[57062,77466],[15742,48559],[89295,97111],[53625,56298],[69594,83802],[54617,72483],[49168,54328],[33176,76217],[10940,63123],[17508,73151],[43397,67245],[82214,93735],[49139,75605],[70796,98879],[71335,99074],[25404,99572],[20000,45481],[75873,76802],[88617,90765],[82985,98447],[45326,66097],[59263,91940],[71138,96897],[75245,93443],[93636,95735],[4515,69330],[39543,90668],[88780,96455],[65570,85719],[48227,97295],[20182,33032],[86514,98009],[27077,52269],[88461,93486],[10858,45837],[95528,99973],[99641,99763],[77191,82744],[80524,95831],[91268,91423],[47679,70851],[39057,78138],[75409,78113],[85416,90640],[51960,64255],[8601,49370],[30539,79879],[21101,67585],[8876,96114],[86898,94820],[37906,78749],[63763,78099],[54995,63278],[7996,90759],[39797,86625],[53191,73504],[9898,56266],[87594,91642],[54657,96754],[33132,90475],[36295,61302],[67492,97693],[21349,35178],[46651,88148],[3628,12486],[10857,57880],[37225,93682],[18809,56798],[51231,99875],[7208,68005],[73400,86998],[95767,98996],[8524,38550],[98236,99301],[89219,91150],[46434,93767],[2764,47804],[62414,78316],[1676,9076],[56578,62583],[19158,72274],[9294,76549],[53519,91808],[6899,52865],[9602,42446],[63506,74147],[21740,28320],[31262,77436],[97531,99700],[27964,42430],[33420,82283],[22264,76859],[85345,87159],[1909,8627],[79785,91506],[55024,88022],[59801,88764],[30047,99531],[5818,54954],[49223,84248],[69528,81167],[39876,59747],[18568,42220],[54149,92890],[81200,82598],[70502,85938],[47156,56462],[9588,88694],[5657,69090],[11780,15580],[13468,62265],[6142,45563],[58636,77002],[14493,69983],[29618,87396],[38138,56444],[48068,53929],[67553,72696],[70452,83159],[11834,84223],[62236,90448],[47383,47984],[31009,51972],[51790,83639],[61350,74495],[67560,83925],[43988,68096],[99791,99995],[26577,89155],[33031,47784],[24206,87471],[4195,5638],[65403,80734],[87297,91274],[13784,16773],[93575,98126],[19892,73700],[64024,76965],[69956,94351],[94035,97564],[42949,70310],[83419,86972],[5698,72078],[48972,54123],[79560,90758],[86954,93685],[38851,88663],[21279,38184],[29924,38544],[54563,59519],[70109,72084],[33228,84582],[62015,71293],[3380,64265],[710,41475],[97023,99428],[20895,72779],[1281,19648],[19105,67517],[60107,85361],[64679,76250],[46446,70990],[60690,98843],[34442,68100],[36178,49782],[77219,83282],[2556,96815],[67840,82746],[27610,29094],[62025,88921],[27603,86887],[61735,94521],[42521,44179],[85485,98255],[97691,99636],[71443,79066],[17889,45512],[37499,55426],[18570,94230],[8784,37996],[64274,84165],[10312,41369],[35000,86525],[49809,78613],[80598,85079],[8234,48840],[5527,52172],[64619,73218],[4788,17648],[54714,59811],[60974,79899],[90073,93152],[24503,85730],[19517,76189],[12736,65446],[35938,88971],[99893,99942],[31047,69816],[79066,91432],[79261,90361],[66498,97191],[79845,81371],[83789,86846],[85963,86678],[93282,99858],[74087,92579],[52789,87044],[74581,95973],[85123,91619],[84345,92213],[74419,78331],[19497,48125],[17068,25548],[90704,92935],[73802,75271],[6911,57358],[8977,86614],[31942,39491],[30272,91867],[5146,87438],[4126,56800],[30325,30552],[34414,72375],[34047,53809],[82896,87932],[93436,96183],[16689,46621],[7140,58848],[13118,78472],[81273,93896],[62218,64503],[82763,89413],[75528,90814],[17607,19870],[69410,75161],[92621,95771],[52599,99959],[53410,88998],[33765,46757],[22874,54450],[1492,14834],[23265,92952],[45777,65800],[36893,38139],[35577,71342],[23061,93358],[65102,66189],[72050,73509],[48714,53141],[77189,86567],[36233,78054],[98671,99724],[32382,62869],[91729,99252],[54320,93770],[15599,72188],[8842,17942],[40418,78867],[13573,17176],[57511,90039],[26455,61015],[23468,32275],[40012,84810],[39958,64891],[7883,41677],[7954,28150],[40087,55804],[62268,99095],[96921,97866],[49846,89181],[20018,68802],[11099,59033],[26916,92242],[15013,21094],[56374,89079],[15539,85207],[44217,76530],[20381,30585],[72883,90208],[30603,99667],[21503,48052],[30533,42098],[25695,40134],[32673,97172],[56773,87044],[29801,40298],[37048,88069],[8607,94505],[61485,94884],[91176,97452],[99610,99787],[82436,84745],[42991,74482],[38,27177],[36554,73683],[32035,48110],[83457,98590],[91920,98809],[12195,85262],[22459,43733],[2482,86443],[16569,84439],[17333,64251],[98666,99232],[38216,93454],[32344,47037],[95736,97859],[31761,97071],[70788,99342],[70586,71710],[24700,53789],[3471,61579],[36251,47543],[48001,94609],[32018,92600],[12852,15906],[40403,91569],[2095,42824],[28512,29086],[10372,95520],[73123,81308],[38663,44897],[65939,96674],[20527,87762],[7204,58097],[90005,98822],[46599,83042],[98250,99242],[84074,95379],[94547,96917],[15498,54674],[9546,50302],[87369,92497],[11153,18291],[27266,85900],[61678,64840],[23965,49367],[3359,76795],[85906,99958],[88347,92902],[4249,24290],[19977,75123],[4894,79092],[33495,43413],[50714,51770],[8409,36171],[61253,85050],[90854,92611],[38497,84949],[94156,99493],[88889,93632],[15617,67767],[48916,74641],[98454,99953],[21220,49069],[53372,88332],[29776,62429],[62232,84426],[64967,70946],[58833,65512],[56324,83239],[93440,94506],[26222,73479],[99662,99937],[65116,71349],[65189,91488],[78147,95714],[59694,73145],[28492,57714],[25,36261],[6475,84355],[18224,76143],[63296,69571],[83529,99245],[38491,50319],[28790,42268],[54158,57047],[62729,83281],[56363,82703],[53321,94275],[23027,56528],[55699,95206],[64082,67583],[20470,51451],[89406,91194],[80166,98449],[25657,55093],[55183,64678],[83782,99167],[15688,58075],[77310,91921],[24640,50532],[92848,99291],[42998,99530],[70584,90098],[51094,87843],[85267,88172],[1077,58272],[21666,83383],[60197,96326],[59849,79865],[22646,53219],[45833,62364],[68249,78517],[67862,75762],[86897,97730],[43159,72490],[89590,93601],[70513,85255],[72769,76867],[59860,62715],[41353,72398],[61731,63053],[52533,94368],[91965,96524],[12473,47954],[22253,52289],[38653,57672],[76655,79988],[25531,84023],[87455,88697],[74189,92266],[14480,68730],[22173,32999],[35452,41262],[49433,62224],[23394,99807],[75581,84961],[57092,79907],[40939,74487],[58971,71038],[95746,96646],[70931,73849],[92556,97408],[82423,86150],[99325,99831],[48006,91193],[89537,95418],[69393,80012],[56393,80221],[49230,81792],[9328,19763],[55528,97703],[62682,86966],[84856,92586],[7421,59435],[60228,69312],[6067,66009],[54230,79899],[34238,75495],[99757,99855],[41843,69295],[37196,51458],[31654,42304],[43700,87609],[17308,20974],[82891,87106],[61823,76367],[95962,98024],[43966,71764],[39103,89292],[58112,78941],[15444,80601],[67203,95078],[17336,53660],[46769,87656],[93858,98504],[96134,99231],[49962,79649],[68572,93420],[16223,50884],[41912,88891],[75378,77246],[50329,50367],[21023,67208],[39085,99060],[57241,93060],[40477,94944],[7178,16351],[70503,95975],[64751,92977],[6239,59720],[16943,23773],[60352,78264],[92940,97075],[61816,76808],[14901,85871],[77838,95282],[49530,55382],[20330,84458],[78156,91765],[54878,80713],[37669,77156],[66272,81412],[22615,95334],[88136,96068],[38697,54036],[28210,54767],[98288,98504],[15955,75404],[9218,29385],[77129,81354],[88308,89083],[62500,99580],[60603,89828],[10929,89049],[41728,73571],[22586,35941],[15763,36771],[14385,20058],[74958,78678],[35690,37381],[36837,96463],[92777,98807],[51627,62566],[66043,83437],[89768,90291],[94499,99512],[77404,97508],[64622,88983],[47891,56489],[94856,99147],[82396,86095],[3770,5011],[7410,94846],[36064,94498],[16978,56282],[6984,54571],[36498,60765],[55669,59700],[10743,36818],[50807,89946],[39145,80019],[49521,50390],[55520,74043],[94657,94946],[86461,96819],[64312,98538],[18520,78961],[35866,70770],[52138,52359],[41041,87859],[12164,75081],[91604,95781],[89147,96787],[8425,17941],[71523,91020],[94552,99646],[62369,68605],[6648,47414],[55811,56314],[13202,88513],[68831,74175],[45610,67327],[24949,56769],[75275,81370],[54808,98947],[66537,96493],[93275,93317],[19792,77018],[19990,56031],[26012,53921],[32928,66496],[33767,50425],[20547,48137],[93163,99429],[32527,48939],[4419,8408],[68871,69300],[51954,95348],[86095,98420],[89377,89737],[96074,97514],[61248,78432],[64287,78809],[73714,84288],[18518,30001],[16291,20898],[41456,94654],[55085,88616],[89631,93299],[42792,99219],[33009,51929],[8010,16695],[42086,77167],[7528,56079],[11169,44433],[9820,11862],[81592,98600],[72252,94185],[25860,64429],[20781,44191],[89665,98010],[4675,61828],[48767,51216],[26239,30212],[49714,92558],[90026,97599],[57053,74664],[19368,62580],[27795,58229],[78123,80210],[15652,77985],[2813,43207],[90355,93345],[69776,82759],[64019,83329],[94878,95035],[94629,95983],[92729,99850],[37017,45929],[49650,85588],[12822,91428],[83570,94342],[49989,58694],[80194,93968],[82809,89919],[92627,98764],[92974,97062],[79899,87972],[27210,30147],[75574,95845],[72518,86386],[61677,63582],[47943,54244],[81575,84271],[16914,31753],[12848,65239],[67532,73269],[66137,81234],[16259,59367],[68180,81944],[18094,69883],[23026,55155],[33975,76632],[33321,39684],[74893,97047],[66635,98224],[15034,39338],[11788,14867],[75503,94776],[10941,54133],[18247,27333],[98766,99083],[63849,94496],[54861,75950],[28087,39537],[79915,82111],[15471,47373],[12157,15689],[33357,89058],[45242,67115],[88702,98088],[49162,63683],[1504,40887],[90118,99095],[88047,88115],[5813,21515],[10955,55581],[37386,48287],[81101,92102],[20694,68322],[29967,94281],[50318,51087],[10343,93542],[93972,98797],[55123,85078],[32791,56919],[30920,68901],[43889,43899],[18498,91448],[23701,74741],[54533,65800],[77969,78454],[12155,14333],[55845,60654],[81529,98040],[30196,73125],[71571,97660],[96284,99807],[13261,42208],[59358,71418],[94728,96111],[25957,77640],[98810,98873],[57153,99018],[24743,33599],[27150,38666],[28222,72947],[70932,97325],[36453,78611],[51053,52792],[28275,69755],[18496,33008],[38638,84938],[65967,98229],[22045,77073],[61305,88518],[41087,74005],[57650,58520],[98984,99953],[91796,95379],[79745,81190],[94530,97308],[74754,90958],[60833,85145],[20889,49516],[19960,25305],[61097,80175],[40890,83554],[2671,99742],[84082,93871],[59469,86116],[1419,13684],[47684,58369],[60227,67742],[19564,22133],[21970,82728],[5416,75035],[98608,99521],[49840,59173],[65821,83964],[3673,48581],[22423,27634],[18040,29575],[85108,89789],[1928,8196],[29250,83312],[65295,96974],[34115,93949],[29842,49120],[99714,99810],[60593,76281],[21431,38344],[39612,81553],[12407,24467],[60983,97570],[9645,14015],[14391,81884],[25477,76174],[92637,95606],[48385,84003],[34502,40283],[27034,76158],[98613,99298],[40770,65904],[94242,99289],[52188,87101],[55377,56281],[85311,91042],[77509,78873],[15668,31985],[87654,97847],[12580,50712],[79173,90571],[27141,56554],[14628,69484],[28824,44592],[50991,62601],[30302,63413],[7966,64411],[74843,85791],[86588,91340],[42548,79812],[3251,75449],[71761,76600],[3918,12162],[13890,60260],[52729,80756],[5046,19374],[8751,92046],[95580,97404],[56725,61980],[67442,88858],[50199,77394],[20572,65625],[66844,69599],[25395,68034],[49477,57174],[50971,55839],[25385,71672],[48662,60793],[35426,79000],[20179,97283],[70007,88142],[69,24868],[76159,80500],[6629,11959],[85782,92573],[75682,99201],[14131,98897],[15365,79226],[9605,16823],[59290,71680],[47663,96058],[97037,98312],[7939,32408],[83114,86328],[70030,96592],[19052,52873],[80128,89238],[42392,45737],[73450,94663],[94537,97566],[38501,66552],[69057,90986],[89747,98835],[62385,75784],[80207,94922],[79346,95874],[25064,60131],[50844,82968],[15656,55761],[48778,89273],[40367,51820],[48989,61254],[4173,76881],[28593,43666],[99012,99301],[51346,62715],[36130,93254],[61107,73262],[30880,48433],[70555,96761],[37255,83755],[84646,89142],[21650,28560],[80667,92406],[19589,59507],[96282,97970],[35857,47864],[45328,64936],[6236,39181],[89437,93989],[17399,80815],[74809,90500],[95196,98455],[83861,88714],[86264,97997],[17337,67426],[37612,80265],[17794,24334],[79153,84958],[5136,61616],[92175,96499],[85286,92163],[43242,71762],[67826,72014],[6783,9993],[69215,72477],[38145,95040],[12224,28422],[93907,96947],[16812,75974],[1744,85272],[28829,80054],[14821,90005],[72592,84167],[78801,92345],[66761,85014],[78200,92344],[59634,87653],[9395,58647],[59780,80021],[51289,61038],[23878,70305],[15751,55084],[6347,92348],[23088,68873],[65489,78603],[72308,88521],[50264,61884],[59463,87859],[27825,87165],[74011,79845],[89552,92595],[25991,62980],[16367,76125],[72852,95545],[77227,87611],[6669,12785],[58918,84930],[39666,46348],[12361,14615],[56693,83491],[98640,99675],[4484,17324],[70474,73355],[72824,87775],[43389,63791],[32776,50767],[74317,77600],[54341,75634],[51309,80878],[49836,59611],[53430,64587],[87801,88048],[34689,69184],[26011,99541],[43186,92135],[32023,35384],[433,36638],[34860,46445],[77562,99205],[66420,80335],[44530,72818],[22507,41955],[15795,67549],[97172,98537],[9229,74503],[72287,96605],[84500,94366],[49794,54103],[87546,89921],[87477,90731],[38923,67745],[36565,59054],[26029,29307],[75481,91903],[21049,59162],[52444,89786],[2939,20994],[4037,6283],[29928,40712],[27081,86044],[37181,46097],[35875,57209],[52237,66547],[45660,61691],[17236,72265],[29852,47070],[35911,49298],[81233,92920],[34215,70137],[91125,97953],[84123,94038],[47527,96365],[91107,91755],[21419,43257],[74825,98928],[63657,73545],[79635,95680],[79181,99354],[50945,99289],[20465,58951],[34460,38639],[23725,45506],[29693,82253],[72066,93294],[43068,92593],[37727,48103],[20221,52854],[10153,60740],[30439,82732],[49261,69523],[48525,67820],[79530,94071],[50770,72819],[13217,45081],[87379,87594],[99540,99622],[43960,88528],[82128,89100],[47882,53581],[70172,74998],[64765,91013],[49774,73330],[23655,60645],[6218,73036],[9828,91196],[63830,95835],[44802,75818],[58564,97033],[69235,85311],[84281,87128],[42419,84497],[13531,30954],[39549,44940],[63537,68112],[24386,32336],[55397,65332],[27324,78815],[84748,96935],[70115,79421],[88344,93286],[92497,98896],[79097,88771],[32723,60205],[72867,94047],[79499,94578],[25062,79953],[84609,92852],[98964,99458],[35827,44904],[78475,90938],[79524,83689],[84310,94939],[69948,88922],[16704,75667],[87400,93843],[6486,98521],[90943,92756],[7889,73246],[44963,78541],[31402,90983],[93543,93866],[51528,78518],[96057,98438],[40003,90534],[45967,58366],[57449,57832],[72444,96332],[80562,92438],[92772,94997],[11647,57148],[2713,67074],[67177,73469],[67892,99059],[23800,84269],[26337,42756],[29038,66903],[30702,62411],[78919,96160],[17440,63627],[25481,54001],[92277,99527],[99376,99902],[75825,98234],[12985,79882],[71774,77862],[6897,8242],[79689,80998],[65437,99577],[88251,97781],[5530,27292],[41268,73592],[18814,49212],[41901,72440],[51328,91743],[17619,94342],[754,56298],[17834,22231],[22902,78932],[39017,94372],[57575,89610],[90985,91054],[57322,82712],[84200,87299],[9805,26186],[1547,89775],[32690,86142],[85927,98468],[55146,96631],[67394,80837],[18499,42723],[73152,78457],[95233,97829],[2701,51045],[18800,75481],[969,40038],[62397,93831],[43992,45709],[37176,93855],[16849,94772],[28812,86510],[67514,97003],[10481,32151],[3900,89555],[46812,62471],[26165,50625],[68211,70633],[22335,24172],[29653,34385],[36646,40207],[33346,93746],[46101,67249],[20614,57902],[7592,34351],[29532,98353],[22171,63156],[69269,87066],[81860,86065],[98672,99647],[24857,68353],[43554,82501],[58283,89039],[86708,98888],[90110,93662],[79540,82098],[36915,79323],[9477,44914],[26902,61621],[19252,69464],[53873,77244],[33229,87352],[65842,71359],[83703,98320],[78079,83462],[92987,95073],[83957,93773],[13362,26402],[4006,12935],[29696,94393],[34194,72006],[5472,59367],[47878,49671],[16103,64443],[7785,55663],[21068,99395],[81493,85542],[1023,29384],[57307,58806],[41830,60341],[43327,55679],[93604,94823],[70462,78639],[26216,98916],[85530,89784],[43144,73775],[72512,74086],[66911,84073],[73746,80441],[20627,67506],[46315,65243],[52418,63537],[5625,91180],[84450,91319],[39796,86837],[13421,43654],[24595,94980],[38749,41544],[29173,59140],[34370,89968],[11853,11959],[47048,59528],[90535,92736],[923,5199],[77896,94529],[73277,96136],[78551,90011],[71830,74066],[42118,43054],[48059,73535],[15487,61796],[78090,81643],[93558,96671],[20976,49962],[80100,91283],[85475,97010],[22215,51771],[47739,72142],[13007,53512],[89993,92928],[77844,98107],[27977,31578],[52677,96809],[27378,28483],[88639,98314],[63834,88481],[93343,98029],[96861,97059],[12986,81131],[37308,71162],[4806,85031],[81578,85484],[49080,60964],[13130,58977],[75999,83399],[92879,96538],[14047,57376],[10678,20006],[91846,99313],[72378,99230],[11030,11642],[362,25978],[24720,88413],[98539,99145],[52900,84583],[40044,79176],[76353,89937],[60869,95553],[31251,91259],[64219,90316],[27707,84796],[40482,41547],[98634,99568],[29977,50166],[98249,99780],[78428,93413],[208,35973],[36240,38460],[30359,42714],[53592,70588],[94399,98668],[90503,94268],[23258,85612],[91612,98466],[89367,97090],[40390,59080],[91625,99454],[62680,96267],[31424,79708],[66341,97256],[95088,95983],[18741,46654],[49711,67588],[27043,38949],[1865,91237],[17041,71181],[12887,91263],[10646,94418],[14596,46088],[21083,99338],[46468,86682],[35908,65766],[65329,93421],[92896,97649],[32970,97139],[30695,95455],[77686,81298],[90194,94299],[63344,73256],[80699,96202],[62094,91885],[644,26102],[28695,99063],[60720,74665],[23743,67099],[90842,99570],[69706,85985],[74659,90226],[49302,66932],[80191,85959],[30712,52790],[99040,99968],[5220,82922],[63006,74602],[74252,99762],[16659,29698],[14748,19430],[86016,89699],[88314,93603],[91863,96447],[90857,98365],[81064,85400],[88122,91703],[38775,67369],[74944,96549],[56140,91569],[25046,64096],[90527,94685],[34698,90231],[22232,95596],[38676,59407],[77313,79641],[26254,54928],[95044,99441],[47517,96786],[11910,88687],[23727,27258],[91434,95243],[37954,56001],[77722,83568],[32679,39398],[7389,36010],[30017,68914],[25673,77632],[59177,91637],[7379,30399],[3038,66305],[9283,24048],[87959,88416],[19645,52371],[64984,99350],[60592,97835],[35091,82023],[62517,99448],[69042,86269],[36805,46142],[75111,83146],[4144,25934],[25624,53507],[39136,79359],[16717,40382],[78003,82048],[6109,46323],[38643,51412],[15456,38440],[10739,25605],[53960,95986],[73503,88050],[11181,57124],[74978,85350],[81282,94363],[7228,71921],[30450,69598],[75932,77513],[86743,88644],[99905,99929],[49258,66002],[35735,67190],[65107,77159],[54445,79641],[98521,99487],[55081,78748],[53576,83434],[505,28966],[35041,55850],[35886,71029],[18649,23589],[67419,86527],[46024,61451],[66399,71763],[31189,97209],[93755,99712],[94269,94625],[87045,94726],[3394,45295],[71035,74297],[78278,83759],[94065,97710],[9032,91599],[77621,78969],[41122,81443],[51206,85148],[77724,78179],[69135,98321],[5126,13004],[12426,71413],[20216,72030],[97575,98001],[63369,83255],[14824,61988],[35361,97988],[16195,28168],[5770,9712],[83273,87299],[46390,82733],[94185,97594],[21551,42688],[48115,63415],[93564,99943],[86845,96840],[8585,25779],[67637,91852],[3438,64269],[16592,69515],[77754,87515],[97487,98312],[84976,86214],[34866,99502],[85848,90024],[74577,97602],[62527,95033],[19730,78860],[33736,38501],[17012,34112],[94799,96391],[46719,91632],[82531,88868],[84366,98357],[19608,55538],[35118,89636],[32000,69918],[32659,51080],[28716,87589],[14378,91534],[13344,60345],[76699,98429],[86722,89986],[48585,79408],[58412,78093],[7398,11757],[9052,61345],[59565,69764],[28696,34796],[17644,33784],[70646,97586],[52241,78888],[48124,52220],[84499,99971],[31727,32279],[20729,26139],[69704,78129],[22152,39063],[23520,31537],[20339,53583],[5238,35360],[55141,69156],[43126,54678],[83281,99607],[13042,46731],[63972,76920],[85556,88915],[22702,94449],[51085,70538],[78627,87950],[88488,91168],[77497,91331],[56225,97747],[55791,95350],[43317,84373],[72408,93410],[41042,59366],[13839,53585],[41394,96480],[75853,78402],[25162,72531],[88124,98143],[57201,96345],[8738,98985],[99619,99864],[29790,75297],[35547,75385],[97944,97946],[13843,20821],[1800,16601],[1282,51666],[35173,59853],[22211,35231],[39601,87729],[99881,99965],[16816,24097],[89606,95064],[18357,74838],[63024,90684],[65176,94625],[27279,81494],[26314,70758],[30846,98526],[66716,90136],[24064,90622],[66372,91031],[98111,99014],[68440,79263],[27962,83800],[72354,83654],[24707,94885],[82753,88098],[61630,89196],[89701,97667],[5557,96260],[57478,88021],[28843,97773],[84573,96229],[20109,39590],[65129,79524],[87134,99189],[48704,98500],[84973,87169],[64949,67356],[61887,79384],[19147,46932],[25602,88989],[76049,85010],[1199,61441],[64143,74277],[73129,80642],[61390,66742],[42555,74525],[80702,97072],[46242,49719],[74675,85314],[88323,97083],[78563,81590],[63975,71677],[87679,93176],[26543,55046],[44004,60185],[58679,78981],[91340,91928],[94147,94676],[59185,60035],[97972,98902],[2353,22943],[81957,91881],[86998,89921],[40757,94255],[83738,98162],[36008,69749],[60264,96277],[40066,65968],[48433,80675],[67349,95732],[23136,25755],[79629,87927],[22804,55275],[46702,60366],[50712,96478],[61372,96828],[92933,98827],[72489,96869],[60829,96987],[30799,57699],[81294,90352],[2033,4900],[23051,38690],[28792,50160],[21026,38203],[87988,91191],[18986,96611],[76723,82447],[35128,41924],[53318,64809],[4005,53008],[77553,84319],[68749,95489],[27196,66400],[83701,98374],[42838,86432],[82698,92147],[49579,96497],[36830,62933],[99820,99961],[25329,90531],[89894,90952],[84188,93075],[8241,34499],[31249,85010],[64528,98702],[75325,95464],[22668,47401],[92491,94670],[70174,81016],[95415,98027],[54638,61541],[64401,95061],[83681,93870],[17413,19857],[20738,21289],[64634,89886],[16695,84050],[83792,99611],[69318,75644],[72989,92305],[84360,84373],[46437,96514],[67441,69356],[66377,94167],[8316,26503],[96888,98710],[50642,83598],[74290,78480],[79709,80537],[2269,33297],[78892,88837],[65234,85626],[9710,30465],[15393,53082],[10363,20692],[46207,52970],[9301,85413],[7018,51539],[59808,60361],[18009,51608],[8015,15688],[34431,94330],[69925,96910],[58399,98795],[95354,96622],[53966,64131],[92982,98232],[94694,98812],[87979,93247],[58448,62027],[83099,91635],[85608,96940],[53601,68381],[76290,84096],[72766,91940],[8554,87812],[38284,65400],[10789,90614],[28882,59736],[5453,75274],[32817,91174],[71589,87048],[20208,74192],[51449,97436],[68952,90599],[3687,90991],[32526,41962],[88106,92420],[82692,95237],[55216,72127],[70723,94057],[85897,93776],[59695,65058],[60133,68822],[86111,99791],[91295,91884],[85396,95941],[92919,95841],[78478,80226],[8376,63169],[25594,89154],[49053,98659],[51193,92499],[63266,64753],[32186,76303],[14958,96221],[47315,64047],[50168,56208],[51235,99535],[45517,67549],[36863,93020],[75572,83709],[53574,60061],[30258,47558],[45691,80419],[6504,70130],[99345,99829],[27194,33216],[14274,18415],[38694,44812],[77635,86182],[90513,96500],[13270,90935],[44697,99158],[71391,87998],[80232,99084],[72411,98132],[51437,95016],[70494,74718],[1705,86610],[58639,75360],[1767,10947],[37541,63683],[69483,74463],[50096,51306],[37024,87507],[62893,70631],[13336,85507],[9037,67618],[59747,90894],[49596,83052],[81053,96948],[70652,96610],[24495,30159],[31081,35506],[72875,94025],[61788,64726],[79532,98738],[42586,84023],[96816,97993],[46951,61254],[39012,82626],[44907,75994],[43290,79588],[31199,84932],[49982,62220],[38064,71430],[28403,80919],[54719,61226],[79788,95553],[48484,65001],[65608,68012],[35132,50335],[63388,74177],[75088,78105],[8967,74426],[43329,89672],[63913,70708],[71783,92675],[68703,82418],[30747,62536],[20641,82031],[239,58964],[63736,95829],[50182,97083],[82017,84095],[46531,88950],[78182,98270],[11511,49787],[27326,34428],[895,18630],[29996,41901],[16932,54090],[90831,95239],[16468,27632],[668,63474],[79610,87304],[71471,71686],[24574,43063],[82034,82590],[43658,45850],[40042,95363],[99158,99561],[83089,89484],[47321,99931],[39197,77981],[8577,82293],[34658,51855],[72840,83143],[62357,78583],[85728,89945],[76862,99201],[86559,89571],[60979,66594],[19335,33887],[91518,92498],[66181,85621],[50667,59117],[24684,65683],[41615,86973],[70191,83832],[61457,90618],[10188,87628],[76325,92733],[25856,62079],[2151,58583],[72607,82726],[38044,70418],[17504,34741],[76208,84502],[53868,84063],[52803,67781],[55675,89586],[90263,93931],[25532,79681],[40091,50359],[63247,65846],[54726,69129],[15574,28090],[38480,99808],[6603,52563],[92056,97399],[32512,36965],[60680,79559],[85799,96175],[87026,89046],[62647,65675],[95582,95685],[40999,66288],[24998,26047],[20842,24074],[38809,67794],[52536,77102],[20119,27524],[22512,57458],[45396,57974],[15694,33098],[25716,55875],[43641,72008],[19255,29938],[87405,97546],[15983,60290],[8656,55318],[93268,97909],[2837,80345],[99853,99919],[66411,88714],[23905,78598],[80949,86349],[21992,47778],[93951,94487],[66526,77269],[70250,85928],[52854,88309],[91022,92039],[65039,86112],[55614,56426],[95816,95983],[71551,95227],[86294,97405],[96668,98588],[39262,90508],[30608,74189],[64120,67730],[2885,19320],[42649,97666],[29154,86018],[29000,79821],[2961,95125],[87331,89473],[53379,78915],[28779,36833],[79201,85011],[41,98363],[43611,86359],[99021,99295],[19127,62703],[34393,98515],[6505,32781],[6099,56900],[58774,92815],[22865,84498],[37903,85780],[69845,86317],[34685,72363],[43362,70384],[27542,59285],[77739,98107],[17546,74424],[36802,70297],[51742,98581],[13476,69791],[20845,47419],[70984,74817],[94498,98804],[8566,63323],[38887,83174],[6941,73998],[19728,78213],[24267,28190],[78195,91053],[25200,89506],[59493,64979],[57147,68855],[10451,81842],[91347,92565],[86751,97876],[15891,73629],[68145,87189],[85577,98681],[89749,98367],[41853,72956],[31067,60710],[15271,72283],[42376,51490],[54520,77937],[23105,61906],[66905,74595],[48757,54777],[11903,57927],[75686,87396],[61244,61895],[95637,96794],[18138,25771],[78358,99805],[8391,75486],[88857,98265],[30677,45184],[96031,96982],[22917,88751],[1919,15356],[90784,92700],[3497,84191],[99782,99930],[35621,91273],[50356,96394],[15602,37267],[18793,39317],[42427,80873],[25828,68412],[21778,61184],[45899,90910],[66655,92853],[61825,75989],[20312,91985],[84198,86813],[39779,60669],[68003,98584],[76124,86628],[44417,98521],[94779,96217],[6701,53554],[81739,97973],[1334,64525],[70276,92451],[70498,97920],[62555,81559],[23324,45008],[18328,79453],[92558,99777],[53880,57222],[26601,79423],[67206,94207],[30982,54159],[78800,98035],[19336,92566],[80613,87698],[8330,23955],[22863,76800],[5678,72293],[75327,93581],[64482,90270],[88648,99754],[37286,41337],[68293,93974],[4648,60768],[92483,96652],[96669,98515],[75793,92913],[55418,89989],[56181,93095],[35229,74508],[85627,90527],[13870,27118],[82085,83299],[93905,94089],[21127,85674],[80318,94640],[89422,99747],[90064,91579],[80938,99040],[94663,99602],[237,70567],[56390,56391],[82493,82773],[9453,40102],[96386,97810],[91878,93639],[68985,82639],[10181,56889],[91186,91692],[89635,91002],[84318,94295],[47050,50040],[46519,64672],[46355,71095],[56286,56452],[66165,96803],[50344,84063],[28917,47618],[33676,51022],[40292,73940],[28668,65062],[29771,44010],[95187,99942],[74196,98542],[90387,99272],[95145,99249],[70116,81113],[77073,79675],[70552,94124],[1543,98303],[90446,98141],[13129,33058],[55615,69540],[75367,86146],[88285,89232],[69562,93412],[12788,22505],[82890,90831],[55043,57193],[67228,87630],[19107,44374],[3191,69445],[45364,62555],[84465,95611],[10490,48361],[52846,68908],[96382,99389],[8423,55239],[57424,62471],[25448,73078],[85007,92760],[36797,61734],[70765,72562],[78255,87992],[58096,89854],[80233,96807],[93012,98732],[16010,37971],[27120,58034],[36730,54538],[69857,92731],[54052,91362],[59235,82649],[18896,90093],[55051,94084],[73966,98084],[63812,64964],[71862,73543],[69815,97734],[74876,79419],[65056,88652],[78972,94870],[40112,94301],[11283,91324],[86781,92983],[14125,37469],[14509,16391],[82566,84299],[91809,92117],[72328,95194],[60947,76633],[27245,74643],[81062,81904],[2167,54588],[3124,61283],[97190,97730],[20938,76506],[24914,56138],[76420,96896],[28765,77297],[35665,73681],[72462,97272],[51393,60071],[58757,83814],[12066,64711],[62244,81295],[59890,97338],[40495,53696],[58559,73929],[39216,50296],[36294,47931],[18643,80541],[39135,41060],[59806,86885],[97532,99425],[71104,85551],[69366,70279],[32470,73784],[51892,60419],[28355,63876],[10134,25261],[85750,91429],[12512,83026],[11945,22850],[5468,51245],[47492,75113],[13410,62474],[80584,94016],[92447,94708],[24199,33822],[48143,65990],[79650,98435],[95424,95914],[11497,47464],[88632,94094],[37201,95965],[44536,98853],[23963,29902],[57592,75795],[59090,63045],[70410,96976],[70102,73487],[46254,85996],[77631,80093],[52892,62824],[58313,66645],[77296,81663],[93037,95473],[34251,90210],[99669,99925],[22662,81373],[66907,94950],[54278,82350],[51798,73642],[83177,86323],[24476,56425],[8220,89405],[35548,43255],[88941,99090],[59859,85273],[60128,83384],[52869,53250],[50941,82544],[49965,83221],[10726,57172],[60867,98373],[8206,39025],[78864,91179],[63580,66364],[99368,99458],[32482,60494],[95512,98538],[8657,13023],[10195,55261],[30507,92793],[69011,84818],[63365,76129],[53426,68188],[60745,95856],[98943,99161],[23474,42104],[86800,99739],[17470,34144],[94328,94831],[92455,99042],[40672,89564],[66687,84016],[57893,92556],[80806,97356],[81599,94124],[65759,70694],[95689,95968],[33479,43480],[87528,97368],[32258,82473],[89353,93116],[37234,37973],[30082,81568],[57671,90876],[13397,78879],[21935,60522],[91617,97734],[43321,96003],[55974,96197],[41663,63761],[5095,32718],[24779,74975],[55422,55922],[90863,97640],[60076,72084],[1304,32358],[40354,77847],[45592,74937],[92788,92829],[49956,52556],[92105,92607],[7428,10308],[84009,92396],[16108,68936],[6461,48449],[84141,98510],[7056,19374],[7195,63612],[24394,74604],[81706,82158],[90738,99972],[49198,50914],[54685,99442],[44512,77586],[79158,79601],[60633,93798],[41037,97878],[79685,80574],[89186,95828],[48339,48615],[90626,90751],[47930,58225],[16786,70052],[68168,99516],[92486,98385],[38253,97551],[65358,87099],[88200,93668],[29226,91502],[52971,61710],[65306,92083],[95639,98176],[4424,8052],[86643,98673],[66382,71112],[32098,75003],[77264,90901],[86760,87838],[7922,60496],[15275,86002],[79110,82335],[6565,95110],[37446,81086],[79827,98094],[76431,84134],[76971,94992],[8281,64878],[25270,32129],[71013,80916],[26118,65882],[36115,97969],[33671,88449],[21985,95920],[46337,64333],[22502,82567],[62859,77781],[63526,83398],[26508,71903],[36711,49106],[85117,91948],[35205,48592],[51109,58009],[86970,99845],[61651,96037],[66208,88300],[95200,96617],[92453,92569],[49970,53159],[94261,98329],[95391,98791],[89949,95989],[26541,52739],[54734,77187],[38576,44497],[78463,86849],[97878,99257],[68147,73201],[43293,79518],[81078,96268],[24399,56724],[59013,97712],[79898,82430],[21599,23172],[49604,85550],[35182,42724],[9161,85462],[17883,75567],[40755,93144],[87433,89158],[63543,74149],[59451,79699],[8894,98920],[96522,99100],[10397,50103],[78646,90098],[10683,11797],[95021,98272],[24510,96992],[71872,86095],[51573,54446],[51812,91997],[26914,41680],[37545,98116],[79698,93634],[84225,85346],[17159,54570],[64200,91448],[59278,67597],[55389,73291],[1873,57696],[33740,79379],[163,97714],[75906,97006],[47831,89110],[71423,77290],[97303,97386],[62111,96707],[7660,59356],[45417,75632],[65601,87722],[48741,67437],[13802,55471],[3376,82840],[63197,89296],[96989,99912],[50964,55183],[73597,90180],[77214,89825],[15860,74463],[35130,83499],[99799,99999],[84293,90041],[55847,57498],[31349,62490],[95241,96763],[14957,76095],[27291,97147],[6610,73266],[2293,26879],[88288,89132],[61204,92315],[88746,89278],[76779,91369],[48070,58761],[38807,71525],[191,47932],[4835,40304],[78188,85996],[46256,50774],[87171,95758],[33727,42022],[51679,87313],[14803,82295],[72258,90236],[73751,85771],[77743,79548],[43833,44891],[24367,70565],[30262,91988],[78448,95046],[94366,96837],[78650,83502],[27339,87329],[34602,72024],[28701,77808],[78763,94459],[11942,82085],[26701,31609],[18813,95880],[53587,89504],[79967,84725],[72948,78091],[85439,92717],[13423,35471],[21374,36149],[15164,58712],[40076,94245],[40953,99923],[41734,42416],[16733,98954],[49984,97469],[39784,91988],[88790,93606],[71195,75365],[78934,99811],[24809,65033],[71247,91272],[16493,73804],[34899,90704],[12545,26495],[64499,94294],[26380,57648],[13255,88007],[36999,41697],[7114,7181],[57996,84773],[57917,61901],[14774,22360],[28977,62234],[77601,83134],[65177,87859],[37931,90722],[88558,93489],[59537,95686],[86390,91634],[63217,93126],[3169,23467],[20598,75348],[96962,97306],[30678,55715],[89745,94790],[99293,99841],[74530,80566],[80597,93687],[81483,92260],[46480,99847],[16869,81550],[10123,98865],[98969,99411],[66793,92787],[96095,98713],[29378,68788],[3589,76532],[89504,90472],[45779,59554],[67652,88380],[57444,60240],[61599,76879],[19428,53692],[4639,92014],[39464,73462],[4540,84034],[51089,98021],[3056,66290],[57891,69893],[70575,72910],[8043,51989],[36834,45022],[59176,97449],[39976,52833],[39694,59990],[12076,20250],[14132,29003],[47099,83179],[66470,95301],[10618,16695],[2239,16062],[46906,49691],[95479,99284],[31680,61701],[27729,30703],[80568,83505],[43676,95722],[49527,99117],[16164,97389],[10699,54667],[60481,95417],[34109,81342],[17795,23040],[55866,87512],[3969,23926],[98029,98173],[87323,88352],[34540,45661],[32305,96426],[45458,47886],[66861,71486],[7738,46553],[17420,65827],[97960,99561],[63852,79401],[51367,79782],[98260,99625],[28249,50188],[82912,95889],[61189,74359],[779,72994],[29439,61370],[92881,98227],[4368,7538],[55346,93019],[19637,31889],[95607,97489],[57543,66824],[41329,96598],[84182,85337],[50795,61794],[61856,62162],[75568,79421],[87512,92175],[43770,82641],[73611,86405],[42532,45214],[78869,99736],[60190,67205],[54759,62748],[37523,40955],[83383,84448],[45244,89999],[98263,99811],[95012,97310],[3709,99205],[41194,88469],[5687,71457],[50564,94203],[91225,92929],[52489,89415],[69267,88958],[32570,93514],[60770,84951],[40211,63852],[60822,91094],[80884,97957],[62733,95837],[91351,92085],[50875,56237],[96429,99887],[16068,84593],[89796,90363],[92590,92949],[79567,90329],[11846,35291],[48941,68443],[37350,47668],[68081,87783],[65588,70533],[23729,64497],[33189,68650],[87363,96647],[34586,94098],[85503,96975],[98239,99221],[68708,79345],[97996,99235],[58444,92526],[34165,41703],[78170,85666],[95154,98227],[62582,74688],[12253,23374],[77585,81431],[29720,42380],[23397,33835],[98217,99242],[20554,27807],[63092,71480],[34674,36403],[35732,37409],[9525,25713],[14276,88158],[18316,98261],[90820,98968],[3847,80766],[86644,96504],[36493,89142],[22601,40210],[76763,89680],[82451,86020],[81308,86887],[28044,33562],[95173,96333],[58051,59929],[56979,69135],[18647,60362],[72823,77052],[15501,35043],[90567,91785],[4837,93478],[36451,91537],[1752,80705],[62510,91383],[57518,79388],[53172,66040],[91644,94591],[77117,88566],[51134,57100],[95101,97805],[63766,73630],[93977,99086],[95606,99107],[11987,14469],[73529,95493],[23864,98930],[75881,96678],[22157,29432],[11095,24170],[60323,87857],[87183,89260],[45345,92664],[55789,75196],[36092,89638],[89143,90457],[51635,94221],[98847,99964],[51372,85177],[98424,99038],[78043,79860],[89596,91352],[22144,71512],[72502,91294],[10438,28716],[17348,49557],[80799,99076],[28165,37935],[62470,87285],[22672,33729],[74431,74632],[45279,51421],[29141,30827],[11311,78705],[47476,72297],[60329,95562],[64724,74519],[19443,96949],[55540,83263],[5272,73795],[75940,89546],[8262,38393],[89023,93940],[2845,25911],[21195,72222],[7159,96290],[67015,83527],[43830,57272],[35368,64617],[62482,73007],[12885,59176],[10426,28202],[59332,83240],[23706,64946],[11799,70406],[19037,53439],[6375,47897],[390,68856],[97503,98043],[85817,99044],[13558,90452],[48518,74234],[18770,98193],[14993,96900],[92014,98095],[25142,58007],[97688,98461],[73982,90593],[63066,70561],[4632,32880],[74716,85300],[26375,34894],[76089,76592],[24547,93665],[12106,71773],[64132,88834],[80602,87848],[74536,97829],[80323,90962],[72168,78008],[68407,96339],[24626,48717],[26280,59717],[62948,70630],[66228,82380],[22185,97840],[67530,82251],[92173,93348],[74178,96860],[78260,93254],[1618,78258],[44765,59503],[19294,79988],[11083,22322],[10897,82504],[23847,82193],[56464,67060],[7126,59877],[35225,98737],[87472,88441],[20287,34588],[83727,91468],[23545,87069],[23150,66317],[48175,87478],[60563,79398],[62936,68430],[21225,24519],[90297,98077],[6292,54922],[26556,83193],[66269,84130],[78141,98325],[91096,94020],[92880,95520],[34859,56645],[1821,77518],[75414,92635],[82732,93167],[18186,29272],[894,4775],[65936,89133],[89155,99993],[7179,61575],[72720,86601],[21430,41107],[37653,69543],[52014,64591],[66297,87353],[9373,89817],[29295,29337],[58236,68433],[14038,25226],[73969,82314],[47592,66973],[96051,98233],[38126,91251],[59434,87531],[45753,48466],[58189,76100],[3143,10730],[38988,62295],[38102,61134],[7896,79682],[87240,98776],[94385,94638],[72845,90227],[65125,72295],[55884,70425],[4374,4664],[38473,67041],[9461,91569],[29698,52922],[55787,80484],[28088,75868],[43341,85197],[59874,78368],[74097,84197],[60301,79851],[92189,94077],[96720,99989],[44772,44891],[17968,98110],[10257,55407],[64367,87731],[47206,91771],[39000,52930],[5962,80042],[31822,77211],[71166,92729],[34429,67782],[20610,81595],[36575,62762],[38305,83849],[77650,84908],[16663,76576],[80607,82900],[82455,95419],[30266,44308],[93736,96273],[78518,83960],[93989,94359],[59049,73321],[79944,91528],[95625,97624],[42221,49712],[17130,75541],[51504,71382],[85536,93722],[89523,93651],[85591,96762],[72808,75354],[38006,52970],[7601,34725],[84531,85867],[1830,32914],[61509,86536],[9571,52445],[1828,98288],[70189,99620],[23550,39019],[72920,90413],[17036,81932],[94380,96802],[17759,33065],[74273,92727],[76158,77140],[65679,83434],[34878,45124],[48050,49203],[8615,9103],[74083,79830],[6342,73096],[32209,44786],[81203,95288],[57056,64233],[58202,81711],[56919,81304],[11190,93573],[46290,60005],[65734,89547],[47722,92156],[14705,43182],[37765,92286],[89205,96867],[25988,97439],[32681,43053],[37223,59001],[93073,96894],[31567,56078],[5752,84825],[83545,93472],[7113,95342],[4175,70159],[41010,44579],[94440,95991],[90633,99825],[15323,61023],[22711,64785],[47626,64087],[37292,70090],[98076,98196],[79747,85843],[57880,82368],[89210,91460],[41448,96511],[47560,65802],[24742,97528],[30744,42878],[61918,69029],[16873,92814],[42307,56512],[74182,90267],[82889,88973],[22298,57594],[54173,69864],[23204,44615],[58463,97437],[40496,87584],[80829,98141],[13055,51592],[18199,57026],[98692,98980],[15594,52346],[49307,68167],[16168,55994],[70881,90345],[16526,31808],[71941,78570],[83405,89259],[2746,18531],[48014,93431],[9098,56310],[66477,94114],[86326,90902],[29506,37504],[64246,98193],[37568,55621],[28499,70464],[98115,98500],[67511,95059],[50249,66873],[24236,99434],[46641,51198],[15802,19398],[43134,63752],[67776,76152],[11027,27560],[31334,85867],[27908,48372],[2544,10872],[42160,95060],[50594,70757],[59656,83687],[34998,81291],[59778,65973],[12620,79401],[64829,76833],[14938,92930],[34184,57695],[25319,58735],[70419,87428],[62804,83078],[86763,94400],[87763,97535],[42736,67098],[67698,72046],[46076,61562],[64703,76258],[49257,93937],[57701,59365],[95050,99181],[79160,88038],[92548,94149],[36169,67818],[74626,79351],[36495,73175],[56033,58322],[61543,94991],[43935,99026],[62806,72805],[1544,23265],[41776,53639],[89160,92770],[7700,73030],[81151,91605],[5723,96833],[46107,93572],[42073,47759],[1935,33604],[92316,98948],[89961,99547],[96350,96889],[85306,95704],[71200,80621],[16977,56870],[73375,75641],[63862,75444],[75307,97741],[7843,48915],[95708,97524],[8684,63351],[43883,55685],[74578,78991],[77135,77919],[7620,71891],[86420,91954],[29515,50927],[82517,93862],[33060,58048],[42800,62171],[74113,97483],[88359,96550],[43567,73297],[94162,98105],[75899,92369],[69628,86425],[56061,74879],[85188,90107],[65969,88418],[95271,98206],[4112,5990],[52255,76998],[171,18188],[78174,90429],[85746,88848],[57715,74254],[84292,87066],[12610,62147],[42200,47082],[95525,95591],[24694,38795],[14834,97708],[91790,99487],[31843,75368],[5761,8575],[68216,85269],[15111,22088],[99972,99991],[26358,93454],[61521,61770],[28159,41406],[14775,54289],[77535,96208],[82739,98217],[84792,90424],[60604,82473],[83932,91422],[37382,46391],[49017,97274],[16315,96421],[44683,90009],[43233,73598],[5822,64872],[44733,97609],[49264,60834],[22844,67384],[1155,48938],[94473,96056],[86544,86861],[17523,65216],[71726,72790],[68996,96900],[4135,14948],[28804,94260],[64368,86885],[20822,25089],[29009,68265],[15951,57941],[15017,25922],[92583,92805],[71737,78339],[40541,93084],[59661,70408],[90757,98102],[88514,98635],[95624,98809],[68291,82389],[48897,91676],[70026,94178],[79383,97902],[30311,65953],[91265,96631],[46496,47469],[20777,70367],[14816,58896],[62126,73024],[84468,97288],[57075,74488],[43820,70160],[99337,99424],[68463,94552],[4699,40164],[60127,90144],[98158,99613],[61588,90014],[92143,99172],[99660,99678],[73447,85307],[79834,96103],[16009,81432],[10187,12590],[9615,11781],[4250,31678],[80678,87554],[15053,46748],[731,23810],[32027,79326],[36374,46260],[46993,70988],[60348,99016],[48581,67140],[2828,8575],[61423,74079],[84315,86466],[88418,99598],[30780,42438],[28195,46963],[84947,90223],[10299,76754],[51553,72431],[56541,77291],[73808,81726],[55770,67671],[96491,99037],[4343,70966],[73760,85520],[5007,52011],[99426,99546],[82542,95791],[37015,71528],[64814,78688],[20167,32196],[78144,78312],[34270,50449],[15863,26114],[31973,40068],[35834,92605],[5441,76986],[78461,86086],[39462,69314],[89673,91446],[68784,86375],[77226,94493],[40052,40826],[78643,94298],[7342,76257],[33408,57049],[25382,70970],[73951,93637],[96952,97209],[93403,94707],[5716,33310],[78180,99087],[83530,96024],[6068,86022],[14833,74102],[59918,97107],[77459,99648],[35588,95378],[6905,52534],[67418,70984],[39722,90369],[80984,93171],[71939,77678],[88573,89722],[63192,69807],[48935,93644],[76354,92230],[94441,99582],[57692,74890],[24727,32076],[93476,95068],[23353,97360],[31034,94233],[14756,33558],[7235,80900],[52303,67988],[82598,99435],[68636,75723],[56879,77330],[41021,74490],[44546,82826],[64650,95261],[21653,94786],[93593,95303],[13823,34707],[93136,99054],[89879,98282],[15048,50398],[95159,97325],[61949,69807],[34816,36505],[82136,84645],[61109,97553],[72726,88384],[30433,62549],[39358,99658],[81071,95879],[28879,63075],[6039,22006],[42631,46932],[23749,47877],[77727,81028],[38137,70791],[97559,99508],[27761,77106],[52000,58090],[87617,97102],[28254,31989],[18155,64844],[80031,82100],[70378,92288],[33080,41316],[80259,90525],[38411,53674],[16642,96027],[1029,15452],[56740,67008],[9701,39857],[80129,96273],[25947,51874],[25920,61604],[363,76075],[79000,80614],[85883,98521],[99890,99905],[87422,98434],[22091,46289],[84864,99042],[64931,86608],[79642,84695],[18620,58822],[27308,63792],[59117,95954],[5945,85467],[18674,54180],[35076,79685],[62601,71775],[46628,81208],[1325,46523],[76252,98418],[20574,87394],[75703,82382],[73910,93226],[58188,86314],[27723,95283],[12687,95316],[67925,88427],[8958,27728],[26157,41450],[88935,94969],[10946,96067],[77976,85174],[63308,67129],[1351,41564],[97621,99680],[32780,64035],[62596,83601],[98227,98635],[38962,91802],[42057,53062],[68403,77267],[51941,95570],[93566,97077],[24460,40558],[29721,62576],[57470,77315],[8389,18243],[1788,84142],[14068,96946],[99925,99966],[87276,96643],[22700,50057],[66522,70854],[9732,75823],[1110,42009],[6641,73763],[33528,53425],[69902,74997],[1133,17243],[32303,84562],[67783,96327],[60376,96545],[68442,77990],[76073,86390],[55378,69152],[62051,92984],[34087,87823],[50623,70031],[83393,85769],[39662,86546],[79692,99671],[10892,46982],[37994,42591],[39213,91105],[45640,85064],[65505,93013],[31418,40997],[25861,68432],[84302,87856],[30275,42539],[49634,98056],[12821,89765],[70125,74587],[74378,81107],[70043,80993],[66759,82297],[37543,54109],[79563,98022],[74774,90201],[7687,84390],[8598,63885],[47557,97813],[27474,68739],[80080,87722],[51175,64679],[96223,98540],[98273,99126],[77248,90280],[92582,94820],[43870,57908],[17948,81290],[21858,79225],[99411,99523],[7185,56896],[20346,22749],[51804,81516],[12793,72618],[93958,99855],[95053,98354],[42940,61077],[22438,98994],[77280,89995],[83255,93223],[46892,51637],[26952,77323],[25348,30458],[16221,47489],[72613,75622],[82778,97522],[52960,73208],[31926,93899],[1588,21971],[86658,94640],[39232,53533],[87490,94194],[90248,97716],[29096,56887],[47232,89769],[85620,88511],[20881,80297],[20386,57474],[24599,50422],[61867,73815],[96994,98133],[25212,37931],[50966,79858],[56751,95810],[56358,82574],[3809,11108],[14271,76561],[6555,41632],[92364,92377],[40975,82885],[91528,91603],[84336,86383],[69265,95851],[49241,68946],[76624,87359],[51931,75961],[93668,93712],[94650,98697],[84718,88719],[49536,56391],[50690,54826],[53698,60681],[65653,65983],[33459,59776],[54192,83233],[55297,56051],[82540,98653],[78711,87338],[22420,84775],[28070,80516],[21743,63141],[93731,99738],[81973,97735],[95375,99113],[35105,92681],[9056,42819],[23267,90392],[74537,80579],[53450,78462],[868,19217],[23094,76640],[90609,97291],[9191,90198],[72605,82051],[2733,45503],[36350,79170],[58199,98459],[11791,60085],[14289,80014],[33567,88368],[29749,97787],[80741,93300],[26103,31354],[89666,94465],[52711,64063],[79228,86209],[43859,90063],[98935,99913],[32665,47256],[85383,88616],[84396,99709],[28678,42589],[55175,62365],[50823,55731],[36879,64459],[96867,99845],[47552,90145],[3181,65883],[69383,76586],[60899,95599],[65666,93012],[11117,21571],[13394,67934],[79964,86183],[85153,88238],[66745,78428],[47940,66838],[49700,84043],[53891,94956],[44464,96844],[35092,80682],[75137,76087],[2058,88185],[81337,88703],[20107,44999],[38234,58683],[45892,63099],[18414,82863],[97905,99632],[4100,66026],[61556,64712],[1132,11039],[22196,60623],[49845,83449],[82429,94511],[51848,88890],[113,62918],[79668,93255],[294,27693],[66116,86655],[79949,83832],[66055,99653],[20937,64665],[52009,72322],[99620,99814],[11059,63806],[54541,93472],[82174,92696],[55661,76348],[53665,80153],[27467,64804],[42494,53519],[31386,55426],[69033,83439],[9343,85859],[51129,85328],[17731,38359],[26689,44449],[54824,73316],[85563,89106],[13433,23941],[82205,98964],[77035,88557],[7916,86002],[38227,76412],[14,7092],[37592,58059],[82533,90495],[69537,98140],[77134,91023],[80786,94535],[65589,99964],[43696,63702],[12281,83739],[31096,56935],[32466,74695],[86626,99153],[44984,96332],[38143,53162],[50364,99730],[6681,83634],[87204,92209],[20797,40285],[37680,71297],[43752,76684],[20290,42098],[46188,71312],[44275,47710],[33056,58434],[72127,88698],[14456,62113],[55530,96590],[16158,48946],[48963,81346],[62822,69390],[54712,98549],[65596,66681],[50225,72923],[67270,76231],[65985,79163],[28883,66384],[59722,67850],[40073,42110],[34635,43556],[96276,98206],[81374,86698],[47949,78974],[85222,94975],[56273,90421],[5121,74625],[20671,99471],[85429,98994],[12416,52734],[51263,88160],[19229,19424],[88338,99263],[3126,38625],[88107,96552],[50905,98071],[41033,91935],[41210,55396],[86502,99926],[34915,71096],[27657,76722],[85644,94253],[91860,95869],[87644,88651],[55794,81486],[55077,59143],[50963,60406],[32560,66268],[68856,75262],[81226,95615],[16912,39161],[56056,72741],[80101,86979],[68402,72491],[72983,78626],[31594,85966],[28023,96216],[35957,67457],[79894,88422],[13193,40932],[34239,63368],[88350,91744],[63072,88585],[39557,60237],[47725,69186],[16191,97301],[28298,31689],[42858,57585],[34891,63927],[28147,41367],[73949,79291],[87287,98995],[53051,94996],[40172,69699],[48354,84240],[27855,60381],[38009,75488],[95395,96413],[2080,87736],[88895,92170],[58229,64956],[15225,74305],[67458,87873],[79476,91014],[69304,77625],[70219,99463],[96866,98474],[21117,93751],[20143,72651],[42950,52871],[56321,71393],[29372,33782],[60369,65992],[65031,82223],[92470,99325],[68296,90917],[92236,97602],[95955,96711],[81016,96579],[4601,97547],[75467,87290],[77877,83538],[84053,85793],[71424,78850],[59179,96124],[5239,65161],[68339,74767],[61896,76684],[41916,47673],[60791,82130],[52546,57918],[41423,97303],[88553,97137],[43596,87490],[24021,32763],[49926,78924],[42502,45412],[85131,88521],[69809,75168],[8159,76132],[89113,98555],[4030,83593],[74037,76371],[63934,97729],[61194,65117],[98821,99071],[98491,98570],[88271,93209],[92960,94165],[39300,50796],[39339,80705],[77810,94975],[12780,42247],[28090,31723],[3171,4623],[49839,72483],[48568,49565],[62124,95976],[35610,53517],[6523,96333],[58173,97998],[36281,97587],[79076,87045],[89388,91256],[23102,69013],[10499,65778],[94181,96528],[95192,96970],[23213,80166],[42565,42616],[80278,87977],[39187,51639],[84530,97781],[35645,78128],[28283,97082],[15433,99166],[76278,82797],[28937,62986],[31995,79590],[49618,91608],[50709,80781],[73355,92025],[17555,68179],[35212,86105],[83431,91644],[23405,71940],[35238,78581],[50666,61467],[71895,98051],[18621,43690],[87352,93143],[13233,78262],[84249,92695],[80147,95848],[70071,93039],[28183,35753],[29201,39852],[22583,72870],[1918,9824],[90990,99886],[89004,93625],[51735,84970],[64816,74589],[43296,60272],[15319,21061],[82482,89915],[89888,95155],[30536,37012],[11276,39798],[68951,84953],[21046,49326],[93240,99386],[60408,86203],[67829,85627],[15916,54005],[21324,42625],[98680,98842],[47022,61432],[8966,85174],[2170,76822],[51045,92919],[48079,91168],[97145,99997],[23888,49881],[17381,70009],[15179,32827],[11113,20106],[68233,96719],[95406,96391],[43261,58979],[62995,81609],[42892,70402],[75227,84284],[70810,87485],[50919,97844],[61329,90856],[27753,35882],[66975,92444],[19658,97703],[44369,90082],[50467,94949],[15579,44303],[5372,76467],[61101,68700],[74448,86996],[80849,90368],[62329,96008],[12975,69118],[72416,97768],[62118,93450],[88227,89016],[68726,74304],[88903,96372],[76693,82252],[19420,27216],[86677,99197],[91786,95712],[51121,81244],[94783,99227],[85581,86409],[22041,84045],[5185,62192],[77975,85497],[59704,87932],[36698,79291],[90075,93106],[29762,45036],[46634,59383],[18924,75132],[48002,57610],[70416,76535],[66388,66734],[59026,79092],[52006,81436],[20997,21332],[11639,27155],[11319,66667],[13625,77323],[47533,78385],[57985,92401],[44121,79391],[15503,82889],[43491,67132],[13878,62615],[95432,98951],[28761,38873],[92123,99204],[43848,65304],[16204,24481],[73912,91754],[67387,82021],[62814,83154],[44600,89193],[60734,85467],[84490,97248],[50321,86124],[48036,55810],[98669,99148],[92523,94023],[27176,96743],[68803,73321],[48505,57828],[104,19776],[23454,55607],[32713,56812],[29582,48058],[50705,62294],[55606,86504],[41358,75921],[57277,79613],[99269,99622],[30551,76091],[22330,76859],[19405,82683],[72632,73408],[8462,63418],[33267,66542],[45263,85952],[52552,82152],[70873,99928],[24943,45935],[5492,46312],[77995,79098],[68176,91406],[89812,96440],[56527,74360],[38042,61800],[90313,99600],[54882,68363],[29866,75897],[60205,95223],[99496,99935],[4998,87862],[42744,97358],[55352,59325],[82258,92740],[41209,64999],[57032,85507],[35423,76434],[47763,83838],[5731,51044],[3600,93546],[10713,85214],[31342,49933],[98627,99185],[63353,78737],[24265,43307],[26782,65717],[75480,78372],[56699,85652],[74542,98420],[51720,91848],[20621,52204],[53617,91935],[28415,41788],[81179,95957],[36496,70555],[59683,76310],[1491,22952],[95798,99386],[52196,70595],[35446,92693],[94250,98787],[93444,96168],[64980,81966],[93009,99873],[17478,33161],[30807,80212],[76867,80401],[35913,65427],[91834,96740],[83126,90176],[14916,68442],[62361,95752],[43238,63457],[18542,53165],[42916,62140],[72453,73390],[71341,90658],[32396,39493],[66986,74172],[47085,71505],[59091,95023],[71384,86592],[17806,30908],[62421,81965],[19278,93255],[86265,86591],[56673,88973],[93150,94969],[90647,94826],[48356,84039],[65540,93098],[20535,24695],[13401,93885],[56914,66868],[43181,74895],[81124,95885],[52498,84931],[40498,89829],[90383,97131],[83058,99069],[65740,90168],[20371,45449],[47666,69692],[30501,46841],[62303,89957],[28534,62185],[99613,99693],[80152,92387],[49663,78031],[93856,93968],[60959,66754],[72996,92789],[31475,78732],[83498,84511],[91980,99459],[9481,22513],[77298,79089],[7737,59256],[5055,33073],[44029,51325],[87087,89339],[74736,78680],[37933,61616],[72593,97905],[11339,58028],[28946,87613],[14531,29388],[59523,62426],[45667,64760],[88561,91020],[3269,71861],[39582,57313],[65595,68608],[91102,93049],[18241,66553],[58707,74445],[28331,73518],[56954,90265],[66869,69723],[5983,15523],[39731,52963],[46058,62156],[37730,73411],[33847,52084],[59480,83861],[21135,96804],[8477,13983],[3583,86531],[71560,83182],[12791,50745],[64929,75387],[78344,86399],[83363,94721],[52248,58742],[9899,50354],[45150,95035],[70849,92499],[43221,87734],[59899,63269],[91242,95064],[33811,58010],[99819,99989],[51195,70913],[46243,84400],[51697,64981],[39379,99522],[22595,64298],[65396,65680],[94563,96006],[27369,57018],[32247,88016],[34978,85859],[82022,85256],[36300,40403],[54203,68241],[27422,71056],[70536,94225],[56820,92449],[44408,98658],[35015,99886],[52473,76730],[49163,55057],[18735,30566],[35616,56146],[86823,92809],[88977,93587],[51660,81895],[54654,78018],[68630,90946],[2399,38745],[51314,98912],[94127,99689],[93573,94040],[5053,58641],[86196,90800],[47120,86402],[73000,89446],[12471,90164],[96038,97717],[48883,52391],[68834,92850],[69100,87956],[39411,93232],[42412,65410],[7618,29458],[72604,82537],[33798,85260],[79071,93177],[72456,86184],[52149,96787],[69254,95551],[31090,47733],[39346,93820],[36466,54695],[67720,95470],[61245,93990],[45400,61962],[69636,70046],[61313,87179],[88181,90392],[39201,51705],[41538,67677],[74708,83874],[25942,32648],[79765,83954],[91709,99397],[78710,94680],[19606,22474],[13403,17588],[69000,86937],[67453,69073],[54517,85802],[27965,85197],[36812,89437],[19994,31358],[83572,92849],[73290,87528],[47300,68705],[84427,97117],[39180,45687],[73824,99247],[72661,79676],[4636,93802],[22925,30390],[81317,82789],[32937,74374],[83708,95450],[75803,94935],[76498,79443],[79596,93897],[62067,62699],[11876,43906],[96213,96527],[33890,52976],[54342,84611],[94153,96205],[49719,59228],[92682,96194],[24295,40144],[36344,53973],[65739,90115],[1019,53415],[73038,95521],[81370,92303],[84108,89003],[26354,80976],[35557,43708],[21025,31441],[41919,93953],[66795,94177],[7569,10071],[2371,31223],[19349,47767],[30420,83906],[39693,89311],[94976,96693],[25011,66815],[41949,79052],[35825,51283],[30973,35021],[63919,89609],[61973,89971],[51048,61236],[6836,77225],[50698,61792],[14696,50411],[86368,93039],[18196,25342],[6802,62093],[72137,85227],[25461,41953],[99,63102],[14075,17297],[77910,83256],[67494,85760],[75401,88520],[50037,51231],[51703,88078],[91252,91518],[14953,50471],[51766,60255],[12050,87943],[27579,74967],[58269,63275],[53533,69739],[56692,66890],[65193,85950],[84337,91214],[46934,80597],[37161,42387],[12546,23793],[93510,93975],[5409,8598],[2403,25475],[3310,9478],[77620,85477],[87956,96395],[96731,96828],[7462,7708],[5872,25445],[60654,81851],[409,34283],[74031,85917],[48377,83126],[22603,28053],[21802,40604],[77629,94241],[26964,72069],[97630,99420],[82848,90585],[77983,83205],[2698,4353],[46715,66020],[58509,86315],[5253,25669],[61850,73099],[10044,72359],[2937,27096],[72285,87830],[37112,87907],[90262,98574],[24722,44789],[41174,50172],[46225,72941],[28182,49262],[25265,48680],[24737,47891],[20871,52967],[21160,66798],[12723,75390],[18868,19049],[52605,83370],[76826,94226],[67900,90152],[59626,91664],[91823,93208],[24963,93912],[48044,70989],[8971,16965],[8103,58942],[35288,41745],[66732,97679],[21522,63229],[69350,75526],[87283,99910],[70106,93371],[82846,98572],[28426,97227],[75709,91893],[77859,91657],[17944,94153],[11390,45232],[22683,60927],[10894,36991],[22114,51885],[55756,64189],[2560,57710],[35677,49599],[77791,80116],[75035,90190],[23399,52433],[81509,96584],[17934,66435],[36268,37666],[37905,70665],[49485,78407],[81642,96809],[6445,28750],[82299,86349],[23869,80442],[67213,88006],[40225,66349],[49078,86072],[53245,68458],[28541,89094],[98731,99535],[96824,99438],[99455,99928],[27067,67401],[4780,88031],[65712,78722],[5078,14337],[31088,34683],[54324,60176],[51590,78488],[1815,29475],[73906,99690],[10811,33593],[9419,94922],[15678,80646],[97890,98327],[38601,77529],[68098,99386],[96313,98423],[42172,96881],[6171,65658],[40979,61599],[91549,92597],[42879,97844],[6095,36816],[45884,52546],[56555,97168],[69182,95905],[65275,98557],[3196,47570],[86506,92356],[67869,87382],[17904,33453],[28169,40625],[73638,77022],[68195,92284],[95690,97185],[95224,99811],[87786,89317],[27831,40205],[86142,89297],[7753,91462],[17958,39591],[98789,99036],[70455,86280],[56162,71807],[47076,63439],[11281,72812],[7492,58247],[51465,93194],[43647,79542],[56344,57191],[54807,69479],[65932,66760],[60134,77106],[41066,41081],[28806,56241],[79133,80437],[54129,64512],[93918,98338],[43847,87640],[28740,72216],[88137,97143],[25195,87251],[56455,58573],[77771,89566],[24652,68929],[30524,97693],[2633,46802],[39851,96176],[27479,69425],[4320,56329],[14794,71169],[25157,75108],[92159,98264],[8976,58092],[82850,85701],[26641,57853],[78572,91651],[33826,53578],[58233,89625],[25522,73870],[52961,61219],[98241,98672],[53216,73943],[75991,92666],[91436,98436],[87408,98000],[25971,56101],[54130,63473],[74816,99112],[15211,68361],[69866,92805],[42346,61942],[85771,92763],[54411,98412],[6066,76050],[26123,95606],[12864,85242],[9517,79553],[29107,60794],[78645,84635],[61903,73680],[62290,76672],[74271,78443],[92713,95567],[62711,84600],[55402,84287],[93288,98605],[5051,83513],[54275,81624],[74094,83860],[8494,75301],[72468,96345],[76439,90428],[40331,94046],[81210,96926],[25845,84839],[38742,67343],[8766,41554],[12353,83618],[29343,96305],[21301,65931],[70257,84723],[75493,79856],[77755,78096],[83644,97720],[1501,31473],[1417,25333],[72895,82174],[44314,83435],[92007,97368],[58613,92626],[55582,93379],[2069,95391],[1342,32271],[74920,78529],[70079,90427],[54675,57950],[36965,84605],[630,40309],[39016,59527],[86958,97626],[14101,98330],[57712,99892],[7176,73221],[39623,63686],[3459,15550],[16470,61779],[57045,71689],[14398,81169],[37722,52539],[71497,72664],[70757,81530],[33991,70659],[861,28530],[63857,74923],[87398,96316],[39069,82182],[17310,56933],[48773,60881],[77063,89928],[60189,63212],[5935,33188],[188,45401],[16490,61123],[88824,96164],[55279,77627],[96622,96935],[64883,87210],[90717,96800],[63302,65923],[64417,97670],[29888,68194],[99635,99892],[86164,98900],[10097,16610],[98028,98289],[21717,92833],[73314,83969],[78526,97765],[55191,70168],[97708,99189],[38583,44117],[5862,47665],[6165,54823],[91321,93002],[80578,86234],[36992,66672],[86600,92533],[56671,72797],[90353,93886],[76928,78751],[27732,29844],[33917,85667],[92976,93204],[64054,64568],[37452,54786],[17693,28198],[49548,81563],[17099,93544],[34827,36847],[35496,36511],[69804,70936],[20982,88027],[62153,85957],[5189,64491],[22020,28254],[77224,93039],[58318,70975],[11662,43203],[18180,87013],[12936,50258],[30634,95254],[59216,66894],[11598,57071],[43923,76670],[95489,99700],[44248,92422],[36227,92263],[20690,80388],[23048,98497],[78257,83778],[7105,44735],[51628,89377],[87500,93736],[58895,93871],[84769,94510],[39393,76403],[79266,87811],[38376,80048],[59425,61305],[11461,94344],[30346,33527],[29796,74804],[20907,87393],[88443,91805],[87860,96975],[3901,80001],[40391,58751],[59739,74797],[35775,43176],[20495,73644],[36408,73046],[87495,89071],[95447,96910],[47152,79822],[68603,69241],[92761,93892],[24441,74522],[12348,31327],[94300,96948],[82665,95868],[96202,96539],[22674,52808],[12105,22263],[73744,78138],[8188,52526],[20367,61721],[46478,76149],[936,97413],[56425,60315],[17660,88922],[7626,18818],[40101,72628],[70727,99839],[72475,75470],[21463,76038],[1126,83753],[1524,73737],[73231,73309],[34954,78719],[40155,82008],[77651,90082],[48569,71087],[16942,31304],[24272,61544],[5902,75809],[13052,45679],[71607,78044],[60237,85640],[1727,99822],[90162,98419],[70643,93473],[20461,23699],[44242,52070],[11073,92203],[69786,73173],[53194,96886],[30932,34607],[839,89332],[61284,98616],[22417,37955],[41920,49035],[48748,64933],[37354,85681],[59755,72068],[63983,79346],[5075,72993],[85844,98054],[50864,77397],[45652,53944],[10580,21232],[47726,61194],[40370,83909],[5617,29730],[91492,99729],[27391,95190],[10854,78334],[45835,47358],[67767,90821],[91131,96484],[15812,54911],[79190,83963],[26708,27496],[35679,37750],[57809,86077],[18764,86661],[30562,40756],[82576,91912],[90289,91089],[81876,92959],[27278,62331],[28976,46014],[75869,78257],[14632,86999],[39133,81173],[91069,92253],[30620,33514],[13588,85008],[1220,29030],[46913,75795],[44850,74308],[95570,99396],[69197,98419],[31948,77991],[80619,85748],[97896,98425],[25222,62555],[30503,69046],[8816,45817],[2902,4727],[10003,78382],[15309,47641],[24379,45062],[51086,80342],[26084,66294],[31372,79463],[29408,63578],[6507,95630],[74732,80488],[83933,96752],[17091,88497],[83118,89544],[22706,23808],[39893,78563],[63621,91512],[68372,81034],[70403,88603],[17691,41030],[36765,47035],[81919,83000],[95452,99498],[7163,78363],[66182,92107],[91932,92073],[39202,45999],[35069,77602],[37158,50420],[76648,84657],[82551,87235],[58726,95871],[2043,63172],[64307,76900],[38542,63896],[43278,82063],[29177,45714],[11270,35115],[61218,93985],[48240,61660],[46723,49387],[44307,73918],[94376,98335],[96090,98258],[82929,84449],[74153,90924],[38701,45539],[36217,95083],[48583,91297],[11570,43445],[3118,88034],[15797,56597],[31425,60678],[84926,90030],[34529,57302],[81140,88443],[98486,99281],[53867,61578],[51994,64129],[32691,48743],[80977,87320],[77865,96333],[1563,39280],[64908,96710],[64354,92640],[66011,70859],[7529,13656],[43210,68782],[99243,99496],[38018,54612],[77913,90497],[42039,75871],[24255,57627],[48043,92390],[95830,98069],[43143,50241],[43347,52954],[98995,99282],[23909,96997],[55479,88185],[5542,89731],[68990,75005],[2754,49664],[42689,45338],[12552,42772],[89434,96880],[14235,51549],[41554,61095],[72751,75814],[57927,75041],[43580,48315],[62724,67850],[81487,97466],[8119,94350],[51644,92529],[8140,69092],[28460,58541],[14092,64127],[23677,54053],[98049,98340],[85378,97368],[49541,85513],[34726,90514],[89734,93171],[69053,77688],[39701,61503],[22953,43738],[12938,87115],[44749,63939],[26919,78047],[41613,54510],[32788,46529],[4767,75567],[22430,88217],[98814,99229],[70489,85131],[11285,22568],[9661,29381],[80301,98085],[3547,75862],[3211,31433],[948,28002],[5343,93191],[45182,61979],[14736,36248],[34913,45878],[1252,5291],[2321,22808],[35398,99408],[27370,54745],[52260,87356],[58016,83124],[74918,84167],[64423,77309],[85107,90020],[92663,93185],[51626,74114],[72421,98407],[66167,68559],[28595,93277],[56854,67985],[82604,84386],[27185,81420],[8600,63440],[28201,81078],[51933,96025],[11588,79748],[44467,68144],[19023,19839],[77806,93313],[81841,94423],[69141,80947],[84930,94164],[59028,93890],[58508,95418],[73505,77119],[28105,66318],[29954,47461],[79342,83666],[55831,96447],[90131,94455],[48102,74899],[13534,39943],[47480,92363],[60082,77469],[27128,72089],[89824,98370],[77861,88320],[65551,84928],[41403,54476],[65799,93018],[59372,96515],[48758,73851],[69001,86936],[20124,99002],[64707,66016],[3403,80870],[83031,85989],[75114,87001],[45896,46823],[12333,98409],[98927,99049],[26517,78502],[66048,67191],[16667,82116],[39500,73789],[31982,37413],[53555,86342],[24810,64635],[4459,59563],[66686,69580],[64241,73241],[2279,92808],[80217,95348],[935,49518],[5465,62920],[64484,93016],[32692,64014],[99995,99999],[41657,62856],[86617,89242],[89700,90401],[74607,84571],[8011,42831],[82920,83406],[9001,13122],[35356,46015],[47385,57623],[87224,87375],[92370,99905],[15257,64714],[96321,99568],[7714,25270],[88004,90766],[47218,69357],[79024,86762],[44332,79944],[42951,80693],[32169,96660],[12101,62268],[964,33761],[48370,54458],[45773,66422],[78799,99851],[91691,98634],[60469,96908],[94247,96832],[16962,82848],[48599,73687],[33053,35499],[5140,33028],[8092,22892],[65313,98598],[47020,90689],[74495,99073],[47042,66124],[81526,88322],[91103,95806],[48740,64436],[66677,68037],[47648,70869],[39898,77631],[59058,87971],[45134,48662],[26127,56518],[44844,56945],[11147,74443],[33314,55783],[1395,97900],[84467,89871],[88025,89982],[8490,12018],[60479,88477],[82683,82790],[81870,82412],[97835,98755],[77986,81052],[33932,75125],[2102,92805],[60579,67908],[35743,48873],[23582,80432],[47065,98104],[2138,45190],[24085,68345],[92203,98083],[50439,86826],[51731,59366],[343,4211],[91241,93447],[98615,98838],[27147,64344],[79889,96675],[51457,88017],[49352,96624],[87789,99703],[37494,90102],[64556,67757],[99912,99991],[93149,99784],[59420,82677],[40413,79375],[33172,45582],[25394,86709],[59400,69621],[53835,56539],[92662,93490],[79171,90426],[10243,48033],[22958,87154],[99675,99914],[65271,81732],[79743,79921],[84384,91652],[29946,73163],[91742,98581],[58593,97831],[74898,96444],[39883,85381],[97072,98236],[50718,81726],[5183,25465],[99566,99872],[48065,98555],[97492,98167],[84719,98122],[25960,33769],[18563,42613],[45729,54040],[83130,90750],[82183,95038],[39033,80036],[38397,65910],[68494,80337],[79439,85917],[29012,54752],[62045,86310],[80548,94696],[76637,79817],[23439,23827],[5144,87421],[54711,94965],[67141,84709],[56600,81903],[37953,45031],[89351,90629],[61899,96267],[40950,85207],[62968,96045],[74574,75917],[6368,53673],[12958,43107],[64059,78790],[236,34894],[19377,33235],[94030,99653],[98659,99912],[83812,95720],[56264,60988],[93545,97368],[31571,64430],[43237,73256],[1528,63930],[60468,63007],[41521,62329],[10150,72737],[81780,89663],[73057,92278],[48799,64025],[50,36360],[92701,96608],[10778,32419],[76209,87926],[48822,83337],[66100,93896],[55268,86529],[15621,25522],[87468,95417],[80464,83408],[86202,89823],[89292,90796],[28046,71520],[32080,76884],[65043,93735],[50980,83168],[73710,77529],[1553,6581],[75127,86676],[99428,99568],[15313,70987],[42926,52749],[71420,98542],[12528,85139],[20703,77055],[21994,95619],[25585,26202],[14278,53135],[13621,81774],[50785,56269],[79067,82069],[67365,85317],[95702,96545],[94916,95853],[10604,73960],[85658,87704],[23647,84584],[13072,36950],[45513,52906],[44083,69309],[70040,73235],[92614,97867],[66504,79929],[27016,51464],[29792,89511],[69093,84095],[26590,87884],[41884,89641],[87814,88272],[15832,89421],[52878,53071],[28273,56276],[70629,89437],[67722,93221],[63530,83427],[90989,94508],[21604,74943],[78544,93919],[58733,60715],[94957,97212],[54792,61116],[33113,59333],[58380,62939],[81299,85123],[75098,81592],[35615,70184],[35797,42352],[27882,44959],[68980,98634],[22294,23820],[21065,46788],[48578,82675],[62335,91688],[67981,80445],[68790,74203],[96832,98804],[79242,94835],[80670,94163],[50736,80712],[23007,30420],[71980,74418],[1091,99132],[27772,83820],[56152,86694],[79299,95458],[75496,99881],[4160,74471],[23068,65647],[80549,81333],[30513,51860],[24786,75331],[58473,72762],[36392,75634],[49321,51313],[24762,25130],[41753,71463],[8028,71913],[93157,93499],[91126,94472],[79122,92146],[38713,69030],[47743,68270],[56468,86804],[50475,53315],[36346,97226],[69055,91347],[13095,45370],[43671,58532],[17260,58784],[58215,75937],[47532,66146],[72133,74177],[615,13270],[38442,75828],[68367,79036],[93653,95125],[22617,87952],[57266,76650],[38545,71785],[34732,67175],[82779,90198],[58620,88371],[82204,90597],[56768,89213],[81379,98453],[39319,45703],[92015,92386],[81478,96072],[43804,78229],[62639,93021],[33282,99215],[87667,96971],[87714,88837],[99934,99966],[19682,35576],[73020,97927],[68471,80432],[77753,94587],[11389,50568],[79705,94622],[70541,99897],[27393,97071],[29430,48204],[37166,40683],[12510,71334],[74784,94907],[94542,96519],[90526,98171],[46348,65789],[80442,97238],[71054,75645],[42903,43711],[81524,98423],[38750,93505],[85816,93722],[89235,90325],[23508,95230],[44090,49457],[2651,53944],[92137,99971],[51975,62534],[63074,77787],[22840,80601],[25190,58957],[58642,70242],[43121,50925],[87218,89010],[46248,54948],[71091,72101],[86762,99733],[19841,52466],[14490,24601],[28710,72021],[36919,39481],[42611,90213],[92644,95231],[93570,93989],[87010,91388],[11138,73435],[17852,64132],[83621,96784],[86385,96582],[54234,86047],[86679,89640],[87315,90731],[52221,80834],[73166,80112],[76834,87728],[33682,50501],[88070,92994],[80051,85179],[60861,91819],[99731,99836],[18062,39677],[87742,99641],[98242,98832],[29874,77358],[20158,43869],[91016,98613],[22642,47301],[30299,69841],[72465,95093],[94722,96125],[79848,91097],[79109,85350],[24764,94610],[26897,95208],[76146,94945],[89077,96248],[42466,76450],[95857,96491],[29150,43585],[71793,82274],[91488,92844],[21767,92668],[39068,98532],[36845,79269],[42504,64360],[3991,77348],[28903,64536],[51524,67513],[29317,70027],[87231,90999],[36659,42000],[38679,85435],[80347,88154],[70922,86383],[67393,87922],[71242,91180],[57009,93280],[22652,60132],[93387,93898],[9000,45805],[91922,95625],[70557,88846],[50435,57175],[29920,63921],[54605,87098],[74812,86524],[74806,86918],[27275,68618],[23568,38056],[70651,96770],[27857,63282],[50850,68361],[584,90174],[52201,71868],[75584,79856],[71170,87968],[63719,95374],[50322,67070],[673,7501],[48962,52469],[66184,93489],[71082,99613],[80658,96023],[89797,99406],[31241,79739],[74807,75418],[63337,96675],[34013,90962],[65078,97859],[17158,49479],[95098,97709],[69385,74246],[5516,42628],[68498,79749],[57549,83318],[88636,99172],[57796,68681],[12261,20884],[49550,49789],[42922,52911],[31943,35746],[9408,70080],[25199,98018],[36246,51932],[83235,85795],[5251,53269],[62742,77521],[96815,97399],[57783,94227],[57373,59552],[89850,97763],[66006,76625],[29291,68178],[67166,94119],[40580,55481],[91027,97303],[41585,44164],[84247,96248],[93904,99236],[91493,94630],[87030,91395],[62239,71529],[55901,72524],[8929,69147],[88823,96389],[41952,56560],[78275,83315],[3647,21085],[67078,91475],[68634,70537],[91132,97152],[73132,92931],[26745,42179],[18678,65301],[4489,63219],[57202,58869],[61111,69285],[79726,92011],[29298,64659],[92389,92645],[11878,94188],[65971,66062],[93021,95201],[12654,23393],[13112,83351],[78312,98628],[9723,68480],[83978,85331],[45176,73201],[26886,49340],[78716,79163],[53963,70127],[50701,80041],[67757,79738],[8091,37419],[25941,59334],[95608,96845],[84855,92166],[89459,96415],[32931,61605],[57129,86805],[77746,86162],[77429,79543],[52544,90262],[3046,51003],[9097,64628],[96211,96350],[67914,84515],[30574,48798],[29233,57246],[4815,24741],[19883,50882],[70140,92580],[92829,97020],[85468,97365],[98963,99344],[36489,77219],[27087,61033],[82987,93215],[56591,59651],[72274,89886],[99677,99715],[9831,57343],[63427,84866],[62709,67889],[39163,80690],[51935,86325],[42976,44222],[72690,92580],[36716,90334],[10867,54832],[35278,59054],[64022,76336],[58622,65493],[41230,81937],[22224,88897],[28216,93941],[68121,85934],[9385,88288],[60740,72905],[60762,84594],[34854,37452],[53967,73577],[24478,36109],[45289,75198],[56491,88151],[5838,94719],[15409,18847],[52913,58968],[43872,84828],[56493,94718],[33153,45378],[59099,96266],[85008,97373],[47875,90557],[15715,48872],[12391,37229],[62248,92824],[53727,84752],[22647,41229],[35574,84173],[42006,42867],[69978,87532],[1040,53465],[82176,87166],[10410,96506],[30788,73836],[38204,87057],[49647,66374],[41070,92894],[24373,59423],[31637,98086],[68152,71002],[5738,30872],[39919,83009],[66401,95150],[65749,89496],[31700,81551],[77618,94992],[98246,99438],[87781,95109],[79043,81096],[62055,78567],[79331,82194],[31574,85361],[76311,81092],[22176,35331],[11239,59137],[67666,96950],[80438,86001],[80005,86100],[24948,49456],[98722,99267],[61761,69667],[26499,30685],[2799,91355],[76843,86832],[17581,72254],[15430,47921],[30733,49563],[56287,85136],[14202,43621],[12403,13547],[74919,75957],[76890,80266],[96016,98388],[52435,70594],[99788,99957],[42204,82086],[7481,55877],[54382,61921],[67797,72085],[67054,91858],[60339,99543],[22483,79955],[61847,90423],[75457,98714],[59375,69784],[93314,95950],[4939,32837],[47853,95388],[91981,98828],[11839,30000],[83083,98008],[82545,85292],[47046,58024],[51150,60634],[57882,84681],[65665,66640],[77638,83976],[59328,78545],[62258,84957],[67140,95911],[6634,67074],[8709,95270],[36807,97141],[46491,54785],[94606,98843],[97136,99900],[99870,99974],[15664,42073],[53850,64156],[41933,75545],[48828,70642],[17137,42466],[30895,60260],[47662,94659],[73295,85178],[27202,89418],[27136,82355],[61913,79220],[84044,93835],[6532,90776],[43502,77484],[35060,70152],[14086,41180],[56881,76896],[7174,52761],[19493,80278],[33585,92266],[73013,81693],[34657,49917],[43876,45734],[99159,99929],[17640,65050],[39968,42550],[24876,74483],[73163,75915],[55814,98620],[45868,88705],[60033,65128],[45639,66467],[88367,99451],[14610,49054],[96545,98147],[59668,82838],[17615,99489],[96081,97436],[62871,63671],[48953,77806],[29935,46703],[70620,74565],[8378,90822],[99763,99958],[55993,87725],[58592,62439],[33794,51857],[41025,95041],[24426,57114],[44302,67655],[71845,74379],[52791,59428],[40402,53583],[20128,29844],[79824,96410],[13259,74363],[14851,71078],[28476,98617],[51866,55554],[24471,95458],[80057,88916],[38119,93561],[68361,72017],[55769,99685],[50614,88916],[27765,55912],[46972,78469],[61721,86741],[20522,70612],[90156,90548],[1221,99926],[71101,72513],[26588,54090],[7516,91724],[79807,95749],[13050,36412],[10519,76321],[18073,33757],[81260,85109],[97567,99159],[51373,95466],[46158,88968],[58172,72753],[25159,76869],[15879,70771],[11267,75013],[60600,65701],[43395,48625],[26626,30556],[2373,98603],[11002,82119],[62322,96825],[52370,54865],[14712,56195],[89300,91176],[8836,67795],[54957,59315],[3759,42497],[24109,79378],[35232,50647],[99282,99762],[74451,80844],[68583,85146],[47100,58637],[71649,91983],[88600,94677],[70305,94827],[13527,83081],[46351,90173],[49588,98593],[15964,55482],[54743,68508],[2191,28382],[24333,60890],[92538,95680],[40000,56621],[46249,89259],[94392,94458],[70895,72330],[65718,74310],[45247,80457],[90643,95765],[5922,30144],[58763,98403],[60629,77938],[22056,66743],[27005,27422],[899,1522],[7331,89103],[52374,90982],[11307,16336],[90750,96385],[46608,80293],[35079,76661],[50305,69647],[1191,99719],[46473,99486],[45052,85249],[14203,29375],[97168,98468],[21736,46053],[22135,98354],[26019,57385],[3334,53427],[33994,77547],[59716,95334],[94448,99067],[32044,69554],[67116,68577],[34745,50936],[73067,80640],[96248,98091],[92494,97880],[45741,77382],[26815,86376],[14233,94630],[18465,55006],[10988,58997],[86467,86623],[14855,21984],[13941,35583],[81095,86730],[30154,70926],[85840,87817],[64781,83067],[50739,76742],[41759,56044],[56709,94624],[85898,93804],[48647,82887],[36122,41178],[34479,35019],[59066,84516],[95759,96366],[83254,88095],[13474,51446],[14595,48859],[82926,87908],[43223,70513],[86103,90836],[72038,88964],[93979,96243],[37641,84991],[16342,80689],[25646,80331],[83279,97333],[72700,92162],[1514,28682],[46627,63017],[92505,98545],[69161,99412],[45838,81226],[17030,21490],[80934,92408],[30544,57257],[70224,93479],[88280,92262],[46668,81804],[74640,79490],[15227,34378],[5325,67131],[20594,77595],[10982,20171],[85975,91859],[71373,91812],[56896,97951],[8869,14334],[15373,18678],[12010,99541],[49529,60428],[91204,99769],[68944,88819],[31828,33657],[62705,68143],[13943,86980],[46467,65360],[72919,99806],[8717,75937],[70599,83082],[28951,74851],[79617,92557],[25423,76741],[92885,99828],[34977,80928],[51152,64388],[14484,58811],[4535,83212],[42654,81313],[70214,77286],[16311,45896],[91426,92151],[86416,92507],[88253,90222],[59726,83215],[56081,66055],[46465,61525],[72554,88157],[24458,36568],[59267,84377],[66351,88669],[4493,39040],[77980,90517],[56686,64171],[71062,82167],[14395,80948],[36800,64708],[91341,99615],[52644,53647],[48517,79447],[81688,82740],[73543,87680],[63168,65946],[44145,85221],[39910,66754],[48838,75912],[29592,33057],[3100,53317],[10632,22776],[132,59055],[60564,70977],[37285,97153],[24317,26726],[81356,97686],[49681,52024],[69151,72469],[14533,79855],[54509,94229],[94952,99724],[74019,78733],[60351,84463],[94972,97159],[59302,86790],[35793,69619],[5932,44877],[37569,47368],[7549,90215],[62034,67351],[45070,57202],[71515,99122],[27262,43663],[92511,99270],[2666,27084],[46124,72770],[74632,91218],[32343,52209],[30937,76506],[93875,97123],[71976,93022],[78125,91828],[62526,89267],[18927,77702],[57460,58423],[17168,98387],[6561,97097],[44998,65063],[22528,86361],[23019,34209],[29128,47362],[51600,76173],[11938,30293],[78390,87618],[61212,79991],[73513,95310],[98971,99926],[4340,46684],[66523,73646],[32804,35441],[75950,78452],[1908,51893],[10450,50970],[87362,87429],[19546,45525],[34232,49168],[58443,93133],[59790,85710],[94381,95936],[55313,79304],[63620,87254],[52153,99119],[30398,31107],[12693,44552],[53697,75742],[74733,82903],[81638,89874],[38086,54871],[86761,93650],[19626,42640],[65434,89337],[16970,85041],[20967,31547],[40790,92377],[8049,68719],[23896,39516],[12778,15767],[12571,86093],[93242,97290],[11857,26646],[92658,93537],[85255,97994],[8244,25840],[57965,66804],[96572,97655],[1328,44942],[31229,88005],[93922,99560],[28228,33262],[28001,38541],[77328,96823],[61120,88260],[60110,97873],[3670,71943],[6008,23420],[52139,96323],[60878,67459],[61580,90962],[37650,75334],[45021,93150],[45478,86873],[60337,67138],[95944,99184],[77356,94885],[44258,88993],[39924,59973],[25562,84639],[64415,70517],[6448,58857],[54392,58411],[18495,34271],[60167,85972],[90919,91869],[3615,55254],[87576,95103],[6331,7271],[10907,78970],[77614,77828],[96974,99591],[35608,47912],[25807,45309],[38279,39247],[86102,93102],[35217,85214],[26731,65926],[8680,52875],[70780,93542],[59680,88604],[20613,93218],[67711,73155],[41247,48139],[92769,95120],[40368,76600],[72560,94227],[53498,60650],[94895,98440],[95709,97672],[2749,67088],[43927,52157],[20767,99999],[72882,73087],[65261,96075],[85057,89490],[64199,77885],[24250,72105],[13668,23177],[7683,88513],[38970,73347],[45827,50845],[99878,99979],[12249,29946],[33664,55919],[27812,62034],[15458,38956],[89525,91692],[33828,87120],[80951,86853],[19092,97699],[8765,71668],[14882,21440],[86133,94195],[65217,91799],[79812,84842],[79722,95526],[6999,30470],[922,26757],[17846,31917],[72216,86877],[85438,92080],[18854,44332],[27521,88809],[16172,68559],[12762,24919],[289,32821],[4870,11656],[88318,98472],[22999,95228],[32001,34204],[55623,75682],[96035,97778],[93313,98007],[96913,98515],[48922,93671],[96552,99064],[16891,69537],[28284,33489],[87836,99464],[71413,72693],[76861,95970],[3542,71404],[17028,78960],[39644,94939],[32039,41198],[25822,43914],[85347,95805],[65810,71000],[44247,55671],[52887,96151],[83942,99556],[91948,94117],[51165,78317],[16820,23875],[18210,89134],[87415,92019],[73448,78863],[97384,97685],[1087,60497],[64677,67384],[89517,95939],[98811,99297],[76327,84874],[99182,99277],[40739,65205],[63294,92077],[18227,89624],[44676,52673],[39211,78822],[38450,58861],[57923,66066],[1079,34769],[84163,94863],[45659,68272],[4274,43149],[10636,64237],[38991,66741],[25480,71568],[96833,99111],[19178,91939],[21742,97580],[29084,52610],[87936,89066],[63304,76155],[63993,74340],[19200,35599],[65134,70615],[14703,28269],[27919,32615],[90830,99341],[25245,61636],[80734,90641],[32635,35175],[2527,82093],[15756,60509],[82819,98194],[32505,76700],[28337,99223],[68656,88900],[84624,94798],[9465,90381],[15162,35614],[62955,64093],[88219,95804],[79217,97692],[57974,60984],[78513,91200],[44279,70672],[72627,90525],[1541,39472],[59015,80999],[67184,99988],[95418,95657],[21729,34931],[46064,60986],[841,18509],[77574,93899],[58066,84115],[34405,96252],[89135,90813],[36704,75097],[41159,74449],[77400,81681],[82761,85628],[60255,76041],[25169,91673],[22363,37078],[590,46113],[36602,78831],[14279,80329],[22055,63269],[37405,90744],[74130,74815],[47807,69000],[74035,93477],[20924,48653],[93888,94819],[56369,72539],[47180,55154],[32236,43272],[70227,74642],[76390,90490],[62978,65822],[40546,44711],[37705,98201],[74726,84004],[32096,32575],[59549,72333],[90984,92675],[6598,75998],[51260,72394],[43972,85557],[33675,91371],[87483,96406],[33366,91352],[70090,84906],[218,11121],[31365,88023],[78137,99940],[72792,79429],[14006,75235],[20476,34293],[76101,78259],[46578,83990],[23141,98626],[81810,85367],[26074,54200],[19514,76780],[19119,71996],[68876,97876],[9345,10300],[40944,93388],[43722,55494],[17295,40180],[51962,60432],[94827,96531],[26401,84913],[8116,15267],[57547,72333],[99806,99891],[12565,56686],[23219,82466],[26328,30284],[64366,91719],[25700,66166],[77988,95220],[57966,88370],[63782,65868],[46060,69248],[7612,42589],[63109,73910],[76160,91246],[57872,71862],[55945,91635],[22133,77512],[72642,90229],[860,17976],[12783,45923],[64663,81246],[61922,79490],[29263,89033],[85972,93242],[9564,26487],[21281,55388],[58378,70990],[58438,79399],[97560,99099],[12502,99143],[18508,36967],[72241,86718],[7530,77925],[74246,89381],[42669,74374],[37084,51858],[1453,53697],[29472,77994],[76939,90191],[68770,68771],[7030,9543],[95530,99130],[93022,96817],[71697,77827],[96812,98028],[78782,91549],[12854,60298],[32630,77996],[72773,91470],[98462,99032],[50910,77075],[97946,99700],[41969,63017],[91721,97477],[550,56818],[44688,57758],[37312,50049],[93737,97897],[18982,24619],[16554,56937],[95630,98805],[4049,40206],[42432,90574],[77796,88746],[96814,99537],[22935,39188],[52058,71721],[87229,98120],[82402,97692],[54460,72451],[54787,98730],[35256,62475],[59326,83610],[79630,82735],[25679,40161],[37381,98734],[29926,93780],[46380,57444],[29511,89164],[54393,85238],[89306,97131],[56368,88265],[26172,65900],[4093,44393],[1897,84507],[95100,99220],[87103,91550],[45490,74954],[2849,42383],[91053,95909],[61499,70442],[94335,98473],[27158,92884],[83965,86906],[34962,64761],[40780,74884],[60336,71660],[37648,61002],[60847,95619],[79091,80891],[56170,83759],[40229,58960],[21089,94177],[40314,55033],[52825,91865],[87340,90324],[55128,91791],[58329,64824],[72930,86493],[30090,70159],[5828,39018],[30230,83311],[40967,67114],[40068,58221],[98270,99162],[67830,88271],[53566,58224],[54272,67892],[21712,74376],[74521,77277],[56521,65076],[90108,97536],[38046,88401],[19464,59479],[81815,98054],[26474,79948],[77812,96209],[71034,91616],[30440,34600],[93089,97424],[86937,88611],[72746,79350],[88830,91632],[61614,81489],[48445,95386],[39775,68530],[7948,11477],[49225,83397],[83883,96652],[75349,84276],[28782,38773],[31830,77766],[88352,94693],[1711,55468],[29254,77231],[51951,84072],[30887,78365],[53988,81673],[13916,60218],[9527,19424],[80119,87874],[81307,94657],[93259,95027],[6572,77611],[80508,84950],[1988,82282],[30429,53521],[20498,80104],[60183,91323],[45819,56837],[55214,63574],[77057,88674],[88885,88936],[71431,77503],[49424,56213],[79489,84172],[83377,83632],[66736,86060],[30977,64349],[99395,99885],[4372,67970],[98228,98737],[64985,73758],[88058,88969],[96862,99337],[593,53561],[79512,90587],[33830,75631],[1635,85724],[66271,92419],[6831,55118],[14856,38388],[52409,72622],[60937,85717],[52083,66104],[95988,97336],[73096,92530],[74723,86457],[13066,71056],[78893,87969],[4024,44195],[33888,41309],[93335,97582],[93042,98943],[2762,26218],[1879,72584],[4331,23992],[15199,60749],[53808,73139],[4234,78722],[94017,99024],[51835,66358],[70825,99164],[98366,99263],[93055,99678],[30891,99509],[25683,36129],[67445,81346],[25975,92954],[65913,92368],[84704,94130],[79717,82999],[8832,98673],[98043,99115],[469,92374],[48387,85935],[15947,23855],[663,48841],[10591,19325],[94410,98827],[53488,92472],[71859,97187],[4892,24171],[10506,94557],[35084,87797],[47825,47929],[97464,99730],[4930,32297],[99297,99788],[6064,38357],[54653,55892],[86634,90710],[32573,51708],[23063,71133],[27528,44206],[91055,91498],[9387,71389],[22354,25800],[32114,45278],[51319,66874],[5283,20340],[67587,97239],[45577,74631],[41643,83431],[59065,99989],[14869,52146],[56314,60475],[36864,96786],[27085,56573],[74719,77526],[72307,92281],[95699,98853],[31258,39166],[62702,84587],[15845,79530],[13862,49871],[25556,45967],[69367,84628],[25923,98793],[41863,58254],[19272,88543],[78564,79611],[26650,62149],[1028,87725],[87986,99725],[17172,59834],[23099,80130],[38890,57562],[94170,94172],[59431,99754],[2760,59201],[35312,72333],[58946,84441],[35742,72529],[21433,32833],[32410,34892],[85176,90627],[60634,77003],[80296,99020],[94411,99365],[75504,78420],[87639,93412],[52249,58457],[31846,32156],[91643,91973],[157,31045],[15967,66957],[292,55149],[23143,57806],[91881,93296],[67506,99443],[43060,90143],[24,23266],[18680,48253],[61474,66859],[61829,99057],[15914,18510],[88483,96685],[38072,98281],[14030,62383],[1451,29509],[10919,36165],[93512,99015],[84149,90733],[41074,72679],[26629,71775],[70456,92863],[77627,91725],[7370,88920],[22068,87692],[8930,63957],[46460,60486],[7124,23422],[93316,97199],[55213,84418],[19570,71892],[40043,57800],[78416,98156],[82995,98932],[92324,99565],[30248,61890],[55365,88702],[43276,68450],[32477,58623],[12648,88397],[49587,77124],[72240,87382],[78679,89070],[99429,99590],[80765,82764],[29830,85432],[38674,39599],[19452,49688],[7499,65363],[43373,61769],[26955,71036],[17565,19397],[22174,36599],[43971,63856],[81935,93371],[78657,88039],[96297,97407],[52974,99619],[58920,92302],[15178,92219],[79357,84266],[71695,87813],[67896,75254],[4848,63848],[44871,79786],[32240,61986],[73037,83943],[23434,35151],[6936,50725],[91358,92872],[20903,43732],[99029,99440],[28484,96655],[29248,52091],[65376,77195],[76449,87814],[11671,90544],[69226,73263],[94888,95444],[35181,83589],[66674,81588],[34086,54790],[84699,98286],[65655,71751],[21765,83133],[45637,74425],[68911,84806],[89253,90368],[57318,57497],[69368,72861],[37966,79907],[28612,89906],[83404,99649],[10119,79735],[59428,64163],[21028,35271],[84089,90433],[44426,91008],[70993,79627],[75068,80401],[35889,39146],[56603,81730],[66599,88524],[15679,62196],[21973,67818],[41868,73078],[41110,81440],[5498,65893],[72775,81582],[56669,70082],[4333,40103],[81685,95728],[32450,46609],[83817,85236],[45593,62840],[31967,60538],[62332,67853],[28329,51028],[94573,96796],[72759,96028],[37097,64084],[75992,85685],[86579,97350],[33503,46903],[12558,37835],[26728,90852],[32610,81224],[7083,28167],[93847,97258],[94567,98940],[70764,88614],[54818,80479],[12370,42053],[45041,61378],[90272,98136],[85743,87629],[11535,86340],[83903,87930],[32188,94765],[28401,73916],[49601,64025],[5010,84491],[70687,87485],[16388,83316],[32559,73223],[56986,98919],[49895,72118],[20656,47055],[42509,45526],[92541,99494],[45858,79758],[3861,38624],[71566,92662],[13061,28847],[64472,95365],[50310,99186],[18331,88572],[62811,66682],[71504,90959],[95047,99506],[93928,95178],[73557,89572],[21716,90945],[15961,59718],[30035,31502],[96847,96916],[45011,68317],[75358,75727],[80055,80920],[83660,95543],[28772,90088],[73437,78155],[6551,15274],[77947,92248],[58147,87917],[10599,18677],[16098,34458],[58047,95425],[49723,67983],[19510,76095],[42770,93619],[22120,53284],[87384,87543],[10249,87753],[89411,94784],[26376,67092],[60000,92610],[96332,96368],[43510,80029],[54559,73956],[58788,85317],[8521,62454],[30640,54127],[15494,32621],[76140,91129],[73381,85100],[54132,64023],[89682,90012],[99733,99958],[50685,83141],[96575,98416],[98202,98931],[35848,63426],[56002,96511],[38179,41383],[12880,96858],[19507,82305],[45232,86599],[14120,82611],[77152,78579],[19958,86814],[95874,97934],[76494,86709],[67905,88495],[52837,74873],[30882,40267],[82654,90023],[57476,97860],[6823,49062],[19555,93025],[27690,85837],[86795,98484],[20601,98761],[776,59138],[82210,87004],[53618,91232],[1257,33100],[80457,88593],[9996,81317],[96435,98378],[27288,94022],[19204,31400],[82348,91476],[49101,79856],[59123,85131],[83653,92509],[84520,89154],[75434,96188],[56690,59674],[2750,73366],[86982,99933],[33749,63050],[24785,27948],[2947,86879],[86737,95666],[95011,99029],[63778,94174],[5525,28998],[23341,42392],[70884,96598],[6987,88644],[51490,90975],[87260,92037],[4185,37893],[70792,71666],[11499,75891],[46686,57517],[83473,90164],[66993,91788],[59356,92650],[4122,97047],[1244,18921],[5261,72591],[12025,70800],[78227,83194],[85456,97665],[11297,18556],[98224,98533],[18145,93063],[37098,64090],[39401,45279],[43166,61318],[16436,54758],[91938,95957],[17446,43859],[59162,70461],[8456,27924],[24381,95193],[29560,69448],[93791,96621],[32162,66301],[42523,77241],[3138,32543],[73818,93968],[63504,87274],[17173,43152],[93191,93459],[70559,86658],[3915,15711],[2001,77539],[33592,62971],[80273,97896],[52456,80831],[71549,79952],[76885,79384],[55049,56022],[40704,93154],[95431,99721],[48420,83342],[75323,80816],[27901,95832],[69939,98296],[13310,32223],[99992,99995],[96472,98467],[18703,80274],[39972,70560],[98042,99902],[49754,97251],[32028,99602],[18928,33960],[33681,34905],[20166,66943],[93577,94830],[34787,51821],[15015,26240],[87795,94286],[64442,94016],[85569,85636],[67604,76073],[18533,19359],[32488,84017],[44283,55849],[42179,68707],[93186,93514],[71856,80230],[68105,69227],[75695,98704],[24430,81248],[53044,68153],[36771,92014],[14427,31147],[11264,15934],[46766,82876],[77208,88954],[44360,56229],[42473,99944],[46161,59131],[46540,88116],[58065,95894],[46177,67640],[93718,98575],[32289,85899],[5906,41260],[45410,48646],[76170,80979],[38592,86015],[89491,94388],[43152,64089],[21626,29905],[12148,30161],[45017,60961],[3639,66965],[6113,86580],[78730,84186],[13550,70610],[19239,87309],[4830,72848],[30040,31484],[29661,61761],[74827,79603],[24222,24637],[49103,68004],[52597,88931],[41586,81417],[99929,99953],[45888,83119],[73823,91149],[81794,93701],[89298,99023],[11514,29725],[40605,70069],[48185,93478],[87246,99648],[59845,69324],[11532,39647],[94396,95197],[765,37103],[29668,93806],[86311,99555],[54635,77443],[58089,83347],[71901,91154],[40974,77241],[70404,82039],[43668,46915],[54401,57036],[56732,60057],[97784,99929],[41265,63209],[2178,29405],[29979,49738],[90179,91823],[24437,25262],[27086,43170],[24603,31887],[6697,65410],[29619,97887],[56810,71320],[15375,58046],[7157,59443],[281,42306],[13297,59476],[23118,80645],[64560,66905],[88444,88464],[69670,95060],[95790,98465],[21367,84089],[28339,45640],[61005,83901],[76011,79038],[55133,99780],[78349,94663],[43568,93412],[4186,9773],[44644,97757],[18598,70780],[15933,17729],[67105,79609],[36497,87416],[78826,81462],[13702,26216],[86456,89248],[41745,90991],[10469,11668],[65005,66044],[11265,45703],[93110,96389],[61991,68597],[80534,91296],[97229,98793],[97866,97977],[39121,64229],[56477,85666],[57942,93912],[47107,82153],[76380,82891],[98385,99434],[49050,99686],[13729,47479],[88585,96598],[63065,65770],[20545,90571],[39134,47645],[22081,52619],[5356,91628],[10561,38907],[52292,71855],[92809,96454],[44764,50254],[34751,90975],[54170,69287],[48919,98089],[30563,95791],[75587,98865],[12539,67550],[62728,82338],[82934,87601],[26353,81330],[8501,83494],[54399,63289],[45360,49908],[48042,72311],[96835,97731],[30558,98219],[45643,56175],[71998,74376],[78184,85442],[29256,31873],[33732,62721],[98556,99886],[90152,97870],[80069,84781],[7512,58705],[69362,92956],[38894,59150],[94398,96864],[70152,93060],[1569,41885],[70233,92771],[95160,98396],[31228,87209],[83115,96781],[85230,88939],[49457,81966],[89275,96051],[81444,89206],[4615,31602],[68385,76044],[60195,83504],[90337,91621],[10106,44689],[80199,97324],[63796,91199],[40245,76879],[11828,54648],[10525,49581],[8469,22674],[14681,28204],[88299,97987],[95060,96998],[11937,74936],[41744,63978],[81359,93676],[21548,57908],[23637,27488],[2210,30595],[42535,55197],[96702,98471],[77416,96603],[55087,81591],[59834,99962],[74450,91021],[26288,41089],[28766,79766],[19031,43799],[19326,67930],[79277,84481],[53500,93900],[49896,59109],[28160,82125],[29123,36909],[8172,72147],[55484,78351],[20882,96952],[89584,89700],[4878,85837],[74596,83100],[20150,78804],[39239,83926],[32792,68496],[66753,67201],[98177,98549],[16545,62585],[3383,86241],[60246,74458],[66805,69244],[65242,82585],[42536,55828],[45808,47573],[88000,90133],[58447,68731],[35320,84110],[13927,57567],[97267,99841],[76604,87935],[12356,29374],[1594,43377],[76043,94876],[90826,97045],[58044,86942],[63563,86420],[90190,91425],[98497,99799],[39888,44387],[68652,85177],[79490,88594],[52679,79060],[36499,60209],[13938,70704],[36291,50679],[80113,87871],[11747,31612],[62171,84375],[27047,45924],[45795,70544],[31980,89976],[35719,96728],[96010,99629],[9845,12055],[17026,57620],[29544,63980],[52861,70168],[30466,34444],[50295,77502],[96387,97997],[70991,80192],[71026,94346],[48913,78537],[64977,85434],[57381,89522],[76187,83175],[96521,99189],[83369,84815],[89286,95697],[14282,84218],[64026,69693],[49561,88866],[14011,58462],[2427,72314],[19375,49916],[62578,67231],[59951,90063],[37123,57649],[13587,51006],[443,31844],[31810,67622],[21034,71201],[61917,91390],[93480,97969],[70391,80076],[30059,73101],[50784,81527],[48366,63697],[57010,72875],[34740,93266],[75259,84637],[89547,94103],[85024,90593],[80156,84149],[49866,83363],[13805,27970],[70888,92997],[67440,78436],[87165,90191],[73700,80362],[85424,87420],[19850,66420],[37100,52315],[47808,65293],[96012,97265],[39651,72849],[18426,56650],[94089,97376],[68099,90992],[83658,91226],[72045,72169],[56031,89693],[68504,87799],[35531,44037],[79107,88387],[8062,76215],[19871,49009],[35707,60747],[99974,99990],[2790,65896],[27060,77752],[27345,40670],[33490,59953],[67913,84064],[91633,97858],[7793,58119],[88016,90705],[25463,81151],[12605,19515],[76537,91964],[96500,97391],[61882,99043],[49058,68279],[60916,94351],[9335,60702],[56294,57861],[53331,90249],[55316,66995],[13006,30326],[16678,51105],[16004,26995],[94877,98589],[32672,37709],[61315,89519],[57388,96794],[38942,70525],[3872,47750],[38837,87201],[85064,86853],[76572,93590],[29655,33570],[82845,87006],[96943,97163],[96716,97812],[4682,43452],[30116,49541],[53474,83750],[22374,74523],[26210,62677],[87937,92215],[30784,82382],[80705,92094],[67631,87695],[68320,95965],[8492,81467],[23291,81797],[57744,69454],[97913,99486],[61526,92080],[98009,98710],[55842,79801],[74857,92682],[88552,91659],[55735,74383],[24425,74049],[91532,92723],[4758,94856],[55514,79356],[94477,94802],[28120,73341],[72218,84599],[34009,96857],[17708,81938],[75647,95976],[8308,46437],[37747,42817],[33217,43817],[65563,86237],[13206,54377],[54381,54780],[41314,50237],[82736,99634],[46589,81786],[47376,70083],[25214,66209],[32454,99839],[4821,68642],[59080,61424],[65018,91421],[90188,97100],[41435,70430],[46429,77509],[16988,79909],[71678,93815],[31469,36909],[71604,90373],[35482,53367],[69496,85068],[41948,52788],[5369,10689],[56413,99677],[48224,80187],[47740,65226],[25417,97780],[72309,80465],[28933,89711],[97396,99176],[65620,67894],[27379,94931],[20619,61939],[94816,98509],[26531,55969],[8681,21701],[63891,72095],[63531,93745],[56204,62581],[84532,99030],[24820,98950],[76490,92644],[32087,51673],[94623,95126],[70740,83290],[40167,75149],[27015,99130],[13808,44491],[35260,99844],[45171,55315],[62907,77661],[42687,81037],[76569,84506],[21344,45266],[7,88079],[70879,93391],[13373,97381],[94060,95535],[30944,57967],[74564,91420],[38547,74822],[38523,65243],[81476,96668],[12165,32552],[77780,94170],[22956,29253],[15473,39646],[74239,99442],[12446,60683],[70295,88494],[59658,89819],[42459,93128],[98906,99745],[74621,94408],[46386,67788],[43541,96046],[55415,72921],[25446,30374],[66908,75713],[874,71954],[1080,19739],[29380,46401],[31827,55959],[66779,92952],[82988,85559],[66245,94263],[58372,59789],[81409,89114],[24716,53081],[56850,72687],[1293,33496],[41322,47835],[8936,96817],[9268,73896],[6123,65615],[65573,74817],[49042,66852],[74054,80858],[85524,92466],[56744,70444],[85571,87140],[97730,99202],[31951,59396],[26697,81199],[62222,85316],[38778,98089],[91962,97395],[46488,78949],[30560,87330],[22818,70268],[45797,84469],[25024,62158],[10024,81608],[50462,52552],[49069,79367],[6024,90748],[60434,85272],[52405,94613],[79720,80761],[34248,37458],[55208,73246],[53427,97337],[10846,69081],[5411,32225],[77407,80302],[53193,76392],[36674,79699],[48108,99532],[89838,99612],[12150,47717],[10021,83637],[85285,86791],[83162,93321],[65845,78059],[98595,99306],[64361,70512],[87123,90627],[78966,96377],[65191,70238],[53162,82448],[24207,29537],[83945,85037],[89622,93418],[1266,8050],[91775,98673],[56496,63432],[25603,52810],[15693,75323],[23256,40366],[5333,31727],[49912,94525],[20921,79689],[74447,84253],[34701,52598],[13395,31042],[597,68076],[13844,65448],[83560,98183],[69094,77117],[903,34346],[28493,52098],[67686,71622],[59153,95087],[95951,96401],[30576,88658],[1664,49388],[66616,83332],[95782,99887],[18549,34382],[19097,58676],[57337,84442],[3820,16610],[21181,63264],[15976,47330],[96883,97628],[85336,88343],[75336,95477],[61967,73539],[67000,68043],[64540,99536],[48521,62458],[6357,74100],[83847,86177],[90518,95838],[27685,64731],[4987,88480],[54823,57190],[43506,78002],[84825,99406],[65546,92102],[67749,98687],[8802,21549],[21741,90714],[65391,93758],[99447,99998],[55159,94353],[3728,27082],[83599,89596],[26062,79891],[37539,88051],[41490,64280],[7931,27430],[84152,88162],[16093,97682],[97530,98861],[70338,73419],[29258,79593],[3152,33484],[23451,59109],[96123,96540],[27073,54100],[27808,65714],[70754,99797],[22083,68256],[43725,81525],[96296,99212],[84693,97409],[78805,98199],[67023,79403],[37848,48729],[63096,95101],[1537,10362],[34126,98993],[28584,97699],[69229,98384],[93633,96423],[68812,87063],[26015,83924],[21803,25060],[11522,96090],[53135,74641],[13187,26772],[28353,55049],[72117,79639],[10997,37046],[34940,46694],[11327,12195],[92124,93370],[88194,93703],[10006,69105],[49968,53124],[99911,99950],[71958,80106],[18670,95259],[950,51131],[71623,80970],[97880,99488],[4370,75459],[88974,97647],[37702,97204],[26208,79777],[6115,8110],[5364,52986],[42773,78704],[93261,94231],[56490,74587],[98693,99260],[56574,66009],[74061,97030],[57781,74087],[28711,35361],[63041,81749],[7911,35540],[45669,52501],[13537,70364],[29611,44836],[92187,96208],[61489,82302],[5887,93188],[13950,57121],[72916,74435],[49509,57126],[19859,85552],[2748,51328],[6939,83033],[4206,71424],[44382,67828],[13920,99103],[96823,98538],[56741,74647],[74313,83382],[41040,67837],[50710,87872],[90176,94199],[40572,54990],[51347,60379],[92888,96285],[94330,95609],[22581,66150],[32446,73141],[90867,97589],[77381,95361],[78437,97162],[49599,69097],[55335,82194],[81898,95547],[42333,97234],[71779,92771],[39209,92947],[40650,51197],[71074,94029],[22968,65090],[8274,90455],[60673,61588],[46840,72237],[25067,54530],[88752,94563],[9374,92378],[54838,63919],[57428,61176],[40030,57238],[13339,21648],[30706,53557],[22389,23059],[18602,89037],[36450,45279],[13267,82522],[81980,84836],[85911,94536],[38665,61775],[13803,17019],[75665,82398],[43026,91029],[24657,47077],[70165,86377],[74117,85851],[49523,53451],[37277,54585],[70628,98019],[12738,99556],[98190,98287],[22882,59759],[93963,94070],[45352,81891],[26537,29295],[12103,21785],[57812,61279],[54601,86705],[53586,64298],[98567,99705],[73506,90373],[48299,52945],[21464,72007],[31158,71120],[44225,72540],[47617,88933],[26433,37234],[47570,69044],[97641,99865],[18531,72190],[49493,70684],[10261,35423],[21971,79968],[95409,98311],[25241,42934],[26420,62292],[14724,17796],[36537,69232],[89200,93521],[59228,68998],[29970,43918],[77851,83049],[34320,76993],[38191,83877],[90060,92789],[7543,75085],[79928,89250],[95280,97040],[84714,94336],[7182,64018],[47070,97502],[62880,96935],[90666,99578],[98140,99221],[75694,90863],[98404,99165],[61473,91103],[24190,75140],[88458,96578],[70017,73799],[22054,90910],[68849,95399],[16051,99547],[54915,97906],[84841,87449],[57194,57729],[71938,72205],[51536,68833],[97616,97971],[43863,48769],[49351,69669],[2896,98783],[48703,74383],[47032,86456],[54161,77166],[32536,53316],[29183,29630],[15268,47330],[16676,85706],[25228,72878],[85207,87986],[24730,27572],[68917,81939],[52406,74003],[18775,23447],[65258,69328],[40669,79667],[98905,99898],[69592,81207],[51160,74319],[76502,89066],[45394,86051],[77656,89383],[7668,21775],[19963,37626],[15998,42215],[11975,37252],[19221,32810],[96607,97225],[92714,97392],[39455,84024],[86098,94204],[62096,82218],[39152,84592],[29751,77302],[85759,91127],[70084,94056],[97601,97645],[79763,82860],[53562,84740],[37160,86410],[78640,78745],[88795,90969],[3724,44151],[18270,53290],[34125,93516],[24536,63364],[36327,78222],[82706,89515],[44130,74648],[31503,79404],[22905,76856],[38100,87048],[67495,87506],[71217,90818],[61796,68783],[93895,99986],[28419,79583],[88097,94602],[69921,99082],[7120,77668],[76541,78645],[28705,87441],[2827,49298],[16841,57606],[63485,99880],[58771,81709],[68237,92850],[48140,56503],[232,77125],[41819,80796],[52379,61991],[87443,90693],[16541,35344],[91818,95922],[22730,90952],[86237,93879],[4544,40631],[29308,64614],[69848,86857],[81345,83216],[70750,96460],[16947,33896],[71385,85548],[5178,72340],[61989,73574],[31695,72714],[35563,61250],[2681,28119],[18336,90013],[64489,97446],[42321,57881],[10644,81944],[8327,50518],[41052,67708],[61618,64386],[68077,96217],[87665,96110],[23554,73071],[95219,99738],[69650,98183],[86205,97945],[42291,73350],[62879,86000],[95372,96072],[16254,66965],[15247,66861],[60632,99262],[85555,87013],[15311,97102],[15331,72448],[37242,58363],[71934,95755],[14248,31676],[95729,99924],[22042,34918],[80844,81549],[93623,98448],[15030,31493],[72616,79923],[36503,91356],[63784,97586],[15138,61991],[73930,77227],[19310,37989],[1042,42801],[63461,95308],[16936,57255],[35409,69485],[56696,81432],[75715,88529],[13225,70224],[31866,71671],[84710,91089],[42821,44596],[66684,98019],[89495,93910],[41840,48251],[64356,84555],[66095,68799],[50166,82508],[4507,36741],[76457,99815],[59510,79370],[8982,40328],[75868,87467],[90286,97676],[45957,54946],[31530,76392],[56349,56834],[46710,53315],[3853,65046],[17186,63143],[95135,98824],[95444,97048],[90196,97932],[29230,79631],[97418,99668],[73399,89295],[4202,25876],[27536,96181],[15037,99318],[44450,90064],[24090,49345],[33285,91888],[95216,99559],[42020,49409],[74375,79141],[82387,86572],[24305,73519],[37336,49142],[23563,94631],[35388,49884],[20421,75753],[79132,98884],[20798,85796],[21167,86761],[22271,60531],[59541,72649],[74048,79624],[81435,88697],[72439,99149],[83853,92177],[24081,50501],[91583,97693],[54961,93572],[11290,54505],[31795,33237],[95018,98274],[51914,69220],[92785,96810],[37008,98744],[34148,56455],[51184,51844],[36787,44043],[39890,52025],[49466,57659],[58718,96702],[80255,84059],[74508,84646],[37936,75805],[81677,89466],[60211,83024],[896,97800],[75877,98474],[83294,96372],[26690,74082],[63450,73008],[51843,84621],[85700,94407],[89762,92886],[9051,99014],[26853,82899],[43507,75020],[35831,48745],[33617,44272],[60457,85709],[14615,60013],[36087,87528],[84518,97431],[25605,42137],[43714,56488],[35631,82346],[88757,90794],[75224,87180],[40706,43445],[96390,98128],[79130,84102],[67953,79317],[3304,94712],[6100,81072],[60090,68651],[95592,98653],[91910,96484],[17483,27396],[68045,94109],[51713,55497],[66883,85207],[71812,90747],[55547,86170],[94084,98047],[78765,89656],[1851,85789],[89524,94770],[28382,39087],[94900,99751],[72288,97845],[41646,68287],[46607,99138],[40334,59877],[1933,33339],[53649,68575],[12717,55003],[11477,33554],[34959,99309],[78701,91719],[40710,87832],[58558,82954],[90330,97319],[82640,99908],[58282,62089],[27491,29638],[94358,96424],[93454,93509],[67979,92098],[65776,94287],[66977,79832],[43331,79353],[82368,93325],[77173,88510],[94389,95430],[52505,64972],[20478,90383],[10687,60099],[35150,75388],[29919,37196],[75941,85297],[9095,73943],[9777,53774],[63704,89242],[51630,90367],[23926,30973],[56330,85114],[74828,91169],[66985,93360],[70343,80843]], targetFriend = 8916
+ ```
+- **Output:** 2235
+
+**Example 7:**
+
+- **Input:**
+ ```
+ times = [[2241,100000],[2852,100000],[5733,100000],[9191,100000],[3272,100000],[2018,100000],[6449,100000],[290,100000],[8719,100000],[4836,100000],[3939,100000],[4903,100000],[1936,100000],[9391,100000],[5910,100000],[1511,100000],[1839,100000],[6922,100000],[392,100000],[9233,100000],[8175,100000],[8520,100000],[5904,100000],[490,100000],[2931,100000],[7972,100000],[7522,100000],[9083,100000],[7653,100000],[955,100000],[135,100000],[4501,100000],[7031,100000],[8180,100000],[6694,100000],[6277,100000],[2061,100000],[6337,100000],[4539,100000],[5685,100000],[700,100000],[7568,100000],[1637,100000],[2600,100000],[2647,100000],[9280,100000],[7895,100000],[3114,100000],[14,100000],[9371,100000],[5615,100000],[8578,100000],[7042,100000],[151,100000],[868,100000],[6735,100000],[6199,100000],[8362,100000],[9615,100000],[9705,100000],[2031,100000],[5189,100000],[5767,100000],[4094,100000],[957,100000],[5939,100000],[9193,100000],[9383,100000],[2528,100000],[9222,100000],[676,100000],[5885,100000],[9888,100000],[2452,100000],[7355,100000],[6998,100000],[4061,100000],[5129,100000],[4364,100000],[9348,100000],[3454,100000],[6239,100000],[3491,100000],[968,100000],[3724,100000],[1629,100000],[6180,100000],[8219,100000],[1842,100000],[673,100000],[8101,100000],[2014,100000],[7500,100000],[8231,100000],[8566,100000],[6216,100000],[7999,100000],[6397,100000],[275,100000],[843,100000],[1471,100000],[1627,100000],[1575,100000],[4032,100000],[829,100000],[2601,100000],[3652,100000],[6807,100000],[9286,100000],[6693,100000],[5684,100000],[4125,100000],[3503,100000],[2727,100000],[8897,100000],[3924,100000],[7961,100000],[3027,100000],[655,100000],[7294,100000],[5547,100000],[2790,100000],[6543,100000],[1724,100000],[6478,100000],[7992,100000],[1851,100000],[1779,100000],[4850,100000],[4598,100000],[1948,100000],[8208,100000],[7056,100000],[2313,100000],[8648,100000],[6276,100000],[1156,100000],[6952,100000],[685,100000],[4522,100000],[2365,100000],[4440,100000],[4940,100000],[3322,100000],[1560,100000],[7682,100000],[9425,100000],[6733,100000],[8398,100000],[5025,100000],[543,100000],[178,100000],[3792,100000],[23,100000],[299,100000],[6755,100000],[7153,100000],[7679,100000],[7715,100000],[394,100000],[2213,100000],[8811,100000],[541,100000],[6684,100000],[8552,100000],[9413,100000],[2097,100000],[16,100000],[5612,100000],[469,100000],[4193,100000],[2605,100000],[2692,100000],[8710,100000],[4269,100000],[7520,100000],[9226,100000],[2765,100000],[6833,100000],[9064,100000],[1036,100000],[6245,100000],[8667,100000],[5518,100000],[7586,100000],[4968,100000],[6439,100000],[6928,100000],[5318,100000],[8943,100000],[8882,100000],[4992,100000],[1204,100000],[2371,100000],[3432,100000],[9100,100000],[4035,100000],[9497,100000],[4970,100000],[5446,100000],[6687,100000],[28,100000],[1769,100000],[4211,100000],[8482,100000],[860,100000],[3896,100000],[9927,100000],[7221,100000],[1939,100000],[9783,100000],[2377,100000],[5406,100000],[9991,100000],[5801,100000],[7110,100000],[8744,100000],[1016,100000],[7290,100000],[7975,100000],[680,100000],[9576,100000],[691,100000],[1477,100000],[6296,100000],[3850,100000],[4510,100000],[7574,100000],[5175,100000],[3293,100000],[1127,100000],[4772,100000],[4951,100000],[2063,100000],[4716,100000],[2363,100000],[7404,100000],[3453,100000],[5437,100000],[1187,100000],[6026,100000],[5535,100000],[6125,100000],[2830,100000],[1031,100000],[6573,100000],[7825,100000],[4958,100000],[5841,100000],[4442,100000],[7218,100000],[2899,100000],[1848,100000],[1923,100000],[8638,100000],[2283,100000],[8100,100000],[2348,100000],[3549,100000],[3838,100000],[1573,100000],[6472,100000],[3008,100000],[8523,100000],[8144,100000],[4514,100000],[3758,100000],[1880,100000],[2379,100000],[1017,100000],[8002,100000],[8080,100000],[5264,100000],[6067,100000],[695,100000],[6958,100000],[431,100000],[6141,100000],[549,100000],[424,100000],[6765,100000],[6220,100000],[693,100000],[7123,100000],[2140,100000],[4614,100000],[4063,100000],[1125,100000],[618,100000],[9038,100000],[8681,100000],[4169,100000],[1828,100000],[5012,100000],[5040,100000],[2506,100000],[7372,100000],[7882,100000],[3143,100000],[2942,100000],[8111,100000],[8260,100000],[3227,100000],[2476,100000],[3228,100000],[586,100000],[8258,100000],[2712,100000],[8797,100000],[1894,100000],[3505,100000],[5355,100000],[4274,100000],[9746,100000],[4391,100000],[2859,100000],[8310,100000],[6851,100000],[8211,100000],[1256,100000],[3897,100000],[232,100000],[8522,100000],[2736,100000],[1531,100000],[1542,100000],[1035,100000],[7864,100000],[5058,100000],[4594,100000],[1438,100000],[3002,100000],[3718,100000],[2179,100000],[1267,100000],[2538,100000],[2306,100000],[1882,100000],[9504,100000],[5142,100000],[2878,100000],[7095,100000],[9767,100000],[3934,100000],[4704,100000],[641,100000],[7341,100000],[9130,100000],[908,100000],[3893,100000],[477,100000],[6419,100000],[3602,100000],[4907,100000],[1022,100000],[136,100000],[1870,100000],[935,100000],[3796,100000],[7265,100000],[846,100000],[4078,100000],[4476,100000],[8255,100000],[3524,100000],[9340,100000],[6066,100000],[686,100000],[1395,100000],[9091,100000],[5093,100000],[2818,100000],[7510,100000],[7161,100000],[4378,100000],[1068,100000],[4431,100000],[8550,100000],[4621,100000],[6633,100000],[7634,100000],[814,100000],[4791,100000],[7968,100000],[9144,100000],[6241,100000],[4657,100000],[3923,100000],[6117,100000],[8903,100000],[3779,100000],[8521,100000],[6133,100000],[9206,100000],[5955,100000],[7717,100000],[7002,100000],[5575,100000],[9081,100000],[8649,100000],[6087,100000],[5868,100000],[2728,100000],[9709,100000],[9857,100000],[6708,100000],[334,100000],[3626,100000],[5366,100000],[3527,100000],[5251,100000],[1354,100000],[7425,100000],[4969,100000],[3078,100000],[3133,100000],[1984,100000],[493,100000],[4919,100000],[6120,100000],[1699,100000],[487,100000],[4143,100000],[9420,100000],[8149,100000],[6913,100000],[5277,100000],[289,100000],[2607,100000],[1339,100000],[879,100000],[1269,100000],[857,100000],[8642,100000],[7417,100000],[2324,100000],[8276,100000],[6170,100000],[3269,100000],[4100,100000],[6775,100000],[8060,100000],[4661,100000],[1402,100000],[6111,100000],[2520,100000],[9247,100000],[1893,100000],[2553,100000],[8726,100000],[5066,100000],[7675,100000],[5817,100000],[5439,100000],[6647,100000],[9704,100000],[7819,100000],[8212,100000],[6336,100000],[6498,100000],[3456,100000],[5109,100000],[5875,100000],[189,100000],[3621,100000],[63,100000],[459,100000],[3128,100000],[7763,100000],[743,100000],[6649,100000],[2102,100000],[3894,100000],[5610,100000],[5991,100000],[8245,100000],[9165,100000],[476,100000],[171,100000],[1247,100000],[4536,100000],[3545,100000],[3136,100000],[1085,100000],[6361,100000],[8440,100000],[6035,100000],[8221,100000],[8703,100000],[9988,100000],[1746,100000],[9417,100000],[6231,100000],[5919,100000],[1822,100000],[486,100000],[578,100000],[867,100000],[6217,100000],[1530,100000],[5866,100000],[1913,100000],[7192,100000],[4701,100000],[8130,100000],[5534,100000],[9179,100000],[1876,100000],[2697,100000],[2792,100000],[302,100000],[1174,100000],[7323,100000],[395,100000],[8589,100000],[6376,100000],[4296,100000],[9000,100000],[502,100000],[4380,100000],[8423,100000],[7581,100000],[2111,100000],[3139,100000],[7926,100000],[3916,100000],[3424,100000],[4532,100000],[7021,100000],[1079,100000],[5878,100000],[8865,100000],[2696,100000],[4437,100000],[4453,100000],[5574,100000],[2584,100000],[1483,100000],[3439,100000],[1445,100000],[8672,100000],[5590,100000],[5149,100000],[6939,100000],[1193,100000],[5585,100000],[6252,100000],[5623,100000],[1803,100000],[6941,100000],[7466,100000],[9627,100000],[6583,100000],[4048,100000],[5678,100000],[7700,100000],[2169,100000],[5375,100000],[8214,100000],[8311,100000],[5436,100000],[5981,100000],[6934,100000],[9244,100000],[3354,100000],[8793,100000],[6863,100000],[1273,100000],[3359,100000],[2095,100000],[8704,100000],[5559,100000],[9780,100000],[5671,100000],[7478,100000],[7476,100000],[4028,100000],[3458,100000],[9814,100000],[2574,100000],[538,100000],[6561,100000],[921,100000],[5915,100000],[7494,100000],[5283,100000],[1753,100000],[4565,100000],[4000,100000],[7626,100000],[8119,100000],[5413,100000],[6357,100000],[5038,100000],[252,100000],[4817,100000],[2106,100000],[119,100000],[8659,100000],[5204,100000],[8901,100000],[249,100000],[1863,100000],[8612,100000],[6585,100000],[7092,100000],[3500,100000],[8359,100000],[3071,100000],[3271,100000],[3216,100000],[9184,100000],[7463,100000],[4672,100000],[861,100000],[8895,100000],[1064,100000],[9155,100000],[1707,100000],[7768,100000],[7244,100000],[3804,100000],[2233,100000],[8172,100000],[9197,100000],[651,100000],[6892,100000],[5393,100000],[3591,100000],[8691,100000],[9463,100000],[8495,100000],[5564,100000],[8394,100000],[9121,100000],[6474,100000],[8908,100000],[775,100000],[6422,100000],[8455,100000],[1541,100000],[2744,100000],[8054,100000],[8122,100000],[8775,100000],[6140,100000],[4023,100000],[8562,100000],[2676,100000],[5974,100000],[9634,100000],[7608,100000],[116,100000],[1037,100000],[850,100000],[3504,100000],[413,100000],[8599,100000],[7623,100000],[5865,100000],[393,100000],[8851,100000],[9056,100000],[8535,100000],[271,100000],[4415,100000],[5385,100000],[3476,100000],[3446,100000],[8515,100000],[2646,100000],[5764,100000],[1578,100000],[6795,100000],[7719,100000],[836,100000],[1253,100000],[6424,100000],[4741,100000],[5804,100000],[8218,100000],[2208,100000],[7869,100000],[8904,100000],[5022,100000],[826,100000],[5376,100000],[8588,100000],[8067,100000],[1926,100000],[6790,100000],[598,100000],[632,100000],[7103,100000],[9323,100000],[2614,100000],[547,100000],[1826,100000],[4862,100000],[7303,100000],[8298,100000],[2456,100000],[709,100000],[9149,100000],[8781,100000],[5877,100000],[3250,100000],[2372,100000],[3618,100000],[3330,100000],[66,100000],[2113,100000],[9428,100000],[8902,100000],[6054,100000],[3990,100000],[4768,100000],[439,100000],[3263,100000],[536,100000],[8456,100000],[301,100000],[7509,100000],[8625,100000],[4044,100000],[8303,100000],[1801,100000],[6932,100000],[2320,100000],[2204,100000],[5273,100000],[2132,100000],[2571,100000],[9125,100000],[4095,100000],[1342,100000],[3412,100000],[6128,100000],[7956,100000],[1938,100000],[6495,100000],[2084,100000],[3820,100000],[4613,100000],[8118,100000],[5723,100000],[5349,100000],[7385,100000],[6523,100000],[4009,100000],[4136,100000],[1940,100000],[5946,100000],[2810,100000],[4255,100000],[2554,100000],[2920,100000],[5788,100000],[9928,100000],[1765,100000],[1078,100000],[8309,100000],[6114,100000],[7610,100000],[7010,100000],[7460,100000],[2193,100000],[1224,100000],[6966,100000],[4567,100000],[7256,100000],[3247,100000],[822,100000],[1556,100000],[1823,100000],[8058,100000],[4212,100000],[7443,100000],[2281,100000],[888,100000],[1488,100000],[97,100000],[6308,100000],[8024,100000],[3573,100000],[2784,100000],[4306,100000],[2560,100000],[894,100000],[3696,100000],[9398,100000],[9088,100000],[2638,100000],[4929,100000],[7538,100000],[8250,100000],[7293,100000],[8767,100000],[6993,100000],[158,100000],[2059,100000],[5860,100000],[3714,100000],[7589,100000],[6404,100000],[4361,100000],[6142,100000],[9986,100000],[8297,100000],[2839,100000],[2726,100000],[5728,100000],[8225,100000],[6079,100000],[2762,100000],[8701,100000],[7403,100000],[9429,100000],[1933,100000],[4601,100000],[1759,100000],[9160,100000],[5420,100000],[1945,100000],[3837,100000],[1412,100000],[4300,100000],[6884,100000],[453,100000],[6510,100000],[1690,100000],[3302,100000],[5549,100000],[5390,100000],[1787,100000],[9459,100000],[852,100000],[211,100000],[1112,100000],[1261,100000],[8635,100000],[739,100000],[1528,100000],[9987,100000],[2950,100000],[405,100000],[3212,100000],[7356,100000],[6854,100000],[1236,100000],[2757,100000],[7177,100000],[1935,100000],[414,100000],[3595,100000],[3125,100000],[4371,100000],[6782,100000],[3575,100000],[8677,100000],[5265,100000],[7933,100000],[8126,100000],[5242,100000],[4779,100000],[6061,100000],[9731,100000],[2645,100000],[8503,100000],[5135,100000],[5080,100000],[6440,100000],[8269,100000],[3764,100000],[8343,100000],[2141,100000],[7739,100000],[7989,100000],[3350,100000],[5185,100000],[8996,100000],[6898,100000],[373,100000],[1653,100000],[4983,100000],[8577,100000],[9537,100000],[4374,100000],[5090,100000],[8277,100000],[2791,100000],[3517,100000],[5469,100000],[6365,100000],[7689,100000],[5786,100000],[6333,100000],[186,100000],[7441,100000],[7108,100000],[2307,100000],[2424,100000],[7991,100000],[7331,100000],[3342,100000],[9768,100000],[3242,100000],[2117,100000],[9446,100000],[2200,100000],[9712,100000],[222,100000],[3631,100000],[2428,100000],[9782,100000],[6985,100000],[1369,100000],[8916,100000],[9759,100000],[1754,100000],[9376,100000],[1841,100000],[5943,100000],[9929,100000],[7,100000],[7360,100000],[7701,100000],[9771,100000],[7280,100000],[2065,100000],[5825,100000],[798,100000],[3294,100000],[1648,100000],[3053,100000],[1802,100000],[8335,100000],[9432,100000],[2473,100000],[2809,100000],[4406,100000],[7003,100000],[7770,100000],[6527,100000],[9549,100000],[233,100000],[8825,100000],[601,100000],[1861,100000],[1255,100000],[6083,100000],[6612,100000],[6799,100000],[1034,100000],[1353,100000],[8542,100000],[2925,100000],[4727,100000],[801,100000],[7564,100000],[9293,100000],[628,100000],[6122,100000],[553,100000],[418,100000],[7374,100000],[2870,100000],[8124,100000],[1811,100000],[7184,100000],[5782,100000],[3929,100000],[166,100000],[8391,100000],[5363,100000],[4128,100000],[2466,100000],[5601,100000],[8053,100000],[5909,100000],[664,100000],[3452,100000],[2772,100000],[6073,100000],[722,100000],[7712,100000],[6926,100000],[4103,100000],[3867,100000],[4963,100000],[4519,100000],[2012,100000],[3774,100000],[361,100000],[6014,100000],[6273,100000],[4521,100000],[6904,100000],[237,100000],[8460,100000],[8446,100000],[6645,100000],[5993,100000],[4715,100000],[4091,100000],[7529,100000],[4949,100000],[2082,100000],[6915,100000],[3070,100000],[473,100000],[4901,100000],[9131,100000],[8770,100000],[7857,100000],[5207,100000],[9918,100000],[7402,100000],[6621,100000],[8318,100000],[9737,100000],[6235,100000],[4751,100000],[9343,100000],[1652,100000],[8259,100000],[8334,100000],[3015,100000],[8356,100000],[6130,100000],[597,100000],[5778,100000],[3014,100000],[542,100000],[3747,100000],[6591,100000],[6151,100000],[236,100000],[789,100000],[8194,100000],[2652,100000],[3630,100000],[8120,100000],[9015,100000],[1284,100000],[1952,100000],[902,100000],[7032,100000],[6166,100000],[7971,100000],[167,100000],[5247,100000],[1392,100000],[5274,100000],[1248,100000],[461,100000],[3365,100000],[50,100000],[3174,100000],[9137,100000],[7125,100000],[7722,100000],[1992,100000],[4186,100000],[3725,100000],[3556,100000],[3821,100000],[2735,100000],[4228,100000],[6302,100000],[4417,100000],[5538,100000],[6964,100000],[7901,100000],[1961,100000],[5711,100000],[802,100000],[2836,100000],[7135,100000],[7732,100000],[7132,100000],[9412,100000],[7219,100000],[899,100000],[4404,100000],[3341,100000],[9356,100000],[2819,100000],[9629,100000],[6949,100000],[501,100000],[9058,100000],[5956,100000],[6912,100000],[9313,100000],[6592,100000],[7391,100000],[124,100000],[2708,100000],[9093,100000],[5621,100000],[8720,100000],[9630,100000],[6260,100000],[9602,100000],[9304,100000],[382,100000],[990,100000],[9021,100000],[8097,100000],[7470,100000],[1390,100000],[6802,100000],[8833,100000],[3699,100000],[5400,100000],[8568,100000],[5336,100000],[7537,100000],[7499,100000],[2995,100000],[3026,100000],[72,100000],[1852,100000],[3754,100000],[293,100000],[7951,100000],[2714,100000],[3198,100000],[1632,100000],[2509,100000],[8905,100000],[981,100000],[2492,100000],[8618,100000],[240,100000],[5668,100000],[2961,100000],[2609,100000],[8743,100000],[4819,100000],[6155,100000],[292,100000],[8420,100000],[1106,100000],[5158,100000],[1846,100000],[3336,100000],[4537,100000],[2092,100000],[2763,100000],[5792,100000],[9845,100000],[1306,100000],[922,100000],[9614,100000],[5085,100000],[2369,100000],[2494,100000],[6379,100000],[3762,100000],[1719,100000],[3571,100000],[2443,100000],[9464,100000],[386,100000],[4870,100000],[2669,100000],[9051,100000],[5285,100000],[9200,100000],[5727,100000],[7944,100000],[1063,100000],[1186,100000],[3379,100000],[8055,100000],[2668,100000],[3389,100000],[929,100000],[5638,100000],[1008,100000],[1011,100000],[8043,100000],[4618,100000],[9309,100000],[4251,100000],[9974,100000],[3682,100000],[7958,100000],[1720,100000],[4494,100000],[3519,100000],[1295,100000],[2190,100000],[3563,100000],[4655,100000],[3355,100000],[522,100000],[7397,100000],[6043,100000],[8493,100000],[4246,100000],[7655,100000],[4401,100000],[2325,100000],[5762,100000],[6954,100000],[2367,100000],[9212,100000],[4165,100000],[2285,100000],[9424,100000],[4566,100000],[7497,100000],[4220,100000],[3522,100000],[9103,100000],[6842,100000],[8131,100000],[1804,100000],[1592,100000],[6530,100000],[8322,100000],[1818,100000],[4253,100000],[4115,100000],[831,100000],[9113,100000],[6143,100000],[2923,100000],[2960,100000],[4962,100000],[8524,100000],[6672,100000],[9199,100000],[3171,100000],[5655,100000],[2861,100000],[5248,100000],[1176,100000],[3674,100000],[903,100000],[5552,100000],[6900,100000],[510,100000],[5959,100000],[4343,100000],[8178,100000],[5278,100000],[471,100000],[3769,100000],[9568,100000],[4991,100000],[8977,100000],[6562,100000],[8866,100000],[1902,100000],[9133,100000],[1681,100000],[8564,100000],[9979,100000],[4684,100000],[7902,100000],[2778,100000],[5310,100000],[6890,100000],[9466,100000],[1808,100000],[773,100000],[4761,100000],[7617,100000],[5781,100000],[3494,100000],[9188,100000],[7317,100000],[2467,100000],[5570,100000],[4436,100000],[5326,100000],[9982,100000],[306,100000],[6947,100000],[9476,100000],[5856,100000],[1736,100000],[6477,100000],[8587,100000],[6082,100000],[4468,100000],[2335,100000],[1123,100000],[5783,100000],[7479,100000],[9829,100000],[2518,100000],[4270,100000],[7452,100000],[435,100000],[1577,100000],[806,100000],[6878,100000],[3822,100000],[6588,100000],[86,100000],[6703,100000],[7431,100000],[371,100000],[4553,100000],[6403,100000],[7761,100000],[5541,100000],[9331,100000],[4484,100000],[2359,100000],[1160,100000],[9688,100000],[7820,100000],[1118,100000],[9395,100000],[9873,100000],[6208,100000],[5855,100000],[8405,100000],[1465,100000],[3872,100000],[4794,100000],[133,100000],[9613,100000],[7647,100000],[7900,100000],[9473,100000],[364,100000],[8137,100000],[1554,100000],[2752,100000],[4928,100000],[1871,100000],[3191,100000],[3487,100000],[5117,100000],[2664,100000],[4122,100000],[5999,100000],[2022,100000],[8396,100000],[2588,100000],[1271,100000],[4912,100000],[7514,100000],[8306,100000],[8148,100000],[4330,100000],[654,100000],[8559,100000],[2620,100000],[8556,100000],[2606,100000],[5627,100000],[3108,100000],[6933,100000],[440,100000],[3313,100000],[3592,100000],[8448,100000],[5055,100000],[2833,100000],[3615,100000],[8889,100000],[7492,100000],[4126,100000],[3513,100000],[3285,100000],[7028,100000],[4610,100000],[7544,100000],[5348,100000],[1213,100000],[2210,100000],[5300,100000],[5675,100000],[2906,100000],[6318,100000],[508,100000],[8831,100000],[5950,100000],[1735,100000],[368,100000],[6185,100000],[172,100000],[6822,100000],[1763,100000],[5463,100000],[2807,100000],[5577,100000],[6674,100000],[6938,100000],[5907,100000],[7197,100000],[6686,100000],[930,100000],[6879,100000],[1208,100000],[4397,100000],[38,100000],[6711,100000],[620,100000],[7420,100000],[7746,100000],[4740,100000],[8106,100000],[8910,100000],[5662,100000],[7818,100000],[1496,100000],[3869,100000],[2808,100000],[7503,100000],[8600,100000],[6109,100000],[7186,100000],[9649,100000],[3957,100000],[914,100000],[4304,100000],[3012,100000],[3823,100000],[9454,100000],[6671,100000],[8299,100000],[7668,100000],[4979,100000],[8877,100000],[6295,100000],[7754,100000],[9002,100000],[6667,100000],[5120,100000],[6707,100000],[138,100000],[2635,100000],[1497,100000],[819,100000],[1487,100000],[8358,100000],[9095,100000],[3056,100000],[776,100000],[5823,100000],[3094,100000],[2741,100000],[7049,100000],[8439,100000],[7442,100000],[7924,100000],[9316,100000],[1621,100000],[4898,100000],[3036,100000],[3210,100000],[1899,100000],[6967,100000],[7988,100000],[716,100000],[1027,100000],[9042,100000],[1275,100000],[3295,100000],[272,100000],[9282,100000],[983,100000],[1505,100000],[2655,100000],[5738,100000],[2160,100000],[924,100000],[4348,100000],[8922,100000],[1866,100000],[752,100000],[8662,100000],[8580,100000],[3644,100000],[4944,100000],[8782,100000],[5193,100000],[8475,100000],[3387,100000],[1052,100000],[9752,100000],[996,100000],[3679,100000],[1110,100000],[8105,100000],[717,100000],[5353,100000],[6394,100000],[1038,100000],[6586,100000],[1119,100000],[6767,100000],[7993,100000],[190,100000],[8528,100000],[9218,100000],[3651,100000],[9753,100000],[8548,100000],[4881,100000],[4525,100000],[6219,100000],[4538,100000],[9861,100000],[3835,100000],[3076,100000],[4635,100000],[1385,100000],[5758,100000],[340,100000],[4427,100000],[3794,100000],[2617,100000],[4301,100000],[9427,100000],[4568,100000],[1815,100000],[7445,100000],[9879,100000],[9779,100000],[8141,100000],[5528,100000],[6682,100000],[8860,100000],[6255,100000],[93,100000],[8984,100000],[316,100000],[7495,100000],[5103,100000],[915,100000],[9259,100000],[3749,100000],[7253,100000],[970,100000],[9856,100000],[804,100000],[213,100000],[223,100000],[5042,100000],[6942,100000],[7305,100000],[9584,100000],[277,100000],[1419,100000],[3817,100000],[6678,100000],[1873,100000],[4785,100000],[7107,100000],[9045,100000],[7349,100000],[6757,100000],[8709,100000],[7191,100000],[408,100000],[5958,100000],[5730,100000],[2147,100000],[7000,100000],[1656,100000],[5195,100000],[9540,100000],[9864,100000],[2909,100000],[1009,100000],[650,100000],[9841,100000],[6147,100000],[5111,100000],[2314,100000],[4107,100000],[3982,100000],[6701,100000],[9781,100000],[9900,100000],[8885,100000],[4957,100000],[7772,100000],[6877,100000],[3426,100000],[2232,100000],[6525,100000],[1878,100000],[2504,100000],[2260,100000],[5686,100000],[6800,100000],[8085,100000],[251,100000],[3062,100000],[3815,100000],[2801,100000],[5542,100000],[298,100000],[6212,100000],[4934,100000],[7685,100000],[6303,100000],[4196,100000],[1400,100000],[623,100000],[7029,100000],[8707,100000],[9675,100000],[2657,100000],[5917,100000],[2675,100000],[7225,100000],[7800,100000],[9374,100000],[3983,100000],[7165,100000],[5598,100000],[2429,100000],[5591,100000],[8920,100000],[7660,100000],[984,100000],[8995,100000],[9592,100000],[7863,100000],[3669,100000],[2238,100000],[5614,100000],[20,100000],[1733,100000],[5259,100000],[1266,100000],[5,100000],[800,100000],[4309,100000],[6157,100000],[22,100000],[689,100000],[5351,100000],[9724,100000],[1922,100000],[4697,100000],[1329,100000],[6824,100000],[463,100000],[1305,100000],[7131,100000],[4320,100000],[7313,100000],[8059,100000],[4821,100000],[5112,100000],[163,100000],[1812,100000],[3746,100000],[3422,100000],[8700,100000],[7087,100000],[7591,100000],[2693,100000],[5820,100000],[1640,100000],[2417,100000],[6806,100000],[9998,100000],[3234,100000],[2389,100000],[5382,100000],[2868,100000],[3200,100000],[9043,100000],[6502,100000],[7017,100000],[5742,100000],[13,100000],[2637,100000],[4287,100000],[5669,100000],[3259,100000],[8717,100000],[7687,100000],[7213,100000],[4669,100000],[2616,100000],[7546,100000],[1191,100000],[4602,100000],[7091,100000],[4463,100000],[1539,100000],[5011,100000],[2337,100000],[748,100000],[5693,100000],[8411,100000],[6113,100000],[6224,100000],[5853,100000],[1277,100000],[8017,100000],[6227,100000],[6638,100000],[3220,100000],[2569,100000],[2025,100000],[5299,100000],[6615,100000],[9897,100000],[8224,100000],[2066,100000],[7606,100000],[6460,100000],[5487,100000],[6484,100000],[4170,100000],[6717,100000],[9811,100000],[5927,100000],[8132,100000],[3609,100000],[5834,100000],[75,100000],[6119,100000],[6329,100000],[6271,100000],[4879,100000],[1689,100000],[6005,100000],[9483,100000],[9359,100000],[2887,100000],[6754,100000],[2975,100000],[455,100000],[9872,100000],[6818,100000],[1568,100000],[5626,100000],[6312,100000],[2049,100000],[2864,100000],[1007,100000],[420,100000],[6582,100000],[2875,100000],[5404,100000],[552,100000],[3514,100000],[2532,100000],[3889,100000],[3827,100000],[5965,100000],[6546,100000],[5337,100000],[8108,100000],[5121,100000],[9242,100000],[9662,100000],[573,100000],[6024,100000],[1153,100000],[6580,100000],[3266,100000],[8933,100000],[1711,100000],[627,100000],[7292,100000],[794,100000],[5005,100000],[878,100000],[8873,100000],[1455,100000],[6004,100000],[9948,100000],[9745,100000],[9347,100000],[3579,100000],[5643,100000],[2980,100000],[703,100000],[9876,100000],[1523,100000],[5667,100000],[131,100000],[9470,100000],[4497,100000],[1639,100000],[5097,100000],[4166,100000],[7273,100000],[8473,100000],[3906,100000],[7340,100000],[7943,100000],[4088,100000],[4917,100000],[8457,100000],[4168,100000],[2088,100000],[7113,100000],[7033,100000],[3256,100000],[6595,100000],[7914,100000],[199,100000],[7097,100000],[8496,100000],[7774,100000],[7449,100000],[8470,100000],[1355,100000],[2487,100000],[4016,100000],[3332,100000],[8165,100000],[1494,100000],[1614,100000],[8774,100000],[7876,100000],[9085,100000],[2705,100000],[512,100000],[5828,100000],[774,100000],[4001,100000],[3693,100000],[6291,100000],[2131,100000],[9637,100000],[1262,100000],[9645,100000],[1002,100000],[5315,100000],[168,100000],[326,100000],[3594,100000],[7580,100000],[1616,100000],[110,100000],[3706,100000],[1701,100000],[3213,100000],[2944,100000],[8560,100000],[3347,100000],[4259,100000],[603,100000],[8016,100000],[2834,100000],[2824,100000],[1279,100000],[2048,100000],[1282,100000],[62,100000],[2503,100000],[5526,100000],[8527,100000],[3337,100000],[4699,100000],[1849,100000],[8427,100000],[6341,100000],[1189,100000],[8395,100000],[5625,100000],[9762,100000],[6173,100000],[8068,100000],[9003,100000],[3403,100000],[6323,100000],[55,100000],[1121,100000],[588,100000],[9012,100000],[2183,100000],[720,100000],[4447,100000],[5110,100000],[1116,100000],[5047,100000],[8040,100000],[9337,100000],[8012,100000],[5628,100000],[102,100000],[4386,100000],[3958,100000],[6476,100000],[3100,100000],[8773,100000],[9960,100000],[4660,100000],[9938,100000],[3421,100000],[9216,100000],[3676,100000],[5740,100000],[5694,100000],[9827,100000],[9224,100000],[6209,100000],[4623,100000],[3031,100000],[563,100000],[9764,100000],[9457,100000],[8189,100000],[2780,100000],[3377,100000],[9697,100000],[182,100000],[1229,100000],[5968,100000],[2749,100000],[4998,100000],[2255,100000],[2471,100000],[9887,100000],[2955,100000],[9116,100000],[7096,100000],[5931,100000],[9871,100000],[8917,100000],[3116,100000],[6677,100000],[6211,100000],[6604,100000],[6736,100000],[8779,100000],[6857,100000],[1107,100000],[2707,100000],[1665,100000],[6214,100000],[2631,100000],[2521,100000],[2627,100000],[6000,100000],[675,100000],[2300,100000],[9713,100000],[1597,100000],[5725,100000],[9947,100000],[8843,100000],[9899,100000],[1388,100000],[9693,100000],[4083,100000],[5485,100000],[2085,100000],[8671,100000],[9850,100000],[1884,100000],[1328,100000],[3917,100000],[5308,100000],[3386,100000],[6086,100000],[746,100000],[897,100000],[6697,100000],[9478,100000],[8914,100000],[767,100000],[3612,100000],[8429,100000],[4050,100000],[3329,100000],[8064,100000],[78,100000],[8410,100000],[630,100000],[5743,100000],[65,100000],[6792,100000],[9172,100000],[3037,100000],[875,100000],[5442,100000],[4941,100000],[974,100000],[1951,100000],[4368,100000],[3468,100000],[3950,100000],[4737,100000],[3777,100000],[6287,100000],[5433,100000],[4953,100000],[5133,100000],[5202,100000],[611,100000],[934,100000],[8038,100000],[49,100000],[8074,100000],[5619,100000],[7130,100000],[8823,100000],[7970,100000],[7726,100000],[1436,100000],[2590,100000],[5250,100000],[2589,100000],[8739,100000],[5171,100000],[2472,100000],[5197,100000],[5706,100000],[5411,100000],[5473,100000],[9271,100000],[3123,100000],[7371,100000],[8103,100000],[5606,100000],[3548,100000],[2719,100000],[9092,100000],[447,100000],[812,100000],[1727,100000],[61,100000],[1515,100000],[2937,100000],[6909,100000],[3107,100000],[4709,100000],[6479,100000],[8319,100000],[2136,100000],[4546,100000],[7776,100000],[5430,100000],[7288,100000],[6097,100000],[7921,100000],[9546,100000],[5997,100000],[6426,100000],[6435,100000],[5523,100000],[4345,100000],[8325,100000],[1910,100000],[3930,100000],[2640,100000],[7810,100000],[1857,100000],[3900,100000],[465,100000],[505,100000],[3291,100000],[1636,100000],[438,100000],[2758,100000],[3179,100000],[1450,100000],[2294,100000],[3648,100000],[5311,100000],[9055,100000],[8676,100000],[8092,100000],[7338,100000],[1211,100000],[1216,100000],[401,100000],[96,100000],[8313,100000],[1370,100000],[3007,100000],[2449,100000],[7471,100000],[6558,100000],[4381,100000],[7528,100000],[1745,100000],[5182,100000],[9141,100000],[2874,100000],[3832,100000],[4848,100000],[3863,100000],[397,100000],[2515,100000],[866,100000],[8077,100000],[1657,100000],[7334,100000],[3397,100000],[6107,100000],[5016,100000],[4308,100000],[6002,100000],[6988,100000],[3058,100000],[6355,100000],[7200,100000],[9314,100000],[876,100000],[5399,100000],[1185,100000],[291,100000],[2525,100000],[4720,100000],[2157,100000],[9848,100000],[8417,100000],[9847,100000],[224,100000],[6267,100000],[3311,100000],[9208,100000],[3905,100000],[8970,100000],[2240,100000],[4323,100000],[978,100000],[7677,100000],[5604,100000],[4236,100000],[4325,100000],[9239,100000],[9468,100000],[8278,100000],[6776,100000],[6893,100000],[8337,100000],[3919,100000],[2556,100000],[9894,100000],[2121,100000],[8551,100000],[9999,100000],[9567,100000],[2347,100000],[9448,100000],[8631,100000],[6272,100000],[6656,100000],[5835,100000],[4355,100000],[8113,100000],[7128,100000],[3664,100000],[9714,100000],[3047,100000],[9143,100000],[4398,100000],[539,100000],[1976,100000],[6483,100000],[468,100000],[5862,100000],[3430,100000],[8742,100000],[619,100000],[2513,100000],[3281,100000],[2777,100000],[8567,100000],[9080,100000],[2135,100000],[6504,100000],[1475,100000],[3828,100000],[5017,100000],[1111,100000],[2877,100000],[8081,100000],[3152,100000],[7733,100000],[4739,100000],[6544,100000],[7551,100000],[5034,100000],[4955,100000],[778,100000],[862,100000],[3025,100000],[7738,100000],[205,100000],[8330,100000],[6433,100000],[4470,100000],[7630,100000],[4489,100000],[4746,100000],[6688,100000],[671,100000],[2623,100000],[4014,100000],[764,100000],[9813,100000],[7392,100000],[3760,100000],[2853,100000],[1606,100000],[2970,100000],[3244,100000],[5491,100000],[9739,100000],[2665,100000],[333,100000],[9664,100000],[1668,100000],[2972,100000],[2895,100000],[7119,100000],[6788,100000],[3907,100000],[1346,100000],[7065,100000],[2441,100000],[4153,100000],[5771,100000],[9674,100000],[2207,100000],[3335,100000],[1382,100000],[6745,100000],[9529,100000],[9803,100000],[5551,100000],[348,100000],[2756,100000],[1230,100000],[3755,100000],[8961,100000],[4053,100000],[3115,100000],[8409,100000],[3081,100000],[7044,100000],[1538,100000],[4210,100000],[9878,100000],[5886,100000],[4222,100000],[9538,100000],[4563,100000],[4060,100000],[5072,100000],[3399,100000],[5074,100000],[2667,100000],[9958,100000],[9159,100000],[3680,100000],[3155,100000],[9389,100000],[3841,100000],[6617,100000],[8712,100000],[2276,100000],[1061,100000],[9997,100000],[6178,100000],[57,100000],[1934,100000],[6360,100000],[8761,100000],[9018,100000],[4633,100000],[4645,100000],[5613,100000],[4076,100000],[1070,100000],[2392,100000],[1756,100000],[7567,100000],[2994,100000],[3345,100000],[437,100000],[1427,100000],[8938,100000],[7848,100000],[2658,100000],[4132,100000],[3086,100000],[9097,100000],[2056,100000],[2835,100000],[8003,100000],[5307,100000],[2903,100000],[5343,100000],[6051,100000],[4027,100000],[9706,100000],[1226,100000],[8543,100000],[8384,100000],[6749,100000],[2847,100000],[5588,100000],[5115,100000],[4362,100000],[2152,100000],[6811,100000],[7815,100000],[3694,100000],[1225,100000],[7199,100000],[8952,100000],[8005,100000],[1202,100000],[1836,100000],[4338,100000],[3371,100000],[9838,100000],[9620,100000],[3510,100000],[6662,100000],[9722,100000],[591,100000],[2566,100000],[2543,100000],[8182,100000],[9901,100000],[9600,100000],[9122,100000],[8643,100000],[7791,100000],[1904,100000],[1384,100000],[318,100000],[4714,100000],[704,100000],[4337,100000],[6060,100000],[8424,100000],[5448,100000],[1732,100000],[777,100000],[1679,100000],[3798,100000],[9508,100000],[2886,100000],[7801,100000],[5838,100000],[6262,100000],[402,100000],[1805,100000],[8892,100000],[7981,100000],[5494,100000],[1096,100000],[2421,100000],[4031,100000],[4454,100000],[5984,100000],[3617,100000],[2564,100000],[8911,100000],[1314,100000],[444,100000],[6307,100000],[710,100000],[2917,100000],[2827,100000],[8291,100000],[6189,100000],[7693,100000],[7880,100000],[577,100000],[5338,100000],[8032,100000],[2684,100000],[4624,100000],[6206,100000],[4712,100000],[9465,100000],[4049,100000],[1215,100000],[951,100000],[496,100000],[9461,100000],[9516,100000],[8011,100000],[1042,100000],[7122,100000],[2118,100000],[2529,100000],[3552,100000],[9563,100000],[9439,100000],[2632,100000],[7314,100000],[6195,100000],[8176,100000],[5587,100000],[2269,100000],[2482,100000],[8875,100000],[3708,100000],[647,100000],[9840,100000],[9558,100000],[1781,100000],[8451,100000],[6482,100000],[9306,100000],[1772,100000],[2926,100000],[2624,100000],[5596,100000],[9641,100000],[4996,100000],[5922,100000],[4599,100000],[587,100000],[9500,100000],[1762,100000],[9240,100000],[5857,100000],[8489,100000],[9886,100000],[5506,100000],[5099,100000],[4034,100000],[18,100000],[303,100000],[1379,100000],[834,100000],[9791,100000],[4133,100000],[6284,100000],[5710,100000],[7363,100000],[330,100000],[4469,100000],[2914,100000],[2053,100000],[735,100000],[2656,100000],[4129,100000],[1782,100000],[9512,100000],[7098,100000],[8171,100000],[3891,100000],[4604,100000],[5288,100000],[6610,100000],[8371,100000],[3994,100000],[9477,100000],[9453,100000],[6388,100000],[499,100000],[4064,100000],[5377,100000],[2984,100000],[3303,100000],[6354,100000],[3193,100000],[6540,100000],[7176,100000],[2343,100000],[1797,100000],[3375,100000],[2165,100000],[2148,100000],[310,100000],[3803,100000],[9357,100000],[8465,100000],[1162,100000],[3304,100000],[9046,100000],[274,100000],[1304,100000],[7285,100000],[2604,100000],[9916,100000],[1059,100000],[8852,100000],[1889,100000],[6350,100000],[8837,100000],[6058,100000],[2599,100000],[4547,100000],[1809,100000],[2316,100000],[7162,100000],[5403,100000],[5525,100000],[8071,100000],[495,100000],[1840,100000],[5190,100000],[8022,100000],[4117,100000],[6500,100000],[3684,100000],[3093,100000],[8232,100000],[4542,100000],[9945,100000],[3824,100000],[9292,100000],[2361,100000],[5784,100000],[6408,100000],[7424,100000],[882,100000],[9109,100000],[8511,100000],[449,100000],[8453,100000],[2174,100000],[5183,100000],[6288,100000],[457,100000],[9760,100000],[3463,100000],[5163,100000],[4199,100000],[4939,100000],[161,100000],[8655,100000],[6948,100000],[8154,100000],[1210,100000],[936,100000],[3023,100000],[4511,100000],[4262,100000],[9543,100000],[363,100000],[6481,100000],[7464,100000],[1097,100000],[2501,100000],[2254,100000],[3496,100000],[3886,100000],[4006,100000],[3852,100000],[5824,100000],[7398,100000],[5241,100000],[9581,100000],[4980,100000],[3551,100000],[5470,100000],[8502,100000],[1268,100000],[5645,100000],[6965,100000],[3427,100000],[945,100000],[9524,100000],[8695,100000],[2731,100000],[558,100000],[2633,100000],[3039,100000],[7842,100000],[7487,100000],[6044,100000],[322,100000],[2879,100000],[6929,100000],[3280,100000],[1330,100000],[4223,100000],[3995,100000],[8272,100000],[4921,100000],[6553,100000],[4108,100000],[9170,100000],[1584,100000],[1484,100000],[9035,100000],[6906,100000],[9923,100000],[1696,100000],[3523,100000],[4221,100000],[1458,100000],[817,100000],[4549,100000],[9020,100000],[6606,100000],[2706,100000],[5846,100000],[3859,100000],[4340,100000],[214,100000],[6196,100000],[3033,100000],[579,100000],[8805,100000],[3759,100000],[1744,100000],[5065,100000],[3633,100000],[1702,100000],[7016,100000],[4312,100000],[4872,100000],[8242,100000],[9880,100000],[7319,100000],[7836,100000],[8312,100000],[2440,100000],[7083,100000],[2789,100000],[8145,100000],[5398,100000],[2613,100000],[1687,100000],[9421,100000],[5232,100000],[2445,100000],[9792,100000],[755,100000],[7450,100000],[9368,100000],[5063,100000],[5222,100000],[8348,100000],[8898,100000],[192,100000],[1980,100000],[4900,100000],[4225,100000],[1572,100000],[3273,100000],[5239,100000],[7745,100000],[7941,100000],[5672,100000],[2869,100000],[7540,100000],[4164,100000],[4857,100000],[6991,100000],[6191,100000],[988,100000],[5737,100000],[3561,100000],[1015,100000],[7896,100000],[6660,100000],[5295,100000],[6702,100000],[2310,100000],[4239,100000],[7816,100000],[635,100000],[9550,100000],[1536,100000],[7198,100000],[7752,100000],[9833,100000],[5768,100000],[4257,100000],[8956,100000],[9685,100000],[4767,100000],[5636,100000],[245,100000],[2218,100000],[8949,100000],[6339,100000],[4450,100000],[31,100000],[2109,100000],[9166,100000],[7766,100000],[4776,100000],[1062,100000],[9657,100000],[7656,100000],[1308,100000],[7809,100000],[9096,100000],[6343,100000],[9843,100000],[8206,100000],[4249,100000],[1722,100000],[8152,100000],[4281,100000],[9487,100000],[8900,100000],[9855,100000],[4530,100000],[7583,100000],[4356,100000],[1489,100000],[8205,100000],[4073,100000],[7891,100000],[5187,100000],[8008,100000],[5282,100000],[2910,100000],[4606,100000],[7458,100000],[5492,100000],[6434,100000],[6084,100000],[11,100000],[8864,100000],[7821,100000],[810,100000],[5940,100000],[9161,100000],[3306,100000],[7350,100000],[5468,100000],[5001,100000],[2231,100000],[1875,100000],[6750,100000],[6401,100000],[9951,100000],[6803,100000],[6256,100000],[7062,100000],[9426,100000],[342,100000],[9647,100000],[6629,100000],[4749,100000],[3840,100000],[7947,100000],[5416,100000],[5572,100000],[956,100000],[8365,100000],[9718,100000],[7358,100000],[7897,100000],[4938,100000],[4021,100000],[3962,100000],[795,100000],[6658,100000],[6486,100000],[3704,100000],[7963,100000],[8514,100000],[5421,100000],[6040,100000],[3316,100000],[479,100000],[854,100000],[3975,100000],[3428,100000],[9489,100000],[2011,100000],[115,100000],[4914,100000],[6895,100000],[7106,100000],[5089,100000],[1264,100000],[4967,100000],[1481,100000],[581,100000],[3321,100000],[6270,100000],[9236,100000],[7258,100000],[1624,100000],[7515,100000],[6210,100000],[3482,100000],[7629,100000],[2663,100000],[1508,100000],[2579,100000],[4290,100000],[5003,100000],[360,100000],[3805,100000],[8802,100000],[1313,100000],[5323,100000],[6430,100000],[6639,100000],[3751,100000],[1845,100000],[7795,100000],[3307,100000],[2811,100000],[1595,100000],[2577,100000],[6392,100000],[1159,100000],[8382,100000],[9609,100000],[5954,100000],[1348,100000],[1367,100000],[9273,100000],[7105,100000],[6559,100000],[3118,100000],[7223,100000],[5418,100000],[1990,100000],[1474,100000],[2196,100000],[2463,100000],[5333,100000],[6447,100000],[53,100000],[2001,100000],[3484,100000],[2199,100000],[454,100000],[4752,100000],[5230,100000],[8981,100000],[5895,100000],[8803,100000],[5205,100000],[5568,100000],[8935,100000],[1786,100000],[9884,100000],[1259,100000],[6093,100000],[5852,100000],[8248,100000],[8702,100000],[3785,100000],[2195,100000],[1428,100000],[2352,100000],[6025,100000],[246,100000],[1431,100000],[3478,100000],[9062,100000],[9690,100000],[6317,100000],[1685,100000],[2398,100000],[7354,100000],[9295,100000],[73,100000],[1740,100000],[3606,100000],[9877,100000],[4824,100000],[4261,100000],[5268,100000],[4777,100000],[9118,100000],[3157,100000],[2957,100000],[4339,100000],[2586,100000],[3436,100000],[9812,100000],[3219,100000],[7650,100000],[6724,100000],[5221,100000],[220,100000],[6171,100000],[5818,100000],[8986,100000],[5592,100000],[4654,100000],[1628,100000],[7664,100000],[1557,100000],[944,100000],[4395,100000],[157,100000],[100,100000],[1833,100000],[9073,100000],[1932,100000],[7844,100000],[5077,100000],[2170,100000],[6886,100000],[8459,100000],[7411,100000],[8001,100000],[3521,100000],[7474,100000],[3112,100000],[7216,100000],[5052,100000],[1250,100000],[7045,100000],[2237,100000],[7205,100000],[9289,100000],[3084,100000],[3383,100000],[4753,100000],[3993,100000],[6508,100000],[1318,100000],[9906,100000],[6957,100000],[1326,100000],[9837,100000],[2403,100000],[995,100000],[5530,100000],[8576,100000],[6756,100000],[1780,100000],[7594,100000],[2857,100000],[4952,100000],[9053,100000],[6467,100000],[3253,100000],[6362,100000],[369,100000],[1482,100000],[9962,100000],[734,100000],[1647,100000],[5973,100000],[9573,100000],[9743,100000],[723,100000],[643,100000],[3398,100000],[3151,100000],[3165,100000],[5021,100000],[2930,100000],[9285,100000],[657,100000],[3783,100000],[2519,100000],[8019,100000],[6190,100000],[3425,100000],[3362,100000],[9243,100000],[682,100000],[3720,100000],[6982,100000],[7468,100000],[3433,100000],[9802,100000],[8611,100000],[2998,100000],[7034,100000],[9072,100000],[403,100000],[4197,100000],[8190,100000],[5228,100000],[949,100000],[694,100000],[546,100000],[9955,100000],[7888,100000],[9621,100000],[7406,100000],[5000,100000],[1800,100000],[7419,100000],[7249,100000],[5753,100000],[3240,100000],[1190,100000],[8687,100000],[7845,100000],[5188,100000],[1694,100000],[8699,100000],[1517,100000],[3532,100000],[5254,100000],[3333,100000],[4658,100000],[8414,100000],[1834,100000],[1130,100000],[2822,100000],[9717,100000],[4523,100000],[2932,100000],[9501,100000],[6992,100000],[5184,100000],[3261,100000],[6283,100000],[3788,100000],[391,100000],[1706,100000],[8281,100000],[8887,100000],[1341,100000],[2594,100000],[1246,100000],[6542,100000],[7235,100000],[8151,100000],[7366,100000],[8814,100000],[991,100000],[4687,100000],[8874,100000],[6099,100000],[5582,100000],[4231,100000],[9689,100000],[9230,100000],[1183,100000],[441,100000],[9860,100000],[4664,100000],[2209,100000],[8545,100000],[7784,100000],[6945,100000],[336,100000],[5647,100000],[2077,100000],[7491,100000],[5808,100000],[2527,100000],[9786,100000],[6537,100000],[6734,100000],[719,100000],[2150,100000],[1258,100000],[1977,100000],[1292,100000],[9089,100000],[759,100000],[9800,100000],[3207,100000],[3742,100000],[7127,100000],[8203,100000],[6691,100000],[5651,100000],[6480,100000],[1920,100000],[8512,100000],[3597,100000],[7156,100000],[2087,100000],[5814,100000],[4176,100000],[9963,100000],[2973,100000],[5405,100000],[3418,100000],[2349,100000],[5902,100000],[4298,100000],[5763,100000],[6030,100000],[3485,100000],[6780,100000],[5465,100000],[5484,100000],[6968,100000],[2163,100000],[1404,100000],[5169,100000],[2005,100000],[9809,100000],[8492,100000],[7854,100000],[5873,100000],[6442,100000],[6994,100000],[6505,100000],[7001,100000],[7264,100000],[6022,100000],[8366,100000],[3737,100000],[1498,100000],[5560,100000],[1688,100000],[4734,100000],[5867,100000],[357,100000],[1998,100000],[6056,100000],[480,100000],[1076,100000],[6804,100000],[2737,100000],[4256,100000],[1757,100000],[1617,100000],[1716,100000],[5757,100000],[7553,100000],[3700,100000],[1446,100000],[7196,100000],[1413,100000],[5328,100000],[5859,100000],[6279,100000],[6917,100000],[6037,100000],[1154,100000],[152,100000],[2500,100000],[7345,100000],[6459,100000],[2533,100000],[950,100000],[4769,100000],[9749,100000],[5322,100000],[6569,100000],[5357,100000],[1323,100000],[6420,100000],[2698,100000],[7102,100000],[6663,100000],[6412,100000],[6975,100000],[1320,100000],[4887,100000],[2355,100000],[8034,100000],[5736,100000],[5690,100000],[881,100000],[8724,100000],[5249,100000],[4795,100000],[2572,100000],[3932,100000],[7208,100000],[9364,100000],[3875,100000],[6268,100000],[4229,100000],[7706,100000],[5048,100000],[1730,100000],[7557,100000],[9480,100000],[7024,100000],[5102,100000],[1596,100000],[7202,100000],[5476,100000],[6493,100000],[2107,100000],[159,100000],[7051,100000],[4756,100000],[9729,100000],[3489,100000],[3231,100000],[8042,100000],[9984,100000],[114,100000],[8665,100000],[5362,100000],[4405,100000],[4666,100000],[7386,100000],[8715,100000],[7859,100000],[3498,100000],[8883,100000],[7035,100000],[8462,100000],[6989,100000],[9542,100000],[1750,100000],[2256,100000],[9346,100000],[785,100000],[9727,100000],[3055,100000],[3857,100000],[2036,100000],[9639,100000],[3903,100000],[5464,100000],[3225,100000],[7074,100000],[9875,100000],[3608,100000],[2883,100000],[4148,100000],[8680,100000],[1219,100000],[5923,100000],[1602,100000],[2034,100000],[4815,100000],[9275,100000],[8041,100000],[7814,100000],[3451,100000],[3061,100000],[3417,100000],[953,100000],[8845,100000],[6771,100000],[5395,100000],[25,100000],[6039,100000],[5180,100000],[2943,100000],[4853,100000],[7278,100000],[6517,100000],[8070,100000],[4363,100000],[8419,100000],[7793,100000],[6632,100000],[3641,100000],[1196,100000],[1452,100000],[4202,100000],[9063,100000],[1755,100000],[3695,100000],[2366,100000],[7516,100000],[2674,100000],[7170,100000],[6798,100000],[4224,100000],[7773,100000],[5076,100000],[5616,100000],[5095,100000],[4513,100000],[766,100000],[8960,100000],[427,100000],[3716,100000],[218,100000],[6518,100000],[7115,100000],[8091,100000],[3022,100000],[5689,100000],[4393,100000],[9333,100000],[8082,100000],[8849,100000],[8432,100000],[751,100000],[5215,100000],[6685,100000],[9726,100000],[6036,100000],[5726,100000],[8098,100000],[9154,100000],[4685,100000],[9526,100000],[1274,100000],[1608,100000],[5527,100000],[6016,100000],[7572,100000],[3778,100000],[2837,100000],[6418,100000],[4911,100000],[3952,100000],[5165,100000],[8697,100000],[1514,100000],[8906,100000],[3105,100000],[7929,100000],[4531,100000],[9078,100000],[796,100000],[6613,100000],[9807,100000],[6869,100000],[1081,100000],[8728,100000],[6020,100000],[1500,100000],[4313,100000],[2219,100000],[9107,100000],[6325,100000],[1296,100000],[7539,100000],[7501,100000],[8117,100000],[4428,100000],[3851,100000],[6098,100000],[5252,100000],[8477,100000],[2854,100000],[9158,100000],[268,100000],[2396,100000],[1366,100000],[6443,100000],[3083,100000],[3843,100000],[4157,100000],[6676,100000],[2918,100000],[6468,100000],[1235,100000],[1911,100000],[411,100000],[9769,100000],[2621,100000],[9198,100000],[9823,100000],[7237,100000],[2881,100000],[7645,100000],[9625,100000],[1373,100000],[2253,100000],[6930,100000],[2581,100000],[8746,100000],[3904,100000],[8581,100000],[7400,100000],[5921,100000],[2526,100000],[9925,100000],[1618,100000],[1133,100000],[4612,100000],[9025,100000],[5508,100000],[2430,100000],[8355,100000],[4038,100000],[7665,100000],[3474,100000],[5335,100000],[19,100000],[9167,100000],[6088,100000],[7920,100000],[5539,100000],[7547,100000],[9931,100000],[3752,100000],[8033,100000],[8608,100000],[8740,100000],[1055,100000],[5709,100000],[8449,100000],[5507,100000],[4282,100000],[9385,100000],[6153,100000],[6475,100000],[4194,100000],[2279,100000],[7692,100000],[5813,100000],[7797,100000],[6202,100000],[2271,100000],[9087,100000],[5457,100000],[9732,100000],[617,100000],[2963,100000],[485,100000],[3533,100000],[6465,100000],[1854,100000],[8818,100000],[389,100000],[1524,100000],[6314,100000],[4884,100000],[7916,100000],[977,100000],[958,100000],[7618,100000],[7714,100000],[2626,100000],[7627,100000],[634,100000],[1453,100000],[7316,100000],[3833,100000],[8234,100000],[4673,100000],[4580,100000],[4502,100000],[8651,100000],[9793,100000],[779,100000],[6808,100000],[6622,100000],[8962,100000],[2216,100000],[4387,100000],[4861,100000],[756,100000],[2650,100000],[832,100000],[4037,100000],[4200,100000],[8533,100000],[6534,100000],[1473,100000],[3049,100000],[1574,100000],[7298,100000],[8768,100000],[3117,100000],[2191,100000],[8199,100000],[7271,100000],[9011,100000],[7428,100000],[6706,100000],[8896,100000],[5957,100000],[6720,100000],[2189,100000],[8658,100000],[4276,100000],[931,100000],[3120,100000],[6118,100000],[5717,100000],[6358,100000],[1551,100000],[7461,100000],[3005,100000],[3360,100000],[8200,100000],[1374,100000],[315,100000],[9642,100000],[9978,100000],[7884,100000],[6238,100000],[3925,100000],[3743,100000],[281,100000],[9571,100000],[29,100000],[8501,100000],[7310,100000],[6727,100000],[3829,100000],[3438,100000],[2993,100000],[7013,100000],[8590,100000],[2251,100000],[4572,100000],[1047,100000],[5475,100000],[7282,100000],[8974,100000],[4650,100000],[8509,100000],[7967,100000],[7938,100000],[2738,100000],[2019,100000],[3911,100000],[9397,100000],[3195,100000],[7834,100000],[3122,100000],[9104,100000],[2198,100000],[4569,100000],[1890,100000],[6464,100000],[5114,100000],[9140,100000],[4139,100000],[1703,100000],[797,100000],[6077,100000],[5708,100000],[6031,100000],[6628,100000],[6550,100000],[8223,100000],[7925,100000],[637,100000],[5030,100000],[9842,100000],[7261,100000],[7254,100000],[5276,100000],[5216,100000],[8572,100000],[1831,100000],[27,100000],[4127,100000],[6421,100000],[375,100000],[481,100000],[4265,100000],[9553,100000],[2576,100000],[5765,100000],[3471,100000],[4030,100000],[5211,100000],[2221,100000],[4482,100000],[8716,100000],[4507,100000],[4173,100000],[7053,100000],[8570,100000],[3870,100000],[4065,100000],[1434,100000],[5748,100000],[417,100000],[5424,100000],[2081,100000],[5426,100000],[9076,100000],[2265,100000],[8673,100000],[9893,100000],[937,100000],[7748,100000],[4206,100000],[1571,100000],[3710,100000],[4648,100000],[4873,100000],[6659,100000],[9541,100000],[3,100000],[6032,100000],[9380,100000],[8220,100000],[7976,100000],[8049,100000],[3878,100000],[5789,100000],[5565,100000],[7631,100000],[8282,100000],[4237,100000],[2126,100000],[7325,100000],[3698,100000],[6843,100000],[3248,100000],[9499,100000],[4144,100000],[5495,100000],[4399,100000],[8146,100000],[6960,100000],[904,100000],[3757,100000],[4101,100000],[1080,100000],[6092,100000],[1909,100000],[4904,100000],[3793,100000],[7112,100000],[1734,100000],[8827,100000],[9521,100000],[3736,100000],[8463,100000],[741,100000],[90,100000],[7247,100000],[763,100000],[6581,100000],[2888,100000],[9321,100000],[132,100000],[2286,100000],[7088,100000],[4017,100000],[6218,100000],[7755,100000],[9394,100000],[3449,100000],[5488,100000],[329,100000],[5170,100000],[3516,100000],[4351,100000],[5935,100000],[74,100000],[3415,100000],[8796,100000],[4367,100000],[346,100000],[4141,100000],[7343,100000],[1566,100000],[9334,100000],[3730,100000],[6809,100000],[5905,100000],[5938,100000],[2654,100000],[7066,100000],[4559,100000],[7718,100000],[8015,100000],[4258,100000],[6797,100000],[6200,100000],[6275,100000],[6657,100000],[489,100000],[3173,100000],[2399,100000],[8997,100000],[6012,100000],[7934,100000],[612,100000],[6826,100000],[1824,100000],[4332,100000],[6274,100000],[7507,100000],[8088,100000],[5459,100000],[2786,100000],[4328,100000],[7114,100000],[7502,100000],[699,100000],[5969,100000],[1970,100000],[4693,100000],[747,100000],[8287,100000],[8320,100000],[1018,100000],[1021,100000],[7694,100000],[6652,100000],[7917,100000],[4544,100000],[8764,100000],[7838,100000],[8982,100000],[7511,100000],[8579,100000],[1024,100000],[9044,100000],[8244,100000],[345,100000],[9933,100000],[9210,100000],[6158,100000],[3562,100000],[3593,100000],[4683,100000],[7962,100000],[3639,100000],[3147,100000],[1120,100000],[3060,100000],[2826,100000],[3052,100000],[140,100000],[5863,100000],[9120,100000],[239,100000],[1101,100000],[8990,100000],[7636,100000],[6405,100000],[3099,100000],[1307,100000],[1821,100000],[6213,100000],[1649,100000],[7201,100000],[7488,100000],[2894,100000],[3411,100000],[4130,100000],[6105,100000],[7174,100000],[3933,100000],[7744,100000],[8592,100000],[7552,100000],[7667,100000],[4414,100000],[9658,100000],[8641,100000],[8790,100000],[6298,100000],[5134,100000],[8443,100000],[4465,100000],[979,100000],[7215,100000],[2402,100000],[7287,100000],[5555,100000],[972,100000],[9815,100000],[8791,100000],[753,100000],[8115,100000],[1410,100000],[4154,100000],[5369,100000],[6286,100000],[7554,100000],[5569,100000],[621,100000],[4071,100000],[6492,100000],[3390,100000],[2043,100000],[7983,100000],[3979,100000],[6601,100000],[5791,100000],[7086,100000],[7342,100000],[5107,100000],[5925,100000],[5396,100000],[851,100000],[2188,100000],[3568,100000],[713,100000],[7931,100000],[5346,100000],[8754,100000],[1858,100000],[1901,100000],[2565,100000],[4096,100000],[7778,100000],[1912,100000],[6981,100000],[288,100000],[5815,100000],[8147,100000],[6598,100000],[7260,100000],[48,100000],[9418,100000],[4988,100000],[3255,100000],[4019,100000],[9390,100000],[3997,100000],[3899,100000],[8421,100000],[9510,100000],[5007,100000],[3655,100000],[9611,100000],[3912,100000],[208,100000],[6538,100000],[5159,100000],[8646,100000],[5192,100000],[51,100000],[2832,100000],[2865,100000],[4452,100000],[9852,100000],[7945,100000],[278,100000],[6138,100000],[2882,100000],[9272,100000],[9495,100000],[2205,100000],[569,100000],[7822,100000],[2382,100000],[7396,100000],[5454,100000],[5802,100000],[6090,100000],[9570,100000],[2732,100000],[8389,100000],[6075,100000],[6389,100000],[3931,100000],[9142,100000],[5006,100000],[7212,100000],[909,100000],[9748,100000],[3656,100000],[5524,100000],[9241,100000],[5455,100000],[6221,100000],[7063,100000],[9930,100000],[3540,100000],[2766,100000],[5580,100000],[5100,100000],[3658,100000],[5147,100000],[6778,100000],[6269,100000],[4798,100000],[7377,100000],[1607,100000],[9274,100000],[2258,100000],[3685,100000],[7421,100000],[566,100000],[5944,100000],[2334,100000],[9523,100000],[9382,100000],[3654,100000],[5441,100000],[9946,100000],[1591,100000],[5374,100000],[1053,100000],[4167,100000],[7597,100000],[3351,100000],[8308,100000],[1077,100000],[9030,100000],[6072,100000],[7807,100000],[1386,100000],[8539,100000],[595,100000],[4439,100000],[7477,100000],[9129,100000],[7639,100000],[5113,100000],[2908,100000],[610,100000],[7698,100000],[4233,100000],[4230,100000],[3040,100000],[7498,100000],[5210,100000],[7877,100000],[7422,100000],[3416,100000],[9057,100000],[6078,100000],[6847,100000],[5434,100000],[8435,100000],[6664,100000],[7405,100000],[2105,100000],[7775,100000],[6387,100000],[4441,100000],[2977,100000],[478,100000],[7769,100000],[9406,100000],[273,100000],[762,100000],[4106,100000],[9308,100000],[6618,100000],[4573,100000],[7365,100000],[4774,100000],[3526,100000],[1357,100000],[1739,100000],[7413,100000],[9715,100000],[4608,100000],[264,100000],[6359,100000],[2924,100000],[7084,100000],[5988,100000],[3353,100000],[106,100000],[7333,100000],[6872,100000],[9676,100000],[4790,100000],[9607,100000],[2438,100000],[966,100000],[8644,100000],[6746,100000],[1598,100000],[7448,100000],[8066,100000],[3802,100000],[9302,100000],[1953,100000],[6935,100000],[5041,100000],[2338,100000],[1941,100000],[7980,100000],[5167,100000],[8373,100000],[7812,100000],[771,100000],[2511,100000],[5479,100000],[6009,100000],[5566,100000],[9650,100000],[6041,100000],[1955,100000],[9619,100000],[9969,100000],[7990,100000],[6821,100000],[1180,100000],[2003,100000],[9965,100000],[5810,100000],[1527,100000],[1129,100000],[8215,100000],[134,100000],[1810,100000],[6055,100000],[1217,100000],[916,100000],[3689,100000],[1999,100000],[7100,100000],[9246,100000],[6351,100000],[6547,100000],[4707,100000],[2451,100000],[8959,100000],[969,100000],[1543,100000],[1128,100000],[6625,100000],[9545,100000],[7886,100000],[4641,100000],[5928,100000],[5719,100000],[987,100000],[8177,100000],[7957,100000],[1188,100000],[6520,100000],[7320,100000],[9605,100000],[4910,100000],[7620,100000],[6233,100000],[4407,100000],[3707,100000],[7545,100000],[4665,100000],[2272,100000],[5681,100000],[5334,100000],[7453,100000],[8856,100000],[5141,100000],[5831,100000],[6179,100000],[7145,100000],[4067,100000],[4445,100000],[5593,100000],[3518,100000],[3177,100000],[5561,100000],[526,100000],[331,100000],[4420,100000],[9755,100000],[3701,100000],[7602,100000],[6167,100000],[9772,100000],[6902,100000],[527,100000],[7866,100000],[4832,100000],[2356,100000],[9583,100000],[5367,100000],[191,100000],[129,100000],[5558,100000],[8408,100000],[2388,100000],[9548,100000],[1709,100000],[5026,100000],[8263,100000],[7936,100000],[1605,100000],[2718,100000],[154,100000],[1672,100000],[3740,100000],[9431,100000],[372,100000],[4055,100000],[1887,100000],[5290,100000],[1981,100000],[3497,100000],[5874,100000],[5168,100000],[8315,100000],[4792,100000],[2345,100000],[8056,100000],[2427,100000],[1600,100000],[5302,100000],[8647,100000],[3768,100000],[9513,100000],[6564,100000],[3569,100000],[1827,100000],[1683,100000],[7362,100000],[6182,100000],[3887,100000],[3910,100000],[5451,100000],[7873,100000],[6485,100000],[5576,100000],[4238,100000],[609,100000],[4864,100000],[3018,100000],[2688,100000],[5830,100000],[7262,100000],[5642,100000],[8485,100000],[5196,100000],[3384,100000],[1460,100000],[9696,100000],[9735,100000],[3946,100000],[2270,100000],[7433,100000],[3297,100000],[3338,100000],[5010,100000],[4,100000],[26,100000],[1417,100000],[396,100000],[1604,100000],[2142,100000],[5281,100000],[3334,100000],[7410,100000],[217,100000],[5703,100000],[3241,100000],[3570,100000],[3057,100000],[8292,100000],[4545,100000],[6996,100000],[842,100000],[959,100000],[8466,100000],[9303,100000],[7210,100000],[5556,100000],[9587,100000],[2974,100000],[1239,100000],[5531,100000],[8491,100000],[652,100000],[1184,100000],[1555,100000],[1051,100000],[4923,100000],[9074,100000],[5896,100000],[6669,100000],[4973,100000],[1959,100000],[825,100000],[1549,100000],[649,100000],[491,100000],[1881,100000],[6875,100000],[4587,100000],[9604,100000],[9957,100000],[4902,100000],[2108,100000],[9525,100000],[2821,100000],[2168,100000],[4305,100000],[6049,100000],[7899,100000],[4042,100000],[6253,100000],[6519,100000],[4376,100000],[4462,100000],[9237,100000],[1771,100000],[6264,100000],[6541,100000],[5847,100000],[6732,100000],[8037,100000],[7856,100000],[6321,100000],[8615,100000],[2782,100000],[4960,100000],[8713,100000],[1352,100000],[8368,100000],[5036,100000],[4959,100000],[7480,100000],[2985,100000],[3221,100000],[1776,100000],[7907,100000],[1989,100000],[976,100000],[5263,100000],[2282,100000],[3166,100000],[6261,100000],[7603,100000],[4743,100000],[6668,100000],[4517,100000],[7163,100000],[2517,100000],[5200,100000],[8378,100000],[4964,100000],[8881,100000],[2767,100000],[5644,100000],[276,100000],[3074,100000],[2419,100000],[674,100000],[2580,100000],[1340,100000],[6192,100000],[3066,100000],[853,100000],[5553,100000],[3511,100000],[926,100000],[1847,100000],[1691,100000],[9608,100000],[4191,100000],[1039,100000],[8991,100000],[7892,100000],[9667,100000],[9009,100000],[2729,100000],[7012,100000],[5982,100000],[8732,100000],[3970,100000],[7190,100000],[6874,100000],[9269,100000],[4554,100000],[5287,100000],[5787,100000],[194,100000],[5445,100000],[8156,100000],[6183,100000],[7688,100000],[4571,100000],[6280,100000],[8354,100000],[2114,100000],[7228,100000],[5056,100000],[9300,100000],[1613,100000],[2591,100000],[7939,100000],[5914,100000],[1243,100000],[4927,100000],[6515,100000],[9041,100000],[5509,100000],[3327,100000],[6841,100000],[6881,100000],[1091,100000],[3546,100000],[8222,100000],[5368,100000],[5329,100000],[6146,100000],[8179,100000],[8809,100000],[8254,100000],[7658,100000],[3877,100000],[1799,100000],[9818,100000],[2681,100000],[8104,100000],[2725,100000],[5344,100000],[2287,100000],[9996,100000],[4535,100000],[1630,100000],[5844,100000],[9882,100000],[9294,100000],[2575,100000],[2608,100000],[8569,100000],[5889,100000],[269,100000],[8721,100000],[7444,100000],[8532,100000],[9820,100000],[6425,100000],[4350,100000],[4831,100000],[5800,100000],[5342,100000],[9353,100000],[9291,100000],[3721,100000],[1163,100000],[3079,100000],[1747,100000],[1179,100000],[1100,100000],[8999,100000],[6080,100000],[5780,100000],[5339,100000],[8546,100000],[6838,100000],[7948,100000],[7533,100000],[8973,100000],[9004,100000],[2067,100000],[1444,100000],[34,100000],[4218,100000],[4936,100000],[3203,100000],[4771,100000],[7680,100000],[4135,100000],[1457,100000],[8488,100000],[9668,100000],[1020,100000],[3541,100000],[9219,100000],[3192,100000],[6635,100000],[2046,100000],[8979,100000],[820,100000],[9152,100000],[5799,100000],[2592,100000],[5490,100000],[4358,100000],[1641,100000],[6436,100000],[3865,100000],[906,100000],[582,100000],[1643,100000],[8181,100000],[8518,100000],[5438,100000],[4318,100000],[8302,100000],[5155,100000],[1525,100000],[6560,100000],[6983,100000],[9006,100000],[3726,100000],[9741,100000],[2380,100000],[1411,100000],[2394,100000],[7093,100000],[1623,100000],[4527,100000],[2414,100000],[4201,100000],[8009,100000],[1832,100000],[9527,100000],[3627,100000],[7367,100000],[9139,100000],[6563,100000],[948,100000],[1476,100000],[3853,100000],[9054,100000],[2070,100000],[5869,100000],[5756,100000],[4331,100000],[4575,100000],[7245,100000],[9922,100000],[4589,100000],[3275,100000],[9695,100000],[2461,100000],[6103,100000],[7061,100000],[9844,100000],[1983,100000],[3622,100000],[2041,100000],[5452,100000],[2505,100000],[1612,100000],[8663,100000],[9358,100000],[3634,100000],[8812,100000],[258,100000],[556,100000],[7462,100000],[80,100000],[6299,100000],[6161,100000],[5023,100000],[6396,100000],[8921,100000],[5258,100000],[646,100000],[1312,100000],[2831,100000],[423,100000],[3727,100000],[9849,100000],[1979,100000],[6834,100000],[5933,100000],[7133,100000],[7230,100000],[2280,100000],[8469,100000],[7579,100000],[3735,100000],[339,100000],[3649,100000],[3782,100000],[799,100000],[8402,100000],[2965,100000],[9744,100000],[8243,100000],[7743,100000],[1742,100000],[4807,100000],[4797,100000],[2512,100000],[296,100000],[1930,100000],[2596,100000],[8288,100000],[2610,100000],[8195,100000],[9328,100000],[2666,100000],[3233,100000],[8826,100000],[226,100000],[8730,100000],[1731,100000],[3030,100000],[1103,100000],[8476,100000],[8966,100000],[2239,100000],[4342,100000],[7270,100000],[6112,100000],[6923,100000],[9027,100000],[910,100000],[4562,100000],[1741,100000],[1376,100000],[711,100000],[8271,100000],[70,100000],[1680,100000],[3623,100000],[7416,100000],[4411,100000],[6462,100000],[9532,100000],[8684,100000],[3490,100000],[7069,100000],[6124,100000],[967,100000],[3043,100000],[5916,100000],[8601,100000],[8948,100000],[3763,100000],[7126,100000],[54,100000],[2934,100000],[4085,100000],[1603,100000],[7124,100000],[7982,100000],[7077,100000],[9606,100000],[344,100000],[6861,100000],[3784,100000],[3723,100000],[4089,100000],[849,100000],[8894,100000],[2243,100000],[3535,100000],[8112,100000],[7935,100000],[1067,100000],[9214,100000],[8604,100000],[5453,100000],[3186,100000],[1297,100000],[5631,100000],[7867,100000],[2497,100000],[907,100000],[9049,100000],[2431,100000],[4811,100000],[2720,100000],[8985,100000],[259,100000],[6390,100000],[6289,100000],[6611,100000],[1075,100000],[9067,100000],[2091,100000],[2029,100000],[6789,100000],[9924,100000],[64,100000],[399,100000],[3034,100000],[8540,100000],[8549,100000],[1760,100000],[7472,100000],[6369,100000],[1534,100000],[5793,100000],[3380,100000],[3605,100000],[9182,100000],[287,100000],[8317,100000],[94,100000],[9221,100000],[8372,100000],[2802,100000],[1580,100000],[642,100000],[5401,100000],[4446,100000],[4162,100000],[2710,100000],[6203,100000],[1682,100000],[2263,100000],[4757,100000],[6402,100000],[5317,100000],[3668,100000],[3170,100000],[7291,100000],[4555,100000],[8418,100000],[3095,100000],[1586,100000],[9475,100000],[9352,100000],[1343,100000],[6370,100000],[2897,100000],[6017,100000],[4379,100000],[2052,100000],[6448,100000],[7526,100000],[5094,100000],[2201,100000],[8951,100000],[9307,100000],[4357,100000],[3472,100000],[1695,100000],[1883,100000],[9653,100000],[5444,100000],[1937,100000],[9322,100000],[1552,100000],[2585,100000],[7395,100000],[9151,100000],[4827,100000],[844,100000],[9123,100000],[9311,100000],[335,100000],[8708,100000],[103,100000],[5790,100000],[3461,100000],[422,100000],[4894,100000],[215,100000],[4744,100000],[660,100000],[2713,100000],[9770,100000],[5996,100000],[3013,100000],[7504,100000],[8246,100000],[5131,100000],[4483,100000],[5540,100000],[4242,100000],[1442,100000],[5379,100000],[3469,100000],[7904,100000],[1830,100000],[3309,100000],[5271,100000],[8850,100000],[3378,100000],[9444,100000],[721,100000],[8484,100000],[8340,100000],[337,100000],[1853,100000],[3308,100000],[1173,100000],[7207,100000],[5198,100000],[9577,100000],[7561,100000],[9101,100000],[4178,100000],[9710,100000],[1435,100000],[8682,100000],[410,100000],[6770,100000],[515,100000],[3553,100000],[7765,100000],[2323,100000],[1773,100000],[1375,100000],[8109,100000],[3368,100000],[7078,100000],[2383,100000],[2321,100000],[7329,100000],[7995,100000],[9761,100000],[8247,100000],[3042,100000],[9194,100000],[3406,100000],[589,100000],[7808,100000],[7672,100000],[6698,100000],[4495,100000],[8386,100000],[7885,100000],[5948,100000],[2057,100000],[6259,100000],[3385,100000],[1424,100000],[8834,100000],[2844,100000],[2900,100000],[4686,100000],[3507,100000],[4883,100000],[3795,100000],[3731,100000],[3339,100000],[3064,100000],[1071,100000],[5809,100000],[5350,100000],[4041,100000],[9754,100000],[7152,100000],[1178,100000],[8361,100000],[324,100000],[6787,100000],[181,100000],[9401,100000],[7759,100000],[6101,100000],[8942,100000],[9798,100000],[9287,100000],[5068,100000],[7615,100000],[5352,100000],[1237,100000],[5178,100000],[7588,100000],[7601,100000],[8160,100000],[3218,100000],[8134,100000],[9177,100000],[9832,100000],[5051,100000],[4840,100000],[4782,100000],[5654,100000],[1915,100000],[6831,100000],[4066,100000],[7481,100000],[8771,100000],[9868,100000],[156,100000],[7674,100000],[9682,100000],[4755,100000],[8217,100000],[5292,100000],[1197,100000],[7426,100000],[6856,100000],[7558,100000],[6100,100000],[4976,100000],[2803,100000],[3054,100000],[4844,100000],[2420,100000],[2739,100000],[8610,100000],[7835,100000],[9509,100000],[8563,100000],[1000,100000],[3420,100000],[6793,100000],[2422,100000],[1532,100000],[5213,100000],[1287,100000],[4625,100000],[2468,100000],[3624,100000],[9114,100000],[8458,100000],[5039,100000],[2671,100000],[4695,100000],[2000,100000],[1069,100000],[965,100000],[7436,100000],[4812,100000],[4822,100000],[2331,100000],[3942,100000],[8323,100000],[4013,100000],[21,100000],[4676,100000],[4426,100000],[6033,100000],[7246,100000],[1988,100000],[1198,100000],[6526,100000],[4845,100000],[5805,100000],[3196,100000],[9094,100000],[3382,100000],[928,100000],[5987,100000],[8838,100000],[6050,100000],[15,100000],[2700,100000],[607,100000],[4285,100000],[434,100000],[5289,100000],[2884,100000],[6963,100000],[1645,100000],[6630,100000],[1504,100000],[6616,100000],[2969,100000],[7312,100000],[9632,100000],[5409,100000],[7673,100000],[1109,100000],[5296,100000],[8888,100000],[6461,100000],[4561,100000],[5480,100000],[2405,100000],[45,100000],[5240,100000],[2459,100000],[1816,100000],[3810,100000],[1045,100000],[338,100000],[6894,100000],[9402,100000],[6607,100000],[225,100000],[5127,100000],[8555,100000],[2759,100000],[3258,100000],[7248,100000],[5498,100000],[4891,100000],[1721,100000],[5545,100000],[9819,100000],[6456,100000],[5313,100000],[8159,100000],[3367,100000],[7327,100000],[3344,100000],[9408,100000],[7861,100000],[4702,100000],[7120,100000],[3140,100000],[3092,100000],[8267,100000],[5260,100000],[3050,100000],[2078,100000],[8326,100000],[4833,100000],[9721,100000],[4114,100000],[3590,100000],[6415,100000],[3088,100000],[3404,100000],[4490,100000],[7297,100000],[1240,100000],[5898,100000],[2507,100000],[9804,100000],[7339,100000],[5474,100000],[4334,100000],[4668,100000],[4422,100000],[3860,100000],[2866,100000],[6832,100000],[4738,100000],[8290,100000],[9349,100000],[4805,100000],[7475,100000],[1094,100000],[6910,100000],[727,100000],[2797,100000],[8844,100000],[9415,100000],[7979,100000],[8158,100000],[8375,100000],[7590,100000],[5571,100000],[475,100000],[3544,100000],[7678,100000],[9589,100000],[8425,100000],[6743,100000],[227,100000],[4002,100000],[5032,100000],[6300,100000],[4512,100000],[9517,100000],[3178,100000],[3922,100000],[8279,100000],[3974,100000],[6132,100000],[7741,100000],[5657,100000],[3110,100000],[68,100000],[807,100000],[3819,100000],[5779,100000],[6319,100000],[8123,100000],[3991,100000],[4418,100000],[8196,100000],[4591,100000],[7955,100000],[1974,100000],[872,100000],[8605,100000],[9994,100000],[464,100000],[3643,100000],[1147,100000],[6768,100000],[2252,100000],[4213,100000],[6784,100000],[8006,100000],[9574,100000],[3937,100000],[3890,100000],[8614,100000],[7446,100000],[5607,100000],[2435,100000],[742,100000],[9547,100000],[2145,100000],[692,100000],[3980,100000],[4408,100000],[9379,100000],[9736,100000],[347,100000],[5533,100000],[5423,100000],[5151,100000],[9892,100000],[9507,100000],[4643,100000],[7038,100000],[3816,100000],[5186,100000],[1929,100000],[8143,100000],[4352,100000],[2299,100000],[9817,100000],[6306,100000],[7566,100000],[9531,100000],[1896,100000],[2828,100000],[4252,100000],[6773,100000],[1046,100000],[4982,100000],[7418,100000],[4926,100000],[9071,100000],[6363,100000],[1001,100000],[5775,100000],[4596,100000],[9716,100000],[314,100000],[9037,100000],[5372,100000],[1794,100000],[5890,100000],[701,100000],[8950,100000],[9723,100000],[8627,100000],[3780,100000],[3790,100000],[43,100000],[8083,100000],[5236,100000],[3770,100000],[5340,100000],[3190,100000],[6759,100000],[2536,100000],[2563,100000],[6896,100000],[604,100000],[9790,100000],[8270,100000],[8328,100000],[381,100000],[2375,100000],[6700,100000],[8785,100000],[7998,100000],[3229,100000],[4005,100000],[2123,100000],[3508,100000],[5937,100000],[3470,100000],[6322,100000],[9086,100000],[1032,100000],[7109,100000],[1014,100000],[8274,100000],[8876,100000],[8073,100000],[6905,100000],[1674,100000],[8227,100000],[4893,100000],[4457,100000],[1576,100000],[4735,100000],[9635,100000],[4008,100000],[7239,100000],[307,100000],[5472,100000],[5722,100000],[2315,100000],[1048,100000],[3153,100000],[5140,100000],[6758,100000],[12,100000],[8121,100000],[9026,100000],[141,100000],[9,100000],[6845,100000],[2562,100000],[9173,100000],[3989,100000],[7263,100000],[4149,100000],[7438,100000],[585,100000],[9644,100000],[6144,100000],[2446,100000],[9631,100000],[2478,100000],[8573,100000],[6063,100000],[5075,100000],[325,100000],[3638,100000],[2743,100000],[107,100000],[1837,100000],[7180,100000],[7865,100000],[7827,100000],[6823,100000],[3732,100000],[1795,100000],[3880,100000],[6820,100000],[9065,100000],[2889,100000],[9220,100000],[9776,100000],[5431,100000],[1548,100000],[9255,100000],[2747,100000],[7622,100000],[4302,100000],[1512,100000],[3861,100000],[4118,100000],[7569,100000],[3223,100000],[3130,100000],[8506,100000],[3441,100000],[2625,100000],[6516,100000],[5822,100000],[6168,100000],[4724,100000],[8341,100000],[9551,100000],[2774,100000],[7335,100000],[9252,100000],[6651,100000],[9601,100000],[3753,100000],[4120,100000],[2385,100000],[4713,100000],[9636,100000],[445,100000],[3659,100000],[5649,100000],[4400,100000],[1360,100000],[3495,100000],[6876,100000],[3277,100000],[6028,100000],[5425,100000],[8061,100000],[1519,100000],[5231,100000],[4503,100000],[4097,100000],[6551,100000],[5620,100000],[1659,100000],[1634,100000],[786,100000],[1,100000],[1774,100000],[3565,100000],[3611,100000],[3222,100000],[1885,100000],[5548,100000],[1033,100000],[7304,100000],[1151,100000],[5108,100000],[9458,100000],[2773,100000],[4877,100000],[6865,100000],[4954,100000],[1558,100000],[2535,100000],[9519,100000],[7019,100000],[8835,100000],[8668,100000],[125,100000],[8233,100000],[6577,100000],[2436,100000],[4283,100000],[2214,100000],[9034,100000],[4896,100000],[2846,100000],[3898,100000],[9777,100000],[715,100000],[8519,100000],[1222,100000],[8406,100000],[2284,100000],[2952,100000],[3531,100000],[8332,100000],[7486,100000],[2776,100000],[2317,100000],[6899,100000],[7828,100000],[3466,100000],[6921,100000],[8840,100000],[5832,100000],[1950,100000],[6374,100000],[4455,100000],[9023,100000],[565,100000],[9806,100000],[3405,100000],[3158,100000],[3135,100000],[3249,100000],[2139,100000],[452,100000],[6320,100000],[583,100000],[3635,100000],[412,100000],[5088,100000],[5851,100000],[2075,100000],[6774,100000],[6521,100000],[5724,100000],[2484,100000],[3978,100000],[5932,100000],[183,100000],[7217,100000],[2845,100000],[6139,100000],[4956,100000],[7324,100000],[6901,100000],[5502,100000],[9384,100000],[4863,100000],[9326,100000],[8086,100000],[312,100000],[2922,100000],[4961,100000],[4783,100000],[6466,100000],[6172,100000],[2248,100000],[8808,100000],[9386,100000],[6587,100000],[2378,100000],[7954,100000],[4214,100000],[2172,100000],[8829,100000],[1817,100000],[4583,100000],[668,100000],[3713,100000],[9471,100000],[1969,100000],[1785,100000],[3154,100000],[2477,100000],[1609,100000],[6327,100000],[4595,100000],[6953,100000],[1503,100000],[1049,100000],[6535,100000],[448,100000],[4918,100000],[1234,100000],[4151,100000],[9069,100000],[7777,100000],[6801,100000],[6346,100000],[3509,100000],[8729,100000],[9679,100000],[8404,100000],[2745,100000],[3169,100000],[9257,100000],[8763,100000],[2701,100000],[8678,100000],[5550,100000],[8907,100000],[7144,100000],[8945,100000],[5365,100000],[1327,100000],[4773,100000],[8918,100000],[744,100000],[2549,100000],[1898,100000],[855,100000],[6452,100000],[5004,100000],[4860,100000],[1418,100000],[6603,100000],[1393,100000],[3976,100000],[4585,100000],[1620,100000],[2546,100000],[6880,100000],[4499,100000],[633,100000],[8594,100000],[8745,100000],[6837,100000],[2116,100000],[406,100000],[9202,100000],[5199,100000],[6692,100000],[9146,100000],[8237,100000],[1766,100000],[2678,100000],[7490,100000],[6648,100000],[985,100000],[9108,100000],[128,100000],[179,100000],[2194,100000],[8050,100000],[4478,100000],[144,100000],[4871,100000],[3672,100000],[4875,100000],[1300,100000],[40,100000],[8784,100000],[9939,100000],[1214,100000],[9919,100000],[7513,100000],[9808,100000],[9263,100000],[2407,100000],[889,100000],[665,100000],[8314,100000],[6681,100000],[4179,100000],[9907,100000],[7794,100000],[2311,100000],[5872,100000],[7898,100000],[6666,100000],[4461,100000],[1012,100000],[7359,100000],[9796,100000],[2751,100000],[4046,100000],[4056,100000],[5483,100000],[2274,100000],[6398,100000],[8136,100000],[3073,100000],[9433,100000],[9014,100000],[4099,100000],[2537,100000],[9268,100000],[3866,100000],[5554,100000],[8442,100000],[2047,100000],[3871,100000],[3370,100000],[938,100000],[5138,100000],[9490,100000],[2411,100000],[4841,100000],[912,100000],[7813,100000],[5137,100000],[3224,100000],[2412,100000],[8531,100000],[8300,100000],[1158,100000],[265,100000],[911,100000],[4485,100000],[5306,100000],[3813,100000],[204,100000],[9618,100000],[126,100000],[1050,100000],[6673,100000],[9788,100000],[6089,100000],[5096,100000],[5515,100000],[5692,100000],[9534,100000],[624,100000],[8722,100000],[3159,100000],[3750,100000],[206,100000],[4080,100000],[6198,100000],[5774,100000],[4297,100000],[4373,100000],[8434,100000],[3006,100000],[640,100000],[3262,100000],[8275,100000],[1057,100000],[8686,100000],[6529,100000],[7295,100000],[8426,100000],[4424,100000],[6973,100000],[7211,100000],[6470,100000],[467,100000],[5064,100000],[4423,100000],[419,100000],[890,100000],[7364,100000],[2516,100000],[6215,100000],[39,100000],[6126,100000],[2226,100000],[7175,100000],[3129,100000],[4058,100000],[4057,100000],[5257,100000],[8711,100000],[3299,100000],[9810,100000],[2733,100000],[2949,100000],[9435,100000],[954,100000],[7193,100000],[5164,100000],[2112,100000],[5387,100000],[3926,100000],[243,100000],[5715,100000],[5936,100000],[733,100000],[6986,100000],[2319,100000],[2304,100000],[3948,100000],[2246,100000],[8988,100000],[511,100000],[8262,100000],[8751,100000],[7977,100000],[2876,100000],[2329,100000],[1928,100000],[6444,100000],[7082,100000],[6281,100000],[6197,100000],[8736,100000],[3671,100000],[3080,100000],[9740,100000],[137,100000],[3968,100000],[2641,100000],[4849,100000],[5573,100000],[1908,100000],[6944,100000],[7059,100000],[3274,100000],[1337,100000],[9267,100000],[5992,100000],[7380,100000],[8385,100000],[1927,100000],[2787,100000],[3111,100000],[6871,100000],[1964,100000],[9648,100000],[2275,100000],[6690,100000],[4493,100000],[8870,100000],[5294,100000],[9626,100000],[4796,100000],[4090,100000],[7705,100000],[4706,100000],[5501,100000],[2682,100000],[3588,100000],[6150,100000],[1223,100000],[6578,100000],[4171,100000],[7833,100000],[6524,100000],[311,100000],[6108,100000],[4688,100000],[9238,100000],[9460,100000],[8347,100000],[9853,100000],[6305,100000],[79,100000],[9514,100000],[5677,100000],[6779,100000],[3945,100000],[7473,100000],[7625,100000],[2611,100000],[4950,100000],[574,100000],[3492,100000],[3113,100000],[9708,100000],[5879,100000],[7399,100000],[2470,100000],[9728,100000],[729,100000],[4187,100000],[3825,100000],[4932,100000],[9825,100000],[2775,100000],[7005,100000],[3845,100000],[6169,100000],[353,100000],[5794,100000],[9416,100000],[9437,100000],[5458,100000],[1761,100000],[6301,100000],[8293,100000],[4219,100000],[4799,100000],[7058,100000],[4828,100000],[7094,100000],[4011,100000],[3109,100000],[1725,100000],[8841,100000],[4726,100000],[9019,100000],[5755,100000],[1743,100000],[5408,100000],[1290,100000],[4244,100000],[2685,100000],[3572,100000],[4924,100000],[7612,100000],[3581,100000],[8800,100000],[594,100000],[7531,100000],[4617,100000],[7562,100000],[4800,100000],[8628,100000],[6345,100000],[6232,100000],[2357,100000],[754,100000],[3666,100000],[185,100000],[285,100000],[4047,100000],[1220,100000],[8741,100000],[5414,100000],[2838,100000],[5720,100000],[8930,100000],[7978,100000],[8972,100000],[2618,100000],[9730,100000],[3558,100000],[5157,100000],[279,100000],[5826,100000],[7786,100000],[9555,100000],[2704,100000],[6380,100000],[2096,100000],[8624,100000],[4909,100000],[6324,100000],[6748,100000],[4093,100000],[5687,100000],[1232,100000],[7928,100000],[780,100000],[7179,100000],[1943,100000],[10,100000],[9231,100000],[870,100000],[1693,100000],[2010,100000],[9472,100000],[113,100000],[187,100000],[8571,100000],[2850,100000],[7611,100000],[3920,100000],[4965,100000],[679,100000],[6293,100000],[7007,100000],[7118,100000],[8321,100000],[9373,100000],[4346,100000],[6979,100000],[4993,100000],[9535,100000],[1347,100000],[7423,100000],[4429,100000],[6034,100000],[5543,100000],[8878,100000],[2981,100000],[1601,100000],[46,100000],[3069,100000],[6427,100000],[6962,100000],[9400,100000],[6081,100000],[5741,100000],[9698,100000],[3876,100000],[2695,100000],[6764,100000],[6713,100000],[9449,100000],[3194,100000],[6228,100000],[7138,100000],[9112,100000],[2222,100000],[6791,100000],[758,100000],[169,100000],[8102,100000],[3296,100000],[7206,100000],[378,100000],[4010,100000],[4316,100000],[3996,100000],[2567,100000],[2531,100000],[8756,100000],[8846,100000],[4628,100000],[5622,100000],[8174,100000],[8052,100000],[9942,100000],[7242,100000],[2186,100000],[9909,100000],[2397,100000],[2098,100000],[9423,100000],[4353,100000],[5176,100000],[3943,100000],[4241,100000],[4710,100000],[5219,100000],[7485,100000],[6074,100000],[1322,100000],[4966,100000],[7570,100000],[1149,100000],[4674,100000],[6085,100000],[1006,100000],[6225,100000],[9392,100000],[2703,100000],[4943,100000],[9186,100000],[2643,100000],[7224,100000],[9660,100000],[7181,100000],[3642,100000],[9209,100000],[8304,100000],[2711,100000],[426,100000],[6600,100000],[6855,100000],[9360,100000],[3201,100000],[9033,100000],[241,100000],[7185,100000],[1954,100000],[1371,100000],[2373,100000],[980,100000],[9443,100000],[6545,100000],[1561,100000],[5101,100000],[1675,100000],[6739,100000],[9898,100000],[3800,100000],[8413,100000],[1212,100000],[1610,100000],[5497,100000],[9296,100000],[9344,100000],[3560,100000],[6038,100000],[1139,100000],[207,100000],[8089,100000],[3984,100000],[1892,100000],[5432,100000],[3936,100000],[2457,100000],[3106,100000],[2951,100000],[2488,100000],[7357,100000],[7166,100000],[2178,100000],[1518,100000],[613,100000],[4835,100000],[6548,100000],[3270,100000],[3879,100000],[8226,100000],[8975,100000],[9977,100000],[6023,100000],[8622,100000],[7624,100000],[2227,100000],[4576,100000],[2342,100000],[3238,100000],[6514,100000],[3660,100000],[9052,100000],[8788,100000],[9162,100000],[4488,100000],[708,100000],[887,100000],[7785,100000],[6251,100000],[1660,100000],[9329,100000],[6969,100000],[2068,100000],[3596,100000],[4268,100000],[201,100000],[1126,100000],[376,100000],[8963,100000],[9201,100000],[3011,100000],[1590,100000],[3434,100000],[2410,100000],[8370,100000],[3098,100000],[92,100000],[69,100000],[3268,100000],[2939,100000],[6399,100000],[5816,100000],[5641,100000],[4892,100000],[2155,100000],[5849,100000],[9940,100000],[6047,100000],[1206,100000],[2890,100000],[6683,100000],[9409,100000],[7669,100000],[425,100000],[2964,100000],[3328,100000],[1441,100000],[263,100000],[5209,100000],[5776,100000],[6027,100000],[9138,100000],[6961,100000],[8162,100000],[5699,100000],[4160,100000],[8129,100000],[8069,100000],[4486,100000],[4180,100000],[8629,100000],[8696,100000],[1900,100000],[8454,100000],[84,100000],[1396,100000],[9795,100000],[6417,100000],[9253,100000],[9914,100000],[7767,100000],[4449,100000],[7663,100000],[2959,100000],[343,100000],[2768,100000],[2568,100000],[9040,100000],[4052,100000],[1718,100000],[9372,100000],[8733,100000],[9794,100000],[5676,100000],[3437,100000],[2699,100000],[6549,100000],[7915,100000],[9995,100000],[828,100000],[2508,100000],[8461,100000],[6990,100000],[9010,100000],[8251,100000],[2858,100000],[2929,100000],[625,100000],[7684,100000],[3486,100000],[4787,100000],[8623,100000],[5876,100000],[9111,100000],[8007,100000],[7720,100000],[2860,100000],[3340,100000],[6825,100000],[2552,100000],[300,100000],[9315,100000],[9183,100000],[4754,100000],[98,100000],[5054,100000],[1010,100000],[7781,100000],[9883,100000],[7484,100000],[9578,100000],[4526,100000],[530,100000],[9684,100000],[7959,100000],[1615,100000],[8333,100000],[221,100000],[5122,100000],[7337,100000],[1054,100000],[8450,100000],[9016,100000],[9915,100000],[3097,100000],[3756,100000],[384,100000],[3537,100000],[8718,100000],[1463,100000],[3988,100000],[9438,100000],[2945,100000],[1105,100000],[2622,100000],[2072,100000],[5609,100000],[3598,100000],[5256,100000],[2229,100000],[7937,100000],[4915,100000],[1651,100000],[2464,100000],[7604,100000],[2555,100000],[1972,100000],[1676,100000],[730,100000],[3848,100000],[550,100000],[5002,100000],[5266,100000],[7683,100000],[4885,100000],[9215,100000],[2788,100000],[2491,100000],[4605,100000],[9350,100000],[1324,100000],[3927,100000],[8537,100000],[5729,100000],[1437,100000],[9024,100000],[7189,100000],[681,100000],[833,100000],[3419,100000],[6348,100000],[1095,100000],[6423,100000],[7047,100000],[9870,100000],[942,100000],[1713,100000],[5567,100000],[7697,100000],[456,100000],[88,100000],[8927,100000],[7862,100000],[2004,100000],[1141,100000],[9518,100000],[7257,100000],[7613,100000],[7146,100000],[4456,100000],[5827,100000],[9757,100000],[7751,100000],[6946,100000],[4690,100000],[9256,100000],[1714,100000],[816,100000],[235,100000],[2485,100000],[313,100000],[6977,100000],[1783,100000],[1717,100000],[2989,100000],[8128,100000],[2817,100000],[8660,100000],[2795,100000],[3538,100000],[9485,100000],[3921,100000],[9612,100000],[9264,100000],[91,100000],[5384,100000],[6506,100000],[5391,100000],[4778,100000],[2288,100000],[8637,100000],[1788,100000],[2180,100000],[1165,100000],[109,100000],[8727,100000],[1023,100000],[2391,100000],[4349,100000],[4477,100000],[6980,100000],[8377,100000],[1581,100000],[2946,100000],[830,100000],[2086,100000],[9711,100000],[6810,100000],[1421,100000],[1537,100000],[2867,100000],[1182,100000],[7251,100000],[9763,100000],[4663,100000],[4671,100000],[3826,100000],[8980,100000],[7435,100000],[8342,100000],[3688,100000],[2683,100000],[2298,100000],[5324,100000],[6839,100000],[1161,100000],[374,100000],[1074,100000],[1957,100000],[4498,100000],[3951,100000],[895,100000],[7637,100000],[2454,100000],[1562,100000],[9720,100000],[4432,100000],[145,100000],[8976,100000],[1242,100000],[8547,100000],[7519,100000],[8557,100000],[5229,100000],[5766,100000],[7619,100000],[4051,100000],[3357,100000],[9176,100000],[2175,100000],[4039,100000],[3021,100000],[9586,100000],[3888,100000],[2904,100000],[5845,100000],[4651,100000],[9469,100000],[4942,100000],[5280,100000],[5388,100000],[809,100000],[7534,100000],[9228,100000],[4402,100000],[964,100000],[8670,100000],[5746,100000],[7389,100000],[9336,100000],[2381,100000],[6352,100000],[2911,100000],[8369,100000],[174,100000],[2748,100000],[2099,100000],[488,100000],[139,100000],[8393,100000],[283,100000],[3188,100000],[9559,100000],[2709,100000],[8953,100000],[6471,100000],[5718,100000],[6653,100000],[295,100000],[4989,100000],[1462,100000],[7140,100000],[5663,100000],[4847,100000],[2302,100000],[6490,100000],[6368,100000],[9663,100000],[9700,100000],[6226,100000],[905,100000],[7721,100000],[9622,100000],[7483,100000],[9867,100000],[2913,100000],[7942,100000],[1336,100000],[9319,100000],[4855,100000],[1241,100000],[4667,100000],[6410,100000],[1544,100000],[7903,100000],[8490,100000],[6501,100000],[9493,100000],[1456,100000],[6257,100000],[5118,100000],[725,100000],[9785,100000],[5750,100000],[8238,100000],[4708,100000],[4321,100000],[2362,100000],[2770,100000],[7532,100000],[5777,100000],[280,100000],[745,100000],[2328,100000],[7149,100000],[9005,100000],[4711,100000],[3142,100000],[3479,100000],[7116,100000],[1973,100000],[5106,100000],[7006,100000],[8661,100000],[2893,100000],[5930,100000],[932,100000],[7067,100000],[8801,100000],[5069,100000],[865,100000],[8513,100000],[4448,100000],[4189,100000],[5386,100000],[4438,100000],[4818,100000],[3692,100000],[9964,100000],[7243,100000],[533,100000],[1298,100000],[9575,100000],[1368,100000],[2615,100000],[2940,100000],[8932,100000],[4700,100000],[6557,100000],[1030,100000],[2578,100000],[1619,100000],[1325,100000],[81,100000],[7361,100000],[9756,100000],[8893,100000],[6062,100000],[2235,100000],[2094,100000],[9117,100000],[99,100000],[2816,100000],[8390,100000],[1877,100000],[7171,100000],[1963,100000],[5489,100000],[3625,100000],[6556,100000],[250,100000],[4763,100000],[5754,100000],[9079,100000],[421,100000],[6165,100000],[7930,100000],[9733,100000],[9474,100000],[6451,100000],[5462,100000],[5220,100000],[6263,100000],[7592,100000],[8891,100000],[9646,100000],[2603,100000],[7709,100000],[6455,100000],[560,100000],[8150,100000],[7089,100000],[7052,100000],[2612,100000],[9640,100000],[7730,100000],[5581,100000],[9949,100000],[4020,100000],[5486,100000],[1559,100000],[7075,100000],[7506,100000],[8336,100000],[3973,100000],[3786,100000],[5119,100000],[8912,100000],[7274,100000],[2855,100000],[2154,100000],[684,100000],[7530,100000],[3868,100000],[2038,100000],[9985,100000],[4079,100000],[1860,100000],[9824,100000],[3326,100000],[9565,100000],[2173,100000],[3789,100000],[9579,100000],[9863,100000],[7379,100000],[6326,100000],[8675,100000],[6094,100000],[9616,100000],[544,100000],[3944,100000],[7757,100000],[3589,100000],[6715,100000],[7238,100000],[8516,100000],[1968,100000],[3103,100000],[470,100000],[327,100000],[2089,100000],[592,100000],[7064,100000],[8824,100000],[2783,100000],[9324,100000],[2009,100000],[3267,100000],[3455,100000],[666,100000],[3245,100000],[8063,100000],[6406,100000],[9590,100000],[6829,100000],[1423,100000],[3038,100000],[7004,100000],[4622,100000],[1148,100000],[7518,100000],[7542,100000],[6631,100000],[8307,100000],[210,100000],[4804,100000],[3162,100000],[1429,100000],[4760,100000],[1146,100000],[2384,100000],[6230,100000],[6163,100000],[8487,100000],[319,100000],[8666,100000],[1751,100000],[198,100000],[8230,100000],[3409,100000],[677,100000],[3450,100000],[4435,100000],[2303,100000],[2901,100000],[5312,100000],[6003,100000],[1205,100000],[9989,100000],[6883,100000],[387,100000],[3809,100000],[7783,100000],[1168,100000],[572,100000],[7041,100000],[3072,100000],[385,100000],[247,100000],[3381,100000],[7142,100000],[9127,100000],[8530,100000],[7543,100000],[2353,100000],[1829,100000],[5070,100000],[4974,100000],[8978,100000],[9048,100000],[7302,100000],[1661,100000],[67,100000],[8363,100000],[687,100000],[535,100000],[429,100000],[9921,100000],[5990,100000],[6053,100000],[3211,100000],[9213,100000],[130,100000],[7469,100000],[3283,100000],[663,100000],[8964,100000],[545,100000],[5429,100000],[6188,100000],[5563,100000],[2548,100000],[576,100000],[6522,100000],[2425,100000],[688,100000],[5116,100000],[8164,100000],[2450,100000],[9217,100000],[7849,100000],[8437,100000],[4649,100000],[4311,100000],[5014,100000],[451,100000],[2100,100000],[811,100000],[5145,100000],[7616,100000],[3947,100000],[8669,100000],[5392,100000],[4396,100000],[1086,100000],[1302,100000],[9681,100000],[2146,100000],[6115,100000],[200,100000],[2996,100000],[4029,100000],[5073,100000],[7439,100000],[4172,100000],[1087,100000],[3967,100000],[919,100000],[9993,100000],[5105,100000],[5304,100000],[6716,100000],[7296,100000],[4508,100000],[5218,100000],[7375,100000],[9211,100000],[2182,100000],[3690,100000],[6411,100000],[8619,100000],[3312,100000],[3885,100000],[9414,100000],[2156,100000],[2350,100000],[3467,100000],[7159,100000],[7823,100000],[4843,100000],[3215,100000],[1025,100000],[9168,100000],[9196,100000],[165,100000],[813,100000],[1758,100000],[2400,100000],[1144,100000],[3830,100000],[3319,100000],[1414,100000],[504,100000],[8264,100000],[6162,100000],[7134,100000],[7909,100000],[2390,100000],[6382,100000],[3156,100000],[3874,100000],[2619,100000],[1644,100000],[2680,100000],[9967,100000],[6819,100000],[5536,100000],[1088,100000],[5206,100000],[2202,100000],[9411,100000],[7204,100000],[705,100000],[808,100000],[562,100000],[1919,100000],[8620,100000],[6931,100000],[5963,100000],[366,100000],[8603,100000],[7099,100000],[9836,100000],[2434,100000],[4425,100000],[8685,100000],[4385,100000],[7489,100000],[4541,100000],[9983,100000],[4004,100000],[3734,100000],[3401,100000],[2999,100000],[9369,100000],[5630,100000],[4637,100000],[5634,100000],[9666,100000],[377,100000],[3607,100000],[1044,100000],[8653,100000],[841,100000],[1564,100000],[9789,100000],[1704,100000],[678,100000],[8324,100000],[1807,100000],[2162,100000],[9778,100000],[8584,100000],[6675,100000],[3600,100000],[2489,100000],[1207,100000],[3702,100000],[7872,100000],[1667,100000],[5297,100000],[5092,100000],[9299,100000],[9447,100000],[6654,100000],[7307,100000],[231,100000],[9595,100000],[9492,100000],[6602,100000],[7734,100000],[4138,100000],[4551,100000],[6951,100000],[9751,100000],[2545,100000],[4344,100000],[2982,100000],[7919,100000],[1469,100000],[5499,100000],[8859,100000],[7762,100000],[9773,100000],[7750,100000],[6836,100000],[253,100000],[9205,100000],[7394,100000],[2458,100000],[6817,100000],[267,100000],[5345,100000],[9451,100000],[2051,100000],[8155,100000],[7070,100000],[254,100000],[8656,100000],[7299,100000],[8479,100000],[1906,100000],[2409,100000],[787,100000],[838,100000],[3972,100000],[1398,100000],[4994,100000],[7853,100000],[3077,100000],[6059,100000],[3163,100000],[7505,100000],[1315,100000],[8810,100000],[7493,100000],[2551,100000],[1888,100000],[1588,100000],[3586,100000],[8683,100000],[4365,100000],[8861,100000],[7621,100000],[6721,100000],[9980,100000],[3661,100000],[3004,100000],[6761,100000],[2799,100000],[5244,100000],[1710,100000],[913,100000],[4121,100000],[4837,100000],[8749,100000],[1986,100000],[5214,100000],[998,100000],[9455,100000],[7577,100000],[5162,100000],[3686,100000],[5024,100000],[9355,100000],[2103,100000],[2387,100000],[6342,100000],[3665,100000],[2134,100000],[7346,100000],[8925,100000],[2176,100000],[4630,100000],[2234,100000],[7806,100000],[7129,100000],[5371,100000],[9381,100000],[6187,100000],[7279,100000],[3185,100000],[3940,100000],[4646,100000],[933,100000],[9943,100000],[9175,100000],[622,100000],[7905,100000],[1218,100000],[8994,100000],[8817,100000],[793,100000],[9488,100000],[7306,100000],[6511,100000],[9098,100000],[8828,100000],[5880,100000],[4277,100000],[900,100000],[669,100000],[8706,100000],[7321,100000],[4758,100000],[1529,100000],[2928,100000],[7837,100000],[4865,100000],[8283,100000],[6121,100000],[9404,100000],[9956,100000],[4971,100000],[9008,100000],[6285,100000],[697,100000],[886,100000],[6134,100000],[3320,100000],[3741,100000],[9399,100000],[8777,100000],[6294,100000],[7527,100000],[1192,100000],[7789,100000],[3662,100000],[6395,100000],[9283,100000],[3199,100000],[6864,100000],[4770,100000],[1135,100000],[8679,100000],[8836,100000],[6247,100000],[7376,100000],[6129,100000],[3583,100000],[6096,100000],[8735,100000],[8954,100000],[6021,100000],[2060,100000],[8422,100000],[5579,100000],[9665,100000],[9522,100000],[7927,100000],[1345,100000],[9132,100000],[6848,100000],[9496,100000],[4600,100000],[3251,100000],[17,100000],[3733,100000],[7555,100000],[4659,100000],[760,100000],[3364,100000],[117,100000],[712,100000],[3709,100000],[3346,100000],[1310,100000],[9835,100000],[7593,100000],[6627,100000],[3121,100000],[9223,100000],[2197,100000],[9528,100000],[9298,100000],[4619,100000],[1286,100000],[6830,100000],[3325,100000],[4607,100000],[7874,100000],[5652,100000],[863,100000],[8565,100000],[2024,100000],[5449,100000],[718,100000],[8204,100000],[5595,100000],[3981,100000],[8099,100000],[6246,100000],[9655,100000],[3965,100000],[2753,100000],[6634,100000],[4948,100000],[7826,100000],[3226,100000],[4280,100000],[1533,100000],[1677,100000],[1738,100000],[4392,100000],[1565,100000],[2215,100000],[1089,100000],[6718,100000],[3909,100000],[3550,100000],[1233,100000],[3164,100000],[2268,100000],[5028,100000],[2245,100000],[4922,100000],[1270,100000],[6378,100000],[9628,100000],[7344,100000],[3145,100000],[6868,100000],[5870,100000],[1098,100000],[6104,100000],[9703,100000],[2026,100000],[8400,100000],[7670,100000],[1835,100000],[9908,100000],[784,100000],[8139,100000],[8899,100000],[8613,100000],[4207,100000],[8869,100000],[8597,100000],[2211,100000],[6814,100000],[2760,100000],[1895,100000],[2582,100000],[3998,100000],[8057,100000],[2090,100000],[7525,100000],[6590,100000],[757,100000],[7918,100000],[2413,100000],[823,100000],[2177,100000],[8374,100000],[6160,100000],[8294,100000],[1869,100000],[9375,100000],[6076,100000],[2796,100000],[6835,100000],[1090,100000],[2374,100000],[4652,100000],[7241,100000],[1303,100000],[4183,100000],[5397,100000],[7758,100000],[9310,100000],[7154,100000],[7598,100000],[1897,100000],[6620,100000],[580,100000],[7576,100000],[1278,100000],[7973,100000],[2522,100000],[1820,100000],[6740,100000],[6737,100000],[2660,100000],[3445,100000],[8428,100000],[7596,100000],[5061,100000],[4852,100000],[7887,100000],[5417,100000],[3286,100000],[5670,100000],[6164,100000],[6858,100000],[8872,100000],[4825,100000],[7908,100000],[7434,100000],[2127,100000],[9950,100000],[9633,100000],[6643,100000],[3555,100000],[5884,100000],[7847,100000],[4534,100000],[4174,100000],[4548,100000],[4678,100000],[4068,100000],[2779,100000],[2841,100000],[260,100000],[8357,100000],[3539,100000],[1502,100000],[9225,100000],[2442,100000],[1132,100000],[570,100000],[5174,100000],[7614,100000],[443,100000],[2570,100000],[2164,100000],[3278,100000],[7550,100000],[631,100000],[5253,100000],[3547,100000],[1254,100000],[9669,100000],[5986,100000],[8107,100000],[6450,100000],[2016,100000],[1311,100000],[7843,100000],[6248,100000],[111,100000],[4748,100000],[7187,100000],[5245,100000],[228,100000],[5067,100000],[6057,100000],[4868,100000],[5664,100000],[7966,100000],[7573,100000],[5009,100000],[2376,100000],[5029,100000],[7072,100000],[1547,100000],[59,100000],[4806,100000],[6010,100000],[7771,100000],[5522,100000],[2223,100000],[1789,100000],[7984,100000],[8792,100000],[2293,100000],[7512,100000],[460,100000],[4838,100000],[6999,100000],[9031,100000],[294,100000],[3797,100000],[3388,100000],[9301,100000],[5653,100000],[5325,100000],[2370,100000],[5181,100000],[7272,100000],[524,100000],[3719,100000],[6473,100000],[8412,100000],[1567,100000],[5217,100000],[5050,100000],[5961,100000],[5586,100000],[127,100000],[1697,100000],[1768,100000],[4059,100000],[731,100000],[732,100000],[5381,100000],[8778,100000],[791,100000],[6888,100000],[8776,100000],[7390,100000],[9591,100000],[5194,100000],[4215,100000],[7575,100000],[7407,100000],[1073,100000],[352,100000],[8078,100000],[6015,100000],[1351,100000],[9846,100000],[9007,100000],[6709,100000],[6316,100000],[2228,100000],[3363,100000],[4295,100000],[2073,100000],[8621,100000],[3402,100000],[3009,100000],[5624,100000],[3214,100000],[5212,100000],[5143,100000],[6860,100000],[6533,100000],[6335,100000],[2856,100000],[6242,100000],[4725,100000],[6689,100000],[3715,100000],[5578,100000],[3290,100000],[2662,100000],[6048,100000],[5949,100000],[4703,100000],[896,100000],[6725,100000],[170,100000],[2475,100000],[9341,100000],[3180,100000],[3481,100000],[2902,100000],[4104,100000],[7169,100000],[4826,100000],[7183,100000],[1291,100000],[737,100000],[9456,100000],[840,100000],[5208,100000],[3298,100000],[2730,100000],[9342,100000],[2823,100000],[1844,100000],[9902,100000],[5761,100000],[9290,100000],[7922,100000],[5172,100000],[6555,100000],[3574,100000],[3928,100000],[4717,100000],[9288,100000],[1134,100000],[1925,100000],[9638,100000],[4931,100000],[2953,100000],[7071,100000],[4131,100000],[8748,100000],[2447,100000],[7151,100000],[3287,100000],[9588,100000],[4588,100000],[5617,100000],[4303,100000],[9981,100000],[5769,100000],[87,100000],[5660,100000],[7600,100000],[5086,100000],[5269,100000],[2481,100000],[3063,100000],[8256,100000],[6413,100000],[5316,100000],[616,100000],[5661,100000],[7868,100000],[3230,100000],[7373,100000],[3096,100000],[8062,100000],[1238,100000],[8213,100000],[1142,100000],[9366,100000],[9189,100000],[4451,100000],[5440,100000],[4696,100000],[1924,100000],[6579,100000],[2935,100000],[7985,100000],[9530,100000],[4656,100000],[4480,100000],[9061,100000],[24,100000],[3918,100000],[7832,100000],[6193,100000],[4329,100000],[6006,100000],[5031,100000],[3806,100000],[1381,100000],[2032,100000],[4518,100000],[9128,100000],[4074,100000],[3580,100000],[216,100000],[8783,100000],[8705,100000],[1425,100000],[6156,100000],[2137,100000],[5015,100000],[8228,100000],[2813,100000],[3895,100000],[6334,100000],[5785,100000],[1625,100000],[4479,100000],[6,100000],[769,100000],[661,100000],[8235,100000],[3542,100000],[3085,100000],[197,100000],[1415,100000],[1245,100000],[3141,100000],[4874,100000],[4216,100000],[2941,100000],[7023,100000],[6567,100000],[3046,100000],[2648,100000],[416,100000],[1479,100000],[4916,100000],[9801,100000],[5639,100000],[6844,100000],[672,100000],[5130,100000],[2649,100000],[248,100000],[5330,100000],[6008,100000],[9270,100000],[9670,100000],[5583,100000],[8025,100000],[4419,100000],[858,100000],[5503,100000],[6258,100000],[6145,100000],[9192,100000],[9354,100000],[5812,100000],[7250,100000],[8468,100000],[6330,100000],[5734,100000],[5226,100000],[6001,100000],[2771,100000],[7879,100000],[9701,100000],[104,100000],[6428,100000],[2015,100000],[1175,100000],[8526,100000],[1430,100000],[6135,100000],[2045,100000],[3543,100000],[7695,100000],[2880,100000],[2722,100000],[971,100000],[3873,100000],[370,100000],[7549,100000],[8239,100000],[7940,100000],[6976,100000],[8626,100000],[5380,100000],[4810,100000],[7952,100000],[407,100000],[726,100000],[1582,100000],[8364,100000],[7736,100000],[309,100000],[2642,100000],[9387,100000],[6695,100000],[8497,100000],[3673,100000],[5960,100000],[1177,100000],[202,100000],[2224,100000],[9961,100000],[8183,100000],[5422,100000],[4275,100000],[5977,100000],[6383,100000],[5027,100000],[8830,100000],[4809,100000],[2408,100000],[4254,100000],[8030,100000],[1663,100000],[1334,100000],[9678,100000],[1806,100000],[5227,100000],[2677,100000],[4314,100000],[880,100000],[8185,100000],[4899,100000],[9970,100000],[5861,100000],[947,100000],[6367,100000],[4394,100000],[6571,100000],[3167,100000],[3528,100000],[2557,100000],[3576,100000],[698,100000],[9687,100000],[9060,100000],[8209,100000],[2017,100000],[6409,100000],[1729,100000],[8944,100000],[8538,100000],[8403,100000],[1422,100000],[1965,100000],[5584,100000],[9190,100000],[2862,100000],[1748,100000],[9912,100000],[164,100000],[8765,100000],[6955,100000],[5702,100000],[3010,100000],[1522,100000],[5049,100000],[6148,100000],[5970,100000],[4629,100000],[4327,100000],[9599,100000],[3616,100000],[1363,100000],[1004,100000],[2724,100000],[3960,100000],[1448,100000],[4359,100000],[3028,100000],[9157,100000],[8157,100000],[4036,100000],[893,100000],[9672,100000],[6729,100000],[1793,100000],[5237,100000],[2129,100000],[234,100000],[5261,100000],[9068,100000],[2523,100000],[9378,100000],[559,100000],[8947,100000],[4570,100000],[160,100000],[8020,100000],[7347,100000],[474,100000],[4159,100000],[8586,100000],[7378,100000],[4444,100000],[9869,100000],[999,100000],[3473,100000],[7556,100000],[7841,100000],[9799,100000],[2336,100000],[4742,100000],[564,100000],[9339,100000],[6987,100000],[1775,100000],[3858,100000],[2418,100000],[219,100000],[2332,100000],[7840,100000],[1451,100000],[4823,100000],[8498,100000],[2956,100000],[6463,100000],[2354,100000],[1856,100000],[3564,100000],[1289,100000],[1344,100000],[2715,100000],[4986,100000],[9227,100000],[803,100000],[7792,100000],[2242,100000],[8698,100000],[3184,100000],[7996,100000],[1447,100000],[3999,100000],[2305,100000],[7164,100000],[4003,100000],[3459,100000],[1166,100000],[2851,100000],[5989,100000],[7408,100000],[7369,100000],[6593,100000],[6488,100000],[4181,100000],[3082,100000],[32,100000],[266,100000],[4886,100000],[4627,100000],[1946,100000],[7259,100000],[2936,100000],[8657,100000],[8,100000],[482,100000],[5305,100000],[3745,100000],[3282,100000],[7535,100000],[1708,100000],[884,100000],[8416,100000],[6046,100000],[3172,100000],[4802,100000],[644,100000],[3711,100000],[8286,100000],[768,100000],[4616,100000],[6937,100000],[1480,100000],[5520,100000],[8167,100000],[6712,100000],[5128,100000],[548,100000],[9890,100000],[9156,100000],[8819,100000],[8075,100000],[4675,100000],[8617,100000],[2257,100000],[4590,100000],[782,100000],[8857,100000],[8607,100000],[3204,100000],[885,100000],[3739,100000],[3614,100000],[6827,100000],[7432,100000],[792,100000],[9361,100000],[9911,100000],[783,100000],[8471,100000],[5618,100000],[6223,100000],[229,100000],[7699,100000],[4984,100000],[6236,100000],[2561,100000],[4245,100000],[3148,100000],[6914,100000],[5658,100000],[184,100000],[5966,100000],[6186,100000],[5243,100000],[7300,100000],[188,100000],[3862,100000],[3506,100000],[4933,100000],[521,100000],[7949,100000],[8536,100000],[7649,100000],[4460,100000],[60,100000],[1764,100000],[8383,100000],[7994,100000],[1521,100000],[8301,100000],[4564,100000],[3775,100000],[7805,100000],[9707,100000],[8352,100000],[6655,100000],[8096,100000],[3087,100000],[1283,100000],[1931,100000],[1408,100000],[7286,100000],[2761,100000],[3499,100000],[5291,100000],[415,100000],[1916,100000],[5883,100000],[8474,100000],[3610,100000],[4033,100000],[7652,100000],[4260,100000],[3376,100000],[4579,100000],[7281,100000],[1486,100000],[6614,100000],[6315,100000],[1181,100000],[3001,100000],[7729,100000],[9889,100000],[7565,100000],[8028,100000],[7147,100000],[5807,100000],[5635,100000],[3019,100000],[859,100000],[6974,100000],[3776,100000],[7587,100000],[4692,100000],[7384,100000],[2587,100000],[7953,100000],[3149,100000],[9585,100000],[6204,100000],[856,100000],[1041,100000],[7661,100000],[5837,100000],[3557,100000],[9966,100000],[3044,100000],[7222,100000],[3202,100000],[1056,100000],[8153,100000],[8192,100000],[4638,100000],[3124,100000],[4820,100000],[6364,100000],[6007,100000],[5953,100000],[608,100000],[4574,100000],[5166,100000],[6311,100000],[2653,100000],[8554,100000],[4347,100000],[1491,100000],[7657,100000],[1891,100000],[4897,100000],[8415,100000],[1546,100000],[7267,100000],[9481,100000],[1454,100000],[3977,100000],[1507,100000],[6704,100000],[9203,100000],[5402,100000],[112,100000],[638,100000],[7117,100000],[8216,100000],[8806,100000],[284,100000],[7143,100000],[1872,100000],[2670,100000],[7666,100000],[9580,100000],[9952,100000],[2559,100000],[2115,100000],[9305,100000],[1917,100000],[1726,100000],[8880,100000],[9232,100000],[1294,100000],[351,100000],[5360,100000],[44,100000],[308,100000],[2896,100000],[7141,100000],[7234,100000],[9126,100000],[1171,100000],[5389,100000],[9561,100000],[8478,100000],[7025,100000],[4024,100000],[5225,100000],[3619,100000],[5057,100000],[3187,100000],[2997,100000],[2267,100000],[824,100000],[5983,100000],[1996,100000],[3205,100000],[8879,100000],[3369,100000],[5952,100000],[6494,100000],[433,100000],[483,100000],[5537,100000],[5971,100000],[2514,100000],[4803,100000],[5998,100000],[4723,100000],[5751,100000],[3954,100000],[5608,100000],[1195,100000],[3520,100000],[517,100000],[6445,100000],[5833,100000],[4383,100000],[6375,100000],[9169,100000],[6497,100000],[8822,100000],[750,100000],[7336,100000],[8360,100000],[3955,100000],[1467,100000],[6011,100000],[3801,100000],[2971,100000],[8934,100000],[350,100000],[1669,100000],[5934,100000],[540,100000],[7946,100000],[8868,100000],[6796,100000],[1626,100000],[9554,100000],[8858,100000],[9491,100000],[7703,100000],[1333,100000],[7388,100000],[516,100000],[1583,100000],[6637,100000],[6552,100000],[9797,100000],[5532,100000],[4370,100000],[5356,100000],[3629,100000],[5773,100000],[8595,100000],[584,100000],[3559,100000],[5460,100000],[9953,100000],[8575,100000],[1516,100000],[4524,100000],[8606,100000],[122,100000],[8971,100000],[4935,100000],[2013,100000],[9124,100000],[6816,100000],[2814,100000],[458,100000],[4634,100000],[9187,100000],[6641,100000],[1493,100000],[4082,100000],[6597,100000],[3663,100000],[3670,100000],[6859,100000],[1994,100000],[3284,100000],[1365,100000],[8946,100000],[8197,100000],[848,100000],[4209,100000],[7728,100000],[4248,100000],[4987,100000],[2297,100000],[8804,100000],[1058,100000],[5008,100000],[2437,100000],[4026,100000],[383,100000],[1383,100000],[8652,100000],[7632,100000],[4292,100000],[1122,100000],[6891,100000],[788,100000],[4543,100000],[8815,100000],[4354,100000],[8327,100000],[4315,100000],[3065,100000],[162,100000],[5043,100000],[4906,100000],[5901,100000],[6340,100000],[5081,100000],[4333,100000],[1864,100000],[9248,100000],[3232,100000],[8187,100000],[4788,100000],[2401,100000],[2181,100000],[2187,100000],[1387,100000],[1420,100000],[8553,100000],[6297,100000],[5913,100000],[847,100000],[3413,100000],[1631,100000],[8965,100000],[9185,100000],[1770,100000],[8689,100000],[2686,100000],[2804,100000],[6019,100000],[4834,100000],[8508,100000],[484,100000],[5967,100000],[8694,100000],[961,100000],[8305,100000],[203,100000],[3738,100000],[1416,100000],[7686,100000],[5752,100000],[6509,100000],[5829,100000],[7987,100000],[7798,100000],[9442,100000],[5882,100000],[8786,100000],[6536,100000],[3361,100000],[3017,100000],[7788,100000],[6116,100000],[8596,100000],[7707,100000],[2185,100000],[5871,100000],[6719,100000],[1405,100000],[5942,100000],[4022,100000],[4226,100000],[2415,100000],[3884,100000],[2490,100000],[2661,100000],[6507,100000],[3949,100000],[3915,100000],[6205,100000],[8928,100000],[6644,100000],[7651,100000],[8388,100000],[8926,100000],[4271,100000],[7563,100000],[4603,100000],[2541,100000],[8941,100000],[1859,100000],[8483,100000],[6575,100000],[6925,100000],[4878,100000],[1060,100000],[6491,100000],[1029,100000],[8494,100000],[5602,100000],[1157,100000],[2734,100000],[4092,100000],[9725,100000],[2905,100000],[7020,100000],[8544,100000],[4459,100000],[3126,100000],[7275,100000],[8023,100000],[9610,100000],[7457,100000],[7846,100000],[9816,100000],[5272,100000],[4110,100000],[323,100000],[42,100000],[5298,100000],[6852,100000],[4163,100000],[6646,100000],[3075,100000],[7111,100000],[6266,100000],[5144,100000],[5894,100000],[5881,100000],[8084,100000],[8193,100000],[6045,100000],[317,100000],[1579,100000],[3902,100000],[2161,100000],[518,100000],[3480,100000],[3604,100000],[827,100000],[1099,100000],[7194,100000],[180,100000],[9266,100000],[7911,100000],[4102,100000],[95,100000],[7430,100000],[9330,100000],[3585,100000],[404,100000],[3041,100000],[9258,100000],[6723,100000],[4421,100000],[1513,100000],[8616,100000],[1962,100000],[2755,100000],[3705,100000],[8558,100000],[4084,100000],[8693,100000],[2153,100000],[7878,100000],[4698,100000],[5019,100000],[3603,100000],[2912,100000],[2110,100000],[7427,100000],[5929,100000],[770,100000],[4584,100000],[2967,100000],[6184,100000],[7182,100000],[7790,100000],[1949,100000],[3959,100000],[9277,100000],[6366,100000],[7283,100000],[356,100000],[3567,100000],[8772,100000],[9479,100000],[9325,100000],[1956,100000],[973,100000],[9410,100000],[6052,100000],[5611,100000],[5286,100000],[7284,100000],[8438,100000],[5599,100000],[7055,100000],[1879,100000],[3717,100000],[7022,100000],[355,100000],[8983,100000],[1589,100000],[7830,100000],[5632,100000],[4722,100000],[8241,100000],[2460,100000],[3811,100000],[7756,100000],[3349,100000],[4620,100000],[4990,100000],[2020,100000],[4764,100000],[4560,100000],[8142,100000],[8989,100000],[2547,100000],[8472,100000],[1201,100000],[940,100000],[2863,100000],[8284,100000],[1200,100000],[4087,100000],[8021,100000],[4632,100000],[3987,100000],[7747,100000],[662,100000],[6175,100000],[2679,100000],[3395,100000],[5674,100000],[7276,100000],[7289,100000],[7635,100000],[6429,100000],[1942,100000],[8968,100000],[1692,100000],[2404,100000],[35,100000],[5945,100000],[2530,100000],[9505,100000],[3442,100000],[5428,100000],[2595,100000],[525,100000],[658,100000],[8035,100000],[670,100000],[7724,100000],[4375,100000],[3969,100000],[5637,100000],[2028,100000],[9032,100000],[4721,100000],[5482,100000],[6457,100000],[3003,100000],[2261,100000],[1958,100000],[4235,100000],[8331,100000],[8161,100000],[7401,100000],[1737,100000],[1550,100000],[5341,100000],[36,100000],[1670,100000],[2312,100000],[7160,100000],[5238,100000],[7315,100000],[8937,100000],[5839,100000],[4146,100000],[659,100000],[8993,100000],[8886,100000],[7742,100000],[7839,100000],[5964,100000],[6532,100000],[4781,100000],[7226,100000],[952,100000],[2542,100000],[3235,100000],[2,100000],[5037,100000],[5370,100000],[2143,100000],[5848,100000],[6699,100000],[2339,100000],[1301,100000],[6650,100000],[9944,100000],[4086,100000],[9976,100000],[9683,100000],[4192,100000],[3310,100000],[3683,100000],[9036,100000],[9204,100000],[8381,100000],[5314,100000],[6623,100000],[2842,100000],[1712,100000],[7233,100000],[4858,100000],[6731,100000],[4322,100000],[9652,100000],[877,100000],[4227,100000],[1967,100000],[6763,100000],[3443,100000],[8798,100000],[8609,100000],[5154,100000],[6207,100000],[8266,100000],[9750,100000],[9506,100000],[1698,100000],[1492,100000],[7030,100000],[7740,100000],[5770,100000],[2794,100000],[3657,100000],[8757,100000],[4496,100000],[3675,100000],[7412,100000],[5972,100000],[6924,100000],[3645,100000],[9396,100000],[5321,100000],[993,100000],[3372,100000],[3836,100000],[9102,100000],[3599,100000],[1117,100000],[6018,100000],[7640,100000],[4190,100000],[9564,100000],[7440,100000],[927,100000],[5060,100000],[5712,100000],[7893,100000],[7681,100000],[6984,100000],[997,100000],[3963,100000],[9536,100000],[8240,100000],[76,100000],[9250,100000],[4119,100000],[4866,100000],[8929,100000],[8534,100000],[3667,100000],[3444,100000],[3501,100000],[5947,100000],[8738,100000],[7172,100000],[8585,100000],[9498,100000],[8207,100000],[6531,100000],[7628,100000],[7157,100000],[6353,100000],[7330,100000],[3252,100000],[7451,100000],[3016,100000],[9734,100000],[9992,100000],[2534,100000],[5173,100000],[8441,100000],[4098,100000],[4413,100000],[6786,100000],[1285,100000],[1155,100000],[7090,100000],[9677,100000],[3279,100000],[4208,100000],[9281,100000],[514,100000],[6201,100000],[7447,100000],[7040,100000],[9001,100000],[9450,100000],[5471,100000],[9502,100000],[3134,100000],[6679,100000],[7725,100000],[3206,100000],[4869,100000],[2988,100000],[1319,100000],[5136,100000],[5659,100000],[5513,100000],[354,100000],[9297,100000],[6762,100000],[2289,100000],[1372,100000],[1495,100000],[5510,100000],[6377,100000],[503,100000],[7080,100000],[3391,100000],[7015,100000],[5496,100000],[7548,100000],[9265,100000],[8640,100000],[6936,100000],[5796,100000],[1449,100000],[5892,100000],[9452,100000],[3961,100000],[4377,100000],[8029,100000],[6572,100000],[7054,100000],[5319,100000],[7851,100000],[1728,100000],[8855,100000],[567,100000],[6813,100000],[7691,100000],[1150,100000],[6068,100000],[815,100000],[9066,100000],[2346,100000],[9312,100000],[5546,100000],[1914,100000],[9557,100000],[9515,100000],[3766,100000],[1115,100000],[196,100000],[398,100000],[4626,100000],[341,100000],[5303,100000],[1671,100000],[3161,100000],[7585,100000],[2948,100000],[6513,100000],[3697,100000],[5646,100000],[5435,100000],[513,100000],[5745,100000],[4670,100000],[6313,100000],[2007,100000],[3447,100000],[1108,100000],[2167,100000],[4730,100000],[5082,100000],[593,100000],[8184,100000],[1040,100000],[8000,100000],[3429,100000],[3276,100000],[9656,100000],[1673,100000],[2027,100000],[5858,100000],[9047,100000],[5091,100000],[9440,100000],[5696,100000],[6908,100000],[7702,100000],[9338,100000],[1361,100000],[765,100000],[1443,100000],[8210,100000],[2495,100000],[3653,100000],[2716,100000],[5760,100000],[5557,100000],[6568,100000],[5732,100000],[728,100000],[4272,100000],[5819,100000],[1947,100000],[6753,100000],[5191,100000],[8789,100000],[4177,100000],[8714,100000],[3243,100000],[1995,100000],[9370,100000],[2393,100000],[5078,100000],[5279,100000],[1406,100000],[2358,100000],[3941,100000],[7782,100000],[6642,100000],[7817,100000],[975,100000],[1971,100000],[5146,100000],[9623,100000],[5673,100000],[8114,100000],[4475,100000],[7950,100000],[2040,100000],[9145,100000],[8598,100000],[6862,100000],[5597,100000],[1918,100000],[8525,100000],[8169,100000],[5150,100000],[8593,100000],[1221,100000],[7456,100000],[8955,100000],[4597,100000],[362,100000],[898,100000],[3577,100000],[2069,100000],[8464,100000],[4279,100000],[3324,100000],[1686,100000],[3175,100000],[599,100000],[8039,100000],[596,100000],[6794,100000],[5059,100000],[7203,100000],[8561,100000],[839,100000],[2598,100000],[9895,100000],[2634,100000],[8758,100000],[2573,100000],[3035,100000],[3464,100000],[6432,100000],[2042,100000],[409,100000],[4072,100000],[7860,100000],[6777,100000],[1228,100000],[8201,100000],[2873,100000],[7068,100000],[7214,100000],[5650,100000],[5373,100000],[2444,100000],[9936,100000],[6344,100000],[3613,100000],[3722,100000],[5126,100000],[4854,100000],[4492,100000],[4642,100000],[7871,100000],[5461,100000],[6742,100000],[1359,100000],[9351,100000],[7737,100000],[1715,100000],[2781,100000],[7322,100000],[5456,100000],[4816,100000],[9317,100000],[2954,100000],[1013,100000],[2690,100000],[4732,100000],[47,100000],[3818,100000],[8500,100000],[5864,100000],[494,100000],[5716,100000],[5908,100000],[5267,100000],[7974,100000],[4390,100000],[6840,100000],[1862,100000],[4471,100000],[1072,100000],[5139,100000],[5665,100000],[9148,100000],[257,100000],[5705,100000],[8507,100000],[9136,100000],[4556,100000],[497,100000],[6911,100000],[9367,100000],[706,100000],[149,100000],[6738,100000],[3554,100000],[5098,100000],[1991,100000],[4515,100000],[6149,100000],[6728,100000],[9180,100000],[531,100000],[1114,100000],[101,100000],[8863,100000],[2754,100000],[869,100000],[5160,100000],[2602,100000],[9178,100000],[9971,100000],[5594,100000],[9029,100000],[6458,100000],[195,100000],[2212,100000],[4113,100000],[2101,100000],[1611,100000],[7749,100000],[9885,100000],[2987,100000],[3217,100000],[6249,100000],[4639,100000],[4466,100000],[3475,100000],[6624,100000],[4284,100000],[5517,100000],[4846,100000],[5427,100000],[5327,100000],[7240,100000],[5125,100000],[9174,100000],[5695,100000],[4217,100000],[4557,100000],[4043,100000],[4336,100000],[5514,100000],[7037,100000],[8296,100000],[3892,100000],[1752,100000],[901,100000],[8762,100000],[2308,100000],[3761,100000],[1540,100000],[1263,100000],[7960,100000],[6441,100000],[2159,100000],[3127,100000],[450,100000],[923,100000],[4945,100000],[7517,100000],[7277,100000],[6744,100000],[6747,100000],[3601,100000],[5450,100000],[2125,100000],[4081,100000],[7654,100000],[4851,100000],[2962,100000],[6940,100000],[1535,100000],[9207,100000],[9261,100000],[7997,100000],[5123,100000],[7465,100000],[1321,100000],[1960,100000],[8847,100000],[8813,100000],[740,100000],[5683,100000],[4829,100000],[1658,100000],[4705,100000],[2540,100000],[3020,100000],[4813,100000],[8517,100000],[7803,100000],[7642,100000],[7708,100000],[9742,100000],[4793,100000],[8821,100000],[177,100000],[8529,100000],[4750,100000],[4317,100000],[2206,100000],[8690,100000],[1426,100000],[5223,100000],[3966,100000],[9153,100000],[9059,100000],[590,100000],[1394,100000],[8839,100000],[8431,100000],[8486,100000],[5806,100000],[1767,100000],[9430,100000],[2968,100000],[6194,100000],[5600,100000],[7351,100000],[4142,100000],[146,100000],[2351,100000],[2469,100000],[8027,100000],[8010,100000],[9699,100000],[7057,100000],[1362,100000],[7850,100000],[3985,100000],[9163,100000],[6850,100000],[3687,100000],[82,100000],[8737,100000],[2423,100000],[3842,100000],[9245,100000],[4830,100000],[3032,100000],[2225,100000],[2386,100000],[891,100000],[6661,100000],[4718,100000],[8816,100000],[4116,100000],[8734,100000],[5603,100000],[1332,100000],[52,100000],[7986,100000],[1356,100000],[6437,100000],[5013,100000],[9115,100000],[5394,100000],[4876,100000],[1526,100000],[2021,100000],[6338,100000],[9805,100000],[9150,100000],[4972,100000],[3964,100000],[8014,100000],[1921,100000],[2498,100000],[4947,100000],[3366,100000],[2439,100000],[4266,100000],[3462,100000],[4240,100000],[8090,100000],[4780,100000],[5900,100000],[8004,100000],[5903,100000],[9990,100000],[5941,100000],[8854,100000],[9229,100000],[8345,100000],[4147,100000],[3343,100000],[532,100000],[6290,100000],[656,100000],[150,100000],[1084,100000],[8674,100000],[2433,100000],[9821,100000],[3181,100000],[9077,100000],[4198,100000],[5309,100000],[8583,100000],[5359,100000],[1778,100000],[7731,100000],[6752,100000],[3176,100000],[9569,100000],[9363,100000],[3808,100000],[960,100000],[4808,100000],[5962,100000],[7496,100000],[7541,100000],[4856,100000],[9702,100000],[2273,100000],[6887,100000],[498,100000],[8236,100000],[8444,100000],[123,100000],[7560,100000],[1464,100000],[2455,100000],[696,100000],[8273,100000],[1102,100000],[5735,100000],[3301,100000],[4937,100000],[4234,100000],[2360,100000],[3318,100000],[1433,100000],[8379,100000],[6254,100000],[2976,100000],[605,100000],[5975,100000],[3691,100000],[2480,100000],[9903,100000],[3640,100000],[1825,100000],[9084,100000],[1083,100000],[2064,100000],[1944,100000],[1092,100000],[2927,100000],[7671,100000],[9482,100000],[155,100000],[6503,100000],[1838,100000],[270,100000],[6282,100000],[7043,100000],[3423,100000],[5529,100000],[9881,100000],[1137,100000],[2364,100000],[7136,100000],[2093,100000],[7429,100000],[4981,100000],[2717,100000],[5731,100000],[6812,100000],[1432,100000],[8202,100000],[7269,100000],[9494,100000],[8338,100000],[702,100000],[1293,100000],[4175,100000],[6528,100000],[4985,100000],[2558,100000],[3323,100000],[871,100000],[3483,100000],[2496,100000],[3748,100000],[2843,100000],[864,100000],[400,100000],[5419,100000],[320,100000],[8140,100000],[4416,100000],[3315,100000],[7073,100000],[3799,100000],[5481,100000],[3394,100000],[6487,100000],[7220,100000],[8634,100000],[6070,100000],[523,100000],[2318,100000],[2062,100000],[5153,100000],[6897,100000],[8018,100000],[30,100000],[7662,100000],[4997,100000],[9839,100000],[6599,100000],[2151,100000],[5739,100000],[9673,100000],[5246,100000],[5511,100000],[1594,100000],[5759,100000],[8967,100000],[6152,100000],[3068,100000],[9013,100000],[4500,100000],[462,100000],[3029,100000],[8253,100000],[5148,100000],[1281,100000],[3620,100000],[7352,100000],[2290,100000],[3646,100000],[3854,100000],[4018,100000],[8329,100000],[6730,100000],[9932,100000],[5262,100000],[3182,100000],[9403,100000],[5493,100000],[6328,100000],[4978,100000],[5035,100000],[614,100000],[380,100000],[6636,100000],[9164,100000],[4895,100000],[6512,100000],[4930,100000],[9671,100000],[2723,100000],[3260,100000],[2798,100000],[3628,100000],[4123,100000],[5331,100000],[561,100000],[6670,100000],[5911,100000],[4925,100000],[9874,100000],[1272,100000],[85,100000],[5062,100000],[761,100000],[3986,100000],[1113,100000],[8044,100000],[8110,100000],[4250,100000],[8969,100000],[5203,100000],[837,100000],[3566,100000],[2462,100000],[6997,100000],[5071,100000],[6137,100000],[1124,100000],[8760,100000],[9520,100000],[8467,100000],[8376,100000],[4155,100000],[1003,100000],[7638,100000],[5648,100000],[653,100000],[8452,100000],[3119,100000],[1700,100000],[9866,100000],[2291,100000],[1364,100000],[962,100000],[176,100000],[5407,100000],[873,100000],[9171,100000],[83,100000],[9511,100000],[4679,100000],[8639,100000],[5698,100000],[1813,100000],[2054,100000],[262,100000],[3348,100000],[4694,100000],[7894,100000],[9968,100000],[4611,100000],[6414,100000],[359,100000],[6846,100000],[1309,100000],[571,100000],[4299,100000],[9692,100000],[7932,100000],[1299,100000],[9135,100000],[5020,100000],[7696,100000],[2344,100000],[4458,100000],[1389,100000],[5516,100000],[3254,100000],[4288,100000],[8252,100000],[6943,100000],[6131,100000],[7227,100000],[8367,100000],[1131,100000],[3189,100000],[9441,100000],[7910,100000],[1678,100000],[7852,100000],[1472,100000],[8399,100000],[8766,100000],[1260,100000],[7704,100000],[3331,100000],[8046,100000],[6849,100000],[3773,100000],[2938,100000],[2474,100000],[2023,100000],[6127,100000],[6438,100000],[8750,100000],[6705,100000],[3678,100000],[5293,100000],[2849,100000],[9345,100000],[9377,100000],[5275,100000],[4889,100000],[1798,100000],[963,100000],[4203,100000],[3812,100000],[1499,100000],[3477,100000],[2080,100000],[1982,100000],[4481,100000],[9624,100000],[6489,100000],[9254,100000],[4520,100000],[7799,100000],[6882,100000],[1790,100000],[7578,100000],[5079,100000],[3855,100000],[6176,100000],[7039,100000],[108,100000],[4156,100000],[6680,100000],[8931,100000],[9539,100000],[994,100000],[328,100000],[7536,100000],[4384,100000],[3881,100000],[5994,100000],[6714,100000],[5798,100000],[2166,100000],[3410,100000],[7912,100000],[1377,100000],[4204,100000],[3264,100000],[9251,100000],[2079,100000],[8752,100000],[7571,100000],[2885,100000],[6136,100000],[9082,100000],[1231,100000],[1172,100000],[7167,100000],[6665,100000],[5033,100000],[5087,100000],[6696,100000],[615,100000],[3956,100000],[7764,100000],[918,100000],[8280,100000],[2432,100000],[9904,100000],[5519,100000],[5980,100000],[6751,100000],[845,100000],[9896,100000],[5691,100000],[4054,100000],[568,100000],[3292,100000],[4464,100000],[7454,100000],[8265,100000],[6431,100000],[7332,100000],[1791,100000],[2872,100000],[8261,100000],[6539,100000],[230,100000],[8795,100000],[724,100000],[8407,100000],[1043,100000],[7178,100000],[8759,100000],[667,100000],[2055,100000],[4267,100000],[1317,100000],[8051,100000],[6347,100000],[2426,100000],[8031,100000],[4195,100000],[1635,100000],[2122,100000],[7050,100000],[1905,100000],[6920,100000],[9320,100000],[365,100000],[8753,100000],[7455,100000],[6783,100000],[9828,100000],[9050,100000],[2991,100000],[7079,100000],[193,100000],[3209,100000],[5918,100000],[6372,100000],[7268,100000],[9661,100000],[3448,100000],[5995,100000],[736,100000],[8909,100000],[9195,100000],[3150,100000],[5721,100000],[4372,100000],[8848,100000],[2037,100000],[4682,100000],[2721,100000],[4529,100000],[6453,100000],[9533,100000],[56,100000],[2296,100000],[9119,100000],[6726,100000],[537,100000],[6609,100000],[3138,100000],[2742,100000],[9738,100000],[8316,100000],[1662,100000],[5714,100000],[5500,100000],[3089,100000],[4662,100000],[2171,100000],[7236,100000],[8633,100000],[9249,100000],[5478,100000],[432,100000],[4644,100000],[5358,100000],[5512,100000],[2524,100000],[3374,100000],[5477,100000],[6069,100000],[7881,100000],[1478,100000],[4140,100000],[8645,100000],[5466,100000],[1005,100000],[2039,100000],[8350,100000],[4729,100000],[9834,100000],[8654,100000],[8832,100000],[286,100000],[917,100000],[6873,100000],[7104,100000],[8987,100000],[7716,100000],[5840,100000],[639,100000],[7811,100000],[3265,100000],[6174,100000],[6927,100000],[5084,100000],[8166,100000],[2184,100000],[554,100000],[3882,100000],[5284,100000],[121,100000],[9830,100000],[9975,100000],[4586,100000],[1723,100000],[8940,100000],[4289,100000],[4389,100000],[8510,100000],[9106,100000],[9654,100000],[2074,100000],[8890,100000],[5713,100000],[6594,100000],[8047,100000],[2986,100000],[2266,100000],[6918,100000],[173,100000],[8582,100000],[8133,100000],[3787,100000],[4310,100000],[6154,100000],[2119,100000],[2829,100000],[6766,100000],[4137,100000],[3703,100000],[2368,100000],[1350,100000],[4487,100000],[6091,100000],[4161,100000],[3457,100000],[7076,100000],[7229,100000],[8998,100000],[6042,100000],[2544,100000],[7081,100000],[2921,100000],[3914,100000],[6304,100000],[1378,100000],[9234,100000],[648,100000],[1796,100000],[7829,100000],[1140,100000],[6095,100000],[5666,100000],[7906,100000],[5899,100000],[2979,100000],[1509,100000],[9935,100000],[4801,100000],[1397,100000],[4467,100000],[9822,100000],[4913,100000],[509,100000],[5347,100000],[8430,100000],[3844,100000],[982,100000],[5224,100000],[153,100000],[9544,100000],[3883,100000],[8295,100000],[4291,100000],[2120,100000],[1466,100000],[8186,100000],[175,100000],[9858,100000],[6244,100000],[5688,100000],[1403,100000],[4640,100000],[5795,100000],[772,100000],[1407,100000],[1777,100000],[2203,100000],[9434,100000],[8173,100000],[9070,100000],[7711,100000],[2978,100000],[9659,100000],[7760,100000],[7723,100000],[5270,100000],[6278,100000],[5410,100000],[6769,100000],[7870,100000],[4558,100000],[37,100000],[6013,100000],[4550,100000],[5053,100000],[6222,100000],[2416,100000],[5443,100000],[2340,100000],[5124,100000],[1244,100000],[7195,100000],[2144,100000],[5843,100000],[8630,100000],[2992,100000],[4691,100000],[6853,100000],[6760,100000],[6292,100000],[5978,100000],[4516,100000],[8755,100000],[5842,100000],[244,100000],[2740,100000],[358,100000],[3864,100000],[4581,100000],[4134,100000],[9686,100000],[4247,100000],[874,100000],[3681,100000],[5044,100000],[600,100000],[9276,100000],[1152,100000],[3288,100000],[626,100000],[4814,100000],[2158,100000],[2330,100000],[2050,100000],[9582,100000],[5177,100000],[6371,100000],[1819,100000],[6576,100000],[4335,100000],[683,100000],[5906,100000],[4786,100000],[6064,100000],[9937,100000],[6265,100000],[5233,100000],[9920,100000],[212,100000],[4152,100000],[6608,100000],[8867,100000],[7676,100000],[7659,100000],[1199,100000],[5893,100000],[2502,100000],[1251,100000],[8191,100000],[534,100000],[9651,100000],[9905,100000],[8939,100000],[120,100000],[1203,100000],[5354,100000],[7753,100000],[1975,100000],[821,100000],[3131,100000],[8591,100000],[8353,100000],[8923,100000],[4509,100000],[4286,100000],[1331,100000],[5680,100000],[9973,100000],[2764,100000],[4647,100000],[7158,100000],[1468,100000],[305,100000],[6391,100000],[9099,100000],[3650,100000],[4264,100000],[5701,100000],[5447,100000],[939,100000],[6243,100000],[4552,100000],[1666,100000],[5850,100000],[2035,100000],[1978,100000],[7266,100000],[9691,100000],[8807,100000],[1654,100000],[5589,100000],[2933,100000],[2230,100000],[1349,100000],[9181,100000],[6454,100000],[520,100000],[3992,100000],[9235,100000],[8127,100000],[7459,100000],[8076,100000],[9260,100000],[9327,100000],[9090,100000],[8504,100000],[1167,100000],[2292,100000],[4263,100000],[6781,100000],[7368,100000],[1985,100000],[7173,100000],[8351,100000],[9335,100000],[4124,100000],[6106,100000],[8349,100000],[9851,100000],[5979,100000],[1903,100000],[7648,100000],[5544,100000],[321,100000],[9774,100000],[4075,100000],[1664,100000],[2597,100000],[9719,100000],[9017,100000],[9388,100000],[6385,100000],[3132,100000],[3637,100000],[4677,100000],[707,100000],[4505,100000],[4733,100000],[6554,100000],[519,100000],[7523,100000],[4908,100000],[7802,100000],[8392,100000],[989,100000],[1867,100000],[5605,100000],[3632,100000],[9680,100000],[6722,100000],[2030,100000],[7780,100000],[6710,100000],[8198,100000],[9262,100000],[6237,100000],[1461,100000],[2262,100000],[9022,100000],[4324,100000],[4888,100000],[6866,100000],[8229,100000],[3407,100000],[2002,100000],[4232,100000],[3400,100000],[148,100000],[4528,100000],[7599,100000],[5976,100000],[1784,100000],[3352,100000],[3729,100000],[3839,100000],[2493,100000],[2539,100000],[8188,100000],[2898,100000],[1459,100000],[3677,100000],[472,100000],[3257,100000],[4388,100000],[2453,100000],[9486,100000],[6332,100000],[5926,100000],[1276,100000],[2746,100000],[8541,100000],[41,100000],[8499,100000],[3024,100000],[6110,100000],[3587,100000],[7923,100000],[2124,100000],[835,100000],[1485,100000],[4765,100000],[6870,100000],[992,100000],[8853,100000],[1143,100000],[4040,100000],[2149,100000],[2217,100000],[9365,100000],[2044,100000],[9643,100000],[4007,100000],[6250,100000],[1380,100000],[6626,100000],[5018,100000],[2840,100000],[946,100000],[9594,100000],[1358,100000],[1633,100000],[9419,100000],[3647,100000],[8769,100000],[1104,100000],[4540,100000],[6970,100000],[9831,100000],[528,100000],[5912,100000],[1066,100000],[4775,100000],[1401,100000],[6565,100000],[6181,100000],[9110,100000],[33,100000],[4609,100000],[7311,100000],[9891,100000],[7508,100000],[6310,100000],[6393,100000],[2301,100000],[7046,100000],[9747,100000],[4474,100000],[749,100000],[1026,100000],[6386,100000],[3160,100000],[1082,100000],[1855,100000],[7965,100000],[7858,100000],[9954,100000],[6407,100000],[2448,100000],[466,100000],[8026,100000],[8884,100000],[7009,100000],[8257,100000],[2250,100000],[7607,100000],[2639,100000],[4273,100000],[1194,100000],[4839,100000],[2630,100000],[3237,100000],[6971,100000],[5521,100000],[8799,100000],[2815,100000],[3197,100000],[3435,100000],[3414,100000],[4326,100000],[4278,100000],[6446,100000],[3847,100000],[4747,100000],[4434,100000],[367,100000],[7964,100000],[8285,100000],[7137,100000],[2236,100000],[2076,100000],[442,100000],[920,100000],[5161,100000],[7121,100000],[2138,100000],[7008,100000],[6331,100000],[8650,100000],[5633,100000],[9407,100000],[7011,100000],[5682,100000],[89,100000],[6384,100000],[3146,100000],[1440,100000],[3849,100000],[4360,100000],[7188,100000],[6177,100000],[7414,100000],[6123,100000],[238,100000],[925,100000],[805,100000],[7787,100000],[2825,100000],[4409,100000],[1490,100000],[9596,100000],[6903,100000],[3578,100000],[143,100000],[8505,100000],[1569,100000],[4182,100000],[8632,100000],[6373,100000],[2891,100000],[4728,100000],[2104,100000],[5803,100000],[2848,100000],[6589,100000],[5797,100000],[4631,100000],[6867,100000],[4759,100000],[1570,100000],[892,100000],[4382,100000],[1335,100000],[6381,100000],[6950,100000],[5320,100000],[5505,100000],[3102,100000],[7309,100000],[4578,100000],[7727,100000],[7521,100000],[7883,100000],[2990,100000],[7060,100000],[4890,100000],[602,100000],[6596,100000],[529,100000],[1065,100000],[9279,100000],[3935,100000],[7643,100000],[9284,100000],[2871,100000],[3772,100000],[9560,100000],[3488,100000],[4882,100000],[1843,100000],[3582,100000],[349,100000],[3529,100000],[1655,100000],[8116,100000],[3913,100000],[7913,100000],[629,100000],[446,100000],[7735,100000],[3791,100000],[8787,100000],[2583,100000],[9859,100000],[1145,100000],[4111,100000],[6159,100000],[6229,100000],[1993,100000],[2593,100000],[1164,100000],[8725,100000],[2483,100000],[3512,100000],[7690,100000],[1684,100000],[2907,100000],[2958,100000],[3814,100000],[9039,100000],[4745,100000],[4472,100000],[4766,100000],[2672,100000],[3045,100000],[8094,100000],[5811,100000],[7308,100000],[2295,100000],[738,100000],[105,100000],[8862,100000],[6496,100000],[4491,100000],[7139,100000],[1987,100000],[9318,100000],[1227,100000],[9572,100000],[3305,100000],[4473,100000],[645,100000],[8013,100000],[2192,100000],[4736,100000],[2406,100000],[5707,100000],[2919,100000],[7326,100000],[4636,100000],[3300,100000],[7048,100000],[5179,100000],[3239,100000],[8125,100000],[5562,100000],[2133,100000],[3525,100000],[4243,100000],[3246,100000],[6828,100000],[3515,100000],[2499,100000],[8079,100000],[4582,100000],[500,100000],[1252,100000],[7018,100000],[8915,100000],[6916,100000],[5132,100000],[7232,100000],[9467,100000],[3771,100000],[8045,100000],[3137,100000],[1470,100000],[8095,100000],[3908,100000],[8820,100000],[7393,100000],[1865,100000],[1028,100000],[8445,100000],[4784,100000],[9913,100000],[2820,100000],[2892,100000],[5854,100000],[4681,100000],[8072,100000],[7168,100000],[8065,100000],[6071,100000],[4995,100000],[9422,100000],[1966,100000],[883,100000],[9972,100000],[2793,100000],[3373,100000],[8602,100000],[5415,100000],[2805,100000],[9910,100000],[8135,100000],[6956,100000],[2644,100000],[9147,100000],[2769,100000],[2628,100000],[3393,100000],[4025,100000],[9862,100000],[1585,100000],[5985,100000],[7595,100000],[4185,100000],[9597,100000],[4410,100000],[2694,100000],[4762,100000],[6570,100000],[3728,100000],[7796,100000],[9617,100000],[7605,100000],[8401,100000],[3059,100000],[5679,100000],[2083,100000],[3846,100000],[1019,100000],[7875,100000],[3358,100000],[9503,100000],[3530,100000],[7231,100000],[7085,100000],[3144,100000],[7467,100000],[7155,100000],[818,100000],[4789,100000],[8087,100000],[7101,100000],[4977,100000],[1138,100000],[3051,100000],[3938,100000],[77,100000],[8268,100000],[5104,100000],[255,100000],[1907,100000],[2309,100000],[4593,100000],[6416,100000],[9758,100000],[5924,100000],[7027,100000],[4867,100000],[3465,100000],[6309,100000],[9765,100000],[8919,100000],[3534,100000],[8093,100000],[9332,100000],[7633,100000],[5201,100000],[2071,100000],[8249,100000],[430,100000],[8723,100000],[9865,100000],[3901,100000],[2915,100000],[7482,100000],[7831,100000],[9105,100000],[3392,100000],[9694,100000],[6772,100000],[9462,100000],[4205,100000],[4592,100000],[1170,100000],[7209,100000],[557,100000],[7584,100000],[1280,100000],[492,100000],[4504,100000],[4731,100000],[7318,100000],[4506,100000],[4443,100000],[436,100000],[7779,100000],[6029,100000],[7348,100000],[7824,100000],[9603,100000],[6885,100000],[7713,100000],[1622,100000],[9784,100000],[1646,100000],[5046,100000],[3289,100000],[2651,100000],[256,100000],[4145,100000],[4294,100000],[1705,100000],[1868,100000],[6065,100000],[3493,100000],[4188,100000],[9445,100000],[2673,100000],[5920,100000],[6978,100000],[282,100000],[7969,100000],[9917,100000],[6469,100000],[1749,100000],[3090,100000],[9593,100000],[8447,100000],[7641,100000],[4653,100000],[9934,100000],[3091,100000],[551,100000],[8163,100000],[2058,100000],[5361,100000],[8387,100000],[3636,100000],[8780,100000],[5045,100000],[379,100000],[118,100000],[1850,100000],[4369,100000],[7255,100000],[8688,100000],[790,100000],[3834,100000],[5656,100000],[4105,100000],[71,100000],[4070,100000],[3856,100000],[8433,100000],[5640,100000],[3408,100000],[5364,100000],[8138,100000],[8913,100000],[1642,100000],[261,100000],[3431,100000],[2550,100000],[5772,100000],[1545,100000],[2983,100000],[2806,100000],[5697,100000],[4069,100000],[3236,100000],[5629,100000],[4366,100000],[4533,100000],[575,100000],[4615,100000],[2691,100000],[8289,100000],[3765,100000],[7646,100000],[3781,100000],[5821,100000],[2008,100000],[5255,100000],[3101,100000],[5332,100000],[2327,100000],[4689,100000],[606,100000],[9278,100000],[1399,100000],[2687,100000],[5156,100000],[5378,100000],[7644,100000],[6889,100000],[58,100000],[1650,100000],[142,100000],[2341,100000],[8731,100000],[941,100000],[4293,100000],[3460,100000],[6959,100000],[4307,100000],[8936,100000],[7582,100000],[986,100000],[1886,100000],[4062,100000],[3048,100000],[6240,100000],[8344,100000],[5951,100000],[9436,100000],[6356,100000],[4430,100000],[8636,100000],[1439,100000],[6499,100000],[2659,100000],[1792,100000],[9959,100000],[2278,100000],[242,100000],[2395,100000],[5744,100000],[1316,100000],[1510,100000],[4015,100000],[3767,100000],[2220,100000],[3000,100000],[9134,100000],[9598,100000],[7415,100000],[3712,100000],[8436,100000],[1553,100000],[9393,100000],[1257,100000],[3183,100000],[1338,100000],[2264,100000],[9566,100000],[5301,100000],[5504,100000],[3953,100000],[2785,100000],[7387,100000],[4012,100000],[2947,100000],[5383,100000],[8481,100000],[9075,100000],[4999,100000],[5235,100000],[1814,100000],[7804,100000],[304,100000],[7328,100000],[2479,100000],[555,100000],[9405,100000],[8380,100000],[1638,100000],[9854,100000],[2689,100000],[714,100000],[2510,100000],[3396,100000],[9941,100000],[7353,100000],[332,100000],[6349,100000],[9552,100000],[2259,100000],[4158,100000],[8480,100000],[147,100000],[5887,100000],[7150,100000],[1265,100000],[3744,100000],[8036,100000],[2247,100000],[297,100000],[4112,100000],[6574,100000],[3317,100000],[2249,100000],[2006,100000],[3104,100000],[2277,100000],[8574,100000],[4920,100000],[7855,100000],[8957,100000],[6640,100000],[4184,100000],[4150,100000],[2486,100000],[5897,100000],[209,100000],[1874,100000],[507,100000],[506,100000],[428,100000],[7381,100000],[8794,100000],[1209,100000],[7382,100000],[3168,100000],[7014,100000],[8842,100000],[2326,100000],[1169,100000],[3208,100000],[9775,100000],[5891,100000],[7559,100000],[9556,100000],[6619,100000],[6907,100000],[2702,100000],[4109,100000],[1501,100000],[1587,100000],[9484,100000],[943,100000],[5467,100000],[1520,100000],[7524,100000],[7383,100000],[7890,100000],[4975,100000],[636,100000],[7437,100000],[1409,100000],[6785,100000],[5747,100000],[7370,100000],[1563,100000],[7301,100000],[4077,100000],[1249,100000],[8992,100000],[2033,100000],[6584,100000],[4842,100000],[5749,100000],[1997,100000],[4045,100000],[4719,100000],[6102,100000],[9562,100000],[2244,100000],[390,100000],[8170,100000],[1093,100000],[5836,100000],[1136,100000],[2629,100000],[8871,100000],[5152,100000],[5083,100000],[5704,100000],[5412,100000],[3314,100000],[8924,100000],[7252,100000],[2916,100000],[1593,100000],[781,100000],[690,100000],[3536,100000],[3831,100000],[6566,100000],[4880,100000],[3440,100000],[4905,100000],[8692,100000],[8346,100000],[3067,100000],[1506,100000],[2966,100000],[7889,100000],[6400,100000],[2465,100000],[4341,100000],[6995,100000],[2812,100000],[7148,100000],[388,100000],[6605,100000],[8339,100000],[4403,100000],[4319,100000],[1599,100000],[8048,100000],[5234,100000],[4577,100000],[7609,100000],[4946,100000],[6805,100000],[8664,100000],[9787,100000],[7409,100000],[8958,100000],[2130,100000],[9826,100000],[5888,100000],[1288,100000],[3807,100000],[4412,100000],[6741,100000],[6815,100000],[9766,100000],[1391,100000],[6972,100000],[2333,100000],[4433,100000],[6234,100000],[2636,100000],[2800,100000],[5700,100000],[7710,100000],[6919,100000],[7026,100000],[8747,100000],[2322,100000],[9362,100000],[7036,100000],[3584,100000],[3971,100000],[3356,100000],[4680,100000],[8397,100000],[8168,100000],[4859,100000],[3502,100000],[2750,100000],[9926,100000],[9028,100000],[2128,100000]],
+ targetFriend = 2145
+ ```
+- **Output:** 9998
+
+
+**Example 8:**
+
+- **Input:**
+ ```
+ times = [[22426,84367],[75329,93393],[16727,83539],[36174,69610],[1205,96372],[92090,92614],[54318,68966],[2108,45037],[33071,97071],[10136,32984],[61156,98710],[5352,26672],[69124,73060],[36589,67343],[9489,99078],[56391,79127],[88275,91841],[47717,71477],[77283,94145],[34279,62828],[93594,94673],[86554,86912],[39556,46739],[53675,75259],[49687,76186],[98841,99920],[71395,71859],[77560,90201],[19800,27407],[29293,36331],[19298,69257],[45777,51071],[88102,91436],[13402,91278],[75702,90696],[35933,80138],[5409,76632],[69427,76986],[22387,42315],[81578,87718],[23495,89502],[31443,58262],[78751,84610],[49378,73936],[57481,99920],[63048,83860],[29535,72348],[89222,92226],[91976,96524],[93321,95260],[2985,80101],[33096,45591],[34892,89098],[71393,75635],[71885,97563],[74351,80224],[70755,97651],[31640,57431],[85098,97725],[52658,58804],[45399,66232],[26851,39059],[81438,83643],[12196,47170],[3859,67920],[61379,85651],[28430,95048],[92417,94207],[93228,96597],[6785,41005],[57995,89468],[89198,89557],[83057,86667],[89833,89939],[44572,86692],[7582,67737],[23988,84070],[67055,98564],[17603,19265],[47502,78080],[7604,31781],[39812,71979],[92551,99714],[61947,79505],[24114,49424],[85626,87737],[58459,62467],[82399,98463],[29555,95257],[29806,71224],[53662,88132],[2228,95288],[14511,93026],[81984,85276],[25902,99856],[20185,29203],[98840,99213],[14791,21666],[26187,45536],[3948,23037],[46754,61956],[53204,97607],[73600,91312],[53148,74118],[8123,75415],[72830,81231],[71516,76002],[48913,61144],[53494,94575],[11622,30017],[89469,98388],[86631,90355],[70085,82969],[66542,82854],[53223,76940],[95413,98935],[84331,92617],[59795,96805],[62877,77297],[90834,92357],[98516,99205],[1335,30749],[36134,39169],[10902,15147],[85022,88653],[96363,97541],[46187,84429],[47101,91808],[6381,29128],[90360,93219],[678,14919],[31908,60036],[40179,82797],[67855,74554],[80220,95471],[98962,99729],[3438,37747],[64847,78496],[2696,41421],[31478,31717],[52990,54936],[98901,99063],[29069,43478],[63633,80235],[80021,80679],[48405,62811],[86712,92198],[11335,69289],[63829,96943],[88025,99197],[22243,78723],[39726,47031],[3674,76470],[28839,85178],[49939,98708],[70724,79599],[56434,88892],[46924,78658],[88332,98027],[149,51884],[31693,83801],[2982,8380],[53085,88474],[99479,99603],[74758,96516],[72069,88990],[89990,93045],[62167,84228],[12199,29553],[49976,56856],[67653,98565],[68522,76794],[67032,91388],[81732,92415],[6704,14173],[3813,57358],[30028,82717],[39697,52527],[44618,45237],[74779,96499],[87531,96157],[38515,90279],[57110,66630],[68505,70468],[77731,80244],[81590,99464],[72188,80823],[89076,94703],[10215,70391],[57010,61892],[95915,96351],[30708,80862],[85150,87096],[42273,99632],[17736,65771],[56542,81567],[19812,80923],[8790,96532],[53282,75169],[18460,34017],[73567,90066],[39660,66128],[38350,70951],[58029,97782],[85992,98758],[59643,60330],[9901,18076],[63362,79459],[93036,95316],[27086,66590],[97482,98421],[47159,57106],[68091,87246],[62998,84053],[27993,71008],[65251,89644],[56787,95879],[99756,99929],[90267,91622],[38113,58407],[10195,37281],[34877,40074],[39607,65623],[65455,79900],[36114,68163],[56663,67889],[1417,38537],[61050,61237],[38301,83359],[77916,87780],[87261,93797],[14058,91720],[31330,64165],[55672,81517],[68997,94089],[62667,78867],[3963,19956],[28712,70710],[6394,59813],[9619,64104],[61141,93587],[72435,85919],[67832,80741],[43963,60332],[83984,97463],[57057,95685],[52320,93603],[9756,93411],[54595,85196],[21537,30086],[98223,99192],[39391,83001],[37806,95459],[41221,61849],[66829,80570],[66938,67870],[5486,72858],[77781,83387],[84348,98225],[78065,93490],[31039,33531],[16457,70156],[14209,19784],[82300,97059],[33740,50997],[12047,67020],[28468,36426],[97068,99213],[3478,88527],[22258,49079],[60185,85934],[60584,69068],[57849,84085],[45296,91043],[66611,71188],[58381,97814],[93522,95858],[80234,95868],[37587,95517],[46237,66393],[7576,94206],[96829,99175],[14028,94924],[45511,72924],[64818,87927],[72073,95870],[86003,92808],[10535,90096],[88474,99431],[20265,54090],[44727,61451],[39855,99853],[78058,95770],[7753,33600],[8926,17869],[86175,89666],[48353,56177],[43190,48343],[32344,40408],[86994,91593],[27654,81640],[74482,80041],[4039,96213],[63715,87431],[31703,97470],[49056,99582],[84769,99647],[68154,93419],[76579,81675],[8857,15202],[31108,49021],[99171,99679],[85007,96421],[30875,42270],[5877,70339],[96721,99733],[25672,97795],[43307,76512],[98657,99995],[28967,98878],[48553,51349],[25816,93721],[38008,84766],[19255,35163],[26291,47157],[83208,99644],[62111,90534],[15135,86170],[79427,92868],[32762,81330],[63163,70693],[52714,98380],[65683,75856],[79354,89352],[41190,71774],[12235,62363],[97322,98922],[73708,94491],[55142,70966],[22283,97245],[7589,44241],[92053,95544],[236,31967],[87108,91959],[39624,96555],[46406,66387],[49366,88370],[75910,90240],[43610,54329],[19574,75487],[81398,97450],[12741,53737],[35128,72226],[76140,93207],[75332,97423],[3623,57463],[27586,33365],[43695,46517],[94491,97026],[4129,54210],[37666,72737],[58588,83221],[46739,80421],[64448,83219],[73637,97833],[35702,70470],[68590,69702],[88562,94633],[61090,93669],[48039,98210],[96115,96799],[83313,92443],[75875,86297],[74842,98135],[87830,88154],[15408,94242],[78783,94713],[75124,86556],[41918,73625],[73323,97655],[99722,99781],[52473,89294],[93127,98947],[59835,90666],[56441,73512],[8362,51749],[42497,63152],[21780,44212],[73997,80521],[39735,75892],[75844,93748],[66478,85845],[52432,95174],[67479,68741],[16601,29443],[34713,84904],[41111,74181],[3520,72076],[63876,71387],[82787,92161],[77312,91425],[55888,57472],[74970,90514],[61195,69943],[64505,98932],[76789,77274],[57579,91944],[81522,89328],[62948,91475],[18138,74002],[60097,77909],[1843,53563],[23969,37355],[1591,76309],[2468,79759],[30138,66474],[68490,85041],[97173,99794],[4812,78767],[79389,90575],[15209,33687],[17700,22542],[98238,98544],[54425,73345],[80635,83226],[37929,42925],[88089,96039],[80035,88228],[79645,84094],[50995,73302],[94847,99323],[75165,80519],[73466,96793],[30388,96068],[71442,95296],[40295,70226],[95890,98313],[28783,85570],[94710,96272],[67477,68343],[73300,74197],[87165,89172],[22324,64705],[76010,91079],[64870,90892],[61812,75664],[42197,84428],[95869,97262],[36012,97948],[31605,37549],[45439,86112],[21845,38008],[58580,67547],[23936,63840],[18103,45502],[12343,52009],[52626,66375],[18535,99047],[93829,97962],[34696,88698],[59738,66140],[31037,34036],[72053,88326],[56430,84134],[98125,98950],[48559,73093],[19022,63314],[49319,69016],[58718,80034],[59902,92465],[90353,99399],[61220,88425],[42657,78883],[38373,49348],[88565,96075],[90295,90598],[49206,60790],[37377,99168],[82776,94673],[57571,57917],[10987,92122],[20596,61302],[44192,94474],[42600,74985],[75617,98823],[26481,72104],[26982,74616],[57606,86459],[46626,83258],[71761,90584],[38254,66000],[51452,75779],[30441,51169],[24049,56382],[44917,77657],[78420,85763],[6094,80028],[74826,89880],[24291,44389],[98642,99176],[92535,95973],[23342,24936],[87458,99799],[56148,81201],[45938,58912],[91454,94117],[78124,84572],[73303,73547],[37153,86845],[48771,79396],[60812,99441],[96073,97597],[37142,81673],[7577,49807],[65917,76425],[11717,34475],[15128,46673],[28548,41254],[92586,94406],[38778,60026],[3677,65260],[75701,91572],[75242,80784],[46789,58349],[85767,99516],[65706,99091],[14837,90206],[29640,41288],[31985,48614],[88779,90001],[24757,68416],[47413,50381],[4178,22530],[40352,85901],[19604,71192],[86916,86975],[21171,75560],[65805,80238],[42830,82340],[24899,88054],[56134,97448],[42701,47602],[58363,68270],[89920,92757],[51054,82747],[50721,64513],[41356,88401],[41305,78768],[29497,71908],[14971,46129],[82610,97544],[9918,16694],[61726,82112],[80052,86778],[71643,95856],[71069,85706],[42537,57054],[37321,70356],[38241,46636],[57903,68396],[66913,85123],[48984,84016],[44418,81262],[78504,89629],[41328,66551],[98448,99926],[32716,65094],[73952,78187],[46422,78204],[56842,97022],[83370,92934],[25920,27514],[95634,99101],[44552,89978],[19620,50573],[35553,38433],[48227,50257],[54468,63904],[99614,99925],[30535,60628],[94526,98320],[60388,69792],[90904,98831],[30118,71467],[71775,96570],[56101,98234],[10501,19756],[7117,85719],[58587,71568],[79303,81786],[92409,94340],[65927,74124],[39535,95120],[78138,81117],[31410,96506],[53466,74861],[95491,99590],[97837,98372],[87978,93281],[88619,96970],[90802,97197],[89194,99164],[87645,93682],[23966,29478],[1301,79547],[68014,79451],[68926,76910],[71005,77469],[89581,93182],[69588,70220],[72074,89276],[44580,73163],[59479,76358],[39412,74221],[31804,39709],[23190,29321],[22389,80342],[13846,15387],[22017,37892],[37540,49660],[86329,87637],[81648,99723],[91159,98234],[71493,71583],[70796,81068],[42290,64284],[82604,99813],[81971,91327],[93287,97119],[31435,49207],[62565,86692],[67474,76533],[46357,48400],[79126,83431],[77664,99322],[70349,97088],[35633,45586],[19293,96941],[90968,98931],[99325,99993],[37735,94667],[85578,87619],[78234,87316],[23657,46130],[58471,65969],[90971,97791],[73762,95683],[41681,58801],[80564,88727],[14418,19289],[28874,64764],[46776,64140],[46252,78555],[18428,52269],[35533,82677],[34336,80432],[92680,94109],[3068,62921],[77481,91087],[81477,92134],[86187,95039],[55209,80113],[20755,85501],[89853,95138],[39960,50195],[81683,96827],[30655,39046],[98770,99647],[46438,63042],[53834,78302],[11402,66208],[44836,50345],[86964,95236],[23574,79410],[55747,55928],[96185,98563],[74542,82177],[88802,95129],[71324,96837],[22191,84047],[95597,99004],[42621,94468],[30688,87769],[72987,75551],[49474,85486],[97430,99133],[91886,97468],[35142,87983],[85327,94294],[62910,69504],[35503,50745],[28375,78510],[12281,50185],[66872,83097],[23789,47221],[10402,80443],[83539,86687],[32500,54058],[13690,58156],[88299,88553],[20932,79902],[6687,40927],[7030,40261],[85380,93536],[25870,99270],[84579,84674],[79626,96311],[22717,92528],[4239,92497],[43588,57494],[74622,89623],[35676,42775],[70532,74898],[65599,92629],[66971,84013],[6436,97156],[29231,94651],[125,40738],[28167,78800],[24591,74884],[95737,96026],[93986,99703],[4546,24759],[37130,51345],[89806,93558],[72138,93650],[89421,94458],[54307,62157],[64763,88598],[95423,96149],[57918,94728],[81083,94220],[95562,99610],[91106,91950],[56825,80948],[3222,55038],[19126,52265],[42957,90094],[64684,73237],[8292,27930],[89578,93552],[91428,93574],[92049,98031],[1372,66535],[97026,98842],[57036,74386],[75175,90327],[63523,81607],[35020,39700],[95065,95755],[42371,46834],[29473,45677],[47060,56928],[21076,53830],[75056,93839],[32459,96711],[5714,30743],[75861,83690],[25709,94826],[79957,83923],[41930,99738],[72944,86724],[26591,88582],[87533,88263],[30839,55068],[63063,79913],[81565,83140],[21411,96484],[77220,77837],[23832,25675],[1635,84970],[87004,97515],[12062,81684],[19091,53626],[38027,48337],[24485,51846],[65560,68205],[21592,25574],[90784,95988],[50423,57740],[57516,84845],[40229,41790],[55386,66675],[75863,98459],[95593,98943],[88536,96543],[84598,93165],[50241,61200],[40249,55421],[56612,68501],[34206,98792],[58589,70391],[50144,91796],[31556,64756],[23629,38942],[87270,87477],[54371,84369],[14122,39854],[7307,10295],[76798,90680],[8148,51777],[85400,96761],[95942,99738],[67247,86279],[81044,95316],[1707,85132],[27906,65468],[83789,88109],[2252,55742],[83919,85892],[75515,87686],[88915,91772],[76659,91333],[90598,99030],[6741,25853],[23114,75776],[3559,69016],[17926,61908],[65545,90774],[81264,95027],[33505,39786],[2167,6486],[9849,49274],[55183,65417],[9672,23620],[47766,78472],[96703,98436],[72470,85518],[57091,88917],[16448,41021],[56431,60550],[23563,52211],[51328,59078],[69280,90040],[53102,54862],[89415,92694],[14490,77208],[16047,31903],[36105,38743],[59237,74417],[23466,26539],[45986,68593],[91104,97407],[25201,65621],[28926,45135],[36947,49945],[448,43180],[10049,81539],[62107,77292],[67231,73319],[78136,96011],[22957,44920],[12517,35307],[61339,79880],[37775,89839],[31186,95269],[18805,68101],[88259,90022],[87870,95397],[40358,40372],[80229,88389],[62407,76476],[37867,50656],[58111,95468],[333,73363],[42211,58621],[83289,89534],[58036,99105],[72211,86938],[31492,53501],[6673,85690],[90949,91126],[78626,91246],[64306,84793],[63004,89028],[8233,34542],[26972,27756],[72898,74372],[58611,58768],[65524,74075],[27103,70782],[69292,95218],[3215,23760],[97505,99237],[72148,75375],[82727,95606],[99863,99875],[53141,68779],[55991,61936],[72768,77869],[54709,81475],[2418,41526],[31913,43454],[65742,92004],[2283,33261],[44555,99768],[23993,24665],[10459,83537],[14406,17592],[53707,63765],[32258,50972],[84099,88009],[41116,72538],[39658,99952],[50486,97707],[30581,43472],[81667,96233],[98983,99816],[88552,99244],[83516,90881],[66486,95108],[65293,70674],[73469,75526],[31880,52097],[33940,56537],[40837,67183],[63341,95769],[35248,47637],[2157,11774],[14529,50594],[56064,82779],[68853,89037],[91003,92075],[21135,55839],[90394,98656],[38688,56973],[34278,47547],[20422,20508],[4216,33327],[27758,33968],[38107,51265],[10690,61346],[9975,72108],[40769,79314],[92463,97380],[68184,71245],[20634,40577],[20008,68607],[63482,80250],[15018,23998],[94183,98966],[28854,54198],[73013,80236],[86262,97209],[77118,83145],[15041,54460],[12655,64595],[69719,82453],[3660,15169],[4432,18646],[48833,66816],[56202,67809],[30679,99720],[25359,68562],[52000,78281],[85947,86371],[53554,70929],[43006,60625],[19379,58275],[16294,40336],[33934,84569],[88189,96549],[97338,98590],[53339,55125],[89775,94688],[5732,33911],[87912,91898],[17574,38202],[54106,93643],[69533,87258],[83708,86329],[64481,98824],[55756,87507],[99636,99851],[55497,64622],[20704,80181],[85207,92273],[8225,87462],[17727,25563],[72923,96086],[95089,96251],[39281,64429],[97488,98592],[57078,95657],[72347,89028],[5869,34191],[45318,53885],[84243,94381],[39407,83889],[90545,91295],[90984,99136],[99247,99671],[25952,59723],[64881,98925],[20800,87013],[24154,49811],[48460,92077],[55709,76882],[22858,32491],[33396,34036],[24784,43269],[49627,70032],[41449,85319],[72644,88351],[86240,96273],[76644,94151],[78453,78524],[30155,90556],[2268,60560],[62696,82719],[28947,59678],[20812,56466],[90438,92448],[73558,95435],[86619,97866],[24309,95288],[75698,98800],[18498,93133],[27540,48180],[90839,94337],[66781,95963],[36883,87923],[98479,99089],[99199,99658],[21320,67026],[66553,94266],[49087,55360],[39861,46845],[89357,94817],[9612,32184],[89673,90079],[22646,38309],[19920,62676],[69309,96295],[48324,80018],[32155,43087],[74488,76507],[50059,97273],[90892,93468],[89984,91815],[71172,71805],[575,6976],[41497,96773],[18544,53310],[21482,96391],[13070,32395],[28177,92554],[19101,88150],[32590,98099],[58293,66155],[60032,87060],[89624,93491],[46300,65495],[62220,87836],[30849,47611],[95747,96806],[42231,75772],[16660,87437],[36715,83215],[57836,86332],[19157,35344],[51633,71685],[38697,96417],[77469,88371],[17464,32623],[87666,99499],[66811,88612],[73596,81656],[90441,95768],[21429,54595],[75502,93233],[97523,97675],[57730,65253],[79875,82727],[45169,99525],[96000,97543],[44157,92978],[33920,63437],[41890,81559],[75211,78626],[20686,31794],[80151,83848],[36152,36679],[28669,31900],[53461,94279],[87432,97588],[16301,37620],[76970,77775],[4373,23130],[9833,77794],[66353,81799],[79569,90090],[37864,49826],[22706,70722],[59908,89038],[50176,90229],[93034,93747],[42900,92331],[32215,84593],[61513,77792],[5427,54184],[17511,84485],[70049,84524],[30076,99727],[15173,96950],[96207,98375],[75281,93908],[32034,43939],[84078,86353],[87283,87485],[83308,90532],[64784,92850],[41968,76869],[56058,76485],[15888,91554],[10006,16648],[93412,93643],[23494,32401],[46066,49835],[83449,84106],[38414,87491],[15540,74273],[51895,91436],[82529,89831],[45523,57208],[30192,31489],[3346,74903],[57915,64269],[34759,77001],[63084,73479],[62560,79274],[14099,63541],[75191,95044],[82430,87521],[69044,92284],[91210,97706],[56595,63669],[1803,26968],[2397,56719],[81699,86313],[3776,5200],[23335,25967],[19054,38352],[83684,89342],[61210,83060],[91655,98307],[18056,82254],[91348,99027],[11979,20754],[45995,97290],[63451,66422],[31521,46659],[78041,81431],[93371,96679],[63880,91951],[42783,88187],[27235,79380],[52831,58457],[17409,47401],[56773,86834],[7879,15117],[71441,95597],[53839,58972],[38149,93628],[16104,62081],[97734,98064],[16511,53590],[29236,87454],[75027,96688],[64155,96073],[18796,73189],[3365,22140],[61842,80699],[25141,61498],[11531,32284],[53389,80046],[28313,60880],[85251,93384],[5114,42964],[17681,20940],[76901,86870],[16663,57227],[52721,78805],[18929,26447],[28670,33388],[17265,54024],[70215,72076],[49390,58037],[64377,76797],[4024,91734],[95135,97716],[49741,55632],[43741,46290],[56933,69294],[66252,82828],[26624,43351],[63904,96355],[81000,83426],[8906,51221],[22255,88414],[74405,96915],[90176,97480],[19331,58173],[98346,98871],[7615,74672],[31621,96644],[30974,87886],[15817,56312],[99573,99777],[41414,62179],[8528,97896],[2512,95901],[96675,97525],[50257,73761],[80446,84555],[35953,74538],[39,17568],[72686,85599],[73759,94566],[67111,73889],[80955,84215],[65451,66602],[21752,98254],[91262,92332],[88715,89342],[66234,81442],[66704,98917],[83123,89321],[43424,52544],[88847,96171],[31787,98835],[6637,69599],[11438,64526],[44765,70820],[91748,94578],[74132,87491],[55768,56256],[36512,73308],[622,65796],[93088,94510],[91813,98495],[11956,90341],[89186,99030],[65164,99302],[4395,5014],[84929,86933],[20311,56387],[10235,19881],[66507,88678],[11919,91484],[31764,60000],[25938,59912],[14632,54798],[3311,97263],[18032,74290],[99113,99377],[86417,94156],[7756,72894],[63810,68102],[10246,56133],[4253,77986],[81885,92683],[57892,80742],[83892,99709],[97133,97592],[31516,69787],[12595,56180],[62513,69500],[69102,69116],[56490,96050],[36567,37343],[96582,96725],[46746,72959],[40408,51681],[24486,47476],[99201,99847],[78610,97370],[51817,60138],[23704,84730],[83732,96219],[74890,95052],[5895,28112],[71628,95792],[88878,99055],[47899,79643],[80387,89794],[82837,89875],[97887,98021],[48528,99554],[69255,97214],[51277,66323],[92946,96488],[53012,70484],[15526,71153],[54857,75352],[76382,77249],[51184,59735],[56106,60400],[7534,97961],[10730,73444],[94925,99131],[44808,82695],[89550,91294],[84352,88361],[52623,98090],[40009,84564],[5315,8500],[80594,84424],[65702,97570],[22991,45209],[7207,30649],[43417,51054],[72080,82908],[78224,80640],[33246,94765],[98104,99069],[31451,62554],[77241,77306],[19621,86506],[61612,77568],[97401,98666],[79203,83652],[58347,68849],[39359,85341],[88274,88317],[23316,59008],[40909,77109],[51075,98051],[87600,96476],[22542,64125],[57475,80526],[28205,93166],[63975,74620],[41809,73481],[9674,87444],[84234,98301],[65944,85149],[86925,99845],[68,71947],[61151,68123],[67447,70377],[76860,79945],[27671,38392],[28263,45884],[12683,78646],[31421,76247],[96138,96380],[7717,51054],[32824,75707],[18642,93009],[9538,15670],[31639,35817],[65598,77776],[17591,69691],[67661,74006],[45912,83523],[89941,93522],[67386,89959],[67046,71249],[14757,82983],[98919,99683],[48636,85097],[70451,77537],[89172,99461],[63347,86782],[39640,98657],[90670,91813],[83228,95920],[73937,93864],[21693,61118],[43451,55461],[91786,97477],[57055,61735],[17137,80337],[71563,96822],[28933,75964],[89702,94908],[26908,27588],[16648,96876],[99135,99463],[93092,97223],[84394,91472],[28112,59946],[43053,84241],[81611,88914],[7314,30771],[32418,73929],[68104,96870],[61959,97097],[74625,95493],[19064,76517],[53214,67257],[90384,91321],[71759,95280],[81586,89584],[53271,90633],[88956,97821],[50826,91476],[14199,18065],[43088,95980],[35657,55405],[66399,66499],[68520,76210],[92379,98010],[39521,85875],[45966,99692],[39210,98871],[76830,81340],[91284,94286],[63563,81234],[12099,79689],[52584,89239],[8332,99740],[27735,87082],[95370,98838],[75403,94876],[53765,88467],[94890,95530],[50845,82478],[19066,93728],[92783,95437],[92439,96211],[36127,80335],[40008,68127],[92129,97604],[51621,76091],[97613,98577],[72900,82797],[20118,27426],[62762,98069],[9255,11338],[99350,99937],[27349,43190],[80724,93400],[75728,98717],[471,5598],[51509,85335],[61889,80925],[16112,67908],[65544,69147],[78981,99213],[51444,94703],[31773,43401],[67948,95780],[99275,99457],[69788,82338],[79828,85039],[76388,85928],[50551,61466],[22205,67592],[35527,78333],[7718,27570],[15009,74551],[5066,87208],[83094,83776],[7546,79524],[8961,44170],[22440,27617],[41590,68240],[37081,76946],[54252,67046],[92220,96283],[21960,33060],[94665,99652],[24655,48542],[13446,95218],[9715,43821],[59932,80270],[73572,99026],[7939,81464],[55824,63445],[89888,92387],[20549,87207],[55228,59532],[58659,95730],[72623,83017],[26595,31320],[98500,98810],[70040,97580],[60251,88631],[80378,84122],[24501,85122],[50866,96174],[50710,87904],[97825,98693],[8598,93398],[76680,94100],[32270,95780],[34643,70801],[88621,91506],[38698,62152],[86173,86866],[37139,37247],[52668,78467],[22331,36957],[57476,73806],[55858,95412],[88789,91313],[8575,60717],[5745,81119],[76300,93796],[35871,58372],[24092,69384],[11071,39820],[69959,99590],[55162,83873],[17366,18913],[35936,49623],[43060,94079],[56633,90841],[11192,94261],[88770,91805],[62822,80957],[11519,17391],[64756,75248],[21425,37082],[28020,51584],[35084,92693],[53065,78602],[96750,98018],[31952,73510],[58963,84100],[21258,80926],[44087,83275],[94893,97521],[28134,35971],[36031,38366],[17679,82194],[8912,55787],[34538,97652],[40108,97419],[61515,78171],[87668,95398],[56245,71462],[9378,79503],[51596,63337],[54984,63175],[53960,93664],[57692,61213],[66202,93796],[4088,21464],[22062,74318],[52944,68405],[35061,70174],[40371,69197],[21491,70801],[6853,63638],[7077,64342],[91955,94161],[77244,80193],[49776,69522],[79664,92700],[41050,68216],[8002,30200],[37906,97892],[70373,93541],[48620,87621],[7200,54942],[9863,19599],[63722,83191],[38905,97787],[84566,98917],[15616,84633],[77728,95161],[54548,62698],[78432,83214],[50332,90878],[89140,92888],[4550,15744],[30336,87262],[82069,83909],[82288,91503],[12955,90342],[19915,41539],[46285,73377],[74237,84777],[39256,67919],[39133,95782],[44126,78771],[32488,75165],[23852,45308],[49033,78079],[84764,91800],[72938,92453],[61936,93996],[30724,99096],[29467,51878],[36006,66017],[61548,96086],[39332,96515],[72494,75098],[3826,29002],[52849,79566],[8060,29576],[44878,59757],[22922,48095],[40903,42551],[92341,97680],[32811,62697],[69544,99018],[61503,86628],[60612,76615],[32641,99027],[49455,98189],[38717,68998],[50384,85843],[43390,66018],[74908,95606],[55977,63768],[52292,69100],[78517,94184],[47726,57005],[20186,83212],[80968,88040],[84741,97014],[85155,96376],[91901,99277],[67875,75368],[65165,83497],[25174,40431],[3766,84176],[37171,57813],[88109,90671],[106,32593],[95794,99022],[43993,44946],[32009,87843],[66867,86472],[3219,48596],[99856,99938],[59735,98426],[88386,98152],[97565,98995],[25075,61018],[68190,81266],[13544,17789],[32106,42592],[62881,88062],[62208,90396],[79263,90396],[20814,99136],[97374,97650],[23560,38010],[41807,42681],[27063,46226],[25232,83693],[93340,95477],[58184,60892],[58902,82501],[70770,77259],[38843,91174],[35966,47584],[80360,89944],[45261,54919],[74675,90003],[29523,68344],[68621,77061],[84158,84574],[14219,48358],[91150,93486],[31884,85398],[92391,95962],[25216,42613],[31311,49742],[66459,70398],[33645,61907],[98805,99283],[86323,88975],[54278,93262],[24051,41853],[5338,61939],[44034,71436],[67848,74340],[78010,91287],[30881,44204],[30431,96869],[56719,84011],[66192,77185],[18107,81349],[13772,40461],[55930,91042],[54002,99704],[92970,99526],[83353,90755],[89012,95956],[81380,86438],[44875,47485],[64320,71093],[7721,62189],[54052,77011],[70015,97865],[53757,96004],[96037,99847],[35800,93019],[1144,48820],[60786,61502],[87056,88890],[98170,99490],[32304,83666],[22841,42174],[64286,93009],[89346,96128],[6925,19385],[50538,80961],[90461,92897],[82326,86558],[73238,83915],[45157,98684],[45486,61522],[60416,94832],[81345,88307],[20818,28775],[91037,91240],[99701,99799],[96967,97729],[23912,90835],[28921,83426],[25209,73822],[12380,70151],[80221,84080],[65956,76971],[66005,92915],[57087,65385],[76432,94714],[81550,95273],[3474,72249],[39623,71746],[13314,13995],[6970,22479],[68434,71769],[4673,55926],[43794,84659],[92407,93136],[51212,79321],[33712,39716],[22827,97213],[68532,84828],[3226,48807],[93170,98180],[15379,18946],[76960,90804],[7426,57776],[23647,59572],[93833,96393],[44712,81641],[82654,89005],[23022,51290],[9224,78307],[36231,48915],[91627,94962],[10150,62801],[57796,57829],[10716,28110],[67334,77450],[47893,67953],[42840,73364],[4455,68467],[4578,58282],[59180,74236],[53247,67460],[1073,80486],[9685,44893],[50450,61033],[36260,94002],[3420,97433],[39108,55902],[31069,81591],[54195,58716],[1841,13835],[35098,89546],[76592,98644],[7821,39601],[38714,98484],[72402,79471],[47611,69236],[73482,84011],[53498,85868],[12646,82748],[4973,60191],[41880,91249],[35567,62859],[19705,63796],[20987,63474],[99747,99794],[98318,98471],[80738,81255],[31736,65949],[21365,89916],[45275,53609],[48092,97940],[71467,82492],[81347,98514],[53283,70650],[8421,99802],[37502,46212],[72036,91979],[2631,33425],[70991,86348],[46620,52879],[18895,57899],[75274,80376],[63081,92089],[33067,76220],[72821,91315],[16723,39992],[83569,97773],[58359,69375],[30212,37769],[86339,94766],[18372,80296],[49284,53232],[51020,73183],[99649,99756],[67957,93008],[65819,76965],[88646,92200],[24350,42915],[33508,62971],[77063,95587],[172,60758],[83590,93946],[21210,97903],[6979,29937],[54960,60652],[76759,79871],[38418,39195],[80161,94945],[15205,32412],[50777,81367],[7568,82777],[54325,88900],[87175,87241],[75821,97969],[37932,56650],[6705,47144],[71729,84517],[88028,98381],[27987,53575],[68857,69804],[26485,60043],[52562,65983],[48462,85275],[52750,72106],[7371,69673],[57465,84727],[22509,72105],[86141,94329],[81774,92668],[7098,95655],[49401,89936],[60729,70295],[15880,41157],[62564,98209],[18087,24758],[18868,59779],[60326,67372],[1112,40858],[34865,64334],[15235,27567],[98017,98054],[96644,99561],[82091,90785],[62322,75071],[23476,90150],[46087,96535],[92209,97500],[34899,40214],[97245,99453],[36826,46373],[45602,74714],[72806,78161],[30482,92880],[67113,90016],[17564,71602],[83441,94233],[73512,86575],[8571,23924],[7318,92524],[14182,98673],[28697,88078],[92962,95688],[27704,90662],[70295,90277],[78201,99879],[486,9411],[52773,82833],[41910,69752],[11850,75713],[5711,88705],[79428,81706],[27674,49779],[91196,93105],[40693,76356],[28953,42223],[51692,53123],[56835,89729],[89304,97186],[8258,43887],[14440,69516],[40365,59635],[97546,99853],[98290,98468],[27791,43697],[67954,92436],[92869,95808],[24196,67466],[31182,81412],[56700,59861],[70250,96089],[31950,74639],[41748,54483],[52037,75503],[85725,91212],[98256,99212],[53310,99339],[36694,68031],[79789,85761],[2207,50354],[27652,39922],[745,44822],[83494,96462],[10380,12406],[439,62968],[90627,95378],[60531,98955],[15346,44456],[30444,92253],[71257,78009],[66270,69486],[26625,89665],[67679,96039],[35418,65252],[60805,99419],[52490,83975],[18573,74694],[8734,58600],[19024,91528],[70435,80362],[35849,74723],[58990,73077],[79196,81615],[6948,54299],[36233,48926],[17446,73301],[62195,99230],[55883,94524],[25759,49239],[35560,39823],[42362,92190],[76583,88973],[90875,94310],[45055,53790],[57643,66823],[91212,92750],[59656,91558],[29785,56574],[50189,93780],[65375,84871],[68179,86237],[57893,65822],[58884,69145],[48272,94373],[91085,96571],[78843,87125],[91770,95568],[46342,60850],[49016,79844],[13379,78825],[53002,62893],[99039,99493],[78793,84343],[95995,97052],[9204,78606],[86391,99673],[74382,76491],[82025,88808],[62669,97146],[61566,85359],[64864,99880],[95439,97584],[1376,50189],[35710,51100],[80074,82901],[70625,87733],[50567,54028],[48609,89497],[59521,72790],[49495,55806],[55349,80705],[41166,68217],[78833,87515],[93085,95571],[75302,88837],[70807,96336],[89139,89145],[9530,70504],[93907,96086],[33108,34558],[9569,36823],[98815,99873],[58653,80674],[42306,54352],[84736,97380],[53741,78320],[74736,94514],[7686,77983],[8034,87146],[54342,97103],[46840,75492],[324,64465],[23572,27536],[48079,92968],[6945,66768],[29865,33206],[53323,79041],[60310,69480],[46968,69104],[63074,79303],[97320,99939],[44166,44897],[12870,43513],[45095,78349],[63972,83000],[47632,99676],[40112,90292],[9638,25450],[41703,97460],[93406,96840],[32306,35660],[66738,70587],[68637,91974],[55105,76337],[55107,90724],[40277,48253],[1212,18197],[46694,92741],[20620,77101],[44258,80357],[48133,50823],[57491,65902],[96395,99863],[57500,88437],[76280,97627],[43033,96363],[74387,91952],[92392,97903],[36879,85609],[64368,73950],[48303,64552],[53693,85151],[63214,94144],[84382,84939],[13812,46579],[26896,50831],[71935,89144],[55669,62133],[3080,72619],[70279,70493],[9916,34517],[68188,97707],[86344,88396],[56901,88494],[76203,83721],[12652,72585],[69879,71606],[79949,89479],[24373,99717],[46280,53373],[39803,98444],[21735,71518],[15955,73350],[54976,83285],[5712,82606],[60063,97083],[26799,50349],[5362,6051],[78311,79685],[20192,38068],[56686,83916],[55816,93746],[16563,28985],[9503,90524],[38931,52860],[40705,99913],[92492,92654],[60325,82234],[96886,99297],[51791,77807],[26738,56927],[88827,95479],[99544,99604],[61867,64034],[92885,94990],[53823,64217],[42882,69222],[91358,95864],[51677,77773],[7526,41967],[58448,74641],[65296,76520],[29037,36198],[22919,65688],[87730,88154],[86982,90000],[23927,41863],[93480,99558],[98718,99772],[4508,78044],[16518,56790],[42596,87264],[47321,73606],[61968,64297],[72140,98982],[78055,83745],[29410,44289],[43106,82829],[8834,34577],[19844,54681],[34624,42067],[18924,82132],[3803,94369],[17995,62313],[71640,84731],[11632,55107],[45892,70016],[57586,70617],[82573,93790],[56127,98210],[75545,79004],[46766,77383],[37736,63757],[92528,99101],[14616,17403],[77115,89382],[7692,62547],[26124,80538],[13354,97054],[27092,36608],[97378,99771],[52440,56921],[54822,71449],[2337,77363],[96125,98286],[99802,99996],[88898,97410],[75758,92153],[94566,96331],[11843,41847],[67172,77256],[59907,87189],[20890,76362],[39279,49358],[38464,98733],[10900,85049],[14725,75804],[74795,84339],[59681,70712],[93524,95434],[63409,77326],[87917,95059],[3689,61201],[41384,75712],[35828,57562],[9882,93983],[96359,99835],[72493,83468],[86072,91953],[91555,93686],[91595,96668],[54796,86815],[63999,67920],[82354,95294],[66556,74108],[54160,90579],[36250,69855],[13411,47422],[3716,10120],[20221,47147],[45259,72982],[25214,37809],[24524,93028],[27479,96180],[58820,71859],[4711,68725],[95509,96436],[60881,65615],[44326,72217],[6330,13019],[88070,89087],[33640,58395],[48363,96929],[38126,65842],[77086,96525],[59453,97227],[28069,71605],[42117,72187],[91608,93396],[51494,76229],[52712,80292],[47448,83087],[31322,51546],[97760,98067],[6628,66774],[58525,74844],[26058,87568],[73076,82948],[40043,48610],[86695,93321],[3874,31350],[45288,55527],[12484,21279],[74593,95445],[39872,76118],[85529,92329],[22852,69754],[37272,65467],[73309,73794],[17286,25363],[20421,23311],[43881,51748],[81415,86141],[75772,81687],[47709,47817],[81610,89484],[14967,55952],[29798,87432],[36461,99339],[74685,82455],[60336,75570],[60657,62618],[24593,72610],[43049,54066],[79831,95827],[14901,43524],[46380,62816],[57792,77911],[73966,92570],[20888,53734],[23056,43582],[62681,92104],[39643,44669],[54148,72691],[74712,86018],[33553,90774],[98911,99726],[47810,63094],[21941,71254],[92513,95362],[14696,87075],[55243,68808],[24718,70380],[17338,97256],[3496,79269],[10377,14318],[97088,98970],[92443,99681],[44002,58641],[61582,80239],[5102,72813],[76238,92574],[24559,27532],[60943,61830],[78339,81773],[46428,82549],[33657,79854],[75666,94115],[95756,99552],[34686,82331],[26991,67342],[17078,92447],[83787,88488],[88306,92512],[5507,81113],[93654,96493],[59319,89808],[33321,36268],[8609,94320],[84060,88539],[68517,79731],[28127,89182],[14394,94462],[53194,72570],[86831,91496],[32518,91739],[76867,86339],[57833,92437],[28098,48948],[48211,77023],[34847,66393],[99075,99193],[41211,80546],[43660,77727],[89105,98252],[90093,96305],[29491,48468],[13640,43611],[39992,48174],[58219,68952],[32044,35759],[91528,99280],[80733,83022],[62244,64410],[31220,80989],[86385,91585],[73251,74328],[14088,57319],[15179,46092],[76292,87242],[90590,97985],[51537,77903],[42854,81863],[2085,86623],[89618,96979],[4403,34070],[14681,41229],[40044,80374],[86492,91823],[60988,92910],[76534,86631],[45077,73915],[66039,90840],[97704,99654],[78875,78958],[72044,97317],[84264,93729],[85236,94170],[73003,92361],[63800,88936],[21568,62051],[77042,77696],[77869,93353],[11232,59429],[33413,94385],[3241,29867],[29304,97785],[87333,93159],[58799,59246],[60589,85943],[55322,64419],[85393,89892],[37632,65840],[35647,92961],[19093,91976],[26218,30649],[29476,92462],[13207,91705],[91419,96884],[58108,73244],[44998,53560],[76847,81837],[22154,64065],[55525,72480],[76076,80753],[31762,66486],[26077,72395],[3829,75512],[22364,72965],[23923,60012],[16431,42018],[60356,74938],[74019,97588],[53240,81672],[74385,76502],[84563,99394],[66275,69851],[42797,45873],[71424,80428],[58007,90623],[25392,86372],[57787,78116],[86690,94960],[29770,44530],[45802,94962],[98618,99001],[36813,65874],[23999,37958],[19978,80514],[31430,49341],[75390,92801],[6794,87837],[77893,94075],[27700,94450],[9283,11116],[50044,51477],[8799,78990],[56620,82901],[40360,68348],[42650,63340],[32892,84792],[71866,74283],[64469,65589],[50245,91373],[5359,94124],[97776,98106],[90539,97036],[6111,6272],[7764,14875],[9164,95616],[597,86297],[20110,41120],[39670,94066],[35471,76159],[60782,64769],[46649,95674],[78022,91508],[75046,86337],[70646,82934],[25995,32066],[50689,92604],[49148,80817],[58442,69674],[5182,90604],[94582,98804],[31734,79326],[60456,91198],[28413,58464],[20826,42486],[72832,75881],[56791,72547],[48180,77995],[14756,93045],[24430,82889],[98532,98686],[49955,53478],[33373,63976],[82000,89233],[4461,67687],[50068,73973],[80232,84229],[11314,20352],[8619,42266],[16176,42428],[84256,85182],[66641,77664],[33306,49807],[67403,96219],[25222,81467],[56602,93311],[66122,94076],[5772,13309],[62988,90949],[8696,76994],[23990,41773],[87110,91327],[1686,80590],[56237,95900],[36154,97368],[65264,78141],[65395,67761],[65390,74621],[11552,97432],[14866,25207],[85008,94848],[66667,78908],[75748,95418],[69386,84846],[80192,86688],[53602,64327],[63600,67920],[18515,52878],[27682,47071],[42782,71156],[94131,99751],[88443,97624],[63128,71888],[10421,80029],[78460,97400],[91207,94012],[85359,92016],[61193,89856],[51189,80790],[8608,19393],[12366,36870],[77342,93191],[57232,89690],[83656,87124],[43279,56799],[71306,93346],[52367,94557],[33459,55845],[36943,68217],[48091,70843],[70125,74910],[38587,44776],[12813,39607],[76234,79814],[10877,96081],[6256,58109],[37149,53261],[15330,40061],[46492,82088],[73606,93518],[93366,96536],[88570,92771],[64086,65303],[47161,66153],[78248,92833],[25840,69596],[18556,51149],[90382,91028],[98980,99463],[88716,90867],[98116,98269],[39209,59880],[2291,44945],[70241,84445],[5067,44628],[96459,98241],[90611,94139],[91094,97725],[91179,93326],[87076,89017],[72968,93486],[45981,78612],[34030,77904],[79192,93901],[8555,80657],[99109,99678],[1142,71995],[89020,99376],[30011,69954],[67914,95874],[69389,94674],[95874,97907],[84969,88816],[85447,90295],[31334,35114],[81961,96325],[73874,82498],[25193,96707],[54343,67389],[48366,67790],[25342,34815],[85810,94235],[45685,76168],[20799,47830],[81476,90093],[86610,96382],[18952,95661],[34698,35020],[21809,63151],[33015,73723],[32756,96844],[39571,81711],[36111,59858],[87616,87900],[14993,89626],[95540,96466],[13362,27418],[49327,67451],[85779,95980],[8512,70855],[38378,93523],[2166,60671],[72591,98800],[20380,30077],[42791,97629],[8854,94918],[98799,99712],[94472,96961],[60500,62979],[73050,92833],[8092,54880],[73906,77920],[26690,67140],[60605,93784],[58586,62591],[69441,76914],[28100,40941],[32053,58387],[92761,93884],[7735,79379],[2253,60458],[23197,47019],[7709,90906],[29552,50783],[48576,53495],[79836,92407],[57229,84897],[33195,60149],[25807,57374],[65818,89607],[65061,97837],[86509,96319],[12918,23867],[8815,21055],[86320,89127],[93780,97878],[91026,99112],[26026,46698],[27183,44984],[18243,70838],[70431,82557],[8514,79396],[98321,99882],[60813,98227],[37331,37646],[5278,33584],[5181,80099],[35377,51964],[55932,99449],[23375,55391],[71130,79562],[84199,88724],[58889,89802],[94716,96750],[61230,71081],[78852,85709],[55795,97337],[16482,99084],[29653,63317],[38105,40549],[16551,24263],[78690,91622],[8764,80155],[87202,98967],[5193,58839],[5780,96672],[44985,97704],[29353,91426],[28620,61958],[99128,99901],[38154,43244],[36072,76037],[85087,88001],[1757,62075],[13566,96710],[98896,99312],[98246,99451],[5675,66018],[19632,28551],[24339,94231],[84104,94723],[10957,73675],[24232,32471],[82946,89496],[70742,84607],[46277,83984],[67228,91424],[78165,99316],[13951,80610],[59355,61510],[66166,81452],[70453,94179],[54680,99706],[10339,28110],[19200,82297],[50733,53695],[8940,20774],[93416,96177],[53019,95559],[89600,97464],[51591,65087],[3855,94227],[32014,77111],[85243,97129],[97609,98848],[99894,99952],[30755,75568],[63176,66623],[91202,93913],[20984,94741],[48232,96995],[52793,83190],[6119,35859],[57105,61864],[66642,89607],[97670,98204],[49854,98125],[43197,64377],[27163,65001],[47421,71454],[75872,87868],[88675,94315],[54399,77331],[42041,64935],[89631,99119],[89306,99415],[96913,98857],[390,20755],[99117,99376],[10028,38451],[30095,58045],[50281,53285],[51559,94447],[366,36146],[67061,89175],[10740,70835],[97140,98152],[70020,74610],[66480,90264],[14067,16264],[84530,85360],[94734,98608],[67635,73306],[44064,72654],[57398,88709],[15068,63321],[58916,69305],[9343,36092],[36016,75566],[59579,87707],[37937,89891],[5729,97535],[31145,37204],[60716,84473],[29419,83281],[84011,88156],[6390,12916],[16391,18988],[68714,91808],[17677,22029],[88840,97299],[99309,99510],[50923,61675],[8478,18408],[39257,89013],[87635,98386],[98495,99357],[32849,86539],[21040,79842],[64122,80446],[86482,87548],[67985,94506],[57111,69240],[47777,51765],[74897,87691],[81208,83013],[60092,62633],[75507,87142],[35364,95040],[14512,84324],[34631,80206],[33019,94577],[24126,76917],[59215,62043],[75503,89319],[71300,78765],[73443,89462],[85402,89377],[50625,99282],[21468,62033],[42065,74894],[15784,60873],[26407,49415],[55835,79187],[27393,97200],[99948,99988],[81533,93333],[25925,96933],[29605,71899],[72769,81377],[39507,68887],[41170,58375],[99536,99737],[7346,81025],[28721,62960],[44305,52654],[4319,35667],[78797,89773],[42839,43045],[69450,70874],[93851,94671],[40430,77023],[27404,94973],[77039,84449],[15783,75181],[60041,97658],[79394,87602],[42202,77268],[10978,30056],[52602,68744],[37973,56036],[64509,90143],[57940,90647],[8554,22896],[42844,48166],[60386,82263],[61204,88154],[6715,11796],[24629,41690],[8822,56137],[2801,71390],[60762,73891],[55309,90024],[60607,95005],[98922,99016],[73786,89795],[52126,54875],[81551,87267],[92634,93060],[96010,96910],[60129,89087],[67878,95724],[30599,95333],[34473,69335],[37519,46967],[65241,93562],[27369,43067],[81009,85508],[12005,81727],[55499,84972],[40476,81375],[60789,87737],[15142,75203],[13888,22905],[20922,38893],[89955,95935],[30474,84407],[83875,94629],[89423,93681],[83951,97189],[85504,86575],[81998,94836],[47661,60741],[32176,54613],[69874,93448],[86531,93003],[8831,44308],[52014,90572],[62125,66170],[24316,71267],[70190,88122],[27060,90131],[47623,80946],[33150,49900],[63243,65806],[72897,95795],[19989,41906],[33636,52886],[65320,95991],[35565,85096],[22814,63708],[59727,99341],[94381,95152],[93718,93935],[6128,6906],[21298,96766],[26329,79690],[32448,92188],[97184,99377],[59450,67951],[19415,46591],[37557,39462],[99557,99657],[57096,61495],[99492,99772],[62384,88964],[68161,87479],[90430,91186],[46892,88486],[82712,86121],[93018,99532],[42167,50632],[2863,28475],[8953,48333],[50980,82997],[13313,99777],[42246,51296],[32714,74018],[35515,48036],[1034,48275],[90318,98729],[86394,91791],[63554,90258],[76601,87695],[74267,91118],[91764,99813],[30207,84822],[56283,71729],[51372,68264],[8406,31951],[2146,72986],[82133,89741],[48873,86021],[52125,54862],[14805,55483],[2310,21391],[53511,68778],[91198,93547],[93251,95487],[46822,48762],[6793,68794],[67809,70978],[53947,85948],[74163,96468],[20115,69345],[9951,14222],[37083,54381],[2050,51336],[80475,83987],[68302,69146],[37971,41533],[38863,83646],[17821,88448],[18126,67536],[99943,99953],[47508,95433],[33498,33592],[90819,94641],[60188,65550],[36484,38456],[4108,28609],[68825,78448],[87222,99078],[34416,89041],[34420,72334],[62579,71650],[63413,85431],[36188,86957],[30840,47014],[31449,75432],[73201,89440],[11450,35541],[28903,94473],[47200,55224],[84122,88931],[98165,98999],[54153,76164],[95880,95961],[47229,77957],[38184,59252],[88556,96511],[1499,68039],[22127,63264],[95695,97536],[44922,58207],[16714,20554],[30440,69695],[19548,27593],[16760,49941],[19092,62647],[38345,47222],[87316,89290],[47749,52328],[60363,84637],[67377,94385],[24659,28392],[42137,63008],[6899,8319],[89926,96004],[26410,49792],[56727,73546],[81416,97552],[72653,76009],[49216,94816],[33036,84760],[87330,89961],[74867,88575],[56089,98949],[58129,79726],[60798,76198],[18094,58028],[98711,99144],[42873,60740],[44381,96275],[71174,72889],[40145,49465],[99123,99544],[8699,66257],[1741,97716],[81104,86045],[75348,87798],[90015,99462],[47805,93892],[42176,80874],[32732,53752],[87334,88861],[45138,56913],[39008,43780],[89464,98960],[18500,45032],[11974,67182],[91757,98974],[13394,26395],[93463,99894],[52906,61244],[45122,60499],[71567,83285],[34413,94919],[52569,82262],[68506,90432],[26296,61506],[61575,90585],[90588,91506],[91095,91964],[62568,83274],[95740,96231],[47170,94500],[4419,15238],[96579,99932],[26965,27838],[40953,90478],[96919,98488],[99528,99759],[20894,93768],[28287,64501],[97009,99491],[97147,97300],[9823,18758],[87303,98674],[6216,88093],[95510,98422],[34318,41494],[82491,92461],[16060,98879],[90543,92788],[57310,67868],[1178,35299],[73984,93402],[40200,94087],[97157,97751],[5830,67239],[20112,59033],[44411,53894],[5871,94803],[89986,95761],[25484,56703],[77310,87773],[92830,97596],[17557,80509],[50827,98034],[28747,33451],[9173,35942],[84244,91633],[91035,92584],[75063,78079],[93252,96230],[97810,99215],[81321,95119],[54663,57037],[28294,49849],[90678,91528],[1165,12849],[94688,98444],[74623,89281],[16879,55071],[12155,36493],[63636,63740],[61688,96822],[24974,41521],[85209,99990],[56938,83872],[6162,12664],[75314,90017],[48978,52103],[47442,78421],[81079,85920],[31700,55338],[61762,82449],[22328,48520],[93878,97086],[22420,70023],[58033,78535],[92179,96640],[67515,86434],[2782,76977],[88233,90891],[94686,96787],[4667,60796],[8503,78377],[30230,60394],[64016,76117],[19340,75690],[8765,53694],[41419,43911],[93861,95531],[86090,90263],[89804,96481],[96800,98569],[75367,88531],[25784,39793],[56325,86429],[84414,94745],[54769,84763],[68459,81697],[35842,36649],[20014,68669],[47952,52302],[61731,94303],[68955,94399],[47080,97552],[11235,15069],[69276,93064],[88390,96806],[19969,80145],[96396,98940],[18970,46114],[6062,70979],[3724,71409],[45874,86515],[77472,99023],[61578,92235],[91984,99587],[10057,39647],[4901,69179],[74566,91803],[95904,99448],[98252,98351],[3427,65531],[58550,76030],[44558,52804],[63469,84055],[79301,96271],[47478,48735],[46557,86163],[93606,95070],[90809,91958],[56435,56732],[3473,75274],[11752,57433],[2622,53910],[68456,91931],[77712,78220],[83034,91176],[27263,90620],[39487,55670],[81608,95107],[88472,99877],[11972,64534],[13025,67385],[86950,99709],[7607,17234],[8690,84109],[88860,91529],[26859,42245],[66195,73143],[89870,96950],[72971,91016],[63668,91215],[53228,78099],[61616,90386],[54919,88244],[89290,96767],[54330,70960],[61780,71299],[59969,80303],[4161,79233],[11252,29002],[8979,44257],[30528,53519],[87806,94630],[24108,90990],[18071,56721],[15861,54090],[12777,22376],[48377,93501],[47058,71685],[1956,50411],[14378,70563],[12475,35608],[24675,76503],[14815,80436],[85301,85331],[29402,34968],[84273,90368],[28557,65778],[10467,18136],[12606,36073],[70928,89119],[83438,99140],[68579,82651],[8191,34181],[85699,96529],[73290,82899],[13876,30384],[60118,64172],[13965,67632],[53586,73172],[12210,39263],[25563,37886],[85342,92957],[11187,70380],[47707,80721],[19351,72566],[81927,91734],[75542,88961],[394,91066],[86130,86558],[51665,84241],[93646,98753],[83824,88926],[61933,82473],[11288,74050],[4436,36075],[93298,96247],[19521,39724],[22465,94783],[84924,93927],[72262,82878],[33858,50549],[21048,30603],[57655,64806],[67309,88960],[58055,61550],[87430,95002],[14270,15163],[41377,81049],[89480,91059],[64113,86115],[1120,18294],[8444,46571],[90366,94601],[11446,12370],[97803,99332],[51268,63980],[12040,51161],[96387,97822],[39979,95287],[63245,98283],[57761,90924],[96647,98520],[36342,55537],[63724,93330],[78270,82731],[92383,92484],[36382,75310],[15530,62363],[96409,97886],[31103,66411],[91102,92736],[27857,94634],[75087,91299],[97458,99993],[20963,36763],[48317,65865],[51357,74313],[9781,42674],[78586,81959],[48441,85205],[83259,88855],[41642,48371],[27289,54898],[91366,96286],[11914,36228],[63018,93137],[89992,93390],[36762,78099],[13072,49899],[41308,63866],[18676,30433],[5310,81853],[94196,95019],[29453,93597],[32434,79229],[89569,97425],[58751,66512],[42522,64250],[96490,96905],[6267,27097],[60788,91297],[968,38265],[18335,42990],[80773,81396],[70008,78570],[8229,66114],[60053,67672],[18939,19311],[774,35020],[5082,93754],[77,6687],[85532,85600],[92743,94238],[8339,91838],[78903,95625],[27149,73883],[28617,40233],[23169,83129],[60880,65346],[32575,70452],[10898,52573],[89613,90865],[9829,28699],[36634,81793],[89219,99945],[26478,63065],[66709,81109],[81850,91953],[53965,88309],[11399,73407],[11345,89589],[52198,71746],[17302,23169],[91324,91609],[94204,96446],[62635,95185],[40036,89141],[67078,96141],[76103,86885],[56854,81828],[19576,20002],[43231,86918],[21766,43613],[12388,54485],[4770,63583],[85883,87356],[69525,92362],[94926,97398],[29141,49412],[2815,90800],[57293,74224],[52644,54099],[93844,99148],[51090,75742],[11344,47807],[85880,95790],[36770,39104],[83358,99665],[81865,86824],[14430,45845],[81543,85688],[59417,63870],[51889,54131],[59599,66042],[17932,67747],[31297,50195],[11424,42854],[4017,75254],[91243,97921],[36625,95464],[35548,46372],[18293,36637],[55259,79757],[28161,95081],[26846,94403],[19738,22497],[95885,99911],[34085,84506],[8915,25174],[96741,98002],[787,36498],[25954,36149],[34648,41199],[31368,38118],[41271,83412],[1276,32108],[27347,79846],[59995,83347],[66605,84967],[78096,80551],[56257,86406],[18072,21011],[63583,80433],[89953,99099],[53130,61233],[77661,92155],[10466,33153],[65172,96331],[96760,98757],[29572,87665],[38320,95016],[70273,80985],[93906,94959],[44653,65574],[2338,20909],[41339,90894],[19586,21941],[73390,83553],[58386,71643],[13012,61266],[82011,98620],[84451,90909],[75775,76150],[12085,68772],[41117,44173],[95749,99256],[62494,69636],[81516,91323],[95492,96782],[68920,90759],[38700,79596],[85724,94617],[73843,89142],[35732,75566],[7100,28716],[94565,94920],[3475,92938],[19242,20108],[17445,44815],[67646,92318],[56869,73602],[89177,96351],[50027,53590],[19712,96848],[54617,58457],[76936,88939],[90364,97111],[43274,69101],[55187,95729],[18784,37603],[79826,81710],[2313,19642],[98292,98696],[65504,75798],[19967,33890],[62151,98598],[24263,49793],[28420,95960],[30886,61930],[20471,27412],[25492,76497],[64464,76957],[75529,76242],[98750,99312],[67164,72944],[88622,91136],[10731,29670],[46363,98186],[35793,87261],[55351,72647],[44437,97799],[34709,58385],[69731,90360],[14702,99922],[52472,83628],[43815,96570],[94947,99929],[77614,97925],[5703,54934],[71459,91417],[41421,42262],[13453,21142],[97337,98443],[33278,90119],[7211,68161],[59083,87451],[31415,84139],[16804,88752],[33680,58207],[6789,99910],[90190,99398],[94823,96268],[81305,82755],[26987,71271],[82232,95070],[32222,50876],[81634,89433],[20483,39861],[91891,97603],[98961,99769],[68307,83218],[52809,90709],[60055,86394],[75853,92089],[4288,49088],[96200,97465],[42471,46460],[54134,62746],[77921,81537],[52484,74574],[89741,95054],[94854,99685],[79622,85564],[44646,91436],[35146,74766],[99594,99788],[1858,68256],[81173,89116],[50861,99122],[88069,90927],[58025,74802],[97512,99832],[5091,27780],[10927,86808],[40877,89981],[853,90239],[62099,86174],[9900,51610],[76399,98849],[16762,77944],[12041,49175],[35722,64262],[42379,95278],[99437,99970],[96872,96920],[44777,76362],[3243,10456],[12961,27073],[33513,87271],[72483,89131],[41113,84423],[45176,46472],[69943,87826],[44084,86521],[20003,72134],[43423,85099],[77885,98115],[71188,71308],[76772,99101],[13364,36901],[62073,93563],[13068,49097],[47156,78949],[64526,86028],[27428,39506],[34271,95092],[71146,85775],[14628,30016],[80873,81765],[63308,68267],[82883,93417],[41494,79335],[16700,53030],[83092,98082],[78471,92441],[89790,97865],[78880,99297],[72120,85155],[7736,18391],[58130,93917],[25897,85221],[41437,46683],[14261,69032],[39877,84846],[12045,27443],[97548,97574],[64975,75410],[78933,83189],[39401,63382],[52209,81975],[85488,99986],[84849,88011],[83060,83098],[69839,89380],[40492,79400],[92565,99052],[13497,62427],[85791,94740],[65371,79088],[92503,98187],[11817,56497],[82031,95607],[48768,75038],[63113,68037],[91944,94697],[6983,96139],[62263,73701],[20791,76663],[46407,88736],[78313,95224],[26704,40728],[59614,73376],[89717,90913],[14859,46340],[84221,90718],[84871,85704],[21539,94772],[69878,81624],[27776,34144],[16701,60887],[43551,95730],[84320,98330],[16912,76196],[67355,91920],[74813,96936],[74508,76707],[80130,95520],[797,12378],[97707,99579],[72201,92658],[92496,95231],[58610,64935],[47363,99002],[382,56132],[71082,96937],[48198,92677],[24990,81531],[58760,98427],[9932,85186],[11659,69171],[41178,79253],[49835,68502],[78735,95357],[5492,22489],[65235,65543],[81531,98020],[59307,65076],[28415,31725],[29708,83044],[70148,75532],[87725,96649],[61907,95365],[44856,74708],[92953,96541],[24563,73518],[52245,92588],[41823,50302],[27211,89245],[3355,62369],[16454,57285],[68146,94343],[27159,88634],[81849,93098],[9334,18277],[41738,63039],[25375,61724],[81571,92295],[16565,68696],[80755,94260],[80798,84938],[8675,90545],[96832,98004],[25202,41200],[45315,72896],[48603,74579],[7139,30536],[80154,81130],[65760,76574],[31549,45652],[38949,96692],[44000,56984],[59559,63237],[92134,97783],[69708,75209],[68121,77093],[54112,92594],[4656,4986],[98363,99374],[25198,90776],[95059,96135],[3084,97532],[55002,89708],[32368,94329],[69249,96270],[53655,66207],[48922,74769],[36158,52760],[55630,62967],[5378,21763],[5526,57366],[32537,94728],[89102,94648],[91213,96617],[89704,93226],[93771,94931],[54891,78745],[67570,75161],[86651,98163],[26314,84915],[14336,39137],[94581,98271],[12275,45344],[14911,69233],[57635,79062],[68313,70517],[20316,67544],[30551,56437],[48027,77772],[59539,97828],[13456,49952],[92924,93248],[70047,76887],[37310,47586],[8885,55841],[2573,61391],[48903,72021],[4407,17202],[98062,99645],[71091,86749],[78479,83489],[46803,47286],[74459,82489],[67839,78048],[3604,10087],[90520,97627],[80718,85137],[49692,95449],[23020,43156],[42091,86128],[41107,91755],[47710,53105],[82171,85510],[6667,59787],[73928,76500],[3503,29036],[8720,41551],[12967,54443],[39429,67456],[62395,77180],[53054,70590],[29948,94103],[86902,89779],[31212,97478],[8511,79001],[10775,39494],[39364,99405],[60350,77447],[98053,99244],[51672,63587],[85672,90861],[57662,94128],[29026,30168],[19613,37964],[14839,21480],[56405,99287],[57576,58400],[19215,81643],[33753,35946],[90144,90567],[72700,77296],[56141,58757],[51299,92883],[17578,74056],[55487,89200],[38582,60632],[29642,95419],[13799,75873],[92072,97983],[4693,33801],[93013,96461],[77218,77264],[19049,79308],[72597,88562],[68057,88392],[89786,90876],[96933,97398],[81925,83337],[94091,94836],[76456,94933],[25616,47042],[18828,29215],[24545,94707],[59311,75673],[6495,36844],[36905,81334],[5978,68654],[99633,99794],[83653,88702],[85618,92558],[37060,87104],[98864,99764],[26668,95249],[41306,98690],[69243,76385],[6978,22972],[72273,76124],[48597,62474],[78441,94458],[80478,98229],[29382,80467],[6468,9777],[13717,93774],[89562,98416],[32964,41788],[71800,97066],[74127,99265],[61686,94009],[34712,50899],[60444,73776],[85196,88853],[812,89746],[47420,93335],[53367,62897],[98656,99932],[28379,68696],[96273,98451],[29184,37450],[69096,82762],[31967,60602],[47656,99273],[35036,37303],[13124,45730],[91833,96362],[79771,98022],[43005,54779],[7925,35661],[85014,99265],[79161,83287],[81996,91240],[4382,93381],[2079,39452],[15157,33375],[78266,88469],[90747,95632],[37796,44456],[14908,86449],[48857,75048],[83434,88623],[16702,86280],[28478,91100],[86188,91934],[48285,78777],[58538,74260],[60115,98441],[64729,65892],[93449,98983],[68936,91298],[65518,78548],[62102,73085],[525,18208],[6886,43317],[54859,93769],[57364,98995],[47414,71684],[85167,88405],[39117,53267],[78391,94108],[5823,82388],[33229,68475],[14896,89077],[80740,85274],[63261,82787],[43649,70922],[76666,92479],[3334,26614],[94163,95390],[35889,91155],[64253,83838],[71966,72290],[59640,73592],[74144,79039],[19782,99804],[33977,49974],[85190,94178],[85034,96024],[38019,44208],[71080,88049],[27281,84867],[90533,90851],[95945,99845],[48703,98678],[43140,52489],[27636,73507],[1203,62858],[31221,64073],[98072,99347],[83479,99544],[62121,85823],[5482,69249],[86002,86494],[55804,57706],[7845,29884],[76585,93999],[74803,96000],[67358,85481],[82180,83153],[96116,97423],[45740,78013],[34681,72567],[65858,67848],[70982,82828],[61788,88010],[20762,39597],[97168,98053],[34080,54872],[42603,58499],[62967,79344],[53536,74920],[31662,99204],[30738,58091],[74042,94004],[53037,67434],[22915,25305],[57562,96433],[93785,96175],[56199,77550],[25196,44581],[24441,38961],[30614,79422],[93623,99761],[38644,45594],[12468,87011],[64394,94601],[30960,93748],[6598,29276],[32111,54302],[22189,58229],[37469,49343],[48946,92765],[19208,34711],[17947,49401],[79509,96593],[99797,99900],[7092,68965],[92448,97015],[70286,70975],[53069,60724],[58324,67503],[30004,66804],[48864,77974],[57966,63889],[16061,78492],[10599,90950],[50904,99561],[29706,78433],[69256,70456],[87980,88374],[26657,65428],[81314,84121],[60036,94494],[28560,78201],[11664,57488],[99772,99835],[20374,32101],[34363,59340],[47759,83792],[21129,81163],[64789,80553],[15760,58974],[38171,61712],[18768,43947],[79685,93453],[78708,92369],[66838,67279],[44860,83353],[60923,92070],[95212,96600],[50829,84589],[94033,98600],[1999,52499],[90776,93308],[5728,72761],[44120,54233],[36934,61712],[99629,99955],[53048,55783],[86717,95741],[99924,99981],[76369,78544],[81518,84577],[35042,43351],[89390,91075],[24895,34597],[94860,95950],[47063,75210],[87480,92199],[57839,95427],[20865,30606],[26926,33507],[85813,86833],[99837,99845],[75662,99152],[38867,79786],[37014,57159],[10493,44437],[48289,94505],[31369,92058],[78888,84571],[61572,99199],[29058,89503],[62192,87698],[89256,90666],[30070,69223],[46593,93672],[58275,81994],[67001,81842],[27099,66385],[55850,91768],[72078,79417],[83043,99754],[79019,92634],[80348,86940],[66827,95079],[49486,69741],[18097,95327],[42549,95589],[17596,25834],[39268,97203],[68729,98193],[8115,12565],[45558,59430],[30554,44335],[25169,69309],[74401,98517],[83468,87865],[17096,82560],[29440,42818],[80974,91839],[55029,67698],[50285,70570],[87369,92266],[47944,81219],[95773,98397],[11845,96617],[51557,93025],[74581,90193],[2183,12823],[44824,65669],[9716,65200],[40709,84517],[12520,37454],[47562,94564],[6539,9654],[76884,98105],[4385,15119],[35236,48843],[17116,22823],[58078,84609],[52715,64143],[30180,82119],[38190,91358],[85356,97847],[14450,62918],[24088,45083],[6595,76872],[80122,95804],[66485,74844],[40400,82498],[7734,12861],[37059,83081],[23029,65539],[23381,57807],[43212,48863],[63821,82476],[59454,80446],[36222,96670],[82187,87875],[1637,64495],[40445,89795],[58970,98854],[16895,43961],[5898,44081],[66163,67274],[84623,99832],[99515,99962],[75959,81208],[54048,90486],[68708,94762],[86307,99506],[39750,73731],[76569,99212],[9068,51807],[29125,35413],[26503,99992],[93672,99502],[28383,54287],[73258,73390],[37027,78086],[29861,80573],[98348,99507],[29246,75778],[18703,39620],[82860,87234],[87492,87618],[29064,79847],[7666,74276],[80079,99084],[14770,29434],[57056,98165],[45571,80827],[16716,99980],[47796,67625],[6018,18116],[81997,84039],[57093,63417],[31003,61745],[74655,75959],[25052,68000],[87052,97579],[67455,91231],[66075,78923],[18409,68105],[47645,52906],[91005,98476],[82534,96245],[23933,47378],[5411,32091],[64754,90700],[5499,31601],[55327,67569],[51414,76674],[87423,89636],[11014,64524],[38214,91188],[39568,76943],[89026,96612],[42369,58277],[95728,96957],[93625,97350],[6581,58184],[84154,94168],[36988,61242],[46427,81161],[7160,11607],[1065,82251],[72815,83234],[26365,46215],[88968,89703],[12277,57720],[12629,67519],[24777,67640],[81643,96452],[24322,81277],[59294,95292],[34192,66555],[65285,90946],[77635,86041],[88121,88693],[87454,90608],[63008,71639],[70227,84532],[22679,73334],[99627,99890],[72696,86278],[43237,43818],[22068,96640],[38702,68529],[19300,98852],[80785,95850],[1135,50146],[38015,81083],[55575,94847],[38870,64089],[152,15440],[81447,90469],[91777,93631],[72748,81061],[77591,90820],[87424,98889],[32696,72695],[20436,22051],[17687,61571],[63349,80182],[85733,89113],[9165,44565],[82254,90131],[56188,74683],[46386,54931],[47909,80830],[65980,97076],[21449,46660],[81306,86623],[98056,99249],[35132,97568],[75002,75936],[73489,78965],[16873,55509],[67482,92737],[79178,87708],[69093,87497],[89080,92001],[83324,97961],[90314,92419],[4505,22580],[12681,25087],[54727,72970],[82920,85735],[26083,79518],[58255,94917],[28360,61625],[42275,74819],[92065,94617],[3895,96038],[75044,87304],[46239,93309],[8104,24481],[9414,10948],[86352,98131],[29312,69219],[9475,34369],[96052,98505],[19394,82320],[55045,96165],[97782,99312],[58487,73864],[53326,68496],[33708,70221],[40942,66430],[20441,38223],[15699,88308],[21553,99492],[10142,84229],[63948,88856],[86227,88277],[40438,75134],[4609,80725],[32985,57983],[42760,53277],[77612,96720],[1156,18890],[88020,98443],[59620,92101],[68606,84765],[6655,66718],[39129,77890],[60544,98977],[24110,60719],[94101,98635],[42567,90154],[65574,76366],[96517,96801],[92252,94266],[10807,97271],[14619,70445],[74684,84346],[40913,87756],[26328,78882],[16673,60575],[57599,92599],[22186,78307],[31616,32657],[89195,98920],[87388,87915],[23498,50715],[92363,94105],[40127,67416],[25205,60789],[17382,55027],[28029,67932],[39895,45351],[53075,55951],[64188,84170],[60603,65995],[22077,82086],[31745,63048],[16187,51408],[72059,95801],[32071,77997],[13588,25447],[78938,80085],[11258,35430],[68771,75635],[45541,92369],[39829,62358],[93315,93583],[44566,46081],[62385,91074],[17449,35228],[1668,88415],[58113,75924],[35473,59945],[94317,99204],[37404,44312],[65430,70686],[80647,93239],[60046,79906],[68182,83205],[80653,82416],[17226,18540],[53427,53674],[52268,57924],[30869,66180],[36832,85154],[13681,50023],[13189,28676],[2528,34077],[55527,99416],[49762,77284],[69700,99871],[82642,99070],[29556,52811],[31522,57729],[60069,71478],[18228,30990],[56957,91447],[69203,77259],[74766,93003],[7454,75997],[61449,76785],[38768,55042],[55750,81653],[61394,68661],[30501,85740],[93407,99985],[96621,99764],[65073,94656],[33183,48841],[55591,61246],[44851,60370],[60315,90844],[13801,46386],[7673,76726],[40583,98315],[40368,68822],[96599,99564],[74390,76216],[59407,77215],[36578,82160],[61832,62549],[16735,92504],[65990,87102],[2997,61307],[5301,53612],[65732,87885],[74288,94833],[20260,43832],[31881,81968],[37628,62248],[96626,98772],[21245,33695],[19154,67225],[93882,99542],[54965,91992],[68168,72182],[3959,29301],[99138,99228],[85660,95591],[40746,66519],[39669,47358],[50013,63756],[88142,95889],[45847,82134],[35734,70146],[51833,69448],[15606,24908],[51430,83471],[57838,90467],[69486,80366],[12003,79427],[56400,85510],[23284,89754],[89872,92647],[71217,88584],[72481,99387],[28414,74577],[6129,91825],[21802,29750],[11415,96079],[94194,96367],[72872,77782],[69330,95858],[94678,96433],[32038,60388],[8756,64848],[13118,45925],[49952,65863],[87971,88632],[17865,22938],[55542,76633],[28264,44677],[14654,81289],[12761,87121],[61851,92913],[41212,62972],[12498,38396],[99834,99936],[32848,64858],[42258,61876],[97866,98831],[42288,50927],[83371,85011],[57131,79332],[88884,93061],[56017,83923],[4428,82419],[90551,98952],[49877,97974],[87055,93575],[45456,99885],[67601,80237],[246,34552],[5268,67194],[64785,89759],[51952,78489],[53267,89959],[28248,82324],[23088,78246],[22534,83418],[75638,93072],[55101,98097],[9130,64529],[41620,78311],[64289,74990],[45032,79849],[45896,84207],[49181,59201],[87986,94396],[5657,17907],[22809,43034],[49175,63846],[62033,80801],[1913,67459],[72293,97604],[87802,92573],[31429,82697],[90987,92510],[86466,88545],[83267,96681],[30483,94341],[32844,95681],[57797,89849],[78415,78416],[27848,81548],[70531,99194],[55855,62235],[27290,77306],[14106,70496],[22592,72519],[53407,59456],[69439,78349],[18948,32426],[59253,83830],[30427,87952],[14685,80434],[88380,88740],[56590,80200],[68826,93466],[23058,51624],[31645,65074],[43829,70281],[54341,67833],[72854,76724],[70520,86101],[84787,93579],[12699,13866],[90944,98920],[35166,65694],[75196,81422],[50986,56209],[50820,81555],[70189,74085],[14375,95874],[72497,80394],[18587,46665],[59801,65818],[26915,43676],[58880,85679],[30231,72993],[53976,79680],[33098,69697],[33641,90346],[62802,84715],[27557,83329],[16613,92245],[40072,79933],[61825,80402],[79266,80640],[74034,83806],[13056,92095],[65031,78274],[71948,99487],[88843,97882],[90479,91637],[92063,96520],[3973,49009],[23212,44006],[26507,46309],[2118,85645],[39755,54647],[58950,98138],[40081,45280],[74522,84363],[27513,98107],[73047,83210],[58154,80826],[78447,98638],[16784,23311],[31317,38728],[37701,73536],[83035,94408],[67703,72644],[34635,47473],[50522,83365],[4947,49458],[67770,97081],[54295,55909],[51060,84535],[87024,98070],[31468,93699],[65069,70250],[5934,46143],[21231,97751],[48644,97113],[60887,74212],[75090,99809],[31303,36944],[99796,99865],[96357,98792],[16749,96563],[15311,58996],[68984,73678],[28487,97196],[29590,41407],[53344,88562],[58598,63478],[11429,87165],[6347,48681],[83810,94816],[26210,32117],[13783,34732],[47235,97665],[39792,52991],[14795,38089],[42982,56629],[54650,82638],[68735,81879],[22248,87221],[8567,80226],[62815,70457],[32266,81348],[45465,49333],[87191,93260],[67519,72758],[81436,89697],[14235,46755],[90428,93164],[24131,82952],[47913,77684],[9639,92793],[32997,84166],[49404,53500],[51176,60106],[67275,90574],[36577,64661],[4540,13432],[19006,85727],[22359,50771],[70540,84465],[21337,61777],[54889,85440],[59875,99459],[32801,90350],[97335,97535],[59382,82471],[70255,73609],[87382,95615],[11937,24441],[47689,74099],[11568,49643],[50646,64000],[77434,98152],[56648,76469],[69868,70662],[98663,99139],[31656,61915],[70352,83899],[79775,89669],[98998,99000],[50816,92208],[89997,98094],[70355,93607],[61350,87685],[51515,75432],[72657,77115],[84716,97495],[21825,79357],[38776,62582],[65192,90711],[15168,39959],[82765,83457],[63414,90566],[1554,52487],[75774,78802],[46029,49554],[75261,78490],[14700,41081],[30135,46266],[17952,45891],[6814,20868],[73012,92103],[38619,73248],[82264,85743],[20994,46659],[34774,49719],[6143,72233],[68565,79419],[94776,96109],[14023,16223],[49804,76639],[92036,99227],[38712,58303],[80001,82730],[12215,91213],[33701,62183],[38814,67227],[25962,45568],[70912,72853],[94727,99843],[69460,91744],[93497,94214],[70027,81347],[22087,39871],[77958,91682],[40151,51381],[57097,86026],[74227,82514],[955,73952],[20711,54408],[30981,48540],[99867,99896],[49171,77914],[46520,54849],[75417,94836],[31705,42651],[53171,75668],[8378,10777],[28407,63762],[59517,91571],[81411,85737],[87018,93693],[1277,78138],[21492,87814],[49962,98523],[87186,97344],[81143,94056],[48773,76740],[53651,76843],[4601,83968],[51079,82109],[73358,81337],[19254,89956],[88250,93713],[95077,99204],[79618,82619],[42451,70697],[92803,94421],[3946,17901],[10831,51019],[28367,31735],[44215,82086],[13350,67566],[35584,37734],[25281,46826],[147,95069],[66021,77393],[90569,98224],[97264,97318],[12839,70493],[48590,53966],[26104,33610],[61704,70329],[49748,57089],[21887,71719],[12633,71455],[47727,62422],[24968,53906],[50931,63348],[83947,92071],[89947,97872],[76853,77197],[6832,96603],[43808,59116],[6027,78269],[86556,88908],[40245,65909],[58787,93987],[95870,97253],[81804,97817],[75293,91639],[74088,87484],[63263,76939],[30697,97335],[58738,65612],[49706,89241],[85550,89771],[85085,94817],[77076,89133],[21085,31416],[64018,66297],[88021,99405],[26568,84321],[89691,98657],[80228,87505],[83382,97222],[85489,94490],[42846,64536],[65827,86727],[25632,98726],[28567,68383],[29858,78023],[32678,75478],[69919,81778],[26332,49271],[16099,98611],[88421,89542],[75832,95324],[43742,64930],[90558,91124],[21920,59880],[97725,99662],[19605,90922],[13371,15658],[75888,89293],[36249,94915],[65093,65879],[17913,73328],[13087,27758],[11912,43008],[52102,65789],[86226,86506],[79111,94752],[10985,99763],[97622,99068],[27975,47098],[97441,97770],[60758,81268],[57233,90825],[73738,83291],[2260,25187],[55074,95596],[40015,99593],[60194,92867],[2391,22985],[9958,92386],[78152,87847],[20190,63635],[88961,90155],[93401,97365],[25806,66253],[8116,31281],[86681,97690],[74915,87178],[98242,98329],[86484,95119],[79090,79978],[31561,74171],[82811,84107],[49648,79293],[50283,57923],[64219,69071],[270,59904],[8198,89889],[786,90180],[8036,56127],[21575,98761],[99225,99670],[74302,85708],[99073,99632],[54351,64151],[17769,30222],[4863,38168],[83857,92406],[75459,90877],[73999,87592],[69208,88961],[51948,71826],[51786,94657],[54435,60035],[5294,62231],[32143,53776],[62961,64831],[82333,86670],[41563,55982],[7926,78632],[31828,88042],[35537,93537],[66762,87177],[84960,90054],[78840,99770],[13861,51847],[99346,99625],[67064,96917],[28607,63577],[75171,88051],[54333,63332],[11911,56728],[14633,44243],[54655,83248],[38349,62359],[74965,91503],[16375,92070],[23980,44084],[12061,19441],[14424,46910],[48228,96803],[79061,84564],[87618,98438],[52733,98363],[84858,85236],[92713,99082],[6461,32323],[55920,79802],[1606,10792],[25368,89339],[37055,52893],[18326,76486],[87871,94115],[94052,96948],[16342,20451],[23178,83486],[90021,97848],[87243,92262],[38993,53912],[47462,64166],[66587,87443],[67759,97552],[58628,91777],[3804,64263],[50396,84157],[1308,57651],[76288,85620],[95092,99162],[74584,99276],[42868,50958],[95772,96869],[42033,62495],[60334,98317],[38211,88671],[75796,88502],[4329,81022],[77949,78470],[3114,45679],[21119,54497],[19814,63406],[25776,95670],[86,80758],[4659,16649],[16169,58633],[71919,96272],[70428,95888],[82703,94500],[56683,58364],[60134,62944],[91355,96681],[52541,82067],[99638,99647],[68476,82694],[58602,61140],[68543,73212],[64065,88630],[73389,97569],[44183,73485],[67895,80766],[50586,93839],[55402,86014],[6726,93432],[88742,99874],[23780,92941],[50993,54099],[4853,18262],[11101,33095],[92236,94794],[3671,28017],[69983,70715],[59544,66498],[46869,89021],[6790,85929],[64176,76671],[7752,41839],[28672,89218],[99451,99958],[39738,70952],[46180,49218],[13494,40218],[86824,96306],[62925,86955],[80101,99650],[41283,68882],[81019,93769],[33962,40440],[24939,31316],[99621,99638],[6752,59370],[38966,68885],[64555,87284],[98407,99351],[79164,91190],[85213,97011],[45114,85135],[17478,74566],[7643,92253],[61725,80068],[85483,92442],[90053,94947],[13073,70256],[2941,84113],[23137,59729],[16170,57929],[20141,35928],[16807,89647],[80005,94148],[48955,49663],[9731,51240],[24476,35547],[79615,80707],[20331,58074],[37945,70677],[79273,80660],[5640,12912],[91292,98983],[31046,57363],[43086,65097],[83519,89565],[67115,90799],[94870,96724],[39273,90075],[15206,52165],[92505,96223],[69264,88682],[58308,88242],[68143,70388],[86235,92161],[42027,93161],[72418,98361],[29812,77760],[13886,21608],[24879,48214],[57355,81123],[72330,81499],[39482,49673],[94358,96660],[69500,71583],[94873,98217],[51819,93067],[36317,40200],[74798,98568],[43121,57971],[92219,98947],[50814,84837],[39171,39574],[45234,97047],[86953,89764],[18804,35042],[44733,56451],[50143,77497],[78556,94358],[74040,98031],[81495,94710],[25244,66418],[59999,76320],[57641,86454],[65960,74447],[18864,90905],[41531,60853],[41456,47672],[92351,98313],[62351,66556],[72556,81299],[38182,55706],[34817,87544],[39758,73584],[96543,98517],[98476,99575],[70142,97398],[92315,98821],[59229,82518],[54161,61705],[55933,85286],[71950,91502],[24393,77989],[60255,96524],[82586,97595],[88800,95783],[37138,58636],[8560,86765],[55430,90501],[58743,63238],[61552,65124],[62490,89545],[9679,25995],[5207,11225],[48745,60500],[96606,98470],[91465,96654],[52955,59309],[65022,85558],[92594,96482],[64001,73959],[29547,73928],[33447,82195],[51827,58068],[81440,93164],[98020,99016],[66455,89694],[11727,62919],[66347,89197],[11743,61355],[38716,75266],[4494,85159],[84102,89015],[95301,98771],[96483,96696],[62388,89113],[23583,98913],[16617,81216],[62040,89213],[94487,95243],[60899,84663],[45361,53015],[52587,85543],[54177,95228],[67084,75571],[94904,96582],[65456,99625],[47399,70484],[63118,69649],[86664,97498],[63250,98355],[83582,83829],[27,6995],[35213,91102],[26789,71065],[62403,90674],[9142,94515],[93690,99189],[89054,96369],[14739,77752],[59942,71012],[25919,88256],[16683,92596],[93769,98600],[30009,73983],[12043,48577],[42579,54614],[82260,89356],[44553,82110],[79071,82686],[64830,91427],[79891,85938],[24821,62626],[43599,67254],[71610,89982],[19564,52834],[87020,91376],[7846,97866],[74702,76352],[47797,98469],[40528,78496],[7828,66840],[20247,33734],[26249,89894],[63731,92910],[87521,95678],[2271,22660],[16266,37033],[98648,98827],[24574,39317],[4847,73181],[9314,90893],[59916,91704],[73629,95049],[79649,98043],[60954,96063],[71050,79108],[89497,99113],[27473,81202],[42963,81549],[90077,91591],[99288,99889],[746,16914],[54213,77826],[93173,94078],[36148,38548],[74669,87709],[1816,51084],[96844,97480],[92103,98276],[2320,65500],[50388,94947],[98602,99095],[27010,32806],[81986,95476],[78639,90967],[41679,82689],[16910,47330],[36526,44779],[87292,99736],[26100,75617],[59592,73713],[50146,62375],[88272,99726],[84855,87456],[66909,93137],[87242,88389],[68805,75047],[75326,78463],[79361,95292],[54634,73482],[6363,77880],[36383,84721],[35784,65527],[7456,26603],[97060,97776],[87368,90249],[25421,96244],[45145,48728],[14364,56566],[8745,85727],[60936,84959],[26232,47657],[41259,47117],[28701,52959],[10855,65857],[90794,98168],[96685,99734],[56254,77955],[84418,87743],[17477,48439],[72151,99270],[78300,95929],[56870,68403],[6985,32707],[52168,76637],[3696,87461],[16631,49289],[95201,96650],[76690,84322],[90403,99357],[38512,91897],[80629,85550],[59529,74193],[19766,66502],[24272,75956],[71108,92179],[22876,59300],[30160,67295],[99838,99895],[61992,82303],[30263,95496],[78198,87456],[4356,73594],[29968,42065],[73206,93970],[84050,86940],[48777,93109],[87092,89023],[89496,94997],[56819,84129],[19491,96197],[18246,65003],[20106,26142],[70666,88722],[24872,33619],[36005,41392],[22557,56335],[65154,69283],[56378,66285],[59565,80699],[81459,83395],[51599,54269],[70080,88597],[33561,45493],[95307,98227],[62455,84615],[18519,96249],[40319,51425],[52693,52763],[25792,77337],[5099,83087],[47076,57045],[27695,99315],[71781,77147],[74953,79848],[46301,72690],[72914,94519],[50462,66553],[98060,99332],[57351,72744],[25162,71081],[74392,74900],[97608,98030],[64889,72327],[48625,72545],[85666,87880],[15466,27459],[87798,95053],[95545,97825],[15840,42214],[61654,63318],[56311,75518],[8296,41760],[51010,73920],[30158,61003],[78829,89976],[85846,91705],[13299,65895],[88061,97408],[81296,98705],[75406,76037],[19722,43078],[18706,81654],[10689,87353],[75812,96788],[60285,97748],[67872,74826],[67274,77408],[7015,75481],[60034,85928],[43376,46055],[1864,3403],[73063,75425],[55922,64127],[87810,92133],[82006,92658],[1733,44897],[61627,94619],[14445,87646],[59510,65590],[70732,99209],[67370,90208],[27240,93961],[55307,66953],[34660,59870],[21186,65232],[90336,98578],[47627,94658],[76350,91063],[77534,87210],[11734,55483],[34634,57220],[50846,95105],[20456,63189],[99648,99835],[16297,76055],[22370,28081],[29337,55994],[92004,95028],[40173,58436],[37297,80635],[92837,93363],[61494,67681],[21261,24415],[79681,87693],[77666,80431],[19221,61928],[94793,98968],[3321,95761],[86768,90539],[39047,47926],[67097,74735],[12372,68409],[52380,55567],[15086,70784],[26180,47596],[66120,86928],[14145,43860],[96597,99928],[39616,48182],[50348,55793],[30924,58259],[354,11743],[25406,54607],[29539,41614],[6152,22926],[30579,83740],[9834,50480],[61295,80221],[17192,19742],[49213,70186],[42124,53156],[297,80746],[14787,72405],[17088,32630],[85279,91564],[69380,73924],[69055,86480],[35835,61770],[70898,91291],[61853,78834],[50684,76110],[52936,74347],[51969,69368],[2001,88912],[40521,76064],[15570,35748],[52289,77248],[43500,72708],[59549,61296],[44781,65070],[91421,97949],[50610,98236],[73727,86651],[84365,85283],[79157,88386],[38006,46692],[4143,79480],[80023,81780],[15165,23031],[1353,50171],[20253,24675],[48036,83150],[60995,82858],[96369,99922],[82706,85287],[3490,78612],[95131,99918],[8715,16383],[10669,79573],[56385,80105],[52502,70891],[13160,25842],[66649,98596],[81679,92384],[74931,98139],[33410,83219],[36186,64372],[74691,90507],[23737,83734],[60436,92338],[39890,79146],[83690,83732],[51415,61194],[6429,75364],[30203,39344],[60697,95970],[41912,72315],[58456,75953],[5249,59959],[46283,62786],[23325,39852],[77510,93977],[61700,93696],[87985,91967],[30394,35493],[23287,97953],[47114,73377],[10783,35725],[49332,87799],[80016,92888],[74910,99543],[62449,84290],[81033,99533],[47906,68331],[4389,6871],[77705,78396],[44427,55463],[95444,98238],[73820,92188],[1200,49368],[18622,87211],[57875,99206],[38471,78168],[42507,71491],[37196,95266],[93306,97994],[49548,74324],[93137,94898],[76517,94666],[26424,97740],[10344,21528],[35500,41059],[16712,28745],[17052,99958],[38532,38534],[18992,20826],[80722,95666],[85808,86162],[14090,43163],[5441,82765],[69557,83928],[44251,97069],[25623,70815],[29408,84067],[45084,75207],[82570,83179],[9552,55203],[44774,51916],[26702,62279],[68667,69750],[88952,95503],[52362,83406],[10116,44156],[13629,29752],[33352,67051],[26279,99051],[65910,75009],[98365,99257],[25998,99425],[31234,31965],[66496,91824],[37963,58962],[45520,93265],[94825,97413],[18447,22313],[60058,61381],[8839,56959],[66633,91843],[7339,95018],[83533,97253],[3517,26256],[6592,53132],[68732,83067],[69481,79588],[9642,66112],[15823,98285],[27358,94307],[57483,96316],[87893,96618],[54178,64721],[50566,52575],[38192,93375],[4592,37865],[34732,82074],[37826,83607],[71889,86742],[99445,99519],[20557,50893],[89034,99439],[60205,87518],[13410,99883],[35763,56671],[98455,99657],[18814,87644],[33877,99005],[67897,73398],[84183,88734],[92416,95143],[86880,97216],[91927,96463],[58095,82401],[67912,90759],[71036,74221],[57706,76646],[60059,96064],[54465,71493],[83737,93738],[98853,99772],[60683,84064],[55664,63264],[66319,73152],[86154,97896],[83673,99101],[90074,95076],[15278,32092],[65993,91263],[16851,73650],[14416,69269],[79762,85271],[94632,97413],[79429,88305],[92708,95808],[61392,91766],[85606,91123],[89069,98084],[42383,47800],[41822,82384],[36147,38154],[20969,86692],[62271,88717],[91107,94100],[52545,87871],[13481,68899],[73888,88015],[52121,77934],[93236,96465],[76276,90664],[5402,63157],[81779,82361],[87156,89587],[33592,57810],[7327,85085],[27967,59446],[23485,90430],[31633,58878],[18221,91894],[4257,97824],[17587,87719],[99595,99717],[6286,34901],[76373,85361],[54280,95764],[28202,77054],[43806,87171],[88375,98199],[87036,90145],[73320,77570],[3395,81608],[97901,98385],[27278,92445],[23041,45309],[31748,48375],[95137,95668],[82391,90101],[24401,27198],[11266,70484],[7679,14061],[77774,97831],[4126,92215],[14150,69200],[91136,97723],[25110,70661],[14766,48456],[45587,69079],[78187,99242],[90653,91597],[99203,99509],[48470,75197],[90912,97283],[93926,98864],[65234,76893],[5380,43418],[15953,49913],[47509,60821],[59751,81938],[50701,91112],[42350,46062],[96813,97468],[93968,98479],[91323,96452],[45310,86387],[90565,92991],[87037,99888],[31648,35455],[57375,86570],[1833,37087],[69184,93264],[84163,99270],[61334,94231],[68840,98031],[79810,91668],[63540,96618],[15660,35123],[73875,83868],[21637,73710],[5844,21019],[75670,86757],[18206,51994],[51575,71779],[37278,61788],[83211,86296],[65018,84792],[75513,98295],[59973,72920],[19089,50452],[83635,92870],[86541,91572],[5578,91352],[72487,88665],[98930,99019],[27188,83014],[11044,37501],[89498,94362],[7005,40032],[50436,96285],[95982,98607],[89729,98333],[77643,84995],[51181,98900],[40925,71698],[85556,89015],[32705,39659],[5155,13101],[32227,96728],[83162,97042],[82759,88402],[13063,37209],[36948,48272],[61745,95369],[83927,88916],[61710,77237],[83463,96692],[84269,99279],[52798,70985],[68507,97055],[13304,84149],[46548,94442],[80994,90049],[93115,96072],[2419,7514],[3491,22265],[75150,79690],[72482,76172],[61626,73608],[20836,70788],[7149,12879],[42442,81016],[18433,57747],[70585,72908],[58747,86928],[3461,93665],[83973,87145],[46474,48979],[61873,92456],[70641,74162],[67276,78814],[8358,44090],[15342,25265],[82733,88186],[67168,85372],[48051,70248],[97673,98835],[43433,97743],[14642,23457],[28417,93953],[74108,85786],[34394,38200],[76321,84111],[59951,66849],[54667,78264],[66852,94009],[24596,77134],[95099,96121],[14999,83162],[4617,66470],[14013,24367],[6676,52311],[80580,91820],[57707,62783],[23010,75156],[95856,97804],[82205,92178],[86273,86687],[33466,98847],[3750,82025],[80764,80890],[11707,60996],[31044,92311],[29999,91734],[71577,73284],[29015,32310],[77859,93982],[86046,95527],[55081,93482],[75597,79443],[81958,82013],[27258,84215],[46048,96729],[62738,74920],[18652,99767],[13879,38721],[73483,78656],[9375,37515],[63963,88278],[89151,89614],[62523,67704],[67767,93302],[93076,96225],[65644,71854],[4549,56990],[346,50681],[18764,92994],[28766,50855],[50407,52468],[6326,17171],[37382,96095],[10776,82523],[16173,21460],[60178,99547],[5239,21000],[26195,54353],[6955,69199],[30804,60177],[94440,95028],[58995,74125],[54189,80566],[34182,89802],[60925,99467],[20745,81508],[29958,95249],[70668,90319],[98936,99521],[82656,86573],[59436,83980],[55567,95439],[12365,26849],[79907,92886],[93202,97943],[87955,98029],[50638,68517],[83462,93535],[17405,29982],[969,86421],[9445,93974],[99918,99951],[7447,28831],[2081,30083],[561,46751],[54041,74718],[48043,51510],[35873,80134],[96126,98398],[86423,91817],[70331,76464],[31957,82175],[25054,79048],[82414,97171],[95930,97668],[27628,42825],[14194,22727],[27679,78421],[58823,72818],[67349,77113],[21115,31136],[98651,98971],[73717,90592],[68726,94150],[42838,51075],[35769,60486],[35522,55147],[87718,92613],[77382,93155],[76808,93644],[30487,90721],[89632,92401],[65048,68612],[73599,94406],[42870,72851],[1384,3653],[51943,85848],[13269,46354],[21078,23366],[38521,59362],[61977,65091],[95317,97163],[97730,98043],[2022,47819],[69505,79834],[52667,61061],[91928,96495],[93648,95344],[75949,92068],[10707,81801],[99152,99175],[91422,99980],[67543,98770],[99612,99614],[24395,92274],[51240,83263],[61558,79985],[88483,93249],[52536,94636],[47884,57542],[93839,99205],[88796,96158],[52711,83240],[26892,72317],[6648,71549],[36258,80968],[63659,75133],[33981,52791],[25468,33469],[84013,95646],[40970,61547],[21091,42296],[25228,37280],[13689,94011],[40834,56017],[76966,79537],[91310,99805],[34723,69956],[4256,53852],[79315,90768],[58133,59612],[90668,93163],[13470,76958],[52881,89659],[43429,75757],[96235,96886],[94738,99311],[50532,86027],[20361,28798],[39657,87472],[22171,65542],[61507,72415],[40334,46394],[2745,6840],[58505,68523],[73882,91254],[41398,83648],[74697,84227],[29183,74561],[14175,71561],[12982,52806],[91220,92788],[83654,93648],[96884,99024],[73847,74517],[52248,84969],[75777,80436],[12748,17781],[62724,79045],[27718,95163],[83173,92758],[52755,73837],[53725,80650],[61079,67583],[17941,93399],[95860,96811],[13678,36481],[85551,92371],[57130,92518],[96997,99704],[5336,75908],[29886,77146],[33605,39923],[52310,69555],[52563,68520],[79199,95679],[88120,91999],[27707,79066],[92941,97889],[68956,85839],[28411,77428],[12751,23262],[18718,83597],[52276,93292],[36825,96560],[94903,97131],[98075,98182],[93262,98502],[13053,69269],[93114,97214],[79708,84503],[20653,95217],[74949,78505],[42,18068],[21067,56790],[97111,97329],[47856,97223],[22522,22962],[8308,25512],[44363,74042],[67304,96407],[33341,42608],[6792,74129],[92630,99978],[17159,45284],[82931,94530],[7745,88123],[75319,95207],[95338,98364],[32555,86652],[36389,51672],[96234,96423],[45026,60711],[75586,83583],[97914,98561],[53686,81833],[4779,29545],[23293,23382],[69641,83615],[2826,53433],[4870,70459],[65484,72428],[76371,97197],[53301,58430],[50812,65384],[78191,97812],[85521,94182],[19797,81138],[64860,80865],[74429,84979],[68999,76677],[59240,84579],[91386,93966],[80146,82943],[5770,14818],[98997,99845],[70578,98959],[5993,20403],[56031,95726],[70792,96431],[65266,98414],[71550,86232],[84003,93240],[23314,77950],[61221,97147],[88056,88421],[12077,56598],[18781,51192],[58815,63345],[18495,88373],[26400,52317],[66431,76945],[53265,66093],[8636,69751],[96114,99044],[19901,44654],[78429,87028],[82453,87796],[35888,50492],[15212,22815],[45931,59971],[64282,97088],[29599,94075],[8397,25724],[6972,86170],[52016,78464],[54883,57395],[30798,55335],[83361,99646],[56368,86632],[2582,45952],[56816,78836],[29949,49156],[21678,58529],[26269,57782],[61569,81418],[45877,49222],[21520,67611],[94307,99660],[70673,88708],[68043,86469],[35578,94815],[23085,71949],[88894,93211],[84861,87368],[22434,33242],[49965,77626],[63346,91541],[28761,67061],[98254,99637],[18810,64154],[38942,91964],[41688,96297],[83544,83806],[8667,63093],[14829,14998],[68668,70925],[81554,92144],[79206,90732],[96613,98132],[70921,75915],[99236,99430],[46493,74670],[98341,98549],[87467,98876],[59249,72845],[65391,84742],[49159,58794],[45189,86066],[3337,87031],[16193,88398],[48235,67475],[57117,80926],[74851,93071],[97563,98717],[75814,76934],[74506,93399],[14768,46561],[10997,12299],[14169,69744],[22424,79146],[25798,70526],[5222,9265],[76900,81733],[5401,11368],[29067,39542],[42667,79651],[27990,86703],[73595,82851],[82532,95004],[48205,85361],[73513,99500],[86777,96175],[51313,51579],[5960,81669],[57950,65392],[78113,79432],[64033,97788],[23596,39032],[19858,97932],[18693,89293],[24435,37809],[85227,88697],[6290,50949],[24746,25882],[41665,44328],[34869,51522],[17641,25771],[90248,93059],[91979,93452],[9613,34761],[70716,99231],[58793,99680],[74604,89331],[87792,96140],[15171,59656],[20452,82037],[3407,31992],[15597,88500],[18726,84854],[55475,96924],[23264,72882],[40176,42267],[13652,45637],[60543,67245],[24259,99044],[55013,90923],[33317,48660],[78261,80211],[94230,98944],[601,80374],[64905,74705],[75653,96632],[11180,80234],[4171,31543],[63072,75725],[19418,77351],[87141,90219],[67089,76884],[12557,70830],[68398,83168],[34561,63522],[80933,83060],[92157,99264],[81599,95178],[64221,83506],[29621,40765],[7349,56728],[43733,73297],[91128,97227],[96304,98326],[23239,84325],[83112,90435],[57317,82493],[69809,84777],[62628,76769],[18494,61613],[85401,95489],[74940,75808],[76044,77733],[56421,64643],[78851,97841],[43359,79142],[55049,76519],[19391,41111],[19867,60998],[89692,96248],[79260,92247],[61043,64261],[70263,97921],[35138,41041],[93500,99708],[37732,85885],[26661,66201],[50294,72335],[50431,74351],[96282,99284],[70053,85642],[41856,96637],[34916,90509],[39352,46524],[94020,97182],[37314,80313],[5620,48861],[43945,53293],[61390,73563],[8189,34092],[66886,83604],[71431,71493],[1581,95184],[60283,78689],[89337,93691],[40122,57658],[68135,84338],[39953,84853],[45001,89250],[33194,39465],[7633,33454],[7521,16050],[70929,80687],[78202,90027],[92827,97941],[46265,51152],[36811,43727],[23631,38415],[74209,90195],[7408,92079],[20539,25674],[36965,85613],[26023,40850],[3651,87791],[90339,93180],[69086,87131],[19502,74186],[80385,93919],[58808,61601],[54249,86877],[43613,61386],[54878,81076],[80309,95919],[9526,43458],[26973,74693],[75012,80311],[41433,81833],[15947,69628],[4272,13072],[33326,73698],[95490,98472],[76273,77289],[62187,73220],[16606,73383],[5516,64451],[10082,83353],[96609,99252],[6813,74135],[80351,90407],[5685,21788],[70890,76750],[2023,91941],[53573,77400],[26113,65838],[82515,87453],[88990,92797],[64385,82030],[40114,96293],[49174,91643],[49467,55050],[95219,98511],[20101,46719],[249,57817],[68782,88109],[64378,88742],[52816,55866],[65452,91724],[42110,75149],[1128,19492],[94701,99958],[44597,94303],[79635,84430],[65263,73571],[52959,53012],[89332,89478],[63698,65244],[30920,90745],[64668,87744],[90254,95734],[86109,98367],[1105,3562],[70268,76130],[72184,82374],[27657,29769],[77371,96614],[71349,85998],[6191,20735],[12497,38058],[91278,95645],[45039,74249],[66460,90409],[72166,92394],[88987,95022],[30777,69728],[59789,63570],[73613,81625],[95068,98668],[49516,88142],[15590,60350],[55065,79122],[35980,79103],[69183,84783],[41588,53579],[93756,94248],[27068,39537],[1619,5188],[81366,86053],[68944,82508],[87773,97905],[36083,41714],[5329,41365],[35716,79971],[17725,21752],[57844,96376],[84447,89104],[6083,54086],[31265,86490],[35089,88218],[426,60634],[38005,42231],[8666,84988],[64587,81282],[49941,64067],[96045,98664],[5219,45230],[33998,52093],[82669,90973],[25939,65453],[91471,96414],[2293,23002],[88780,94613],[5949,86269],[25028,70795],[89932,95363],[61746,78141],[67557,92520],[82559,98974],[20695,40552],[94546,96537],[94300,97211],[20833,43249],[97938,98449],[42013,81138],[47249,48210],[66694,88989],[19954,87129],[93382,94066],[38580,51947],[61175,68254],[1773,55318],[67965,67973],[64974,77812],[80519,98146],[14198,66838],[63436,72168],[59977,61663],[20084,42487],[88939,99011],[87188,94971],[28935,47436],[51438,55166],[1186,97758],[74167,74821],[51939,66971],[3277,17885],[25077,72752],[31278,66627],[16757,23758],[92188,94149],[71810,92735],[52048,72721],[66251,93837],[14705,83541],[73954,92970],[15864,78013],[20414,68732],[90450,98578],[6629,41342],[81558,83242],[11206,72814],[60126,80365],[80731,92133],[10491,84582],[58302,94542],[14342,80656],[12688,83575],[61468,70628],[30353,69330],[3615,25251],[3506,34521],[2876,14533],[11297,87686],[75400,76475],[34522,89030],[46379,92792],[69644,70588],[37452,99671],[42201,89190],[68772,74652],[45493,49851],[81404,95139],[31414,74738],[88585,95866],[87547,90353],[71339,90655],[12665,21163],[43383,74524],[18662,35527],[26338,79089],[63977,84050],[17949,59684],[38104,88036],[38509,73068],[98419,99081],[81096,96875],[85281,86139],[78196,86973],[18453,83938],[11565,32167],[11247,21442],[37290,78173],[86954,91158],[40912,85015],[62504,90571],[13050,93753],[88680,93859],[60139,98665],[13361,15592],[76474,91331],[42988,86373],[27288,57833],[13972,83889],[91472,98339],[25396,32292],[57318,58947],[19026,75194],[8499,36297],[35168,89309],[44705,92063],[26266,68174],[36833,61401],[72225,72540],[6172,70295],[78958,95425],[38454,75858],[21467,95382],[81391,94726],[40093,92626],[10624,66674],[93015,95902],[61643,81555],[10884,79236],[10193,48325],[38703,61824],[50365,69901],[26732,95400],[90613,94212],[41205,98255],[61458,83358],[84370,92460],[98898,99896],[52319,62409],[43506,79164],[2744,99348],[89429,90270],[2722,17450],[42949,69532],[87479,93229],[91157,93192],[47799,48846],[35532,79597],[43643,97040],[25517,96103],[67178,85452],[61309,82505],[83258,91137],[34802,47816],[73467,80970],[23165,72881],[58930,91736],[1597,36721],[52878,80288],[60587,99310],[62034,93458],[75576,92419],[45308,60589],[28238,36955],[24353,32849],[29795,86886],[54524,94100],[76887,87205],[33400,76512],[38807,81214],[52972,78857],[54072,86995],[95472,97630],[32432,49382],[7335,39790],[20496,21321],[96853,97735],[55757,81563],[4890,37368],[51959,99966],[60075,62819],[46404,60047],[47460,96440],[70543,94298],[6953,35368],[35991,76880],[11260,87448],[23803,26206],[16877,44211],[41347,92328],[15354,57314],[71990,98485],[38433,58181],[89789,92654],[2687,64290],[88921,97636],[84280,89440],[23066,47661],[78716,82641],[19344,41201],[96408,98469],[46931,79003],[39142,88305],[36931,87981],[2319,75222],[4304,94444],[98209,98489],[38106,78076],[81739,90753],[2229,31485],[37562,61957],[72467,78300],[60513,82743],[13387,65609],[50490,52632],[69415,93946],[44440,58312],[73766,96375],[5226,7140],[91939,94628],[61550,93526],[71168,93306],[71001,95112],[49559,77175],[22626,63528],[40979,60593],[1848,26751],[72650,97115],[86169,90273],[68029,79768],[13249,75223],[37532,67878],[66582,66827],[87343,91167],[98696,99595],[57699,60264],[73451,81602],[63721,71670],[57512,77540],[89889,99956],[29102,96371],[50270,59844],[8773,55526],[83837,90162],[44035,73534],[88924,91517],[42769,95020],[10281,92171],[10798,11883],[42058,49799],[38033,70535],[71689,78664],[77543,86853],[92589,94826],[26079,42927],[74610,90042],[97313,98532],[93199,98188],[1430,27471],[64230,80081],[60431,99492],[59647,63671],[16972,92122],[14444,15951],[13243,47041],[83021,89937],[31201,80150],[69278,81493],[87469,91199],[15577,85225],[80077,91024],[30459,42459],[11208,59823],[4807,37976],[6093,91446],[76431,87145],[47695,59960],[93325,93913],[44325,46978],[72392,88983],[95528,97842],[27061,82579],[43107,84348],[91232,95720],[35329,94267],[85116,88511],[16444,98567],[24951,82594],[70063,77528],[45652,51381],[73863,77254],[65376,94173],[6996,15103],[93737,95188],[59003,76449],[58202,79852],[77412,90750],[3416,36296],[3889,81559],[62746,68031],[33292,44133],[38754,45485],[94659,98344],[88550,88725],[43892,47702],[54208,65570],[34726,91968],[21356,51164],[74335,92231],[54,56165],[1026,43706],[15090,77982],[83481,95766],[15766,95122],[69132,76641],[88804,99752],[88150,96930],[66992,90784],[62478,95973],[90624,99374],[67783,98376],[16989,64867],[53146,91442],[41972,51737],[74843,93460],[54911,61727],[11401,30485],[20397,20493],[7137,89688],[63581,94497],[97385,99610],[47385,88667],[5593,87468],[95785,97919],[84444,85280],[52567,58076],[12042,19229],[37313,90840],[97570,98195],[17323,82743],[48202,94717],[36767,37138],[57810,85729],[74133,86792],[13604,64051],[41634,91719],[67141,77267],[43980,99642],[63448,80310],[51436,95312],[37291,97637],[74031,92947],[18295,62296],[29791,63901],[83349,99975],[50963,56661],[57141,92398],[8865,64685],[19053,81043],[52595,74930],[20013,77224],[83470,85289],[33616,86928],[84765,99168],[59655,70390],[10349,37447],[12104,39615],[79612,85585],[24679,32773],[60471,89514],[74324,88485],[8766,56430],[20167,81178],[27465,54577],[11818,85766],[34446,38477],[26581,85810],[88260,89245],[79000,88351],[74261,85674],[13541,26540],[48400,69777],[28054,98537],[49067,76463],[1164,88579],[57841,77246],[14723,69809],[74170,95854],[8119,82358],[63115,71612],[10580,38930],[86060,86957],[33404,71036],[40163,53409],[44906,52655],[51803,62328],[85409,94145],[44302,50857],[56081,97976],[26989,80200],[43276,70832],[67799,74754],[86591,98716],[45089,73515],[27478,61859],[83974,93648],[66079,80675],[46000,61775],[12444,75689],[1479,81541],[42825,67853],[48843,54497],[56839,59776],[16436,70093],[23979,87142],[68882,92853],[17948,26560],[34727,79745],[83469,90936],[46488,53769],[85399,85958],[71133,84385],[57659,61337],[11674,71773],[63318,90888],[46791,58219],[63322,70224],[32686,99886],[99773,99779],[13642,75817],[24689,44832],[99874,99989],[80545,81212],[40452,45511],[43361,99629],[77654,98968],[66284,86366],[50622,72353],[71425,83068],[42867,87555],[39092,93838],[22815,35336],[26467,36055],[66978,86127],[3161,56435],[26304,58887],[87232,99413],[34164,76039],[21325,66507],[49753,73764],[60408,74837],[35185,35861],[87952,99010],[36650,65677],[14952,96852],[2396,10869],[91318,98441],[27107,70930],[88445,89933],[10047,59057],[57276,85118],[90023,94790],[12774,71328],[73814,95199],[5999,74299],[88692,95603],[2044,28537],[56972,71113],[83022,87918],[39988,58496],[35466,70226],[29634,84759],[81278,83951],[24145,70342],[92587,99611],[48847,86593],[33315,34008],[17108,72901],[88754,92347],[1589,38086],[6684,84543],[20011,90322],[19499,63005],[27007,96412],[26035,40340],[7212,67181],[83666,99614],[81454,95028],[27222,39156],[69028,81015],[92478,95106],[30602,71193],[49673,96672],[85275,98823],[32462,77718],[8113,22267],[28698,38980],[13335,59593],[80133,87044],[32000,79107],[17801,30910],[77853,89702],[89534,95062],[63062,75069],[73205,87811],[97534,98555],[83504,96020],[44973,95594],[12660,93905],[35414,40813],[74689,81817],[60668,84609],[10781,85677],[73899,80287],[60638,81352],[96055,98247],[67017,97240],[86770,88385],[47857,74700],[15654,40593],[15208,71456],[110,66483],[70667,90383],[96365,98933],[2046,77761],[67033,69490],[16068,56044],[95216,98206],[87781,94279],[67692,98776],[80963,85357],[87023,93730],[21497,95915],[57623,99445],[49188,92254],[7570,84283],[45240,67622],[85067,96040],[85861,89341],[86638,87117],[14055,57428],[74989,88835],[97717,99702],[43317,53170],[46583,76143],[2778,5200],[14389,20839],[71144,79745],[84703,85334],[12787,74330],[69105,91160],[26038,92073],[22947,41573],[60804,83963],[76894,77965],[77386,78836],[92305,97586],[15356,48573],[35404,88624],[61269,85110],[226,92941],[47252,97523],[94978,95088],[60406,68903],[78980,91842],[90164,94748],[43657,72608],[9151,99992],[95441,95465],[51052,63322],[89238,91344],[18599,97797],[31842,50997],[28235,29523],[46829,94812],[37568,38708],[20045,73292],[78518,86890],[21142,66758],[71438,93837],[82320,95585],[46103,75787],[49463,53113],[89637,93895],[99397,99534],[45543,55345],[42247,73289],[27893,84644],[43480,49747],[38082,43124],[1196,38035],[69978,83736],[56133,72306],[92883,98743],[96321,96384],[83798,87985],[41694,45457],[7084,70054],[55604,85067],[2777,24137],[73124,78952],[23670,30309],[67688,83584],[94644,95929],[41206,45424],[76424,92865],[33244,54764],[46643,61370],[60170,84982],[97333,97536],[85908,94667],[91830,93177],[79547,98453],[67204,79246],[24220,29101],[1905,87769],[38594,57721],[84695,93598],[50443,71987],[77429,86458],[24328,60755],[57361,75844],[94626,99425],[37713,64960],[39315,67635],[74624,84106],[69279,94540],[41096,76995],[32017,82735],[52661,84640],[54702,65451],[92216,99234],[79881,83260],[31359,95859],[77146,90304],[12430,62292],[45332,65447],[43908,75136],[17491,70848],[42492,46966],[33204,57261],[62510,97462],[32142,81883],[386,47791],[83157,84964],[70152,70347],[44952,58887],[35508,94371],[68799,94511],[81591,97266],[96274,97312],[4681,94365],[43045,63339],[60832,76866],[66406,67252],[81035,93354],[36454,48705],[87100,96435],[10320,70337],[97841,99172],[45339,47919],[12417,19146],[30366,81088],[34263,52230],[23276,49506],[97437,99370],[54683,66659],[78575,93496],[60885,99325],[46655,65243],[52101,92278],[83739,88536],[41734,64242],[53294,72705],[38083,41480],[3133,19655],[96474,97011],[9123,35505],[87723,97621],[98754,99573],[27335,33532],[17708,19725],[97675,99276],[94766,99884],[70986,72032],[64300,65059],[98946,99211],[52,95286],[45009,50668],[96996,98076],[35984,85507],[59994,81280],[75076,91391],[17318,34838],[96098,99961],[87591,87597],[89652,92790],[65494,78979],[981,16446],[90173,95471],[19603,85764],[65984,94352],[14216,89496],[99641,99696],[94145,97120],[83100,92539],[79897,99198],[23848,35003],[70369,85577],[88404,96447],[33320,58929],[30249,81618],[728,80276],[5143,66078],[38649,71191],[10003,79630],[76984,85738],[88479,96444],[2245,28313],[87961,94463],[7024,76209],[42228,43512],[65782,96057],[41273,56866],[36493,94563],[50989,80277],[88537,88568],[11119,34785],[78179,88905],[42556,86643],[34700,91648],[4048,26387],[73946,75221],[74721,77135],[61033,99782],[87229,93284],[23786,95228],[10074,82963],[65850,96764],[72308,82690],[7020,25600],[91099,97407],[82313,82819],[85715,95501],[86429,99043],[8109,48989],[97571,97676],[31799,61486],[13142,15920],[42948,94461],[6866,18478],[79832,92184],[24437,86304],[57129,94163],[70613,89473],[42208,97739],[12147,22435],[4848,32152],[45914,68773],[85909,93479],[6509,26640],[11639,42758],[43201,92234],[31805,84961],[56993,85715],[34563,75384],[96827,98767],[10062,79832],[50817,78063],[22737,77410],[27354,42787],[5367,53413],[81160,99198],[1990,40513],[89418,91619],[83618,95789],[23434,94561],[86431,86990],[98313,98656],[75470,77171],[88506,96874],[68300,89140],[10445,88164],[54470,86855],[88038,88942],[65359,91097],[620,45362],[38581,68055],[41005,63653],[20068,78453],[43756,86331],[92573,98857],[48820,99635],[95093,97733],[98189,98702],[94621,97770],[49689,85958],[2388,59651],[92310,92573],[98908,99785],[16278,68264],[55516,90004],[81326,94323],[33427,89256],[18096,70022],[90069,90321],[48700,74854],[86236,90381],[96081,97781],[5156,45032],[10470,38521],[53948,57659],[14922,78528],[43481,75847],[9750,85388],[53695,72919],[284,21841],[65327,97951],[2862,42694],[15315,61775],[62313,73734],[17581,69906],[66348,99420],[48471,84491],[95450,99846],[90252,99872],[49801,62322],[60250,94332],[83171,99667],[79437,94340],[7914,33961],[1689,70616],[95343,96198],[25010,37361],[9487,81782],[91657,93114],[66861,85367],[23210,39694],[74544,96339],[24953,32412],[28601,44177],[22159,93012],[91535,96913],[1216,85325],[11544,53122],[11921,93416],[56358,86210],[60628,78266],[14146,96548],[98965,99489],[25910,69795],[24978,34097],[44022,49117],[69382,84163],[1385,34696],[41992,97189],[31058,86566],[62119,70342],[65669,68655],[64613,73140],[62743,78328],[13909,81353],[24644,96028],[49650,56402],[5351,48226],[28250,30078],[65584,90032],[58858,63254],[62612,70502],[52314,64739],[58913,68274],[89259,91516],[11963,36508],[42602,76909],[54645,87349],[21463,54702],[69206,89294],[38457,90577],[62993,69771],[50455,60698],[10843,69810],[73154,75022],[51149,79725],[74639,89340],[34496,68491],[4627,51038],[73903,80610],[38834,67296],[53779,99310],[39832,40672],[61213,80587],[80288,85475],[53889,87525],[61890,84077],[57760,78356],[29303,57117],[60907,93705],[47099,55143],[25699,27663],[91199,97074],[48904,58212],[2335,86221],[15936,26215],[66188,71116],[6171,26200],[1947,24264],[76557,86836],[70750,74079],[53398,76862],[16458,85229],[92536,95028],[53392,92957],[32207,63928],[94682,99912],[30456,75067],[1747,92885],[38455,98886],[65799,88852],[27859,68481],[78262,96350],[94725,96566],[76742,88889],[32564,59480],[67873,87257],[9558,79034],[58213,66303],[61767,82022],[20009,76498],[43412,53990],[93799,97531],[84326,87838],[19190,74828],[68024,72896],[86222,97968],[60259,90089],[71429,88655],[18649,28225],[7350,84181],[98561,99773],[593,4393],[83354,88499],[83940,85983],[56156,68634],[91964,92688],[32290,81813],[81297,82043],[19652,92937],[50503,52125],[95150,97268],[81308,88217],[73809,99407],[42401,44895],[37942,57024],[95883,97462],[86461,99943],[48069,79498],[23552,48006],[10883,71720],[77845,95159],[34283,98593],[25438,35888],[83741,96170],[81357,94540],[7818,15108],[13380,41810],[38058,41455],[8606,31423],[5879,34156],[31402,47097],[93042,95513],[68848,70160],[20278,75020],[30638,72315],[19609,71402],[16153,82010],[40336,78330],[70204,85697],[77907,78866],[84115,91486],[60368,89897],[84100,98229],[75559,87363],[56605,94390],[77155,92509],[15242,29215],[78796,95959],[92534,98068],[94222,97707],[6038,35198],[81630,86261],[54720,64196],[69228,98893],[22743,78138],[26292,61313],[56011,56183],[91489,94823],[97114,98516],[45080,74079],[38689,63802],[49792,63771],[97830,98792],[25700,80738],[18666,69183],[17457,53026],[65771,77005],[98506,98753],[1887,20799],[54982,58631],[13921,31558],[22447,90882],[5501,10022],[29650,81090],[64948,66392],[26531,30156],[94152,95712],[50499,69832],[27559,52849],[85903,88788],[17667,41021],[23496,28617],[83464,86190],[47580,58112],[31790,64342],[78053,87880],[91261,95099],[3464,99612],[64617,94893],[54832,70818],[40136,84031],[97767,98305],[98655,99211],[49290,77433],[28016,92346],[85197,85652],[10639,67266],[49407,50622],[89737,95635],[76066,86722],[27847,65055],[40380,85808],[8154,45246],[85183,86493],[30499,92261],[40032,43164],[58724,78324],[56153,93024],[26465,43782],[76268,90962],[69820,92227],[64592,89931],[43802,93400],[84380,86311],[90519,90655],[93087,99595],[60262,66008],[36062,92509],[50475,95146],[30477,72577],[90332,94085],[77900,96344],[41765,49880],[72306,73324],[21182,30779],[93161,93854],[20128,41127],[55889,97648],[25078,31360],[15740,27575],[93647,98312],[40723,83278],[6677,11127],[1505,64649],[11352,32745],[12678,91997],[1496,40165],[92187,99997],[64932,99714],[90955,92072],[3244,86871],[3885,4119],[9851,10743],[37437,40791],[13270,68904],[27350,77736],[74715,80456],[23188,35577],[9510,91240],[28879,73176],[31298,63108],[72215,89546],[20048,22011],[7266,17606],[1404,62445],[42742,45017],[78177,85442],[9767,47857],[81720,96654],[44542,62021],[25861,66998],[84213,93174],[52411,65999],[49362,79258],[12789,61552],[53016,53831],[28470,34204],[77980,82308],[17113,84306],[61247,85707],[30084,53526],[28494,82007],[19692,99667],[76099,84379],[77789,83095],[40971,87163],[5169,33767],[30301,33922],[25306,58565],[60524,82018],[13474,66254],[45844,65549],[9950,69286],[11515,34542],[49622,87594],[89883,96057],[58321,70999],[95712,98594],[58083,74385],[49268,61142],[2860,5468],[7538,61506],[32991,56318],[6352,18057],[42279,68404],[74189,78817],[18181,80662],[88460,93496],[35536,63138],[68865,89092],[91561,99327],[3626,55254],[65862,90035],[7050,18617],[92193,98310],[47210,71995],[1477,79388],[73751,85166],[40996,41125],[26551,31212],[9582,45026],[72730,87607],[65999,82901],[50352,75443],[77433,87685],[46671,47761],[7332,15012],[15399,69040],[83861,99010],[1756,98404],[57656,94823],[95644,99755],[15641,15881],[64636,84193],[12524,48409],[37959,46920],[75792,97464],[47131,53927],[43637,85452],[91679,94713],[21652,81917],[64458,77151],[13986,62253],[51600,62227],[34462,93690],[66377,81966],[87420,87426],[35041,96228],[17264,43273],[16496,65146],[29644,73444],[41375,64927],[73586,82142],[22480,30902],[59528,95228],[89594,97345],[84073,92039],[68349,89196],[927,20789],[79337,95962],[43038,54568],[25105,49623],[79371,89936],[88963,96210],[96531,98764],[78705,90573],[82882,83868],[73388,95631],[58439,92615],[22627,83306],[82957,92070],[58512,95798],[50078,74448],[88407,94177],[57048,78451],[85598,91383],[1712,15443],[72893,89520],[20508,85204],[1831,47231],[48507,65455],[2684,91652],[90781,91326],[61331,80123],[46179,75874],[24304,87571],[70022,82872],[91356,95169],[17863,92938],[40816,78135],[72485,94773],[86178,93445],[61591,80654],[88760,99288],[81233,96780],[15824,16702],[61096,61449],[49750,84908],[1633,9807],[13713,85772],[61450,62077],[17033,76861],[90244,92872],[94721,94863],[66881,70601],[94820,98856],[4107,11506],[84507,96202],[35463,60386],[8064,21368],[10089,91816],[60487,88742],[12126,76034],[26835,91188],[75754,87109],[1878,40975],[75483,75837],[93481,98749],[48113,64248],[81086,87642],[92225,96795],[54884,96427],[69283,94253],[57290,86228],[49069,63315],[81576,96612],[47000,47623],[70466,78530],[33241,35319],[46525,77973],[43199,45234],[22321,81984],[94337,96549],[36374,41587],[60330,76667],[66723,93309],[15513,75417],[4684,55508],[51687,96825],[18857,67447],[30774,74384],[69530,98925],[56802,90632],[23032,71994],[69739,78552],[11124,23739],[31608,80222],[96074,96540],[61597,65816],[42391,87653],[76805,94825],[23973,30395],[23278,91906],[72270,90114],[57783,75225],[29360,87073],[69467,85002],[63121,66650],[64197,80964],[54185,79970],[31930,48230],[7618,49978],[6242,18797],[81114,95426],[61782,67150],[97633,99349],[82545,82617],[25958,91853],[96733,97139],[83674,87527],[63125,80942],[8747,35643],[41906,70824],[28192,51638],[36300,88600],[86001,95506],[79467,95110],[89662,95222],[72182,99930],[94949,99509],[97900,98300],[79447,86065],[48195,52682],[47463,50406],[78596,86595],[86974,93008],[28378,83026],[46648,61476],[65226,89482],[24812,74926],[67306,92111],[98058,98402],[50577,89493],[37343,65631],[34949,79213],[23149,81273],[10071,48365],[7780,73682],[37251,63459],[14826,28891],[17486,96155],[82653,98787],[69205,77919],[87953,96331],[73624,77929],[96236,96590],[90654,91362],[27030,95348],[6108,89486],[19018,45067],[24005,41374],[11804,34576],[21169,72284],[52841,78748],[90368,96820],[1148,74333],[79672,85424],[68140,72845],[77799,81215],[15262,38722],[80842,83357],[39350,53683],[18055,37728],[51375,99898],[4985,73477],[2849,95100],[36294,93578],[79923,98500],[71354,92405],[97733,98040],[23376,23790],[28023,55729],[4752,8403],[27174,49792],[18237,27431],[3581,89963],[21088,74892],[50540,66309],[39101,83013],[69706,90718],[32722,68605],[27011,46189],[7363,91761],[51748,88144],[13123,85202],[85441,93614],[80121,98572],[43337,94495],[9635,14781],[68340,83329],[8360,19459],[63572,94992],[65709,70746],[23317,96753],[68262,98841],[713,9865],[70488,90687],[76059,84037],[16357,42953],[18584,74741],[99657,99929],[91016,93357],[55517,79518],[19892,31403],[95689,95792],[77184,88423],[39915,60073],[982,28736],[39339,89291],[70834,76613],[52034,94193],[26978,67303],[74360,99970],[54400,79195],[25891,83352],[77339,88923],[86501,92864],[79231,85627],[18093,94461],[45094,52141],[4916,7271],[6082,93850],[47434,90647],[36306,99170],[88756,88858],[72465,98602],[99632,99744],[39538,76223],[3281,64745],[76982,81179],[24484,50589],[72704,72959],[93626,97490],[26944,87499],[67384,97785],[47725,86378],[19342,44162],[1852,63869],[21932,42877],[46147,61300],[27668,49806],[87794,88693],[49862,84374],[88330,89420],[27647,30599],[27697,55101],[11434,64521],[90556,92001],[2048,77104],[63147,93466],[75235,96991],[71721,97249],[26205,53861],[57547,61728],[30017,89472],[49515,80300],[35237,67621],[82593,88023],[75444,90886],[27853,29881],[86213,97506],[50632,89893],[77250,78340],[46182,78619],[90518,91535],[11980,76801],[51213,69885],[43018,98469],[97602,98000],[60484,97330],[58952,60041],[83182,91967],[66677,84959],[96640,97216],[48836,98679],[83977,95995],[37177,68538],[29529,68642],[30193,59653],[42828,87934],[178,74033],[50593,94235],[61536,64110],[95284,95679],[38029,59035],[76782,93695],[4308,71383],[72888,72900],[61100,76236],[70303,81360],[72663,91798],[12526,89112],[25376,29241],[42400,85671],[21526,52418],[54192,58356],[73568,96049],[12193,31438],[26139,44479],[78442,95933],[77448,83432],[60209,68926],[69123,91211],[69631,83811],[94275,96635],[56485,67599],[56615,59379],[3230,62216],[63797,96960],[27495,69544],[93813,94767],[11753,50235],[42933,92031],[99441,99666],[55674,57258],[55113,56680],[43015,86315],[14929,36429],[50878,54851],[51088,70298],[32116,65880],[13837,43839],[26381,35683],[2857,46116],[40868,42384],[56868,56979],[96471,98430],[94667,97843],[3056,41609],[52149,89783],[805,61857],[87204,94219],[75664,87470],[90107,92945],[80292,90445],[31647,74475],[55558,77905],[89174,91553],[2574,79432],[17180,74107],[8435,26089],[18539,69340],[87494,98486],[70421,78690],[42511,85210],[59338,85615],[14588,91563],[14478,83029],[1504,83785],[37684,56513],[37232,59359],[17992,82725],[46109,92308],[29212,37529],[92909,94393],[12111,61907],[86008,92965],[88405,95338],[31196,57147],[88276,98769],[70265,77166],[47521,79768],[14045,53034],[19510,24554],[87114,92881],[71252,96210],[4148,22352],[40618,88183],[58498,88169],[36652,97178],[93157,99729],[45503,72413],[76422,80542],[34370,52751],[32024,69364],[82904,87960],[75628,80488],[3385,22806],[39373,89147],[92355,96996],[87919,94828],[48078,88515],[44329,53401],[51912,89995],[87532,90862],[50900,65997],[94691,95211],[97210,98414],[4398,77553],[93783,94523],[43444,90003],[58150,63090],[1213,42316],[24168,77478],[60620,63385],[24431,78158],[23861,75404],[29096,89552],[94783,96850],[59,7235],[36876,79458],[97206,97502],[41183,61851],[9562,24960],[13551,28887],[85419,95977],[96948,99986],[42293,68952],[8665,40981],[19966,55503],[24993,68819],[61713,69615],[40564,60740],[73913,77896],[46170,86759],[26174,86755],[78741,99334],[20738,79934],[26480,97363],[5528,43734],[15588,87299],[16630,32593],[66437,95559],[39207,87205],[30961,90739],[18798,84866],[83820,92723],[95455,96350],[53542,56117],[4414,69143],[55266,59585],[52386,88993],[26236,84550],[32443,52822],[66879,83626],[70648,80212],[73563,85041],[79018,91957],[78861,93460],[85476,94377],[22621,88335],[80481,91415],[78926,99790],[23131,37089],[15005,28282],[91831,94526],[12862,29549],[98432,99845],[72982,83975],[32987,63279],[87803,95449],[89334,94410],[48164,84498],[86829,89800],[25243,92738],[53580,55162],[26612,31224],[24323,41713],[908,60122],[45868,79159],[82318,89219],[10967,41825],[98043,98748],[28272,46808],[522,2485],[13960,67836],[59193,64892],[11104,81752],[18661,72236],[66656,75061],[69716,80243],[17419,19381],[80025,91359],[9738,53972],[67387,89406],[56761,73282],[43671,99845],[13925,81343],[64206,72149],[54029,73884],[72390,93584],[22419,88514],[16882,24099],[88657,95602],[19202,38689],[42777,79819],[55766,59816],[62558,95352],[72634,86945],[19641,31581],[23502,35419],[74513,97601],[45487,88108],[59406,79694],[81068,96268],[75999,99025],[53077,94910],[59959,73908],[2281,2413],[17509,19283],[67958,81626],[34832,84589],[67321,75373],[19023,69874],[1070,85164],[82522,90371],[1175,3640],[29264,85934],[22294,43264],[53058,98689],[61623,82430],[30229,88535],[87013,87569],[9494,14621],[10334,33258],[62759,91819],[59219,87760],[75547,99082],[44179,69454],[29771,90677],[70107,89937],[93586,93982],[95802,99477],[84618,94369],[48037,97717],[37187,98505],[37162,52213],[87435,87795],[5044,92420],[18148,73622],[63108,94236],[23350,49485],[64032,83105],[5707,62360],[51860,77239],[44086,73694],[43031,87429],[75385,97532],[36291,82210],[29095,57501],[28057,59177],[37619,59540],[57943,83581],[29677,87307],[65331,85644],[93845,97760],[1447,97598],[99851,99925],[7198,79067],[59686,98441],[77894,79939],[66554,85738],[52061,65866],[42705,57569],[11143,65252],[74752,84208],[47141,55395],[17656,71313],[33083,91652],[98033,99165],[94169,97722],[87992,91667],[74086,86908],[58099,85999],[92498,95488],[35160,48910],[13119,28755],[63946,85572],[47752,95570],[30311,55423],[80603,97833],[69899,84870],[58707,71375],[72784,97039],[35795,70556],[16520,23060],[30524,75371],[25447,82206],[11498,59459],[23319,99534],[50769,74601],[58960,98423],[51136,74027],[77611,85495],[30033,54478],[20698,98709],[54985,97027],[27014,99850],[55897,78381],[71638,89834],[45246,51616],[73334,94900],[88653,89179],[74627,95238],[99327,99692],[29348,78209],[47910,75832],[31008,85287],[84751,96061],[89710,98684],[17290,41647],[84356,96991],[10507,13591],[72480,93813],[80556,95666],[25062,97002],[80316,91895],[52457,55499],[79710,85789],[99263,99415],[44591,48050],[65807,93695],[78184,80610],[13213,57567],[65646,77611],[35413,86565],[25695,39316],[27246,96601],[75984,80330],[98051,98150],[87680,93378],[10569,12743],[9337,52477],[88022,95200],[67099,90598],[33132,65657],[98615,99946],[35737,62558],[16690,20437],[53827,79100],[71792,87622],[50502,92825],[83001,86722],[46963,86488],[67123,89898],[50767,67603],[41729,65004],[65016,88289],[37133,55184],[1920,18716],[21569,95728],[43755,74974],[23656,86057],[29757,73636],[11188,65526],[20076,59232],[12914,26587],[17565,18735],[21668,59385],[31154,73811],[35066,92085],[37841,53529],[86363,95115],[6230,87018],[12494,56984],[39237,85978],[27362,92670],[36423,80723],[97601,99152],[20967,37758],[25463,34033],[59594,96223],[84403,94333],[73580,85026],[26828,31446],[36695,78748],[71092,83900],[37795,86368],[24371,97892],[64683,87213],[51172,97929],[81364,85835],[73158,80004],[3154,82312],[97991,98137],[98555,98765],[6711,77553],[95881,98477],[19883,60174],[79409,82814],[97529,99957],[76173,84191],[88471,98958],[17184,42829],[75791,93345],[40670,95612],[66539,75195],[98851,99352],[85127,93555],[72976,94515],[77535,89528],[96082,97196],[89960,90161],[67345,68630],[14353,25322],[20637,20823],[64229,86539],[8005,82047],[17470,22118],[3631,16833],[27864,65512],[99547,99782],[66534,94856],[37579,86665],[68756,72319],[2279,50052],[12722,89048],[31798,80901],[29863,61669],[21466,58446],[44921,98989],[55510,76225],[68250,77422],[84590,92045],[77409,85236],[58437,68329],[94881,97251],[55306,76230],[82012,92719],[67853,95335],[20102,57651],[63591,70378],[39833,55483],[8183,43491],[52162,89609],[78051,78202],[46663,56910],[61763,96203],[68280,79255],[26655,97942],[33589,67497],[52224,98435],[18778,93369],[94670,95186],[73333,73993],[64849,74196],[52340,73966],[59947,96269],[84652,94308],[17871,90347],[64330,78096],[67255,99606],[20108,83056],[78983,88655],[45214,52455],[7639,99251],[15340,51669],[47093,81574],[15028,55828],[68066,77091],[46356,48365],[32652,77641],[70154,94007],[94757,96684],[91892,98543],[5477,36952],[15083,82681],[61504,85825],[96392,99553],[11557,88083],[61326,80755],[66009,77918],[345,94800],[61714,89631],[77492,97965],[99779,99926],[77880,90862],[42631,83305],[38228,64218],[65146,92127],[37311,97482],[59196,80290],[60675,82993],[48070,56146],[15111,71227],[33552,36002],[21763,68977],[95960,97053],[44369,83448],[50185,64635],[86856,99486],[43112,82242],[31747,62256],[56725,80366],[36753,97182],[33578,84960],[84473,98347],[53444,76069],[41127,46389],[11106,94689],[39730,41099],[70481,91473],[66637,89389],[76734,91651],[77110,90370],[49842,78182],[25725,82011],[19887,37952],[22183,86831],[12760,17192],[17642,25446],[9825,36673],[94967,98159],[82903,85917],[90812,92033],[63815,88582],[32392,90392],[98939,99699],[8713,63268],[33146,66507],[90145,99821],[12419,62301],[34931,84520],[21587,75639],[8978,47634],[42229,80917],[20990,76011],[29300,54121],[51139,79069],[67949,84893],[40035,71272],[90629,92080],[5791,57884],[34345,99808],[57686,94629],[30681,47682],[86219,95022],[45532,58656],[33864,95660],[46353,51076],[47696,85003],[896,4307],[74918,80021],[13721,17731],[17018,64847],[15991,48547],[93206,97088],[80888,96546],[84836,87750],[265,92874],[93294,94299],[87029,87366],[86251,92669],[59244,80300],[95518,96613],[85431,93246],[92154,96069],[2510,56313],[10875,78239],[61498,77785],[50915,56570],[84475,92435],[88284,98347],[41861,75986],[6214,19841],[72227,87456],[27002,77680],[82875,87653],[39442,93632],[25112,53200],[39014,44383],[18581,31951],[61775,80093],[91671,95652],[41617,52028],[10549,77507],[12286,97129],[46481,93951],[26917,54341],[22318,24835],[7492,8217],[73179,76328],[81625,93434],[34175,53234],[62814,88781],[3961,73542],[99019,99701],[58621,99907],[42587,60900],[56745,59762],[78988,81965],[5746,9675],[7289,69103],[65670,72312],[62616,69707],[19950,24399],[28213,32006],[19129,21757],[61838,87593],[67414,67639],[55939,95162],[71159,89961],[91632,94102],[1279,35540],[6231,64121],[20203,98414],[30718,70070],[86242,92829],[5543,11265],[98285,99631],[73918,91121],[99731,99855],[45277,67008],[45110,97102],[28062,78809],[35541,39431],[67936,74138],[27810,68471],[95388,97923],[52164,54722],[51066,64649],[55042,56449],[74937,79978],[13069,45716],[76457,77736],[85224,91632],[2666,12466],[79113,99478],[49123,94935],[22987,76893],[45782,78707],[34592,93723],[34800,43025],[5444,52235],[41100,61381],[2223,31913],[13080,71988],[90437,94240],[10252,61098],[56454,69990],[91998,95959],[71332,82376],[91148,95101],[60712,90834],[69608,73003],[63188,99125],[62777,74273],[48331,82472],[81056,82682],[13769,58847],[60246,77987],[55741,58995],[8568,62257],[11263,55383],[41487,77773],[61697,80531],[25204,49114],[90926,92865],[79668,99430],[80103,96997],[18337,50750],[46874,53715],[54611,57788],[93370,99921],[79892,99225],[45808,98976],[75802,93394],[9140,75549],[36698,57806],[10890,85580],[97223,98267],[87196,93667],[58217,86546],[67924,80013],[57402,59328],[87796,88958],[88340,90814],[99807,99922],[92357,97796],[7444,7466],[15367,94621],[65116,72363],[88354,91903],[86504,93273],[27429,46866],[78148,93003],[91709,92454],[74054,96172],[91707,93003],[72107,76879],[57672,67584],[24975,57031],[74595,98111],[53873,60332],[72678,96145],[69625,97532],[78506,79719],[58920,79879],[52087,82357],[27821,58312],[11330,80482],[63132,86938],[70465,98513],[88357,91378],[47667,89682],[71694,99422],[80638,97096],[23261,50451],[24119,37149],[95956,99659],[74297,80116],[4491,61436],[86601,99367],[94733,94748],[19299,68132],[68417,94422],[303,30267],[78963,90647],[22095,44235],[74120,84208],[1126,85014],[90131,95166],[46369,69012],[75951,77170],[38132,56205],[14363,29911],[85933,95598],[95612,97780],[94346,96247],[4799,59363],[90874,96219],[18069,85467],[96982,98541],[66144,93967],[35973,98580],[21964,22019],[3256,10856],[34491,96673],[74946,90146],[16726,47407],[52092,89602],[60287,74932],[37424,56702],[48254,56189],[3541,24393],[98547,99812],[23600,91324],[44140,67233],[5204,27932],[24969,30774],[71482,92196],[11381,38712],[28760,87536],[8111,83855],[56917,81480],[747,38564],[5793,60542],[75112,79850],[23682,41015],[15841,50034],[31867,85508],[19585,58395],[64514,75731],[88718,91352],[27907,73038],[75519,80061],[3810,64609],[80693,92778],[92686,97620],[90255,95569],[87588,98962],[55077,57708],[14492,47970],[27840,38638],[94159,94950],[57177,92506],[57257,93053],[74887,75745],[3282,14340],[60825,97924],[72388,88833],[28835,75662],[37997,81516],[81015,82323],[92397,94661],[80553,83373],[50891,86820],[34836,85263],[86004,97514],[47457,87806],[49583,99388],[4378,46588],[98427,99307],[43717,52790],[56016,92358],[83365,87889],[39443,46551],[93661,97704],[23421,48464],[18869,83525],[21063,49156],[626,49818],[82708,99987],[96697,97761],[7046,90524],[23019,99925],[41216,95442],[30443,77356],[29622,92859],[63036,88432],[61073,63632],[10828,46678],[60056,83220],[43568,44692],[84064,99427],[54628,79970],[92995,95083],[7504,61750],[93684,97060],[85023,93743],[27158,30675],[89445,98379],[83130,96887],[73579,90973],[1104,15978],[14128,85596],[26007,62734],[13633,54789],[71984,84670],[88495,90141],[27814,82058],[71023,74569],[67820,81317],[22547,90502],[74280,86356],[5788,50784],[65600,86175],[19878,61215],[51381,61484],[96822,98984],[40019,79094],[35884,77929],[29550,87052],[69920,92651],[55653,83771],[64482,79540],[35120,61121],[14275,71069],[56794,80895],[70188,83396],[28775,98248],[4577,21130],[83572,85275],[83694,87646],[63276,88451],[38010,46731],[92601,98433],[9927,52936],[65259,75883],[88358,92099],[28671,58475],[13393,32262],[53903,90823],[53543,69133],[93708,98624],[48788,60100],[61286,65124],[50695,80926],[84979,91040],[7766,77556],[2821,14040],[66475,99523],[99645,99677],[31577,63948],[28996,70861],[86261,92082],[78986,79283],[98792,99099],[91490,97224],[82204,86281],[60182,76824],[99935,99980],[78521,96597],[18253,73784],[49183,65711],[29739,95067],[45799,75355],[30659,71267],[82704,91172],[84842,94312],[94576,95155],[89855,92598],[99883,99887],[2970,76922],[84832,85886],[91602,96396],[19426,58458],[40855,85461],[2220,47322],[44362,50417],[12705,27052],[93734,96901],[31051,40638],[38114,52973],[72495,95929],[78304,87320],[26760,31199],[3399,10194],[43729,46966],[14493,30356],[19389,68607],[16359,92213],[46836,90475],[37129,52476],[92120,93664],[71830,72434],[22158,31735],[7982,84820],[30662,44949],[75884,90617],[21806,46055],[53670,70882],[96962,96987],[39665,46402],[47991,61376],[66616,74155],[46375,65131],[66339,77783],[9669,22370],[42771,72234],[58958,98627],[27754,39498],[68458,90531],[89802,90674],[38841,69310],[18626,53862],[42626,54235],[93460,96866],[51840,81010],[48530,77378],[88954,92107],[71586,80676],[72790,90976],[46884,83689],[46633,77263],[43987,54161],[63123,66226],[34178,48032],[12221,40760],[92881,96887],[24440,24465],[27388,90146],[96451,97519],[46162,61624],[20891,86113],[75007,76152],[20493,94992],[28841,54054],[71150,90341],[3370,75919],[75279,87852],[66027,91128],[15780,75585],[19343,63005],[75760,94701],[3691,6635],[15990,22110],[66840,95010],[9107,65947],[61002,74033],[44972,48502],[57624,78129],[91389,97487],[37570,95560],[65215,75545],[79582,82271],[16258,63649],[24691,59972],[28461,85203],[11657,54116],[6302,49949],[30342,80120],[41733,69917],[62013,72671],[78668,91970],[34854,75216],[44999,83873],[40318,93825],[23965,99223],[88041,97311],[60688,71307],[5950,41724],[86316,94066],[47199,86520],[76156,97411],[14644,62319],[59358,99760],[64437,97921],[17352,38736],[51321,87154],[6530,37922],[492,3435],[82438,99832],[95692,95745],[61810,85078],[46775,49583],[20901,28533],[70720,91586],[62874,85621],[32751,92017],[24054,76361],[87025,93841],[55442,77597],[65593,83817],[87834,95135],[82933,90981],[39636,65906],[60631,70815],[84442,92067],[1048,34732],[74228,80100],[62016,93690],[40343,90550],[20591,43547],[86757,90961],[12072,72332],[44105,78347],[38740,46125],[22954,49577],[42294,88896],[81635,85074],[40906,77262],[39809,63912],[28483,68870],[73077,92161],[14786,81738],[25561,80800],[61360,68527],[98621,99121],[17426,19436],[37757,76704],[7028,66295],[94258,98575],[21339,80084],[34157,53930],[45516,58992],[95369,95730],[56238,87440],[9697,26750],[66602,97598],[26009,76824],[73452,96321],[59612,67621],[52618,76341],[59246,90417],[39427,61224],[55298,67732],[94109,94196],[71369,98932],[5105,32446],[76692,86291],[78862,84777],[39478,61644],[39476,82219],[92386,96595],[64960,77272],[25150,65242],[43768,60201],[85374,96644],[62829,73708],[10763,23994],[95042,96933],[42337,75303],[92558,98116],[85897,87385],[37361,40593],[49392,52004],[56181,57719],[50667,52430],[17064,34396],[27492,29368],[7075,53843],[62930,71613],[48452,82133],[82202,93413],[5905,29639],[55272,99710],[99575,99726],[87640,97292],[32065,76088],[35110,92064],[84161,98504],[62638,99730],[81433,81461],[65751,76592],[83843,90119],[84232,93575],[95987,97753],[15256,40704],[7490,70862],[96182,96594],[45488,67090],[77150,87009],[76390,87959],[93939,98344],[8216,57867],[47040,76965],[517,3014],[9413,64776],[34445,46103],[57898,84524],[46842,73172],[69152,84023],[62842,63604],[99581,99890],[97727,98378],[10931,38738],[34147,39265],[60801,86780],[42269,98325],[66084,89903],[35124,35423],[49822,62014],[84634,87284],[78400,91980],[59596,91144],[94877,98479],[47162,49702],[96211,99615],[37063,88646],[89411,94228],[53457,56886],[53802,89905],[13595,36052],[14446,53651],[67139,96649],[47365,49476],[66037,96711],[37281,65598],[37388,87704],[77453,91636],[68311,90769],[93537,94642],[93125,98111],[19951,29093],[74971,96379],[13667,79195],[18698,67762],[22790,27951],[80411,87178],[3428,52924],[43137,49965],[27085,53686],[3499,19622],[94122,98114],[86390,90089],[50730,69046],[5591,59435],[43556,56473],[11795,76967],[54870,58946],[88534,90470],[96672,97458],[47188,93986],[34903,67419],[39012,48703],[41647,52034],[57154,62147],[65462,94326],[74310,82137],[97834,99967],[49919,80366],[77920,98685],[78738,85991],[17929,94798],[90465,93443],[40456,82363],[45886,50409],[29325,80760],[45249,91573],[17516,83776],[35046,47207],[1062,4955],[25781,59430],[94997,97734],[13499,33796],[63599,81512],[30267,51691],[3265,78656],[56422,73754],[49934,98547],[65404,82974],[5953,37467],[17364,99256],[18334,94078],[2333,4107],[14995,75176],[9630,15766],[25990,70747],[44739,90865],[8368,19130],[31409,98471],[83377,94722],[82536,97300],[69990,94815],[78012,90733],[3117,30323],[92624,98756],[23161,63018],[82955,99960],[12382,92668],[27441,85839],[23764,31683],[63606,65963],[64388,85556],[61769,78031],[75528,76057],[58554,90213],[7704,92301],[46702,53848],[67725,82790],[40183,88604],[94373,98351],[56107,64046],[12959,14152],[88023,99423],[43118,98119],[67905,81048],[56331,75512],[43906,79805],[48852,69777],[8427,57349],[2236,60567],[28589,39727],[58938,73107],[18232,25668],[31976,39400],[95082,96570],[35183,37034],[6829,48468],[46573,86802],[85704,94972],[78677,88502],[27392,57043],[80134,88940],[5238,29634],[11186,30395],[50158,93505],[88042,91537],[88783,98980],[26133,62669],[18399,29721],[20885,56202],[16037,33314],[87365,95737],[59601,92068],[70050,82054],[16975,55849],[76616,81391],[36146,96602],[91185,95214],[96309,97814],[71534,83792],[179,53670],[26123,92326],[75742,96754],[26443,66275],[21548,73545],[76050,97652],[36293,84905],[22142,81760],[15756,79253],[76866,89741],[86402,88975],[16908,82956],[12141,96001],[56383,97391],[88348,99577],[29902,75519],[30676,74808],[51727,75102],[97823,98639],[72439,87965],[30803,50683],[85080,99484],[5594,98106],[2760,46296],[47533,58246],[8205,97838],[89763,94389],[52443,99806],[79542,88687],[58294,78592],[14867,60027],[63943,91010],[61364,82127],[12415,68451],[51201,81319],[71053,73948],[38624,91359],[31837,81904],[26192,59235],[910,5941],[8997,35937],[97872,99876],[50810,71635],[58404,68669],[57331,75482],[76809,96131],[45222,87848],[37678,85939],[10276,95259],[28021,92089],[16815,25747],[16753,44683],[78350,82642],[30645,75860],[32956,95138],[4878,34844],[76412,99306],[62419,89924],[88176,90122],[12259,57976],[68789,88137],[96540,99603],[99448,99478],[56739,72778],[11204,70095],[58026,70548],[60751,72412],[33580,96973],[6075,59970],[38577,45848],[34323,67859],[86827,87402],[61345,78458],[3288,95411],[91989,92843],[12024,96666],[1548,36314],[15916,22047],[72570,77735],[3150,82730],[29938,87419],[3259,73004],[77533,85848],[21380,64022],[38280,50124],[33591,96391],[89157,99054],[514,45720],[72333,89914],[59863,76925],[76504,88928],[12658,76955],[71416,94709],[50688,55862],[70752,99646],[11366,34159],[23326,45511],[25235,56951],[69981,90685],[6387,50320],[47369,86588],[48504,62014],[56028,90391],[32531,95597],[82542,84609],[40562,54965],[69144,89454],[62872,94585],[23544,70197],[7742,51211],[47831,49821],[30882,79725],[49911,78727],[36600,90185],[84831,91524],[82799,91672],[15403,75264],[18159,35614],[97743,99184],[74134,80378],[19177,79124],[49928,50712],[90685,93031],[77049,98537],[57192,62318],[175,90210],[14185,93802],[99926,99999],[31635,72386],[35055,77002],[24277,58889],[44638,69958],[40992,46376],[66426,83197],[82149,85405],[43221,46434],[15842,39863],[84878,93250],[78779,97415],[32062,57135],[12561,19492],[97688,98441],[22138,35671],[34486,71909],[17053,92600],[81763,93799],[10332,75076],[38403,79615],[6559,65363],[65118,98174],[71241,86369],[61130,73017],[16396,25454],[56244,61739],[2581,24905],[91238,95759],[65756,70863],[86472,86698],[63986,92213],[31815,74986],[33236,86796],[15702,55328],[88435,98366],[16040,20426],[96775,97770],[87907,92673],[38735,93474],[73250,91658],[60026,89471],[85060,99326],[16321,28564],[78516,99612],[43870,68015],[40883,53790],[99723,99857],[96972,98246],[76536,96819],[73413,89954],[30890,91764],[93803,93905],[44175,87670],[5947,11087],[48144,78965],[28399,29088],[95263,98942],[98467,98755],[74973,80724],[69391,99535],[43253,89939],[62899,72585],[43142,94506],[38742,66714],[44119,92088],[23718,48531],[98780,99803],[98598,99663],[44102,95170],[21608,48488],[54276,89149],[72569,93670],[89797,95460],[17132,65971],[54420,55361],[31676,89354],[16455,93963],[27198,75756],[55367,62289],[16026,95306],[814,13814],[84846,94736],[90381,96223],[25787,51823],[46228,76487],[35544,65634],[84459,97365],[48958,69281],[57050,97515],[77436,83350],[42271,65612],[26336,36556],[42788,46192],[35234,68920],[21939,79289],[23668,31216],[4671,22183],[1482,17773],[55438,90597],[54202,64322],[12303,35280],[56451,85541],[1207,86552],[88841,94124],[55083,82837],[12649,46258],[52977,92437],[24011,59875],[85585,91318],[8247,14036],[37252,42100],[5018,44180],[70729,78397],[7641,55338],[81004,99115],[46608,61213],[53489,92708],[85961,96470],[61759,70428],[40233,69229],[30238,85388],[11078,37603],[6014,7270],[14997,78021],[95926,95968],[88706,97429],[159,56720],[5406,91488],[86719,91254],[91926,95190],[9037,74317],[61781,99537],[33024,67192],[19464,97619],[33020,44145],[50983,79473],[36662,54465],[18734,30731],[43891,74908],[16189,36429],[94591,99869],[88912,89543],[70943,81713],[33230,86426],[96792,97726],[82015,93509],[7305,11356],[27016,56735],[48432,83357],[63427,71560],[16979,21772],[51978,56625],[66358,94179],[8524,60584],[22022,76610],[30246,92057],[71913,98265],[85481,93136],[30985,79469],[18106,52156],[64138,81166],[42513,71033],[21469,53072],[51108,67633],[33416,67012],[16788,71081],[53493,74531],[15333,28405],[39502,98665],[7150,68008],[82240,88406],[72204,96773],[82257,95971],[44407,58529],[58431,83741],[22270,26375],[75364,91548],[41176,58874],[14463,65864],[66001,86594],[36679,47141],[81072,98669],[28239,71527],[34111,57190],[38663,62644],[95335,97583],[83699,97090],[20081,79025],[76976,95944],[82059,85633],[56621,69005],[93304,98341],[41662,92206],[72135,78469],[69695,70560],[53198,86724],[17436,76667],[2899,94522],[56167,97985],[39454,72853],[11739,58953],[63189,73818],[30144,55452],[49238,61167],[66772,84596],[75333,79150],[80082,89729],[87350,96717],[44333,89884],[53810,98412],[65463,83084],[14054,76941],[73655,96303],[98854,99481],[82495,90541],[99212,99761],[58863,62943],[24282,79009],[41345,60866],[77036,92121],[16118,58862],[53008,79797],[87109,99696],[14006,70512],[3463,25761],[10983,16066],[33852,49087],[51106,80670],[68159,98247],[17860,65766],[6622,45122],[72226,84403],[25948,36703],[93368,93637],[29080,35214],[79572,94529],[50734,94593],[43666,90464],[81540,93564],[71102,76045],[15471,33475],[10298,14516],[14797,63284],[56575,78124],[98667,98679],[37782,76096],[93247,95070],[75633,95552],[49560,78743],[74061,94509],[92015,96465],[12025,15094],[56185,83194],[61830,69299],[22513,44393],[53963,72500],[12713,24071],[59361,88779],[92044,96038],[29068,84068],[57597,93437],[95026,95284],[56538,71994],[71242,96363],[88364,98544],[77186,95557],[76170,89686],[36508,97790],[85299,99080],[72617,77632],[16254,17529],[78878,85681],[11216,84380],[22752,62573],[47866,86752],[26560,64386],[60956,95279],[9687,44844],[43203,93371],[90573,95804],[49763,88879],[59461,94562],[55,48398],[5537,22175],[54372,88352],[45583,66946],[69968,75765],[28187,37145],[98023,99561],[31472,49551],[90743,98962],[82347,91843],[41403,59842],[15007,19343],[84217,98503],[14228,63284],[47228,87782],[90546,97115],[9804,62778],[63153,68028],[54475,85218],[97729,99270],[6059,52585],[98466,98947],[96732,96843],[54565,66559],[69314,70771],[68603,92273],[21605,89629],[94436,99825],[41785,63032],[63045,77337],[27785,30452],[15959,72417],[79359,96794],[93408,95865],[26088,69215],[25622,74172],[63483,72165],[63638,89335],[12729,22167],[67796,85495],[58165,94150],[14242,32256],[30183,60913],[45042,98414],[86078,96914],[14585,87116],[97614,98901],[60737,93515],[48756,83097],[18549,84857],[16419,93220],[49165,78242],[26230,88353],[60910,80361],[13267,82699],[3348,23171],[96549,97209],[27312,87909],[27497,44170],[27598,57247],[68625,98438],[23251,39769],[62853,89852],[59120,89960],[52074,74908],[41857,75660],[25013,35751],[59122,78309],[16010,20492],[85501,87306],[57858,64663],[6577,99839],[89607,99155],[17313,96043],[70315,96183],[20415,76185],[50000,81970],[13927,38067],[40087,94976],[58433,68351],[67672,72257],[15886,17140],[14620,70999],[41286,94396],[98514,99620],[82724,96236],[83122,92141],[61683,93276],[32366,82984],[45691,53301],[11736,39355],[17697,39890],[96624,97854],[20188,48083],[4075,78460],[41641,93368],[52139,75045],[85858,90196],[16141,71187],[26511,96830],[59685,65998],[45713,99019],[91495,91983],[10188,60261],[36990,77584],[69751,98455],[21461,35273],[72431,83686],[57253,71576],[22910,25586],[50982,76566],[53962,95546],[12470,90060],[45344,74177],[76979,99770],[17608,82176],[32888,37449],[45367,93833],[78873,96313],[69244,81190],[89397,95045],[59765,62412],[94344,96557],[12700,46049],[34255,95874],[3470,44452],[77281,99016],[38861,98635],[82339,86919],[32839,70247],[25189,81152],[96260,98692],[48601,70836],[13377,14555],[984,27046],[20501,75021],[87090,88483],[71284,94798],[37720,53661],[11829,21325],[45471,65253],[5839,74266],[81954,90789],[51810,78493],[78826,87349],[78599,81472],[72057,99195],[11350,86164],[87392,92368],[8650,37671],[14265,75448],[85911,91708],[10563,39988],[8761,58705],[20542,54799],[42578,91998],[49662,64917],[59384,92791],[3909,97563],[52226,97854],[31546,56161],[71134,91907],[55978,89241],[15872,27610],[12401,33563],[75042,97045],[12815,73359],[89773,99152],[54759,83346],[74892,95999],[13658,58830],[87896,91825],[25388,37729],[77720,97146],[42906,49379],[20349,32224],[97930,99893],[17695,38450],[93677,96459],[98742,99379],[9311,22435],[48241,62080],[85047,95115],[3284,26309],[35588,82665],[73774,77006],[46099,72652],[60143,82329],[71075,93983],[6139,11940],[15612,90825],[92758,98319],[81700,93952],[97492,98271],[36035,41902],[79713,98646],[6619,28330],[80809,81933],[89719,95047],[96915,98484],[56116,88690],[62182,99034],[16390,52705],[26684,91765],[84994,97955],[87754,89567],[50529,93115],[57829,91835],[17998,74644],[67556,90458],[37442,44317],[47008,58930],[8788,49418],[5321,18306],[2376,41826],[12258,29058],[50139,72988],[22920,46273],[87763,90059],[60349,81681],[44255,88460],[93452,94080],[77726,86666],[75899,89759],[24320,25872],[75644,84894],[3408,13499],[70738,95982],[11951,66605],[94299,96683],[17576,90013],[63429,91410],[42553,68346],[72401,87954],[66632,97299],[95847,96095],[71048,77223],[71038,71358],[46951,85606],[33725,85131],[76754,95406],[1021,5034],[52300,72875],[21071,44131],[22647,55531],[31406,76458],[51814,85229],[26405,34535],[72221,99860],[26561,31164],[6831,73823],[30813,77808],[96406,99867],[97926,99876],[75291,91876],[65310,65905],[68880,70319],[29593,39950],[84042,97786],[10386,14500],[53848,69554],[98172,99529],[15701,80499],[76001,84484],[33356,37158],[69593,77600],[95130,99838],[8482,64384],[9149,80333],[95936,98574],[24035,80560],[33710,43979],[87200,96128],[84891,92613],[35263,40989],[40599,47654],[27742,97413],[24248,70494],[86842,97667],[21598,42057],[27932,65162],[2611,67658],[53127,88553],[11176,28159],[9036,17382],[78237,82078],[4796,19453],[71649,79735],[6608,83209],[1569,44717],[50560,95426],[73777,84564],[31728,92420],[18981,36878],[88376,97394],[46053,77422],[28639,77317],[14257,70802],[14580,34003],[17784,18456],[52858,69603],[79876,91895],[93678,97188],[1470,3599],[22825,34180],[39919,86226],[73705,74171],[98047,98439],[72758,76140],[83019,93092],[37229,39236],[11584,50127],[42382,48664],[61978,95533],[96519,98751],[67433,73530],[19894,64695],[90780,92485],[40611,53802],[8782,84321],[27919,90131],[67997,89518],[76849,83781],[63870,93871],[96757,96927],[57035,90902],[37746,46207],[52456,91128],[30531,74211],[84157,92551],[79035,81830],[86441,93870],[8705,31735],[42903,48101],[40089,46974],[12962,21729],[3785,57995],[40846,75291],[92461,93259],[57912,86184],[16320,42201],[13231,51682],[73548,76410],[47750,95627],[16265,84084],[99051,99255],[90187,91234],[73433,76456],[96881,97928],[13676,78398],[11440,53661],[89507,95840],[72542,90044],[67520,76011],[2520,91861],[79451,97190],[75290,86127],[78965,85404],[85410,92594],[82660,84450],[22572,47150],[47213,67501],[64815,76639],[9207,74013],[78587,81676],[29019,69622],[36994,94478],[78226,84624],[44434,88575],[47934,93778],[92757,98635],[45358,82379],[87027,96159],[59788,97254],[81542,84553],[89130,94870],[41089,57230],[4910,70944],[20702,77756],[12083,48947],[1982,67319],[52910,79567],[79448,87660],[32793,88685],[69098,81558],[48688,58174],[23939,56104],[66382,71705],[16607,28508],[55598,95187],[84080,94549],[94754,99347],[28554,75525],[30633,86799],[55617,95549],[8281,8541],[36405,81228],[14836,93129],[44616,55527],[2346,26182],[79678,83479],[34432,39384],[10058,13874],[44133,91094],[99798,99921],[84758,85649],[37400,92177],[89208,99362],[33056,71511],[70335,90449],[67067,71339],[61271,82705],[69234,92787],[19771,64807],[1994,41357],[68797,70712],[17996,33150],[87211,90081],[9212,69801],[10934,17781],[71618,73375],[58829,90368],[13320,37774],[89031,89949],[30223,35800],[90198,95485],[44026,63725],[44164,61316],[10268,38468],[48448,95832],[7955,76751],[61684,97586],[28243,90818],[85840,97846],[73490,98136],[13842,96818],[73791,84527],[61970,93296],[81463,97067],[4686,55036],[53984,80137],[53844,72029],[33738,73258],[80111,82629],[65507,67509],[52494,92626],[18332,82791],[67015,99477],[86680,91871],[21368,41390],[32123,42273],[58643,79347],[87701,96330],[26985,86069],[49328,65131],[31341,55675],[81660,83840],[28679,32325],[62646,71476],[57622,65773],[27028,30375],[9056,48649],[77773,80473],[272,34726],[75285,96308],[9017,61836],[87434,97212],[89037,93199],[16034,64371],[57108,85908],[45755,60381],[32035,61080],[51690,85926],[29145,30888],[44648,96814],[53160,77292],[87022,97089],[73592,74991],[30938,39313],[49489,97856],[69620,91040],[1011,18077],[84392,85070],[87272,94231],[16335,93002],[49364,60041],[28710,56180],[59052,83621],[27686,79269],[74416,90928],[87357,92766],[80774,87802],[14076,90746],[94339,99332],[75763,86596],[63383,93769],[59895,73139],[83540,88443],[96623,99150],[69150,78386],[61429,67945],[13919,14015],[39728,64230],[76180,81498],[91780,99729],[86548,96274],[63234,67411],[84270,96047],[40285,66600],[13748,24491],[4312,62378],[38226,58094],[42589,84335],[32568,37037],[4737,68165],[81276,90528],[11229,88057],[28774,78938],[92910,97647],[43287,46058],[39620,46487],[77929,94902],[36157,44786],[68495,93186],[27447,46666],[44783,56524],[39702,93491],[7791,32096],[86498,97564],[94954,95205],[80138,83000],[24697,96806],[96591,99575],[24198,75648],[29049,96667],[73627,76349],[35714,68834],[45866,71800],[20923,62307],[47528,81140],[50537,91615],[32054,61642],[79662,95219],[50106,84006],[94468,95076],[53176,93717],[13375,66767],[32381,87250],[26756,96235],[69469,74822],[34224,49015],[85980,97996],[35221,68853],[55624,86046],[15042,16664],[94595,96418],[43974,92547],[85189,91509],[96348,99366],[22357,78119],[59320,66960],[86399,92181],[20131,60347],[40743,62120],[36257,76302],[99944,99945],[89604,90347],[48381,75857],[31929,37570],[47785,96032],[42848,66985],[51979,89937],[93165,95468],[72549,75195],[10705,34954],[62236,85752],[12842,24323],[60274,71433],[60790,81470],[74453,97975],[85539,99189],[55379,87354],[72712,99647],[67369,97492],[10359,25025],[34167,64858],[97297,97695],[39325,80268],[24046,89216],[70660,89661],[10346,65711],[97703,98757],[72019,81633],[67881,85499],[38989,45535],[30462,51983],[93420,99013],[81852,89801],[23592,84614],[25322,50101],[71411,96166],[80823,93814],[52807,85935],[49200,71161],[89104,91384],[93268,96483],[99864,99874],[5544,83853],[30805,61641],[10341,61439],[46482,74062],[76637,97125],[10024,12036],[30514,83647],[68939,94235],[48084,89459],[16998,82805],[90274,98154],[533,43354],[27316,61628],[65682,69511],[13705,52980],[37807,80290],[2169,36901],[36046,84215],[23199,69856],[22639,63704],[60598,80014],[57538,81494],[48430,55677],[85105,93377],[15868,88183],[15006,42307],[50325,99723],[67893,69296],[64088,64973],[22791,94011],[47247,59178],[77072,87094],[85238,95248],[19315,39650],[55335,87588],[92684,93604],[27018,62313],[61370,93303],[83505,94290],[9855,66263],[28804,56949],[34360,85936],[57324,99024],[73707,98170],[85546,89725],[49480,83039],[5331,49757],[17281,62338],[85043,88187],[49658,67659],[71593,89875],[78597,83263],[67735,73518],[28502,44035],[91999,93977],[74107,92145],[42234,68754],[34683,42127],[83903,97296],[20905,70688],[85520,92607],[74663,93556],[11751,61642],[57528,73186],[19444,28262],[13297,87392],[70579,92875],[37935,44350],[13758,89781],[51884,84491],[96215,99733],[229,2029],[85371,96934],[11118,80772],[94288,98680],[1191,10224],[26534,55573],[69549,95592],[54766,62266],[33669,34271],[88876,88928],[18933,66247],[763,21777],[99921,99968],[56767,79229],[80269,98879],[17193,47571],[65085,91969],[10164,80635],[54031,74963],[59540,95484],[3048,26407],[77022,98224],[48022,72115],[67393,70805],[92986,94959],[72203,80462],[86317,87659],[70582,72108],[1918,63103],[73575,82849],[56530,87778],[64256,77416],[1711,55738],[90032,92781],[21243,49960],[68833,92535],[74307,87866],[83064,95162],[28616,56840]],
+ targetFriend = 2304
+ ```
+- **Output:** 2027
+
+
+**Constraints:**
+
+- `n == times.length`
+- 2 <= n <= 104
+- `times[i].length == 2`
+- 1 <= arrivali < leavingi <= 105
+- `0 <= targetFriend <= n - 1`
+- Each arrivali
time is **distinct**.
+
+
+**Hint:**
+1. Sort times by arrival time.
+2. for each `arrival_i` find the smallest unoccupied chair and mark it as occupied until `leaving_i`.
+
+
+
+**Solution:**
+
+We need to simulate the arrival and departure of friends at a party and manage the assignment of the smallest available chairs. Here's the step-by-step approach:
+
+### Approach:
+
+1. **Sort by Arrival Times**:
+ - Sort the `times` array based on the arrival time.
+ - This helps process friends in the order of their arrivals.
+
+2. **Use Priority Queues (Min-Heaps)**:
+ - Use a min-heap to manage unoccupied chairs (`availableChairs`).
+ - Use another min-heap to track the occupied chairs along with their release times (`occupiedChairs`).
+
+3. **Iterate Through Sorted Times**:
+ - For each friend's arrival:
+ - Free up chairs of friends who have already left by comparing the current time to the friends' departure times. Move those chairs back to `availableChairs`.
+ - Assign the smallest available chair (from `availableChairs`) to the arriving friend. If no chairs are free, assign the next smallest unused chair.
+ - Push this chair and the friend's leaving time into `occupiedChairs`.
+
+4. **Track Target Friend's Chair**:
+ - When the `targetFriend` arrives, record the chair assigned to them.
+
+5. **Edge Cases**:
+ - Consider cases where multiple friends arrive at the same time.
+ - Ensure that the smallest chair is always assigned.
+
+Let's implement this solution in PHP: **[1942. The Number of the Smallest Unoccupied Chair](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/001942-the-number-of-the-smallest-unoccupied-chair/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+- **Sorting** ensures friends are processed in order of their arrival times.
+- **Using a min-heap for available chairs** allows us to always assign the smallest unoccupied chair.
+- **Occupied chairs heap** helps track when friends leave, making their chairs available again.
+- When the `targetFriend` arrives, we directly return the chair assigned to them.
+
+### Complexity:
+
+- **Time Complexity**: _**O(n log n)**_, primarily due to sorting the `times` array and managing the heaps.
+- **Space Complexity**: _**O(n)**_, for storing chairs in the heaps.
+
+This solution efficiently handles the assignment of chairs, ensuring that each friend gets the smallest available chair, and accurately tracks when chairs become available again.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
+
+
+
diff --git a/algorithms/001942-the-number-of-the-smallest-unoccupied-chair/solution.php b/algorithms/001942-the-number-of-the-smallest-unoccupied-chair/solution.php
new file mode 100644
index 000000000..18070658c
--- /dev/null
+++ b/algorithms/001942-the-number-of-the-smallest-unoccupied-chair/solution.php
@@ -0,0 +1,52 @@
+ $time) {
+ $times[$i][] = $i; // Add index to the end of each time entry
+ }
+
+ // Sort times based on arrival time
+ usort($times, function($a, $b) {
+ return $a[0] - $b[0];
+ });
+
+ foreach ($times as $time) {
+ $arrival = $time[0];
+ $leaving = $time[1];
+ $i = $time[2];
+
+ // Free chairs that have become available
+ while (!$occupied->isEmpty() && $occupied->top()[0] <= $arrival) {
+ $emptyChairs->insert($occupied->top()[1]);
+ $occupied->extract(); // Remove the top element
+ }
+
+ // Check if this is the target friend
+ if ($i == $targetFriend) {
+ return $emptyChairs->isEmpty() ? $nextUnsatChair : $emptyChairs->top();
+ }
+
+ // Allocate chair
+ if ($emptyChairs->isEmpty()) {
+ $occupied->insert([$leaving, $nextUnsatChair++]);
+ } else {
+ $occupied->insert([$leaving, $emptyChairs->top()]);
+ $emptyChairs->extract(); // Remove the used chair
+ }
+ }
+
+ return -1; // This should never happen
+ }
+}
\ No newline at end of file
diff --git a/algorithms/001945-sum-of-digits-of-string-after-convert/README.md b/algorithms/001945-sum-of-digits-of-string-after-convert/README.md
new file mode 100644
index 000000000..3803f7aad
--- /dev/null
+++ b/algorithms/001945-sum-of-digits-of-string-after-convert/README.md
@@ -0,0 +1,117 @@
+1945\. Sum of Digits of String After Convert
+
+**Difficulty:** Easy
+
+**Topics:** `String`, `Simulation`
+
+You are given a string `s` consisting of lowercase English letters, and an integer `k`.
+
+First, **convert** `s` into an integer by replacing each letter with its position in the alphabet (i.e., replace `'a'` with `1`, `'b'` with `2`, ..., `'z'` with `26`). Then, **transform** the integer by replacing it with the **sum of its digits**. Repeat the **transform** operation `k` **times** in total.
+
+For example, if `s = "zbax"` and `k = 2`, then the resulting integer would be `8` by the following operations:
+
+- **Convert:** `"zbax" β "(26)(2)(1)(24)" β "262124" β 262124`
+- **Transform #1:** `262124 β 2 + 6 + 2 + 1 + 2 + 4 β 17`
+- **Transform #2:** `17 β 1 + 7 β 8`
+
+Return _the resulting integer after performing the operations described above_.
+
+**Example 1:**
+
+- **Input:** s = "iiii", k = 1
+- **Output:** 36
+- **Explanation:** The operations are as follows:
+ - Convert: "iiii" β "(9)(9)(9)(9)" β "9999" β 9999
+ - Transform #1: 9999 β 9 + 9 + 9 + 9 β 36
+ - Thus the resulting integer is 36.
+
+**Example 2:**
+
+- **Input:** s = "leetcode", k = 2
+- **Output:** 6
+- **Explanation:** The operations are as follows:
+ - Convert: "leetcode" β "(12)(5)(5)(20)(3)(15)(4)(5)" β "12552031545" β 12552031545
+ - Transform #1: 12552031545 β 1 + 2 + 5 + 5 + 2 + 0 + 3 + 1 + 5 + 4 + 5 β 33
+ - Transform #2: 33 β 3 + 3 β 6
+ - Thus the resulting integer is 6.
+
+
+**Example 3:**
+
+- **Input:** s = "zbax", k = 2
+- **Output:** 8
+
+
+
+**Constraints:**
+
+- 1 <= s.length <= 100
+- 1 <= k <= 10
+- s
consists of lowercase English letters.
+
+
+**Hint:**
+1. First, let's note that after the first transform the value will be at most `100 * 10` which is not much
+2. After The first transform, we can just do the rest of the transforms by brute force
+
+
+
+**Solution:**
+
+We can break down the solution into two main steps:
+
+1. **Convert the string `s` into an integer**:
+ - Each character in the string is replaced with its corresponding position in the alphabet (e.g., 'a' -> 1, 'b' -> 2, ..., 'z' -> 26).
+ - Concatenate all these numbers to form a large integer.
+
+2. **Transform the integer by summing its digits `k` times**:
+ - For each transformation, sum all the digits of the current number.
+ - Repeat this transformation process `k` times.
+
+Let's implement this solution in PHP: **[1945. Sum of Digits of String After Convert](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/001945-sum-of-digits-of-string-after-convert/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+1. **Convert the String:**
+ - We loop through each character in the string `s` and calculate its corresponding alphabet position using `ord($s[$i]) - ord('a') + 1`.
+ - These values are concatenated to form a large string `numStr` representing the number.
+
+2. **Transform the Number:**
+ - We loop `k` times, each time summing the digits of the current `numStr`.
+ - The result of this summing operation is stored back in `numStr` as a string to allow for further transformations.
+ - After `k` transformations, we return the final integer value.
+
+### Test Cases:
+
+- `"iiii"` with `k = 1` converts to `"9999"`, sums to `36`, and since `k=1`, the final result is `36`.
+- `"leetcode"` with `k = 2` converts to `"12552031545"`, sums to `33` in the first transform, and to `6` in the second transform, resulting in `6`.
+- `"zbax"` with `k = 2` converts to `"262124"`, sums to `17` in the first transform, and to `8` in the second transform, resulting in `8`.
+
+This solution is efficient given the constraints and will work well within the provided limits.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
diff --git a/algorithms/001945-sum-of-digits-of-string-after-convert/solution.php b/algorithms/001945-sum-of-digits-of-string-after-convert/solution.php
new file mode 100644
index 000000000..450fe9328
--- /dev/null
+++ b/algorithms/001945-sum-of-digits-of-string-after-convert/solution.php
@@ -0,0 +1,28 @@
+1 <= s.length <= 105
+- `s` consists only of lowercase English letters.
+
+
+**Hint:**
+1. What's the optimal way to delete characters if three or more consecutive characters are equal?
+2. If three or more consecutive characters are equal, keep two of them and delete the rest.
+
+
+
+**Solution:**
+
+We need to ensure that no three consecutive characters are the same in the final string. We'll iterate through the input string and build a new "fancy" string by keeping track of the previous two characters. If a third consecutive character matches the last two, we skip it. Otherwise, we add it to the output.
+
+Let's implement this solution in PHP: **[1957. Delete Characters to Make Fancy String](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/001957-delete-characters-to-make-fancy-string/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+1. **Initialize Variables**:
+ - `$result`: This will store the final "fancy" string.
+
+2. **Iterate through the String**:
+ - For each character, check if it forms a trio with the last two characters in the result.
+ - If it does, skip adding it to `$result`.
+ - If not, add it to `$result`.
+
+3. **Return the Result**:
+ - The `$result` string now contains no three consecutive identical characters.
+
+### Complexity Analysis
+
+- **Time Complexity**: _**O(n)**_, where _**n**_ is the length of the input string, as we process each character once.
+- **Space Complexity**: _**O(n)**_, for storing the output string.
+
+This solution meets the constraints efficiently and ensures that the final string has no three consecutive identical characters.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
\ No newline at end of file
diff --git a/algorithms/001957-delete-characters-to-make-fancy-string/solution.php b/algorithms/001957-delete-characters-to-make-fancy-string/solution.php
new file mode 100644
index 000000000..32485168f
--- /dev/null
+++ b/algorithms/001957-delete-characters-to-make-fancy-string/solution.php
@@ -0,0 +1,24 @@
+ 1 && $s[$i] == $result[strlen($result) - 1] && $s[$i] == $result[strlen($result) - 2]) {
+ continue; // Skip adding the character if it makes three consecutive
+ }
+
+ $result .= $s[$i]; // Add character to result
+ }
+
+ return $result;
+ }
+}
\ No newline at end of file
diff --git a/algorithms/001963-minimum-number-of-swaps-to-make-the-string-balanced/README.md b/algorithms/001963-minimum-number-of-swaps-to-make-the-string-balanced/README.md
new file mode 100644
index 000000000..d3a5184a2
--- /dev/null
+++ b/algorithms/001963-minimum-number-of-swaps-to-make-the-string-balanced/README.md
@@ -0,0 +1,129 @@
+1963\. Minimum Number of Swaps to Make the String Balanced
+
+**Difficulty:** Medium
+
+**Topics:** `Two Pointers`, `String`, `Stack`, `Greedy`
+
+You are given a **0-indexed** string `s` of **even** length `n`. The string consists of **exactly** `n / 2` opening brackets `'['` and `n / 2` closing brackets `']'`.
+
+A string is called **balanced** if and only if:
+
+- It is the empty string, or
+- It can be written as `AB`, where both `A` and `B` are **balanced** strings, or
+- It can be written as `[C]`, where `C` is a **balanced** string.
+
+You may swap the brackets at **any** two indices **any** number of times.
+
+Return _the **minimum** number of swaps to make `s` **balanced**_.
+
+**Example 1:**
+
+- **Input:** s = "][]["
+- **Output:** 1
+- **Explanation:** You can make the string balanced by swapping index 0 with index 3.\
+ The resulting string is "[[]]".
+
+**Example 2:**
+
+- **Input:** s = "]]][[["
+- **Output:** 2
+- **Explanation:** You can do the following to make the string balanced:
+ - Swap index 0 with index 4. s = "[]][][".
+ - Swap index 1 with index 5. s = "[[][]]".\
+ The resulting string is "[[][]]".
+
+
+**Example 3:**
+
+- **Input:** s = "[]"
+- **Output:** 0
+- **Explanation:** The string is already balanced.
+
+
+**Constraints:**
+
+- `n == s.length`
+- 2 <= n <= 106
+- `n` is even.
+- `s[i]` is either `'['` or `']'`.
+- The number of opening brackets `'['` equals `n / 2`, and the number of closing brackets `']'` equals `n / 2`.
+
+
+**Hint:**
+1. Iterate over the string and keep track of the number of opening and closing brackets on each step.
+2. If the number of closing brackets is ever larger, you need to make a swap.
+3. Swap it with the opening bracket closest to the end of s.
+
+
+
+**Solution:**
+
+We can use a **two-pointers** approach, which is efficient given the constraints.
+
+### Approach:
+1. **Track Balance**: As we iterate through the string, we can track the balance between opening and closing brackets:
+ - Increment the balance when encountering `'['`.
+ - Decrement the balance when encountering `']'`.
+
+2. **Identify Imbalance**: When the balance becomes negative, it indicates that there are more closing brackets `']'` than opening brackets `'['` at that point in the string. This is where we need to swap brackets to make the string balanced.
+
+3. **Count Swaps**: To correct an imbalance:
+ - Keep a counter `max_imbalance` to track the maximum imbalance observed during the iteration.
+ - The required number of swaps is equal to `(max_imbalance + 1) / 2`, which effectively gives the minimum number of swaps needed to make the string balanced.
+
+Let's implement this solution in PHP: **[1963. Minimum Number of Swaps to Make the String Balanced](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/001963-minimum-number-of-swaps-to-make-the-string-balanced/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+1. **Balance Tracking**:
+ - `balance` keeps track of the difference between the number of `'['` and `']'`.
+ - If `balance` becomes negative, it indicates that there are more `']'` than `'['` at that point.
+
+2. **Calculate Maximum Imbalance**:
+ - `max_imbalance` stores the largest imbalance encountered during the iteration.
+ - If `balance` becomes negative, update `max_imbalance` with the larger of `max_imbalance` or `-balance`.
+
+3. **Calculate Swaps**:
+ - To fix an imbalance, the minimum swaps required is `(max_imbalance + 1) / 2`.
+
+### Time Complexity:
+- The time complexity is **O(n)**, where `n` is the length of the string. We iterate through the string once to determine the `max_imbalance`.
+- The space complexity is **O(1)** as we use a few variables for tracking the balance and maximum imbalance.
+
+This solution ensures that we meet the constraints, even for large inputs.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
\ No newline at end of file
diff --git a/algorithms/001963-minimum-number-of-swaps-to-make-the-string-balanced/solution.php b/algorithms/001963-minimum-number-of-swaps-to-make-the-string-balanced/solution.php
new file mode 100644
index 000000000..8770ccd0e
--- /dev/null
+++ b/algorithms/001963-minimum-number-of-swaps-to-make-the-string-balanced/solution.php
@@ -0,0 +1,30 @@
+-105 <= matrix[i][j] <= 105
+
+**Hint:**
+1. Try to use the operation so that each row has only one negative number.
+2. If you have only one negative element you cannot convert it to positive.
+
+
+
+**Solution:**
+
+To maximize the matrix's sum using the operation, we need to minimize the absolute value of the negative contributions to the sum. Here's the plan:
+
+1. **Count Negative Numbers:** Track how many negative numbers are present in the matrix.
+2. **Find Minimum Absolute Value:** Determine the smallest absolute value in the matrix, which will help if the number of negatives is odd.
+3. **Adjust for Odd Negatives:** If the count of negative numbers is odd, the smallest absolute value cannot be flipped to positive, and this limits the maximum possible sum.
+
+Let's implement this solution in PHP: **[1975. Maximum Matrix Sum](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/001975-maximum-matrix-sum/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+1. **Summation of Absolute Values:** Compute the sum of absolute values of all elements since the optimal configuration flips as many negative numbers to positive as possible.
+2. **Track Smallest Absolute Value:** Use this to adjust the sum when the count of negative numbers is odd.
+3. **Handle Odd Negatives:** Subtract twice the smallest absolute value from the sum to account for the unavoidable negative element when the negatives cannot be fully neutralized.
+
+### Complexity
+- **Time Complexity:** _**O(n^2)**_, as the matrix is traversed once.
+- **Space Complexity:** _**O(1)**_, since no additional space is used apart from variables.
+
+This solution works efficiently within the given constraints.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
diff --git a/algorithms/001975-maximum-matrix-sum/solution.php b/algorithms/001975-maximum-matrix-sum/solution.php
new file mode 100644
index 000000000..ebea37884
--- /dev/null
+++ b/algorithms/001975-maximum-matrix-sum/solution.php
@@ -0,0 +1,33 @@
+1 <= original.length <= 5 * 104
+- 1 <= original[i] <= 105
+- 1 <= m, n <= 4 * 104
+
+
+**Hint:**
+1. When is it possible to convert original into a 2D array and when is it impossible?
+2. It is possible if and only if m * n == original.length
+3. If it is possible to convert original to a 2D array, keep an index i such that original[i] is the next element to add to the 2D array.
+
+
+
+**Solution:**
+
+We need to follow these steps:
+
+1. **Check if Conversion is Possible**: The conversion from a 1D array to a 2D array is only possible if the total number of elements in the 1D array (`original.length`) is exactly equal to `m * n`, where `m` is the number of rows and `n` is the number of columns. If this condition is not met, return an empty array.
+
+2. **Create the 2D Array**: If the conversion is possible, initialize a 2D array with `m` rows and `n` columns, and populate it by iterating over the 1D array and filling in the 2D array row by row.
+
+Let's implement this solution in PHP: **[2022. Convert 1D Array Into 2D Array](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/002022-convert-1d-array-into-2d-array/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+- **Input Validation**:
+ - We first calculate the length of the original array.
+ - If the length does not equal `m * n`, the conversion is impossible, and we return an empty array.
+
+- **2D Array Construction**:
+ - We initialize a 2D array named `$result`.
+ - We use a nested loop where the outer loop runs `m` times (for each row) and the inner loop runs `n` times (for each column in a row).
+ - We maintain an index `$index` that tracks our position in the original array, incrementing it as we place elements into the 2D array.
+
+### Example Output:
+
+For the provided example:
+
+```php
+$original = array(1, 2, 3, 4);
+$m = 2;
+$n = 2;
+print_r(construct2DArray($original, $m, $n));
+```
+
+The output will be:
+
+```php
+Array
+(
+ [0] => Array
+ (
+ [0] => 1
+ [1] => 2
+ )
+
+ [1] => Array
+ (
+ [0] => 3
+ [1] => 4
+ )
+)
+```
+
+This approach ensures that the 1D array is correctly converted into the desired 2D array, and it efficiently handles edge cases by checking whether the conversion is possible.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
diff --git a/algorithms/002022-convert-1d-array-into-2d-array/solution.php b/algorithms/002022-convert-1d-array-into-2d-array/solution.php
new file mode 100644
index 000000000..bd9e4b451
--- /dev/null
+++ b/algorithms/002022-convert-1d-array-into-2d-array/solution.php
@@ -0,0 +1,34 @@
+ith observation. You are also given the two integers `mean` and `n`.
+
+Return _an array of length `n` containing the missing observations such that the average value of the `n + m` rolls is exactly `mean`_. If there are multiple valid answers, return any of them. If no such array exists, return _an empty array_.
+
+The **average value** of a set of `k` numbers is the sum of the numbers divided by `k`.
+
+**Note** that `mean` is an integer, so the sum of the `n + m `rolls should be divisible by `n + m`.
+
+**Example 1:**
+
+- **Input:** rolls = [3,2,4,3], mean = 4, n = 2
+- **Output:** [6,6]
+- **Explanation:** The mean of all n + m rolls is (3 + 2 + 4 + 3 + 6 + 6) / 6 = 4.
+
+**Example 2:**
+
+- **Input:** rolls = [1,5,6], mean = 3, n = 4
+- **Output:** [2,3,2,2]
+- **Explanation:** The mean of all n + m rolls is (1 + 5 + 6 + 2 + 3 + 2 + 2) / 7 = 3.
+
+**Example 3:**
+
+- **Input:** rolls = [1,2,3,4], mean = 6, n = 4
+- **Output:** []
+- **Explanation:** It is impossible for the mean to be 6 no matter what the 4 missing rolls are.
+
+
+
+**Constraints:**
+
+- m == rolls.length
+- 1 <= n, m <= 105
+- 1 <= rolls[i], mean <= 6
+
+
+**Hint:**
+1. What should the sum of the n rolls be?
+2. Could you generate an array of size n such that each element is between 1 and 6?
+
+
+
+**Solution:**
+
+We need to determine an array of missing rolls such that the average of all `n + m` dice rolls is exactly equal to `mean`. Here's the step-by-step breakdown of the solution:
+
+### Steps to Approach:
+
+1. **Calculate the total sum for `n + m` rolls:**
+ Given that the average value of `n + m` rolls is `mean`, the total sum of all the rolls should be `total_sum = (n + m) * mean`.
+
+2. **Determine the missing sum:**
+ The sum of the `m` rolls is already known. Thus, the sum of the missing `n` rolls should be:
+ ```
+ missing_sum = total_sum - β(rolls)
+ ```
+ where `β(rolls)` is the sum of the elements in the `rolls` array.
+
+3. **Check for feasibility:**
+ Each roll is a 6-sided die, so the missing values must be between 1 and 6 (inclusive). Therefore, the sum of the missing `n` rolls must be between:
+ ```
+ min_sum = n X 1 = n
+ ```
+ and
+ ```
+ max_sum = n X 6 = 6n
+ ```
+ If the `missing_sum` is outside this range, it's impossible to form valid missing observations, and we should return an empty array.
+
+4. **Distribute the missing sum:**
+ If `missing_sum` is valid, we distribute it across the `n` rolls by initially filling each element with `1` (the minimum possible value). Then, we increment elements from 1 to 6 until we reach the required `missing_sum`.
+
+Let's implement this solution in PHP: **[2028. Find Missing Observations](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/002028-find-missing-observations/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+1. **Input:**
+ - `rolls = [3, 2, 4, 3]`
+ - `mean = 4`
+ - `n = 2`
+
+2. **Steps:**
+ - The total number of rolls is `n + m = 6`.
+ - The total sum needed is `6 * 4 = 24`.
+ - The sum of the given rolls is `3 + 2 + 4 + 3 = 12`.
+ - The sum required for the missing rolls is `24 - 12 = 12`.
+
+ We need two missing rolls that sum up to 12, and the only possibility is `[6, 6]`.
+
+3. **Result:**
+ - For example 1: The output is `[6, 6]`.
+ - For example 2: The output is `[2, 3, 2, 2]`.
+ - For example 3: No valid solution, so the output is `[]`.
+
+### Time Complexity:
+- Calculating the sum of `rolls` takes O(m), and distributing the `missing_sum` takes O(n). Hence, the overall time complexity is **O(n + m)**, which is efficient for the input constraints.
+
+This solution ensures that we either find valid missing rolls or return an empty array when no solution exists.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
diff --git a/algorithms/002028-find-missing-observations/solution.php b/algorithms/002028-find-missing-observations/solution.php
new file mode 100644
index 000000000..3d6aacf29
--- /dev/null
+++ b/algorithms/002028-find-missing-observations/solution.php
@@ -0,0 +1,41 @@
+ 6 * $n) {
+ return []; // Impossible to find valid missing rolls
+ }
+
+ // Initialize the result array with the minimum value 1
+ $result = array_fill(0, $n, 1);
+ $remaining_sum = $missing_sum - $n; // Already allocated n * 1 = n, so subtract this
+
+ // Distribute the remaining sum to the rolls
+ for ($i = 0; $i < $n && $remaining_sum > 0; $i++) {
+ $add = min(5, $remaining_sum); // Add at most 5 to keep the value <= 6
+ $result[$i] += $add;
+ $remaining_sum -= $add;
+ }
+
+ return $result;
+
+ }
+}
\ No newline at end of file
diff --git a/algorithms/002044-count-number-of-maximum-bitwise-or-subsets/README.md b/algorithms/002044-count-number-of-maximum-bitwise-or-subsets/README.md
new file mode 100644
index 000000000..e315623ca
--- /dev/null
+++ b/algorithms/002044-count-number-of-maximum-bitwise-or-subsets/README.md
@@ -0,0 +1,113 @@
+2044\. Count Number of Maximum Bitwise-OR Subsets
+
+**Difficulty:** Medium
+
+**Topics:** `Array`, `Backtracking`, `Bit Manipulation`, `Enumeration`
+
+Given an integer array `nums`, find the **maximum** possible **bitwise OR** of a subset of `nums` and return _the **number of different non-empty subsets** with the maximum bitwise OR_.
+
+An array `a` is a **subset** of an array `b` if `a` can be obtained from `b` by deleting some (possibly zero) elements of `b`. Two subsets are considered **different** if the indices of the elements chosen are different.
+
+The bitwise OR of an array `a` is equal to `a[0] OR a[1] OR ... OR a[a.length - 1]` (**0-indexed**).
+
+**Example 1:**
+
+- **Input:** nums = [3,1]
+- **Output:** 2
+- **Explanation:** The maximum possible bitwise OR of a subset is 3. There are 2 subsets with a bitwise OR of 3:
+ - [3]
+ - [3,1]
+
+**Example 2:**
+
+- **Input:** nums = [2,2,2]
+- **Output:** 7
+- **Explanation:** All non-empty subsets of [2,2,2] have a bitwise OR of 2. There are 23 - 1 = 7 total subsets.
+
+
+**Example 3:**
+
+- **Input:** nums = [3,2,1,5]
+- **Output:** 6
+- **Explanation:** The maximum possible bitwise OR of a subset is 7. There are 6 subsets with a bitwise OR of 7:
+ - [3,5]
+ - [3,1,5]
+ - [3,2,5]
+ - [3,2,1,5]
+ - [2,5]
+ - [2,1,5]
+
+
+
+**Constraints:**
+
+- `1 <= nums.length <= 16`
+- 1 <= nums[i] <= 105
+
+
+**Hint:**
+1. Can we enumerate all possible subsets?
+2. The maximum bitwise-OR is the bitwise-OR of the whole array.
+
+
+
+**Solution:**
+
+We can follow these steps:
+
+1. **Calculate the Maximum Bitwise OR**: The maximum bitwise OR of a subset can be determined by performing a bitwise OR operation across all elements of the array. This gives us the maximum possible bitwise OR.
+
+2. **Enumerate All Subsets**: Since the size of the array is small (up to 16), we can enumerate all possible subsets using a bit manipulation technique. For an array of size `n`, there are `2^n` possible subsets.
+
+3. **Count Valid Subsets**: For each subset, compute its bitwise OR and check if it matches the maximum bitwise OR. If it does, increment a counter.
+
+Let's implement this solution in PHP: **[2044. Count Number of Maximum Bitwise-OR Subsets](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/002044-count-number-of-maximum-bitwise-or-subsets/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+1. **Maximum Bitwise OR Calculation**:
+ - We use a loop to calculate the maximum bitwise OR of the array by performing a bitwise OR on each element.
+
+2. **Subset Enumeration**:
+ - We loop through all numbers from `1` to `2^n - 1` (where `n` is the length of `nums`), representing all non-empty subsets.
+ - For each number, we check each bit to see which elements are included in the subset.
+
+3. **Valid Subset Count**:
+ - After calculating the bitwise OR for the current subset, we check if it equals `maxOR`. If it does, we increment our counter.
+
+This solution is efficient given the constraints and should work well for arrays of size up to 16, resulting in at most 65,535 subsets to evaluate.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
diff --git a/algorithms/002044-count-number-of-maximum-bitwise-or-subsets/solution.php b/algorithms/002044-count-number-of-maximum-bitwise-or-subsets/solution.php
new file mode 100644
index 000000000..89a77c459
--- /dev/null
+++ b/algorithms/002044-count-number-of-maximum-bitwise-or-subsets/solution.php
@@ -0,0 +1,38 @@
+events[i] = [startTimei, endTimei, valuei]. The ith
event starts at startTimei
and ends at endTimei
, and if you attend this event, you will receive a value of valuei
. You can choose **at most two non-overlapping** events to attend such that the sum of their values is **maximized**.
+
+Return this **maximum** sum.
+
+Note that the start time and end time is **inclusive**: that is, you cannot attend two events where one of them starts and the other ends at the same time. More specifically, if you attend an event with end time `t`, the next event must start at or after `t + 1`.
+
+**Example 1:**
+
+
+
+- **Input:** events = [[1,3,2],[4,5,2],[2,4,3]]
+- **Output:** 4
+- **Explanation:** Choose the green events, 0 and 1 for a sum of 2 + 2 = 4.
+
+**Example 2:**
+
+
+
+- **Input:** events = [[1,3,2],[4,5,2],[1,5,5]]
+- **Output:** 5
+- **Explanation:** Choose event 2 for a sum of 5.
+
+
+**Example 3:**
+
+
+
+- **Input:** events = [[1,5,3],[1,5,1],[6,6,5]]
+- **Output:** 8
+- **Explanation:** Choose events 0 and 2 for a sum of 3 + 5 = 8.
+
+
+
+**Constraints:**
+
+- 2 <= events.length <= 105
+- `events[i].length == 3`
+- 1 <= startTimei <= endTimei <= 109
+- 1 <= valuei <= 106
+
+
+**Hint:**
+1. How can sorting the events on the basis of their start times help? How about end times?
+2. How can we quickly get the maximum score of an interval not intersecting with the interval we chose?
+
+
+
+**Solution:**
+
+We can use the following approach:
+
+### Approach
+1. **Sort Events by End Time**:
+ - Sorting helps us efficiently find non-overlapping events using binary search.
+
+2. **Binary Search for Non-Overlapping Events**:
+ - Use binary search to find the latest event that ends before the current event's start time. This ensures non-overlapping.
+
+3. **Dynamic Programming with Max Tracking**:
+ - While iterating through the sorted events, maintain the maximum value of events up to the current one. This allows us to quickly compute the maximum sum of two events.
+
+4. **Iterate and Calculate the Maximum Sum**:
+ - For each event, calculate the possible sum using:
+ - Only the current event.
+ - The current event combined with the best non-overlapping event found using binary search.
+
+Let's implement this solution in PHP: **[2054. Two Best Non-Overlapping Events](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/002054-two-best-non-overlapping-events/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+1. **Sorting**:
+ - The events are sorted by their end time, which allows for efficient searching of the last non-overlapping event.
+
+2. **Binary Search**:
+ - For each event, binary search determines the latest event that ends before the current event starts.
+
+3. **Max Tracking**:
+ - We maintain an array `maxUpTo`, which stores the maximum value of events up to the current index. This avoids recalculating the maximum for earlier indices.
+
+4. **Max Sum Calculation**:
+ - For each event, calculate the sum of its value and the best non-overlapping event's value. Update the global maximum sum accordingly.
+
+### Complexity Analysis
+- **Sorting**: _**O(n log n)**_
+- **Binary Search for Each Event**: _**O(log n)**_, repeated _**n**_ times β _**O(n log n)**_
+- **Overall**: _**O(n log n)**_
+
+This solution is efficient and works well within the constraints.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
diff --git a/algorithms/002054-two-best-non-overlapping-events/solution.php b/algorithms/002054-two-best-non-overlapping-events/solution.php
new file mode 100644
index 000000000..6ac9a35bb
--- /dev/null
+++ b/algorithms/002054-two-best-non-overlapping-events/solution.php
@@ -0,0 +1,60 @@
+ $event) {
+ $startTime = $event[0];
+ $endTime = $event[1];
+ $value = $event[2];
+
+ // Use binary search to find the latest non-overlapping event
+ $left = 0;
+ $right = $i - 1;
+ $bestIndex = -1;
+
+ while ($left <= $right) {
+ $mid = intval(($left + $right) / 2);
+ if ($endTimes[$mid] < $startTime) {
+ $bestIndex = $mid; // Potential candidate
+ $left = $mid + 1;
+ } else {
+ $right = $mid - 1;
+ }
+ }
+
+ // If a non-overlapping event is found, use its maximum value
+ $currentSum = $value;
+ if ($bestIndex != -1) {
+ $currentSum += $maxUpTo[$bestIndex];
+ }
+
+ // Update the maximum sum found so far
+ $maxSum = max($maxSum, $currentSum);
+
+ // Update the max value up to the current index
+ $maxValueSoFar = max($maxValueSoFar, $value);
+ $maxUpTo[$i] = $maxValueSoFar;
+ }
+
+ return $maxSum;
+ }
+}
\ No newline at end of file
diff --git a/algorithms/002064-minimized-maximum-of-products-distributed-to-any-store/README.md b/algorithms/002064-minimized-maximum-of-products-distributed-to-any-store/README.md
new file mode 100644
index 000000000..18b1043d9
--- /dev/null
+++ b/algorithms/002064-minimized-maximum-of-products-distributed-to-any-store/README.md
@@ -0,0 +1,150 @@
+2064\. Minimized Maximum of Products Distributed to Any Store
+
+**Difficulty:** Medium
+
+**Topics:** `Array`, `Binary Search`
+
+You are given an integer `n` indicating there are `n` specialty retail stores. There are `m` product types of varying amounts, which are given as a **0-indexed** integer array `quantities`, where `quantities[i]` represents the number of products of the ith
product type.
+
+You need to distribute **all products** to the retail stores following these rules:
+
+- A store can only be given **at most one product type** but can be given any amount of it.
+- After distribution, each store will have been given some number of products (possibly `0`). Let `x` represent the maximum number of products given to any store. You want `x` to be as small as possible, i.e., you want to **minimize** the **maximum** number of products that are given to any store.
+
+Return _the minimum possible `x`_.
+
+**Example 1:**
+
+- **Input:** n = 6, quantities = [11,6]
+- **Output:** 3
+- **Explanation:** One optimal way is:
+ - The 11 products of type 0 are distributed to the first four stores in these amounts: 2, 3, 3, 3
+ - The 6 products of type 1 are distributed to the other two stores in these amounts: 3, 3
+ The maximum number of products given to any store is max(2, 3, 3, 3, 3, 3) = 3.
+
+**Example 2:**
+
+- **Input:** n = 7, quantities = [15,10,10]
+- **Output:** 5
+- **Explanation:** One optimal way is:
+ - The 15 products of type 0 are distributed to the first three stores in these amounts: 5, 5, 5
+ - The 10 products of type 1 are distributed to the next two stores in these amounts: 5, 5
+ - The 10 products of type 2 are distributed to the last two stores in these amounts: 5, 5
+ The maximum number of products given to any store is max(5, 5, 5, 5, 5, 5, 5) = 5.
+
+
+**Example 3:**
+
+- **Input:** n = 1, quantities = [100000]
+- **Output:** 100000
+- **Explanation:** The only optimal way is:
+ - The 100000 products of type 0 are distributed to the only store.
+ The maximum number of products given to any store is max(100000) = 100000.
+
+
+
+**Constraints:**
+
+- `m == quantities.length`
+- 1 <= m <= n <= 105
+- 1 <= quantities[i] <= 105
+
+
+**Hint:**
+1. There exists a monotonic nature such that when x is smaller than some number, there will be no way to distribute, and when x is not smaller than that number, there will always be a way to distribute.
+2. If you are given a number k, where the number of products given to any store does not exceed k, could you determine if all products can be distributed?
+3. Implement a function canDistribute(k), which returns true if you can distribute all products such that any store will not be given more than k products, and returns false if you cannot. Use this function to binary search for the smallest possible k.
+
+
+
+**Solution:**
+
+We can use a binary search on the maximum possible number of products assigned to any store (`x`). Hereβs a step-by-step explanation and the PHP solution:
+
+### Approach
+
+1. **Binary Search Setup**:
+ - Set the lower bound (`left`) as 1 (since each store can get at least 1 product).
+ - Set the upper bound (`right`) as the maximum quantity in `quantities` array (in the worst case, one store gets all products of a type).
+ - Our goal is to minimize the value of `x` (maximum products given to any store).
+
+2. **Binary Search Logic**:
+ - For each mid-point `x`, check if itβs feasible to distribute all products such that no store has more than `x` products.
+ - Use a helper function `canDistribute(x)` to determine feasibility.
+
+3. **Feasibility Check (canDistribute)**:
+ - For each product type in `quantities`, calculate the minimum number of stores needed to distribute that product type without exceeding `x` products per store.
+ - Sum the required stores for all product types.
+ - If the total required stores is less than or equal to `n`, the distribution is possible with `x` as the maximum load per store; otherwise, it is not feasible.
+
+4. **Binary Search Adjustment**:
+ - If `canDistribute(x)` returns `true`, it means `x` is a feasible solution, but we want to minimize `x`, so adjust the `right` bound.
+ - If it returns `false`, increase the `left` bound since `x` is too small.
+
+5. **Result**:
+ - Once the binary search completes, `left` will hold the minimum possible `x`.
+
+Let's implement this solution in PHP: **[2064. Minimized Maximum of Products Distributed to Any Store](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/002064-minimized-maximum-of-products-distributed-to-any-store/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+1. **`canDistribute` function**:
+ - For each `quantity`, it calculates the minimum stores required by dividing the `quantity` by `x` (using `ceil` to round up since each store can get a whole number of products).
+ - It returns `false` if the cumulative required stores exceed `n`.
+
+2. **Binary Search on `x`**:
+ - The binary search iteratively reduces the range for `x` until it converges on the minimal feasible value.
+
+3. **Efficiency**:
+ - This solution is efficient for large input sizes (`n` and `m` up to `10^5`) because binary search runs in `O(log(max_quantity) * m)`, which is feasible within the given constraints.
+
+This approach minimizes `x`, ensuring the products are distributed as evenly as possible across the stores.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
diff --git a/algorithms/002064-minimized-maximum-of-products-distributed-to-any-store/solution.php b/algorithms/002064-minimized-maximum-of-products-distributed-to-any-store/solution.php
new file mode 100644
index 000000000..0724b4f34
--- /dev/null
+++ b/algorithms/002064-minimized-maximum-of-products-distributed-to-any-store/solution.php
@@ -0,0 +1,52 @@
+canDistribute($mid, $quantities, $n)) {
+ // If possible to distribute with `mid` as the maximum, try smaller values
+ $right = $mid;
+ } else {
+ // Otherwise, increase `mid`
+ $left = $mid + 1;
+ }
+ }
+
+ return $left;
+ }
+
+ /**
+ * Helper function to check if we can distribute products with maximum `x` per store
+ *
+ * @param $x
+ * @param $quantities
+ * @param $n
+ * @return bool
+ */
+ function canDistribute($x, $quantities, $n) {
+ $requiredStores = 0;
+
+ foreach ($quantities as $quantity) {
+ // Calculate minimum stores needed for this product type
+ $requiredStores += ceil($quantity / $x);
+ // If we need more stores than available, return false
+ if ($requiredStores > $n) {
+ return false;
+ }
+ }
+
+ return $requiredStores <= $n;
+ }
+}
\ No newline at end of file
diff --git a/algorithms/002070-most-beautiful-item-for-each-query/README.md b/algorithms/002070-most-beautiful-item-for-each-query/README.md
new file mode 100644
index 000000000..1c5408948
--- /dev/null
+++ b/algorithms/002070-most-beautiful-item-for-each-query/README.md
@@ -0,0 +1,125 @@
+2070\. Most Beautiful Item for Each Query
+
+**Difficulty:** Medium
+
+**Topics:** `Array`, `Binary Search`, `Sorting`
+
+You are given a 2D integer array `items` where items[i] = [pricei, beautyi]
denotes the **price** and **beauty** of an item respectively.
+
+You are also given a **0-indexed** integer array `queries`. For each `queries[j]`, you want to determine the **maximum beauty** of an item whose **price** is **less than or equal** to `queries[j]`. If no such item exists, then the answer to this query is `0`.
+
+Return _an array `answer` of the same length as `queries` where `answer[j]` is the answer to the j
th query_.
+
+**Example 1:**
+
+- **Input:** items = [[1,2],[3,2],[2,4],[5,6],[3,5]], queries = [1,2,3,4,5,6]
+- **Output:** [2,4,5,5,6,6]
+- **Explanation:**
+ - For queries[0]=1, [1,2] is the only item which has price <= 1. Hence, the answer for this query is 2.
+ - For queries[1]=2, the items which can be considered are [1,2] and [2,4].
+ - The maximum beauty among them is 4.
+ - For queries[2]=3 and queries[3]=4, the items which can be considered are [1,2], [3,2], [2,4], and [3,5].
+ - The maximum beauty among them is 5.
+ - For queries[4]=5 and queries[5]=6, all items can be considered.
+ - Hence, the answer for them is the maximum beauty of all items, i.e., 6.
+
+**Example 2:**
+
+- **Input:** items = [[1,2],[1,2],[1,3],[1,4]], queries = [1]
+- **Output:** [4]
+- **Explanation:** The price of every item is equal to 1, so we choose the item with the maximum beauty 4.
+ - Note that multiple items can have the same price and/or beauty.
+
+
+**Example 3:**
+
+- **Input:** items = [[10,1000]], queries = [5]
+- **Output:** [0]
+- **Explanation:** No item has a price less than or equal to 5, so no item can be chosen.
+ - Hence, the answer to the query is 0.
+
+
+
+**Constraints:**
+
+- 1 <= items.length, queries.length <= 105
+- `items[i].length == 2`
+- 1 <= pricei, beautyi, queries[j] <= 109
+
+
+**Hint:**
+1. Can we process the queries in a smart order to avoid repeatedly checking the same items?
+2. How can we use the answer to a query for other queries?
+
+
+
+**Solution:**
+
+We can use sorting and binary search techniques. Hereβs the plan:
+
+### Approach
+
+1. **Sort the Items by Price**:
+ - First, sort `items` by their `price`. This way, as we iterate through the items, we can keep track of the maximum beauty seen so far for items up to any given price.
+
+2. **Sort the Queries with their Original Indices**:
+ - Create an array of queries paired with their original indices, then sort this array by the query values.
+ - Sorting helps because we can process queries in increasing order of `price` and avoid recalculating beauty values for lower prices repeatedly.
+
+3. **Iterate through Items and Queries Simultaneously**:
+ - Using two pointers, process each query:
+ - For each query, move the pointer through items with a price less than or equal to the queryβs price.
+ - Track the maximum beauty as you go through these items, and use this value to answer the current query.
+ - This avoids repeatedly checking items for multiple queries.
+
+4. **Store and Return Results**:
+ - Once processed, store the maximum beauty result for each query based on the original index to maintain the order.
+ - Return the `answer` array.
+
+Let's implement this solution in PHP: **[2070. Most Beautiful Item for Each Query](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/002070-most-beautiful-item-for-each-query/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+- **Sorting `items` and `queries`**: This allows efficient processing without redundant calculations.
+- **Two-pointer technique**: Moving through `items` only once for each query avoids excessive computations.
+- **Track `maxBeauty`**: We update `maxBeauty` progressively, allowing each query to access the highest beauty seen so far.
+
+### Complexity
+
+- **Time Complexity**: _**O(n log n + m log m)**_ for sorting `items` and `queries`, and _**O(n + m)**_ for processing, where _**n**_ is the length of `items` and _**m**_ is the length of `queries`.
+- **Space Complexity**: _**O(m)**_ for storing the results.
+
+This solution is efficient and meets the constraints of the problem.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
diff --git a/algorithms/002070-most-beautiful-item-for-each-query/solution.php b/algorithms/002070-most-beautiful-item-for-each-query/solution.php
new file mode 100644
index 000000000..bcf1df199
--- /dev/null
+++ b/algorithms/002070-most-beautiful-item-for-each-query/solution.php
@@ -0,0 +1,46 @@
+ $query) {
+ $indexedQueries[] = [$query, $index];
+ }
+ // Sort queries by price
+ usort($indexedQueries, function($a, $b) {
+ return $a[0] - $b[0];
+ });
+
+ $maxBeauty = 0;
+ $itemIndex = 0;
+ $answer = array_fill(0, count($queries), 0);
+
+ // Process each query
+ foreach ($indexedQueries as $query) {
+ list($queryPrice, $queryIndex) = $query;
+
+ // Move the item pointer to include all items with price <= queryPrice
+ while ($itemIndex < count($items) && $items[$itemIndex][0] <= $queryPrice) {
+ $maxBeauty = max($maxBeauty, $items[$itemIndex][1]);
+ $itemIndex++;
+ }
+
+ // Set the result for this query's original index
+ $answer[$queryIndex] = $maxBeauty;
+ }
+
+ return $answer;
+ }
+}
\ No newline at end of file
diff --git a/algorithms/002097-valid-arrangement-of-pairs/README.md b/algorithms/002097-valid-arrangement-of-pairs/README.md
new file mode 100644
index 000000000..99dc09d0f
--- /dev/null
+++ b/algorithms/002097-valid-arrangement-of-pairs/README.md
@@ -0,0 +1,167 @@
+2097\. Valid Arrangement of Pairs
+
+**Difficulty:** Hard
+
+**Topics:** `Depth-First Search`, `Graph`, `Eulerian Circuit`
+
+You are given a **0-indexed** 2D integer array `pairs` where pairs[i] = [starti, endi]
. An arrangement of `pairs` is **valid** if for every index `i` where `1 <= i < pairs.length`, we have endi-1 == starti
.
+
+Return _**any** valid arrangement of `pairs`_.
+
+**Note**: The inputs will be generated such that there exists a valid arrangement of `pairs`.
+
+**Example 1:**
+
+- **Input:** pairs = [[5,1],[4,5],[11,9],[9,4]]
+- **Output:** [[11,9],[9,4],[4,5],[5,1]]
+- **Explanation:** This is a valid arrangement since endi-1 always equals starti.
+ - end0 = 9 == 9 = start1
+ - end1 = 4 == 4 = start2
+ - end2 = 5 == 5 = start3
+
+**Example 2:**
+
+- **Input:** pairs = [[1,3],[3,2],[2,1]]
+- **Output:** [[1,3],[3,2],[2,1]]
+- **Explanation:** This is a valid arrangement since endi-1 always equals starti.
+ - end0 = 3 == 3 = start1
+ - end1 = 2 == 2 = start2
+ - The arrangements [[2,1],[1,3],[3,2]] and [[3,2],[2,1],[1,3]] are also valid.
+
+
+**Example 3:**
+
+- **Input:** pairs = [[1,2],[1,3],[2,1]]
+- **Output:** [[1,2],[2,1],[1,3]]
+- **Explanation:** This is a valid arrangement since endi-1 always equals starti.
+ - end0 = 2 == 2 = start1
+ - end1 = 1 == 1 = start2
+
+
+
+**Constraints:**
+
+- 1 <= pairs.length <= 105
+- `pairs[i].length == 2`
+- 0 <= starti, endi <= 109
+- starti != endi
+- No two pairs are exactly the same.
+- There **exists** a valid arrangement of `pairs`.
+
+
+**Hint:**
+1. Could you convert this into a graph problem?
+2. Consider the pairs as edges and each number as a node.
+3. We have to find an Eulerian path of this graph. Hierholzerβs algorithm can be used.
+
+
+
+**Solution:**
+
+We can approach it as an Eulerian Path problem in graph theory. In this case, the pairs can be treated as edges, and the values within the pairs (the `start` and `end`) can be treated as nodes. We need to find an Eulerian path, which is a path that uses every edge exactly once, and the end of one edge must match the start of the next edge.
+
+### Key Steps:
+1. **Graph Representation**: Each unique number in the pairs will be a node, and each pair will be an edge from `start[i]` to `end[i]`.
+2. **Eulerian Path Criteria**:
+ - An Eulerian path exists if there are exactly two nodes with odd degrees, and the rest must have even degrees.
+ - We need to make sure that the graph is connected (though this is guaranteed by the problem statement).
+3. **Hierholzer's Algorithm**: This algorithm can be used to find the Eulerian path. It involves:
+ - Starting at a node with an odd degree (if any).
+ - Traversing through the edges, marking them as visited.
+ - If a node is reached with unused edges, continue traversing until all edges are used.
+
+### Plan:
+- Build a graph using a hash map to store the adjacency list (each node and its connected nodes).
+- Track the degree (in-degree and out-degree) of each node.
+- Use Hierholzer's algorithm to find the Eulerian path.
+
+Let's implement this solution in PHP: **[2097. Valid Arrangement of Pairs](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/002097-valid-arrangement-of-pairs/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+1. **Graph Construction**:
+ - We build the graph using an adjacency list where each key is a `start` node, and the value is a list of `end` nodes.
+ - We also maintain the out-degree and in-degree for each node, which will help us find the start node for the Eulerian path.
+
+2. **Finding the Start Node**:
+ - An Eulerian path starts at a node where the out-degree is greater than the in-degree by 1 (if such a node exists).
+ - If no such node exists, the graph is balanced, and we can start at any node.
+
+3. **Hierholzer's Algorithm**:
+ - We start from the `startNode` and repeatedly follow edges, marking them as visited by removing them from the adjacency list.
+ - Once we reach a node with no more outgoing edges, we backtrack and build the result.
+
+4. **Return the Result**:
+ - The result is constructed in reverse order because of the way we backtrack, so we reverse it at the end.
+
+### Example Output:
+```php
+Array
+(
+ [0] => Array
+ (
+ [0] => 11
+ [1] => 9
+ )
+
+ [1] => Array
+ (
+ [0] => 9
+ [1] => 4
+ )
+
+ [2] => Array
+ (
+ [0] => 4
+ [1] => 5
+ )
+
+ [3] => Array
+ (
+ [0] => 5
+ [1] => 1
+ )
+)
+```
+
+### Time Complexity:
+- **Building the graph**: O(n), where n is the number of pairs.
+- **Hierholzer's Algorithm**: O(n), because each edge is visited once.
+- **Overall Time Complexity**: O(n).
+
+This approach efficiently finds a valid arrangement of pairs by treating the problem as an Eulerian path problem in a directed graph.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
diff --git a/algorithms/002097-valid-arrangement-of-pairs/solution.php b/algorithms/002097-valid-arrangement-of-pairs/solution.php
new file mode 100644
index 000000000..51f0d6d45
--- /dev/null
+++ b/algorithms/002097-valid-arrangement-of-pairs/solution.php
@@ -0,0 +1,59 @@
+ $outCount) {
+ $inCount = isset($inDegree[$node]) ? $inDegree[$node] : 0;
+ if ($outCount - $inCount == 1) {
+ $startNode = $node;
+ break;
+ }
+ }
+
+ // If no start node found, use any node from the graph as a start
+ if ($startNode === null) {
+ $startNode = key($graph); // Just get the first key
+ }
+
+ // Hierholzer's algorithm to find the Eulerian path
+ $stack = [];
+ $result = [];
+ $currentNode = $startNode;
+ while (count($stack) > 0 || isset($graph[$currentNode]) && count($graph[$currentNode]) > 0) {
+ if (isset($graph[$currentNode]) && count($graph[$currentNode]) > 0) {
+ // There are still edges to visit from current node
+ $stack[] = $currentNode;
+ $nextNode = array_pop($graph[$currentNode]);
+ $currentNode = $nextNode;
+ } else {
+ // No more edges, add current node to result
+ $result[] = [$stack[count($stack) - 1], $currentNode];
+ $currentNode = array_pop($stack);
+ }
+ }
+
+ // Since we built the path backwards, reverse it
+ return array_reverse($result);
+ }
+}
\ No newline at end of file
diff --git a/algorithms/002109-adding-spaces-to-a-string/README.md b/algorithms/002109-adding-spaces-to-a-string/README.md
new file mode 100644
index 000000000..ab3bc6263
--- /dev/null
+++ b/algorithms/002109-adding-spaces-to-a-string/README.md
@@ -0,0 +1,121 @@
+2109\. Adding Spaces to a String
+
+**Difficulty:** Medium
+
+**Topics:** `Array`, `Two Pointers`, `String`, `Simulation`
+
+You are given a 0-indexed string s and a 0-indexed integer array spaces that describes the indices in the original string where spaces will be added. Each space should be inserted before the character at the given index.
+
+- For example, given `s = "EnjoyYourCoffee"` and `spaces = [5, 9]`, we place spaces before `'Y'` and `'C'`, which are at indices `5` and `9` respectively. Thus, we obtain `"Enjoy Your Coffee"`.
+
+Return _the modified string **after** the spaces have been added_.
+
+**Example 1:**
+
+- **Input:** s = "LeetcodeHelpsMeLearn", spaces = [8,13,15]
+- **Output:** "Leetcode Helps Me Learn"
+- **Explanation:** The indices 8, 13, and 15 correspond to the underlined characters in "LeetcodeHelpsMeLearn".
+ We then place spaces before those characters.
+
+**Example 2:**
+
+- **Input:** s = "icodeinpython", spaces = [1,5,7,9]
+- **Output:** "i code in py thon"
+- **Explanation:** The indices 1, 5, 7, and 9 correspond to the underlined characters in "icodeinpython".
+ We then place spaces before those characters.
+
+
+**Example 3:**
+
+- **Input:** s = "spacing", spaces = [0,1,2,3,4,5,6]
+- **Output:** " s p a c i n g"
+- **Explanation:** We are also able to place spaces before the first character of the string.
+
+
+
+**Constraints:**
+
+- 1 <= s.length <= 3 * 105
+- `s` consists only of lowercase and uppercase English letters.
+- 1 <= spaces.length <= 3 * 105
+- `0 <= spaces[i] <= s.length - 1`
+- All the values of `spaces` are **strictly increasing**.
+
+
+**Hint:**
+1. Create a new string, initially empty, as the modified string. Iterate through the original string and append each character of the original string to the new string. However, each time you reach a character that requires a space before it, append a space before appending the character.
+2. Since the array of indices for the space locations is sorted, use a pointer to keep track of the next index to place a space. Only increment the pointer once a space has been appended.
+3. Ensure that your append operation can be done in O(1).
+
+
+
+**Solution:**
+
+We can use an efficient approach with two pointers. Here's how the implementation in PHP 5.6 would look:
+
+### Solution Explanation:
+1. Use a pointer `spaceIndex` to track the current position in the `spaces` array.
+2. Iterate through the string `s` using a loop.
+3. Check whether the current index in the string matches the current value in the `spaces` array. If it does, append a space to the result and move the `spaceIndex` pointer forward.
+4. Append the current character of the string to the result.
+5. Return the final result as a single string.
+
+This approach ensures that we process the input efficiently, taking advantage of the sorted order of the `spaces` array.
+
+Let's implement this solution in PHP: **[2109. Adding Spaces to a String](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/002109-adding-spaces-to-a-string/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+1. **Efficient Appending:** The `.` operator in PHP is used to append strings efficiently.
+2. **Two Pointers:** The `spaceIndex` pointer ensures we only process the `spaces` array once.
+3. **Time Complexity:**
+ - Iterating over the string takes _**O(n)**_, where _**n**_ is the length of the string.
+ - Checking against the `spaces` array pointer takes _**O(m)**_, where _**m**_ is the length of the `spaces` array.
+ - Combined: _**O(n + m)**_, which is optimal given the constraints.
+
+This solution adheres to the constraints and is efficient even for large inputs.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
+
+
+
diff --git a/algorithms/002109-adding-spaces-to-a-string/solution.php b/algorithms/002109-adding-spaces-to-a-string/solution.php
new file mode 100644
index 000000000..08d426565
--- /dev/null
+++ b/algorithms/002109-adding-spaces-to-a-string/solution.php
@@ -0,0 +1,28 @@
+1 <= n <= 105
+- `s[i]` is either `'('` or `')'`.
+- `locked[i]` is either `'0'` or `'1'`.
+
+
+**Hint:**
+1. Can an odd length string ever be valid?
+2. From left to right, if a locked ')' is encountered, it must be balanced with either a locked '(' or an unlocked index on its left. If neither exist, what conclusion can be drawn? If both exist, which one is more preferable to use?
+3. After the above, we may have locked indices of '(' and additional unlocked indices. How can you balance out the locked '(' now? What if you cannot balance any locked '('?
+
+
+
+**Solution:**
+
+We can approach it step by step, keeping in mind the constraints and the behavior of the locked positions.
+
+### Key Points:
+1. If the length of the string is odd, we can immediately return `false` because a valid parentheses string must have an even length (each opening `(` needs a closing `)`).
+2. We need to keep track of the number of open parentheses (`(`) and closed parentheses (`)`) as we iterate through the string. If at any point the number of closing parentheses exceeds the number of opening ones, it's impossible to balance the string and we return `false`.
+3. We must carefully handle the positions that are locked (`locked[i] == '1'`) and unlocked (`locked[i] == '0'`). Unlocked positions allow us to change the character, but locked positions do not.
+
+### Algorithm:
+- **Step 1:** Check if the length of the string `s` is odd. If so, return `false` immediately.
+- **Step 2:** Loop through the string from left to right to track the balance of parentheses.
+ - Use a counter to track the balance between opening `(` and closing `)` parentheses.
+ - If at any point, the number of closing parentheses exceeds the opening parentheses, check if the locked positions have enough flexibility to balance it.
+ - After processing the entire string, check if the parentheses are balanced, i.e., if there are no leftover unmatched opening parentheses.
+
+Let's implement this solution in PHP: **[2116. Check if a Parentheses String Can Be Valid](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/002116-check-if-a-parentheses-string-can-be-valid/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+1. **First pass (left to right):**
+ - We iterate through the string and track the balance of open parentheses. Each time we encounter an open parenthesis `(`, we increment the `open` counter. For a closed parenthesis `)`, we decrement the `open` counter.
+ - If the current character is unlocked (`locked[i] == '0'`), we can assume it to be `(` if needed to balance the parentheses.
+ - If at any point the `open` counter goes negative, it means we have more closing parentheses than opening ones, and we return `false`.
+
+2. **Second pass (right to left):**
+ - We perform a similar operation in reverse to handle the scenario of unmatched opening parentheses that might be at the end of the string.
+ - Here we track closing parentheses (`)`) with the `close` counter and ensure that no unbalanced parentheses exist.
+
+3. **Edge Case:** If the string length is odd, we immediately return `false` because it cannot form a valid parentheses string.
+
+### Time Complexity:
+- Both passes (left-to-right and right-to-left) take linear time, O(n), where `n` is the length of the string. Thus, the overall time complexity is O(n), which is efficient for the input size constraints.
+
+This solution correctly handles the problem within the given constraints.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
diff --git a/algorithms/002116-check-if-a-parentheses-string-can-be-valid/solution.php b/algorithms/002116-check-if-a-parentheses-string-can-be-valid/solution.php
new file mode 100644
index 000000000..7c17a0758
--- /dev/null
+++ b/algorithms/002116-check-if-a-parentheses-string-can-be-valid/solution.php
@@ -0,0 +1,55 @@
+= 0; $i--) {
+ // If `locked` is 0, we can treat it as either '(' or ')'
+ if ($locked[$i] === '0' || $s[$i] === ')') {
+ $close++;
+ } else {
+ // If locked[i] is '1' and s[i] is '(', it reduces the balance
+ $close--;
+ }
+
+ // At any point, if closeBalance is negative, it's invalid
+ if ($close < 0) {
+ return false;
+ }
+ }
+
+ // If both checks pass, the string can be valid
+ return true;
+ }
+}
\ No newline at end of file
diff --git a/algorithms/002182-construct-string-with-repeat-limit/README.md b/algorithms/002182-construct-string-with-repeat-limit/README.md
new file mode 100644
index 000000000..f24f5cc61
--- /dev/null
+++ b/algorithms/002182-construct-string-with-repeat-limit/README.md
@@ -0,0 +1,172 @@
+2182\. Construct String With Repeat Limit
+
+**Difficulty:** Medium
+
+**Topics:** `Hash Table`, `String`, `Greedy`, `Heap (Priority Queue)`, `Counting`
+
+You are given a string `s` and an integer `repeatLimit`. Construct a new string `repeatLimitedString` using the characters of `s` such that no letter appears **more than** `repeatLimit` times **in a row**. You do **not** have to use all characters from `s`.
+
+Return _the **lexicographically largest** `repeatLimitedString` possible_.
+
+A string `a` is **lexicographically larger** than a string `b` if in the first position where `a` and `b` differ, string `a` has a letter that appears later in the alphabet than the corresponding letter in `b`. If the first `min(a.length, b.length)` characters do not differ, then the longer string is the lexicographically larger one.
+
+**Example 1:**
+
+- **Input:** s = "cczazcc", repeatLimit = 3
+- **Output:** "zzcccac"
+- **Explanation:** We use all of the characters from s to construct the repeatLimitedString "zzcccac".
+ - The letter 'a' appears at most 1 time in a row.
+ - The letter 'c' appears at most 3 times in a row.
+ - The letter 'z' appears at most 2 times in a row.
+ - Hence, no letter appears more than repeatLimit times in a row and the string is a valid repeatLimitedString.
+ - The string is the lexicographically largest repeatLimitedString possible so we return "zzcccac".
+ - Note that the string "zzcccca" is lexicographically larger but the letter 'c' appears more than 3 times in a row, so it is not a valid repeatLimitedString.
+
+**Example 2:**
+
+- **Input:** s = "aababab", repeatLimit = 2
+- **Output:** "bbabaa"
+- **Explanation:** We use only some of the characters from s to construct the repeatLimitedString "bbabaa".
+ - The letter 'a' appears at most 2 times in a row.
+ - The letter 'b' appears at most 2 times in a row.
+ - Hence, no letter appears more than repeatLimit times in a row and the string is a valid repeatLimitedString.
+ - The string is the lexicographically largest repeatLimitedString possible so we return "bbabaa".
+ - Note that the string "bbabaaa" is lexicographically larger but the letter 'a' appears more than 2 times in a row, so it is not a valid repeatLimitedString.
+
+
+
+**Constraints:**
+
+- 1 <= repeatLimit <= s.length <= 105
+- `s` consists of lowercase English letters.
+
+
+**Hint:**
+1. Start constructing the string in descending order of characters.
+2. When repeatLimit is reached, pick the next largest character.
+
+
+
+**Solution:**
+
+We use a **greedy approach** to prioritize lexicographically larger characters while ensuring that no character exceeds the `repeatLimit` consecutively. The approach uses a **priority queue** (max heap) to process characters in lexicographically descending order and ensures that no character appears more than the `repeatLimit` times consecutively.
+
+### **Solution Explanation**
+
+1. **Count Characters**: Count the frequency of each character in the string `s` using an array.
+2. **Max Heap**: Use a max heap (priority queue) to sort and extract characters in descending lexicographical order.
+3. **Greedy Construction**:
+ - Add the largest character available up to `repeatLimit` times.
+ - If the `repeatLimit` for the current character is reached, switch to the next largest character, insert it once, and then reinsert the first character into the heap for further use.
+4. **Edge Handling**: Properly manage when a character cannot be used any further.
+
+Let's implement this solution in PHP: **[2182. Construct String With Repeat Limit](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/002182-construct-string-with-repeat-limit/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+1. **Frequency Count:**
+ - Iterate through the string `s` to count the occurrences of each character.
+ - Store the frequency in an associative array `$freq`.
+
+2. **Sort in Descending Order:**
+ - Use `krsort()` to sort the characters by their lexicographical order in **descending** order.
+
+3. **Build the Result:**
+ - Continuously append the lexicographically largest character (`$char`) to the result string.
+ - If a character reaches its `repeatLimit`, stop appending it temporarily.
+ - Use a temporary queue to hold characters that still have remaining counts but are temporarily skipped.
+
+4. **Handle Repeat Limit:**
+ - If the current character has hit the `repeatLimit`, use the next lexicographically largest character from the queue.
+ - Reinsert the previous character back into the frequency map to continue processing it later.
+
+5. **Edge Cases:**
+ - Characters may not use up their full counts initially.
+ - After handling a backup character from the queue, the current character resumes processing.
+
+### **How It Works**
+
+1. **Character Count**: `$freq` keeps track of occurrences for all characters.
+2. **Max Heap**: `SplPriorityQueue` is used as a max heap, where characters are prioritized by their ASCII value (descending order).
+3. **String Construction**:
+ - If the current character has reached its `repeatLimit`, fetch the next largest character.
+ - Use the next largest character once and reinstate the previous character into the heap for future use.
+4. **Final Result**: The resulting string is the lexicographically largest string that satisfies the `repeatLimit` constraint.
+
+### **Example Walkthrough**
+
+**Input:**
+`s = "cczazcc"`, `repeatLimit = 3`
+
+**Steps:**
+1. Frequency count:
+ `['a' => 1, 'c' => 4, 'z' => 2]`
+
+2. Sort in descending order:
+ `['z' => 2, 'c' => 4, 'a' => 1]`
+
+3. Append characters while respecting `repeatLimit`:
+ - Append `'z'` β "zz" (`z` count = 0)
+ - Append `'c'` 3 times β "zzccc" (`c` count = 1)
+ - Append `'a'` β "zzccca" (`a` count = 0)
+ - Append remaining `'c'` β "zzcccac"
+
+**Output:** `"zzcccac"`
+
+### **Time Complexity**
+- **Character Frequency Counting**: _**O(n)**_, where _**n**_ is the length of the string.
+- **Heap Operations**: _**O(26 log 26)**_, as the heap can hold up to 26 characters.
+- **Overall Complexity**: _**O(n + 26 log 26) β O(n)**_.
+
+### **Space Complexity**
+- _**O(26)**_ for the frequency array.
+- _**O(26)**_ for the heap.
+
+### **Test Cases**
+
+```php
+echo repeatLimitedString("cczazcc", 3) . "\n"; // Output: "zzcccac"
+echo repeatLimitedString("aababab", 2) . "\n"; // Output: "bbabaa"
+echo repeatLimitedString("aaaaaaa", 2) . "\n"; // Output: "aa"
+echo repeatLimitedString("abcabc", 1) . "\n"; // Output: "cba"
+```
+
+### **Edge Cases**
+
+1. `s` contains only one unique character (e.g., `"aaaaaaa"`, `repeatLimit = 2`): The output will only include as many characters as allowed consecutively.
+2. `repeatLimit = 1`: The output alternates between available characters.
+3. All characters in `s` are unique: The output is sorted in descending order.
+
+This implementation works efficiently within the constraints.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
diff --git a/algorithms/002182-construct-string-with-repeat-limit/solution.php b/algorithms/002182-construct-string-with-repeat-limit/solution.php
new file mode 100644
index 000000000..222fe30b2
--- /dev/null
+++ b/algorithms/002182-construct-string-with-repeat-limit/solution.php
@@ -0,0 +1,76 @@
+= 0; $i--) {
+ if ($freq[$i] > 0) {
+ $heap->insert(chr($i + ord('a')), $i);
+ }
+ }
+
+ $result = '';
+ $lastChar = ''; // Last character added to result
+ $lastCharCount = 0; // Count of consecutive lastChar added
+
+ while (!$heap->isEmpty()) {
+ // Step 3: Get the current largest character
+ $char = $heap->extract();
+ $charFreq = $freq[ord($char) - ord('a')];
+
+ if ($char == $lastChar && $lastCharCount == $repeatLimit) {
+ // If current char is same as lastChar and repeatLimit is reached,
+ // pick the next largest character
+ if ($heap->isEmpty()) {
+ break; // No other character to use
+ }
+ $nextChar = $heap->extract();
+ $result .= $nextChar; // Use the next largest char once
+ $freq[ord($nextChar) - ord('a')]--;
+
+ // Reinsert nextChar back into heap if it still has occurrences
+ if ($freq[ord($nextChar) - ord('a')] > 0) {
+ $heap->insert($nextChar, ord($nextChar) - ord('a'));
+ }
+
+ // Reinsert the original char into heap for later use
+ $heap->insert($char, ord($char) - ord('a'));
+
+ // Reset lastChar tracking
+ $lastChar = $nextChar;
+ $lastCharCount = 1;
+
+ } else {
+ // Use the current largest character
+ $useCount = min($repeatLimit, $charFreq);
+ $result .= str_repeat($char, $useCount);
+ $freq[ord($char) - ord('a')] -= $useCount;
+
+ // Update lastChar tracking
+ $lastChar = $char;
+ $lastCharCount = $useCount;
+
+ // Reinsert char back into heap if it still has occurrences
+ if ($freq[ord($char) - ord('a')] > 0) {
+ $heap->insert($char, ord($char) - ord('a'));
+ }
+ }
+ }
+
+ return $result;
+ }
+}
\ No newline at end of file
diff --git a/algorithms/002185-counting-words-with-a-given-prefix/README.md b/algorithms/002185-counting-words-with-a-given-prefix/README.md
new file mode 100644
index 000000000..e887e79ec
--- /dev/null
+++ b/algorithms/002185-counting-words-with-a-given-prefix/README.md
@@ -0,0 +1,104 @@
+2185\. Counting Words With a Given Prefix
+
+**Difficulty:** Easy
+
+**Topics:** `Array`, `String`, `tring Matching`
+
+You are given an array of strings `words` and a string `pref`.
+
+Return _the number of strings in `words` that contain `pref` as a **prefix**_.
+
+A **prefix** of a string `s` is any leading contiguous substring of `s`.
+
+**Example 1:**
+
+- **Input:** words = ["pay","**at**tention","practice","**at**tend"], pref = "at"
+- **Output:** 2
+- **Explanation:** The 2 strings that contain "at" as a prefix are: "**at**tention" and "**at**tend".
+
+**Example 2:**
+
+- **Input:** words = ["leetcode","win","loops","success"], pref = "code"
+- **Output:** 0
+- **Explanation:** There are no strings that contain "code" as a prefix.
+
+
+
+**Constraints:**
+
+- `1 <= words.length <= 100`
+- `1 <= words[i].length, pref.length <= 100`
+- `words[i]` and `pref` consist of lowercase English letters.
+
+
+**Hint:**
+1. Go through each word in words and increment the answer if `pref` is a **prefix** of the word.
+
+
+
+**Solution:**
+
+We can iterate through the `words` array and check if the given `pref` is a prefix of each word. You can use the `substr` function to check the beginning part of each string and compare it with `pref`. If they match, you increment the count.
+
+Let's implement this solution in PHP: **[2185. Counting Words With a Given Prefix](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/002185-counting-words-with-a-given-prefix/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+1. **Function Definition:**
+ - The function `countWordsWithPrefix` accepts an array `$words` and a string `$pref`.
+ - A counter `$count` is initialized to 0, which will hold the number of words that match the given prefix.
+
+2. **Loop Through Words:**
+ - The function loops through each word in the `$words` array.
+
+3. **Check for Prefix:**
+ - The `substr($word, 0, strlen($pref))` function extracts the first `strlen($pref)` characters from the word.
+ - If this substring matches `$pref`, the count is incremented.
+
+4. **Return Count:**
+ - Finally, the function returns the total count of words that contain the prefix.
+
+### Time Complexity:
+- The time complexity is O(n * m), where:
+ - `n` is the number of words in the array.
+ - `m` is the length of the prefix.
+ - For each word, we check the first `m` characters.
+
+This solution is efficient for the input constraints.
+
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
diff --git a/algorithms/002185-counting-words-with-a-given-prefix/solution.php b/algorithms/002185-counting-words-with-a-given-prefix/solution.php
new file mode 100644
index 000000000..5eeb13596
--- /dev/null
+++ b/algorithms/002185-counting-words-with-a-given-prefix/solution.php
@@ -0,0 +1,26 @@
+ 1011.
+ - Flip the third bit from the right: 1011 -> 1111.
+ - Flip the fourth bit from the right: 1111 -> 0111.\
+ It can be shown we cannot convert 10 to 7 in less than 3 steps. Hence, we return 3.
+
+**Example 2:**
+
+- **Input:** start = 3, goal = 4
+- **Output:** 3
+- **Explanation:** The binary representation of 3 and 4 are 011 and 100 respectively. We can convert 3 to 4 in 3 steps:
+ - Flip the first bit from the right: 011 -> 010.
+ - Flip the second bit from the right: 010 -> 000.
+ - Flip the third bit from the right: 000 -> 100.\
+ It can be shown we cannot convert 3 to 4 in less than 3 steps. Hence, we return 3.
+
+
+**Constraints:**
+
+- 0 <= start, goal <= 109
+
+**Hint:**
+1. If the value of a bit in start and goal differ, then we need to flip that bit.
+2. Consider using the XOR operation to determine which bits need a bit flip.
+
+
+
+**Solution:**
+
+We need to determine how many bit positions differ between `start` and `goal`. This can be easily achieved using the XOR operation (`^`), which returns a 1 for each bit position where the two numbers differ.
+
+### Steps:
+1. Perform the XOR operation between `start` and `goal`. The result will be a number that has `1`s in all the positions where `start` and `goal` differ.
+2. Count how many `1`s are present in the binary representation of the result (i.e., the Hamming distance).
+3. The number of `1`s will give us the minimum number of bit flips needed.
+
+Let's implement this solution in PHP: **[2220. Minimum Bit Flips to Convert Number](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/002220-minimum-bit-flips-to-convert-number/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+1. The `^` (XOR) operation compares each bit of `start` and `goal`. If the bits are different, the corresponding bit in the result will be `1`.
+2. We then count the number of `1`s in the result, which gives the number of differing bits, i.e., the number of bit flips required.
+3. The `& 1` operation checks if the last bit is `1`, and `>>= 1` shifts the number right to process the next bit.
+
+### Time Complexity:
+- The time complexity is \(O(\log N)\), where \(N\) is the larger of `start` or `goal`, because we're checking each bit of the number. In the worst case, we will loop through all bits of a 32-bit integer (since PHP 5.6 works with 32-bit or 64-bit integers depending on the system).
+
+### Output:
+- For `start = 10` and `goal = 7`, the output is `3`.
+- For `start = 3` and `goal = 4`, the output is `3`.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
diff --git a/algorithms/002220-minimum-bit-flips-to-convert-number/solution.php b/algorithms/002220-minimum-bit-flips-to-convert-number/solution.php
new file mode 100644
index 000000000..4c02755f3
--- /dev/null
+++ b/algorithms/002220-minimum-bit-flips-to-convert-number/solution.php
@@ -0,0 +1,26 @@
+ 0) {
+ // Increment count if the last bit is 1
+ $bitFlips += $xor & 1;
+ // Shift xor to the right by 1 bit
+ $xor >>= 1;
+ }
+
+ return $bitFlips;
+
+ }
+}
\ No newline at end of file
diff --git a/algorithms/002257-count-unguarded-cells-in-the-grid/README.md b/algorithms/002257-count-unguarded-cells-in-the-grid/README.md
new file mode 100644
index 000000000..76d549abb
--- /dev/null
+++ b/algorithms/002257-count-unguarded-cells-in-the-grid/README.md
@@ -0,0 +1,138 @@
+2257\. Count Unguarded Cells in the Grid
+
+**Difficulty:** Medium
+
+**Topics:** `Array`, `Matrix`, `Simulation`
+
+You are given two integers `m` and `n` representing a **0-indexed** `m x n` grid. You are also given two 2D integer arrays `guards` and `walls` where guards[i] = [rowi, coli]
and walls[j] = [rowj, colj]
represent the positions of the ith
guard and jth
wall respectively.
+
+A guard can see **every** cell in the four cardinal directions (north, east, south, or west) starting from their position unless **obstructed** by a wall or another guard. A cell is **guarded** if there is **at least** one guard that can see it.
+
+Return _the number of unoccupied cells that are **not guarded**_.
+
+**Example 1:**
+
+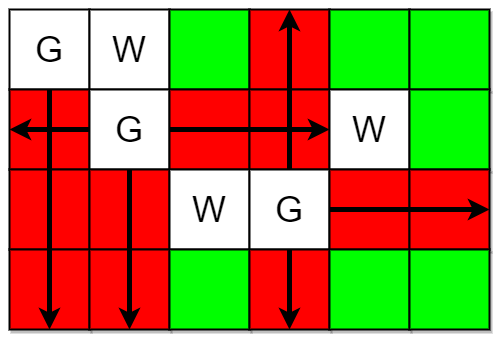
+
+- **Input:** m = 4, n = 6, guards = [[0,0],[1,1],[2,3]], walls = [[0,1],[2,2],[1,4]]
+- **Output:** 7
+- **Explanation:** The guarded and unguarded cells are shown in red and green respectively in the above diagram.
+ There are a total of 7 unguarded cells, so we return 7.
+
+**Example 2:**
+
+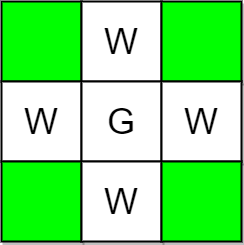
+
+- **Input:** m = 3, n = 3, guards = [[1,1]], walls = [[0,1],[1,0],[2,1],[1,2]]
+- **Output:** 4
+- **Explanation:** The unguarded cells are shown in green in the above diagram.
+ There are a total of 4 unguarded cells, so we return 4.
+
+
+
+**Constraints:**
+
+- 1 <= m, n <= 105
+- 2 <= m * n <= 105
+- 1 <= guards.length, walls.length <= 5 * 104
+- `2 <= guards.length + walls.length <= m * n`
+- `guards[i].length == walls[j].length == 2`
+- 0 <= rowi, rowj < m
+- 0 <= coli, colj < n
+- All the positions in `guards` and `walls` are **unique**.
+
+
+**Hint:**
+1. Create a 2D array to represent the grid. Can you mark the tiles that can be seen by a guard?
+2. Iterate over the guards, and for each of the 4 directions, advance the current tile and mark the tile. When should you stop advancing?
+
+
+
+**Solution:**
+
+We need to mark the cells that are guarded by at least one guard. The guards can see in four cardinal directions (north, south, east, and west), but their vision is blocked by walls. We can simulate this process and count the number of cells that are unguarded.
+
+### Approach:
+1. **Create a grid**: We can represent the grid as a 2D array where each cell can either be a wall, a guard, or empty.
+2. **Mark guarded cells**: For each guard, iterate in the four directions (north, south, east, west) and mark all the cells it can see, stopping when we encounter a wall or another guard.
+3. **Count unguarded cells**: After marking the guarded cells, count how many cells are not guarded and are not walls.
+
+### Steps:
+1. **Grid Initialization**: Create a 2D array to represent the grid. Mark cells with walls, guards, and guarded areas as we iterate.
+
+2. **Simulating Guard Coverage**:
+ - Guards can see in four directions (north, south, east, west).
+ - Mark cells guarded by each guard until encountering a wall or another guard.
+
+3. **Counting Unguarded Cells**: After processing all guards, count cells that are neither walls, guards, nor guarded.
+
+Let's implement this solution in PHP: **[2257. Count Unguarded Cells in the Grid](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/002257-count-unguarded-cells-in-the-grid/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+1. **Initialization**:
+ - The grid is initialized with `0` for empty cells. Walls and guards are marked with unique constants.
+
+2. **Guard Simulation**:
+ - For each guard, simulate movement in all four directions, marking cells as guarded until hitting a wall or another guard.
+
+3. **Counting Unguarded Cells**:
+ - After processing all guards, iterate through the grid and count cells still marked as `0`.
+
+### Performance:
+- Time complexity: _**O(m x n + g x d)**_, where _**g**_ is the number of guards and _**d**_ is the number of cells in the direction each guard can travel.
+- Space complexity: _**O(m x n)**_ for the grid.
+
+### Time Complexity:
+- **Grid initialization**: **_O(m * n)**_, where _**m**_ and _**n**_ are the dimensions of the grid.
+- **Marking guard vision**: Each guard checks at most the length of the row or column in the four directions. So, for each guard, it's _**O(m + n)**_.
+- **Counting unguarded cells**: _**O(m * n)**_.
+
+Thus, the overall complexity is _**O(m * n)**_, which is efficient given the problem constraints.
+
+### Edge Cases:
+- If no `guards` or `walls` exist, the entire grid will be unguarded.
+- If all cells are either guarded or walls, the result will be `0` unguarded cells.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
diff --git a/algorithms/002257-count-unguarded-cells-in-the-grid/solution.php b/algorithms/002257-count-unguarded-cells-in-the-grid/solution.php
new file mode 100644
index 000000000..03df6dd71
--- /dev/null
+++ b/algorithms/002257-count-unguarded-cells-in-the-grid/solution.php
@@ -0,0 +1,71 @@
+= $m || $y < 0 || $y >= $n || $grid[$x][$y] == $WALL || $grid[$x][$y] == $GUARD) {
+ break;
+ }
+
+ // Mark the cell as guarded
+ if ($grid[$x][$y] == 0) {
+ $grid[$x][$y] = $GUARDED;
+ }
+ }
+ }
+ }
+
+ // Count unguarded cells
+ $unguardedCount = 0;
+ for ($i = 0; $i < $m; $i++) {
+ for ($j = 0; $j < $n; $j++) {
+ if ($grid[$i][$j] == 0) {
+ $unguardedCount++;
+ }
+ }
+ }
+
+ return $unguardedCount;
+ }
+}
\ No newline at end of file
diff --git a/algorithms/002270-number-of-ways-to-split-array/README.md b/algorithms/002270-number-of-ways-to-split-array/README.md
new file mode 100644
index 000000000..e2c256c48
--- /dev/null
+++ b/algorithms/002270-number-of-ways-to-split-array/README.md
@@ -0,0 +1,105 @@
+2270\. Number of Ways to Split Array
+
+**Difficulty:** Medium
+
+**Topics:** `Array`, `Prefix Sum`
+
+You are given a **0-indexed** integer array `nums` of length `n`.
+
+`nums` contains a **valid split** at index `i` if the following are true:
+
+- The sum of the first `i + 1` elements is **greater than or equal to** the sum of the last `n - i - 1` elements.
+- There is **at least one** element to the right of `i`. That is, `0 <= i < n - 1`.
+
+Return _the number of **valid splits** in `nums`_.
+
+**Example 1:**
+
+- **Input:** nums = [10,4,-8,7]
+- **Output:** 2
+- **Explanation:** There are three ways of splitting nums into two non-empty parts:
+ - Split nums at index `0`. Then, the first part is `[10]`, and its sum is `10`. The second part is `[4,-8,7]`, and its sum is `3`. Since `10 >= 3`, `i = 0` is a valid split.
+ - Split nums at index `1`. Then, the first part is `[10,4]`, and its sum is `14`. The second part is `[-8,7]`, and its sum is `-1`. Since `14 >= -1`, `i = 1` is a valid split.
+ - Split nums at index `2`. Then, the first part is `[10,4,-8]`, and its sum is `6`. The second part is `[7]`, and its sum is `7`. Since `6 < 7`, `i = 2` is not a valid split.
+ Thus, the number of valid splits in nums is `2`.
+
+**Example 2:**
+
+- **Input:** nums = [2,3,1,0]
+- **Output:** 2
+- **Explanation:** There are two valid splits in nums:
+ - Split nums at index `1`. Then, the first part is `[2,3]`, and its sum is `5`. The second part is `[1,0]`, and its sum is `1`. Since `5 >= 1`, `i = 1` is a valid split.
+ - Split nums at index `2`. Then, the first part is `[2,3,1]`, and its sum is `6`. The second part is `[0]`, and its sum is `0`. Since `6 >= 0`, `i = 2` is a valid split.
+
+
+
+**Constraints:**
+
+- 2 <= nums.length <= 105
+- -105 <= nums[i] <= 105
+
+
+**Hint:**
+1. For any index `i`, how can we find the `sum` of the first `(i+1)` elements from the `sum` of the first `i` elements?
+2. If the total `sum` of the array is known, how can we check if the `sum` of the first `(i+1)` elements `greater than or equal to` the remaining elements?
+
+
+
+**Solution:**
+
+We can approach it using the following steps:
+
+### Approach:
+
+1. **Prefix Sum**: First, we compute the cumulative sum of the array from the left, which helps in checking the sum of the first `i+1` elements.
+2. **Total Sum**: Compute the total sum of the array, which is useful in checking if the sum of the remaining elements is less than or equal to the sum of the first `i+1` elements.
+3. **Iterate over the array**: For each valid index `i` (where `0 <= i < n-1`), we check if the sum of the first `i+1` elements is greater than or equal to the sum of the last `n-i-1` elements.
+4. **Efficiency**: Instead of recalculating the sums repeatedly, use the prefix sum and the total sum for efficient comparisons.
+
+Let's implement this solution in PHP: **[2270. Number of Ways to Split Array](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/002270-number-of-ways-to-split-array/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+1. **$totalSum**: This variable stores the sum of all elements in the `nums` array.
+2. **$prefixSum**: This variable keeps track of the cumulative sum of elements from the left (up to index `i`).
+3. **$remainingSum**: This is the sum of the remaining elements from index `i+1` to the end of the array. It is calculated by subtracting `$prefixSum` from `$totalSum`.
+4. **Valid Split Check**: For each index `i`, we check if the prefix sum is greater than or equal to the remaining sum.
+
+### Time Complexity:
+- **O(n)**: We loop through the array once to compute the sum and once again to check for valid splits. Therefore, the time complexity is linear with respect to the length of the array.
+
+### Space Complexity:
+- **O(1)**: We are using only a few extra variables (`$totalSum`, `$prefixSum`, `$remainingSum`), so the space complexity is constant.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
diff --git a/algorithms/002270-number-of-ways-to-split-array/solution.php b/algorithms/002270-number-of-ways-to-split-array/solution.php
new file mode 100644
index 000000000..5e343e0e5
--- /dev/null
+++ b/algorithms/002270-number-of-ways-to-split-array/solution.php
@@ -0,0 +1,28 @@
+= $remainingSum) {
+ $validSplits++;
+ }
+ }
+
+ return $validSplits;
+ }
+}
\ No newline at end of file
diff --git a/algorithms/002275-largest-combination-with-bitwise-and-greater-than-zero/README.md b/algorithms/002275-largest-combination-with-bitwise-and-greater-than-zero/README.md
new file mode 100644
index 000000000..7ab27d3bf
--- /dev/null
+++ b/algorithms/002275-largest-combination-with-bitwise-and-greater-than-zero/README.md
@@ -0,0 +1,109 @@
+2275\. Largest Combination With Bitwise AND Greater Than Zero
+
+**Difficulty:** Medium
+
+**Topics:** `Array`, `Hash Table`, `Bit Manipulation`, `Counting`
+
+The **bitwise AND** of an array `nums` is the bitwise AND of all integers in `nums`.
+
+- For example, for `nums = [1, 5, 3]`, the bitwise AND is equal to `1 & 5 & 3 = 1`.
+- Also, for `nums = [7]`, the bitwise AND is `7`.
+
+You are given an array of positive integers `candidates`. Evaluate the **bitwise AND** of every **combination** of numbers of `candidates`. Each number in `candidates` may only be used **once** in each combination.
+
+Return _the size of the **largest** combination of candidates with a bitwise AND **greater** than `0`_.
+
+**Example 1:**
+
+- **Input:** candidates = [16,17,71,62,12,24,14]
+- **Output:** 4
+- **Explanation:** The combination [16,17,62,24] has a bitwise AND of 16 & 17 & 62 & 24 = 16 > 0.
+ The size of the combination is 4.
+ It can be shown that no combination with a size greater than 4 has a bitwise AND greater than 0.
+ Note that more than one combination may have the largest size.
+ For example, the combination [62,12,24,14] has a bitwise AND of 62 & 12 & 24 & 14 = 8 > 0.
+
+**Example 2:**
+
+- **Input:** candidates = [8,8]
+- **Output:** 2
+- **Explanation:** The largest combination [8,8] has a bitwise AND of 8 & 8 = 8 > 0.
+ The size of the combination is 2, so we return 2.
+
+**Constraints:**
+
+- 1 <= candidates.length <= 105
+- 1 <= candidates[i] <= 107
+
+
+**Hint:**
+1. For the bitwise AND to be greater than zero, at least one bit should be 1 for every number in the combination.
+2. The candidates are 24 bits long, so for every bit position, we can calculate the size of the largest combination such that the bitwise AND will have a 1 at that bit position.
+
+
+
+**Solution:**
+
+We need to focus on identifying groups of numbers where at least one bit position in their binary representation remains set (1) across all numbers in the combination.
+
+### Solution Outline
+
+1. **Bit Analysis**: Since each number in `candidates` can be represented by a binary number with up to 24 bits (as `1 <= candidates[i] <= 10^7`), we only need to examine each bit position from 0 to 23.
+
+2. **Count Set Bits at Each Position**: For each bit position, count how many numbers in `candidates` have that bit set to 1. If multiple numbers share a bit in the same position, they could potentially form a combination with a bitwise AND greater than zero at that bit position.
+
+3. **Find the Largest Count**: The largest number of numbers with a set bit at any given position will be the answer, as it represents the largest possible combination where the bitwise AND result is greater than zero.
+
+### Example
+
+Consider `candidates = [16, 17, 71, 62, 12, 24, 14]`:
+
+- Convert each number to binary and analyze bit positions.
+- Count how many times each bit is set across all numbers.
+- Find the maximum count across all bit positions.
+
+Let's implement this solution in PHP: **[2275. Largest Combination With Bitwise AND Greater Than Zero](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/002275-largest-combination-with-bitwise-and-greater-than-zero/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+1. **Loop Through Each Bit Position**: We iterate over each bit position from 0 to 23.
+2. **Count Numbers with Bit Set**: For each position, count how many numbers in `candidates` have that specific bit set.
+3. **Update Maximum Combination Size**: Track the highest count across all bit positions.
+4. **Return the Result**: The result is the largest combination size with a bitwise AND greater than zero, as required.
+
+### Complexity Analysis
+
+- **Time Complexity**: _**O(n x 24) = O(n)**_, where _**n**_ is the number of elements in `candidates`, because we perform 24 operations (one for each bit position) for each number.
+- **Space Complexity**: _**O(1)**_, since we are only using a fixed amount of extra space.
+
+This approach is efficient enough to handle the input size limit (candidates.length <= 105
).
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
diff --git a/algorithms/002275-largest-combination-with-bitwise-and-greater-than-zero/solution.php b/algorithms/002275-largest-combination-with-bitwise-and-greater-than-zero/solution.php
new file mode 100644
index 000000000..b8424661a
--- /dev/null
+++ b/algorithms/002275-largest-combination-with-bitwise-and-greater-than-zero/solution.php
@@ -0,0 +1,29 @@
+1 <= m, n <= 105
+- 2 <= m * n <= 105
+- `grid[i][j]` is either `0` or `1`.
+- `grid[0][0] == grid[m - 1][n - 1] == 0`
+
+
+**Hint:**
+1. Model the grid as a graph where cells are nodes and edges are between adjacent cells. Edges to cells with obstacles have a cost of 1 and all other edges have a cost of 0.
+2. Could you use 0-1 Breadth-First Search or Dijkstraβs algorithm?
+
+
+
+**Solution:**
+
+We need to model this problem using a graph where each cell in the grid is a node. The goal is to navigate from the top-left corner `(0, 0)` to the bottom-right corner `(m-1, n-1)`, while minimizing the number of obstacles (1s) we need to remove.
+
+### Approach:
+
+1. **Graph Representation:**
+ - Each cell in the grid is a node.
+ - Movement between adjacent cells (up, down, left, right) is treated as an edge.
+ - If an edge moves through a cell with a `1` (obstacle), the cost is `1` (remove the obstacle), and if it moves through a `0` (empty cell), the cost is `0`.
+
+2. **Algorithm Choice:**
+ - Since we need to minimize the number of obstacles removed, we can use **0-1 BFS** (Breadth-First Search with a deque) or **Dijkstra's algorithm** with a priority queue.
+ - **0-1 BFS** is suitable here because each edge has a cost of either `0` or `1`.
+
+3. **0-1 BFS:**
+ - We use a deque (double-ended queue) to process nodes with different costs:
+ - Push cells with cost `0` to the front of the deque.
+ - Push cells with cost `1` to the back of the deque.
+ - The idea is to explore the grid and always expand the path that does not require obstacle removal first, and only remove obstacles when necessary.
+
+Let's implement this solution in PHP: **[2290. Minimum Obstacle Removal to Reach Corner](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/002290-minimum-obstacle-removal-to-reach-corner/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+1. **Input Parsing:**
+ - The `grid` is taken as a 2D array.
+ - Rows and columns are calculated for bounds checking.
+
+2. **Deque Implementation:**
+ - `SplDoublyLinkedList` is used to simulate the deque. It supports adding elements at the front (`unshift`) or the back (`push`).
+
+3. **Visited Array:**
+ - Keeps track of cells already visited to avoid redundant processing.
+
+4. **0-1 BFS Logic:**
+ - Start from `(0, 0)` with a cost of 0.
+ - For each neighboring cell:
+ - If it's empty (`grid[nx][ny] == 0`), add it to the front of the deque with the same cost.
+ - If it's an obstacle (`grid[nx][ny] == 1`), add it to the back of the deque with an incremented cost.
+
+5. **Return the Result:**
+ - When the bottom-right corner is reached, return the cost.
+ - If no valid path exists (though the problem guarantees one), return `-1`.
+
+---
+
+### Complexity:
+- **Time Complexity:** _**O(m x n)**_, where _**m**_ is the number of rows and _**n**_ is the number of columns. Each cell is processed once.
+- **Space Complexity:** _**O(m x n)**_, for the visited array and deque.
+
+This implementation works efficiently within the given constraints.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
diff --git a/algorithms/002290-minimum-obstacle-removal-to-reach-corner/solution.php b/algorithms/002290-minimum-obstacle-removal-to-reach-corner/solution.php
new file mode 100644
index 000000000..0a5cad88d
--- /dev/null
+++ b/algorithms/002290-minimum-obstacle-removal-to-reach-corner/solution.php
@@ -0,0 +1,55 @@
+push([0, 0, 0]); // [row, col, cost]
+
+ // Visited array
+ $visited = array_fill(0, $rows, array_fill(0, $cols, false));
+ $visited[0][0] = true;
+
+ // 0-1 BFS
+ while (!$deque->isEmpty()) {
+ list($x, $y, $cost) = $deque->shift();
+
+ // If we reach the bottom-right corner, return the cost
+ if ($x == $rows - 1 && $y == $cols - 1) {
+ return $cost;
+ }
+
+ // Explore neighbors
+ foreach ($directions as $direction) {
+ $nx = $x + $direction[0];
+ $ny = $y + $direction[1];
+
+ // Check if the new position is within bounds and not visited
+ if ($nx >= 0 && $nx < $rows && $ny >= 0 && $ny < $cols && !$visited[$nx][$ny]) {
+ $visited[$nx][$ny] = true;
+
+ // If no obstacle, add to the front of the deque
+ if ($grid[$nx][$ny] == 0) {
+ $deque->unshift([$nx, $ny, $cost]);
+ } else {
+ // If obstacle, add to the back of the deque
+ $deque->push([$nx, $ny, $cost + 1]);
+ }
+ }
+ }
+ }
+
+ return -1; // Should not reach here if there's a valid path
+ }
+}
\ No newline at end of file
diff --git a/algorithms/002326-spiral-matrix-iv/README.md b/algorithms/002326-spiral-matrix-iv/README.md
new file mode 100644
index 000000000..6197fdd13
--- /dev/null
+++ b/algorithms/002326-spiral-matrix-iv/README.md
@@ -0,0 +1,151 @@
+2326\. Spiral Matrix IV
+
+**Difficulty:** Medium
+
+**Topics:** `Array`, `Linked List`, `Matrix`, `Simulation`
+
+You are given two integers `m` and `n`, which represent the dimensions of a matrix.
+
+You are also given the `head` of a linked list of integers.
+
+Generate an `m x n` matrix that contains the integers in the linked list presented in **spiral** order (**clockwise**), starting from the **top-left** of the matrix. If there are remaining empty spaces, fill them with `-1`.
+
+Return _the generated matrix_.
+
+**Example 1:**
+
+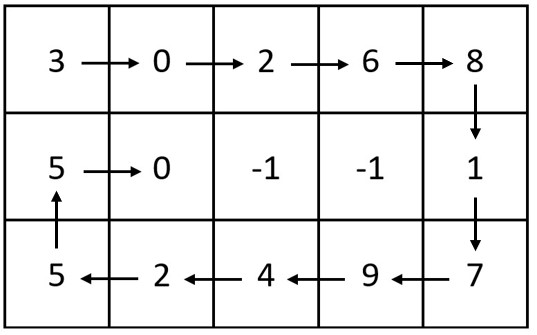
+
+- **Input:** m = 3, n = 5, head = [3,0,2,6,8,1,7,9,4,2,5,5,0]
+- **Output:** [[3,0,2,6,8],[5,0,-1,-1,1],[5,2,4,9,7]]
+- **Explanation:** The diagram above shows how the values are printed in the matrix.\
+ Note that the remaining spaces in the matrix are filled with -1.
+
+**Example 2:**
+
+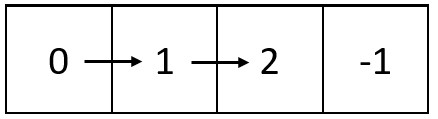
+
+- **Input:** m = 1, n = 4, head = [0,1,2]
+- **Output:** [[0,1,2,-1]]
+- **Explanation:** The diagram above shows how the values are printed from left to right in the matrix.\
+ The last space in the matrix is set to -1.
+
+
+**Example 3:**
+
+- **Input:** cost = [[2, 5, 1], [3, 4, 7], [8, 1, 2], [6, 2, 4], [3, 8, 8]]
+- **Output:** 10
+
+
+
+**Constraints:**
+
+- 1 <= m, n <= 105
+- 1 <= m, n <= 105
+- The number of nodes in the list is in the range `[1, m * n]`.
+- 0 <= Node.val <= 1000
+
+
+**Hint:**
+1. First, generate an m x n matrix filled with -1s.
+2. Navigate within the matrix at (i, j) with the help of a direction vector β¨di, djβ©. At (i, j), you need to decide if you can keep going in the current direction.
+3. If you cannot keep going, rotate the direction vector clockwise by 90 degrees.
+
+
+
+**Solution:**
+
+We will simulate a spiral traversal of an `m x n` matrix, filling it with values from a linked list. The remaining positions that don't have corresponding linked list values will be filled with `-1`.
+
+Here's how the solution is structured:
+
+1. **Matrix Initialization**: We first create an `m x n` matrix initialized with `-1`.
+2. **Direction Vectors**: The spiral movement can be controlled using a direction vector that cycles through the right, down, left, and up directions. This ensures that we are traversing the matrix in a spiral manner.
+3. **Linked List Iteration**: We traverse through the linked list, placing values in the matrix in spiral order.
+4. **Boundary Handling**: We check if we've reached the boundary or encountered an already filled cell. If so, we change direction (clockwise).
+
+Let's implement this solution in PHP: **[2326. Spiral Matrix IV](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/002326-spiral-matrix-iv/solution.php)**
+
+```php
+val = $val;
+ $this->next = $next;
+ }
+}
+/**
+ * @param Integer $m
+ * @param Integer $n
+ * @param ListNode $head
+ * @return Integer[][]
+ */
+function spiralMatrix($m, $n, $head) {
+ ...
+ ...
+ ...
+ /**
+ * go to ./solution.php
+ */
+}
+
+// Helper function to print the matrix (for debugging)
+function printMatrix($matrix) {
+ foreach ($matrix as $row) {
+ echo implode(" ", $row) . "\n";
+ }
+}
+
+// Example usage:
+// Create the linked list: [3,0,2,6,8,1,7,9,4,2,5,5,0]
+$head = new ListNode(3);
+$head->next = new ListNode(0);
+$head->next->next = new ListNode(2);
+$head->next->next->next = new ListNode(6);
+$head->next->next->next->next = new ListNode(8);
+$head->next->next->next->next->next = new ListNode(1);
+$head->next->next->next->next->next->next = new ListNode(7);
+$head->next->next->next->next->next->next->next = new ListNode(9);
+$head->next->next->next->next->next->next->next->next = new ListNode(4);
+$head->next->next->next->next->next->next->next->next->next = new ListNode(2);
+$head->next->next->next->next->next->next->next->next->next->next = new ListNode(5);
+$head->next->next->next->next->next->next->next->next->next->next->next = new ListNode(5);
+$head->next->next->next->next->next->next->next->next->next->next->next->next = new ListNode(0);
+
+$m = 3;
+$n = 5;
+
+$matrix = spiralMatrix($m, $n, $head);
+printMatrix($matrix);
+?>
+```
+
+### Explanation:
+
+1. **Matrix Initialization**: The matrix is initialized with `-1` so that any unfilled spaces will remain `-1` by default.
+
+2. **Spiral Movement**:
+ - The direction vector `dirs` manages movement in four directions: right, down, left, and up.
+ - The index `dirIndex` keeps track of the current direction. After moving in one direction, we calculate the next position and check if it's valid. If not, we change the direction.
+
+3. **Linked List Traversal**:
+ - We traverse through the linked list nodes, placing values in the matrix one by one, following the spiral order.
+
+4. **Boundary and Direction Change**:
+ - When we encounter an invalid position (out of bounds or already filled), we rotate the direction by 90 degrees (i.e., change the direction vector).
+
+### Time Complexity:
+
+- Filling the matrix takes O(m \* n) because we are traversing every cell once. Hence, the time complexity is O(m \* n), which is efficient given the constraints.
+
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
diff --git a/algorithms/002326-spiral-matrix-iv/solution.php b/algorithms/002326-spiral-matrix-iv/solution.php
new file mode 100644
index 000000000..bb671e79b
--- /dev/null
+++ b/algorithms/002326-spiral-matrix-iv/solution.php
@@ -0,0 +1,63 @@
+val = $val;
+ * $this->next = $next;
+ * }
+ * }
+ */
+class Solution {
+
+ /**
+ * @param Integer $m
+ * @param Integer $n
+ * @param ListNode $head
+ * @return Integer[][]
+ */
+ function spiralMatrix($m, $n, $head) {
+ // Step 1: Initialize an m x n matrix filled with -1
+ $matrix = array_fill(0, $m, array_fill(0, $n, -1));
+
+ // Step 2: Define the direction vectors for right, down, left, and up movements
+ $dirs = [[0, 1], [1, 0], [0, -1], [-1, 0]]; // right, down, left, up
+ $dirIndex = 0; // Start with the direction moving right
+ $row = 0;
+ $col = 0;
+
+ // Step 3: Traverse the linked list
+ $curr = $head;
+
+ while ($curr) {
+ // Step 4: Fill the current position in the matrix with the current node's value
+ $matrix[$row][$col] = $curr->val;
+
+ // Move to the next node in the linked list
+ $curr = $curr->next;
+
+ // Calculate the next position
+ $nextRow = $row + $dirs[$dirIndex][0];
+ $nextCol = $col + $dirs[$dirIndex][1];
+
+ // Step 5: Check if the next position is out of bounds or already filled
+ if ($nextRow < 0 || $nextRow >= $m || $nextCol < 0 || $nextCol >= $n || $matrix[$nextRow][$nextCol] != -1) {
+ // Change direction (rotate clockwise)
+ $dirIndex = ($dirIndex + 1) % 4;
+ // Update the next position after changing direction
+ $nextRow = $row + $dirs[$dirIndex][0];
+ $nextCol = $col + $dirs[$dirIndex][1];
+ }
+
+ // Step 6: Update row and col to the next position
+ $row = $nextRow;
+ $col = $nextCol;
+ }
+
+ return $matrix;
+
+ }
+}
\ No newline at end of file
diff --git a/algorithms/002337-move-pieces-to-obtain-a-string/README.md b/algorithms/002337-move-pieces-to-obtain-a-string/README.md
new file mode 100644
index 000000000..817ad8492
--- /dev/null
+++ b/algorithms/002337-move-pieces-to-obtain-a-string/README.md
@@ -0,0 +1,168 @@
+2337\. Move Pieces to Obtain a String
+
+**Difficulty:** Medium
+
+**Topics:** `Two Pointers`, `String`
+
+You are given two strings `start` and `target`, both of length `n`. Each string consists only of the characters `'L'`, `'R'`, and `'_'` where:
+
+- The characters `'L'` and `'R'` represent pieces, where a piece `'L'` can move to the **left** only if there is a **blank** space directly to its left, and a piece `'R'` can move to the **right** only if there is a **blank** space directly to its right.
+- The character `'_'` represents a blank space that can be occupied by **any** of the `'L'` or `'R'` pieces.
+
+Return _`true` if it is possible to obtain the string `target` by moving the pieces of the string `start` any number of times. Otherwise, return `false`_.
+
+**Example 1:**
+
+- **Input:** start = "_L__R__R_", target = "L______RR"
+- **Output:** true
+- **Explanation:** We can obtain the string target from start by doing the following moves:
+ - Move the first piece one step to the left, start becomes equal to "**L**___R__R_".
+ - Move the last piece one step to the right, start becomes equal to "L___R___R".
+ - Move the second piece three steps to the right, start becomes equal to "L______RR".
+ Since it is possible to get the string target from start, we return true.
+
+**Example 2:**
+
+- **Input:** start = "R_L_", target = "__LR"
+- **Output:** false
+- **Explanation:** The 'R' piece in the string start can move one step to the right to obtain "_**R**L_".
+ After that, no pieces can move anymore, so it is impossible to obtain the string target from start.
+
+
+**Example 3:**
+
+- **Input:** start = "_R", target = "R_"
+- **Output:** false
+- **Output:** The piece in the string start can move only to the right, so it is impossible to obtain the string target from start.
+
+
+
+**Constraints:**
+
+- `n == start.length == target.length`
+- 1 <= n <= 105
+- `start` and `target` consist of the characters `'L'`, `'R'`, and `'_'`.
+
+
+**Hint:**
+1. After some sequence of moves, can the order of the pieces change?
+2. Try to match each piece in s with a piece in e.
+
+
+
+**Solution:**
+
+We need to check if we can transform the string `start` into the string `target` by moving pieces ('L' and 'R') as per the given rules. The main constraints to consider are:
+
+- 'L' can only move left (and cannot cross other 'L' or 'R' pieces).
+- 'R' can only move right (and cannot cross other 'L' or 'R' pieces).
+- We can use any blank spaces ('_') to move pieces around.
+
+### Plan:
+
+1. **Length Check**: First, check if both strings have the same length. If they don't, return `false` immediately.
+
+2. **Character Frequency Check**: The number of 'L's, 'R's, and '_' in the `start` string must match the respective counts in the `target` string because the pieces cannot be duplicated or destroyed, only moved.
+
+3. **Piece Matching Using Two Pointers**:
+ - Traverse through both strings (`start` and `target`).
+ - Check if each piece ('L' or 'R') in `start` can move to its corresponding position in `target`.
+ - Specifically:
+ - 'L' should always move to the left (i.e., it must not be in a position where a piece in `target` should move right).
+ - 'R' should always move to the right (i.e., it must not be in a position where a piece in `target` should move left).
+
+### Algorithm Explanation:
+
+1. **Filter `L` and `R` Positions:**
+ - Remove all `_` from both strings `start` and `target` to compare the sequence of `L` and `R`. If the sequences differ, return `false` immediately.
+
+2. **Two-Pointer Traversal:**
+ - Use two pointers to iterate over the characters in `start` and `target`.
+ - Ensure that:
+ - For `L`, the piece in `start` can only move **left**, so its position in `start` should be greater than or equal to its position in `target`.
+ - For `R`, the piece in `start` can only move **right**, so its position in `start` should be less than or equal to its position in `target`.
+
+3. **Output Result:**
+ - If all conditions are satisfied during traversal, return `true`. Otherwise, return `false`.
+
+Let's implement this solution in PHP: **[2337. Move Pieces to Obtain a String](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/002337-move-pieces-to-obtain-a-string/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+1. **Initial Checks**: First, we check the length of both strings and ensure the counts of 'L' and 'R' are the same in both strings. If not, return `false`.
+
+2. **Two Pointers Logic**: We use two pointers, `i` and `j`, to traverse both strings:
+ - Skip over the blank spaces ('_') since they don't affect the movement of the pieces.
+ - If the characters at `i` and `j` in `start` and `target` differ, return `false` (as we cannot move pieces to different characters).
+ - If an 'L' is found in `start` and is at a position greater than its target position, or if an 'R' is found in `start` and is at a position smaller than its target position, return `false` (since 'L' can only move left and 'R' can only move right).
+
+3. **Edge Cases**: The solution handles all possible edge cases, such as:
+ - Different counts of 'L' or 'R' in `start` and `target`.
+ - Inability to move pieces due to constraints.
+
+### Time Complexity:
+
+- The time complexity is O(n), where `n` is the length of the input strings. This is because we traverse each string only once.
+
+### Space Complexity:
+
+- The space complexity is O(1), as we are only using a fixed amount of extra space.
+
+### Complexity Analysis:
+1. **Time Complexity:**
+ - Filtering underscores takes _**O(n)**_.
+ - Two-pointer traversal takes _**O(n)**_.
+ - Overall: _**O(n)**_.
+
+2. **Space Complexity:**
+ - Two strings (`$startNoUnderscore` and `$targetNoUnderscore`) are created, each of size _**O(n)**_.
+ - Overall: _**O(n)**_.
+
+### Explanation of Test Cases:
+1. **Input:** `_L__R__R_`, `L______RR`
+ - The sequence of `L` and `R` matches.
+ - Each piece can be moved to the required position following the rules.
+ - **Output:** `true`.
+
+2. **Input:** `R_L_`, `__LR`
+ - The sequence of `L` and `R` matches.
+ - The `R` piece cannot move left; hence, the transformation is impossible.
+ - **Output:** `false`.
+
+3. **Input:** `_R`, `R_`
+ - The `R` piece cannot move left; hence, the transformation is impossible.
+ - **Output:** `false`.
+
+This implementation is efficient and adheres to the problem constraints, making it suitable for large input sizes.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
diff --git a/algorithms/002337-move-pieces-to-obtain-a-string/solution.php b/algorithms/002337-move-pieces-to-obtain-a-string/solution.php
new file mode 100644
index 000000000..90c059148
--- /dev/null
+++ b/algorithms/002337-move-pieces-to-obtain-a-string/solution.php
@@ -0,0 +1,45 @@
+ $j)) {
+ return false;
+ }
+ }
+
+ $i++;
+ $j++;
+ }
+
+ return true;
+ }
+}
\ No newline at end of file
diff --git a/algorithms/002381-shifting-letters-ii/README.md b/algorithms/002381-shifting-letters-ii/README.md
new file mode 100644
index 000000000..692106714
--- /dev/null
+++ b/algorithms/002381-shifting-letters-ii/README.md
@@ -0,0 +1,109 @@
+2381\. Shifting Letters II
+
+**Difficulty:** Medium
+
+**Topics:** `Array`, `String`, `Prefix Sum`
+
+You are given a string `s` of lowercase English letters and a 2D integer array `shifts` where shifts[i] = [starti, endi, directioni]
. For every `i`, **shift** the characters in `s` from the index starti
to the index endi
(**inclusive**) forward if directioni = 1
, or shift the characters backward if directioni = 0
.
+
+Shifting a character **forward** means replacing it with the next letter in the alphabet (wrapping around so that `'z'` becomes `'a'`). Similarly, shifting a character **backward** means replacing it with the **previous** letter in the alphabet (wrapping around so that `'a'` becomes `'z'`).
+
+Return _the final string after all such shifts to `s` are applied_.
+
+**Example 1:**
+
+- **Input:** s = "abc", shifts = [[0,1,0],[1,2,1],[0,2,1]]
+- **Output:** "ace"
+- **Explanation:** Firstly, shift the characters from index 0 to index 1 backward. Now s = "zac".
+ Secondly, shift the characters from index 1 to index 2 forward. Now s = "zbd".
+ Finally, shift the characters from index 0 to index 2 forward. Now s = "ace".
+
+**Example 2:**
+
+- **Input:** s = "dztz", shifts = [[0,0,0],[1,1,1]]
+- **Output:** "catz"
+- **Explanation:** Firstly, shift the characters from index 0 to index 0 backward. Now s = "cztz".
+ Finally, shift the characters from index 1 to index 1 forward. Now s = "catz".
+
+
+
+**Constraints:**
+
+- 1 <= s.length, shifts.length <= 5 * 104
+- `shifts[i].length == 3`
+- 0 <= starti <= endi < s.length
+- 0 <= directioni <= 1
+- `s` consists of lowercase English letters.
+
+
+**Hint:**
+1. Instead of shifting every character in each shift, could you keep track of which characters are shifted and by how much across all shifts?
+2. Try marking the start and ends of each shift, then perform a prefix sum of the shifts.
+
+
+
+**Solution:**
+
+We need to avoid shifting the characters one by one for each shift, as this would be too slow for large inputs. Instead, we can use a more optimal approach by leveraging a technique called the **prefix sum**.
+
+### Steps:
+1. **Mark the shift boundaries**: Instead of shifting each character immediately, we mark the shift effects at the start and end of each range.
+2. **Apply prefix sum**: After marking all the shifts, we can compute the cumulative shifts at each character using the prefix sum technique. This allows us to efficiently apply the cumulative shifts to each character.
+3. **Perform the shifts**: Once we know the total shift for each character, we can apply the shifts (either forward or backward) to the string.
+
+Let's implement this solution in PHP: **[2381. Shifting Letters II](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/002381-shifting-letters-ii/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+1. For each shift `[start, end, direction]`, we'll increment a `shift` array at `start` and decrement at `end + 1`. This allows us to track the start and end of the shift range.
+2. After processing all the shifts, we apply a prefix sum on the `shift` array to get the cumulative shift at each index.
+3. Finally, we apply the cumulative shift to each character in the string.
+
+### Explanation of Code:
+1. **Input Parsing**: We convert the input string `s` into an array of characters for easier manipulation.
+2. **Shift Array**: We initialize a `shift` array of size `n + 1` to zero. This array is used to track the shift effects. For each shift `[start, end, direction]`, we adjust the values at `shift[start]` and `shift[end + 1]` to reflect the start and end of the shift.
+3. **Prefix Sum**: We calculate the total shift for each character by iterating over the `shift` array and maintaining a cumulative sum of shifts.
+4. **Character Shifting**: For each character in the string, we compute the final shifted character using the formula `(ord(currentChar) - ord('a') + totalShift) % 26`, which accounts for the circular nature of the alphabet.
+5. **Return Result**: The final string is obtained by converting the character array back into a string and returning it.
+
+### Time Complexity:
+- **Time complexity**: `O(n + m)`, where `n` is the length of the string `s` and `m` is the number of shifts. This is because we iterate through the string and the list of shifts once each.
+- **Space complexity**: `O(n)`, where `n` is the length of the string `s`, due to the space needed for the `shift` array.
+
+This solution efficiently handles the problem even with the upper limits of the input constraints.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
diff --git a/algorithms/002381-shifting-letters-ii/solution.php b/algorithms/002381-shifting-letters-ii/solution.php
new file mode 100644
index 000000000..052fd02c8
--- /dev/null
+++ b/algorithms/002381-shifting-letters-ii/solution.php
@@ -0,0 +1,40 @@
+[lefti, righti].
+
+You have to divide the intervals into one or more **groups** such that each interval is in **exactly** one group, and no two intervals that are in the same group **intersect** each other.
+
+Return _the **minimum** number of groups you need to make_.
+
+Two intervals **intersect** if there is at least one common number between them. For example, the intervals `[1, 5]` and `[5, 8]` intersect.
+
+**Example 1:**
+
+- **Input:** intervals = [[5,10],[6,8],[1,5],[2,3],[1,10]]
+- **Output:** 3
+- **Explanation:** We can divide the intervals into the following groups:
+ - Group 1: [1, 5], [6, 8].
+ - Group 2: [2, 3], [5, 10].
+ - Group 3: [1, 10].\
+ It can be proven that it is not possible to divide the intervals into fewer than 3 groups.
+
+**Example 2:**
+
+- **Input:** intervals = [[1,3],[5,6],[8,10],[11,13]]
+- **Output:** 1
+- **Explanation:** None of the intervals overlap, so we can put all of them in one group.
+
+**Constraints:**
+
+- 1 <= intervals.length <= 105
+- `intervals[i].length == 2`
+- 1 <= lefti <= righti <= 106
+
+
+**Hint:**
+1. Can you find a different way to describe the question?
+2. The minimum number of groups we need is equivalent to the maximum number of intervals that overlap at some point. How can you find that?
+
+
+
+**Solution:**
+
+To solve the problem of dividing intervals into the minimum number of groups such that no two intervals in the same group intersect, we can interpret it in a different way:
+
+### Explanation:
+The minimum number of groups needed is equivalent to the **maximum number of intervals that overlap at any point in time**. This approach is based on the fact that if multiple intervals overlap at a particular point, each one will require a separate group.
+
+### Approach:
+1. **Sorting Events:** We can convert each interval into two events:
+ - A start event (`+1`) at the `left` point.
+ - An end event (`-1`) at the `right + 1` point (to ensure the interval is closed).
+
+ These events will help track when intervals start and stop overlapping.
+
+2. **Sweep Line Algorithm:** Using a sweep line approach:
+ - Sort all events based on their time.
+ - Use a counter to track the number of ongoing intervals at each point.
+ - Keep track of the maximum count of overlapping intervals as we process each event.
+
+3. **Result:** The maximum count of overlapping intervals at any point is the minimum number of groups required.
+
+Let's implement this solution in PHP: **[2406. Divide Intervals Into Minimum Number of Groups](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/002406-divide-intervals-into-minimum-number-of-groups/solution.php)**
+
+```php
+
+```
+
+### Explanation of the Code:
+
+1. **Events Array:** We build an array of events where each interval contributes two events (`[start, +1]` and `[end+1, -1]`).
+2. **Sorting:** We sort these events. When two events have the same time, we prioritize ending intervals (`-1`) over starting intervals (`+1`).
+3. **Sweep Line Processing:** We iterate through sorted events, adjusting the `currentGroups` as intervals start or end:
+ - Increment for `+1` (start of an interval).
+ - Decrement for `-1` (end of an interval).
+4. **Track Maximum:** The `maxGroups` stores the peak number of simultaneous overlapping intervals, which is our answer.
+
+### Complexity:
+- **Time Complexity:** _**O(n log n)**_ due to sorting of events.
+- **Space Complexity:** _**O(n)**_ for storing the events.
+
+This solution efficiently determines the minimum number of groups required by finding the peak number of overlapping intervals.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
diff --git a/algorithms/002406-divide-intervals-into-minimum-number-of-groups/solution.php b/algorithms/002406-divide-intervals-into-minimum-number-of-groups/solution.php
new file mode 100644
index 000000000..7cdc3c6cc
--- /dev/null
+++ b/algorithms/002406-divide-intervals-into-minimum-number-of-groups/solution.php
@@ -0,0 +1,37 @@
+[1, 214].
+- 0 <= Node.val <= 105
+- `root` is a perfect binary tree.
+
+
+**Hint:**
+1. Try to solve recursively for each level independently.
+2. While performing a depth-first search, pass the left and right nodes (which should be paired) to the next level. If the current level is odd, then reverse their values, or else recursively move to the next level.
+
+
+
+**Solution:**
+
+We need to perform a depth-first traversal on the binary tree. The task is to reverse the node values at odd levels. A perfect binary tree means all non-leaf nodes have two children, and all leaf nodes are at the same level.
+
+We will use a DFS (Depth-First Search) approach, and on each odd level, we will reverse the node values. Below is the solution that accomplishes this.
+
+### Key Points:
+- The tree is perfect, meaning it is completely balanced, and all leaf nodes are at the same level.
+- Only nodes at odd levels need to be reversed. Odd levels are indexed starting from level 1 (1st, 3rd, 5th, etc.).
+- A DFS can be used to traverse the tree and identify the levels of nodes. When we encounter an odd level, we swap the values of the nodes at that level.
+- At each level, we traverse two child nodes: the left child and right child.
+
+### Approach:
+1. Perform a depth-first traversal of the tree.
+2. For each pair of nodes at the current level:
+ - If the level is odd, swap the node values.
+3. Recursively process the left and right children of the current node, passing the updated level information.
+4. Return the root node after processing the entire tree.
+
+### Plan:
+1. Start from the root node.
+2. Use a recursive function `dfs` to traverse the tree and reverse node values at odd levels.
+3. Keep track of the current level to identify odd levels.
+4. Swap values at odd levels and continue the DFS traversal for the children.
+5. Return the root after processing.
+
+### Solution Steps:
+1. Define a recursive function `dfs($left, $right, $isOddLevel)`:
+ - `left`: Left child node.
+ - `right`: Right child node.
+ - `isOddLevel`: Boolean indicating whether the current level is odd.
+2. Check if `left` is null. If it is, return, as there are no further nodes to process.
+3. If `isOddLevel` is true, swap the values of `left` and `right` nodes.
+4. Recursively call the `dfs` function for:
+ - Left child of `left` and right child of `right` (next level).
+ - Right child of `left` and left child of `right` (next level).
+5. Start the recursion with `dfs($root->left, $root->right, true)` and return the root.
+
+Let's implement this solution in PHP: **[2415. Reverse Odd Levels of Binary Tree](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/002415-reverse-odd-levels-of-binary-tree/solution.php)**
+
+```php
+val = $val;
+ $this->left = $left;
+ $this->right = $right;
+ }
+}
+
+class Solution {
+ /**
+ * @param TreeNode $root
+ * @return TreeNode
+ */
+ public function reverseOddLevels($root) {
+ ...
+ ...
+ ...
+ /**
+ * go to ./solution.php
+ */
+ }
+
+ /**
+ * Helper function to perform DFS
+ *
+ * @param $left
+ * @param $right
+ * @param $isOddLevel
+ * @return void
+ */
+ private function dfs($left, $right, $isOddLevel) {
+ ...
+ ...
+ ...
+ /**
+ * go to ./solution.php
+ */
+ }
+}
+
+// Example usage:
+$root = new TreeNode(2);
+$root->left = new TreeNode(3);
+$root->right = new TreeNode(5);
+$root->left->left = new TreeNode(8);
+$root->left->right = new TreeNode(13);
+$root->right->left = new TreeNode(21);
+$root->right->right = new TreeNode(34);
+
+$solution = new Solution();
+$reversedRoot = $solution->reverseOddLevels($root);
+
+// Function to print the tree for testing
+function printTree($root) {
+ if ($root === null) {
+ return;
+ }
+ echo $root->val . " ";
+ printTree($root->left);
+ printTree($root->right);
+}
+
+printTree($reversedRoot); // Output: 2 5 3 8 13 21 34
+?>
+```
+
+### Explanation:
+
+1. **TreeNode Class**: Represents the structure of a binary tree node, which has a value (`val`), and two children (`left`, `right`).
+2. **reverseOddLevels Function**: Initiates the DFS with the left and right child of the root node and starts at level 1 (odd level).
+3. **dfs Function**:
+ - Takes in two nodes (`left` and `right`) and a boolean `isOddLevel` to determine if the current level is odd.
+ - If the current level is odd, the values of `left` and `right` are swapped.
+ - Recursively calls itself for the next level, alternating the `isOddLevel` value (`true` becomes `false` and vice versa).
+4. The recursion proceeds to process the next pair of nodes at each level, ensuring only nodes at odd levels are reversed.
+
+### Example Walkthrough:
+
+**Example 1:**
+
+Input:
+```
+ 2
+ / \
+ 3 5
+ / \ / \
+ 8 13 21 34
+```
+
+- Level 0: `[2]` (even, no change).
+- Level 1: `[3, 5]` (odd, reverse to `[5, 3]`).
+- Level 2: `[8, 13, 21, 34]` (even, no change).
+
+Output:
+```
+ 2
+ / \
+ 5 3
+ / \ / \
+ 8 13 21 34
+```
+
+**Example 2:**
+
+Input:
+```
+ 7
+ / \
+ 13 11
+```
+
+- Level 0: `[7]` (even, no change).
+- Level 1: `[13, 11]` (odd, reverse to `[11, 13]`).
+
+Output:
+```
+ 7
+ / \
+ 11 13
+```
+
+### Time Complexity:
+- **Time Complexity**: O(n), where n is the number of nodes in the binary tree. We visit each node exactly once in a depth-first manner.
+- **Space Complexity**: O(h), where h is the height of the tree. The recursion depth corresponds to the height of the tree, and in the worst case (for a skewed tree), it would be O(n), but for a perfect binary tree, it's O(log n).
+
+This solution efficiently reverses the nodes at odd levels of a perfect binary tree using depth-first search with a time complexity of O(n). The code swaps values at odd levels and uses a recursive approach to process the tree.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
diff --git a/algorithms/002415-reverse-odd-levels-of-binary-tree/solution.php b/algorithms/002415-reverse-odd-levels-of-binary-tree/solution.php
new file mode 100644
index 000000000..1f85e15c1
--- /dev/null
+++ b/algorithms/002415-reverse-odd-levels-of-binary-tree/solution.php
@@ -0,0 +1,57 @@
+val = $val;
+ * $this->left = $left;
+ * $this->right = $right;
+ * }
+ * }
+ */
+class Solution {
+
+ /**
+ * @param TreeNode $root
+ * @return TreeNode
+ */
+ function reverseOddLevels($root) {
+ if ($root === null) {
+ return $root;
+ }
+
+ // Call the helper function with root's left and right children
+ $this->dfs($root->left, $root->right, true);
+ return $root;
+ }
+
+ /**
+ * Helper function to perform DFS
+ *
+ * @param $left
+ * @param $right
+ * @param $isOddLevel
+ * @return void
+ */
+ private function dfs($left, $right, $isOddLevel) {
+ // If we reach null nodes, return
+ if ($left == null) {
+ return;
+ }
+
+ // If it's an odd level, swap the values
+ if ($isOddLevel) {
+ $temp = $left->val;
+ $left->val = $right->val;
+ $right->val = $temp;
+ }
+
+ // Recursively move to the next level
+ $this->dfs($left->left, $right->right, !$isOddLevel);
+ $this->dfs($left->right, $right->left, !$isOddLevel);
+ }
+}
\ No newline at end of file
diff --git a/algorithms/002416-sum-of-prefix-scores-of-strings/README.md b/algorithms/002416-sum-of-prefix-scores-of-strings/README.md
new file mode 100644
index 000000000..298511402
--- /dev/null
+++ b/algorithms/002416-sum-of-prefix-scores-of-strings/README.md
@@ -0,0 +1,202 @@
+2416\. Sum of Prefix Scores of Strings
+
+**Difficulty:** Hard
+
+**Topics:** `Array`, `String`, `Trie`, `Counting`
+
+You are given an array `words` of size `n` consisting of **non-empty** strings.
+
+We define the **score** of a string `word` as the **number** of strings `words[i]` such that `word` is a **prefix** of `words[i]`.
+
+- For example, if `words = ["a", "ab", "abc", "cab"]`, then the score of `"ab"` is `2`, since `"ab"` is a prefix of both `"ab"` and `"abc"`.
+
+Return _an array `answer` of size `n` where `answer[i]` is the **sum** of scores of every **non-empty** prefix of `words[i]`_.
+
+**Note** that a string is considered as a prefix of itself.
+
+**Example 1:**
+
+- **Input:** words = ["abc","ab","bc","b"]
+- **Output:** [5,4,3,2]
+- **Explanation:** The answer for each string is the following:
+ - "abc" has 3 prefixes: "a", "ab", and "abc".
+ - There are 2 strings with the prefix "a", 2 strings with the prefix "ab", and 1 string with the prefix "abc".
+ - The total is answer[0] = 2 + 2 + 1 = 5.
+ - "ab" has 2 prefixes: "a" and "ab".
+ - There are 2 strings with the prefix "a", and 2 strings with the prefix "ab".
+ - The total is answer[1] = 2 + 2 = 4.
+ - "bc" has 2 prefixes: "b" and "bc".
+ - There are 2 strings with the prefix "b", and 1 string with the prefix "bc".
+ - The total is answer[2] = 2 + 1 = 3.
+ - "b" has 1 prefix: "b".
+ - There are 2 strings with the prefix "b".
+ - The total is answer[3] = 2.
+
+**Example 2:**
+
+- **Input:** words = ["abcd"]
+- **Output:** [4]
+- **Explanation:**
+ - "abcd" has 4 prefixes: "a", "ab", "abc", and "abcd".
+ - Each prefix has a score of one, so the total is answer[0] = 1 + 1 + 1 + 1 = 4.
+
+
+
+**Constraints:**
+
+- `1 <= words.length <= 1000`
+- `1 <= words[i].length <= 1000`
+- `words[i]` consists of lowercase English letters.
+
+
+
+**Hint:**
+1. What data structure will allow you to efficiently keep track of the score of each prefix?
+2. Use a Trie. Insert all the words into it, and keep a counter at each node that will tell you how many times we have visited each prefix.
+
+
+
+**Solution:**
+
+We can use a **Trie** data structure, which is particularly efficient for working with prefixes. Each node in the Trie will represent a letter of the words, and we'll maintain a counter at each node to store how many times that prefix has been encountered. This allows us to efficiently calculate the score of each prefix by counting how many words start with that prefix.
+
+### Approach:
+
+1. **Insert Words into Trie:**
+ - We'll insert each word into the Trie character by character.
+ - At each node (representing a character), we maintain a counter that keeps track of how many words pass through that prefix.
+
+2. **Calculate Prefix Scores:**
+ - For each word, as we traverse its prefixes in the Trie, we'll sum the counters stored at each node to compute the score for each prefix.
+
+3. **Build Answer Array:**
+ - For each word, we'll calculate the sum of the scores for all its prefixes and store it in the result array.
+
+Let's implement this solution in PHP: **[2416. Sum of Prefix Scores of Strings](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/002416-sum-of-prefix-scores-of-strings/solution.php)**
+
+```php
+children = [];
+ $this->count = 0;
+ }
+}
+
+class Trie {
+ /**
+ * @var TrieNode
+ */
+ private $root;
+
+ public function __construct() {
+ $this->root = new TrieNode();
+ }
+
+ /**
+ * Insert a word into the Trie and update the prefix counts
+ *
+ * @param $word
+ * @return void
+ */
+ public function insert($word) {
+ ...
+ ...
+ ...
+ /**
+ * go to ./solution.php
+ */
+ }
+
+ /**
+ * Get the sum of prefix scores for a given word
+ *
+ * @param $word
+ * @return int
+ */
+ public function getPrefixScores($word) {
+ ...
+ ...
+ ...
+ /**
+ * go to ./solution.php
+ */
+ }
+}
+
+/**
+ * @param String[] $words
+ * @return Integer[]
+ */
+function sumOfPrefixScores($words) {
+ ...
+ ...
+ ...
+ /**
+ * go to ./solution.php
+ */
+}
+
+// Example usage:
+$words1 = ["abc", "ab", "bc", "b"];
+$words2 = ["abcd"];
+
+print_r(sumOfPrefixScores($words1)); // Output: [5, 4, 3, 2]
+print_r(sumOfPrefixScores($words2)); // Output: [4]
+?>
+```
+
+### Explanation:
+
+1. **TrieNode Class:**
+ - Each node has an array of children (representing the next characters in the word) and a `count` to keep track of how many words share this prefix.
+
+2. **Trie Class:**
+ - The `insert` method adds a word to the Trie. As we insert each character, we increment the `count` at each node, representing how many words have this prefix.
+ - The `getPrefixScores` method calculates the sum of scores for all prefixes of a given word. It traverses through the Trie, adding up the `count` of each node corresponding to a character in the word.
+
+3. **Main Function (`sumOfPrefixScores`):**
+ - First, we insert all words into the Trie.
+ - Then, for each word, we calculate the sum of scores for its prefixes by querying the Trie and store the result in the `result` array.
+
+### Example:
+
+For `words = ["abc", "ab", "bc", "b"]`, the output will be:
+```
+Array
+(
+ [0] => 5
+ [1] => 4
+ [2] => 3
+ [3] => 2
+)
+```
+
+- "abc" has 3 prefixes: "a" (2 words), "ab" (2 words), "abc" (1 word) -> total = 5.
+- "ab" has 2 prefixes: "a" (2 words), "ab" (2 words) -> total = 4.
+- "bc" has 2 prefixes: "b" (2 words), "bc" (1 word) -> total = 3.
+- "b" has 1 prefix: "b" (2 words) -> total = 2.
+
+### Time Complexity:
+- **Trie Construction:** O(n * m) where `n` is the number of words and `m` is the average length of the words.
+- **Prefix Score Calculation:** O(n * m) as we traverse each word's prefix in the Trie.
+
+This approach ensures that we efficiently compute the prefix scores in linear time relative to the total number of characters in all words.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
diff --git a/algorithms/002416-sum-of-prefix-scores-of-strings/solution.php b/algorithms/002416-sum-of-prefix-scores-of-strings/solution.php
new file mode 100644
index 000000000..4c4d7fbbd
--- /dev/null
+++ b/algorithms/002416-sum-of-prefix-scores-of-strings/solution.php
@@ -0,0 +1,88 @@
+insert($word);
+ }
+
+ // Step 2: Calculate prefix scores for each word
+ $result = [];
+ foreach ($words as $word) {
+ $result[] = $trie->getPrefixScores($word);
+ }
+
+ return $result;
+ }
+}
+
+class TrieNode {
+ /**
+ * @var array
+ */
+ public $children;
+ /**
+ * @var int
+ */
+ public $count;
+
+ public function __construct() {
+ $this->children = [];
+ $this->count = 0;
+ }
+}
+
+class Trie {
+ /**
+ * @var TrieNode
+ */
+ private $root;
+
+ public function __construct() {
+ $this->root = new TrieNode();
+ }
+
+ /**
+ * Insert a word into the Trie and update the prefix counts
+ *
+ * @param $word
+ * @return void
+ */
+ public function insert($word) {
+ $node = $this->root;
+ foreach (str_split($word) as $char) {
+ if (!isset($node->children[$char])) {
+ $node->children[$char] = new TrieNode();
+ }
+ $node = $node->children[$char];
+ $node->count++; // Increase the count at this node
+ }
+ }
+
+ /**
+ * Get the sum of prefix scores for a given word
+ *
+ * @param $word
+ * @return int
+ */
+ public function getPrefixScores($word) {
+ $node = $this->root;
+ $prefixScore = 0;
+ foreach (str_split($word) as $char) {
+ if (!isset($node->children[$char])) {
+ break;
+ }
+ $node = $node->children[$char];
+ $prefixScore += $node->count;
+ }
+ return $prefixScore;
+ }
+}
diff --git a/algorithms/002419-longest-subarray-with-maximum-bitwise-and/README.md b/algorithms/002419-longest-subarray-with-maximum-bitwise-and/README.md
new file mode 100644
index 000000000..da1453c8c
--- /dev/null
+++ b/algorithms/002419-longest-subarray-with-maximum-bitwise-and/README.md
@@ -0,0 +1,120 @@
+2419\. Longest Subarray With Maximum Bitwise AND
+
+**Difficulty:** Medium
+
+**Topics:** `Array`, `Bit Manipulation`, `Brainteaser`
+
+You are given an integer array `nums` of size `n`.
+
+Consider a **non-empty** subarray from nums that has the **maximum** possible **bitwise AND**.
+
+- In other words, let `k` be the maximum value of the bitwise AND of **any** subarray of `nums`. Then, only subarrays with a bitwise AND equal to `k` should be considered.
+
+Return _the length of the **longest** such subarray_.
+
+The bitwise AND of an array is the bitwise AND of all the numbers in it.
+
+A **subarray** is a contiguous sequence of elements within an array.
+
+**Example 1:**
+
+- **Input:** nums = [1,2,3,3,2,2]
+- **Output:** 2
+- **Explanation:**
+ The maximum possible bitwise AND of a subarray is 3.\
+ The longest subarray with that value is [3,3], so we return 2.
+
+**Example 2:**
+
+- **Input:** nums = [1,2,3,4]
+- **Output:** 1
+- **Explanation:**
+ The maximum possible bitwise AND of a subarray is 4.\
+ The longest subarray with that value is [4], so we return 1.
+
+**Constraints:**
+
+- 1 <= nums.length <= 101
+- 1 <= nums[i] <= 106
+
+
+**Hint:**
+1. Notice that the bitwise AND of two different numbers will always be strictly less than the maximum of those two numbers.
+2. What does that tell us about the nature of the subarray that we should choose?
+
+
+
+**Solution:**
+
+Let's first break down the problem step by step:
+
+### Key Insights:
+1. **Bitwise AND Properties**:
+ - The bitwise AND of two numbers is generally smaller than or equal to both numbers.
+ - Therefore, if we find a maximum value in the array, the subarray that will achieve this maximum bitwise AND value must consist of this maximum value repeated.
+
+2. **Objective**:
+ - Find the maximum value in the array.
+ - Find the longest contiguous subarray of that maximum value, because any other number in the subarray would reduce the overall bitwise AND result.
+
+### Plan:
+1. Traverse the array and determine the maximum value.
+2. Traverse the array again to find the longest contiguous subarray where all elements are equal to this maximum value.
+
+### Example:
+For the input array `[1,2,3,3,2,2]`, the maximum value is `3`. The longest contiguous subarray with only `3`s is `[3,3]`, which has a length of 2.
+
+Let's implement this solution in PHP: **[2419. Longest Subarray With Maximum Bitwise AND](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/002419-longest-subarray-with-maximum-bitwise-and/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+1. **Step 1**: We first find the maximum value in the array using PHP's built-in `max()` function.
+2. **Step 2**: We initialize two variables, `$maxLength` to store the length of the longest subarray and `$currentLength` to track the length of the current contiguous subarray of the maximum value.
+3. **Step 3**: We iterate through the array:
+ - If the current number equals the maximum value, we increment the length of the current subarray.
+ - If the current number does not equal the maximum value, we check if the current subarray is the longest so far and reset the length.
+4. **Final Step**: After the loop, we ensure that if the longest subarray is at the end of the array, we still consider it.
+5. Finally, we return the length of the longest subarray that contains only the maximum value.
+
+### Time Complexity:
+- Finding the maximum value takes \(O(n)\).
+- Traversing the array to find the longest subarray takes \(O(n)\).
+- Overall time complexity: \(O(n)\), where \(n\) is the length of the array.
+
+### Test Cases:
+For the input `[1, 2, 3, 3, 2, 2]`, the output is `2`, and for `[1, 2, 3, 4]`, the output is `1`, as expected.
+
+This solution handles the constraints and efficiently solves the problem.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
diff --git a/algorithms/002419-longest-subarray-with-maximum-bitwise-and/solution.php b/algorithms/002419-longest-subarray-with-maximum-bitwise-and/solution.php
new file mode 100644
index 000000000..15371dfb8
--- /dev/null
+++ b/algorithms/002419-longest-subarray-with-maximum-bitwise-and/solution.php
@@ -0,0 +1,41 @@
+ $maxLength) {
+ $maxLength = $currentLength;
+ }
+ // Reset the current length since we encountered a different number
+ $currentLength = 0;
+ }
+ }
+
+ // Final check at the end in case the longest subarray is at the end of the array
+ if ($currentLength > $maxLength) {
+ $maxLength = $currentLength;
+ }
+
+ // Return the longest length found
+ return $maxLength;
+
+ }
+}
\ No newline at end of file
diff --git a/algorithms/002425-bitwise-xor-of-all-pairings/README.md b/algorithms/002425-bitwise-xor-of-all-pairings/README.md
new file mode 100644
index 000000000..c953f4334
--- /dev/null
+++ b/algorithms/002425-bitwise-xor-of-all-pairings/README.md
@@ -0,0 +1,128 @@
+2425\. Bitwise XOR of All Pairings
+
+**Difficulty:** Medium
+
+**Topics:** `Array`, `Bit Manipulation`, `Brainteaser`
+
+You are given two **0-indexed** arrays, `nums1` and `nums2`, consisting of non-negative integers. There exists another array, `nums3`, which contains the bitwise XOR of **all pairings** of integers between `nums1` and `nums2` (every integer in `nums1` is paired with every integer in `nums2` **exactly once**).
+
+Return _the **bitwise XOR** of all integers in `nums3`_.
+
+**Example 1:**
+
+- **Input:** nums1 = [2,1,3], nums2 = [10,2,5,0]
+- **Output:** 13
+- **Explanation:**
+ A possible nums3 array is [8,0,7,2,11,3,4,1,9,1,6,3].
+ The bitwise XOR of all these numbers is 13, so we return 13.
+
+**Example 2:**
+
+- **Input:** nums1 = [1,2], nums2 = [3,4]
+- **Output:** 0
+- **Explanation:**
+ All possible pairs of bitwise XORs are nums1[0] ^ nums2[0], nums1[0] ^ nums2[1], nums1[1] ^ nums2[0], and nums1[1] ^ nums2[1].
+ Thus, one possible nums3 array is [2,5,1,6].
+ 2 ^ 5 ^ 1 ^ 6 = 0, so we return 0.
+
+
+
+**Constraints:**
+
+- 1 <= nums1.length, nums2.length <= 105
+- 0 <= nums1[i], nums2[j] <= 109
+
+
+**Hint:**
+1. Think how the count of each individual integer affects the final answer.
+2. If the length of nums1 is m and the length of nums2 is n, then each number in nums1 is repeated n times and each number in nums2 is repeated m times.
+
+
+
+**Solution:**
+
+We need to think carefully about how the bitwise XOR operates and how the pairing of elements in `nums1` and `nums2` affects the result.
+
+### Key Observations:
+1. Each element in `nums1` pairs with every element in `nums2`, and vice versa.
+2. In `nums3`, each number from `nums1` and `nums2` appears multiple times:
+ - Each number from `nums1` appears `n` times (where `n` is the length of `nums2`).
+ - Each number from `nums2` appears `m` times (where `m` is the length of `nums1`).
+
+3. The XOR operation has a property that if a number appears an even number of times, it will cancel out (because `x ^ x = 0`), and if it appears an odd number of times, it contributes to the final XOR result.
+
+### Approach:
+- For each bit position (from 0 to 31, since `nums1[i]` and `nums2[j]` are at most `10^9`), we will calculate how many times this bit is set (i.e., `1`) in the XOR result of all pairings.
+- Each number in `nums1` contributes to the final XOR `n` times, and each number in `nums2` contributes to the final XOR `m` times.
+
+Using these observations, we can reduce the problem to counting the number of times each bit is set (odd number of times) across all pairings.
+
+### Plan:
+1. Count the number of `1`s in each bit position for `nums1` and `nums2`.
+2. For each bit, check whether the total count of `1`s (from both `nums1` and `nums2`) for that bit is odd or even.
+3. If the count is odd, set that bit in the final XOR result.
+
+Let's implement this solution in PHP: **[2425. Bitwise XOR of All Pairings](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/002425-bitwise-xor-of-all-pairings/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+- **`$m` and `$n`:** Store the lengths of `nums1` and `nums2` respectively.
+- **`$result`:** This variable will store the final XOR result.
+- **Loop over 32 bits:** Since each number is at most `10^9`, we only need to consider the first 32 bits.
+- **Counting bits:** For each bit position, we count how many times this bit is set in `nums1` and `nums2`.
+- **Calculate total counts:** Each bit from `nums1` contributes `n` times to the total count, and each bit from `nums2` contributes `m` times.
+- **XOR result update:** If the total count for a particular bit is odd, we update the result to set that bit.
+
+### Complexity:
+- **Time Complexity**: _**O(m + n)**_, where _**m**_ and _**n**_ are the lengths of `nums1` and `nums2`, respectively.
+- **Space Complexity**: _**O(1)**_, as we use constant extra space.
+
+### Example Walkthrough:
+
+**Example 1:**
+```php
+$nums1 = [2, 1, 3];
+$nums2 = [10, 2, 5, 0];
+echo xorAllPairings($nums1, $nums2); // Output: 13
+```
+
+**Example 2:**
+```php
+$nums1 = [1, 2];
+$nums2 = [3, 4];
+echo xorAllPairings($nums1, $nums2); // Output: 0
+```
+
+This solution is efficient and works within the problem's constraints.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
diff --git a/algorithms/002425-bitwise-xor-of-all-pairings/solution.php b/algorithms/002425-bitwise-xor-of-all-pairings/solution.php
new file mode 100644
index 000000000..93f4c08a4
--- /dev/null
+++ b/algorithms/002425-bitwise-xor-of-all-pairings/solution.php
@@ -0,0 +1,45 @@
+> $bit) & 1) {
+ $count1++;
+ }
+ }
+
+ // Count 1's in nums2 for the $bit-th position
+ foreach ($nums2 as $num) {
+ if (($num >> $bit) & 1) {
+ $count2++;
+ }
+ }
+
+ // Calculate the number of 1's in the $bit-th position in nums3
+ $totalCount = $count1 * $n + $count2 * $m;
+
+ // If totalCount is odd, set the $bit-th bit in result
+ if ($totalCount % 2 == 1) {
+ $result |= (1 << $bit);
+ }
+ }
+
+ return $result;
+ }
+}
\ No newline at end of file
diff --git a/algorithms/002429-minimize-xor/README.md b/algorithms/002429-minimize-xor/README.md
new file mode 100644
index 000000000..fd2e238e5
--- /dev/null
+++ b/algorithms/002429-minimize-xor/README.md
@@ -0,0 +1,140 @@
+2429\. Minimize XOR
+
+**Difficulty:** Medium
+
+**Topics:** `Greedy`, `Bit Manipulation`
+
+Given two positive integers `num1` and `num2`, find the positive integer `x` such that:
+
+- `x` has the same number of set bits as `num2`, and
+- The value `x XOR num1` is **minimal**.
+
+**Note** that `XOR` is the bitwise XOR operation.
+
+Return _the integer `x`. The test cases are generated such that `x` is **uniquely determined**_.
+
+The number of **set bits** of an integer is the number of `1`'s in its binary representation.
+
+**Example 1:**
+
+- **Input:** num1 = 3, num2 = 5
+- **Output:** 3
+- **Explanation:** The binary representations of num1 and num2 are 0011 and 0101, respectively.
+ The integer 3 has the same number of set bits as num2, and the value 3 XOR 3 = 0 is minimal.
+
+**Example 2:**
+
+- **Input:** num1 = 1, num2 = 12
+- **Output:** 3
+- **Explanation:** The binary representations of num1 and num2 are 0001 and 1100, respectively.
+ The integer 3 has the same number of set bits as num2, and the value 3 XOR 1 = 2 is minimal.
+
+
+
+**Constraints:**
+- 1 <= num1, num2 <= 109
+
+**Hint:**
+1. To arrive at a small xor, try to turn off some bits from num1
+2. If there are still left bits to set, try to set them from the least significant bit
+
+
+
+**Solution:**
+
+The idea is to manipulate the bits of `num1` and match the number of set bits (`1`s) in `num2` while minimizing the XOR result. Here's the step-by-step approach:
+
+### Steps:
+1. **Count the Set Bits in `num2`:**
+ - Find the number of `1`s in the binary representation of `num2`. Let's call this `setBitsCount`.
+
+2. **Create a Result Number `x`:**
+ - Start with `x = 0`.
+ - From the binary representation of `num1`, preserve the `1`s in the most significant positions that match `setBitsCount`.
+ - If there are not enough `1`s in `num1`, add extra `1`s starting from the least significant bit.
+
+3. **Optimize XOR Result:**
+ - By aligning the set bits of `x` with the most significant `1`s of `num1`, the XOR value will be minimized.
+
+4. **Return the Result:**
+ - Return the computed value of `x`.
+
+Let's implement this solution in PHP: **[2429. Minimize XOR](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/002429-minimize-xor/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+1. **`countSetBits` Function:**
+ - This function counts the number of `1`s in the binary representation of a number using a loop.
+
+2. **Building `x`:**
+ - First, the most significant `1`s from `num1` are retained in `x` to minimize the XOR value.
+ - If more `1`s are required to match `setBitsCount`, they are added starting from the least significant bit (to keep the XOR minimal).
+
+3. **Optimization:**
+ - The XOR value is minimized by aligning the significant bits as much as possible between `num1` and `x`.
+
+### Complexity:
+- **Time Complexity:** _**O(32)**_, since the loop iterates a constant number of times (32 bits for integers).
+- **Space Complexity:** _**O(1)**_, as no extra space is used apart from variables.
+
+### Example Walkthrough:
+#### Example 1:
+- `num1 = 3 (0011)` and `num2 = 5 (0101)`.
+- `setBitsCount = 2`.
+- `x` starts as `0`.
+- From `num1`, keep two most significant `1`s: `x = 3 (0011)`.
+- `x XOR num1 = 0`, which is minimal.
+
+#### Example 2:
+- `num1 = 1 (0001)` and `num2 = 12 (1100)`.
+- `setBitsCount = 2`.
+- From `num1`, retain `1` and add one more bit to `x`: `x = 3 (0011)`.
+- `x XOR num1 = 2`, which is minimal.
+
+This ensures the correct and efficient computation of `x`.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
diff --git a/algorithms/002429-minimize-xor/solution.php b/algorithms/002429-minimize-xor/solution.php
new file mode 100644
index 000000000..cd515b556
--- /dev/null
+++ b/algorithms/002429-minimize-xor/solution.php
@@ -0,0 +1,50 @@
+countSetBits($num2);
+
+ // Create the result x
+ $x = 0;
+
+ // Preserve the most significant bits from num1
+ for ($i = 31; $i >= 0 && $setBitsCount > 0; $i--) {
+ if (($num1 & (1 << $i)) != 0) {
+ $x |= (1 << $i);
+ $setBitsCount--;
+ }
+ }
+
+ // If there are still bits to set, set them from the least significant bit
+ for ($i = 0; $i <= 31 && $setBitsCount > 0; $i++) {
+ if (($x & (1 << $i)) == 0) {
+ $x |= (1 << $i);
+ $setBitsCount--;
+ }
+ }
+
+ return $x;
+ }
+
+ /**
+ * Helper function to count the number of set bits in a number
+ *
+ * @param $num
+ * @return int
+ */
+ function countSetBits($num) {
+ $count = 0;
+ while ($num > 0) {
+ $count += $num & 1;
+ $num >>= 1;
+ }
+ return $count;
+ }
+}
\ No newline at end of file
diff --git a/algorithms/002458-height-of-binary-tree-after-subtree-removal-queries/README.md b/algorithms/002458-height-of-binary-tree-after-subtree-removal-queries/README.md
new file mode 100644
index 000000000..15bcf9cf3
--- /dev/null
+++ b/algorithms/002458-height-of-binary-tree-after-subtree-removal-queries/README.md
@@ -0,0 +1,193 @@
+2458\. Height of Binary Tree After Subtree Removal Queries
+
+**Difficulty:** Hard
+
+**Topics:** `Array`, `Tree`, `Depth-First Search`, `Breadth-First Search`, `Binary Tree`
+
+You are given the `root` of a **binary tree** with `n` nodes. Each node is assigned a unique value from `1` to `n`. You are also given an array `queries` of size `m`.
+
+You have to perform `m` **independent** queries on the tree where in the ith
query you do the following:
+
+- **Remove** the subtree rooted at the node with the value `queries[i]` from the tree. It is **guaranteed** that `queries[i]` will **not** be equal to the value of the root.
+
+Return _an array `answer` of size `m` where `answer[i]` is the height of the tree after performing the ith
query_.
+
+**Note:**
+
+- The queries are independent, so the tree returns to its **initial** state after each query.
+- The height of a tree is the **number of edges in the longest simple path** from the root to some node in the tree.
+
+
+**Example 1:**
+
+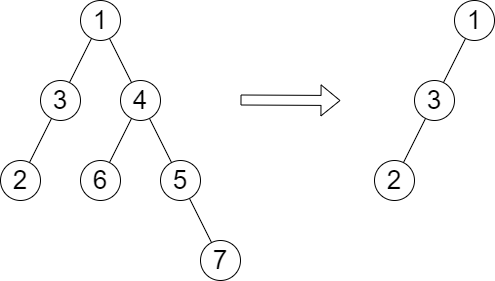
+
+- **Input:** root = [1,3,4,2,null,6,5,null,null,null,null,null,7], queries = [4]
+- **Output:** [2]
+- **Explanation:** The diagram above shows the tree after removing the subtree rooted at node with value 4.\
+ The height of the tree is 2 (The path 1 -> 3 -> 2).
+
+**Example 2:**
+
+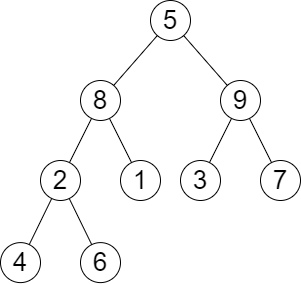
+
+- **Input:** root = [5,8,9,2,1,3,7,4,6], queries = [3,2,4,8]
+- **Output:** [3,2,3,2]
+- **Explanation:** We have the following queries:
+ - Removing the subtree rooted at node with value 3. The height of the tree becomes 3 (The path 5 -> 8 -> 2 -> 4).
+ - Removing the subtree rooted at node with value 2. The height of the tree becomes 2 (The path 5 -> 8 -> 1).
+ - Removing the subtree rooted at node with value 4. The height of the tree becomes 3 (The path 5 -> 8 -> 2 -> 6).
+ - Removing the subtree rooted at node with value 8. The height of the tree becomes 2 (The path 5 -> 9 -> 3).
+
+
+**Constraints:**
+
+- The number of nodes in the tree is `n`.
+- 2 <= n <= 105
+- `1 <= Node.val <= n`
+- All the values in the tree are **unique**.
+- `m == queries.length`
+- 1 <= m <= min(n, 104)
+- `1 <= queries[i] <= n`
+- `queries[i] != root.val`
+
+
+**Hint:**
+1. Try pre-computing the answer for each node from 1 to n, and answer each query in O(1).
+2. The answers can be precomputed in a single tree traversal after computing the height of each subtree.
+
+
+
+**Solution:**
+
+The solution employs a two-pass approach:
+1. **Calculate the height of each node** in the tree.
+2. **Determine the maximum height** of the tree after removing the subtree rooted at each queried node.
+
+Let's implement this solution in PHP: **[2458. Height of Binary Tree After Subtree Removal Queries](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/002458-height-of-binary-tree-after-subtree-removal-queries/solution.php)**
+
+#### Code Breakdown
+
+**1. Class Definition and Properties:**
+```php
+class Solution {
+
+ private $valToMaxHeight = [];
+ private $valToHeight = [];
+```
+- `valToMaxHeight`: This array will store the maximum height of the tree after removing each node's subtree.
+- `valToHeight`: This array will store the height of each node's subtree.
+
+**2. Main Function:**
+```php
+function treeQueries($root, $queries) {
+ ...
+ ...
+ ...
+ /**
+ * go to ./solution.php
+ */
+}
+```
+- The function `treeQueries` takes the `root` of the tree and the `queries` array.
+- It first calls the `height` function to compute the height of each node.
+- Then, it calls the `dfs` (depth-first search) function to compute the maximum heights after subtree removals.
+- Finally, it populates the `answer` array with the results for each query.
+
+**3. Height Calculation:**
+```php
+private function height($node) {
+ ...
+ ...
+ ...
+ /**
+ * go to ./solution.php
+ */
+}
+```
+- This function computes the height of each node recursively.
+- If the node is `null`, it returns 0.
+- If the height of the node is already computed, it retrieves it from the cache (`valToHeight`).
+- It calculates the height based on the heights of its left and right children and stores it in `valToHeight`.
+
+**4. Depth-First Search for Maximum Height:**
+```php
+private function dfs($node, $depth, $maxHeight) {
+ ...
+ ...
+ ...
+ /**
+ * go to ./solution.php
+ */
+}
+```
+- This function performs a DFS to compute the maximum height of the tree after each node's subtree is removed.
+- It records the current maximum height in `valToMaxHeight` for the current node.
+- It calculates the heights of the left and right subtrees and updates the maximum height accordingly.
+- It recursively calls itself for the left and right children, updating the depth and maximum height.
+
+### Example Walkthrough
+
+Let's go through an example step-by-step.
+
+**Example Input:**
+```php
+// Tree Structure
+// 1
+// / \
+// 3 4
+// / / \
+// 2 6 5
+// \
+// 7
+
+$root = [1, 3, 4, 2, null, 6, 5, null, null, null, null, null, 7];
+$queries = [4];
+```
+
+**Initial Height Calculation:**
+- The height of the tree rooted at 1: `3`
+- The height of the tree rooted at 3: `2`
+- The height of the tree rooted at 4: `2` (height of subtrees rooted at 6 and 5)
+- The height of the tree rooted at 6: `1` (just node 7)
+- The height of the tree rooted at 2: `0` (leaf node)
+
+After the height computation, `valToHeight` would look like this:
+```php
+$valToHeight = [
+ 1 => 3,
+ 2 => 0,
+ 3 => 2,
+ 4 => 2,
+ 5 => 0,
+ 6 => 1,
+ 7 => 0
+];
+```
+
+**DFS for Max Heights:**
+- For the root (1), removing subtree 4 leaves:
+ - Left Height: 2 (rooted at 3)
+ - Right Height: 1 (rooted at 5)
+- Thus, the maximum height after removing 4 is `2`.
+
+**Result Array After Queries:**
+- For the query `4`, the result would be `[2]`.
+
+### Final Output
+The result for the input provided will be:
+```php
+// Output
+[2]
+```
+
+This structured approach ensures that we efficiently compute the necessary heights and answer each query in constant time after the initial preprocessing. The overall complexity is _**O(n + m)**_, where _**n**_ is the number of nodes in the tree and _**m**_ is the number of queries.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
diff --git a/algorithms/002458-height-of-binary-tree-after-subtree-removal-queries/solution.php b/algorithms/002458-height-of-binary-tree-after-subtree-removal-queries/solution.php
new file mode 100644
index 000000000..4d8a4713a
--- /dev/null
+++ b/algorithms/002458-height-of-binary-tree-after-subtree-removal-queries/solution.php
@@ -0,0 +1,84 @@
+val = $val;
+ * $this->left = $left;
+ * $this->right = $right;
+ * }
+ * }
+ */
+
+class Solution {
+
+ private $valToMaxHeight = [];
+ private $valToHeight = [];
+
+ /**
+ * @param TreeNode $root
+ * @param Integer[] $queries
+ * @return Integer[]
+ */
+ function treeQueries($root, $queries) {
+ $this->height($root);
+ $this->dfs($root, 0, 0);
+
+ $answer = [];
+ foreach ($queries as $query) {
+ $answer[] = $this->valToMaxHeight[$query];
+ }
+
+ return $answer;
+ }
+
+ /**
+ * Calculate the height of each node and store it in $valToHeight
+ *
+ * @param $node
+ * @return int|mixed
+ */
+ private function height($node) {
+ if ($node === null) {
+ return 0;
+ }
+
+ if (isset($this->valToHeight[$node->val])) {
+ return $this->valToHeight[$node->val];
+ }
+
+ $leftHeight = $this->height($node->left);
+ $rightHeight = $this->height($node->right);
+
+ $this->valToHeight[$node->val] = 1 + max($leftHeight, $rightHeight);
+ return $this->valToHeight[$node->val];
+ }
+
+ /**
+ * Depth-first traversal to calculate the max height of the tree without each node
+ *
+ * @param $node
+ * @param $depth
+ * @param $maxHeight
+ * @return void
+ */
+ private function dfs($node, $depth, $maxHeight) {
+ if ($node === null) {
+ return;
+ }
+
+ $this->valToMaxHeight[$node->val] = $maxHeight;
+
+ // Update heights for left and right subtree
+ $leftHeight = isset($this->valToHeight[$node->right->val]) ? $this->valToHeight[$node->right->val] : 0;
+ $rightHeight = isset($this->valToHeight[$node->left->val]) ? $this->valToHeight[$node->left->val] : 0;
+
+ // Recursive DFS call for left and right subtrees
+ $this->dfs($node->left, $depth + 1, max($maxHeight, $depth + $leftHeight));
+ $this->dfs($node->right, $depth + 1, max($maxHeight, $depth + $rightHeight));
+ }
+}
\ No newline at end of file
diff --git a/algorithms/002461-maximum-sum-of-distinct-subarrays-with-length-k/README.md b/algorithms/002461-maximum-sum-of-distinct-subarrays-with-length-k/README.md
new file mode 100644
index 000000000..97162b66f
--- /dev/null
+++ b/algorithms/002461-maximum-sum-of-distinct-subarrays-with-length-k/README.md
@@ -0,0 +1,130 @@
+2461\. Maximum Sum of Distinct Subarrays With Length K
+
+**Difficulty:** Medium
+
+**Topics:** `Array`, `Hash Table`, `Sliding Window`
+
+You are given an integer array `nums` and an integer `k`. Find the maximum subarray sum of all the **subarrays** of `nums` that meet the following conditions:
+
+- The length of the subarray is `k`, and
+- All the elements of the subarray are **distinct**.
+
+Return _the maximum subarray sum of all the **subarrays** that meet the conditions_. If no subarray meets the conditions, return _`0`_.
+
+_A **subarray** is a contiguous non-empty sequence of elements within an array_.
+
+**Example 1:**
+
+- **Input:** nums = [1,5,4,2,9,9,9], k = 3
+- **Output:** 15
+- **Explanation:** The **subarrays** of nums with length `3` are:
+ - [1,5,4] which meets the requirements and has a sum of `10`.
+ - [5,4,2] which meets the requirements and has a sum of `11`.
+ - [4,2,9] which meets the requirements and has a sum of `15`.
+ - [2,9,9] which does not meet the requirements because the element `9` is repeated.
+ - [9,9,9] which does not meet the requirements because the element `9` is repeated.
+ We return `15` because it is the maximum subarray sum of all the **subarrays** that meet the conditions
+
+**Example 2:**
+
+- **Input:** nums = [4,4,4], k = 3
+- **Output:** 0
+- **Explanation:** The **subarrays** of nums with length `3` are:
+ - `[4,4,4]` which does not meet the requirements because the element `4` is repeated.
+ We return `0` because no **subarrays** meet the conditions.
+
+
+
+**Constraints:**
+
+- 1 <= k <= nums.length <= 105
+- 1 <= nums[i] <= 105
+
+
+**Hint:**
+1. Which elements change when moving from the subarray of size `k` that ends at index `i` to the subarray of size `k` that ends at index `i + 1`?
+2. Only two elements change, the element at `i + 1` is added into the subarray, and the element at `i - k + 1` gets removed from the subarray.
+3. Iterate through each subarray of size k and keep track of the sum of the subarray and the frequency of each element.
+
+
+**Solution:**
+
+We can follow these steps:
+
+### Approach:
+1. **Sliding Window**: The window size is `k`, and we slide the window through the array while maintaining the sum of the current window and checking if all elements in the window are distinct.
+2. **Hash Table (or associative array)**: Use an associative array (hash table) to track the frequency of elements in the current window. If any element appears more than once, the window is invalid.
+3. **Updating the Window**: As we slide the window, add the new element (i.e., the element coming into the window), and remove the old element (i.e., the element leaving the window). Update the sum accordingly and check if the window is valid (i.e., all elements are distinct).
+4. **Return the Maximum Sum**: We need to keep track of the maximum sum encountered among valid subarrays.
+
+### Algorithm:
+1. Initialize a hash table `freq` to store the frequency of elements in the current window.
+2. Start by calculating the sum for the first window of size `k` and store the result if the window contains distinct elements.
+3. Slide the window from left to right by:
+ - Removing the element that leaves the window from the left.
+ - Adding the element that enters the window from the right.
+ - Update the sum and the hash table, and check if the window still contains only distinct elements.
+4. If the window has valid distinct elements, update the maximum sum.
+5. If no valid subarray is found, return `0`.
+
+Let's implement this solution in PHP: **[2461. Maximum Sum of Distinct Subarrays With Length K](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/002461-maximum-sum-of-distinct-subarrays-with-length-k/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+1. **Variables**:
+ - `$maxSum`: Tracks the maximum sum of a valid subarray found so far.
+ - `$currentSum`: Holds the sum of the current sliding window of size `k`.
+ - `$freq`: A hash table (or associative array) that stores the frequency of elements in the current window.
+
+2. **Sliding Window**:
+ - We iterate through the array with a loop. As we move the window, we:
+ - Add the element at index `$i` to the sum and update its frequency.
+ - If the window size exceeds `k`, we remove the element at index `$i - k` from the sum and reduce its frequency.
+
+3. **Valid Window Check**:
+ - Once the window size reaches `k` (i.e., when `$i >= k - 1`), we check if all elements in the window are distinct by ensuring that the number of distinct keys in the frequency map is equal to `k`. If it is, we update the maximum sum.
+
+4. **Return the Result**:
+ - After iterating through the array, we return the maximum sum found. If no valid subarray was found, `maxSum` will remain `0`.
+
+### Time Complexity:
+- **O(n)**, where `n` is the length of the `nums` array. Each element is added and removed from the sliding window once, and the hash table operations (insert, remove, check count) are O(1) on average.
+
+### Space Complexity:
+- **O(k)** for the hash table that stores the frequency of elements in the current window.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
diff --git a/algorithms/002461-maximum-sum-of-distinct-subarrays-with-length-k/solution.php b/algorithms/002461-maximum-sum-of-distinct-subarrays-with-length-k/solution.php
new file mode 100644
index 000000000..0f0729b61
--- /dev/null
+++ b/algorithms/002461-maximum-sum-of-distinct-subarrays-with-length-k/solution.php
@@ -0,0 +1,40 @@
+= $k) {
+ $currentSum -= $nums[$i - $k];
+ $freq[$nums[$i - $k]]--;
+ if ($freq[$nums[$i - $k]] === 0) {
+ unset($freq[$nums[$i - $k]]);
+ }
+ }
+
+ // If the current window has exactly k elements and all are distinct
+ if ($i >= $k - 1) {
+ if (count($freq) === $k) {
+ $maxSum = max($maxSum, $currentSum);
+ }
+ }
+ }
+
+ return $maxSum;
+ }
+}
\ No newline at end of file
diff --git a/algorithms/002463-minimum-total-distance-traveled/README.md b/algorithms/002463-minimum-total-distance-traveled/README.md
new file mode 100644
index 000000000..c2f379ca0
--- /dev/null
+++ b/algorithms/002463-minimum-total-distance-traveled/README.md
@@ -0,0 +1,137 @@
+2463\. Minimum Total Distance Traveled
+
+**Difficulty:** Hard
+
+**Topics:** `Array`, `Dynamic Programming`, `Sorting`
+
+There are some robots and factories on the X-axis. You are given an integer array `robot` where `robot[i]` is the position of the ith
robot. You are also given a 2D integer array factory where factory[j] = [positionj, limitj]
indicates that positionj
is the position of the jth
factory and that the jth
factory can repair at most limitj
robots.
+
+The positions of each robot are **unique**. The positions of each factory are also **unique**. Note that a robot can be **in the same position** as a factory initially.
+
+All the robots are initially broken; they keep moving in one direction. The direction could be the negative or the positive direction of the X-axis. When a robot reaches a factory that did not reach its limit, the factory repairs the robot, and it stops moving.
+
+**At any moment**, you can set the initial direction of moving for **some** robot. Your target is to minimize the total distance traveled by all the robots.
+
+Return _the minimum total distance traveled by all the robots_. The test cases are generated such that all the robots can be repaired.
+
+**Note that**
+
+- All robots move at the same speed.
+- If two robots move in the same direction, they will never collide.
+- If two robots move in opposite directions and they meet at some point, they do not collide. They cross each other.
+- If a robot passes by a factory that reached its limits, it crosses it as if it does not exist.
+- If the robot moved from a position `x` to a position `y`, the distance it moved is `|y - x|`.
+
+
+**Example 1:**
+
+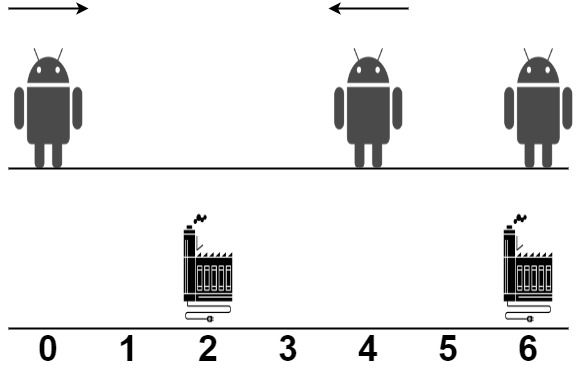
+
+- **Input:** robot = [0,4,6], factory = [[2,2],[6,2]]
+- **Output:** 4
+- **Explanation:** As shown in the figure:
+ - The first robot at position 0 moves in the positive direction. It will be repaired at the first factory.
+ - The second robot at position 4 moves in the negative direction. It will be repaired at the first factory.
+ - The third robot at position 6 will be repaired at the second factory. It does not need to move.
+ - The limit of the first factory is 2, and it fixed 2 robots.
+ - The limit of the second factory is 2, and it fixed 1 robot.
+ - The total distance is |2 - 0| + |2 - 4| + |6 - 6| = 4. It can be shown that we cannot achieve a better total distance than 4.
+
+**Example 2:**
+
+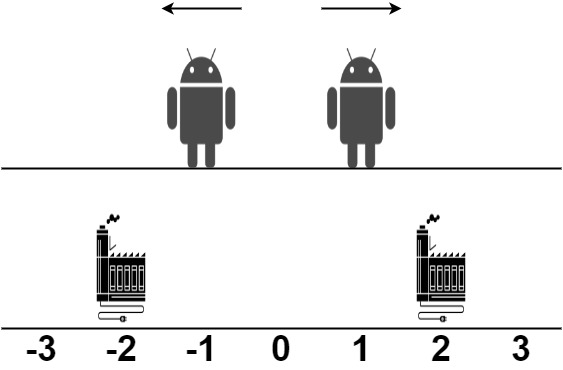
+
+- **Input:** robot = [1,-1], factory = [[-2,1],[2,1]]
+- **Output:** 2
+- **Explanation:** As shown in the figure:
+ - The first robot at position 1 moves in the positive direction. It will be repaired at the second factory.
+ - The second robot at position -1 moves in the negative direction. It will be repaired at the first factory.
+ - The limit of the first factory is 1, and it fixed 1 robot.
+ - The limit of the second factory is 1, and it fixed 1 robot.
+ - The total distance is |2 - 1| + |(-2) - (-1)| = 2. It can be shown that we cannot achieve a better total distance than 2.
+
+
+**Constraints:**
+
+- `1 <= robot.length, factory.length <= 100`
+- `factory[j].length == 2`
+- -109 <= robot[i], positionj <= 109
+- 0 <= limitj <= robot.length
+- The input will be generated such that it is always possible to repair every robot.
+
+
+**Hint:**
+1. Sort robots and factories by their positions.
+2. After sorting, notice that each factory should repair some subsegment of robots.
+3. Find the minimum total distance to repair first i robots with first j factories.
+
+
+
+**Solution:**
+
+We can use dynamic programming with sorted `robot` and `factory` arrays. The idea is to minimize the distance each robot must travel to be repaired by a factory, respecting each factoryβs repair capacity. Hereβs a step-by-step breakdown of the approach:
+
+1. **Sort the `robot` and `factory` arrays by position**. Sorting helps in minimizing the travel distance as we can assign nearby robots to nearby factories.
+
+2. **Dynamic Programming Approach**: We define a 2D DP table `dp[i][j]` where:
+ - `i` represents the first `i` robots.
+ - `j` represents the first `j` factories.
+ - `dp[i][j]` stores the minimum total distance for repairing these `i` robots using these `j` factories.
+
+3. **State Transition**:
+ - For each factory, try to repair a subset of consecutive robots within its limit.
+ - For a factory `j` at position `p`, calculate the minimum distance required for assigning `k` robots to it by summing the distances from each robot to the factory's position.
+ - Update the DP state by selecting the minimum between repairing fewer robots or utilizing the factory capacity to the fullest.
+
+Let's implement this solution in PHP: **[2463. Minimum Total Distance Traveled](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/002463-minimum-total-distance-traveled/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+- **Sorting**: We sort `robot` and `factory` by positions to ensure we assign nearby robots to nearby factories.
+- **DP Initialization**: Initialize `dp[0][0] = 0` since no robots repaired by no factories means zero distance.
+- **Dynamic Programming Transition**:
+ - For each factory `j`, we try repairing the `k` robots preceding it within its limit.
+ - The total distance is accumulated in `sumDist`.
+ - We update `dp[i][j]` with the minimum value after repairing `k` robots, considering the distance and previous states.
+
+### Complexity
+- **Time Complexity**: `O(n * m * L)` where `n` is the number of robots, `m` is the number of factories, and `L` is the maximum limit of repairs any factory can handle.
+- **Space Complexity**: `O(n * m)` for the DP table.
+
+This solution efficiently calculates the minimum travel distance for all robots to be repaired within their factory limits.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
diff --git a/algorithms/002463-minimum-total-distance-traveled/solution.php b/algorithms/002463-minimum-total-distance-traveled/solution.php
new file mode 100644
index 000000000..8cfbef75c
--- /dev/null
+++ b/algorithms/002463-minimum-total-distance-traveled/solution.php
@@ -0,0 +1,36 @@
+109 + 7.
+
+**Example 1:**
+
+- **Input:** low = 3, high = 3, zero = 1, one = 1
+- **Output:** 8
+- **Explanation:**
+ One possible valid good string is "011".
+ It can be constructed as follows: "" -> "0" -> "01" -> "011".
+ All binary strings from "000" to "111" are good strings in this example.
+
+**Example 2:**
+
+- **Input:** low = 2, high = 3, zero = 1, one = 2
+- **Output:** 5
+- **Explanation:** The good strings are "00", "11", "000", "110", and "011".
+
+
+
+**Constraints:**
+
+- 1 <= low <= high <= 105
+- `1 <= zero, one <= low`
+
+
+**Hint:**
+1. Calculate the number of good strings with length less or equal to some constant `x`.
+2. Apply dynamic programming using the group size of consecutive zeros and ones.
+
+
+
+**Solution:**
+
+We need to focus on constructing strings of different lengths and counting the number of valid strings that meet the given conditions. Let's break down the approach:
+
+### Problem Breakdown
+1. **State Definition:**
+ Let `dp[i]` represent the number of valid strings of length `i` that can be constructed using the provided `zero` and `one` values.
+
+2. **Recurrence Relation:**
+ - From any length `i`, we can append either:
+ - `zero` consecutive `0`s, so the previous string would be of length `i-zero`, and we would add `dp[i-zero]` ways.
+ - `one` consecutive `1`s, so the previous string would be of length `i-one`, and we would add `dp[i-one]` ways.
+
+ The recurrence relation becomes:
+ dp[i] = dp[i - zero] + dp[i - one] (mod 109 + 7)
+
+3. **Base Case:**
+ - There is exactly one way to construct an empty string: `dp[0] = 1`.
+
+4. **Final Computation:**
+ - The total number of valid strings of length between `low` and `high` is the sum of `dp[i]` for all `i` from `low` to `high`.
+
+### Solution Steps
+1. Initialize a `dp` array of size `high + 1` where each index `i` represents the number of valid strings of length `i`.
+2. Loop through each possible length `i` from 1 to `high`, updating `dp[i]` based on the recurrence relation.
+3. Compute the sum of `dp[i]` from `low` to `high` to get the final result.
+
+Let's implement this solution in PHP: **[2466. Count Ways To Build Good Strings](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/002466-count-ways-to-build-good-strings/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+1. **Initialization:** We start by setting the base case `dp[0] = 1`, meaning that there is one way to form a string of length `0` (the empty string).
+2. **Dynamic Programming Update:** For each possible string length `i` from 1 to `high`, we check if we can append a string of length `zero` or `one` to a smaller string. We update `dp[i]` accordingly by adding the number of ways to form a string of length `i-zero` and `i-one`, ensuring that the result is taken modulo _**109 + 7**_.
+3. **Final Result Calculation:** We sum up all values of `dp[i]` for `i` in the range `[low, high]` to get the final count of valid strings.
+
+### Time Complexity:
+- Filling the `dp` array takes `O(high)`.
+- Summing the valid results between `low` and `high` takes `O(high - low + 1)`.
+
+Thus, the overall time complexity is _** O(high) **_, which is efficient enough for the input limits.
+
+### Space Complexity:
+- We use an array `dp` of size `high + 1`, so the space complexity is _**O(high)**_.
+
+This solution efficiently solves the problem within the constraints.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
diff --git a/algorithms/002466-count-ways-to-build-good-strings/solution.php b/algorithms/002466-count-ways-to-build-good-strings/solution.php
new file mode 100644
index 000000000..15622fe03
--- /dev/null
+++ b/algorithms/002466-count-ways-to-build-good-strings/solution.php
@@ -0,0 +1,39 @@
+= 0) {
+ $dp[$i] = ($dp[$i] + $dp[$i - $zero]) % $MOD;
+ }
+ if ($i - $one >= 0) {
+ $dp[$i] = ($dp[$i] + $dp[$i - $one]) % $MOD;
+ }
+ }
+
+ // Calculate the result as the sum of dp[i] for i in the range [low, high]
+ $result = 0;
+ for ($i = $low; $i <= $high; $i++) {
+ $result = ($result + $dp[$i]) % $MOD;
+ }
+
+ return $result;
+ }
+}
\ No newline at end of file
diff --git a/algorithms/002471-minimum-number-of-operations-to-sort-a-binary-tree-by-level/README.md b/algorithms/002471-minimum-number-of-operations-to-sort-a-binary-tree-by-level/README.md
new file mode 100644
index 000000000..291e5757c
--- /dev/null
+++ b/algorithms/002471-minimum-number-of-operations-to-sort-a-binary-tree-by-level/README.md
@@ -0,0 +1,239 @@
+2471\. Minimum Number of Operations to Sort a Binary Tree by Level
+
+**Difficulty:** Medium
+
+**Topics:** `Tree`, `Breadth-First Search`, `Binary Tree`
+
+You are given the `root` of a binary tree with **unique values**.
+
+In one operation, you can choose any two nodes **at the same level** and swap their values.
+
+Return _the minimum number of operations needed to make the values at each level sorted in a **strictly increasing order**_.
+
+The **level** of a node is the number of edges along the path between it and the root node.
+
+**Example 1:**
+
+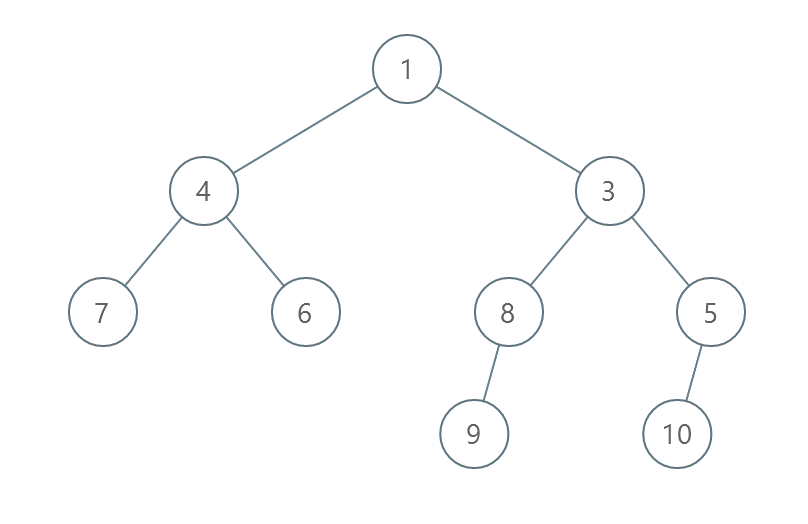
+
+- **Input:** root = [1,4,3,7,6,8,5,null,null,null,null,9,null,10]
+- **Output:** 3
+- **Explanation:**
+ - Swap 4 and 3. The 2nd level becomes [3,4].
+ - Swap 7 and 5. The 3rd level becomes [5,6,8,7].
+ - Swap 8 and 7. The 3rd level becomes [5,6,7,8].
+ We used 3 operations so return 3.
+ It can be proven that 3 is the minimum number of operations needed.
+
+**Example 2:**
+
+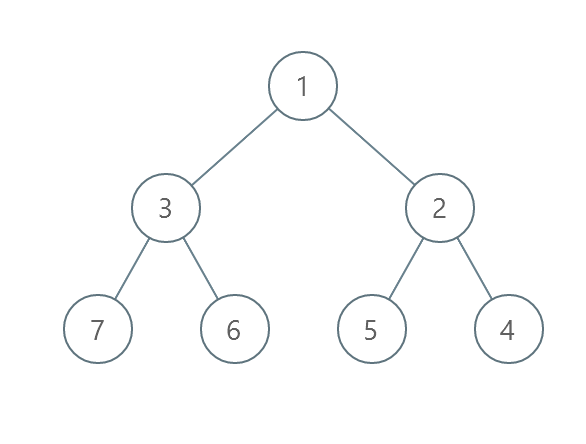
+
+- **Input:** root = [1,3,2,7,6,5,4]
+- **Output:** 3
+- **Explanation:**
+ - Swap 3 and 2. The 2nd level becomes [2,3].
+ - Swap 7 and 4. The 3rd level becomes [4,6,5,7].
+ - Swap 6 and 5. The 3rd level becomes [4,5,6,7].
+ We used 3 operations so return 3.
+ It can be proven that 3 is the minimum number of operations needed.
+
+
+**Example 3:**
+
+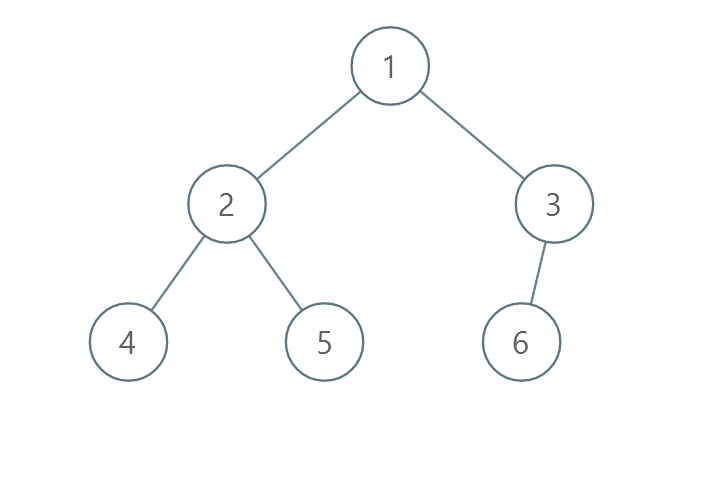
+
+- **Input:** root = [1,2,3,4,5,6]
+- **Output:** 0
+- **Explanation:** Each level is already sorted in increasing order so return 0.
+
+
+
+**Constraints:**
+
+- The number of nodes in the tree is in the range [1, 105]
.
+- 1 <= Node.val <= 105
+- All the values of the tree are **unique**.
+
+
+**Hint:**
+1. We can group the values level by level and solve each group independently.
+2. Do BFS to group the value level by level.
+3. Find the minimum number of swaps to sort the array of each level.
+4. While iterating over the array, check the current element, and if not in the correct index, replace that element with the index of the element which should have come.
+
+
+
+**Solution:**
+
+The problem is about sorting the values of a binary tree level by level in strictly increasing order with the minimum number of operations. In each operation, we can swap the values of two nodes that are at the same level. The goal is to determine the minimum number of such operations required to achieve the sorted order.
+
+### Key Points:
+1. **Binary Tree Levels**: Each level of the binary tree should have values sorted in strictly increasing order.
+2. **Unique Values**: All nodes have unique values, making sorting easier as there are no duplicates.
+3. **BFS for Level Grouping**: Use Breadth-First Search (BFS) to traverse the tree and group nodes by their levels.
+4. **Minimum Swaps**: For each level, we need to find the minimum number of swaps required to sort the node values at that level.
+5. **Constraints**: The tree can have up to _**10^5**_ nodes, so the solution must be efficient.
+
+### Approach:
+
+1. **BFS Traversal**: Perform a BFS to traverse the tree and collect the values of nodes at each level.
+2. **Sorting Each Level**: For each level, calculate the minimum number of swaps to sort the values in strictly increasing order.
+3. **Cycle Decomposition**: The key observation to minimize swaps is that sorting an array can be seen as swapping elements in cycles. The minimum number of swaps for each cycle of misplaced elements is the length of the cycle minus one.
+4. **Accumulating Swaps**: Sum the swaps for each level to get the total number of swaps required.
+
+### Plan:
+
+1. **BFS**: Traverse the tree using BFS to collect nodes at each level.
+2. **Sorting Each Level**: Sort the values at each level and compute the minimum number of swaps.
+3. **Calculate Swaps**: Use cycle decomposition to find the minimum swaps needed to sort the nodes at each level.
+
+Let's implement this solution in PHP: **[2471. Minimum Number of Operations to Sort a Binary Tree by Level](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/002471-minimum-number-of-operations-to-sort-a-binary-tree-by-level/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+1. **Step 1: Perform BFS to group nodes by level**:
+ - Start from the root and traverse the tree level by level.
+ - For each node, append its value to the corresponding level in the `levels` array.
+
+2. **Step 2: For each level, calculate the minimum swaps to sort the values**:
+ - Sort the values at each level.
+ - Use a cycle-based approach to calculate the minimum swaps required to transform the current level into a sorted level.
+
+3. **Cycle Decomposition**:
+ - For each unsorted element, trace its cycle (i.e., where it should go) and mark elements as visited.
+ - For each cycle, the number of swaps required is the length of the cycle minus one.
+
+4. **Return the total number of swaps**:
+ - Sum the swaps needed for each level and return the total.
+
+### Example Walkthrough:
+
+#### Example 1:
+
+Input tree:
+```
+ 1
+ / \
+ 4 3
+ / \ / \
+ 7 6 8 5
+ \
+ 9
+ \
+ 10
+```
+
+**Levels**:
+- Level 0: [1]
+- Level 1: [4, 3]
+- Level 2: [7, 6, 8, 5]
+- Level 3: [9, 10]
+
+1. **Level 1**: [4, 3]
+ - Sort it to [3, 4] with 1 swap (swap 4 and 3).
+
+2. **Level 2**: [7, 6, 8, 5]
+ - Sort it to [5, 6, 7, 8] with 2 swaps (swap 7 and 5, swap 8 and 7).
+
+3. **Level 3**: [9, 10]
+ - Already sorted, no swaps needed.
+
+Total swaps = 1 (Level 1) + 2 (Level 2) = 3 swaps.
+
+Output: 3
+
+#### Example 2:
+
+Input tree:
+```
+ 1
+ / \
+ 3 2
+ / \ / \
+ 7 6 5 4
+```
+
+**Levels**:
+- Level 0: [1]
+- Level 1: [3, 2]
+- Level 2: [7, 6, 5, 4]
+
+1. **Level 1**: [3, 2]
+ - Sort it to [2, 3] with 1 swap (swap 3 and 2).
+
+2. **Level 2**: [7, 6, 5, 4]
+ - Sort it to [4, 5, 6, 7] with 2 swaps (swap 7 and 4, swap 6 and 5).
+
+Total swaps = 1 (Level 1) + 2 (Level 2) = 3 swaps.
+
+Output: 3
+
+### Time Complexity:
+
+1. **BFS**: _**O(N)**_ where _**N**_ is the number of nodes in the tree.
+2. **Sorting Each Level**: Sorting the values at each level takes _**O(L log L)**_ where _**L**_ is the number of nodes at the current level. In the worst case, the total sorting complexity is _**O(N log N)**_.
+3. **Cycle Decomposition**: _**O(L)**_ for each level.
+
+Thus, the overall time complexity is _**O(N log N)**_, which is efficient enough given the constraints.
+
+### Output for Example:
+
+For the input tree:
+
+```
+ 1
+ / \
+ 4 3
+ / \ / \
+ 7 6 8 5
+ \
+ 9
+ \
+ 10
+```
+
+The output is `3` swaps, as detailed in the example.
+
+This solution efficiently calculates the minimum number of swaps needed to sort each level of the binary tree by using BFS to group nodes by level and cycle decomposition to minimize the number of swaps. The time complexity of _**O(N log N)**_ is optimal for handling trees with up to _**10^5**_ nodes.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
diff --git a/algorithms/002471-minimum-number-of-operations-to-sort-a-binary-tree-by-level/solution.php b/algorithms/002471-minimum-number-of-operations-to-sort-a-binary-tree-by-level/solution.php
new file mode 100644
index 000000000..613deb717
--- /dev/null
+++ b/algorithms/002471-minimum-number-of-operations-to-sort-a-binary-tree-by-level/solution.php
@@ -0,0 +1,91 @@
+val = $val;
+ * $this->left = $left;
+ * $this->right = $right;
+ * }
+ * }
+ */
+class Solution {
+
+ /**
+ * @param TreeNode $root
+ * @return Integer
+ */
+ function minimumOperations($root) {
+ if (!$root) {
+ return 0;
+ }
+
+ // Step 1: Perform BFS to get nodes at each level
+ $levels = [];
+ $queue = [[$root, 0]]; // Node and level
+
+ while (!empty($queue)) {
+ list($node, $level) = array_shift($queue);
+
+ if (!isset($levels[$level])) {
+ $levels[$level] = [];
+ }
+ $levels[$level][] = $node->val;
+
+ if ($node->left) {
+ $queue[] = [$node->left, $level + 1];
+ }
+ if ($node->right) {
+ $queue[] = [$node->right, $level + 1];
+ }
+ }
+
+ // Step 2: Calculate the minimum swaps for each level
+ $totalSwaps = 0;
+ foreach ($levels as $level) {
+ $totalSwaps += $this->minSwapsToSort($level);
+ }
+
+ return $totalSwaps;
+ }
+
+ /**
+ * Function to calculate minimum swaps to sort an array
+ *
+ * @param $arr
+ * @return int
+ */
+ function minSwapsToSort($arr) {
+ $n = count($arr);
+ $sortedArr = $arr;
+ sort($sortedArr);
+ $indexMap = array_flip($arr);
+ $visited = array_fill(0, $n, false);
+ $swaps = 0;
+
+ for ($i = 0; $i < $n; $i++) {
+ if ($visited[$i] || $sortedArr[$i] == $arr[$i]) {
+ continue;
+ }
+
+ $cycleSize = 0;
+ $x = $i;
+
+ while (!$visited[$x]) {
+ $visited[$x] = true;
+ $cycleSize++;
+ $x = $indexMap[$sortedArr[$x]];
+ }
+
+ if ($cycleSize > 1) {
+ $swaps += $cycleSize - 1;
+ }
+ }
+
+ return $swaps;
+ }
+}
\ No newline at end of file
diff --git a/algorithms/002490-circular-sentence/README.md b/algorithms/002490-circular-sentence/README.md
new file mode 100644
index 000000000..e71d057e6
--- /dev/null
+++ b/algorithms/002490-circular-sentence/README.md
@@ -0,0 +1,118 @@
+2490\. Circular Sentence
+
+**Difficulty:** Easy
+
+**Topics:** `String`
+
+A **sentence** is a list of words that are separated by a **single** space with no leading or trailing spaces.
+
+- For example, `"Hello World"`, `"HELLO"`, `"hello world hello world"` are all sentences.
+
+Words consist of **only** uppercase and lowercase English letters. Uppercase and lowercase English letters are considered different.
+
+A sentence is **circular** if:
+
+- The last character of a word is equal to the first character of the next word.
+- The last character of the last word is equal to the first character of the first word.
+
+For example, `"leetcode exercises sound delightful"`, `"eetcode"`, `"leetcode eats soul"` are all circular sentences. However, `"Leetcode is cool"`, `"happy Leetcode"`, `"Leetcode"` and `"I like Leetcode"` are **not** circular sentences.
+
+Given a string `sentence`, return `_true` if it is circular_. Otherwise, return _`false`_.
+
+**Example 1:**
+
+- **Input:** sentence = "leetcode exercises sound delightful"
+- **Output:** true
+- **Explanation:** The words in sentence are ["leetcode", "exercises", "sound", "delightful"].
+ - leetcode's last character is equal to exercises's first character.
+ - exercises's last character is equal to sound's first character.
+ - sound's last character is equal to delightful's first character.
+ - delightful's last character is equal to leetcode's first character.
+ The sentence is circular.
+
+**Example 2:**
+
+- **Input:** sentence = "eetcode"
+- **Output:** true
+- **Explanation:** The words in sentence are ["eetcode"].
+ - eetcode's last character is equal to eetcode's first character.
+ The sentence is circular.
+
+
+**Example 3:**
+
+- **Input:** sentence = "Leetcode is cool"
+- **Output:** false
+- **Explanation:** The words in sentence are ["Leetcode", "is", "cool"].
+ - Leetcode's last character is not equal to is's first character.
+ The sentence is not circular.
+
+
+**Constraints:**
+
+- `1 <= sentence.length <= 500`
+- `sentence` consist of only lowercase and uppercase English letters and spaces.
+- The words in `sentence` are separated by a single space.
+- There are no leading or trailing spaces.
+
+**Hint:**
+1. Check the character before the empty space and the character after the empty space.
+2. Check the first character and the last character of the sentence.
+
+
+
+**Solution:**
+
+We need to verify two conditions:
+
+1. Each wordβs last character should match the next wordβs first character.
+2. The last character of the final word should match the first character of the first word, making it circular.
+
+Let's implement this solution in PHP: **[2490. Circular Sentence](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/002490-circular-sentence/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+1. **Splitting the Sentence**: Use `explode(" ", $sentence)` to split the sentence into words.
+2. **Looping through Words**:
+ - For each word, get its last character using `substr($words[$i], -1)`.
+ - Get the first character of the next word. For the last word, we use modulo (`%`) to wrap around to the first word.
+3. **Comparison**:
+ - If the last character of a word doesnβt match the first character of the next word, return `false`.
+ - If the loop completes without finding any mismatch, the sentence is circular, so return `true`.
+
+This code efficiently checks the circular condition for each word pair, making it simple and optimal.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
diff --git a/algorithms/002490-circular-sentence/solution.php b/algorithms/002490-circular-sentence/solution.php
new file mode 100644
index 000000000..2991506c1
--- /dev/null
+++ b/algorithms/002490-circular-sentence/solution.php
@@ -0,0 +1,32 @@
+ith player. Divide the players into `n / 2` teams of size `2` such that the total skill of each team is **equal**.
+
+The **chemistry** of a team is equal to the **product** of the skills of the players on that team.
+
+Return _the sum of the **chemistry** of all the teams, or return `-1` if there is no way to divide the players into teams such that the total skill of each team is equal_.
+
+**Example 1:**
+
+- **Input:** skill = [3,2,5,1,3,4]
+- **Output:** 22
+- **Explanation:**\
+ Divide the players into the following teams: (1, 5), (2, 4), (3, 3), where each team has a total skill of 6.\
+ The sum of the chemistry of all the teams is: 1 * 5 + 2 * 4 + 3 * 3 = 5 + 8 + 9 = 22.
+
+**Example 2:**
+
+- **Input:** skill = [3,4]
+- **Output:** 112
+- **Explanation:**\
+ The two players form a team with a total skill of 7.\
+ The chemistry of the team is 3 * 4 = 12.
+
+
+**Example 3:**
+
+- **Input:** skill = [1,1,2,3]
+- **Output:** -1
+- **Explanation:** There is no way to divide the players into teams such that the total skill of each team is equal.
+
+
+**Constraints:**
+
+- 2 <= skill.length <= 105
+- `skill.length` is even.
+- `1 <= skill[i] <= 1000`
+
+**Hint:**
+1. Try sorting the skill array.
+2. It is always optimal to pair the weakest available player with the strongest available player.
+
+
+
+**Solution:**
+
+We can follow the hint provided and use a greedy approach. Here's the detailed breakdown of the solution:
+
+### Steps:
+1. **Sort the Skill Array**: Sorting allows us to efficiently pair the weakest player (smallest value) with the strongest player (largest value).
+
+2. **Check for Valid Pairing**: The sum of the skills of each team should be equal. After sorting, we will pair the smallest and the largest element, then the second smallest with the second largest, and so on. If at any point, the sum of a pair differs from the previous sums, it's impossible to divide the players into valid teams, and we should return `-1`.
+
+3. **Calculate the Chemistry**: The chemistry of each team is the product of the two skills in that team. Sum up all the chemistry values for each valid team.
+
+4. **Return the Total Chemistry**: If all the teams have the same total skill, return the sum of their chemistry.
+
+Let's implement this solution in PHP: **[2491. Divide Players Into Teams of Equal Skill](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/002491-divide-players-into-teams-of-equal-skill/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+1. **Sorting**: The array `skill` is sorted to ensure that we can efficiently pair the smallest and largest values.
+
+2. **Two Pointers**: We use two pointers (`$i` starting from the beginning and `$j` starting from the end). For each valid pair (smallest and largest), we check if their sum is the same as the expected `teamSkillSum`. If not, it's impossible to divide the players into teams.
+
+3. **Chemistry Calculation**: If the pair is valid, the chemistry is calculated as the product of the two values (`$skill[$i] * $skill[$j]`), and we keep adding it to the total chemistry.
+
+4. **Edge Cases**:
+ - If the team cannot be formed due to unequal sums, we return `-1`.
+ - The code handles cases with even length and ensures that all players are paired up correctly.
+
+### Time Complexity:
+- Sorting the array takes _**O(n log n)**_, and the two-pointer traversal takes _**O(n)**_. Hence, the overall time complexity is _**O(n log n)**_, which is efficient given the constraints.
+
+This solution works within the given constraint of up to **105
** players.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
diff --git a/algorithms/002491-divide-players-into-teams-of-equal-skill/solution.php b/algorithms/002491-divide-players-into-teams-of-equal-skill/solution.php
new file mode 100644
index 000000000..a77b15769
--- /dev/null
+++ b/algorithms/002491-divide-players-into-teams-of-equal-skill/solution.php
@@ -0,0 +1,31 @@
+2 <= nums.length <= 105
+- 2 <= nums[i] <= 105
+
+
+**Hint:**
+1. With the constraints, the length of the longest square streak possible is 5.
+2. Store the elements of nums in a set to quickly check if it exists.
+
+
+
+**Solution:**
+
+We need to identify the longest square streak in the `nums` array. A square streak is a subsequence where each subsequent element is the square of the previous element, and it must be at least two elements long.
+
+Here's the solution approach:
+
+1. **Use a Set for Quick Lookup**:
+ - Store the numbers in a set to quickly verify if an element's square is also in the array.
+
+2. **Iterate Through the Array**:
+ - For each number in the array, try to build a square streak starting from that number.
+ - Check if the square of the current number exists in the set and keep extending the streak until thereβs no further square match.
+
+3. **Track Maximum Length**:
+ - Track the maximum length of all possible square streaks encountered. If no square streak is found, return `-1`.
+
+4. **Optimization**:
+ - Sort the array before checking each element to ensure the subsequence is checked in ascending order. This will help avoid redundant checks.
+
+Let's implement this solution in PHP: **[2501. Longest Square Streak in an Array](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/002501-longest-square-streak-in-an-array/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+- **Sorting**: Sorting `nums` ensures we can check sequences in ascending order.
+- **Set Lookup**: Using `array_flip` creates a set-like structure for `$numSet` with `$nums` as keys, allowing fast existence checks.
+- **Loop through each number**: For each `num` in `nums`, check if the square of the current number is in the set. If it is, continue the streak. Otherwise, break the streak and check if itβs the longest found.
+
+### Complexity Analysis
+
+- **Time Complexity**: _**O(n log n)**_ due to sorting, where _**n**_ is the number of elements in `nums`. The subsequent lookups and square streak checks are _**O(n)**_.
+- **Space Complexity**: _**O(n)**_, mainly for storing `nums` in the set.
+
+This solution efficiently finds the longest square streak or returns `-1` if no valid streak exists.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
diff --git a/algorithms/002501-longest-square-streak-in-an-array/solution.php b/algorithms/002501-longest-square-streak-in-an-array/solution.php
new file mode 100644
index 000000000..ac5a5e6bd
--- /dev/null
+++ b/algorithms/002501-longest-square-streak-in-an-array/solution.php
@@ -0,0 +1,38 @@
+= 2) {
+ $maxStreak = max($maxStreak, $currentStreak);
+ }
+ }
+
+ // Step 4: Return result
+ return $maxStreak >= 2 ? $maxStreak : -1;
+ }
+}
\ No newline at end of file
diff --git a/algorithms/002516-take-k-of-each-character-from-left-and-right/README.md b/algorithms/002516-take-k-of-each-character-from-left-and-right/README.md
new file mode 100644
index 000000000..e236bc2fd
--- /dev/null
+++ b/algorithms/002516-take-k-of-each-character-from-left-and-right/README.md
@@ -0,0 +1,123 @@
+2516\. Take K of Each Character From Left and Right
+
+**Difficulty:** Medium
+
+**Topics:** `Hash Table`, `String`, `Sliding Window`
+
+You are given a string `s` consisting of the characters `'a'`, `'b'`, and `'c'` and a non-negative integer `k`. Each minute, you may take either the **leftmost** character of `s`, or the **rightmost** character of `s`.
+
+Return _the **minimum** number of minutes needed for you to take **at least** `k` of each character, or return `-1` if it is not possible to take `k` of each character_.
+
+**Example 1:**
+
+- **Input:** s = "aabaaaacaabc", k = 2
+- **Output:** 8
+- **Explanation:**
+ Take three characters from the left of s. You now have two 'a' characters, and one 'b' character.
+ Take five characters from the right of s. You now have four 'a' characters, two 'b' characters, and two 'c' characters.
+ A total of 3 + 5 = 8 minutes is needed.
+ It can be proven that 8 is the minimum number of minutes needed.
+
+**Example 2:**
+
+- **Input:** s = "a", k = 1
+- **Output:** -1
+- **Explanation:** It is not possible to take one 'b' or 'c' so return -1.
+
+**Constraints:**
+
+- 1 <= s.length <= 105
+- `s` consists of only the letters `'a'`, `'b'`, and `'c'`.
+- `0 <= k <= s.length`
+
+
+**Hint:**
+1. Start by counting the frequency of each character and checking if it is possible.
+2. If you take x characters from the left side, what is the minimum number of characters you need to take from the right side? Find this for all values of x in the range 0 β€ x β€ s.length.
+3. Use a two-pointers approach to avoid computing the same information multiple times.
+
+
+
+**Solution:**
+
+We can use a sliding window technique with two pointers to find the minimum number of minutes needed to take at least `k` of each character ('a', 'b', 'c') from both the left and right of the string.
+
+### Problem Breakdown:
+- We are given a string `s` containing only 'a', 'b', and 'c'.
+- We need to take at least `k` occurrences of each character, either from the leftmost or rightmost characters of the string.
+- We need to determine the **minimum number of minutes** required to achieve this or return `-1` if it's impossible.
+
+### Approach:
+
+1. **Initial Checks**:
+ - If `k == 0`, we can directly return `0` since no characters are required.
+ - If `k` exceeds the number of occurrences of any character in the string, return `-1` immediately.
+
+2. **Frequency Count**:
+ - We need to count how many times 'a', 'b', and 'c' appear in the string `s` to ensure that it's even possible to gather `k` of each character.
+
+3. **Sliding Window Technique**:
+ - Use a sliding window approach with two pointers (`left` and `right`).
+ - Maintain two pointers and slide them from both ends of the string to gather the required characters.
+ - For every number of characters taken from the left, calculate the minimum number of characters that need to be taken from the right to satisfy the requirement.
+
+4. **Optimization**:
+ - Instead of recalculating the character counts repeatedly for each window, we can keep track of character counts as we expand or contract the window.
+
+Let's implement this solution in PHP: **[2516. Take K of Each Character From Left and Right](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/002516-take-k-of-each-character-from-left-and-right/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+1. **Initial Setup**:
+ - We count the occurrences of `'a'`, `'b'`, and `'c'` in the entire string to ensure it's possible to gather at least `k` of each character.
+ - If any character count is less than `k`, return `-1`.
+
+2. **Sliding Window**:
+ - We use two pointers (`left` and `right`) to create a sliding window from both ends.
+ - We expand the window by moving the `right` pointer and increment the count of the characters encountered.
+ - Once we have at least `k` of each character in the current window, we try to shrink the window from the left to minimize the number of minutes (characters taken).
+
+3. **Minimize Time**:
+ - We track the minimum number of minutes required by comparing the size of the window each time we collect `k` characters of all types.
+
+### Time Complexity:
+- Counting characters initially takes O(n).
+- The sliding window operation takes O(n), as both `left` and `right` pointers move across the string once.
+- Overall time complexity is O(n).
+
+### Edge Cases:
+- If `k == 0`, return `0`.
+- If it's impossible to take `k` of each character, return `-1`.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
diff --git a/algorithms/002516-take-k-of-each-character-from-left-and-right/solution.php b/algorithms/002516-take-k-of-each-character-from-left-and-right/solution.php
new file mode 100644
index 000000000..c65c7440d
--- /dev/null
+++ b/algorithms/002516-take-k-of-each-character-from-left-and-right/solution.php
@@ -0,0 +1,38 @@
+1 <= nums.length, k <= 105
+- 1 <= nums[i] <= 109
+
+**Hint:**
+1. It is always optimal to select the greatest element in the array.
+2. Use a heap to query for the maximum in O(log n) time.
+
+
+**Solution:**
+
+Let's break down the solution for the "Maximal Score After Applying K Operations" problem:
+
+### Approach:
+
+1. **Use a Max Heap**: The problem requires us to maximize the score by selecting the largest available number in `nums` for `k` operations. A Max Heap (priority queue) is ideal here since it allows us to efficiently extract the largest element and then insert updated values.
+
+2. **Extract the Maximum Element**: For each operation, extract the largest element from the heap. This element will be added to the score.
+
+3. **Update the Extracted Element**: After using the largest element, replace it with `ceil(num / 3)` (rounding up). This simulates the reduction in the value of the chosen element as per the problem's requirement.
+
+4. **Insert the Updated Element Back into the Heap**: Insert the updated value back into the heap so that it can be used in subsequent operations if it remains among the largest values.
+
+5. **Repeat for `k` Operations**: Perform this process exactly `k` times to ensure the maximum possible score is achieved after `k` operations.
+
+### Detailed Explanation:
+
+- **Initialization**:
+ - `SplMaxHeap` is used to maintain a max-heap (priority queue) to efficiently get the maximum element at each step.
+ - `ans` keeps track of the cumulative score.
+ - Insert all elements of `nums` into the max heap.
+
+- **Processing Each Operation**:
+ - Extract the largest element using `extract()`.
+ - Add this value to the `sum`.
+ - Calculate the new value of this element as `ceil(t / 3)`. Since PHP `int` division rounds down, we use `(int)($t + 2) / 3` to simulate the ceiling.
+ - Insert the updated value back into the heap.
+
+- **Return the Final Score**: After `k` operations, return the accumulated `sum`.
+
+Let's implement this solution in PHP: **[2530. Maximal Score After Applying K Operations](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/002530-maximal-score-after-applying-k-operations/solution.php)**
+
+```php
+
+```
+
+### Example Walkthrough:
+
+**Example 1**: `nums = [10, 10, 10, 10, 10], k = 5`
+- Initial Heap: `[10, 10, 10, 10, 10]`
+- Extract 10, score += 10, insert `ceil(10/3) = 4`: New Heap: `[10, 10, 10, 10, 4]`
+- Extract 10, score += 10, insert `ceil(10/3) = 4`: New Heap: `[10, 10, 4, 10, 4]`
+- Repeat similarly for all elements. The final score will be 50.
+
+**Example 2**: `nums = [1, 10, 3, 3, 3], k = 3`
+- Initial Heap: `[10, 3, 3, 3, 1]`
+- Extract 10, score += 10, insert `ceil(10/3) = 4`: New Heap: `[4, 3, 3, 3, 1]`
+- Extract 4, score += 4, insert `ceil(4/3) = 2`: New Heap: `[3, 3, 3, 2, 1]`
+- Extract 3, score += 3, insert `ceil(3/3) = 1`: New Heap: `[3, 3, 2, 1, 1]`
+- Final score is 17.
+
+### Complexity:
+
+- **Time Complexity**: `O(k log n)`, where `k` is the number of operations and `n` is the size of the heap. Each extraction and insertion operation on the heap takes `O(log n)` time, and this is done `k` times.
+- **Space Complexity**: `O(n)` for storing the elements in the max heap.
+
+This solution efficiently maximizes the score by always choosing the largest available number and managing the decreasing values using a priority queue.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
\ No newline at end of file
diff --git a/algorithms/002530-maximal-score-after-applying-k-operations/solution.php b/algorithms/002530-maximal-score-after-applying-k-operations/solution.php
index 6b4260cf9..a92a55a9f 100644
--- a/algorithms/002530-maximal-score-after-applying-k-operations/solution.php
+++ b/algorithms/002530-maximal-score-after-applying-k-operations/solution.php
@@ -7,19 +7,30 @@ class Solution {
* @param Integer $k
* @return Integer
*/
- function maxKelements(array $nums, int $k): int
- {
- $pq = new SplMaxHeap(); // Building Max Heap
- $ans = 0;
- foreach($nums as $num){
+ function maxKelements($nums, $k) {
+ $pq = new SplMaxHeap(); // Building a max heap
+ $sum = 0;
+
+ // Insert all elements into the max heap
+ foreach ($nums as $num) {
$pq->insert($num);
}
- $sum = 0;
- for($i=0; $i<$k; $i++){
+
+ // Perform k operations
+ for ($i = 0; $i < $k; $i++) {
+ // Extract the largest element
$t = $pq->extract();
- $sum += (int)$t;
- $pq->insert((int)($t+2)/3); // taking ceil value
+
+ // Add it to the score
+ $sum += $t;
+
+ // Calculate the new value using ceil(t / 3)
+ $newVal = (int)(($t + 2) / 3);
+
+ // Insert the updated value back into the heap
+ $pq->insert($newVal);
}
+
return $sum;
}
}
\ No newline at end of file
diff --git a/algorithms/002554-maximum-number-of-integers-to-choose-from-a-range-i/README.md b/algorithms/002554-maximum-number-of-integers-to-choose-from-a-range-i/README.md
new file mode 100644
index 000000000..77bc58d00
--- /dev/null
+++ b/algorithms/002554-maximum-number-of-integers-to-choose-from-a-range-i/README.md
@@ -0,0 +1,152 @@
+2554\. Maximum Number of Integers to Choose From a Range I
+
+**Difficulty:** Medium
+
+**Topics:** `Array`, `Hash Table`, `Binary Search`, `Greedy`, `Sorting`
+
+You are given an integer array `banned` and two integers `n` and `maxSum`. You are choosing some number of integers following the below rules:
+
+- The chosen integers have to be in the range `[1, n]`.
+- Each integer can be chosen **at most once**.
+- The chosen integers should not be in the array `banned`.
+- The sum of the chosen integers should not exceed `maxSum`.
+
+Return _the **maximum** number of integers you can choose following the mentioned rules_.
+
+**Example 1:**
+
+- **Input:** banned = [1,6,5], n = 5, maxSum = 6
+- **Output:** 2
+- **Explanation:** You can choose the integers 2 and 4.
+ 2 and 4 are from the range [1, 5], both did not appear in banned, and their sum is 6, which did not exceed maxSum.
+
+**Example 2:**
+
+- **Input:** banned = [1,2,3,4,5,6,7], n = 8, maxSum = 1
+- **Output:** 0
+- **Explanation:** You cannot choose any integer while following the mentioned conditions.
+
+
+**Example 3:**
+
+- **Input:** banned = [11], n = 7, maxSum = 50
+- **Output:** 7
+- **Explanation:** You can choose the integers 1, 2, 3, 4, 5, 6, and 7.
+ They are from the range [1, 7], all did not appear in banned, and their sum is 28, which did not exceed maxSum.
+
+
+
+**Constraints:**
+
+- 1 <= banned.length <= 104
+- 1 <= banned[i], n <= 104
+- 1 <= maxSum <= 109
+
+
+**Hint:**
+1. Keep the banned numbers that are less than n in a set.
+2. Loop over the numbers from 1 to n and if the number is not banned, use it.
+3. Keep adding numbers while they are not banned, and their sum is less than k.
+
+
+
+**Solution:**
+
+We can use a greedy approach where we iterate over the numbers from `1` to `n`, skipping the banned numbers, and keep adding the valid numbers (those not in the `banned` array) to a running sum until we reach the `maxSum`.
+
+Here's the step-by-step solution:
+
+### Steps:
+1. **Convert banned array to a set for quick lookups:** Using `array_flip()` can convert the `banned` array into a set for O(1) average-time complexity lookups.
+2. **Iterate from 1 to n:** Check each number, if it's not in the banned set and if adding it doesn't exceed `maxSum`, add it to the sum and increase the count.
+3. **Stop once adding the next number exceeds `maxSum`:** Since the goal is to maximize the number of chosen integers without exceeding the sum, this greedy approach ensures we take the smallest available numbers first.
+
+### Approach:
+1. **Exclude Banned Numbers:** We'll keep track of the banned numbers in a set (or an associative array) for fast lookups.
+2. **Greedy Selection:** Start selecting numbers from `1` to `n` in ascending order, as this will allow us to maximize the number of integers selected. Each time we select a number, we'll add it to the sum and check if it exceeds `maxSum`. If it does, stop.
+3. **Efficiency Considerations:** Since we are iterating over numbers from `1` to `n`, and checking if each is in the banned set (which can be done in constant time), the approach runs in linear time relative to `n` and the size of the banned list.
+
+Let's implement this solution in PHP: **[2554. Maximum Number of Integers to Choose From a Range I](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/002554-maximum-number-of-integers-to-choose-from-a-range-i/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+1. **Convert banned array to set:**
+ We use `array_flip($banned)` to create a set from the `banned` array, which allows for O(1) lookups to check if a number is banned.
+
+2. **Iterate from `1` to `n`:**
+ We iterate through numbers from `1` to `n`. For each number:
+ - If the number is not in the banned set (checked using `isset($bannedSet[$i])`),
+ - And if adding the number to the sum does not exceed `maxSum`,
+ - We include that number and update the sum and count.
+
+3. **Return the count:**
+ After the loop, we return the number of integers selected (`$count`).
+4. **`$bannedSet = array_flip($banned);`**: This converts the banned list into a set (associative array) for fast lookups.
+5. **`for ($i = 1; $i <= $n; $i++)`**: We iterate over all integers from 1 to `n`.
+6. **`if (isset($bannedSet[$i])) { continue; }`**: This checks if the current number is in the banned set. If it is, we skip it.
+7. **`if ($sum + $i > $maxSum) { break; }`**: If adding the current number exceeds `maxSum`, we stop the process.
+8. **`$sum += $i; $count++;`**: If the number is valid and adding it doesn't exceed `maxSum`, we include it in our sum and increase the count.
+
+### Time Complexity:
+- The creation of the banned set (`array_flip`) is O(b), where `b` is the length of the banned array.
+- The loop iterates `n` times (for numbers from 1 to `n`), and each lookup into the banned set takes O(1) time. So, the time complexity of the loop is O(n).
+- Thus, the overall time complexity is O(n + b), which is efficient given the problem constraints.
+
+### Example Walkthrough:
+
+For the input:
+
+- **Input 1:** `banned = [1, 6, 5], n = 5, maxSum = 6`
+ - We create the banned set: `{1, 5, 6}`.
+ - We iterate through numbers 1 to 5:
+ - 1 is banned, skip it.
+ - 2 is not banned, add it to sum (`sum = 2`, `count = 1`).
+ - 3 is not banned, add it to sum (`sum = 5`, `count = 2`).
+ - 4 is not banned, but adding it to the sum would exceed `maxSum` (5 + 4 = 9), so skip it.
+ - The result is 2.
+
+- **Input 2:** `banned = [1, 2, 3, 4, 5, 6, 7], n = 8, maxSum = 1`
+ - All numbers from 1 to 7 are banned, so no valid numbers can be chosen.
+ - The result is 0.
+
+- **Input 3:** `banned = [11], n = 7, maxSum = 50`
+ - The only banned number is 11, which is outside the range 1 to 7.
+ - We can select all numbers from 1 to 7, and their sum is 28, which is less than `maxSum`.
+ - The result is 7.
+
+This solution efficiently handles the problem within the given constraints.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
diff --git a/algorithms/002554-maximum-number-of-integers-to-choose-from-a-range-i/solution.php b/algorithms/002554-maximum-number-of-integers-to-choose-from-a-range-i/solution.php
new file mode 100644
index 000000000..dc996907d
--- /dev/null
+++ b/algorithms/002554-maximum-number-of-integers-to-choose-from-a-range-i/solution.php
@@ -0,0 +1,37 @@
+ $maxSum) {
+ break;
+ }
+
+ // Add this number to the sum and increment the count
+ $sum += $i;
+ $count++;
+ }
+
+ return $count;
+ }
+}
\ No newline at end of file
diff --git a/algorithms/002558-take-gifts-from-the-richest-pile/README.md b/algorithms/002558-take-gifts-from-the-richest-pile/README.md
new file mode 100644
index 000000000..be292f0b9
--- /dev/null
+++ b/algorithms/002558-take-gifts-from-the-richest-pile/README.md
@@ -0,0 +1,148 @@
+2558\. Take Gifts From the Richest Pile
+
+**Difficulty:** Easy
+
+**Topics:** `Array`, `Heap (Priority Queue)`, `Simulation`
+
+You are given an integer array `gifts` denoting the number of gifts in various piles. Every second, you do the following:
+
+- Choose the pile with the maximum number of gifts.
+- If there is more than one pile with the maximum number of gifts, choose any.
+- Leave behind the floor of the square root of the number of gifts in the pile. Take the rest of the gifts.
+
+Return _the number of gifts remaining after `k` seconds_.
+
+**Example 1:**
+
+- **Input:** gifts = [25,64,9,4,100], k = 4
+- **Output:** 29
+- **Explanation:** The gifts are taken in the following way:
+ - In the first second, the last pile is chosen and 10 gifts are left behind.
+ - Then the second pile is chosen and 8 gifts are left behind.
+ - After that the first pile is chosen and 5 gifts are left behind.
+ - Finally, the last pile is chosen again and 3 gifts are left behind.
+ The final remaining gifts are [5,8,9,4,3], so the total number of gifts remaining is 29.
+
+**Example 2:**
+
+- **Input:** gifts = [1,1,1,1], k = 4
+- **Output:** 4
+- **Explanation:** In this case, regardless which pile you choose, you have to leave behind 1 gift in each pile.
+ That is, you can't take any pile with you.
+ So, the total gifts remaining are 4.
+
+
+
+**Constraints:**
+
+- 1 <= gifts.length <= 103
+- 1 <= gifts[i] <= 109
+- 1 <= k <= 103
+
+
+**Hint:**
+1. How can you keep track of the largest gifts in the array
+2. What is an efficient way to find the square root of a number?
+3. Can you keep adding up the values of the gifts while ensuring they are in a certain order?
+4. Can we use a priority queue or heap here?
+
+
+
+**Solution:**
+
+We can utilize a max-heap (priority queue) since we need to repeatedly pick the pile with the maximum number of gifts. A max-heap will allow us to efficiently access the largest pile in constant time and update the heap after taking gifts from the pile.
+
+### Approach:
+
+1. **Use a Max-Heap:**
+ - Since we need to repeatedly get the pile with the maximum number of gifts, a max-heap (priority queue) is ideal. In PHP, we can use `SplPriorityQueue`, which is a priority queue that by default works as a max-heap.
+ - To simulate a max-heap, we will insert the number of gifts as a negative value, since `SplPriorityQueue` is a min-heap by default. By inserting negative values, the smallest negative value will represent the largest original number.
+
+2. **Process Each Second:**
+ - In each second, pop the pile with the maximum number of gifts from the heap.
+ - Take all the gifts except the floor of the square root of the number of gifts in that pile.
+ - Push the modified pile back into the heap.
+
+3. **Termination:**
+ - We stop after `k` seconds or once we've processed all the seconds.
+
+Let's implement this solution in PHP: **[2558. Take Gifts From the Richest Pile](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/002558-take-gifts-from-the-richest-pile/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+1. **Heap Initialization**:
+ - `SplPriorityQueue` is used to simulate a max-heap. The `insert` method allows us to push elements into the heap with a priority.
+
+2. **Processing the Largest Pile**:
+ - For `k` iterations, the largest pile is extracted using `extract`.
+ - The number of gifts left behind is calculated as the floor of the square root of the current largest pile using `floor(sqrt(...))`.
+ - The reduced pile is re-inserted into the heap.
+
+3. **Summing Remaining Gifts**:
+ - After the `k` operations, all elements in the heap are extracted and summed up to get the total number of remaining gifts.
+
+4. **Edge Cases**:
+ - If `gifts` is empty, the result is `0`.
+ - If `k` is larger than the number of operations possible, the algorithm gracefully handles it.
+
+### Time Complexity:
+
+- **Heap operations (insert and extract)**: Each heap operation (insertion and extraction) takes _**O(log n)**_, where _**n**_ is the number of piles.
+- **Looping through `k` operations**: We perform _**k**_ operations, each involving heap extraction and insertion, both taking _**O(log n)**_.
+
+Thus, the total time complexity is _**O(k log n)**_, where _**n**_ is the number of piles and _**k**_ is the number of seconds.
+
+### Example Walkthrough:
+
+For the input:
+
+```php
+$gifts = [25, 64, 9, 4, 100];
+$k = 4;
+```
+
+1. Initially, the priority queue has the piles: `[25, 64, 9, 4, 100]`.
+2. After 1 second: Choose 100, leave 10 behind. The remaining gifts are: `[25, 64, 9, 4, 10]`.
+3. After 2 seconds: Choose 64, leave 8 behind. The remaining gifts are: `[25, 8, 9, 4, 10]`.
+4. After 3 seconds: Choose 25, leave 5 behind. The remaining gifts are: `[5, 8, 9, 4, 10]`.
+5. After 4 seconds: Choose 10, leave 3 behind. The remaining gifts are: `[5, 8, 9, 4, 3]`.
+
+The sum of the remaining gifts is _**5 + 8 + 9 + 4 + 3 = 29**_.
+
+This approach efficiently solves the problem using a max-heap and performs well within the given constraints.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
diff --git a/algorithms/002558-take-gifts-from-the-richest-pile/solution.php b/algorithms/002558-take-gifts-from-the-richest-pile/solution.php
new file mode 100644
index 000000000..8a2cedceb
--- /dev/null
+++ b/algorithms/002558-take-gifts-from-the-richest-pile/solution.php
@@ -0,0 +1,41 @@
+insert($gift, $gift); // Value and priority are the same
+ }
+
+ // Perform the operation k times
+ for ($i = 0; $i < $k; ++$i) {
+ if ($maxHeap->isEmpty()) break;
+
+ // Get the largest pile
+ $largestPile = $maxHeap->extract();
+
+ // Compute the floor of the square root of the largest pile
+ $reducedPile = floor(sqrt($largestPile));
+
+ // Put the reduced pile back into the heap
+ $maxHeap->insert($reducedPile, $reducedPile);
+ }
+
+ // Sum up the remaining gifts
+ $remainingGifts = 0;
+ while (!$maxHeap->isEmpty()) {
+ $remainingGifts += $maxHeap->extract();
+ }
+
+ return $remainingGifts;
+ }
+}
\ No newline at end of file
diff --git a/algorithms/002559-count-vowel-strings-in-ranges/README.md b/algorithms/002559-count-vowel-strings-in-ranges/README.md
new file mode 100644
index 000000000..804c78317
--- /dev/null
+++ b/algorithms/002559-count-vowel-strings-in-ranges/README.md
@@ -0,0 +1,134 @@
+2559\. Count Vowel Strings in Ranges
+
+**Difficulty:** Medium
+
+**Topics:** `Array`, `String`, `Prefix Sum`
+
+You are given a **0-indexed** array of strings `words` and a 2D array of integers `queries`.
+
+Each query queries[i] = [li, ri]
asks us to find the number of strings present in the range li
to ri
(both inclusive) of `words` that start and end with a vowel.
+
+Return _an array `ans` of size `queries.length`, where `ans[i]` is the answer to the ith
query_.
+
+**Note** that the vowel letters are `'a'`, `'e'`, `'i'`, `'o'`, and `'u'`.
+
+**Example 1:**
+
+- **Input:** words = ["aba","bcb","ece","aa","e"], queries = [[0,2],[1,4],[1,1]]
+- **Output:** [2,3,0]
+- **Explanation:** The strings starting and ending with a vowel are "aba", "ece", "aa" and "e".
+ The answer to the query [0,2] is 2 (strings "aba" and "ece").
+ to query [1,4] is 3 (strings "ece", "aa", "e").
+ to query [1,1] is 0.
+ We return [2,3,0].
+
+**Example 2:**
+
+- **Input:** words = ["a","e","i"], queries = [[0,2],[0,1],[2,2]]
+- **Output:** [3,2,1]
+- **Explanation:** Every string satisfies the conditions, so we return [3,2,1].
+
+
+**Constraints:**
+
+- 1 <= words.length <= 105
+- `1 <= words[i].length <= 40`
+- `words[i]` consists only of lowercase English letters.
+- sum(words[i].length) <= 3 * 105
+- 1 <= queries.length <= 105
+- 0 <= li <= ri < words.length
+
+
+**Hint:**
+1. Precompute the prefix sum of strings that start and end with vowels.
+2. Use unordered_set to store vowels.
+3. Check if the first and last characters of the string are present in the vowels set.
+4. Subtract prefix sum for range `[l-1, r]` to find the number of strings starting and ending with vowels.
+
+
+
+**Solution:**
+
+We can follow these steps:
+
+1. **Check for Vowel Strings:** Create a helper function to determine whether a string starts and ends with a vowel.
+2. **Precompute Prefix Sums:** Use a prefix sum array to store the cumulative count of strings that start and end with vowels.
+3. **Answer Queries:** Use the prefix sum array to efficiently calculate the number of such strings within the specified range for each query.
+
+Let's implement this solution in PHP: **[2559. Count Vowel Strings in Ranges](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/002559-count-vowel-strings-in-ranges/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+1. **`isVowelString` Function:**
+ - Checks if the first and last characters of the string are vowels.
+ - Uses `in_array` to determine if the characters are in the predefined list of vowels.
+
+2. **Prefix Sum Array:**
+ - `prefixSum[i]` stores the cumulative count of vowel strings up to index `i-1`.
+ - If the current word satisfies the condition, increment the count.
+
+3. **Query Resolution:**
+ - For a range `[l, r]`, the count of vowel strings is `prefixSum[r + 1] - prefixSum[l]`.
+
+4. **Efficiency:**
+ - Constructing the prefix sum array takes _**O(n)**_, where _**n**_ is the number of words.
+ - Resolving each query takes _**O(1)**_, making the overall complexity _**O(n + q)**_, where _**q**_ is the number of queries.
+
+### Edge Cases:
+- All strings start and end with vowels.
+- No strings start and end with vowels.
+- Single-element ranges in the queries.
+
+This approach efficiently handles the constraints of the problem.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
diff --git a/algorithms/002559-count-vowel-strings-in-ranges/solution.php b/algorithms/002559-count-vowel-strings-in-ranges/solution.php
new file mode 100644
index 000000000..30de87140
--- /dev/null
+++ b/algorithms/002559-count-vowel-strings-in-ranges/solution.php
@@ -0,0 +1,39 @@
+isVowelString($words[$i]) ? 1 : 0);
+ }
+
+ // Step 2: Answer queries using the prefix sum
+ $result = [];
+ foreach ($queries as $query) {
+ list($l, $r) = $query;
+ $result[] = $prefixSum[$r + 1] - $prefixSum[$l];
+ }
+
+ return $result;
+ }
+
+ /**
+ * Helper function to check if a string starts and ends with a vowel
+ *
+ * @param $word
+ * @return bool
+ */
+ function isVowelString($word) {
+ $vowels = ['a', 'e', 'i', 'o', 'u'];
+ $n = strlen($word);
+ return in_array($word[0], $vowels) && in_array($word[$n - 1], $vowels);
+ }
+}
\ No newline at end of file
diff --git a/algorithms/002563-count-the-number-of-fair-pairs/README.md b/algorithms/002563-count-the-number-of-fair-pairs/README.md
new file mode 100644
index 000000000..c0f30cebb
--- /dev/null
+++ b/algorithms/002563-count-the-number-of-fair-pairs/README.md
@@ -0,0 +1,129 @@
+2563\. Count the Number of Fair Pairs
+
+**Difficulty:** Medium
+
+**Topics:** `Array`, `Two Pointers`, `Binary Search`, `Sorting`
+
+Given a **0-indexed** integer array `nums` of size `n` and two integers `lower` and `upper`, return _the number of fair pairs_.
+
+A pair `(i, j)` is **fair** if:
+
+- `0 <= i < j < n`, and
+- `lower <= nums[i] + nums[j] <= upper`
+
+
+**Example 1:**
+
+- **Input:** nums = [0,1,7,4,4,5], lower = 3, upper = 6
+- **Output:** 6
+- **Explanation:** There are 6 fair pairs: (0,3), (0,4), (0,5), (1,3), (1,4), and (1,5).
+
+**Example 2:**
+
+- **Input:** nums = [1,7,9,2,5], lower = 11, upper = 11
+- **Output:** 1
+- **Explanation:** There is a single fair pair: (2,3).
+
+
+**Constraints:**
+
+- 1 <= nums.length <= 105
+- nums.length == n
+- -109 <= nums[i] <= 109
+- -109 <= lower <= upper <= 109
+
+
+**Hint:**
+1. Sort the array in ascending order.
+2. For each number in the array, keep track of the smallest and largest numbers in the array that can form a fair pair with this number.
+3. As you move to larger number, both boundaries move down.
+
+
+
+**Solution:**
+
+We can use the following approach:
+
+1. **Sort the Array**: Sorting helps us leverage the two-pointer technique and perform binary searches more effectively.
+2. **Two-Pointer Technique**: For each element in the sorted array, we can calculate the range of elements that can form a fair pair with it. We use binary search to find this range.
+3. **Binary Search for Bounds**: For each element `nums[i]`, find the range `[lower, upper] - nums[i]` for `j > i`. We use binary search to find the smallest and largest indices that satisfy this range.
+
+Let's implement this solution in PHP: **[2563. Count the Number of Fair Pairs](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/002563-count-the-number-of-fair-pairs/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+1. **Sorting**: We sort the array `nums` to make it easier to find valid pairs with binary search.
+2. **Binary Search Bounds**:
+ - For each element `nums[i]`, we find the `low` and `high` values, which are the bounds we want the sum to fall within.
+ - We use two binary searches to find the range of indices `[left, right)` where `nums[i] + nums[j]` falls within `[lower, upper]`.
+3. **Counting Pairs**: We add the count of valid indices between `left` and `right` for each `i`.
+
+This approach has a time complexity of _**O(n log n)**_ due to sorting and binary search for each element, which is efficient enough for large inputs.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
diff --git a/algorithms/002563-count-the-number-of-fair-pairs/solution.php b/algorithms/002563-count-the-number-of-fair-pairs/solution.php
new file mode 100644
index 000000000..11f702c25
--- /dev/null
+++ b/algorithms/002563-count-the-number-of-fair-pairs/solution.php
@@ -0,0 +1,72 @@
+lowerBound($nums, $low, $i + 1);
+ $right = $this->upperBound($nums, $high, $i + 1);
+
+ $count += $right - $left;
+ }
+
+ return $count;
+ }
+
+ /**
+ * Helper function for binary search to find left boundary
+ *
+ * @param $arr
+ * @param $target
+ * @param $start
+ * @return int|mixed
+ */
+ function lowerBound($arr, $target, $start) {
+ $left = $start;
+ $right = count($arr);
+ while ($left < $right) {
+ $mid = intval(($left + $right) / 2);
+ if ($arr[$mid] < $target) {
+ $left = $mid + 1;
+ } else {
+ $right = $mid;
+ }
+ }
+ return $left;
+ }
+
+ /**
+ * Helper function for binary search to find right boundary
+ *
+ * @param $arr
+ * @param $target
+ * @param $start
+ * @return int|mixed
+ */
+ function upperBound($arr, $target, $start) {
+ $left = $start;
+ $right = count($arr);
+ while ($left < $right) {
+ $mid = intval(($left + $right) / 2);
+ if ($arr[$mid] <= $target) {
+ $left = $mid + 1;
+ } else {
+ $right = $mid;
+ }
+ }
+ return $left;
+ }
+}
\ No newline at end of file
diff --git a/algorithms/002577-minimum-time-to-visit-a-cell-in-a-grid/README.md b/algorithms/002577-minimum-time-to-visit-a-cell-in-a-grid/README.md
new file mode 100644
index 000000000..5f5275a81
--- /dev/null
+++ b/algorithms/002577-minimum-time-to-visit-a-cell-in-a-grid/README.md
@@ -0,0 +1,166 @@
+2577\. Minimum Time to Visit a Cell In a Grid
+
+**Difficulty:** Hard
+
+**Topics:** `Array`, `Breadth-First Search`, `Graph`, `Heap (Priority Queue)`, `Matrix`, `Shortest Path`
+
+You are given a `m x n` matrix `grid` consisting of **non-negative** integers where `grid[row][col]` represents the **minimum** time required to be able to visit the cell `(row, col)`, which means you can visit the cell `(row, col)` only when the time you visit it is greater than or equal to `grid[row][col]`.
+
+You are standing in the **top-left** cell of the matrix in the 0th
second, and you must move to **any** adjacent cell in the four directions: up, down, left, and right. Each move you make takes 1 second.
+
+Return _the **minimum** time required in which you can visit the bottom-right cell of the matrix. If you cannot visit the bottom-right cell, then return `-1`_.
+
+**Example 1:**
+
+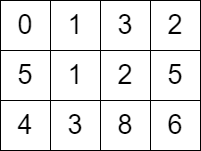
+
+- **Input:** grid = [[0,1,3,2],[5,1,2,5],[4,3,8,6]]
+- **Output:** 7
+- **Explanation:** One of the paths that we can take is the following:
+ - at t = 0, we are on the cell (0,0).
+ - at t = 1, we move to the cell (0,1). It is possible because grid[0][1] <= 1.
+ - at t = 2, we move to the cell (1,1). It is possible because grid[1][1] <= 2.
+ - at t = 3, we move to the cell (1,2). It is possible because grid[1][2] <= 3.
+ - at t = 4, we move to the cell (1,1). It is possible because grid[1][1] <= 4.
+ - at t = 5, we move to the cell (1,2). It is possible because grid[1][2] <= 5.
+ - at t = 6, we move to the cell (1,3). It is possible because grid[1][3] <= 6.
+ - at t = 7, we move to the cell (2,3). It is possible because grid[2][3] <= 7.
+ The final time is 7. It can be shown that it is the minimum time possible.
+
+**Example 2:**
+
+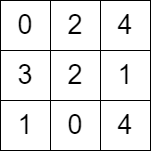
+
+- **Input:** grid = [[0,2,4],[3,2,1],[1,0,4]]
+- **Output:** -1
+- **Explanation:** There is no path from the top left to the bottom-right cell.
+
+**Constraints:**
+
+- `m == grid.length`
+- `n == grid[i].length`
+- `2 <= m, n <= 1000`
+- 4 <= m * n <= 10>sup>5
+- 0 <= grid[i][j] <= 10>sup>5
+- `grid[0][0] == 0`
+
+
+**Hint:**
+1. Try using some algorithm that can find the shortest paths on a graph.
+2. Consider the case where you have to go back and forth between two cells of the matrix to unlock some other cells.
+
+
+
+**Solution:**
+
+We can apply a modified version of **Dijkstra's algorithm** using a **priority queue**. This problem essentially asks us to find the shortest time required to visit the bottom-right cell from the top-left cell, where each move has a time constraint based on the values in the grid.
+
+### Approach:
+
+1. **Graph Representation**: Treat each cell in the grid as a node. The edges are the adjacent cells (up, down, left, right) that you can move to.
+
+2. **Priority Queue (Min-Heap)**: Use a priority queue to always explore the cell with the least time required. This ensures that we are processing the cells in order of the earliest time we can reach them.
+
+3. **Modified BFS**: For each cell, we will check if we can move to its neighboring cells and update the time at which we can visit them. If a cell is visited at a later time than the current, we add it back into the queue with the new time.
+
+4. **Early Exit**: Once we reach the bottom-right cell, we can return the time. If we exhaust the queue without reaching it, return `-1`.
+
+Let's implement this solution in PHP: **[2577. Minimum Time to Visit a Cell In a Grid](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/002577-minimum-time-to-visit-a-cell-in-a-grid/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+1. **Priority Queue**:
+ The `SplPriorityQueue` is used to ensure that the cells with the minimum time are processed first. The priority is stored as `-time` because PHP's `SplPriorityQueue` is a max-heap by default.
+
+2. **Traversal**:
+ Starting from the top-left cell `(0, 0)`, the algorithm processes all reachable cells, considering the earliest time each can be visited (`max(0, grid[newRow][newCol] - (time + 1))`).
+
+3. **Visited Cells**:
+ A `visited` array keeps track of cells that have already been processed to avoid redundant computations and infinite loops.
+
+4. **Boundary and Validity Check**:
+ The algorithm ensures we stay within the bounds of the grid and processes only valid neighbors.
+
+5. **Time Calculation**:
+ Each move takes one second, and if the cell requires waiting (i.e., `grid[newRow][newCol] > time + 1`), the algorithm waits until the required time.
+
+6. **Edge Case**:
+ If the queue is exhausted and the bottom-right cell is not reached, the function returns `-1`.
+
+---
+
+### Complexity Analysis
+
+1. **Time Complexity**:
+ - Each cell is added to the priority queue at most once: _**O(m x n x log(m x n))**_, where _**m**_ and _**n**_ are the grid dimensions.
+
+2. **Space Complexity**:
+ - The space for the priority queue and the `visited` array is _**O(m x n)**_.
+
+---
+
+### Example Runs
+
+#### Input:
+```php
+$grid = [
+ [0, 1, 3, 2],
+ [5, 1, 2, 5],
+ [4, 3, 8, 6]
+];
+echo minimumTime($grid); // Output: 7
+```
+
+#### Input:
+```php
+$grid = [
+ [0, 2, 4],
+ [3, 2, 1],
+ [1, 0, 4]
+];
+echo minimumTime($grid); // Output: -1
+```
+
+This solution is efficient and works well within the constraints.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
diff --git a/algorithms/002577-minimum-time-to-visit-a-cell-in-a-grid/solution.php b/algorithms/002577-minimum-time-to-visit-a-cell-in-a-grid/solution.php
new file mode 100644
index 000000000..e4c31d752
--- /dev/null
+++ b/algorithms/002577-minimum-time-to-visit-a-cell-in-a-grid/solution.php
@@ -0,0 +1,56 @@
+ 1 && $grid[1][0] > 1) {
+ return -1;
+ }
+
+ // Directional movements: right, down, left, up
+ $dirs = [[0, 1], [1, 0], [0, -1], [-1, 0]];
+
+ // Priority queue to store (time, row, col)
+ $minHeap = new SplPriorityQueue();
+ $minHeap->setExtractFlags(SplPriorityQueue::EXTR_DATA); // Extract data only
+ $minHeap->insert([0, 0, 0], 0); // (time, i, j)
+
+ // Seen array to track visited cells
+ $seen = array_fill(0, $m, array_fill(0, $n, false));
+ $seen[0][0] = true;
+
+ while (!$minHeap->isEmpty()) {
+ list($time, $i, $j) = $minHeap->extract();
+ if ($i === $m - 1 && $j === $n - 1) {
+ return $time; // Reached the bottom-right cell
+ }
+
+ foreach ($dirs as $dir) {
+ $x = $i + $dir[0];
+ $y = $j + $dir[1];
+
+ // Skip invalid or already visited cells
+ if ($x < 0 || $x >= $m || $y < 0 || $y >= $n || $seen[$x][$y]) {
+ continue;
+ }
+
+ // Calculate the waiting time if needed
+ $extraWait = ($grid[$x][$y] - $time) % 2 === 0 ? 1 : 0;
+ $nextTime = max($time + 1, $grid[$x][$y] + $extraWait);
+
+ $minHeap->insert([$nextTime, $x, $y], -$nextTime); // Use -$nextTime to mimic min-heap
+ $seen[$x][$y] = true;
+ }
+ }
+
+ return -1; // Unable to reach the bottom-right cell
+ }
+}
\ No newline at end of file
diff --git a/algorithms/002583-kth-largest-sum-in-a-binary-tree/README.md b/algorithms/002583-kth-largest-sum-in-a-binary-tree/README.md
new file mode 100644
index 000000000..13c9d96e7
--- /dev/null
+++ b/algorithms/002583-kth-largest-sum-in-a-binary-tree/README.md
@@ -0,0 +1,141 @@
+2583\. Kth Largest Sum in a Binary Tree
+
+**Difficulty:** Medium
+
+**Topics:** `Tree`, `Breadth-First Search`, `Sorting`, `Binary Tree`
+
+You are given the `root` of a binary tree and a positive integer `k`.
+
+The **level sum** in the tree is the sum of the values of the nodes that are on the **same** level.
+
+Return _the kth
**largest** level sum in the tree (not necessarily distinct)_. If there are fewer than `k` levels in the tree, return _`-1`_.
+
+**Note** that two nodes are on the same level if they have the same distance from the root.
+
+**Example 1:**
+
+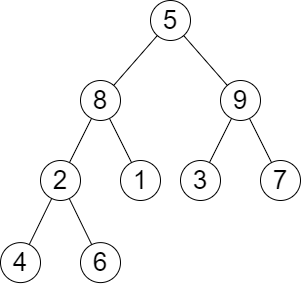
+
+- **Input:** root = [5,8,9,2,1,3,7,4,6], k = 2
+- **Output:** 13
+- **Explanation:** The level sums are the following:
+ - Level 1: 5.
+ - Level 2: 8 + 9 = 17.
+ - Level 3: 2 + 1 + 3 + 7 = 13.
+ - Level 4: 4 + 6 = 10.
+ The 2nd largest level sum is 13.
+
+**Example 2:**
+
+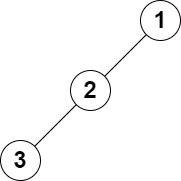
+
+- **Input:** root = [1,2,null,3], k = 1
+- **Output:** 3
+- **Explanation:** The largest level sum is 3.
+
+
+**Constraints:**
+
+- The number of nodes in the tree is `n`.
+- 2 <= n <= 105
+- 1 <= Node.val <= 106
+- `1 <= k <= n`
+
+
+**Hint:**
+1. Find the sum of values of nodes on each level and return the kth largest one.
+2. To find the sum of the values of nodes on each level, you can use a DFS or BFS algorithm to traverse the tree and keep track of the level of each node.
+
+
+
+**Solution:**
+
+We'll follow these steps:
+
+1. **Level Order Traversal**: We will use a Breadth-First Search (BFS) to traverse the tree level by level.
+2. **Calculate Level Sums**: During the BFS traversal, we'll calculate the sum of node values at each level.
+3. **Sort and Find k-th Largest Sum**: After calculating the sums for all levels, we'll sort the sums and retrieve the k-th largest value.
+
+Let's implement this solution in PHP: **[2583. Kth Largest Sum in a Binary Tree](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/002583-kth-largest-sum-in-a-binary-tree/solution.php)**
+
+```php
+val = $val;
+ $this->left = $left;
+ $this->right = $right;
+ }
+}
+
+/**
+ * @param TreeNode $root
+ * @param Integer $k
+ * @return Integer
+ */
+function kthLargestLevelSum($root, $k) {
+ ...
+ ...
+ ...
+ /**
+ * go to ./solution.php
+ */
+}
+
+// Example 1:
+// Input: root = [5,8,9,2,1,3,7,4,6], k = 2
+$root1 = new TreeNode(5);
+$root1->left = new TreeNode(8);
+$root1->right = new TreeNode(9);
+$root1->left->left = new TreeNode(2);
+$root1->left->right = new TreeNode(1);
+$root1->right->left = new TreeNode(3);
+$root1->right->right = new TreeNode(7);
+$root1->left->left->left = new TreeNode(4);
+$root1->left->left->right = new TreeNode(6);
+echo kthLargestLevelSum($root1, 2); // Output: 13
+
+// Example 2:
+// Input: root = [1,2,null,3], k = 1
+$root2 = new TreeNode(1);
+$root2->left = new TreeNode(2);
+$root2->left->left = new TreeNode(3);
+echo kthLargestLevelSum($root2, 1); // Output: 3
+?>
+```
+
+### Explanation:
+
+1. **TreeNode Class**: We define the `TreeNode` class to represent a node in the binary tree, where each node has a value (`val`), a left child (`left`), and a right child (`right`).
+
+2. **BFS Traversal**: The function `kthLargestLevelSum` uses a queue to perform a BFS traversal. For each level, we sum the values of the nodes and store the result in an array (`$levelSums`).
+
+3. **Sorting Level Sums**: After traversing the entire tree and calculating the level sums, we sort the sums in descending order using `rsort`. This allows us to easily access the k-th largest sum.
+
+4. **Edge Case Handling**: If there are fewer than `k` levels, we return `-1`.
+
+### Time Complexity:
+- **BFS Traversal**: O(n), where n is the number of nodes in the tree.
+- **Sorting**: O(m log m), where m is the number of levels in the tree. Since m is much smaller than n, sorting is relatively fast.
+- Overall time complexity: O(n + m log m).
+
+### Space Complexity:
+- **Queue**: O(n), for storing nodes during BFS.
+- **Level Sums**: O(m), for storing the sum of each level.
+- Overall space complexity: O(n).
+
+This approach ensures that we traverse the tree efficiently and return the correct kth **largest** level sum.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
+
diff --git a/algorithms/002583-kth-largest-sum-in-a-binary-tree/solution.php b/algorithms/002583-kth-largest-sum-in-a-binary-tree/solution.php
new file mode 100644
index 000000000..1b52358cf
--- /dev/null
+++ b/algorithms/002583-kth-largest-sum-in-a-binary-tree/solution.php
@@ -0,0 +1,62 @@
+val = $val;
+ * $this->left = $left;
+ * $this->right = $right;
+ * }
+ * }
+ */
+class Solution {
+
+ /**
+ * @param TreeNode $root
+ * @param Integer $k
+ * @return Integer
+ */
+ function kthLargestLevelSum($root, $k) {
+ if ($root === null) {
+ return -1;
+ }
+
+ // Use BFS to traverse the tree level by level
+ $queue = [];
+ array_push($queue, $root);
+ $levelSums = [];
+
+ // BFS loop
+ while (!empty($queue)) {
+ $levelSize = count($queue);
+ $currentLevelSum = 0;
+
+ // Process each node in the current level
+ for ($i = 0; $i < $levelSize; $i++) {
+ $node = array_shift($queue);
+ $currentLevelSum += $node->val;
+
+ // Add left and right children to the queue for the next level
+ if ($node->left !== null) {
+ array_push($queue, $node->left);
+ }
+ if ($node->right !== null) {
+ array_push($queue, $node->right);
+ }
+ }
+
+ // Store the sum of the current level
+ array_push($levelSums, $currentLevelSum);
+ }
+
+ // Sort the level sums in descending order
+ rsort($levelSums);
+
+ // Return the k-th largest level sum
+ return isset($levelSums[$k - 1]) ? $levelSums[$k - 1] : -1;
+ }
+}
\ No newline at end of file
diff --git a/algorithms/002593-find-score-of-an-array-after-marking-all-elements/README.md b/algorithms/002593-find-score-of-an-array-after-marking-all-elements/README.md
new file mode 100644
index 000000000..6d77e1896
--- /dev/null
+++ b/algorithms/002593-find-score-of-an-array-after-marking-all-elements/README.md
@@ -0,0 +1,125 @@
+2593\. Find Score of an Array After Marking All Elements
+
+**Difficulty:** Medium
+
+**Topics:** `Heap (Priority Queue)`, `Sorting`, `Array`, `Simulation`, `Hash Table`, `Ordered Set`, `Ordered Map`, `Greedy`, `Monotonic Stack`, `Sliding Window`, `Two Pointers`, `Stack`, `Queue`, `Bit Manipulation`, `Divide and Conquer`, `Dynamic Programming`, `Doubly-Linked List`, `Data Stream`, `Radix Sort`, `Backtracking`, `Bitmask`, `Tree`, `Design`, `Hash Function`, `String`, `Iterator`, `Counting Sort`, `Linked List`
+
+You are given an array `nums` consisting of positive integers.
+
+Starting with `score = 0`, apply the following algorithm:
+
+- Choose the smallest integer of the array that is not marked. If there is a tie, choose the one with the smallest index.
+- Add the value of the chosen integer to `score`.
+- Mark **the chosen element and its two adjacent elements if they exist**.
+- Repeat until all the array elements are marked.
+
+Return _the score you get after applying the above algorithm_.
+
+**Example 1:**
+
+- **Input:** nums = [2,1,3,4,5,2]
+- **Output:** 7
+- **Explanation:** We mark the elements as follows:
+ - 1 is the smallest unmarked element, so we mark it and its two adjacent elements: [2,1,3,4,5,2].
+ - 2 is the smallest unmarked element, so we mark it and its left adjacent element: [2,1,3,4,5,2].
+ - 4 is the only remaining unmarked element, so we mark it: [2,1,3,4,5,2].
+ Our score is 1 + 2 + 4 = 7.
+
+**Example 2:**
+
+- **Input:** nums = [2,3,5,1,3,2]
+- **Output:** 5
+- **Explanation:** We mark the elements as follows:
+ - 1 is the smallest unmarked element, so we mark it and its two adjacent elements: [2,3,5,1,3,2].
+ - 2 is the smallest unmarked element, since there are two of them, we choose the left-most one, so we mark the one at index 0 and its right adjacent element: [2,3,5,1,3,2].
+ - 2 is the only remaining unmarked element, so we mark it: [2,3,5,1,3,2].
+ Our score is 1 + 2 + 2 = 5.
+
+
+
+**Constraints:**
+
+- 1 <= nums.length <= 105
+- 1 <= nums[i] <= 106
+
+
+**Hint:**
+1. Try simulating the process of marking the elements and their adjacent.
+2. If there is an element that was already marked, then you skip it.
+
+
+
+**Solution:**
+
+We can simulate the marking process efficiently by using a sorted array or priority queue to keep track of the smallest unmarked element. So we can use the following approach:
+
+### Plan:
+
+1. **Input Parsing**: Read the array `nums` and initialize variables for the score and marking status.
+2. **Heap (Priority Queue)**:
+ - Use a **min-heap** to efficiently extract the smallest unmarked element in each step.
+ - Insert each element into the heap along with its index `(value, index)` to manage ties based on the smallest index.
+3. **Marking Elements**:
+ - Maintain a `marked` array to track whether an element and its adjacent ones are marked.
+ - When processing an element from the heap, skip it if it is already marked.
+ - Mark the current element and its two adjacent elements (if they exist).
+ - Add the value of the current element to the score.
+4. **Repeat**: Continue until all elements are marked.
+5. **Output**: Return the accumulated score.
+
+Let's implement this solution in PHP: **[2593. Find Score of an Array After Marking All Elements](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/002593-find-score-of-an-array-after-marking-all-elements/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+1. **Heap Construction**:
+ - The `usort` function sorts the array based on values, and by index when values are tied.
+ - This ensures that we always process the smallest unmarked element with the smallest index.
+
+2. **Marking Logic**:
+ - For each unmarked element, we mark it and its adjacent elements using the `marked` array.
+ - This ensures that we skip previously marked elements efficiently.
+
+3. **Time Complexity**:
+ - Sorting the heap: _**O(n log n)**_
+ - Processing the heap: _**O(n)**_
+ - Overall: _**O(n log n)**_, which is efficient for the given constraints.
+
+4. **Space Complexity**:
+ - Marked array: _**O(n)**_
+ - Heap: _**O(n)**_
+ - Total: _**O(n)**_
+
+This solution meets the constraints and works efficiently for large inputs.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
diff --git a/algorithms/002593-find-score-of-an-array-after-marking-all-elements/solution.php b/algorithms/002593-find-score-of-an-array-after-marking-all-elements/solution.php
new file mode 100644
index 000000000..a9ee1974c
--- /dev/null
+++ b/algorithms/002593-find-score-of-an-array-after-marking-all-elements/solution.php
@@ -0,0 +1,52 @@
+ 0) {
+ $marked[$index - 1] = true;
+ }
+ if ($index < $n - 1) {
+ $marked[$index + 1] = true;
+ }
+ }
+
+ return $score;
+ }
+}
\ No newline at end of file
diff --git a/algorithms/002601-prime-subtraction-operation/README.md b/algorithms/002601-prime-subtraction-operation/README.md
new file mode 100644
index 000000000..449cca8b6
--- /dev/null
+++ b/algorithms/002601-prime-subtraction-operation/README.md
@@ -0,0 +1,145 @@
+2601\. Prime Subtraction Operation
+
+**Difficulty:** Medium
+
+**Topics:** `Array`, `Math`, `Binary Search`, `Greedy`, `Number Theory`
+
+You are given a **0-indexed** integer array `nums` of length `n`.
+
+You can perform the following operation as many times as you want:
+
+- Pick an index `i` that you havenβt picked before, and pick a prime `p` **strictly less than** `nums[i]`, then subtract `p` from `nums[i]`.
+
+Return _true if you can make `nums` a strictly increasing array using the above operation and false otherwise_.
+
+A **strictly increasing array** is an array whose each element is strictly greater than its preceding element.
+
+**Example 1:**
+
+- **Input:** nums = [4,9,6,10]
+- **Output:** true
+- **Explanation:** In the first operation: Pick i = 0 and p = 3, and then subtract 3 from nums[0], so that nums becomes [1,9,6,10].
+ - In the second operation: i = 1, p = 7, subtract 7 from nums[1], so nums becomes equal to [1,2,6,10].
+ - After the second operation, nums is sorted in strictly increasing order, so the answer is true.
+
+**Example 2:**
+
+- **Input:** nums = [6,8,11,12]
+- **Output:** true
+- **Explanation:** Initially nums is sorted in strictly increasing order, so we don't need to make any operations.
+
+
+**Example 3:**
+
+- **Input:** nums = [5,8,3]
+- **Output:** false
+- **Explanation:** It can be proven that there is no way to perform operations to make nums sorted in strictly increasing order, so the answer is false.
+
+
+**Constraints:**
+
+- `1 <= nums.length <= 1000`
+- `1 <= nums[i] <= 1000`
+- `nums.length == n`
+
+
+**Hint:**
+1. Think about if we have many primes to subtract from `nums[i]`. Which prime is more optimal?
+2. The most optimal prime to subtract from `nums[i]` is the one that makes `nums[i]` the smallest as possible and greater than `nums[i-1]`.
+
+
+
+**Solution:**
+
+We need to break down the algorithm and adapt it to PHP syntax and functionality. The solution primarily involves the following steps:
+
+1. **Generating Primes (Sieve of Eratosthenes):** Generate a list of all primes up to the maximum possible value in `nums` (1000).
+2. **Prime Subtraction Operation:** For each number in `nums`, check if we can subtract a prime to make the array strictly increasing.
+3. **Binary Search for Prime:** Use a binary search to find the largest prime less than the current number that would still keep the sequence strictly increasing.
+
+Let's implement this solution in PHP: **[2601. Prime Subtraction Operation](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/002601-prime-subtraction-operation/solution.php)**
+
+```php
+primeSubOperation([4, 9, 6, 10]) ? 'true' : 'false'; // Output: true
+echo $solution->primeSubOperation([6, 8, 11, 12]) ? 'true' : 'false'; // Output: true
+echo $solution->primeSubOperation([5, 8, 3]) ? 'true' : 'false'; // Output: false
+?>
+```
+
+### Explanation:
+
+1. **primeSubOperation**: Loops through each element in `nums` and checks if we can make each element greater than the previous one by subtracting an appropriate prime.
+ - We use `$this->findLargestPrimeLessThan` to find the largest prime less than `num - prevNum`.
+ - If such a prime exists, we subtract it from the current `num`.
+ - If after subtracting the prime, the current `num` is not greater than `prevNum`, we return `false`.
+ - Otherwise, we update `prevNum` to the current `num`.
+
+2. **sieveEratosthenes**: Generates all primes up to 1000 using the Sieve of Eratosthenes and returns them as an array.
+
+3. **findLargestPrimeLessThan**: Uses binary search to find the largest prime less than a given limit, ensuring we find the optimal prime for subtraction.
+
+### Complexity Analysis
+
+- **Time Complexity**: _**O(n . √m)**_, where _**n**_ is the length of `nums` and m is the maximum value of an element in `nums` (here _**m = 1000**_).
+- **Space Complexity**: _**O(m)**_, used to store the list of primes up to `1000`.
+
+This solution will return `true` or `false` based on whether it is possible to make `nums` strictly increasing by performing the described prime subtraction operations.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
diff --git a/algorithms/002601-prime-subtraction-operation/solution.php b/algorithms/002601-prime-subtraction-operation/solution.php
new file mode 100644
index 000000000..d6717a110
--- /dev/null
+++ b/algorithms/002601-prime-subtraction-operation/solution.php
@@ -0,0 +1,77 @@
+sieveEratosthenes($maxNum);
+
+ $prevNum = 0;
+ foreach ($nums as &$num) {
+ // Find the largest prime `p` that makes `num - p > prevNum`
+ $prime = $this->findLargestPrimeLessThan($primes, $num - $prevNum);
+ if ($prime !== null) {
+ $num -= $prime;
+ }
+
+ // Check if the current number is greater than the previous number
+ if ($num <= $prevNum) {
+ return false;
+ }
+
+ $prevNum = $num;
+ }
+
+ return true;
+ }
+
+ /**
+ * Helper function to generate all primes up to n using Sieve of Eratosthenes
+ *
+ * @param $n
+ * @return array
+ */
+ private function sieveEratosthenes($n) {
+ $isPrime = array_fill(0, $n + 1, true);
+ $isPrime[0] = $isPrime[1] = false;
+ $primes = [];
+
+ for ($i = 2; $i <= $n; $i++) {
+ if ($isPrime[$i]) {
+ $primes[] = $i;
+ for ($j = $i * $i; $j <= $n; $j += $i) {
+ $isPrime[$j] = false;
+ }
+ }
+ }
+
+ return $primes;
+ }
+
+ /**
+ * Helper function to find the largest prime less than a given limit using binary search
+ *
+ * @param $primes
+ * @param $limit
+ * @return mixed|null
+ */
+ private function findLargestPrimeLessThan($primes, $limit) {
+ $left = 0;
+ $right = count($primes) - 1;
+
+ while ($left <= $right) {
+ $mid = $left + (int)(($right - $left) / 2);
+ if ($primes[$mid] < $limit) {
+ $left = $mid + 1;
+ } else {
+ $right = $mid - 1;
+ }
+ }
+
+ return $right >= 0 ? $primes[$right] : null;
+ }
+}
\ No newline at end of file
diff --git a/algorithms/002641-cousins-in-binary-tree-ii/README.md b/algorithms/002641-cousins-in-binary-tree-ii/README.md
new file mode 100644
index 000000000..076c8d4ba
--- /dev/null
+++ b/algorithms/002641-cousins-in-binary-tree-ii/README.md
@@ -0,0 +1,243 @@
+2641\. Cousins in Binary Tree II
+
+**Difficulty:** Medium
+
+**Topics:** `Hash Table`, `Tree`, `Depth-First Search`, `Breadth-First Search`, `Binary Tree`
+
+Given the `root` of a binary tree, replace the value of each node in the tree with the **sum of all its cousins' values**.
+
+Two nodes of a binary tree are **cousins** if they have the same depth with different parents.
+
+Return _the `root` of the modified tree_.
+
+**Note** that the depth of a node is the number of edges in the path from the root node to it.
+
+**Example 1:**
+
+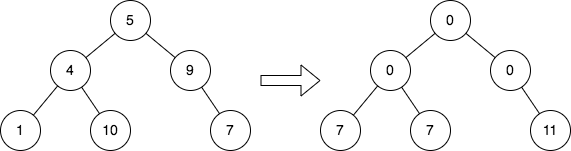
+
+- **Input:** root = [5,4,9,1,10,null,7]
+- **Output:** [0,0,0,7,7,null,11]
+- **Explanation:** The diagram above shows the initial binary tree and the binary tree after changing the value of each node.
+ - Node with value 5 does not have any cousins so its sum is 0.
+ - Node with value 4 does not have any cousins so its sum is 0.
+ - Node with value 9 does not have any cousins so its sum is 0.
+ - Node with value 1 has a cousin with value 7 so its sum is 7.
+ - Node with value 10 has a cousin with value 7 so its sum is 7.
+ - Node with value 7 has cousins with values 1 and 10 so its sum is 11.
+
+**Example 2:**
+
+
+
+- **Input:** root = [3,1,2]
+- **Output:** [0,0,0]
+- **Explanation:** The diagram above shows the initial binary tree and the binary tree after changing the value of each node.
+ - Node with value 3 does not have any cousins so its sum is 0.
+ - Node with value 1 does not have any cousins so its sum is 0.
+ - Node with value 2 does not have any cousins so its sum is 0.
+
+
+**Constraints:**
+
+- The number of nodes in the tree is in the range [1, 105]
.
+- 1 <= Node.val <= 104
+
+
+**Hint:**
+1. Use DFS two times.
+2. For the first time, find the sum of values of all the levels of the binary tree.
+3. For the second time, update the value of the node with the sum of the values of the current level - sibling nodeβs values.
+
+
+
+**Solution:**
+
+The solution uses a Depth-First Search (DFS) approach twice:
+1. **First DFS:** Calculate the sum of all node values at each level of the tree.
+2. **Second DFS:** Update each node's value with the sum of its cousins' values by subtracting its siblings' values from the total for that level.
+
+Let's implement this solution in PHP: **[2641. Cousins in Binary Tree II](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/002641-cousins-in-binary-tree-ii/solution.php)**
+
+```php
+val = $val;
+ $this->left = $left;
+ $this->right = $right;
+ }
+}
+
+class Solution {
+
+ /**
+ * @param TreeNode $root
+ * @return TreeNode
+ */
+ public function replaceValueInTree($root) {
+ ...
+ ...
+ ...
+ /**
+ * go to ./solution.php
+ */
+ }
+
+ /**
+ * DFS to calculate the sum of node values at each level.
+ * @param $root - current node
+ * @param $level - current depth level in the tree
+ * @param $levelSums - array holding the sum of values at each level
+ * @return void
+ */
+ private function dfs($root, $level, &$levelSums) {
+ ...
+ ...
+ ...
+ /**
+ * go to ./solution.php
+ */
+ }
+
+ /**
+ * DFS to replace the node values with the sum of cousins' values.
+ * @param $root - current node in the original tree
+ * @param $level - current depth level in the tree
+ * @param $curr - node being modified in the new tree
+ * @param $levelSums - array holding the sum of values at each level
+ * @return mixed
+ */
+ private function replace($root, $level, $curr, $levelSums) {
+ ...
+ ...
+ ...
+ /**
+ * go to ./solution.php
+ */
+ }
+}
+
+// Helper function to print the tree (for testing purpose)
+function printTree($root) {
+ if ($root === null) return [];
+
+ $result = [];
+ $queue = [$root];
+
+ while (!empty($queue)) {
+ $node = array_shift($queue);
+ if ($node !== null) {
+ $result[] = $node->val;
+ $queue[] = $node->left;
+ $queue[] = $node->right;
+ } else {
+ $result[] = null;
+ }
+ }
+
+ // Remove trailing null values for clean output
+ while (end($result) === null) {
+ array_pop($result);
+ }
+
+ return $result;
+}
+
+// Example usage:
+
+// Tree: [5,4,9,1,10,null,7]
+$root = new TreeNode(5);
+$root->left = new TreeNode(4);
+$root->right = new TreeNode(9);
+$root->left->left = new TreeNode(1);
+$root->left->right = new TreeNode(10);
+$root->right->right = new TreeNode(7);
+
+$solution = new Solution();
+$modifiedTree = $solution->replaceValueInTree($root);
+
+print_r(printTree($modifiedTree)); // Output: [0, 0, 0, 7, 7, null, 11]
+?>
+```
+
+### Breakdown of the Code
+
+#### 1. `replaceValueInTree` Method
+- This is the main method that initializes the process.
+- It creates an empty array, `$levelSums`, to hold the sum of values at each level.
+- It calls the first DFS to populate `$levelSums` and then calls the second DFS to replace the values in the tree.
+
+#### 2. `dfs` Method (First DFS)
+- **Parameters:**
+ - `$root`: Current node.
+ - `$level`: Current depth level of the tree.
+ - `&$levelSums`: Reference to the array where sums will be stored.
+
+- **Base Case:** If the node is `null`, return.
+- If the current level's sum is not initialized (i.e., the level does not exist in the array), initialize it to `0`.
+- Add the current node's value to the sum for its level.
+- Recursively call `dfs` for the left and right children, incrementing the level by 1.
+
+#### 3. `replace` Method (Second DFS)
+- **Parameters:**
+ - `$root`: Current node.
+ - `$level`: Current depth level.
+ - `$curr`: Current node in the modified tree.
+ - `$levelSums`: The array with sums for each level.
+
+- Calculate the sum of cousins' values:
+ - Get the total sum for the next level.
+ - Subtract the values of the current node's children (siblings) from this total to get the cousins' sum.
+
+- If the left child exists, create a new `TreeNode` with the cousins' sum and recursively call `replace` for it.
+- If the right child exists, do the same for the right child.
+
+### Example Walkthrough
+
+Let's use the first example from the prompt:
+
+#### Input
+```
+root = [5,4,9,1,10,null,7]
+```
+
+1. **First DFS (Calculate Level Sums):**
+ - Level 0: `5` β Sum = `5`
+ - Level 1: `4 + 9` β Sum = `13`
+ - Level 2: `1 + 10 + 7` β Sum = `18`
+ - Final `levelSums`: `[5, 13, 18]`
+
+2. **Second DFS (Replace Values):**
+ - At Level 0: `5` has no cousins β Replace with `0`.
+ - At Level 1:
+ - `4` β Cousins = `9` β Replace with `9` (from Level 1 total, no siblings).
+ - `9` β Cousins = `4` β Replace with `4`.
+ - At Level 2:
+ - `1` β Cousins = `10 + 7 = 17` β Replace with `17`.
+ - `10` β Cousins = `1 + 7 = 8` β Replace with `8`.
+ - `7` β Cousins = `1 + 10 = 11` β Replace with `11`.
+
+#### Output
+```
+Output: [0, 0, 0, 7, 7, null, 11]
+```
+
+This step-by-step replacement based on cousin values results in the modified tree as shown in the example.
+
+### Summary
+- The solution uses two DFS traversals: one for calculating the sums and the other for replacing node values.
+- The logic ensures each node is updated based on its cousin nodes' values while maintaining the structure of the binary tree.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
diff --git a/algorithms/002641-cousins-in-binary-tree-ii/solution.php b/algorithms/002641-cousins-in-binary-tree-ii/solution.php
new file mode 100644
index 000000000..031481167
--- /dev/null
+++ b/algorithms/002641-cousins-in-binary-tree-ii/solution.php
@@ -0,0 +1,84 @@
+val = $val;
+ * $this->left = $left;
+ * $this->right = $right;
+ * }
+ * }
+ */
+
+
+class Solution {
+
+ /**
+ * @param TreeNode $root
+ * @return TreeNode
+ */
+ public function replaceValueInTree($root) {
+ $levelSums = [];
+ $this->dfs($root, 0, $levelSums);
+ return $this->replace($root, 0, new TreeNode(0), $levelSums);
+ }
+
+ /**
+ * DFS to calculate the sum of node values at each level.
+ * @param $root - current node
+ * @param $level - current depth level in the tree
+ * @param $levelSums - array holding the sum of values at each level
+ * @return void
+ */
+ private function dfs($root, $level, &$levelSums) {
+ if ($root === null) {
+ return;
+ }
+
+ if (count($levelSums) == $level) {
+ $levelSums[] = 0; // Initialize the sum for this level
+ }
+
+ $levelSums[$level] += $root->val; // Add current node's value to level sum
+
+ // Recursive DFS calls for left and right children
+ $this->dfs($root->left, $level + 1, $levelSums);
+ $this->dfs($root->right, $level + 1, $levelSums);
+ }
+
+ /**
+ * DFS to replace the node values with the sum of cousins' values.
+ * @param $root - current node in the original tree
+ * @param $level - current depth level in the tree
+ * @param $curr - node being modified in the new tree
+ * @param $levelSums - array holding the sum of values at each level
+ * @return mixed
+ */
+ private function replace($root, $level, $curr, $levelSums) {
+ $nextLevel = $level + 1;
+
+ // Calculate the sum of cousins' values
+ $nextLevelCousinsSum = ($nextLevel >= count($levelSums)) ? 0 :
+ $levelSums[$nextLevel] -
+ (($root->left === null) ? 0 : $root->left->val) -
+ (($root->right === null) ? 0 : $root->right->val);
+
+ // Update left child if it exists
+ if ($root->left !== null) {
+ $curr->left = new TreeNode($nextLevelCousinsSum);
+ $this->replace($root->left, $level + 1, $curr->left, $levelSums);
+ }
+
+ // Update right child if it exists
+ if ($root->right !== null) {
+ $curr->right = new TreeNode($nextLevelCousinsSum);
+ $this->replace($root->right, $level + 1, $curr->right, $levelSums);
+ }
+
+ return $curr;
+ }
+}
\ No newline at end of file
diff --git a/algorithms/002657-find-the-prefix-common-array-of-two-arrays/README.md b/algorithms/002657-find-the-prefix-common-array-of-two-arrays/README.md
new file mode 100644
index 000000000..93f75d4e0
--- /dev/null
+++ b/algorithms/002657-find-the-prefix-common-array-of-two-arrays/README.md
@@ -0,0 +1,119 @@
+2657\. Find the Prefix Common Array of Two Arrays
+
+**Difficulty:** Medium
+
+**Topics:** `Array`, `Hash Table`, `Bit Manipulation`
+
+You are given two **0-indexed** integer permutations `A` and `B` of length `n`.
+
+A **prefix common array** of `A` and `B` is an array `C` such that `C[i]` is equal to the count of numbers that are present at or before the index `i` in both `A` and `B`.
+
+Return _the **prefix common array** of `A` and `B`_.
+
+A sequence of `n` integers is called a **permutation** if it contains all integers from `1` to `n` exactly once.
+
+**Example 1:**
+
+- **Input:** A = [1,3,2,4], B = [3,1,2,4]
+- **Output:** [0,2,3,4]
+- **Explanation:** At i = 0: no number is common, so C[0] = 0.
+ At i = 1: 1 and 3 are common in A and B, so C[1] = 2.
+ At i = 2: 1, 2, and 3 are common in A and B, so C[2] = 3.
+ At i = 3: 1, 2, 3, and 4 are common in A and B, so C[3] = 4.
+
+**Example 2:**
+
+- **Input:** A = [2,3,1], B = [3,1,2]
+- **Output:** [0,1,3]
+- **Explanation:** At i = 0: no number is common, so C[0] = 0.
+ At i = 1: only 3 is common in A and B, so C[1] = 1.
+ At i = 2: 1, 2, and 3 are common in A and B, so C[2] = 3.
+
+
+
+**Constraints:**
+
+- `1 <= A.length == B.length == n <= 50`
+- `1 <= A[i], B[i] <= n`
+- `It is guaranteed that A and B are both a permutation of n integers.`
+
+
+**Hint:**
+1. Consider keeping a frequency array that stores the count of occurrences of each number till index i.
+2. If a number occurred two times, it means it occurred in both A and B since theyβre both permutations so add one to the answer.
+
+
+
+**Solution:**
+
+We can iterate over the two arrays A and B while keeping track of the numbers that have occurred at or before the current index in both arrays. Since both arrays are permutations of the same set of numbers, we can utilize two hash sets (or arrays) to store which numbers have appeared at or before the current index in both arrays. For each index, we can count the common numbers that have appeared in both arrays up to that point.
+
+### Solution Approach:
+1. Use two arrays to keep track of the occurrences of numbers in both `A` and `B` up to index `i`.
+2. For each index `i`, check if both `A[i]` and `B[i]` have been seen before. If so, increment the common count.
+3. Use a frequency array to track the presence of numbers from `1` to `n` in both arrays.
+
+Let's implement this solution in PHP: **[2657. Find the Prefix Common Array of Two Arrays](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/002657-find-the-prefix-common-array-of-two-arrays/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+1. **Frequency Arrays**: We maintain two frequency arrays, `freqA` and `freqB`, where each index represents a number in the permutation.
+ - When we encounter a number in `A[i]` or `B[i]`, we increase the corresponding value in the frequency array.
+2. **Common Count**: After updating the frequency arrays for both `A[i]` and `B[i]`, we check for each number if it has appeared in both arrays up to index `i`. If so, we increase the `commonCount`.
+3. **Result**: The common count is stored in the `result` array for each index.
+
+### Example Walkthrough:
+
+For the input:
+```php
+$A = [1, 3, 2, 4];
+$B = [3, 1, 2, 4];
+```
+
+- At `i = 0`: No common numbers yet β `C[0] = 0`
+- At `i = 1`: Numbers `1` and `3` are common β `C[1] = 2`
+- At `i = 2`: Numbers `1`, `2`, and `3` are common β `C[2] = 3`
+- At `i = 3`: Numbers `1`, `2`, `3`, and `4` are common β `C[3] = 4`
+
+Output: `[0, 2, 3, 4]`
+
+### Time Complexity:
+- **O(n2)**: For each index `i`, we are checking every element from `1` to `n` to see if it's common, making this solution quadratic in time complexity. This is acceptable given the constraint _**n β€ 50**_.
+
+This should work within the given constraints effectively.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
diff --git a/algorithms/002657-find-the-prefix-common-array-of-two-arrays/solution.php b/algorithms/002657-find-the-prefix-common-array-of-two-arrays/solution.php
new file mode 100644
index 000000000..2f664c55d
--- /dev/null
+++ b/algorithms/002657-find-the-prefix-common-array-of-two-arrays/solution.php
@@ -0,0 +1,36 @@
+ 0 && $freqB[$j] > 0) {
+ $commonCount++;
+ }
+ }
+
+ // Store the common count in the result array
+ $result[] = $commonCount;
+ }
+
+ return $result;
+ }
+}
\ No newline at end of file
diff --git a/algorithms/002683-neighboring-bitwise-xor/README.md b/algorithms/002683-neighboring-bitwise-xor/README.md
new file mode 100644
index 000000000..a1fdc3aa7
--- /dev/null
+++ b/algorithms/002683-neighboring-bitwise-xor/README.md
@@ -0,0 +1,136 @@
+2683\. Neighboring Bitwise XOR
+
+**Difficulty:** Medium
+
+**Topics:** `Array`, `Bit Manipulation`
+
+A **0-indexed** array `derived` with length `n` is derived by computing the **bitwise XOR** (β) of adjacent values in a **binary array** `original` of length `n`.
+
+Specifically, for each index `i` in the range `[0, n - 1]`:
+
+- If `i = n - 1`, then `derived[i] = original[i] β original[0]`.
+- Otherwise, `derived[i] = original[i] β original[i + 1]`.
+
+Given an array `derived`, your task is to determine whether there exists a **valid binary array** `original` that could have formed `derived`.
+
+Return _**true** if such an array exists or **false** otherwise_.
+
+- A binary array is an array containing only **0's** and **1's**
+
+
+**Example 1:**
+
+- **Input:** derived = [1,1,0]
+- **Output:** true
+- **Explanation:** A valid original array that gives derived is [0,1,0].
+ derived[0] = original[0] β original[1] = 0 β 1 = 1
+ derived[1] = original[1] β original[2] = 1 β 0 = 1
+ derived[2] = original[2] β original[0] = 0 β 0 = 0
+
+**Example 2:**
+
+- **Input:** derived = [1,1]
+- **Output:** true
+- **Explanation:** A valid original array that gives derived is [0,1].
+ derived[0] = original[0] β original[1] = 1
+ derived[1] = original[1] β original[0] = 1
+
+
+**Example 3:**
+
+- **Input:** derived = [1,0]
+- **Output:** false
+- **Explanation:** There is no valid original array that gives derived.
+
+
+
+**Constraints:**
+
+- `n == derived.length`
+- 1 <= n <= 105
+- The values in `derived` are either **0's** or **1's**
+
+
+**Hint:**
+1. Understand that from the original element, we are using each element twice to construct the derived array
+2. The xor-sum of the derived array should be 0 since there is always a duplicate occurrence of each element.
+
+
+
+**Solution:**
+
+To determine whether a valid binary array `original` exists that could form the given `derived` array, we can use the properties of XOR:
+
+### Key Observations:
+1. Each `derived[i]` is the XOR of two adjacent elements in `original`:
+ - For `i = n - 1`, `derived[i] = original[n-1] β original[0]`.
+ - Otherwise, `derived[i] = original[i] β original[i+1]`.
+
+2. XOR properties:
+ - `a β a = 0` (XOR of a number with itself is 0).
+ - `a β 0 = a` (XOR of a number with 0 is the number itself).
+ - XOR is commutative and associative.
+
+3. To construct a valid `original`, the XOR of all elements in `derived` must equal 0. Why? Because for a circular XOR relationship to work (start and end wrap back to the beginning), the cumulative XOR of `derived` should cancel out.
+
+### Steps:
+1. Compute the XOR of all elements in `derived`.
+2. If the result is 0, a valid `original` exists; otherwise, it does not.
+
+### Algorithm:
+- Compute the XOR of all elements in `derived`.
+- If the XOR is 0, return `true`.
+- Otherwise, return `false`.
+
+Let's implement this solution in PHP: **[2683. Neighboring Bitwise XOR](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/002683-neighboring-bitwise-xor/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+1. We initialize `$xorSum` to 0.
+2. For each element in `derived`, we XOR it with `$xorSum`.
+3. At the end of the loop, `$xorSum` will contain the XOR of all elements in `derived`.
+4. If `$xorSum` is 0, return `true`; otherwise, return `false`.
+
+### Complexity:
+- **Time Complexity:** _**O(n)**_, where _**n**_ is the length of `derived`. We only iterate through the array once.
+- **Space Complexity:** _**O(1)**_, as we only use a single variable to compute the XOR.
+
+This approach efficiently checks for the existence of a valid `original` array within the given constraints.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
diff --git a/algorithms/002683-neighboring-bitwise-xor/solution.php b/algorithms/002683-neighboring-bitwise-xor/solution.php
new file mode 100644
index 000000000..e4422fec2
--- /dev/null
+++ b/algorithms/002683-neighboring-bitwise-xor/solution.php
@@ -0,0 +1,20 @@
+ (0, 1).
+ - (0, 1) -> (1, 2).
+ - (1, 2) -> (2, 3).
+ It can be shown that it is the maximum number of moves that can be made.
+
+**Example 2:**
+
+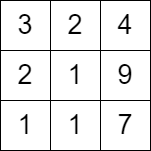
+
+- **Input:** grid = [[3,2,4],[2,1,9],[1,1,7]]
+- **Output:** 0
+- **Explanation:** Starting from any cell in the first column we cannot perform any moves.
+
+
+
+**Constraints:**
+
+- `m == grid.length`
+- `n == grid[i].length`
+- `2 <= m, n <= 1000`
+- 4 <= m * n <= 105
+- 1 <= grid[i][j] <= 106
+
+
+**Hint:**
+1. Consider using dynamic programming to find the maximum number of moves that can be made from each cell.
+2. The final answer will be the maximum value in cells of the first column.
+
+
+
+**Solution:**
+
+We can use **Dynamic Programming** (DP) to keep track of the maximum number of moves from each cell, starting from any cell in the first column. Hereβs the step-by-step approach:
+
+### Approach:
+
+1. **Define DP Array**: Let `dp[row][col]` represent the maximum number of moves possible starting from `grid[row][col]`. Initialize this with `0` for all cells.
+
+2. **Traverse the Grid**:
+ - Start from the last column and move backward to the first column. For each cell in column `col`, calculate possible moves for `col-1`.
+ - Update `dp[row][col]` based on possible moves `(row - 1, col + 1)`, `(row, col + 1)`, and `(row + 1, col + 1)`, only if the value of the destination cell is **strictly greater** than the current cell.
+
+3. **Calculate the Maximum Moves**:
+ - After filling out the `dp` table, the result will be the maximum value in the first column of `dp`, as it represents the maximum moves starting from any cell in the first column.
+
+4. **Edge Cases**:
+ - Handle cases where no moves are possible (e.g., when all paths are blocked by lower or equal values in neighboring cells).
+
+Let's implement this solution in PHP: **[2684. Maximum Number of Moves in a Grid](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/002684-maximum-number-of-moves-in-a-grid/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+1. **`dp` Initialization**: We create a 2D array `dp` to store the maximum moves from each cell.
+2. **Loop through Columns**: We iterate from the second-last column to the first, updating `dp[row][col]` based on possible moves to neighboring cells in the next column.
+3. **Maximum Moves Calculation**: Finally, the maximum value in the first column of `dp` gives the result.
+
+### Complexity Analysis:
+- **Time Complexity**: _**O(m x n)**_ since we process each cell once.
+- **Space Complexity**: _**O(m x n)**_ for the `dp` array.
+
+This solution is efficient given the constraints and will work within the provided limits.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
diff --git a/algorithms/002684-maximum-number-of-moves-in-a-grid/solution.php b/algorithms/002684-maximum-number-of-moves-in-a-grid/solution.php
new file mode 100644
index 000000000..ff9b0d63e
--- /dev/null
+++ b/algorithms/002684-maximum-number-of-moves-in-a-grid/solution.php
@@ -0,0 +1,43 @@
+= 0; $col--) {
+ for ($row = 0; $row < $m; $row++) {
+ // Possible moves (right-up, right, right-down)
+ $moves = [
+ [$row - 1, $col + 1],
+ [$row, $col + 1],
+ [$row + 1, $col + 1]
+ ];
+
+ foreach ($moves as $move) {
+ list($newRow, $newCol) = $move;
+ if ($newRow >= 0 && $newRow < $m && $grid[$newRow][$newCol] > $grid[$row][$col]) {
+ $dp[$row][$col] = max($dp[$row][$col], $dp[$newRow][$newCol] + 1);
+ }
+ }
+ }
+ }
+
+ // Find the maximum moves starting from any cell in the first column
+ $maxMoves = 0;
+ for ($row = 0; $row < $m; $row++) {
+ $maxMoves = max($maxMoves, $dp[$row][0]);
+ }
+
+ return $maxMoves;
+ }
+}
\ No newline at end of file
diff --git a/algorithms/002696-minimum-string-length-after-removing-substrings/README.md b/algorithms/002696-minimum-string-length-after-removing-substrings/README.md
new file mode 100644
index 000000000..2a6761f12
--- /dev/null
+++ b/algorithms/002696-minimum-string-length-after-removing-substrings/README.md
@@ -0,0 +1,108 @@
+2696\. Minimum String Length After Removing Substrings
+
+**Difficulty:** Easy
+
+**Topics:** `String`, `Stack`, `Simulation`
+
+You are given a string s consisting only of **uppercase** English letters.
+
+You can apply some operations to this string where, in one operation, you can remove any occurrence of one of the substrings `"AB"` or `"CD"` from `s`.
+
+Return _the **minimum** possible length of the resulting string that you can obtain_.
+
+**Note** that the string concatenates after removing the substring and could produce new `"AB"` or `"CD"` substrings.
+
+**Example 1:**
+
+- **Input:** s = "ABFCACDB"
+- **Output:** 2
+- **Explanation:** We can do the following operations:
+ - Remove the substring "ABFCACDB", so s = "FCACDB".
+ - Remove the substring "FCACDB", so s = "FCAB".
+ - Remove the substring "FCAB", so s = "FC".\
+ So the resulting length of the string is 2.\
+ It can be shown that it is the minimum length that we can obtain.
+
+**Example 2:**
+
+- **Input:** s = "ACBBD"
+- **Output:** 5
+- **Explanation:** We cannot do any operations on the string so the length remains the same.
+
+
+**Constraints:**
+
+- `1 <= s.length <= 100`
+- `s` consists only of uppercase English letters.
+
+
+**Hint:**
+1. Can we use brute force to solve the problem?
+2. Repeatedly traverse the string to find and remove the substrings βABβ and βCDβ until no more occurrences exist.
+
+
+
+**Solution:**
+
+We'll use a **stack** to handle the removal of substrings `"AB"` and `"CD"`. The stack approach ensures that we efficiently remove these substrings as they occur during traversal of the string.
+
+### Approach:
+
+1. **Use a Stack**:
+ - Traverse the string character by character.
+ - Push each character onto the stack.
+ - If the top two characters on the stack form the substring `"AB"` or `"CD"`, pop these two characters from the stack (remove them).
+ - Continue this process for all characters in the input string.
+2. **Final String**:
+ - At the end of the traversal, the stack will contain the reduced string.
+ - The minimum possible length will be the size of the stack.
+
+Let's implement this solution in PHP: **[2696. Minimum String Length After Removing Substrings](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/002696-minimum-string-length-after-removing-substrings/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+- We initialize an empty stack (`$stack`).
+- Loop through each character of the string `s`.
+- Check the top character of the stack:
+ - If the top character and the current character form the substrings `"AB"` or `"CD"`, we remove the top character using `array_pop`.
+ - Otherwise, push the current character onto the stack.
+- The stack will hold the characters that remain after all possible removals.
+- Finally, `count($stack)` gives the length of the resulting string.
+
+### Complexity:
+
+- **Time Complexity**: `O(n)`, where `n` is the length of the string. Each character is processed at most twice (once pushed, once popped).
+- **Space Complexity**: `O(n)` for the stack, in the worst case where no removals are possible.
+
+This solution effectively minimizes the string by removing all possible occurrences of `"AB"` and `"CD"` until no more can be found.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
diff --git a/algorithms/002696-minimum-string-length-after-removing-substrings/solution.php b/algorithms/002696-minimum-string-length-after-removing-substrings/solution.php
new file mode 100644
index 000000000..3924f1492
--- /dev/null
+++ b/algorithms/002696-minimum-string-length-after-removing-substrings/solution.php
@@ -0,0 +1,35 @@
+edges[i] = [ai, bi, wi] indicates that there is an edge between nodes ai
and bi
with weight wi
.
+
+Some edges have a weight of `-1` (wi = -1
), while others have a **positive** weight (wi > 0
).
+
+Your task is to modify **all edges** with a weight of `-1` by assigning them **positive integer values** in the range [1, 2 * 109]
so that the **shortest distance** between the nodes `source` and `destination` becomes equal to an integer `target`. If there are **multiple modifications** that make the shortest distance between `source` and `destination` equal to `target`, any of them will be considered correct.
+
+Return _an array containing all edges (even unmodified ones) in any order if it is possible to make the shortest distance from `source` to `destination` equal to `target`, or an **empty array** if it's impossible_.
+
+**Note:** You are not allowed to modify the weights of edges with initial positive weights.
+
+**Example 1:**
+
+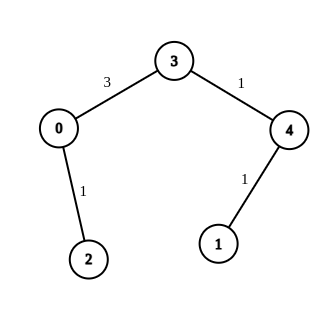
+
+- **Input:** n = 5, edges = [[4,1,-1],[2,0,-1],[0,3,-1],[4,3,-1]], source = 0, destination = 1, target = 5
+- **Output:** [[4,1,1],[2,0,1],[0,3,3],[4,3,1]]
+- **Explanation:** The graph above shows a possible modification to the edges, making the distance from 0 to 1 equal to 5.
+
+**Example 2:**
+
+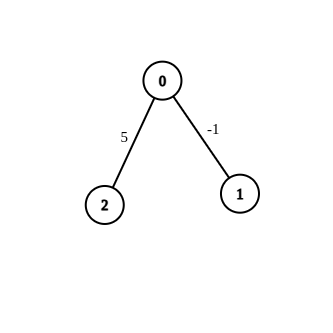
+
+- **Input:** n = 3, edges = [[0,1,-1],[0,2,5]], source = 0, destination = 2, target = 6
+- **Output:** []
+- **Explanation:** The graph above contains the initial edges. It is not possible to make the distance from 0 to 2 equal to 6 by modifying the edge with weight -1. So, an empty array is returned.
+
+
+**Example 3:**
+
+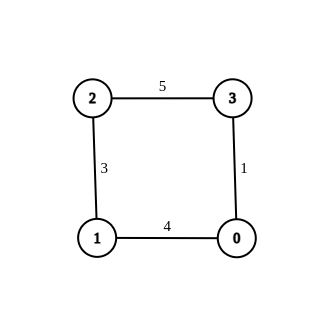
+
+- **Input:** n = 4, edges = [[1,0,4],[1,2,3],[2,3,5],[0,3,-1]], source = 0, destination = 2, target = 6
+- **Output:** [[1,0,4],[1,2,3],[2,3,5],[0,3,1]]
+- **Explanation:** The graph above shows a modified graph having the shortest distance from 0 to 2 as 6.
+
+
+
+**Constraints:**
+
+- `1 <= n <= 100`
+- `1 <= edges.length <= n * (n - 1) / 2`
+- `edges[i].length == 3`
+- 0 <= ai, bi < n
+- wi = -1
or 1 <= wi <= 107
+- ai != bi
+- `0 <= source, destination < n`
+- `source != destination`
+- 1 <= target <= 109
+- The graph is connected, and there are no self-loops or repeated edges
+
+**Hint:**
+1. Firstly, check that itβs actually possible to make the shortest path from source to destination equal to the target.
+2. If the shortest path from source to destination without the edges to be modified, is less than the target, then it is not possible.
+3. If the shortest path from source to destination including the edges to be modified and assigning them a temporary weight of `1`, is greater than the target, then it is also not possible.
+4. Suppose we can find a modifiable edge `(u, v)` such that the length of the shortest path from source to `u` `(dis1)` plus the length of the shortest path from `v` to destination (`dis2`) is less than target (`dis1 + dis2 < target`), then we can change its weight to β`target - dis1 - dis2`β.
+5. For all the other edges that still have the weight `β-1β`, change the weights into sufficient large number (`target`, `target + 1` or `200000000` etc.).
+
+
+
+**Solution:**
+
+We can break down the approach as follows:
+
+### Approach:
+
+1. **Initial Check with Existing Weights:**
+ - First, we compute the shortest path from `source` to `destination` using only the edges with positive weights, ignoring the edges with weight `-1`.
+ - If this distance is already greater than `target`, then it's impossible to modify the `-1` edges to achieve the target, so we return an empty array.
+
+2. **Temporary Assignment of Weight 1:**
+ - Next, assign a temporary weight of `1` to all edges with weight `-1` and recompute the shortest path.
+ - If this shortest path is still greater than `target`, then it's impossible to achieve the target, so we return an empty array.
+
+3. **Modify Specific Edge Weights:**
+ - Iterate through the edges with weight `-1` and identify edges that can be adjusted to exactly match the target distance. This is done by adjusting the weight of an edge such that the combined distances of paths leading to and from this edge result in the exact `target` distance.
+ - For any remaining `-1` edges, assign a large enough weight (e.g., `2 * 10^9`) to ensure they don't impact the shortest path.
+
+4. **Return Modified Edges:**
+ - Finally, return the modified list of edges.
+
+
+Let's implement this solution in PHP: **[2699. Modify Graph Edge Weights](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/002699-modify-graph-edge-weights/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+- The dijkstra function calculates the shortest path from the source to all other nodes.
+- We initially compute the shortest path ignoring `-1` edges.
+- If the path without `-1` edges is less than the target, the function returns an empty array, indicating it's impossible to adjust the weights to meet the target.
+- Otherwise, we temporarily set all `-1` edges to `1` and check if the shortest path exceeds the target.
+- If it does, it's again impossible to reach the target, and we return an empty array.
+- We then modify the weights of `-1` edges strategically to achieve the desired shortest path of exactly target.
+
+This approach efficiently checks and adjusts edge weights, ensuring that the target distance is met if possible.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
diff --git a/algorithms/002699-modify-graph-edge-weights/solution.php b/algorithms/002699-modify-graph-edge-weights/solution.php
new file mode 100644
index 000000000..77c464d17
--- /dev/null
+++ b/algorithms/002699-modify-graph-edge-weights/solution.php
@@ -0,0 +1,92 @@
+dijkstra($graph, $source, $destination);
+ if ($distToDestination < $target) return [];
+ if ($distToDestination == $target) {
+ // Change the weights of negative edges to an impossible value
+ foreach ($edges as &$edge) {
+ if ($edge[2] == -1) {
+ $edge[2] = $this->kMax;
+ }
+ }
+ return $edges;
+ }
+
+ // Modify the graph and adjust weights
+ for ($i = 0; $i < count($edges); ++$i) {
+ list($u, $v, $w) = $edges[$i];
+ if ($w != -1) continue;
+ $edges[$i][2] = 1;
+ $graph[$u][] = [$v, 1];
+ $graph[$v][] = [$u, 1];
+ $distToDestination = $this->dijkstra($graph, $source, $destination);
+ if ($distToDestination <= $target) {
+ $edges[$i][2] += $target - $distToDestination;
+ // Change the weights of negative edges to an impossible value
+ for ($j = $i + 1; $j < count($edges); ++$j) {
+ if ($edges[$j][2] == -1) {
+ $edges[$j][2] = $this->kMax;
+ }
+ }
+ return $edges;
+ }
+ }
+
+ return [];
+ }
+
+ /**
+ * @param $graph
+ * @param $src
+ * @param $dst
+ * @return int|mixed
+ */
+ private function dijkstra($graph, $src, $dst) {
+ $dist = array_fill(0, count($graph), PHP_INT_MAX);
+ $minHeap = new SplPriorityQueue();
+ $minHeap->setExtractFlags(SplPriorityQueue::EXTR_BOTH);
+
+ $dist[$src] = 0;
+ $minHeap->insert($src, 0);
+
+ while (!$minHeap->isEmpty()) {
+ $node = $minHeap->extract();
+ $u = $node['data'];
+ $d = -$node['priority'];
+
+ if ($d > $dist[$u]) continue;
+
+ foreach ($graph[$u] as list($v, $w)) {
+ if ($d + $w < $dist[$v]) {
+ $dist[$v] = $d + $w;
+ $minHeap->insert($v, -$dist[$v]);
+ }
+ }
+ }
+
+ return $dist[$dst];
+ }
+}
diff --git a/algorithms/002707-extra-characters-in-a-string/README.md b/algorithms/002707-extra-characters-in-a-string/README.md
new file mode 100644
index 000000000..23ef741ca
--- /dev/null
+++ b/algorithms/002707-extra-characters-in-a-string/README.md
@@ -0,0 +1,110 @@
+2707\. Extra Characters in a String
+
+**Difficulty:** Medium
+
+**Topics:** `Array`, `Hash Table`, `String`, `Dynamic Programming`, `Trie`
+
+You are given a **0-indexed** string `s` and a dictionary of words `dictionary`. You have to break `s` into one or more **non-overlapping** substrings such that each substring is present in `dictionary`. There may be some **extra characters** in `s` which are not present in any of the substrings.
+
+Return _the **minimum** number of extra characters left over if you break up `s` optimally_.
+
+**Example 1:**
+
+- **Input:** s = "leetscode", dictionary = ["leet","code","leetcode"]
+- **Output:** 1
+- **Explanation:** We can break s in two substrings: "leet" from index 0 to 3 and "code" from index 5 to 8. There is only 1 unused character (at index 4), so we return 1.
+
+**Example 2:**
+
+- **Input:** s = "sayhelloworld", dictionary = ["hello","world"]
+- **Output:** 3
+- **Explanation:** We can break s in two substrings: "hello" from index 3 to 7 and "world" from index 8 to 12. The characters at indices 0, 1, 2 are not used in any substring and thus are considered as extra characters. Hence, we return 3.
+
+
+
+**Constraints:**
+
+- `1 <= s.length <= 50`
+- `1 <= dictionary.length <= 50`
+- `1 <= dictionary[i].length <= 50`
+- `dictionary[i]` and `s` consists of only lowercase English letters
+- `dictionary` contains distinct words
+
+
+**Hint:**
+1. Can we use Dynamic Programming here?
+2. Define DP[i] as the min extra character if breaking up s[0:i] optimally.
+
+
+
+**Solution:**
+
+We can define a `dp` array where `dp[i]` represents the minimum number of extra characters in the substring `s[0:i]` after optimal segmentation.
+
+### Approach:
+1. **Dynamic Programming Definition:**
+ - Let `dp[i]` be the minimum number of extra characters in the substring `s[0:i]`.
+ - To calculate `dp[i]`, we can:
+ - Either consider the character `s[i-1]` as an extra character and move to the next index.
+ - Or check if some substring ending at index `i` exists in the dictionary, and if it does, then use it to reduce extra characters.
+
+2. **Transition:**
+ - For each index `i`, we either:
+ - Add one to `dp[i-1]` if we treat `s[i]` as an extra character.
+ - Check every possible substring `s[j:i]` (for `j < i`) and if `s[j:i]` is in the dictionary, we update `dp[i]` based on `dp[j]`.
+
+3. **Result:**
+ - The value of `dp[len(s)]` will give us the minimum number of extra characters in the entire string `s`.
+
+Let's implement this solution in PHP: **[2707. Extra Characters in a String](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/002707-extra-characters-in-a-string/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+1. **Base Case:**
+ - `dp[0] = 0` since no extra characters exist in an empty string.
+
+2. **Dictionary Lookup:**
+ - We store the dictionary words in a hash map using `array_flip()` for constant-time lookup.
+
+3. **Transition:**
+ - For each position `i`, we check all possible substrings `s[j:i]`. If a substring exists in the dictionary, we update the `dp[i]` value.
+
+4. **Time Complexity:**
+ - The time complexity is `O(n^2)` where `n` is the length of the string `s` because for each index, we check all previous indices to form substrings.
+
+### Test Results:
+For the input `"leetscode"` with dictionary `["leet","code","leetcode"]`, the function correctly returns `1`, as only 1 extra character (`"s"`) remains.
+
+For the input `"sayhelloworld"` with dictionary `["hello","world"]`, the function returns `3`, as the first three characters (`"say"`) are extra.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
diff --git a/algorithms/002707-extra-characters-in-a-string/solution.php b/algorithms/002707-extra-characters-in-a-string/solution.php
new file mode 100644
index 000000000..ec38ce5a3
--- /dev/null
+++ b/algorithms/002707-extra-characters-in-a-string/solution.php
@@ -0,0 +1,36 @@
+i <= i1, i2 <= j, 0 <= |nums[i1] - nums[i2]| <= 2
.
+
+Return _the total number of **continuous** subarrays_.
+
+A subarray is a contiguous **non-empty** sequence of elements within an array.
+
+**Example 1:**
+
+- **Input:** nums = [5,4,2,4]
+- **Output:** 8
+- **Explanation:**
+ Continuous subarray of size 1: [5], [4], [2], [4].
+ Continuous subarray of size 2: [5,4], [4,2], [2,4].
+ Continuous subarray of size 3: [4,2,4].
+ Thereare no subarrys of size 4.
+ Total continuous subarrays = 4 + 3 + 1 = 8.
+ It can be shown that there are no more continuous subarrays.
+
+**Example 2:**
+
+- **Input:** nums = [1,2,3]
+- **Output:** 6
+- **Explanation:**
+ Continuous subarray of size 1: [1], [2], [3].
+ Continuous subarray of size 2: [1,2], [2,3].
+ Continuous subarray of size 3: [1,2,3].
+ Total continuous subarrays = 3 + 2 + 1 = 6.
+
+
+
+**Constraints:**
+
+- 1 <= nums.length <= 105
+- 1 <= nums[i] <= 109
+
+
+**Hint:**
+1. Try using the sliding window technique.
+2. Use a set or map to keep track of the maximum and minimum of subarrays.
+
+
+
+**Solution:**
+
+We can use the **sliding window technique** to efficiently calculate the number of continuous subarrays. We'll maintain a valid window where the difference between the maximum and minimum values in the subarray is at most 2. To efficiently track the maximum and minimum values within the current window, we can use two **deques** (one for the maximum and one for the minimum).
+
+### Approach
+1. Use the sliding window technique with two pointers: `left` and `right`.
+2. Use two **deques**:
+ - One to track indices of elements in descending order for the maximum value.
+ - One to track indices of elements in ascending order for the minimum value.
+3. For each index `right`:
+ - Update the deques to reflect the current window.
+ - Ensure the window remains valid (difference between maximum and minimum β€ 2). If invalid, increment the `left` pointer to shrink the window.
+ - Count the number of valid subarrays ending at index `right` as `(right - left + 1)`.
+4. Return the total count of subarrays.
+
+Let's implement this solution in PHP: **[2762. Continuous Subarrays](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/002762-continuous-subarrays/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+1. **Sliding Window:**
+ The `left` pointer moves forward only when the window becomes invalid (i.e., the difference between the maximum and minimum values exceeds 2). The `right` pointer expands the window by iterating through the array.
+
+2. **Deques for Maximum and Minimum:**
+ - The `maxDeque` always holds indices of elements in descending order, ensuring the maximum value in the current window is at the front (`maxDeque[0]`).
+ - The `minDeque` always holds indices of elements in ascending order, ensuring the minimum value in the current window is at the front (`minDeque[0]`).
+
+3. **Counting Subarrays:**
+ For each `right`, the number of valid subarrays ending at `right` is equal to `(right - left + 1)`, as all subarrays from `left` to `right` are valid.
+
+4. **Efficiency:**
+ Each element is added to and removed from the deques at most once, making the time complexity **O(n)**. Space complexity is **O(n)** due to the deques.
+
+---
+
+### Output
+
+```text
+8
+6
+```
+
+---
+
+### Complexity Analysis
+
+1. **Time Complexity:**
+ - Each element is pushed and popped from the deques at most once.
+ - Total time complexity is **O(n)**.
+
+2. **Space Complexity:**
+ - Deques store indices, with a maximum size of `n`.
+ - Space complexity is **O(n)**.
+
+This implementation is efficient and works within the constraints provided.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
diff --git a/algorithms/002762-continuous-subarrays/solution.php b/algorithms/002762-continuous-subarrays/solution.php
new file mode 100644
index 000000000..4f23a5adc
--- /dev/null
+++ b/algorithms/002762-continuous-subarrays/solution.php
@@ -0,0 +1,44 @@
+= $nums[$right]) {
+ array_pop($minDeque);
+ }
+ $minDeque[] = $right;
+
+ // Shrink the window if the condition is violated
+ while ($nums[$maxDeque[0]] - $nums[$minDeque[0]] > 2) {
+ $left++;
+ // Remove indices that are outside the current window
+ if ($maxDeque[0] < $left) array_shift($maxDeque);
+ if ($minDeque[0] < $left) array_shift($minDeque);
+ }
+
+ // Add the number of valid subarrays ending at `right`
+ $count += $right - $left + 1;
+ }
+
+ return $count;
+ }
+}
\ No newline at end of file
diff --git a/algorithms/002779-maximum-beauty-of-an-array-after-applying-operation/README.md b/algorithms/002779-maximum-beauty-of-an-array-after-applying-operation/README.md
new file mode 100644
index 000000000..500ccf419
--- /dev/null
+++ b/algorithms/002779-maximum-beauty-of-an-array-after-applying-operation/README.md
@@ -0,0 +1,134 @@
+2779\. Maximum Beauty of an Array After Applying Operation
+
+**Difficulty:** Medium
+
+**Topics:** `Array`, `Binary Search`, `Sliding Window`, `Sorting`
+
+You are given a **0-indexed** array `nums` and a **non-negative** integer `k`.
+
+In one operation, you can do the following:
+
+- Choose an index `i` that **hasn't been chosen before** from the range `[0, nums.length - 1]`.
+- Replace `nums[i]` with any integer from the range `[nums[i] - k, nums[i] + k]`.
+
+The **beauty** of the array is the length of the longest subsequence consisting of equal elements.
+
+Return _the **maximum** possible beauty of the array `nums` after applying the operation any number of times_.
+
+**Note** that you can apply the operation to each index only once.
+
+A **subsequence** of an array is a new array generated from the original array by deleting some elements (possibly none) without changing the order of the remaining elements.
+
+**Example 1:**
+
+- **Input:** nums = [4,6,1,2], k = 2
+- **Output:** 3
+- **Explanation:** In this example, we apply the following operations:
+ - Choose index 1, replace it with 4 (from range [4,8]), nums = [4,4,1,2].
+ - Choose index 3, replace it with 4 (from range [0,4]), nums = [4,4,1,4].
+ After the applied operations, the beauty of the array nums is 3 (subsequence consisting of indices 0, 1, and 3).
+ It can be proven that 3 is the maximum possible length we can achieve.
+
+**Example 2:**
+
+- **Input:** nums = [1,1,1,1], k = 10
+- **Output:** 4
+- **Explanation:** In this example we don't have to apply any operations.
+ The beauty of the array nums is 4 (whole array).
+
+
+**Constraints:**
+
+- 1 <= nums.length <= 105
+- 0 <= nums[i], k <= 105
+
+
+**Hint:**
+1. Sort the array.
+2. The problem becomes the following: find maximum subarray A[i β¦ j] such that A[j] - A[i] β€ 2 * k.
+
+
+
+**Solution:**
+
+We can utilize sorting and a sliding window approach.
+
+### Approach:
+1. **Sort the array**: Sorting simplifies identifying subsequences where the difference between the largest and smallest element does not exceed _**2k**_.
+2. **Sliding window technique**: Maintain a window of indices _**[i, j]**_ where the difference _**nums[j] - nums[i] <= 2k**_. Adjust _**i**_ or _**j**_ to maximize the window size.
+
+Let's implement this solution in PHP: **[2779. Maximum Beauty of an Array After Applying Operation](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/002779-maximum-beauty-of-an-array-after-applying-operation/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+1. **Sorting the Array**:
+ - Sorting ensures that the window defined by indices _**[i, j]**_ has all elements in increasing order, which makes it easier to check the difference between the smallest and largest values in the window.
+2. **Sliding Window**:
+ - Start with both `i` and `j` at the beginning.
+ - Expand the window by incrementing `j` and keep the window valid by incrementing `i` whenever the condition _**nums[j] - nums[i] > 2k**_ is violated.
+ - At each step, calculate the size of the current valid window _**j - i + 1**_ and update the `maxBeauty`.
+
+---
+
+### Complexity Analysis:
+1. **Time Complexity**:
+ - Sorting the array: _**O(n log n)**_.
+ - Sliding window traversal: _**O(n)**_.
+ - Overall: _**O(n log n)**_.
+2. **Space Complexity**:
+ - _**O(1)**_, as the solution uses only a few additional variables.
+
+---
+
+### Examples:
+#### Input 1:
+```php
+$nums = [4, 6, 1, 2];
+$k = 2;
+echo maximumBeauty($nums, $k); // Output: 3
+```
+
+#### Input 2:
+```php
+$nums = [1, 1, 1, 1];
+$k = 10;
+echo maximumBeauty($nums, $k); // Output: 4
+```
+
+This solution adheres to the constraints and efficiently computes the result for large inputs.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
diff --git a/algorithms/002779-maximum-beauty-of-an-array-after-applying-operation/solution.php b/algorithms/002779-maximum-beauty-of-an-array-after-applying-operation/solution.php
new file mode 100644
index 000000000..678d8bff5
--- /dev/null
+++ b/algorithms/002779-maximum-beauty-of-an-array-after-applying-operation/solution.php
@@ -0,0 +1,29 @@
+ 2 * $k) {
+ $i++; // Shrink the window from the left
+ }
+ // Update the maximum beauty (window size)
+ $maxBeauty = max($maxBeauty, $j - $i + 1);
+ }
+
+ return $maxBeauty;
+ }
+}
\ No newline at end of file
diff --git a/algorithms/002807-insert-greatest-common-divisors-in-linked-list/README.md b/algorithms/002807-insert-greatest-common-divisors-in-linked-list/README.md
new file mode 100644
index 000000000..9b5546953
--- /dev/null
+++ b/algorithms/002807-insert-greatest-common-divisors-in-linked-list/README.md
@@ -0,0 +1,176 @@
+2807\. Insert Greatest Common Divisors in Linked List
+
+**Difficulty:** Medium
+
+**Topics:** `Linked List`, `Math`, `Number Theory`
+
+Given the head of a linked list `head`, in which each node contains an integer value.
+
+Between every pair of adjacent nodes, insert a new node with a value equal to the **greatest common divisor** of them.
+
+Return _the linked list after insertion_.
+
+The **greatest common divisor** of two numbers is the largest positive integer that evenly divides both numbers.
+
+**Example 1:**
+
+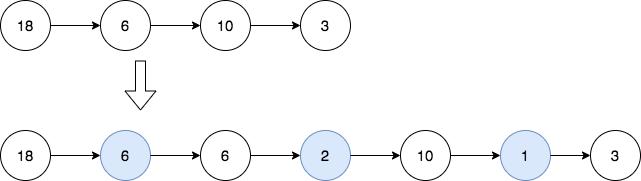
+
+- **Input:** head = [18,6,10,3]
+- **Output:** [18,6,6,2,10,1,3]
+- **Explanation:** The 1st diagram denotes the initial linked list and the 2nd diagram denotes the linked list after inserting the new nodes (nodes in blue are the inserted nodes).
+ - We insert the greatest common divisor of 18 and 6 = 6 between the 1st and the 2nd nodes.
+ - We insert the greatest common divisor of 6 and 10 = 2 between the 2nd and the 3rd nodes.
+ - We insert the greatest common divisor of 10 and 3 = 1 between the 3rd and the 4th nodes.\
+ There are no more adjacent nodes, so we return the linked list.
+
+**Example 2:**
+
+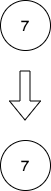
+
+- **Input:** head = [7]
+- **Output:** [7]
+- **Explanation:** The 1st diagram denotes the initial linked list and the 2nd diagram denotes the linked list after inserting the new nodes.\
+ There are no pairs of adjacent nodes, so we return the initial linked list.
+
+
+**Example 3:**
+
+- **Input:** cost = [[2, 5, 1], [3, 4, 7], [8, 1, 2], [6, 2, 4], [3, 8, 8]]
+- **Output:** 10
+
+
+
+**Constraints:**
+
+- The number of nodes in the list is in the range `[1, 5000]`.
+- `1 <= Node.val <= 1000`
+
+
+**Hint:**
+1. Each point on the left would either be connected to exactly point already connected to some left node, or a subset of the nodes on the right which are not connected to any node
+2. Use dynamic programming with bitmasking, where the state will be (number of points assigned in first group, bitmask of points assigned in second group).
+
+
+
+**Solution:**
+
+We need to insert nodes between every pair of adjacent nodes in a linked list. The value of the inserted node should be the greatest common divisor (GCD) of the two adjacent nodes' values. We'll traverse the linked list, calculate the GCD for every pair of adjacent nodes, and then insert the new node accordingly.
+
+Here's how you can approach this:
+
+1. **Define a ListNode Class**: This class will represent each node in the linked list.
+2. **Traverse the List**: We'll iterate through the list to find each pair of adjacent nodes.
+3. **Insert GCD Nodes**: For each pair of adjacent nodes, we'll insert a new node whose value is the GCD of the two adjacent nodes.
+4. **Return the modified list**.
+
+Let's implement this solution in PHP: **[2807. Insert Greatest Common Divisors in Linked List](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/002807-insert-greatest-common-divisors-in-linked-list/solution.php)**
+
+```php
+val = $val;
+ $this->next = $next;
+ }
+}
+
+/**
+ * Function to calculate the GCD of two numbers.
+ *
+ * @param $a
+ * @param $b
+ * @return mixed
+ */
+function gcd($a, $b) {
+ ...
+ ...
+ ...
+ /**
+ * go to ./solution.php
+ */
+
+}
+
+/**
+ * @param ListNode $head
+ * @return ListNode
+ */
+function insertGreatestCommonDivisors($head) {
+ ...
+ ...
+ ...
+ /**
+ * go to ./solution.php
+ */
+}
+
+/**
+ * Function to print the linked list for testing purposes.
+ *
+ * @param $head
+ * @return void
+ */
+function printList($head) {
+ $current = $head;
+ while ($current != null) {
+ echo $current->val . " ";
+ $current = $current->next;
+ }
+ echo "\n";
+}
+
+// Example usage:
+
+// Create the linked list: 18 -> 6 -> 10 -> 3
+$head = new ListNode(18);
+$head->next = new ListNode(6);
+$head->next->next = new ListNode(10);
+$head->next->next->next = new ListNode(3);
+
+// Insert GCD nodes.
+$modifiedHead = insertGreatestCommonDivisors($head);
+
+// Print the modified linked list.
+printList($modifiedHead);
+
+// Output should be: 18 -> 6 -> 6 -> 2 -> 10 -> 1 -> 3
+?>
+```
+
+### Explanation:
+
+1. **ListNode Class**: This class represents the structure of a node in the linked list, with properties for the value (`$val`) and the next node (`$next`).
+
+2. **GCD Calculation**: The `gcd` function uses the Euclidean algorithm to compute the greatest common divisor of two integers.
+
+3. **Main Logic (`insertGreatestCommonDivisors`)**:
+ - We loop through the linked list until we reach the second-to-last node.
+ - For each pair of adjacent nodes, we compute the GCD of their values.
+ - We create a new node with this GCD value and insert it between the current node and the next node.
+
+4. **Edge Case**: If the list has only one node, we return it as is without making any changes since there are no adjacent nodes.
+
+5. **Testing**: The function `printList` is a helper function used to output the values of the linked list for verification.
+
+### Time Complexity:
+- The time complexity of this solution is \(O(n)\), where \(n\) is the number of nodes in the linked list. Calculating the GCD for each adjacent pair takes constant time on average, and we perform a linear traversal of the list.
+
+### Example:
+For the input list `[18, 6, 10, 3]`, the output is:
+```
+18 -> 6 -> 6 -> 2 -> 10 -> 1 -> 3
+```
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
diff --git a/algorithms/002807-insert-greatest-common-divisors-in-linked-list/solution.php b/algorithms/002807-insert-greatest-common-divisors-in-linked-list/solution.php
new file mode 100644
index 000000000..c3d1e2cc5
--- /dev/null
+++ b/algorithms/002807-insert-greatest-common-divisors-in-linked-list/solution.php
@@ -0,0 +1,63 @@
+val = $val;
+ * $this->next = $next;
+ * }
+ * }
+ */
+class Solution {
+
+ /**
+ * @param ListNode $head
+ * @return ListNode
+ */
+ function insertGreatestCommonDivisors($head) {
+ // Edge case: If the list has only one node, return the list as is.
+ if ($head == null || $head->next == null) {
+ return $head;
+ }
+
+ // Start traversing the linked list from the head node.
+ $current = $head;
+
+ while ($current != null && $current->next != null) {
+ // Calculate GCD of current node's value and the next node's value.
+ $gcdValue = $this->gcd($current->val, $current->next->val);
+
+ // Create a new node with the GCD value.
+ $gcdNode = new ListNode($gcdValue);
+
+ // Insert the GCD node between current and next node.
+ $gcdNode->next = $current->next;
+ $current->next = $gcdNode;
+
+ // Move to the node after the newly inserted GCD node.
+ $current = $gcdNode->next;
+ }
+
+ // Return the modified head of the linked list.
+ return $head;
+
+ }
+
+ /**
+ * Function to calculate the GCD of two numbers.
+ *
+ * @param $a
+ * @param $b
+ * @return mixed
+ */
+ function gcd($a, $b) {
+ if ($b == 0) {
+ return $a;
+ }
+ return self::gcd($b, $a % $b);
+ }
+
+}
\ No newline at end of file
diff --git a/algorithms/002825-make-string-a-subsequence-using-cyclic-increments/README.md b/algorithms/002825-make-string-a-subsequence-using-cyclic-increments/README.md
new file mode 100644
index 000000000..9252aa7ea
--- /dev/null
+++ b/algorithms/002825-make-string-a-subsequence-using-cyclic-increments/README.md
@@ -0,0 +1,123 @@
+2825\. Make String a Subsequence Using Cyclic Increments
+
+**Difficulty:** Medium
+
+**Topics:** `Two Pointers`, `String`
+
+You are given two **0-indexed** strings `str1` and `str2`.
+
+In an operation, you select a **set** of indices in `str1`, and for each index `i` in the set, increment `str1[i]` to the next character **cyclically**. That is `'a'` becomes `'b'`, `'b'` becomes `'c'`, and so on, and `'z'` becomes `'a'`.
+
+Return _`true` if it is possible to make `str2` a subsequence of `str1` by performing the operation **at most once**, and `false` otherwise_.
+
+**Note:** A subsequence of a string is a new string that is formed from the original string by deleting some (possibly none) of the characters without disturbing the relative positions of the remaining characters.
+
+**Example 1:**
+
+- **Input:** str1 = "abc", str2 = "ad"
+- **Output:** true
+- **Explanation:** Select index 2 in str1.
+ Increment str1[2] to become 'd'.
+ Hence, str1 becomes "abd" and str2 is now a subsequence. Therefore, true is returned.
+
+**Example 2:**
+
+- **Input:** str1 = "zc", str2 = "ad"
+- **Output:** true
+- **Explanation:** Select indices 0 and 1 in str1.
+ Increment str1[0] to become 'a'.
+ Increment str1[1] to become 'd'.
+ Hence, str1 becomes "ad" and str2 is now a subsequence. Therefore, true is returned.
+
+
+**Example 3:**
+
+- **Input:** str1 = "ab", str2 = "d"
+- **Output:** false
+- **Explanation:** In this example, it can be shown that it is impossible to make str2 a subsequence of str1 using the operation at most once.
+ Therefore, false is returned.
+
+
+
+**Constraints:**
+
+- 1 <= str1.length <= 105
+- 1 <= str2.length <= 105
+- `str1` and `str2` consist of only lowercase English letters.
+
+
+**Hint:**
+1. Consider the indices we will increment separately.
+2. We can maintain two pointers: pointer `i` for `str1` and pointer `j` for `str2`, while ensuring they remain within the bounds of the strings.
+3. If both `str1[i]` and `str2[j]` match, or if incrementing `str1[i]` matches `str2[j]`, we increase both pointers; otherwise, we increment only pointer `i`.
+4. It is possible to make `str2` a subsequence of `str1` if `j` is at the end of `str2`, after we can no longer find a match.
+
+
+
+**Solution:**
+
+We need to check if we can make `str2` a subsequence of `str1` by performing at most one cyclic increment operation on any characters in `str1`:
+
+### Explanation:
+- We will use two pointers, `i` for `str1` and `j` for `str2`.
+- If the character at `str1[i]` matches `str2[j]`, we move both pointers forward.
+- If `str1[i]` can be incremented to match `str2[j]` (cyclically), we try to match them and then move both pointers.
+- If neither of the above conditions holds, we only move the pointer `i` for `str1`.
+- Finally, if we can match all characters of `str2`, then it is possible to make `str2` a subsequence of `str1`, otherwise not.
+
+Let's implement this solution in PHP: **[2825. Make String a Subsequence Using Cyclic Increments](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/002825-make-string-a-subsequence-using-cyclic-increments/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+1. **Two Pointers**: `i` and `j` are initialized to the start of `str1` and `str2`, respectively.
+2. **Matching Logic**: Inside the loop, we check if the characters at `str1[i]` and `str2[j]` are the same or if we can increment `str1[i]` to match `str2[j]` cyclically.
+ - The cyclic increment condition is handled using `(ord($str1[$i]) + 1 - ord('a')) % 26` which checks if `str1[i]` can be incremented to match `str2[j]`.
+3. **Subsequence Check**: If we have iterated through `str2` completely (i.e., `j == m`), it means `str2` is a subsequence of `str1`. Otherwise, it's not.
+
+### Time Complexity:
+- The algorithm iterates through `str1` once, and each character in `str2` is checked only once, so the time complexity is **O(n)**, where `n` is the length of `str1`.
+
+### Space Complexity:
+- The space complexity is **O(1)** since we only use a few pointers and do not need extra space dependent on the input size.
+
+This solution efficiently checks if it's possible to make `str2` a subsequence of `str1` with at most one cyclic increment operation.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
diff --git a/algorithms/002825-make-string-a-subsequence-using-cyclic-increments/solution.php b/algorithms/002825-make-string-a-subsequence-using-cyclic-increments/solution.php
new file mode 100644
index 000000000..ac4df8e06
--- /dev/null
+++ b/algorithms/002825-make-string-a-subsequence-using-cyclic-increments/solution.php
@@ -0,0 +1,32 @@
+edges[i] = [ai, bi] indicates that there is an edge between nodes ai
and bi
in the tree.
+
+You are also given a **0-indexed** integer array `values` of length `n`, where `values[i]` is the value associated with the ith
node, and an integer `k`.
+
+A **valid split** of the tree is obtained by removing any set of edges, possibly empty, from the tree such that the resulting components all have values that are divisible by `k`, where the **value of a connected component** is the sum of the values of its nodes.
+
+Return _the **maximum number of components** in any valid split_.
+
+**Example 1:**
+
+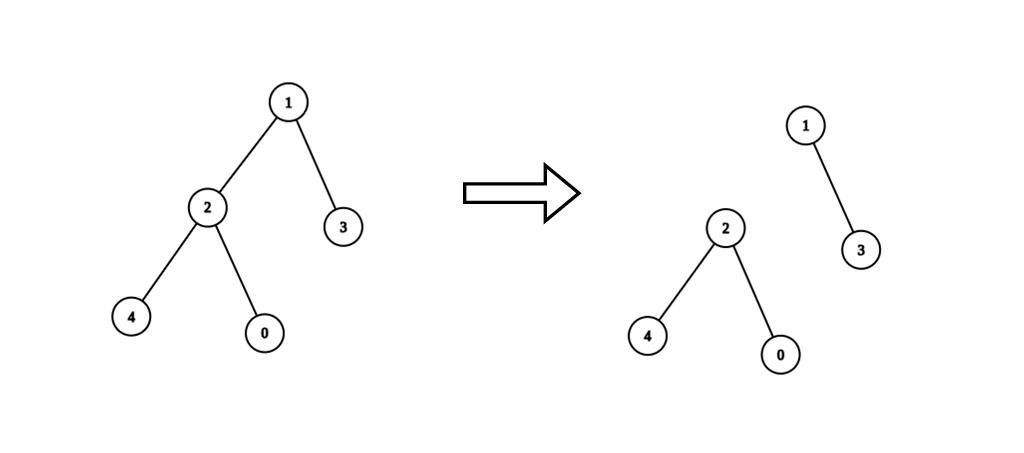
+
+- **Input:** n = 5, edges = [[0,2],[1,2],[1,3],[2,4]], values = [1,8,1,4,4], k = 6
+- **Output:** 2
+- **Explanation:** We remove the edge connecting node 1 with 2. The resulting split is valid because:
+ - The value of the component containing nodes 1 and 3 is values[1] + values[3] = 12.
+ - The value of the component containing nodes 0, 2, and 4 is values[0] + values[2] + values[4] = 6.
+ It can be shown that no other valid split has more than 2 connected components.
+
+**Example 2:**
+
+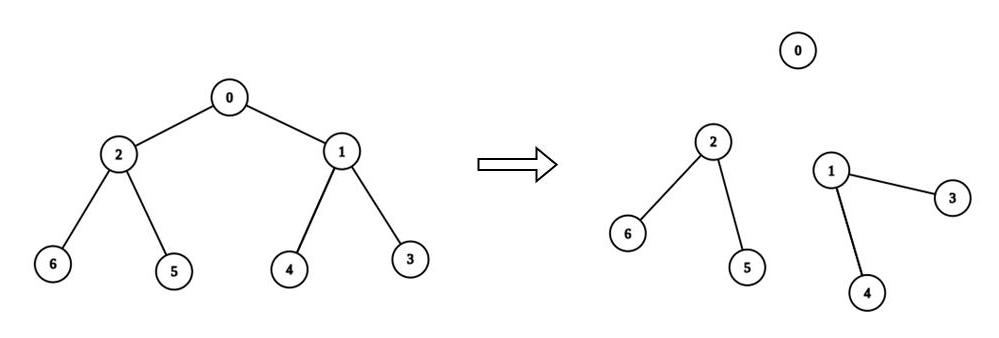
+
+- **Input:** n = 7, edges = [[0,1],[0,2],[1,3],[1,4],[2,5],[2,6]], values = [3,0,6,1,5,2,1], k = 3
+- **Output:** 3
+- **Explanation:** We remove the edge connecting node 0 with 2, and the edge connecting node 0 with 1. The resulting split is valid because:
+ - The value of the component containing node 0 is values[0] = 3.
+ - The value of the component containing nodes 2, 5, and 6 is values[2] + values[5] + values[6] = 9.
+ - The value of the component containing nodes 1, 3, and 4 is values[1] + values[3] + values[4] = 6.
+ It can be shown that no other valid split has more than 3 connected components.
+
+
+
+**Constraints:**
+
+- 1 <= n <= 3 * 104
+- `edges.length == n - 1`
+- `edges[i].length == 2`
+- `0 <= ai, bi < n`
+- `values.length == n`
+- 0 <= values[i] <= 109
+- 1 <= k <= 109
+- Sum of `values` is divisible by `k`.
+- The input is generated such that `edges` represents a valid tree.
+
+
+**Hint:**
+1. Root the tree at node `0`.
+2. If a leaf node is not divisible by `k`, it must be in the same component as its parent node so we merge it with its parent node.
+3. If a leaf node is divisible by `k`, it will be in its own components so we separate it from its parent node.
+4. In each step, we either cut a leaf node down or merge a leaf node. The number of nodes on the tree reduces by one. Repeat this process until only one node is left.
+
+
+
+**Solution:**
+
+We can implement a Depth-First Search (DFS) approach to explore the tree, track the values of components, and find the maximum number of valid splits.
+
+### Key Points:
+
+- **Tree Structure:** We are working with an undirected tree where each node has an associated value. We need to find the maximum number of connected components we can get by splitting the tree such that the sum of values of each component is divisible by `k`.
+- **DFS Traversal:** We use Depth-First Search (DFS) to traverse the tree and calculate the subtree sums.
+- **Divisibility Check:** After calculating the sum of a subtree, if it's divisible by `k`, it means the subtree can be considered as a valid component by itself.
+- **Edge Removal:** By removing edges that connect nodes whose subtree sums aren't divisible by `k`, we can maximize the number of valid components.
+
+### Approach:
+
+1. **Tree Representation:** Convert the `edges` list into an adjacency list to represent the tree.
+2. **DFS Traversal:** Start from node 0 and recursively calculate the sum of values in each subtree. If the sum is divisible by `k`, we can cut that subtree off from its parent, effectively forming a valid component.
+3. **Global Count:** Maintain a global counter (`result`) that tracks the number of valid components formed by cutting edges.
+4. **Final Check:** At the end of the DFS traversal, ensure that if the root's total subtree sum is divisible by `k`, it counts as a valid component.
+
+### Plan:
+
+1. **Input Parsing:** Convert the input into a usable form. Specifically, create an adjacency list representation for the tree.
+2. **DFS Function:** Write a recursive function `dfs(node)` that computes the sum of values in the subtree rooted at `node`. If this sum is divisible by `k`, increment the `result` counter and "cut" the edge by returning 0 to prevent merging back into the parent.
+3. **Start DFS from Root:** Call `dfs(0)` and then check the final value of `result`.
+
+### Solution Steps:
+
+1. **Build the Tree:** Convert the edge list into an adjacency list for easier traversal.
+2. **Initialize Visited Array:** Use a `visited` array to ensure we don't revisit nodes.
+3. **DFS Traversal:** Perform DFS starting from node `0`. For each node, calculate the sum of values of its subtree.
+4. **Check Divisibility:** If the sum of a subtree is divisible by `k`, increment the `result` and reset the subtree sum to 0.
+5. **Final Component Check:** After the DFS traversal, check if the entire tree (rooted at node 0) has a sum divisible by `k` and account for it as a separate component if necessary.
+
+Let's implement this solution in PHP: **[2872. Maximum Number of K-Divisible Components](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/002872-maximum-number-of-k-divisible-components/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+1. **Tree Construction:** We build an adjacency list to represent the tree structure. Each edge connects two nodes, and we use this adjacency list to traverse the tree.
+2. **DFS Traversal:** The DFS function recursively calculates the sum of the subtree rooted at each node. If the sum of the subtree is divisible by `k`, we increment the `result` and consider the subtree as a separate valid component.
+3. **Returning Subtree Sum:** For each node, the DFS function returns the sum of values for its subtree. If a subtree is divisible by `k`, we effectively "cut" the edge by returning a sum of 0, preventing further merging back with the parent node.
+4. **Final Check:** At the end of the DFS, we ensure that if the sum of the entire tree is divisible by `k`, it counts as a valid component.
+
+### Example Walkthrough:
+
+**Example 1:**
+
+Input:
+- `n = 5`, `edges = [[0,2],[1,2],[1,3],[2,4]]`, `values = [1,8,1,4,4]`, `k = 6`.
+
+DFS starts from node 0:
+- Node 0: subtree sum = 1
+- Node 2: subtree sum = 1 + 1 + 4 = 6 (divisible by 6, so we can cut this edge)
+- Node 1: subtree sum = 8 + 4 = 12 (divisible by 6, cut this edge)
+- Final count of components = 2.
+
+**Example 2:**
+
+Input:
+- `n = 7`, `edges = [[0,1],[0,2],[1,3],[1,4],[2,5],[2,6]]`, `values = [3,0,6,1,5,2,1]`, `k = 3`.
+
+DFS starts from node 0:
+- Node 0: subtree sum = 3
+- Node 2: subtree sum = 6 + 2 + 1 = 9 (divisible by 3, cut this edge)
+- Node 1: subtree sum = 0 + 5 = 5 (not divisible by 3, merge this sum)
+- Final count of components = 3.
+
+### Time Complexity:
+
+- **DFS Time Complexity:** O(n), where `n` is the number of nodes. We visit each node once and perform constant-time operations at each node.
+- **Space Complexity:** O(n) for the adjacency list, visited array, and recursion stack.
+
+Thus, the overall time and space complexity is O(n), making this approach efficient for the given problem constraints.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
diff --git a/algorithms/002872-maximum-number-of-k-divisible-components/solution.php b/algorithms/002872-maximum-number-of-k-divisible-components/solution.php
new file mode 100644
index 000000000..95c1cba16
--- /dev/null
+++ b/algorithms/002872-maximum-number-of-k-divisible-components/solution.php
@@ -0,0 +1,57 @@
+dfs($graph, 0, -1, $values, $k, $ans);
+ return $ans;
+ }
+
+ /**
+ * DFS Function
+ *
+ * @param $graph
+ * @param $node
+ * @param $parent
+ * @param $values
+ * @param $k
+ * @param $ans
+ * @return array|bool|int|int[]|mixed|null
+ */
+ private function dfs($graph, $node, $parent, &$values, $k, &$ans) {
+ $treeSum = $values[$node];
+
+ foreach ($graph[$node] as $neighbor) {
+ if ($neighbor !== $parent) {
+ $treeSum += $this->dfs($graph, $neighbor, $node, $values, $k, $ans);
+ }
+ }
+
+ // If the subtree sum is divisible by k, it forms a valid component
+ if ($treeSum % $k === 0) {
+ $ans++;
+ return 0; // Reset sum for the current component
+ }
+
+ return $treeSum;
+ }
+}
\ No newline at end of file
diff --git a/algorithms/002914-minimum-number-of-changes-to-make-binary-string-beautiful/README.md b/algorithms/002914-minimum-number-of-changes-to-make-binary-string-beautiful/README.md
new file mode 100644
index 000000000..7b5758599
--- /dev/null
+++ b/algorithms/002914-minimum-number-of-changes-to-make-binary-string-beautiful/README.md
@@ -0,0 +1,118 @@
+2914\. Minimum Number of Changes to Make Binary String Beautiful
+
+**Difficulty:** Medium
+
+**Topics:** `String`
+
+You are given a **0-indexed** binary string `s` having an even length.
+
+A string is **beautiful** if it's possible to partition it into one or more substrings such that:
+
+- Each substring has an **even length**.
+- Each substring contains **only** `1`'s or only `0`'s.
+
+You can change any character in `s` to `0` or `1`.
+
+Return _the **minimum** number of changes required to make the string `s` beautiful_.
+
+**Example 1:**
+
+- **Input:** s = "1001"
+- **Output:** 2
+- **Explanation:** We change s[1] to 1 and s[3] to 0 to get string "1100".
+ - It can be seen that the string "1100" is beautiful because we can partition it into "11|00".
+ - It can be proven that 2 is the minimum number of changes needed to make the string beautiful.
+
+**Example 2:**
+
+- **Input:** s = "10"
+- **Output:** 1
+- **Explanation:** We change s[1] to 1 to get string "11".
+ - It can be seen that the string "11" is beautiful because we can partition it into "11".
+ - It can be proven that 1 is the minimum number of changes needed to make the string beautiful.
+
+
+**Example 3:**
+
+- **Input:** s = "0000"
+- **Output:** 0
+- **Explanation:** We don't need to make any changes as the string "0000" is beautiful already.
+
+
+**Constraints:**
+
+- 2 <= s.length <= 105
+- `s` has an even length.
+- `s[i]` is either `'0'` or `'1'`.
+
+
+**Hint:**
+1. For any valid partition, since each part consists of an even number of the same characters, we can further partition each part into lengths of exactly `2`.
+2. After noticing the first hint, we can decompose the whole string into disjoint blocks of size `2` and find the minimum number of changes required to make those blocks beautiful.
+
+
+
+**Solution:**
+
+We need to ensure that every pair of characters in the binary string `s` is either `"00"` or `"11"`. If a pair is not in one of these two patterns, we will need to change one of the characters to make it match.
+
+Here's the step-by-step solution approach:
+
+1. **Divide the String into Blocks:** Since a beautiful string can be formed from blocks of length 2, we can iterate through the string in steps of 2.
+
+2. **Count Changes:** For each block of 2 characters, we need to determine the majority character (either `0` or `1`). We will change the minority character in the block to match the majority character.
+
+3. **Calculate Minimum Changes:** For each block, if both characters are different, we will need 1 change; if they are the same, no changes are required.
+
+Let's implement this solution in PHP: **[2914. Minimum Number of Changes to Make Binary String Beautiful](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/002914-minimum-number-of-changes-to-make-binary-string-beautiful/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+1. **Function Definition:** We define a function `minChanges` that takes a binary string `s`.
+
+2. **Initialization:** We initialize a variable `$changes` to keep track of the number of changes required.
+
+3. **Iterate Over the String:** We loop through the string, incrementing by 2 each time to check each block of two characters:
+ - `$first` is the character at the current position.
+ - `$second` is the character at the next position.
+
+4. **Check for Changes:** If the characters in the current block are different, we increment the `$changes` counter by 1.
+
+5. **Return Result:** Finally, we return the total number of changes required.
+
+### Complexity:
+- **Time Complexity**: _**O(n)**_, where _**n**_ is the length of the string, as we are iterating over the string once.
+- **Space Complexity**: _**O(1)**_, since we are using a constant amount of extra space.
+
+This solution operates in _**O(n)**_ time complexity, where n is the length of the string, making it efficient for the given constraints.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
diff --git a/algorithms/002914-minimum-number-of-changes-to-make-binary-string-beautiful/solution.php b/algorithms/002914-minimum-number-of-changes-to-make-binary-string-beautiful/solution.php
new file mode 100644
index 000000000..19a409ae1
--- /dev/null
+++ b/algorithms/002914-minimum-number-of-changes-to-make-binary-string-beautiful/solution.php
@@ -0,0 +1,27 @@
+edges[i] = [ui, vi] indicates that there is a directed edge from team ui
to team vi
in the graph.
+
+A directed edge from `a` to `b` in the graph means that team `a` is **stronger** than team `b` and team `b` is **weaker** than team `a`.
+
+Team `a` will be the **champion** of the tournament if there is no team `b` that is **stronger** than team `a`.
+
+Return _the team that will be the **champion** of the tournament if there is a **unique** champion, otherwise, return `-1`_.
+
+**Notes**
+
+- A **cycle** is a series of nodes a1, a2, ..., an, an+1
such that node a1
is the same node as node an+1
, the nodes a1, a2, ..., an
are distinct, and there is a directed edge from the node ai
to node ai+1
for every `i` in the range `[1, n]`.
+- A **DAG** is a directed graph that does not have any **cycle**.
+
+
+**Example 1:**
+
+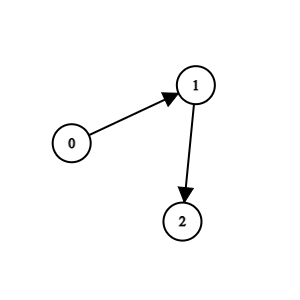
+
+- **Input:** n = 3, edges = [[0,1],[1,2]]
+- **Output:** 0
+- **Explanation:** Team 1 is weaker than team 0. Team 2 is weaker than team 1. So the champion is team 0.
+
+**Example 2:**
+
+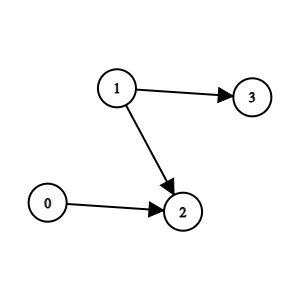
+
+- **Input:** n = 4, edges = [[0,2],[1,3],[1,2]]
+- **Output:** -1
+- **Explanation:** Team 2 is weaker than team 0 and team 1. Team 3 is weaker than team 1. But team 1 and team 0 are not weaker than any other teams. So the answer is -1.
+
+
+**Constraints:**
+
+- `1 <= n <= 100`
+- `m == edges.length`
+- `0 <= m <= n * (n - 1) / 2`
+- `edges[i].length == 2`
+- `0 <= edge[i][j] <= n - 1`
+- `edges[i][0] != edges[i][1]`
+- The input is generated such that if team `a` is stronger than team `b`, team `b` is not stronger than team `a`.
+- The input is generated such that if team `a` is stronger than team `b` and team `b` is stronger than team `c`, then team `a` is stronger than team `c`.
+
+
+**Hint:**
+1. The champion(s) should have in-degree `0` in the DAG.
+
+
+
+**Solution:**
+
+We need to identify the team(s) with an **in-degree** of `0` in the given Directed Acyclic Graph (DAG). Teams with no incoming edges represent teams that no other team is stronger than, making them candidates for being the champion. If there is exactly one team with an in-degree of `0`, it is the unique champion. If there are multiple or no such teams, the result is `-1`.
+
+Let's implement this solution in PHP: **[2924. Find Champion II](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/002924-find-champion-ii/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+
+1. **Input Parsing**:
+ - `n` is the number of teams.
+ - `edges` is the list of directed edges in the graph.
+
+2. **Initialize In-degree**:
+ - Create an array `inDegree` of size `n` initialized to `0`.
+
+3. **Calculate In-degree**:
+ - For each edge `[u, v]`, increment the in-degree of `v` (team `v` has one more incoming edge).
+
+4. **Find Candidates**:
+ - Iterate through the `inDegree` array and collect indices where the in-degree is `0`. These indices represent teams with no other stronger teams.
+
+5. **Determine Champion**:
+ - If exactly one team has in-degree `0`, it is the unique champion.
+ - If multiple teams or no teams have in-degree `0`, return `-1`.
+
+### Example Walkthrough
+
+#### Example 1:
+- Input: `n = 3`, `edges = [[0, 1], [1, 2]]`
+- In-degree: `[0, 1, 1]`
+- Teams with in-degree `0`: `[0]`
+- Output: `0` (Team `0` is the unique champion).
+
+#### Example 2:
+- Input: `n = 4`, `edges = [[0, 2], [1, 3], [1, 2]]`
+- In-degree: `[0, 0, 2, 1]`
+- Teams with in-degree `0`: `[0, 1]`
+- Output: `-1` (There are multiple potential champions).
+
+### Complexity Analysis
+
+1. **Time Complexity**:
+ - Computing in-degrees: _**O(m)**_, where _**m**_ is the number of edges.
+ - Checking teams: _**O(n)**_, where _**n**_ is the number of teams.
+ - Total: _**O(n + m)**_.
+
+2. **Space Complexity**:
+ - `inDegree` array: _**O(n)**_.
+
+This solution is efficient and works within the given constraints.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
diff --git a/algorithms/002924-find-champion-ii/solution.php b/algorithms/002924-find-champion-ii/solution.php
new file mode 100644
index 000000000..e4a2b715f
--- /dev/null
+++ b/algorithms/002924-find-champion-ii/solution.php
@@ -0,0 +1,30 @@
+1 <= n == s.length <= 105
+- `s[i]` is either `'0'` or `'1'`.
+
+
+**Hint:**
+1. Every `1` in the string `s` should be swapped with every `0` on its right side.
+2. Iterate right to left and count the number of `0` that have already occurred, whenever you iterate on `1` add that counter to the answer.
+
+
+
+**Solution:**
+
+To solve this problem efficiently, we can use a greedy approach with a two-pointer-like strategy. The key insight is that every `1` (black ball) should be moved past the `0`s (white balls) that are to its right, minimizing the total number of swaps.
+
+### Approach
+1. **Track the Number of `0`s Encountered**:
+ - Iterate through the string from right to left.
+ - Count the number of `0`s encountered so far as you iterate.
+ - When you encounter a `1`, each `0` that is to its right contributes to a swap needed to move this `1` past those `0`s.
+ - Add the count of `0`s to the total swaps each time you encounter a `1`.
+
+2. **Calculate the Total Swaps**:
+ - The total number of swaps required will be the sum of the number of `0`s encountered when processing each `1`.
+
+Let's implement this solution in PHP: **[2938. Separate Black and White Balls](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/002938-separate-black-and-white-balls/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+1. **Initialize Counters**:
+ - `zeroCount` is initialized to `0` and tracks the number of `0`s encountered while iterating from right to left.
+ - `swaps` keeps track of the minimum swaps needed to group the `1`s (black balls) together.
+
+2. **Iterate Through the String**:
+ - Loop through the string from right to left using a for-loop.
+ - If the current character is `0`, increment `zeroCount` as it represents a white ball that will need to be swapped with a `1` to its left.
+ - If the current character is `1`, add `zeroCount` to `swaps` because each `0` encountered after this `1` contributes to a swap.
+
+3. **Return the Total Swaps**:
+ - The accumulated value of `swaps` represents the minimum number of swaps required to arrange all `1`s to the right.
+
+### Time Complexity
+- **Time Complexity**: `O(n)` where `n` is the length of the string `s`. We iterate through the string once and perform constant-time operations for each character.
+- **Space Complexity**: `O(1)` as we only use a few variables (`zeroCount` and `swaps`).
+
+### Example Analysis
+- **Example 1**: Input: `"101"`
+ - Iteration from right to left:
+ - `s[2] = '1'`: zeroCount = 0, swaps = 0
+ - `s[1] = '0'`: zeroCount = 1, swaps = 0
+ - `s[0] = '1'`: zeroCount = 1, add `1` to swaps, swaps = 1
+ - Output: `1`.
+
+- **Example 2**: Input: `"100"`
+ - Iteration from right to left:
+ - `s[2] = '0'`: zeroCount = 1, swaps = 0
+ - `s[1] = '0'`: zeroCount = 2, swaps = 0
+ - `s[0] = '1'`: zeroCount = 2, add `2` to swaps, swaps = 2
+ - Output: `2`.
+
+- **Example 3**: Input: `"0111"`
+ - Iteration from right to left:
+ - `s[3] = '1'`: zeroCount = 0, swaps = 0
+ - `s[2] = '1'`: zeroCount = 0, swaps = 0
+ - `s[1] = '1'`: zeroCount = 0, swaps = 0
+ - `s[0] = '0'`: zeroCount = 1, swaps = 0
+ - Output: `0` (All `1`s are already grouped).
+
+This solution provides an efficient way to determine the minimum steps to separate black and white balls using PHP.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
diff --git a/algorithms/002938-separate-black-and-white-balls/solution.php b/algorithms/002938-separate-black-and-white-balls/solution.php
new file mode 100644
index 000000000..f2315228e
--- /dev/null
+++ b/algorithms/002938-separate-black-and-white-balls/solution.php
@@ -0,0 +1,27 @@
+= 0; $i--) {
+ if ($s[$i] == '0') {
+ // Increment the count of zeros when encountering '0'
+ $zeroCount++;
+ } elseif ($s[$i] == '1') {
+ // When encountering '1', add the count of '0's to swaps
+ $swaps += $zeroCount;
+ }
+ }
+
+ return $swaps;
+ }
+}
\ No newline at end of file
diff --git a/algorithms/002940-find-building-where-alice-and-bob-can-meet/README.md b/algorithms/002940-find-building-where-alice-and-bob-can-meet/README.md
new file mode 100644
index 000000000..0cda6f5dd
--- /dev/null
+++ b/algorithms/002940-find-building-where-alice-and-bob-can-meet/README.md
@@ -0,0 +1,248 @@
+2940\. Find Building Where Alice and Bob Can Meet
+
+**Difficulty:** Hard
+
+**Topics:** `Array`, `Binary Search`, `Stack`, `Binary Indexed Tree`, `Segment Tree`, `Heap (Priority Queue)`, `Monotonic Stack`
+
+You are given a **0-indexed** array `heights` of positive integers, where `heights[i]` represents the height of the ith
building.
+
+If a person is in building `i`, they can move to any other building `j` if and only if `i < j` and `heights[i] < heights[j]`.
+
+You are also given another array `queries` where queries[i] = [ai, bi]
. On the ith
query, Alice is in building ai
while Bob is in building bi
.
+
+Return an array `ans` where `ans[i]` is **the index of the leftmost building** where Alice and Bob can meet on the ith
query. If Alice and Bob cannot move to a common building on query `i`, set `ans[i]` to `-1`.
+
+**Example 1:**
+
+- **Input:** heights = [6,4,8,5,2,7], queries = [[0,1],[0,3],[2,4],[3,4],[2,2]]
+- **Output:** [2,5,-1,5,2]
+- **Explanation:** In the first query, Alice and Bob can move to building 2 since heights[0] < heights[2] and heights[1] < heights[2].
+ In the second query, Alice and Bob can move to building 5 since heights[0] < heights[5] and heights[3] < heights[5].
+ In the third query, Alice cannot meet Bob since Alice cannot move to any other building.
+ In the fourth query, Alice and Bob can move to building 5 since heights[3] < heights[5] and heights[4] < heights[5].
+ In the fifth query, Alice and Bob are already in the same building.
+ For ans[i] != -1, It can be shown that ans[i] is the leftmost building where Alice and Bob can meet.
+ For ans[i] == -1, It can be shown that there is no building where Alice and Bob can meet.
+
+**Example 2:**
+
+- **Input:** heights = [5,3,8,2,6,1,4,6], queries = [[0,7],[3,5],[5,2],[3,0],[1,6]]
+- **Output:** [7,6,-1,4,6]
+- **Explanation:** In the first query, Alice can directly move to Bob's building since heights[0] < heights[7].
+ In the second query, Alice and Bob can move to building 6 since heights[3] < heights[6] and heights[5] < heights[6].
+ In the third query, Alice cannot meet Bob since Bob cannot move to any other building.
+ In the fourth query, Alice and Bob can move to building 4 since heights[3] < heights[4] and heights[0] < heights[4].
+ In the fifth query, Alice can directly move to Bob's building since heights[1] < heights[6].
+ For ans[i] != -1, It can be shown that ans[i] is the leftmost building where Alice and Bob can meet.
+ For ans[i] == -1, It can be shown that there is no building where Alice and Bob can meet.
+
+
+
+**Constraints:**
+
+- 1 <= heights.length <= 5 * 104
+- 1 <= heights[i] <= 109
+- 1 <= queries.length <= 5 * 104
+- queries[i] = [ai, bi]
+- 0 <= ai, bi <= heights.length - 1
+
+
+**Hint:**
+1. For each query `[x, y]`, if `x > y`, swap `x` and `y`. Now, we can assume that `x <= y`.
+2. For each query `[x, y]`, if `x == y` or `heights[x] < heights[y]`, then the answer is `y` since `x β€ y`.
+3. Otherwise, we need to find the smallest index `t` such that `y < t` and `heights[x] < heights[t]`. Note that `heights[y] <= heights[x]`, so `heights[x] < heights[t]` is a sufficient condition.
+4. To find index `t` for each query, sort the queries in descending order of `y`. Iterate over the queries while maintaining a monotonic stack which we can binary search over to find index `t`.
+
+
+
+**Solution:**
+
+The problem requires determining the **leftmost building** where Alice and Bob can meet given their starting buildings and movement rules. Each query involves finding a meeting point based on building heights. This is challenging due to the constraints on movement and the need for efficient computation.
+
+### **Key Points**
+1. Alice and Bob can move to another building if its height is strictly greater than the current building.
+2. For each query, find the **leftmost valid meeting point**, or return `-1` if no such building exists.
+3. The constraints demand a solution better than a naive O(nΒ²) approach.
+
+### **Approach**
+
+1. **Observations:**
+ - If `a == b`, Alice and Bob are already at the same building.
+ - If `heights[a] < heights[b]`, Bob's building is the meeting point.
+ - Otherwise, find the smallest building index `t > b` where:
+ - `heights[a] < heights[t]`
+ - `heights[b] <= heights[t]` (as `b` is already less than `a` in height comparison).
+
+2. **Optimization Using Monotonic Stack:**
+ - A **monotonic stack** helps efficiently track the valid buildings Alice and Bob can move to. Buildings are added to the stack in a way that ensures heights are in decreasing order, enabling fast binary searches.
+
+3. **Query Sorting:**
+ - Sort the queries in descending order of `b` to process buildings with larger indices first. This ensures that we build the stack efficiently as we move from higher to lower indices.
+
+4. **Binary Search on Stack:**
+ - For each query, use binary search on the monotonic stack to find the smallest index `t` that satisfies the conditions.
+
+### **Plan**
+
+1. Sort queries based on the larger of the two indices (`b`) in descending order.
+2. Traverse the array backward while maintaining a monotonic stack of valid indices.
+3. For each query, check trivial cases (`a == b` or `heights[a] < heights[b]`).
+4. For non-trivial cases, use the stack to find the leftmost valid building via binary search.
+5. Return the results in the original query order.
+
+### **Solution Steps**
+
+1. **Preprocess Queries:**
+ - Ensure `a <= b` in each query for consistency.
+ - Sort queries by `b` in descending order.
+
+2. **Iterate Through Queries:**
+ - Maintain a monotonic stack as we traverse the array.
+ - For each query:
+ - If `a == b`, the answer is `b`.
+ - If `heights[a] < heights[b]`, the answer is `b`.
+ - Otherwise, use the stack to find the smallest valid index `t > b`.
+
+3. **Binary Search on Stack:**
+ - Use binary search to quickly find the smallest index `t` on the stack that satisfies `heights[t] > heights[a]`.
+
+4. **Restore Original Order:**
+ - Map results back to the original query indices.
+
+5. **Return Results.**
+
+Let's implement this solution in PHP: **[2940. Find Building Where Alice and Bob Can Meet](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/002940-find-building-where-alice-and-bob-can-meet/solution.php)**
+
+```php
+queryIndex = $queryIndex;
+ $this->a = $a;
+ $this->b = $b;
+ }
+}
+
+// Test the function
+$heights = [6, 4, 8, 5, 2, 7];
+$queries = [[0, 1], [0, 3], [2, 4], [3, 4], [2, 2]];
+print_r(leftmostBuildingQueries($heights, $queries));
+
+$heights = [5, 3, 8, 2, 6, 1, 4, 6];
+$queries = [[0, 7], [3, 5], [5, 2], [3, 0], [1, 6]];
+print_r(leftmostBuildingQueries($heights, $queries));
+?>
+```
+
+### Explanation:
+
+1. **Sorting Queries:** The queries are sorted by `b` in descending order to process larger indices first, which allows us to update our monotonic stack as we process.
+2. **Monotonic Stack:** The stack is used to keep track of building indices where Alice and Bob can meet. We only keep buildings that have a height larger than any previously seen buildings in the stack.
+3. **Binary Search:** When answering each query, we use binary search to efficiently find the smallest index `t` where the conditions are met.
+
+### **Example Walkthrough**
+
+#### Input:
+- `heights = [6,4,8,5,2,7]`
+- `queries = [[0,1],[0,3],[2,4],[3,4],[2,2]]`
+
+#### Process:
+
+1. **Sort Queries:**
+ - Indexed queries: `[(2,4), (3,4), (0,3), (0,1), (2,2)]`
+
+2. **Build Monotonic Stack:**
+ - Start at the highest index and add indices to the stack:
+ - At index 5: Stack = `[5]`
+ - At index 4: Stack = `[5, 4]`
+ - ...
+
+3. **Query Processing:**
+ - For query `[0,1]`, `heights[0] < heights[1]`: Result = 2.
+ - ...
+
+#### Output:
+`[2, 5, -1, 5, 2]`
+
+### **Time Complexity**
+
+1. **Query Sorting:** `O(Q log Q)` where Q is the number of queries.
+2. **Monotonic Stack Construction:** `O(N)` where N is the length of `heights`.
+3. **Binary Search for Each Query:** `O(Q log N)`.
+
+**Overall:** `O(N + Q log (Q + N))`.
+
+### **Output for Example**
+
+**Input:**
+```php
+$heights = [6, 4, 8, 5, 2, 7];
+$queries = [[0, 1], [0, 3], [2, 4], [3, 4], [2, 2]];
+```
+
+**Output:**
+```php
+print_r(findBuilding($heights, $queries)); // [2, 5, -1, 5, 2]
+```
+
+This approach efficiently handles large constraints by leveraging a monotonic stack and binary search. It ensures optimal query processing while maintaining correctness.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
diff --git a/algorithms/002940-find-building-where-alice-and-bob-can-meet/solution.php b/algorithms/002940-find-building-where-alice-and-bob-can-meet/solution.php
new file mode 100644
index 000000000..3158aaa38
--- /dev/null
+++ b/algorithms/002940-find-building-where-alice-and-bob-can-meet/solution.php
@@ -0,0 +1,101 @@
+getIndexedQueries($queries) as $indexedQuery) {
+ $queryIndex = $indexedQuery->queryIndex;
+ $a = $indexedQuery->a;
+ $b = $indexedQuery->b;
+
+ if ($a == $b || $heights[$a] < $heights[$b]) {
+ // 1. Alice and Bob are already in the same index (a == b) or
+ // 2. Alice can jump from a -> b (heights[a] < heights[b]).
+ $ans[$queryIndex] = $b;
+ } else {
+ // Now, a < b and heights[a] >= heights[b].
+ // Gradually add heights with an index > b to the monotonic stack.
+ while ($heightsIndex > $b) {
+ // heights[heightsIndex] is a better candidate.
+ while (!empty($stack) && $heights[end($stack)] <= $heights[$heightsIndex]) {
+ array_pop($stack);
+ }
+ $stack[] = $heightsIndex--;
+ }
+
+ // Binary search to find the smallest index j such that j > b and
+ // heights[j] > heights[a], ensuring heights[j] > heights[b].
+ $it = $this->findUpperBound($stack, $a, $heights);
+ if ($it !== null) {
+ $ans[$queryIndex] = $it;
+ }
+ }
+ }
+
+ return $ans;
+ }
+
+ /**
+ * @param $queries
+ * @return array
+ */
+ private function getIndexedQueries($queries) {
+ $indexedQueries = [];
+ foreach ($queries as $i => $query) {
+ // Make sure that a <= b.
+ $a = min($query[0], $query[1]);
+ $b = max($query[0], $query[1]);
+ $indexedQueries[] = new IndexedQuery($i, $a, $b);
+ }
+
+ // Sort queries in descending order of b.
+ usort($indexedQueries, function ($a, $b) {
+ return $b->b - $a->b;
+ });
+
+ return $indexedQueries;
+ }
+
+ /**
+ * @param $stack
+ * @param $a
+ * @param $heights
+ * @return mixed|null
+ */
+ private function findUpperBound($stack, $a, $heights) {
+ foreach (array_reverse($stack) as $index) {
+ if ($heights[$index] > $heights[$a]) {
+ return $index;
+ }
+ }
+ return null;
+ }
+}
+
+class IndexedQuery {
+ public $queryIndex;
+ public $a; // Alice's index
+ public $b; // Bob's index
+
+ /**
+ * @param $queryIndex
+ * @param $a
+ * @param $b
+ */
+ public function __construct($queryIndex, $a, $b) {
+ $this->queryIndex = $queryIndex;
+ $this->a = $a;
+ $this->b = $b;
+ }
+}
\ No newline at end of file
diff --git a/algorithms/002981-find-longest-special-substring-that-occurs-thrice-i/README.md b/algorithms/002981-find-longest-special-substring-that-occurs-thrice-i/README.md
new file mode 100644
index 000000000..028fe58ca
--- /dev/null
+++ b/algorithms/002981-find-longest-special-substring-that-occurs-thrice-i/README.md
@@ -0,0 +1,122 @@
+2981\. Find Longest Special Substring That Occurs Thrice I
+
+**Difficulty:** Medium
+
+**Topics:** `Hash Table`, `String`, `Binary Search`, `Sliding Window`, `Counting`
+
+You are given a string `s` that consists of lowercase English letters.
+
+A string is called **special** if it is made up of only a single character. For example, the string `"abc"` is not special, whereas the strings `"ddd"`, `"zz"`, and `"f"` are special.
+
+Return _the length of the **longest special substring** of `s` which occurs **at least thrice**, or `-1` if no special substring occurs at least thrice_.
+
+A **substring** is a contiguous **non-empty** sequence of characters within a string.
+
+**Example 1:**
+
+- **Input:** s = "aaaa"
+- **Output:** 2
+- **Explanation:** The longest special substring which occurs thrice is "aa": substrings "aaaa", "aaaa", and "aaaa".
+ It can be shown that the maximum length achievable is 2.
+
+**Example 2:**
+
+- **Input:** s = "abcdef"
+- **Output:** -1
+- **Explanation:** There exists no special substring which occurs at least thrice. Hence return -1.
+
+
+**Example 3:**
+
+- **Input:** s = "abcdef"
+- **Output:** 1
+- **Explanation:** The longest special substring which occurs thrice is "a": substrings "abcaba", "abcaba", and "abcaba".
+ It can be shown that the maximum length achievable is 1.
+
+
+
+**Constraints:**
+
+- `3 <= s.length <= 50`
+- `s` consists of only lowercase English letters.
+
+
+**Hint:**
+1. The constraints are small.
+2. Brute force checking all substrings.
+
+
+
+**Solution:**
+
+We can use a brute force approach due to the small constraints of `s` (length of up to 50). We'll:
+
+1. Iterate over possible lengths of substrings (from longest to shortest).
+2. Check all substrings of the given length and count their occurrences.
+3. If a substring occurs at least three times, check if it is special (made of one repeated character).
+4. Return the length of the longest such substring. If no substring satisfies the conditions, return `-1`.
+
+Let's implement this solution in PHP: **[2981. Find Longest Special Substring That Occurs Thrice I](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/002981-find-longest-special-substring-that-occurs-thrice-i/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+1. **Outer Loop**: We iterate over the possible lengths of substrings, starting with the longest. This ensures we return the longest special substring as soon as we find it.
+2. **Sliding Window**: For each substring length, we use a sliding window approach to extract all substrings of that length.
+3. **Counting Substrings**: We use an associative array (`$countMap`) to store and count occurrences of each substring.
+4. **Checking Special**: A helper function `isSpecial` checks if the substring is made up of only one repeated character.
+5. **Returning the Result**: If a valid substring is found, we return its length; otherwise, we return `-1`.
+
+### Complexity
+- **Time Complexity**: _**O(n3)**_ in the worst case because we:
+ 1. Iterate over _**n**_ substring lengths.
+ 2. Extract _**O(n)**_ substrings for each length.
+ 3. Check if each substring is special, which takes _**O(n)**_ time.
+- **Space Complexity**: _**O(n2)**_ due to the substring counting map.
+
+This brute force approach is feasible given the constraints (_**n <= 50**_).
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
diff --git a/algorithms/002981-find-longest-special-substring-that-occurs-thrice-i/solution.php b/algorithms/002981-find-longest-special-substring-that-occurs-thrice-i/solution.php
new file mode 100644
index 000000000..7f93a5844
--- /dev/null
+++ b/algorithms/002981-find-longest-special-substring-that-occurs-thrice-i/solution.php
@@ -0,0 +1,54 @@
+= 1; $len--) {
+ $countMap = [];
+
+ // Sliding window to collect substrings of length $len
+ for ($i = 0; $i <= $n - $len; $i++) {
+ $substring = substr($s, $i, $len);
+
+ // Count the occurrences of each substring
+ if (!isset($countMap[$substring])) {
+ $countMap[$substring] = 0;
+ }
+ $countMap[$substring]++;
+ }
+
+ // Check if any special substring occurs at least 3 times
+ foreach ($countMap as $substring => $count) {
+ if ($count >= 3 && $this->isSpecial($substring)) {
+ return $len; // Return the length of the substring
+ }
+ }
+ }
+
+ // If no special substring occurs at least 3 times
+ return -1;
+ }
+
+ /**
+ * Helper function to check if a substring is special
+ *
+ * @param $substring
+ * @return bool
+ */
+ function isSpecial($substring) {
+ $char = $substring[0];
+ for ($i = 1; $i < strlen($substring); $i++) {
+ if ($substring[$i] !== $char) {
+ return false;
+ }
+ }
+ return true;
+ }
+}
\ No newline at end of file
diff --git a/algorithms/003011-find-if-array-can-be-sorted/README.md b/algorithms/003011-find-if-array-can-be-sorted/README.md
new file mode 100644
index 000000000..2bdf8444e
--- /dev/null
+++ b/algorithms/003011-find-if-array-can-be-sorted/README.md
@@ -0,0 +1,151 @@
+3011\. Find if Array Can Be Sorted
+
+**Difficulty:** Medium
+
+**Topics:** `Array`, `Bit Manipulation`, `Sorting`
+
+You are given a **0-indexed** array of **positive** integers `nums`.
+
+In one **operation**, you can swap any two **adjacent** elements if they have the **same** number of set bits[^1]. You are allowed to do this operation **any** number of times (**including zero**).
+
+Return _`true` if you can sort the array, else return `false`_.
+
+**Example 1:**
+
+- **Input:** nums = [8,4,2,30,15]
+- **Output:** true
+- **Explanation:** Let's look at the binary representation of every element. The numbers 2, 4, and 8 have one set bit each with binary representation "10", "100", and "1000" respectively. The numbers 15 and 30 have four set bits each with binary representation "1111" and "11110".
+ We can sort the array using 4 operations:
+ - Swap nums[0] with nums[1]. This operation is valid because 8 and 4 have one set bit each. The array becomes [4,8,2,30,15].
+ - Swap nums[1] with nums[2]. This operation is valid because 8 and 2 have one set bit each. The array becomes [4,2,8,30,15].
+ - Swap nums[0] with nums[1]. This operation is valid because 4 and 2 have one set bit each. The array becomes [2,4,8,30,15].
+ - Swap nums[3] with nums[4]. This operation is valid because 30 and 15 have four set bits each. The array becomes [2,4,8,15,30].
+ The array has become sorted, hence we return true.
+ Note that there may be other sequences of operations which also sort the array.
+
+**Example 2:**
+
+- **Input:** nums = [1,2,3,4,5]
+- **Output:** true
+- **Explanation:** The array is already sorted, hence we return true.
+
+
+**Example 3:**
+
+- **Input:** nums = [3,16,8,4,2]
+- **Output:** false
+- **Explanation:** It can be shown that it is not possible to sort the input array using any number of operations.
+
+**Example 4:**
+
+- **Input:** nums = [75,34,30]
+- **Output:** false
+- **Explanation:** It can be shown that it is not possible to sort the input array using any number of operations.
+
+
+**Constraints:**
+
+- `1 <= nums.length <= 100`
+- 1 <= nums[i] <= 28
+
+
+**Hint:**
+1. Split the array into segments. Each segment contains consecutive elements with the same number of set bits.
+2. From left to right, the previous segmentβs largest element should be smaller than the current segmentβs smallest element.
+
+[^1]: **Set Bit** A set bit refers to a bit in the binary representation of a number that has a value of `1`.
+
+**Solution:**
+
+We need to determine if the array can be sorted by only swapping adjacent elements that have the same number of set bits in their binary representation. Hereβs the plan:
+
+### Solution Steps:
+
+1. **Key Observation**: The operation allows us to swap adjacent elements only if they have the same number of set bits. This restricts swapping across elements with different numbers of set bits.
+
+2. **Plan**:
+ - Group elements by the number of set bits in their binary representation.
+ - Sort each group individually, since within a group, elements can be rearranged by swaps.
+ - After sorting each group, merge the sorted groups back together.
+ - Check if this merged array is sorted. If it is, then sorting the array using the allowed operations is possible.
+
+3. **Steps**:
+ - Count the set bits in each number and group numbers with the same set bit count.
+ - Sort each group individually.
+ - Reconstruct the array from these sorted groups and verify if the result is sorted.
+
+Let's implement this solution in PHP: **[3011. Find if Array Can Be Sorted](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/003011-find-if-array-can-be-sorted/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+1. **countSetBits Function**: Counts the number of set bits in a number using bitwise operations.
+2. **Grouping Elements**: `bitGroups` is an associative array where each key represents the set bit count, and each value is an array of numbers with that many set bits.
+3. **Sorting and Rebuilding**:
+ - We iterate over `nums` to group elements by their set bit count.
+ - We sort each group independently.
+ - We then reconstruct the array by inserting each sorted group element in its original order.
+ - Finally, we check if the reconstructed array is sorted in non-decreasing order. If it is, return `true`; otherwise, return `false`.
+
+4. **Final Comparison**: Compare the rebuilt array with a fully sorted version of `nums`. If they match, return `true`; otherwise, return `false`.
+
+### Complexity Analysis
+
+- **Time Complexity**: _**O(n log n)**_ due to the sorting within each group and the final comparison.
+- **Space Complexity**: _**O(n)**_ for storing the bit groups.
+
+This solution ensures that we only swap adjacent elements with the same set bit count, achieving a sorted order if possible.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
diff --git a/algorithms/003011-find-if-array-can-be-sorted/solution.php b/algorithms/003011-find-if-array-can-be-sorted/solution.php
new file mode 100644
index 000000000..c456e7310
--- /dev/null
+++ b/algorithms/003011-find-if-array-can-be-sorted/solution.php
@@ -0,0 +1,55 @@
+countSetBits($num);
+
+ // If we're in a new segment (set bit count changed)
+ if ($setBits !== $prevSetBits) {
+ // Check if previous segmentβs max is greater than current segmentβs min
+ if ($prevMax > $currMin) {
+ return false;
+ }
+
+ // Start a new segment
+ $prevSetBits = $setBits;
+ $prevMax = $currMax;
+ $currMax = $num;
+ $currMin = $num;
+ } else {
+ // Continue with the current segment
+ $currMax = max($currMax, $num);
+ $currMin = min($currMin, $num);
+ }
+ }
+
+ // Final check: last segment's max should be <= last segment's min
+ return $prevMax <= $currMin;
+ }
+
+ /**
+ * Helper function to count set bits in a number
+ *
+ * @param $n
+ * @return int
+ */
+ private function countSetBits($num) {
+ $count = 0;
+ while ($num > 0) {
+ $count += $num & 1;
+ $num >>= 1;
+ }
+ return $count;
+ }
+}
\ No newline at end of file
diff --git a/algorithms/003042-count-prefix-and-suffix-pairs-i/README.md b/algorithms/003042-count-prefix-and-suffix-pairs-i/README.md
new file mode 100644
index 000000000..acc4047c5
--- /dev/null
+++ b/algorithms/003042-count-prefix-and-suffix-pairs-i/README.md
@@ -0,0 +1,139 @@
+3042\. Count Prefix and Suffix Pairs I
+
+**Difficulty:** Easy
+
+**Topics:** `Array`, `String`, `Trie`, `Rolling Hash`, `String Matching`, `Hash Function`
+
+You are given a **0-indexed** string array words.
+
+Let's define a **boolean** function `isPrefixAndSuffix` that takes two strings, `str1` and `str2`:
+
+- `isPrefixAndSuffix(str1, str2)` returns `true` if `str1` is **both** a prefix[^1] and a suffix[^2] of `str2`, and `false` otherwise.
+
+For example, `isPrefixAndSuffix("aba", "ababa")` is `true` because `"aba"` is a prefix of `"ababa"` and also a suffix, but `isPrefixAndSuffix("abc", "abcd")` is `false`.
+
+Return an integer denoting the **number** of index pairs `(i, j)` such that `i < j`, and `isPrefixAndSuffix(words[i], words[j])` is `true`.
+
+**Example 1:**
+
+- **Input:** words = ["a","aba","ababa","aa"]
+- **Output:** 4
+- **Explanation:** In this example, the counted index pairs are:
+ i = 0 and j = 1 because isPrefixAndSuffix("a", "aba") is true.
+ i = 0 and j = 2 because isPrefixAndSuffix("a", "ababa") is true.
+ i = 0 and j = 3 because isPrefixAndSuffix("a", "aa") is true.
+ i = 1 and j = 2 because isPrefixAndSuffix("aba", "ababa") is true.
+ Therefore, the answer is 4.
+
+**Example 2:**
+
+- **Input:** words = ["pa","papa","ma","mama"]
+- **Output:** 2
+- **Explanation:** In this example, the counted index pairs are:
+ i = 0 and j = 1 because isPrefixAndSuffix("pa", "papa") is true.
+ i = 2 and j = 3 because isPrefixAndSuffix("ma", "mama") is true.
+ Therefore, the answer is 2.
+
+
+**Example 3:**
+
+- **Input:** words = ["abab","ab"]
+- **Output:** 0
+- **Explanation:** In this example, the only valid index pair is i = 0 and j = 1, and isPrefixAndSuffix("abab", "ab") is false.
+ Therefore, the answer is 0.
+
+
+
+**Constraints:**
+
+- `1 <= words.length <= 50`
+- `1 <= words[i].length <= 10`
+- `words[i]` consists only of lowercase English letters.
+
+
+**Hint:**
+1. Iterate through all index pairs `(i, j)`, such that `i < j`, and check `isPrefixAndSuffix(words[i], words[j])`.
+2. The answer is the total number of pairs where `isPrefixAndSuffix(words[i], words[j]) == true`.
+
+[^1]: **Prefix** `A prefix of a string is a substring that starts from the beginning of the string and extends to any point within it.`
+[^2]: **Suffix** `A suffix of a string is a substring that begins at any point in the string and extends to its end.`
+
+**Solution:**
+
+We need to iterate through all index pairs `(i, j)` where `i < j` and check whether the string `words[i]` is both a prefix and a suffix of `words[j]`. For each pair, we can use PHP's built-in functions `substr()` to check for prefixes and suffixes.
+
+Let's implement this solution in PHP: **[3042. Count Prefix and Suffix Pairs I](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/003042-count-prefix-and-suffix-pairs-i/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+1. **countPrefixAndSuffixPairs($words):**
+ - This function loops through all possible index pairs `(i, j)` such that `i < j`.
+ - It calls `isPrefixAndSuffix()` to check if `words[i]` is both a prefix and a suffix of `words[j]`.
+ - If the condition is true, it increments the count.
+
+2. **isPrefixAndSuffix($str1, $str2):**
+ - This helper function checks whether `str1` is both a prefix and a suffix of `str2`.
+ - It uses `substr()` to extract the prefix and suffix of `str2` and compares them with `str1`.
+ - If both conditions are true, it returns `true`, otherwise, it returns `false`.
+
+### Time Complexity:
+- The time complexity is _**O(n2 x m)**_, where `n` is the length of the `words` array, and `m` is the average length of the strings in the array. This is due to the nested loops and the `substr()` operations.
+
+### Example Output:
+For the given input arrays:
+- `["a", "aba", "ababa", "aa"]` -> Output: `4`
+- `["pa", "papa", "ma", "mama"]` -> Output: `2`
+- `["abab", "ab"]` -> Output: `0`
+
+This solution should work efficiently within the given constraints.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
diff --git a/algorithms/003042-count-prefix-and-suffix-pairs-i/solution.php b/algorithms/003042-count-prefix-and-suffix-pairs-i/solution.php
new file mode 100644
index 000000000..34682c0e3
--- /dev/null
+++ b/algorithms/003042-count-prefix-and-suffix-pairs-i/solution.php
@@ -0,0 +1,37 @@
+isPrefixAndSuffix($words[$i], $words[$j])) {
+ $count++;
+ }
+ }
+ }
+
+ return $count;
+ }
+
+ /**
+ * Function to check if str1 is both a prefix and a suffix of str2
+ *
+ * @param $str1
+ * @param $str2
+ * @return bool
+ */
+ function isPrefixAndSuffix($str1, $str2) {
+ // Check if str1 is a prefix and a suffix of str2
+ return (substr($str2, 0, strlen($str1)) === $str1 && substr($str2, -strlen($str1)) === $str1);
+ }
+}
\ No newline at end of file
diff --git a/algorithms/003043-find-the-length-of-the-longest-common-prefix/README.md b/algorithms/003043-find-the-length-of-the-longest-common-prefix/README.md
new file mode 100644
index 000000000..a2bf8b650
--- /dev/null
+++ b/algorithms/003043-find-the-length-of-the-longest-common-prefix/README.md
@@ -0,0 +1,118 @@
+3043\. Find the Length of the Longest Common Prefix
+
+**Difficulty:** Medium
+
+**Topics:** `Array`, `Hash Table`, `String`, `Trie`
+
+You are given two arrays with **positive** integers `arr1` and `arr2`.
+
+A **prefix** of a positive integer is an integer formed by one or more of its digits, starting from its **leftmost** digit. For example, `123` is a prefix of the integer `12345`, while `234` is **not**.
+
+A **common prefix** of two integers `a` and `b` is an integer `c`, such that `c` is a prefix of both `a` and `b`. For example, `5655359` and `56554` have a common prefix `565` while `1223` and `43456` **do not** have a common prefix.
+
+You need to find the length of the **longest common prefix** between all pairs of integers `(x, y)` such that `x` belongs to `arr1` and `y` belongs to `arr2`.
+
+Return _the length of the **longest** common prefix among all pairs. If no common prefix exists among them, return `0`_.
+
+**Example 1:**
+
+- **Input:** arr1 = [1,10,100], arr2 = [1000]
+- **Output:** 3
+- **Explanation:** There are 3 pairs (arr1[i], arr2[j]):
+ - The longest common prefix of (1, 1000) is 1.
+ - The longest common prefix of (10, 1000) is 10.
+ - The longest common prefix of (100, 1000) is 100.\
+ The longest common prefix is 100 with a length of 3.
+
+**Example 2:**
+
+- **Input:** arr1 = [1,2,3], arr2 = [4,4,4]
+- **Output:** 4
+- **Explanation:** There exists no common prefix for any pair (arr1[i], arr2[j]), hence we return 0.\
+ Note that common prefixes between elements of the same array do not count.
+
+
+
+**Constraints:**
+
+- 1 <= arr1.length, arr2.length <= 5 * 104
+- 1 <= arr1[i], arr2[i] <= 108
+
+
+**Hint:**
+1. Put all the possible prefixes of each element in `arr1` into a HashSet.
+2. For all the possible prefixes of each element in `arr2`, check if it exists in the HashSet.
+
+
+
+**Solution:**
+
+We can utilize a HashSet to store the prefixes from one array and then check for those prefixes in the second array.
+
+### Approach:
+
+1. **Generate Prefixes**: For each number in `arr1` and `arr2`, generate all possible prefixes. A prefix is formed by one or more digits starting from the leftmost digit.
+
+2. **Store Prefixes of `arr1` in a Set**: Using a `HashSet` to store all prefixes of numbers in `arr1` ensures fast lookups when checking prefixes from `arr2`.
+
+3. **Find Longest Common Prefix**: For each number in `arr2`, generate its prefixes and check if any of these prefixes exist in the `HashSet` from step 2. Track the longest prefix found.
+
+4. **Return the Length of the Longest Common Prefix**: If a common prefix is found, return its length; otherwise, return `0`.
+
+Let's implement this solution in PHP: **[3043. Find the Length of the Longest Common Prefix](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/003043-find-the-length-of-the-longest-common-prefix/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+1. **HashSet Creation**:
+ - We first create an associative array `$prefixSet` to hold all possible prefixes of numbers in `arr1`.
+ - We iterate through each number in `arr1`, convert it to a string, and extract all its prefixes using the `substr` function. Each prefix is stored in the `$prefixSet`.
+
+2. **Prefix Checking**:
+ - Next, we loop through each number in `arr2`, converting it to a string as well.
+ - For each number in `arr2`, we again extract all possible prefixes.
+ - If a prefix exists in `$prefixSet`, we check if its length is greater than the current maximum length found (`$maxLength`).
+
+3. **Return the Result**:
+ - Finally, we return the length of the longest common prefix found.
+
+### Complexity:
+- **Time Complexity**: O(n * m) where n and m are the lengths of `arr1` and `arr2` respectively. This is because we are processing every number and their prefixes.
+- **Space Complexity**: O(p) where p is the total number of prefixes stored in the HashSet.
+
+This solution is efficient and works well within the provided constraints.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
diff --git a/algorithms/003043-find-the-length-of-the-longest-common-prefix/solution.php b/algorithms/003043-find-the-length-of-the-longest-common-prefix/solution.php
new file mode 100644
index 000000000..69fc5a548
--- /dev/null
+++ b/algorithms/003043-find-the-length-of-the-longest-common-prefix/solution.php
@@ -0,0 +1,37 @@
+1 <= nums.length <= 2 * 105
+- 0 <= nums[i] <= 109
+- 0 <= k <= 109
+
+
+**Hint:**
+1. For each `nums[i]`, we can maintain each subarrayβs bitwise `OR` result ending with it.
+2. The property of bitwise `OR` is that it never unsets any bits and only sets new bits
+3. So the number of different results for each `nums[i]` is at most the number of bits 32.
+
+[^1]: _**Subarray** : A **subarray** is a contiguous **non-empty** sequence of elements within an array._
+
+
+**Solution:**
+
+We can use a sliding window approach combined with bit manipulation to keep track of the OR of elements in the window.
+
+### Plan:
+1. **Sliding Window Approach**: Iterate over the array using two pointers, maintaining a subarray whose OR value is checked.
+2. **Bitwise OR**: The OR operation accumulates values. It never reduces the result (i.e., once a bit is set to `1`, it cannot be unset). This means as we extend the window, the OR value only increases or stays the same.
+3. **Efficiency**: We can use a deque (double-ended queue) to maintain indices of the subarrays. This allows us to efficiently slide the window while keeping track of the minimum subarray length.
+
+### Steps:
+1. Traverse the array, for each element, maintain a running OR.
+2. For each element, check if the OR exceeds or equals `k`. If it does, try to shrink the window from the left side.
+3. The sliding window should be moved efficiently by keeping track of the OR value in a deque structure to allow constant time sliding and shrinking.
+
+Let's implement this solution in PHP: **[3097. Shortest Subarray With OR at Least K II](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/003097-shortest-subarray-with-or-at-least-k-ii/solution.php)**
+
+```php
+minimumSubarrayLength($nums1, $k1) . "\n"; // Output: 1
+
+$nums2 = [2, 1, 8];
+$k2 = 10;
+echo $solution->minimumSubarrayLength($nums2, $k2) . "\n"; // Output: 3
+
+$nums3 = [1, 2];
+$k3 = 0;
+echo $solution->minimumSubarrayLength($nums3, $k3) . "\n"; // Output: 1
+?>
+```
+
+### Explanation:
+
+1. **`minimumSubarrayLength` Method**:
+ - Initialize `ans` to an impossible high value (`$n + 1`).
+ - Use two pointers `l` (left) and `r` (right) to expand and shrink the window.
+ - Calculate the OR of the subarray as you expand the window with `orNum` and reduce it with `undoOrNum` when shrinking.
+ - Whenever the OR result meets or exceeds `k`, check if the current window size is smaller than `ans`.
+
+2. **`orNum` and `undoOrNum` Methods**:
+ - `orNum` method: Adds bits to the cumulative OR by updating the `count` array. If a bit is newly set in the window (meaning `count[i]` becomes `1`), that bit is added to `ors`.
+ - `undoOrNum` method: Removes bits from the cumulative OR when sliding the window leftward. If a bit is no longer set in any of the numbers in the window (meaning `count[i]` becomes `0`), that bit is removed from `ors`.
+
+3. **Time Complexity**:
+ - The time complexity is O(n) because each index is added and removed from the deque at most once.
+ - `n` is the length of the input array.
+
+4**Time Complexity**:
+ - The space complexity is O(n) for storing the prefix OR array and the deque.
+
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
diff --git a/algorithms/003097-shortest-subarray-with-or-at-least-k-ii/solution.php b/algorithms/003097-shortest-subarray-with-or-at-least-k-ii/solution.php
new file mode 100644
index 000000000..f7c26370d
--- /dev/null
+++ b/algorithms/003097-shortest-subarray-with-or-at-least-k-ii/solution.php
@@ -0,0 +1,68 @@
+orNum($ors, $nums[$r], $count);
+ // Shrink window from the left as long as ors >= k
+ while ($ors >= $k && $l <= $r) {
+ $ans = min($ans, $r - $l + 1);
+ $ors = $this->undoOrNum($ors, $nums[$l], $count);
+ $l++;
+ }
+ }
+
+ return ($ans == $n + 1) ? -1 : $ans;
+ }
+
+ /**
+ * @param $ors
+ * @param $num
+ * @param $count
+ * @return int
+ */
+ private function orNum($ors, $num, &$count) {
+ // Update the ors value and count bits that are set
+ for ($i = 0; $i < self::K_MAX_BIT; $i++) {
+ if (($num >> $i) & 1) { // Check if the i-th bit is set
+ $count[$i]++;
+ if ($count[$i] == 1) { // Only add to ors if this bit is newly set in the window
+ $ors += (1 << $i); // Add this bit to the cumulative OR
+ }
+ }
+ }
+ return $ors;
+ }
+
+ /**
+ * @param $ors
+ * @param $num
+ * @param $count
+ * @return int
+ */
+ private function undoOrNum($ors, $num, &$count) {
+ // Reverse the update on ors and count bits that are reset
+ for ($i = 0; $i < self::K_MAX_BIT; $i++) {
+ if (($num >> $i) & 1) { // Check if the i-th bit is set
+ $count[$i]--;
+ if ($count[$i] == 0) { // Only remove from ors if this bit is no longer set in the window
+ $ors -= (1 << $i); // Remove this bit from the cumulative OR
+ }
+ }
+ }
+ return $ors;
+ }
+}
\ No newline at end of file
diff --git a/algorithms/003133-minimum-array-end/README.md b/algorithms/003133-minimum-array-end/README.md
new file mode 100644
index 000000000..0a91b1cbb
--- /dev/null
+++ b/algorithms/003133-minimum-array-end/README.md
@@ -0,0 +1,106 @@
+3133\. Minimum Array End
+
+**Difficulty:** Medium
+
+**Topics:** `Bit Manipulation`
+
+You are given two integers `n` and `x`. You have to construct an array of **positive** integers `nums` of size `n` where for every `0 <= i < n - 1`, `nums[i + 1]` is **greater than** `nums[i]`, and the result of the bitwise `AND` operation between all elements of `nums` is `x`.
+
+Return _the **minimum** possible value of `nums[n - 1]`_.
+
+**Example 1:**
+
+- **Input:** n = 3, x = 4
+- **Output:** 6
+- **Explanation:** `nums` can be `[4,5,6]` and its last element is 6.
+
+**Example 2:**
+
+- **Input:** n = 2, x = 7
+- **Output:** 15
+- **Explanation:** `nums` can be `[7,15]` and its last element is 15.
+
+
+**Example 3:**
+
+- **Input:** cost = [[2, 5, 1], [3, 4, 7], [8, 1, 2], [6, 2, 4], [3, 8, 8]]
+- **Output:** 10
+
+
+
+**Constraints:**
+
+- 1 <= n, x <= 108
+
+
+**Hint:**
+1. Each element of the array should be obtained by βmergingβ `x` and `v` where `v = 0, 1, 2, β¦(n - 1)`.
+2. To merge `x` with another number `v`, keep the set bits of `x` untouched, for all the other bits, fill the set bits of `v` from right to left in order one by one.
+3. So the final answer is the βmergeβ of `x` and `n - 1`.
+
+
+
+**Solution:**
+
+We need to construct an array `nums` of positive integers of size `n`, where each successive element is greater than the previous. The bitwise `AND` of all elements in `nums` should yield `x`. We are asked to find the minimum possible value of `nums[n-1]`.
+
+Hereβs the breakdown:
+
+1. **Bit Manipulation Insight**: We can observe that `nums[i]` should be built by merging `x` with integers `0, 1, ..., n-1`. This will help ensure the bitwise `AND` result yields `x` since we start with a base of `x`.
+
+2. **Building the Array Elements**: Each element can be thought of as `x` merged with some integer, and we aim to keep `x`'s bits intact. We fill in additional bits from the integer to get increasing numbers while maintaining the `AND` outcome as `x`.
+
+3. **Merging Strategy**: To find the minimum `nums[n-1]`, we only need to merge `x` with `n-1`. Merging in this context means that if any bit in `x` is `1`, it remains `1`. We use bits from `n-1` to add any required additional bits without altering the bits set in `x`.
+
+
+Let's implement this solution in PHP: **[3133. Minimum Array End](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/003133-minimum-array-end/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+1. **Bit Checking and Setting**:
+ - We check each bit of `ans` (starting from `x`), and if a bit is `0` in `ans`, we look to the corresponding bit in `k` (which is `n-1`).
+ - If that bit in `k` is `1`, we set the bit in `ans` to `1`. This process ensures the minimum increment in value while preserving bits set in `x`.
+
+2. **Loop Constraints**:
+ - We iterate through each bit position up to a calculated maximum (`kMaxBit`), ensuring that we cover the bits necessary from both `x` and `n`.
+
+3. **Result**:
+ - The final value of `ans` is the minimum possible value for `nums[n-1]` that satisfies the conditions.
+
+### Complexity:
+- The algorithm operates in constant time since the number of bits in any integer in this range is bounded by 32, making this approach efficient even for large values of `n` and `x`.
+
+This solution yields the desired minimum `nums[n-1]` while maintaining the required properties.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
diff --git a/algorithms/003133-minimum-array-end/solution.php b/algorithms/003133-minimum-array-end/solution.php
new file mode 100644
index 000000000..1b221e5a9
--- /dev/null
+++ b/algorithms/003133-minimum-array-end/solution.php
@@ -0,0 +1,31 @@
+> $i) & 1) == 0) {
+ // Set the bit in $ans if the corresponding bit in $k is 1
+ if (($k >> $kBinaryIndex) & 1) {
+ $ans |= (1 << $i);
+ }
+ ++$kBinaryIndex;
+ }
+ }
+
+ return $ans;
+ }
+}
\ No newline at end of file
diff --git a/algorithms/003152-special-array-ii/README.md b/algorithms/003152-special-array-ii/README.md
new file mode 100644
index 000000000..8a7115308
--- /dev/null
+++ b/algorithms/003152-special-array-ii/README.md
@@ -0,0 +1,127 @@
+3152\. Special Array II
+
+**Difficulty:** Medium
+
+**Topics:** `Array`, `Binary Search`, `Prefix Sum`
+
+An array is considered **special** if every pair of its adjacent elements contains two numbers with different parity.
+
+You are given an array of integer `nums` and a 2D integer matrix `queries`, where for queries[i] = [fromi, toi]
your task is to check that subarray[^1] nums[fromi..toi]
is special or not.
+
+Return _an array of booleans `answer` such that `answer[i]` is true if nums[fromi..toi]
is special_.
+
+**Example 1:**
+
+- **Input:** nums = [3,4,1,2,6], queries = [[0,4]]
+- **Output:** [false]
+- **Explanation:** The subarray is `[3,4,1,2,6]`. `2` and `6` are both even.
+
+**Example 2:**
+
+- **Input:** nums = [4,3,1,6], queries = [[0,2],[2,3]]
+- **Output:** [false,true]
+- **Explanation:**
+
+ 1. The subarray is `[4,3,1]`. `3` and `1` are both odd. So the answer to this query is `false`.
+ 2. The subarray is `[1,6]`. There is only one pair: `(1,6)` and it contains numbers with different parity. So the answer to this query is `true`.
+
+
+**Constraints:**
+
+- 1 <= nums.length <= 105
+- 1 <= nums[i] <= 105
+- 1 <= queries.length <= 105
+- `queries[i].length == 2`
+- `0 <= queries[i][0] <= queries[i][1] <= nums.length - 1`
+
+
+**Hint:**
+1. Try to split the array into some non-intersected continuous special subarrays.
+2. For each query check that the first and the last elements of that query are in the same subarray or not.
+
+[^1]: **Subarray** A **subarray** is a contiguous sequence of elements within an array
.
+
+
+
+**Solution:**
+
+We need to determine whether a subarray of `nums` is "special," i.e., every pair of adjacent elements in the subarray must have different parity (one must be odd, and the other must be even).
+
+### Approach:
+
+1. **Identify Parity Transitions:**
+ We can preprocess the array to mark positions where the parity changes. For example:
+ - `0` represents an even number.
+ - `1` represents an odd number.
+
+ The idea is to identify all positions where adjacent elements have different parity. This will help us efficiently determine if a subarray is special by checking if the positions in the query are part of the same "special" block.
+
+2. **Preprocessing:**
+ Create a binary array `parity_change` where each element is `1` if the adjacent elements have different parity, otherwise `0`. For example:
+ - If `nums[i]` and `nums[i+1]` have different parity, set `parity_change[i] = 1`, otherwise `0`.
+
+3. **Prefix Sum Array:**
+ Construct a prefix sum array `prefix_sum` where each entry at index `i` represents the cumulative number of parity transitions up to that index. This helps quickly check if all pairs within a subarray have different parity.
+
+4. **Query Processing:**
+ For each query `[from, to]`, check if there is any position in the range `[from, to-1]` where the parity doesn't change. This can be done by checking the difference in the prefix sum values: `prefix_sum[to] - prefix_sum[from]`.
+
+Let's implement this solution in PHP: **[3152. Special Array II](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/003152-special-array-ii/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+1. **Preprocessing Parity Transitions:**
+ We calculate `parity_change[i] = 1` if the elements `nums[i]` and `nums[i+1]` have different parity. Otherwise, we set it to `0`.
+
+2. **Prefix Sum Array:**
+ The `prefix_sum[i]` stores the cumulative count of parity transitions from the start of the array up to index `i`. This allows us to compute how many transitions occurred in any subarray `[from, to]` in constant time using the formula:
+ ```php
+ $transition_count = $prefix_sum[$to] - $prefix_sum[$from];
+ ```
+
+3. **Query Evaluation:**
+ For each query, if the number of transitions is equal to the length of the subarray minus 1, the subarray is special, and we return `true`. Otherwise, we return `false`.
+
+### Time Complexity:
+- Preprocessing the parity transitions takes O(n).
+- Constructing the prefix sum array takes O(n).
+- Each query can be answered in O(1) using the prefix sum array.
+- Hence, the total time complexity is O(n + q), where `n` is the length of the array and `q` is the number of queries.
+
+This solution efficiently handles the problem constraints with an optimized approach.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
diff --git a/algorithms/003152-special-array-ii/solution.php b/algorithms/003152-special-array-ii/solution.php
new file mode 100644
index 000000000..2308aa746
--- /dev/null
+++ b/algorithms/003152-special-array-ii/solution.php
@@ -0,0 +1,42 @@
+1 <= word.length <= 2 * 105
+- `word` consists only of lowercase English letters.
+
+
+**Hint:**
+1. Each time, just cut the same character in prefix up to at max 9 times. Itβs always better to cut a bigger prefix.
+
+
+
+**Solution:**
+
+We can use a greedy approach to compress the string by taking the longest possible prefix of repeating characters (up to 9 occurrences at a time) and then appending the length of the prefix along with the character to the result.
+
+Here's the step-by-step solution:
+
+1. **Initialize Variables**:
+ - `comp` (the compressed string) starts as an empty string.
+ - Use a pointer or index `i` to track the position in the `word`.
+
+2. **Loop through `word`**:
+ - While there are characters left in `word`, find the longest prefix of repeating characters that does not exceed 9 characters.
+ - Count how many times the current character repeats consecutively, up to a maximum of 9.
+
+3. **Append to Compressed String**:
+ - Append the count followed by the character to `comp`.
+ - Move the pointer `i` forward by the number of characters processed.
+
+4. **Return Result**:
+ - After processing the entire string, return the compressed string `comp`.
+
+Let's implement this solution in PHP: **[3163. String Compression III](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/003163-string-compression-iii/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+- **Counting Loop**: We use a `while` loop inside the main loop to count consecutive characters (up to 9) for each unique character in `word`.
+- **Appending Results**: After counting each sequence, we append the count and character directly to `comp`.
+- **Pointer Update**: The main loop pointer `i` moves forward by the number of characters counted, effectively reducing the size of the remaining string in each iteration.
+
+### Complexity Analysis
+
+- **Time Complexity**: _**O(n)**_, where _**n**_ is the length of `word`. Each character is processed once.
+- **Space Complexity**: _**O(n)**_ for the compressed result stored in `comp`.
+
+This solution is efficient and handles edge cases, such as sequences shorter than 9 characters or a single occurrence of each character.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
\ No newline at end of file
diff --git a/algorithms/003163-string-compression-iii/solution.php b/algorithms/003163-string-compression-iii/solution.php
new file mode 100644
index 000000000..d2193ef76
--- /dev/null
+++ b/algorithms/003163-string-compression-iii/solution.php
@@ -0,0 +1,30 @@
+edges1[i] = [ai, bi] indicates that there is an edge between nodes ai
and bi
in the first tree and edges2[i] = [ui, vi]
indicates that there is an edge between nodes ui
and vi
in the second tree.
+
+You must connect one node from the first tree with another node from the second tree with an edge.
+
+Return _the **minimum** possible **diameter** of the resulting tree_.
+
+The **diameter** of a tree is the length of the _longest_ path between any two nodes in the tree.
+
+**Example 1:**
+
+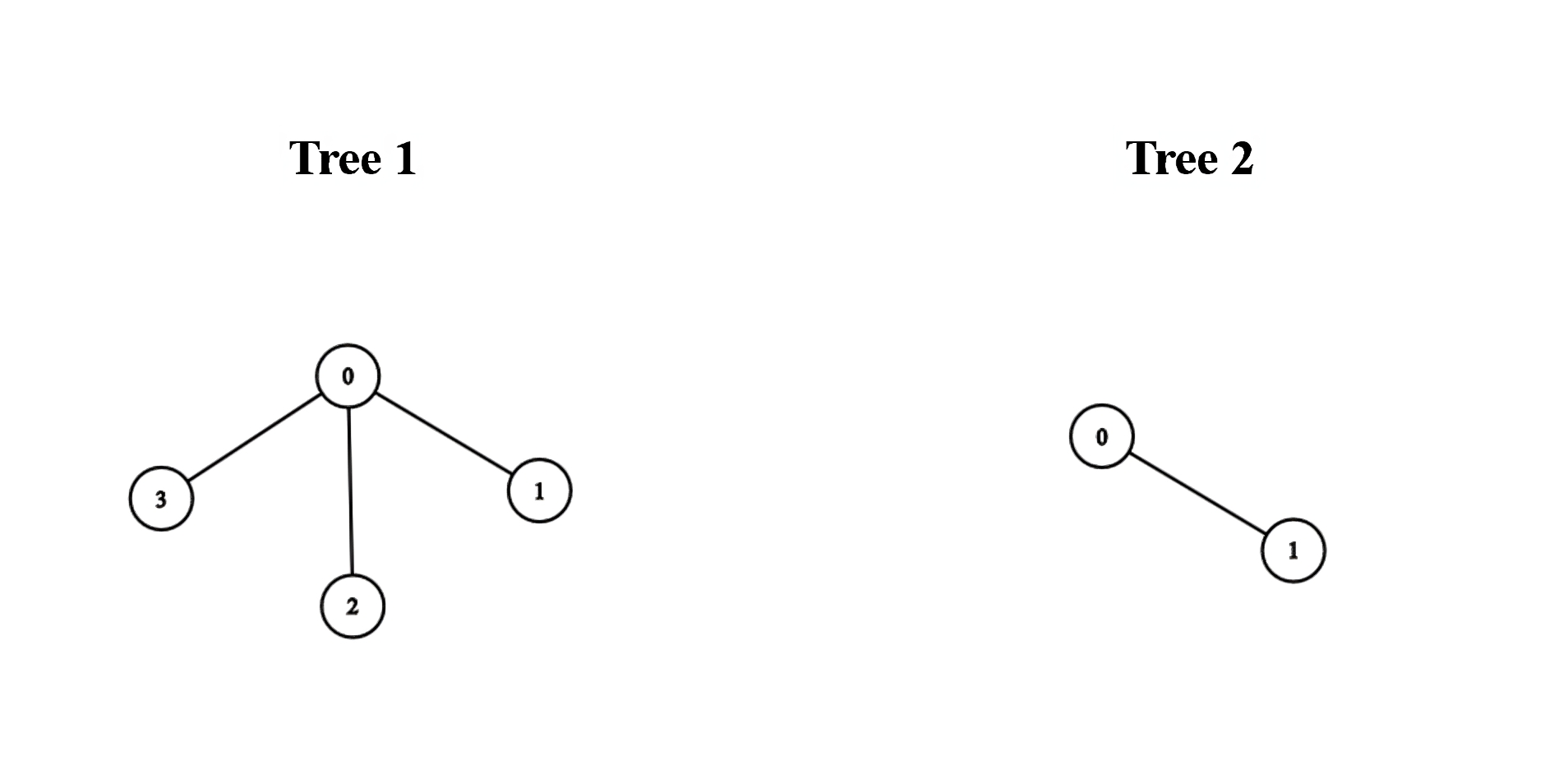
+
+- **Input:** edges1 = [[0,1],[0,2],[0,3]], edges2 = [[0,1]]
+- **Output:** 3
+- **Explanation:** We can obtain a tree of diameter 3 by connecting node 0 from the first tree with any node from the second tree.
+
+**Example 2:**
+
+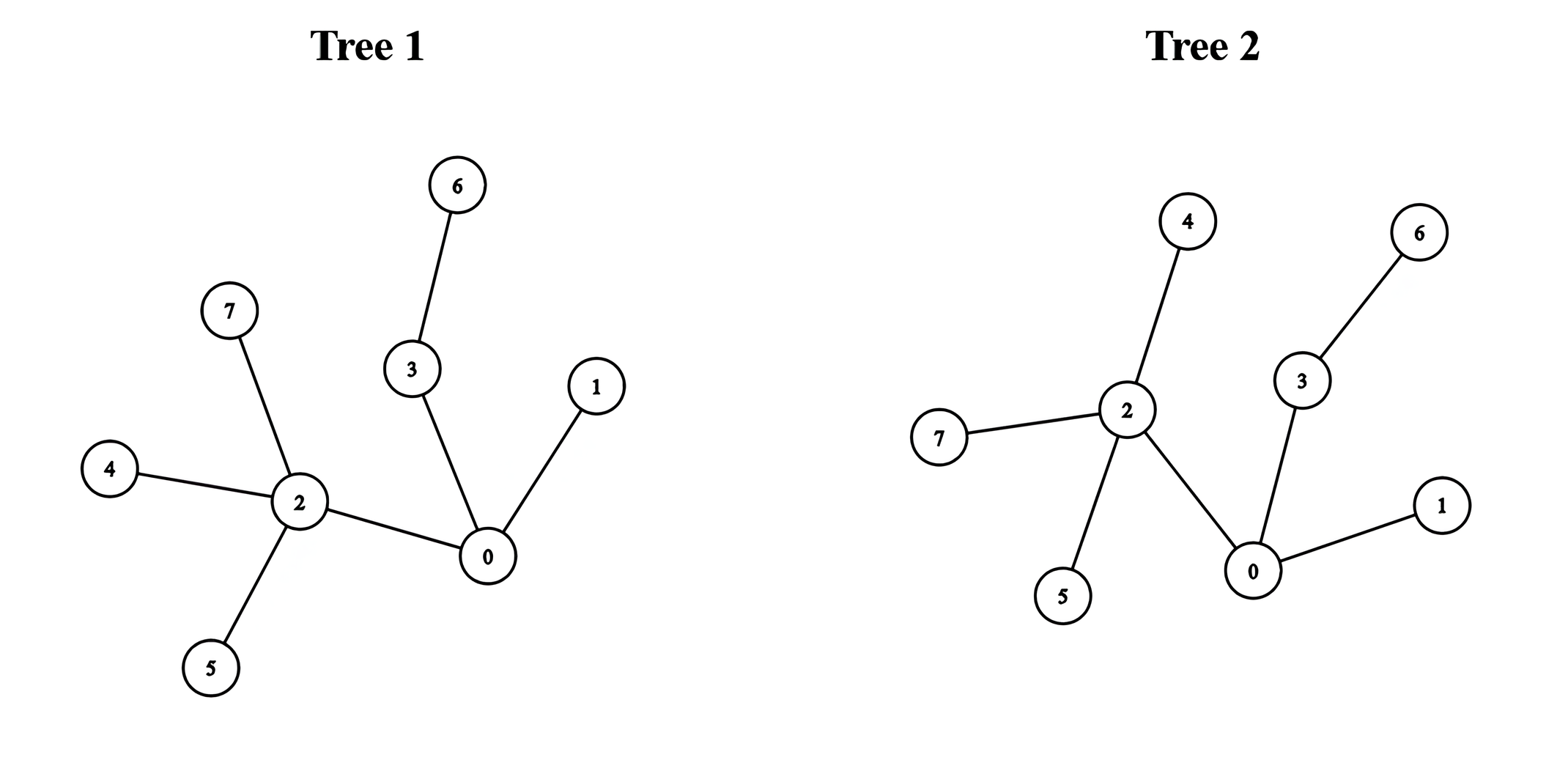
+
+- **Input:** edges1 = [[0,1],[0,2],[0,3],[2,4],[2,5],[3,6],[2,7]], edges2 = [[0,1],[0,2],[0,3],[2,4],[2,5],[3,6],[2,7]]
+- **Output:** 5
+- **Explanation:** We can obtain a tree of diameter 5 by connecting node 0 from the first tree with node 0 from the second tree.
+
+
+**Constraints:**
+
+- 1 <= n, m <= 105
+- `edges1.length == n - 1`
+- `edges2.length == m - 1`
+- `edges1[i].length == edges2[i].length == 2`
+- edges1[i] = [ai, bi]
+- 0 <= ai, bi < n
+- edges2[i] = [ui, vi]
+- 0 <= ui, vi < m
+- The input is generated such that `edges1` and `edges2` represent valid trees.
+
+
+**Hint:**
+1. Suppose that we connected node a in tree1 with node b in tree2. The diameter length of the resulting tree will be the largest of the following 3 values:
+ 1. The diameter of tree 1.
+ 2. The diameter of tree 2.
+ 3. The length of the longest path that starts at node a and that is completely within Tree 1 + The length of the longest path that starts at node b and that is completely within Tree 2 + 1.
+ The added one in the third value is due to the additional edge that we have added between trees 1 and 2.
+2. Values 1 and 2 are constant regardless of our choice of a and b. Therefore, we need to pick a and b in such a way that minimizes value 3.
+3. If we pick `a` and `b` optimally, they will be in the diameters of Tree 1 and Tree 2, respectively. Exactly which nodes of the diameter should we pick?
+4. `a` is the center of the diameter of tree 1, and `b` is the center of the diameter of tree 2.
+
+
+
+**Solution:**
+
+We need to approach it step-by-step with a focus on understanding how to calculate the diameter of a tree and how connecting the two trees influences the overall diameter.
+
+### Steps to solve:
+
+1. **Find the diameter of each tree**:
+ - The diameter of a tree is the longest path between any two nodes. To find it, we can use the following two-step process:
+ 1. Perform a BFS (or DFS) from an arbitrary node to find the farthest node (let's call this node `A`).
+ 2. Perform another BFS (or DFS) starting from `A` to find the farthest node from `A` (let's call this node `B`), and the distance from `A` to `B` will be the diameter of the tree.
+
+2. **Determine the optimal nodes to connect**:
+ - From the hint in the problem, the best way to minimize the additional diameter when connecting two trees is to connect the centers of the diameters of both trees. This will minimize the longest path caused by the new edge.
+ - The optimal node in a tree's diameter is typically the "center", which can be found by performing a BFS from the endpoints of the diameter and finding the middle of the longest path.
+
+3. **Minimize the total diameter**:
+ - Once we find the centers of both trees, the new diameter is the maximum of:
+ - The diameter of Tree 1.
+ - The diameter of Tree 2.
+ - The sum of the longest path within Tree 1, the longest path within Tree 2, and 1 for the new connecting edge.
+
+Let's implement this solution in PHP: **[3203. Find Minimum Diameter After Merging Two Trees](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/003203-find-minimum-diameter-after-merging-two-trees/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+1. **BFS Helper Function**: The `bfs` function calculates the farthest node from a given starting node and returns the distance array and the farthest node found. This is essential for calculating the diameter of the tree.
+
+2. **Get Diameter and Center**: The `getDiameterAndCenter` function finds the diameter of a tree and its center. The center of the tree is crucial for minimizing the new tree's diameter when merging two trees.
+
+3. **Main Solution**:
+ - We first build adjacency lists for both trees.
+ - We calculate the diameter and center for both trees.
+ - We perform BFS from the centers of both trees to get the longest paths within each tree.
+ - Finally, we calculate the maximum of the three values to get the minimum diameter of the merged tree.
+
+### Time Complexity:
+- Constructing the adjacency list: O(n + m)
+- BFS traversal: O(n + m)
+- Overall time complexity is O(n + m), which is efficient for the input size limit of 105
.
+
+This approach ensures we find the minimum possible diameter when merging the two trees.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
diff --git a/algorithms/003203-find-minimum-diameter-after-merging-two-trees/solution.php b/algorithms/003203-find-minimum-diameter-after-merging-two-trees/solution.php
new file mode 100644
index 000000000..39ea25f39
--- /dev/null
+++ b/algorithms/003203-find-minimum-diameter-after-merging-two-trees/solution.php
@@ -0,0 +1,102 @@
+getDiameterAndCenter($n, $edgesTree1);
+ list($diameter2, $center2) = $this->getDiameterAndCenter($m, $edgesTree2);
+
+ // Now find the longest paths in each tree from their respective centers
+ list($distFromCenter1, $farthestFromCenter1) = $this->bfs($n, $edgesTree1, $center1);
+ list($distFromCenter2, $farthestFromCenter2) = $this->bfs($m, $edgesTree2, $center2);
+
+ // The result is the maximum of the 3 diameters
+ return max($diameter1, $diameter2, $distFromCenter1[$farthestFromCenter1] + 1 + $distFromCenter2[$farthestFromCenter2]);
+ }
+
+ /**
+ * Helper function to find the diameter of a tree and the farthest node from a start node
+ *
+ * @param $n
+ * @param $edges
+ * @param $start
+ * @return array
+ */
+ function bfs($n, $edges, $start) {
+ $dist = array_fill(0, $n, -1);
+ $dist[$start] = 0;
+ $queue = [$start];
+ $farthestNode = $start;
+
+ while (count($queue) > 0) {
+ $node = array_shift($queue);
+ foreach ($edges[$node] as $neighbor) {
+ if ($dist[$neighbor] == -1) {
+ $dist[$neighbor] = $dist[$node] + 1;
+ $queue[] = $neighbor;
+ if ($dist[$neighbor] > $dist[$farthestNode]) {
+ $farthestNode = $neighbor;
+ }
+ }
+ }
+ }
+ return [$dist, $farthestNode];
+ }
+
+ /**
+ * Helper function to find the diameter of a tree and its center
+ *
+ * @param $n
+ * @param $edges
+ * @return array
+ */
+ function getDiameterAndCenter($n, $edges) {
+ // Find farthest node from an arbitrary node (node 0)
+ list($distFromFirst, $farthestFromFirst) = $this->bfs($n, $edges, 0);
+
+ // Find farthest node from the farthest node found (this gives the diameter's length)
+ list($distFromFarthest, $farthestFromFarthest) = $this->bfs($n, $edges, $farthestFromFirst);
+
+ // Now find the center (middle of the diameter)
+ $path = [];
+ $current = $farthestFromFarthest;
+ while ($current != $farthestFromFirst) {
+ $path[] = $current;
+ foreach ($edges[$current] as $neighbor) {
+ if ($distFromFarthest[$neighbor] == $distFromFarthest[$current] - 1) {
+ $current = $neighbor;
+ break;
+ }
+ }
+ }
+ $path[] = $farthestFromFirst;
+ $diameterLength = $distFromFarthest[$farthestFromFarthest];
+ $center = $path[floor(count($path) / 2)];
+
+ return [$diameterLength, $center];
+ }
+}
\ No newline at end of file
diff --git a/algorithms/003217-delete-nodes-from-linked-list-present-in-array/README.md b/algorithms/003217-delete-nodes-from-linked-list-present-in-array/README.md
new file mode 100644
index 000000000..0addd1fc5
--- /dev/null
+++ b/algorithms/003217-delete-nodes-from-linked-list-present-in-array/README.md
@@ -0,0 +1,157 @@
+3217\. Delete Nodes From Linked List Present in Array
+
+**Difficulty:** Medium
+
+**Topics:** `Array`, `Hash Table`, `Linked List`
+
+You are given an array of integers `nums` and the `head` of a linked list. Return _the `head` of the modified linked list after `removing` all nodes from the linked list that have a value that exists in `nums`_.
+
+**Example 1:**
+
+- **Input:** nums = [1,2,3], head = [1,2,3,4,5]
+- **Output:** [4,5]
+- **Explanation:**
+ 
+ Remove the nodes with values 1, 2, and 3.
+
+**Example 2:**
+
+- **Input:** Input: nums = [1], head = [1,2,1,2,1,2]
+- **Output:** [2,2,2]
+- **Explanation:**
+
+ Remove the nodes with value 1.
+
+**Example 3:**
+
+- **Input:** nums = [5], head = [1,2,3,4]
+- **Output:** [1,2,3,4]
+ 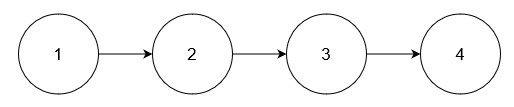
+ No node has value 5.
+
+
+
+**Constraints:**
+
+- 1 <= nums.length <= 105
+- 1 <= nums[i] <= 105
+- All elements in `nums` are unique.
+- The number of nodes in the given list is in the range [1, 105]
.
+- 1 <= Node.val <= 105
+- The input is generated such that there is at least one node in the linked list that has a value not present in `nums`.
+
+
+**Hint:**
+1. Add all elements of `nums` into a Set.
+2. Scan the list to check if the current element should be deleted by checking the Set.
+
+
+
+**Solution:**
+
+We need to traverse through the linked list and remove any nodes that have a value present in the array `nums`.
+
+### Approach:
+1. **Hash Set for Fast Lookup**: Since checking if a value exists in `nums` needs to be efficient, we will convert `nums` into a hash set. This allows O(1) lookup for each value.
+2. **Iterate Through the Linked List**: We will iterate through the linked list and remove nodes whose values are present in the hash set.
+3. **Pointer Manipulation**: While iterating, we will adjust the pointers to "skip" nodes that match values in the `nums` array.
+
+### Steps:
+1. Convert `nums` to a hash set for O(1) lookup.
+2. Traverse the linked list with two pointers: one for the current node and one for the previous node to help remove nodes efficiently.
+3. For each node, check if the value is in the hash set. If it is, update the previous nodeβs `next` to skip the current node.
+
+Let's implement this solution in PHP: **[3217. Delete Nodes From Linked List Present in Array](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/003217-delete-nodes-from-linked-list-present-in-array/solution.php)**
+
+```php
+val = $val;
+ $this->next = $next;
+ }
+}
+
+class Solution {
+
+ /**
+ * @param Integer[] $nums
+ * @param ListNode $head
+ * @return ListNode
+ */
+ function removeElements($head, $nums) {
+ ...
+ ...
+ ...
+ /**
+ * go to ./solution.php
+ */
+ }
+}
+
+// Example usage:
+
+// Linked List: 1 -> 2 -> 3 -> 4 -> 5
+$head = new ListNode(1);
+$head->next = new ListNode(2);
+$head->next->next = new ListNode(3);
+$head->next->next->next = new ListNode(4);
+$head->next->next->next->next = new ListNode(5);
+
+// Array nums: [1, 2, 3]
+$nums = [1, 2, 3];
+
+$solution = new Solution();
+$result = $solution->removeElements($head, $nums);
+
+// Function to print the linked list
+function printList($node) {
+ while ($node !== null) {
+ echo $node->val . " ";
+ $node = $node->next;
+ }
+}
+
+// Print the resulting linked list
+printList($result); // Output: 4 5
+?>
+```
+
+### Explanation:
+
+1. **`removeElements($head, $nums)`**:
+ - We first convert `nums` into a hash set (`$numSet = array_flip($nums);`) for fast lookups.
+ - A dummy node is created and linked to the head of the list. This helps simplify edge cases like removing the head node.
+ - The `prev` pointer tracks the node before the current one, allowing us to remove the current node by skipping it in the list.
+ - For each node, we check if its value is in `numSet`. If so, we remove it by adjusting the `prev->next` pointer to skip the current node.
+
+2. **Edge Cases**:
+ - If the head node needs to be removed, the dummy node ensures the head can be cleanly removed and still return the correct list.
+ - Handles cases where multiple consecutive nodes need to be removed.
+
+3. **Complexity**:
+ - **Time Complexity**: O(n), where `n` is the number of nodes in the linked list. We visit each node once. Converting `nums` to a set takes O(m), where `m` is the size of `nums`.
+ - **Space Complexity**: O(m) for storing the `nums` set.
+
+### Example Walkthrough:
+
+For input `nums = [1, 2, 3]` and `head = [1, 2, 3, 4, 5]`, the algorithm will:
+- Start at node 1, check if 1 is in `nums`, and remove it.
+- Move to node 2, check if 2 is in `nums`, and remove it.
+- Move to node 3, check if 3 is in `nums`, and remove it.
+- Move to node 4 and 5, which are not in `nums`, so they remain in the list.
+
+The resulting linked list is `[4, 5]`.
+
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
diff --git a/algorithms/003217-delete-nodes-from-linked-list-present-in-array/solution.php b/algorithms/003217-delete-nodes-from-linked-list-present-in-array/solution.php
new file mode 100644
index 000000000..7f7bcf3fb
--- /dev/null
+++ b/algorithms/003217-delete-nodes-from-linked-list-present-in-array/solution.php
@@ -0,0 +1,47 @@
+val = $val;
+ * $this->next = $next;
+ * }
+ * }
+ */
+class Solution {
+
+ /**
+ * @param Integer[] $nums
+ * @param ListNode $head
+ * @return ListNode
+ */
+ function modifiedList($nums, $head) {
+ // Convert nums array to a hash set for O(1) lookups
+ $numSet = array_flip($nums);
+
+ // Create a dummy node to handle edge cases (e.g. removing the head)
+ $dummy = new ListNode(0);
+ $dummy->next = $head;
+
+ // Initialize pointers
+ $prev = $dummy;
+ $current = $head;
+
+ // Traverse the linked list
+ while ($current !== null) {
+ // If the current node's value exists in numSet, remove it
+ if (isset($numSet[$current->val])) {
+ $prev->next = $current->next; // Skip the current node
+ } else {
+ $prev = $current; // Move prev pointer forward
+ }
+ $current = $current->next; // Move current pointer forward
+ }
+
+ // Return the modified list starting from the dummy's next node
+ return $dummy->next;
+ }
+}
\ No newline at end of file
diff --git a/algorithms/003223-minimum-length-of-string-after-operations/README.md b/algorithms/003223-minimum-length-of-string-after-operations/README.md
new file mode 100644
index 000000000..ccce542fd
--- /dev/null
+++ b/algorithms/003223-minimum-length-of-string-after-operations/README.md
@@ -0,0 +1,137 @@
+3223\. Minimum Length of String After Operations
+
+**Difficulty:** Medium
+
+**Topics:** `Hash Table`, `String`, `Counting`
+
+You are given a string `s`.
+
+You can perform the following process on `s` **any** number of times:
+
+- Choose an index `i` in the string such that there is **at least** one character to the left of index `i` that is equal to `s[i]`, and **at least** one character to the right that is also equal to `s[i]`.
+- Delete the **closest** character to the **left** of index `i` that is equal to `s[i]`.
+- Delete the **closest** character to the **right** of index `i` that is equal to `s[i]`.
+
+Return _the **minimum** length of the final string `s` that you can achieve_.
+
+**Example 1:**
+
+- **Input:** s = "abaacbcbb"
+- **Output:** 5
+- **Explanation:** We do the following operations:
+ - Choose index 2, then remove the characters at indices 0 and 3. The resulting string is s = "bacbcbb".
+ - Choose index 3, then remove the characters at indices 0 and 5. The resulting string is s = "acbcb".
+
+
+**Example 2:**
+
+- **Input:** s = "aa"
+- **Output:** 2
+- **Explanation:** We cannot perform any operations, so we return the length of the original string.
+
+
+
+**Constraints:**
+
+- 1 <= s.length <= 2 * 105
+- `s` consists only of lowercase English letters.
+
+
+**Hint:**
+1. Only the frequency of each character matters in finding the final answer.
+2. If a character occurs less than 3 times, we cannot perform any process with it.
+3. Suppose there is a character that occurs at least 3 times in the string, we can repeatedly delete two of these characters until there are at most 2 occurrences left of it.
+
+
+
+**Solution:**
+
+We need to focus on the frequency of each character in the string. Hereβs the approach to solve it:
+
+### Approach:
+1. **Count Character Frequencies**:
+ - Use a frequency table to count how many times each character appears in the string.
+
+2. **Reduce Characters with Frequency >= 3**:
+ - If a character appears 3 or more times, we can repeatedly delete two of them until only 2 occurrences are left.
+
+3. **Calculate the Minimum Length**:
+ - Sum up the remaining counts of all characters after reducing the frequencies.
+
+Let's implement this solution in PHP: **[3223. Minimum Length of String After Operations](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/003223-minimum-length-of-string-after-operations/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+1. **Frequency Count**:
+ - Iterate through the string, and for each character, increment its count in the `$frequency` array.
+
+2. **Reduction of Characters**:
+ - For each character in the `$frequency` array, check if its count is 3 or more. If so, reduce it to at most 2.
+
+3. **Calculate the Result**:
+ - Sum the values in the `$frequency` array to get the minimum possible length of the string.
+
+### Example Walkthrough:
+
+#### Example 1:
+- Input: `s = "abaacbcbb"`
+- Frequencies: `['a' => 3, 'b' => 4, 'c' => 2]`
+- After reduction:
+ - `'a' => 2` (reduced from 3),
+ - `'b' => 2` (reduced from 4),
+ - `'c' => 2` (no reduction needed).
+- Minimum length: `2 + 2 + 2 = 6`.
+
+#### Example 2:
+- Input: `s = "aa"`
+- Frequencies: `['a' => 2]`
+- No reduction needed since no character has a frequency of 3 or more.
+- Minimum length: `2`.
+
+### Complexity:
+1. **Time Complexity**:
+ - Counting frequencies: _**O(n)**_, where _**n**_ is the length of the string.
+ - Reduction: _**O(1)**_ (constant time, as there are only 26 lowercase letters).
+ - Summing frequencies: _**O(1)**_.
+ - Overall: _**O(n)**_.
+
+2. **Space Complexity**:
+ - _**O(1)**_, since the frequency array has at most 26 entries.
+
+This solution is efficient and works well within the problem's constraints.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
diff --git a/algorithms/003223-minimum-length-of-string-after-operations/solution.php b/algorithms/003223-minimum-length-of-string-after-operations/solution.php
new file mode 100644
index 000000000..8c6b5d2b2
--- /dev/null
+++ b/algorithms/003223-minimum-length-of-string-after-operations/solution.php
@@ -0,0 +1,38 @@
+= 3
+ foreach ($frequency as $char => $count) {
+ if ($count >= 3) {
+ // Remove pairs of characters
+ $frequency[$char] = $count % 2 == 0 ? 2 : 1;
+ }
+ }
+
+ // Step 3: Calculate the minimum length
+ $minLength = 0;
+ foreach ($frequency as $count) {
+ $minLength += $count;
+ }
+
+ return $minLength;
+ }
+}
\ No newline at end of file
diff --git a/algorithms/003243-shortest-distance-after-road-addition-queries-i/README.md b/algorithms/003243-shortest-distance-after-road-addition-queries-i/README.md
new file mode 100644
index 000000000..3afb497d7
--- /dev/null
+++ b/algorithms/003243-shortest-distance-after-road-addition-queries-i/README.md
@@ -0,0 +1,164 @@
+3243\. Shortest Distance After Road Addition Queries I
+
+**Difficulty:** Medium
+
+**Topics:** `Array`, `Breadth-First Search`, `Graph`
+
+You are given an integer `n` and a 2D integer array `queries`.
+
+There are `n` cities numbered from `0` to `n - 1`. Initially, there is a **unidirectional** road from city `i` to city `i + 1` for all `0 <= i < n - 1`.
+
+queries[i] = [ui, vi]
represents the addition of a new **unidirectional** road from city ui
to city vi
. After each query, you need to find the **length** of the **shortest path** from city `0` to city `n - 1`.
+
+Return _an array `answer` where for each `i` in the range `[0, queries.length - 1]`, `answer[i]` is the length of the shortest path from city `0` to city `n - 1` after processing the **first** `i + 1` queries_.
+
+**Example 1:**
+
+- **Input:** n = 5, queries = [[2,4],[0,2],[0,4]]
+- **Output:** [3,2,1]
+- **Explanation:**
+ 
+ After the addition of the road from 2 to 4, the length of the shortest path from 0 to 4 is 3.
+ 
+ After the addition of the road from 0 to 2, the length of the shortest path from 0 to 4 is 2.
+ 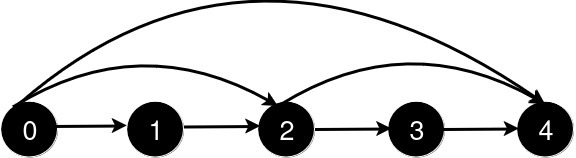
+ After the addition of the road from 0 to 4, the length of the shortest path from 0 to 4 is 1.
+
+**Example 2:**
+
+- **Input:** n = 4, queries = [[0,3],[0,2]]
+- **Output:** [1,1]
+- **Explanation:**
+ 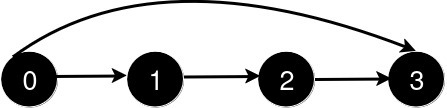
+ After the addition of the road from 0 to 3, the length of the shortest path from 0 to 3 is 1.
+ 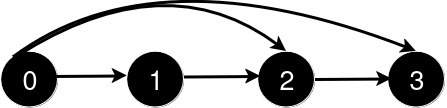
+ After the addition of the road from 0 to 2, the length of the shortest path remains 1.
+
+
+
+**Constraints:**
+
+- `3 <= n <= 500`
+- `1 <= queries.length <= 500`
+- `queries[i].length == 2`
+- `0 <= queries[i][0] < queries[i][1] < n`
+- `1 < queries[i][1] - queries[i][0]`
+- There are no repeated roads among the queries.
+
+
+**Hint:**
+1. Maintain the graph and use an efficient shortest path algorithm after each update.
+2. We use BFS/Dijkstra for each query.
+
+
+
+**Solution:**
+
+We need to simulate adding roads between cities and calculate the shortest path from city `0` to city `n - 1` after each road addition. Given the constraints and the nature of the problem, we can use **Breadth-First Search (BFS)** for unweighted graphs.
+
+### Approach:
+1. **Graph Representation:**
+ - We can represent the cities and roads using an adjacency list. Initially, each city `i` will have a road to city `i + 1` for all `0 <= i < n - 1`.
+ - After each query, we add the road from `u_i` to `v_i` into the graph.
+
+2. **Shortest Path Calculation (BFS):**
+ - We can use BFS to calculate the shortest path from city `0` to city `n - 1`. BFS works well here because all roads have equal weight (each road has a length of 1).
+
+3. **Iterating over Queries:**
+ - For each query, we will add the new road to the graph and then use BFS to find the shortest path from city `0` to city `n - 1`. After processing each query, we store the result in the output array.
+
+4. **Efficiency:**
+ - Since we are using BFS after each query, and the graph size can be at most `500` cities with up to `500` queries, the time complexity for each BFS is `O(n + m)`, where `n` is the number of cities and `m` is the number of roads. We need to perform BFS up to 500 times, so the solution will run efficiently within the problem's constraints.
+
+Let's implement this solution in PHP: **[3243. Shortest Distance After Road Addition Queries I](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/003243-shortest-distance-after-road-addition-queries-i/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+
+1. **Graph Initialization:**
+ - An adjacency list `graph` is used to represent the cities and roads.
+ - Initially, roads exist only between consecutive cities (`i` to `i+1`).
+
+2. **BFS Function:**
+ - BFS is used to compute the shortest distance from city `0` to city `n - 1`. We maintain a queue for BFS and an array `distances` to store the minimum number of roads (edges) to reach each city.
+ - Initially, the distance to city `0` is set to `0`, and all other cities have a distance of infinity (`PHP_INT_MAX`).
+ - As we process each city in the BFS queue, we update the distances for its neighboring cities and continue until all reachable cities are visited.
+
+3. **Query Processing:**
+ - For each query, the new road is added to the graph (`u -> v`).
+ - BFS is called to calculate the shortest path from city `0` to city `n-1` after the update.
+ - The result of BFS is stored in the `result` array.
+
+4. **Output**:
+ - The `result` array contains the shortest path lengths after each query.
+
+5. **Time Complexity:**
+ - Each BFS takes `O(n + m)`, where `n` is the number of cities and `m` is the number of roads. Since the number of queries is `q`, the overall time complexity is `O(q * (n + m))`, which should be efficient for the given constraints.
+
+### Example Walkthrough:
+
+For the input `n = 5` and `queries = [[2, 4], [0, 2], [0, 4]]`:
+- Initially, the roads are `[0 -> 1 -> 2 -> 3 -> 4]`.
+- After the first query `[2, 4]`, the roads are `[0 -> 1 -> 2 -> 3 -> 4]` and the shortest path from `0` to `4` is `3` (using the path `0 -> 1 -> 2 -> 4`).
+- After the second query `[0, 2]`, the roads are `[0 -> 2, 1 -> 2 -> 3 -> 4]`, and the shortest path from `0` to `4` is `2` (using the path `0 -> 2 -> 4`).
+- After the third query `[0, 4]`, the roads are `[0 -> 2, 1 -> 2 -> 3 -> 4]`, and the shortest path from `0` to `4` is `1` (direct road `0 -> 4`).
+
+Thus, the output is `[3, 2, 1]`.
+
+### Final Thoughts:
+- The solution uses BFS for each query to efficiently calculate the shortest path.
+- The graph is dynamically updated as new roads are added in each query.
+- The solution works well within the problem's constraints and efficiently handles up to 500 queries with up to 500 cities.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
diff --git a/algorithms/003243-shortest-distance-after-road-addition-queries-i/solution.php b/algorithms/003243-shortest-distance-after-road-addition-queries-i/solution.php
new file mode 100644
index 000000000..82e1f7172
--- /dev/null
+++ b/algorithms/003243-shortest-distance-after-road-addition-queries-i/solution.php
@@ -0,0 +1,61 @@
+bfs($graph, $n);
+ }
+
+ return $result;
+ }
+
+ /**
+ * Function to find the shortest path using BFS
+ *
+ * @param $graph
+ * @param $n
+ * @return int
+ */
+ function bfs($graph, $n) {
+ $queue = [[0, 0]]; // [current node, distance from source]
+ $visited = array_fill(0, $n, false);
+ $visited[0] = true;
+
+ while (!empty($queue)) {
+ list($node, $dist) = array_shift($queue);
+
+ if ($node == $n - 1) {
+ return $dist; // Found the shortest path to city n-1
+ }
+
+ foreach ($graph[$node] as $neighbor) {
+ if (!$visited[$neighbor]) {
+ $visited[$neighbor] = true;
+ $queue[] = [$neighbor, $dist + 1];
+ }
+ }
+ }
+
+ return -1; // If there's no path (shouldn't happen in this problem)
+ }
+
+}
\ No newline at end of file
diff --git a/algorithms/003254-find-the-power-of-k-size-subarrays-i/README.md b/algorithms/003254-find-the-power-of-k-size-subarrays-i/README.md
new file mode 100644
index 000000000..2ab1b61b9
--- /dev/null
+++ b/algorithms/003254-find-the-power-of-k-size-subarrays-i/README.md
@@ -0,0 +1,134 @@
+3254\. Find the Power of K-Size Subarrays I
+
+**Difficulty:** Medium
+
+**Topics:** `Array`, `Sliding Window`
+
+You are given an array of integers `nums` of length `n` and a _positive_ integer `k`.
+
+The **power** of an array is defined as:
+
+- Its **maximum** element if all of its elements are **consecutive** and sorted in **ascending** order.
+- `-1` otherwise.
+
+You need to find the **power** of all subarrays[^1] of nums of size k.
+
+Return an integer array `results` of size `n - k + 1`, where `results[i]` is the _power_ of `nums[i..(i + k - 1)]`.
+
+**Example 1:**
+
+- **Input:** nums = [1,2,3,4,3,2,5], k = 3
+- **Output:** [3,4,-1,-1,-1]
+- **Explanation:** There are 5 subarrays of `nums` of size 3:
+ - `[1, 2, 3]` with the maximum element 3.
+ - `[2, 3, 4]` with the maximum element 4.
+ - `[3, 4, 3]` whose elements are **not** consecutive.
+ - `[4, 3, 2]` whose elements are **not** sorted.
+ - `[3, 2, 5]` whose elements are **not** consecutive.
+
+
+**Example 2:**
+
+- **Input:** nums = [2,2,2,2,2], k = 4
+- **Output:** [-1,-1]
+
+
+**Example 3:**
+
+- **Input:** nums = [3,2,3,2,3,2], k = 2
+- **Output:** [-1,3,-1,3,-1]
+
+
+
+**Constraints:**
+
+- `1 <= n == nums.length <= 500`
+- 1 <= nums[i] <= 105
+- `1 <= k <= n`
+
+
+**Hint:**
+1. Can we use a brute force solution with nested loops and HashSet?
+
+[^1]: **Subarray**:
+A subarray is a contiguous non-empty sequence of elements within an array.
+
+
+
+**Solution:**
+
+We can break down the task as follows:
+
+### Problem Breakdown:
+1. We are given an array `nums` of length `n`, and a positive integer `k`. We need to consider all subarrays of size `k` and compute their **power**.
+2. The **power** of a subarray is:
+ - The **maximum** element of the subarray if all the elements are **consecutive** and sorted in **ascending** order.
+ - `-1` otherwise.
+3. We need to return an array of size `n - k + 1`, where each element corresponds to the power of the respective subarray.
+
+### Plan:
+1. **Sliding Window Approach**: We will slide over the array and check each subarray of length `k`.
+2. **Check if the Subarray is Sorted**: We need to check if the subarray has elements that are consecutive and sorted in ascending order.
+3. **Return Maximum or -1**: If the subarray is valid, we return the maximum element. Otherwise, return `-1`.
+
+### Steps:
+1. **Check if the subarray is sorted**:
+ - A sorted subarray with consecutive elements should have the property: `nums[i+1] - nums[i] == 1` for every `i` in the subarray.
+2. **Sliding Window**:
+ - For each subarray of length `k`, check if it is sorted and return the maximum element if valid, otherwise return `-1`.
+
+Let's implement this solution in PHP: **[3254. Find the Power of K-Size Subarrays I](https://github.com/mah-shamim/leet-code-in-php/tree/main/algorithms/003254-find-the-power-of-k-size-subarrays-i/solution.php)**
+
+```php
+
+```
+
+### Explanation:
+
+- **Sliding Window**: We use a `for` loop from `i = 0` to `i = n - k` to consider all subarrays of size `k`. For each subarray, we use `array_slice()` to extract the subarray.
+- **Sorting Check**: For each subarray, we check if it is sorted with consecutive elements by iterating through the subarray and checking if each pair of consecutive elements has a difference of `1`.
+- **Result**: If the subarray is valid, we append the maximum value of the subarray to the result. Otherwise, we append `-1`.
+
+### Time Complexity:
+- We are iterating over `n - k + 1` subarrays.
+- For each subarray, we check if the elements are consecutive, which takes `O(k)` time.
+- Thus, the overall time complexity is `O((n - k + 1) * k)` which simplifies to `O(n * k)`.
+
+### Edge Case Considerations:
+- If `k = 1`, every subarray is trivially sorted (it only contains one element), and the power of each subarray will be the element itself.
+- If the subarray is not consecutive, it will immediately return `-1`.
+
+### Example Outputs:
+1. For `nums = [1, 2, 3, 4, 3, 2, 5], k = 3`, the output is `[3, 4, -1, -1, -1]`.
+2. For `nums = [2, 2, 2, 2, 2], k = 4`, the output is `[-1, -1]`.
+3. For `nums = [3, 2, 3, 2, 3, 2], k = 2`, the output is `[-1, 3, -1, 3, -1]`.
+
+This solution should efficiently work for the problem constraints.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
diff --git a/algorithms/003254-find-the-power-of-k-size-subarrays-i/solution.php b/algorithms/003254-find-the-power-of-k-size-subarrays-i/solution.php
new file mode 100644
index 000000000..6d4ae4231
--- /dev/null
+++ b/algorithms/003254-find-the-power-of-k-size-subarrays-i/solution.php
@@ -0,0 +1,37 @@
+
+```
+
+### Explanation:
+
+
+1. **Initialization**: Loop `k` times since you need to perform `k` operations.
+2. **Find Minimum Value**:
+ - Iterate over the array `nums` to find the smallest value and its **first occurrence index**.
+3. **Multiply Minimum Value**:
+ - Replace the value at the identified index with the product of the current value and `multiplier`.
+4. **Repeat**:
+ - Repeat the above steps for `k` iterations.
+5. **Return the Final Array**:
+ - Return the modified array after all operations.
+
+### Test Output
+
+For the provided test cases:
+
+#### Test Case 1:
+Input:
+```php
+$nums = [2, 1, 3, 5, 6];
+$k = 5;
+$multiplier = 2;
+```
+Output:
+```text
+Output: [8, 4, 6, 5, 6]
+```
+
+#### Test Case 2:
+Input:
+```php
+$nums = [1, 2];
+$k = 3;
+$multiplier = 4;
+```
+Output:
+```text
+Output: [16, 8]
+```
+
+### Complexity
+
+1. **Time Complexity**:
+ - For each of the `k` operations, finding the minimum value in the array requires `O(n)`.
+ - Total: _**O(k x n)**_, where _**n**_ is the size of the array.
+
+2. **Space Complexity**:
+ - The solution uses _**O(1)**_ extra space.
+
+This solution adheres to the constraints and provides the expected results for all test cases.
+
+**Contact Links**
+
+If you found this series helpful, please consider giving the **[repository](https://github.com/mah-shamim/leet-code-in-php)** a star on GitHub or sharing the post on your favorite social networks π. Your support would mean a lot to me!
+
+If you want more helpful content like this, feel free to follow me:
+
+- **[LinkedIn](https://www.linkedin.com/in/arifulhaque/)**
+- **[GitHub](https://github.com/mah-shamim)**
diff --git a/algorithms/003264-final-array-state-after-k-multiplication-operations-i/solution.php b/algorithms/003264-final-array-state-after-k-multiplication-operations-i/solution.php
new file mode 100644
index 000000000..2d89ba765
--- /dev/null
+++ b/algorithms/003264-final-array-state-after-k-multiplication-operations-i/solution.php
@@ -0,0 +1,28 @@
+