You signed in with another tab or window. Reload to refresh your session.You signed out in another tab or window. Reload to refresh your session.You switched accounts on another tab or window. Reload to refresh your session.Dismiss alert
{{ message }}
This repository has been archived by the owner on Feb 17, 2022. It is now read-only.
I'm working on porting a C++ program that uses SDL2 to Emscripten. I have gotten most things to work, but I'm having trouble making HiDPI support work. This is what the program looks like on desktop with HiDPI:
This is the exact same code running in an Emscripten environment using this SDL2 port, on the same HiDPI screen:
Notice how the entire screen is rendered only in the top left corner of the screen, and that everything is 2x smaller. If I change the code to render the screen at 2x resolution, it looks like this:
The text is at the correct size, but only a quarter of the screen is showing up. If I make the created window 2x larger, it makes the canvas much larger than the border:
For reference, here's what it looks like without HiDPI enabled:
I looked at the source a bit and found that:
The size of the image data is 4x smaller than it would be with the full size (in this case, it's 620x350 pixels long instead of 1240x700 pixels long). This could be fixed by applying data->pixel_ratio to the width and height, but I don't believe this is a portable solution since the normal SDL behavior doesn't require the application to do 2x rendering itself. (?)
The actual image data is not scaled up to 2x size anywhere in Emscripten_UpdateWindowFramebuffer, which under normal circumstances I would expect, but due to the way SDL2 seems to handle HiDPI (at least from my experience) the image should be integer scaled up to the correct resolution.
Note that I don't really understand how HiDPI works with normal SDL (I just messed with code until I got a result), so I may be wrong on how to handle this; but I know for sure that more than just a quarter of the canvas should be accessible with HiDPI.
The text was updated successfully, but these errors were encountered:
Hmm, it looks like most of the other backends with HiDPI support don't have framebuffer support and fall back to using a renderer internally, which handles the scale automatically. It looks like we would need to scale in Emscripten_UpdateFramebuffer to handle this correctly.
As a workaround, you can use SDL_SetHint(SDL_HINT_FRAMEBUFFER_ACCELERATION, "1"); before you call SDL_Init to force going through the renderer.
EDIT: Hmm, since the framebuffer seems to always be non-HiDPI you could just disable HiDPI and use CSS image-rendering on the canvas to control the scaling...
MCJack123
added a commit
to MCJack123/craftos2
that referenced
this issue
Mar 26, 2020
Due to a bug in SDL2 (emscripten-ports/SDL2#109), HiDPI mode forces the
canvas to be larger on HiDPI monitors. To work around this, use the
following CSS rules on the canvas:
```
@media (min-resolution: 144dpi) and (max-resolution: 240dpi) {
canvas.emscripten {
clip-path: polygon(0% 0%, 0% 50%, 50% 50%, 50% 0%);
margin-left: 620px;
margin-top: 350px;
}
}
@media (min-resolution: 240dpi) and (max-resolution: 336dpi) {
canvas.emscripten {
clip-path: polygon(0% 0%, 0% 33.33333333%, 33.33333333% 33.33333333%, 33.33333333% 0%);
margin-left: 1240px;
margin-top: 700px;
}
}
```
HiDPI can be disabled by adding `-DNO_EMSCRIPTEN_HIDPI` to CPPFLAGS.
I'm working on porting a C++ program that uses SDL2 to Emscripten. I have gotten most things to work, but I'm having trouble making HiDPI support work. This is what the program looks like on desktop with HiDPI:
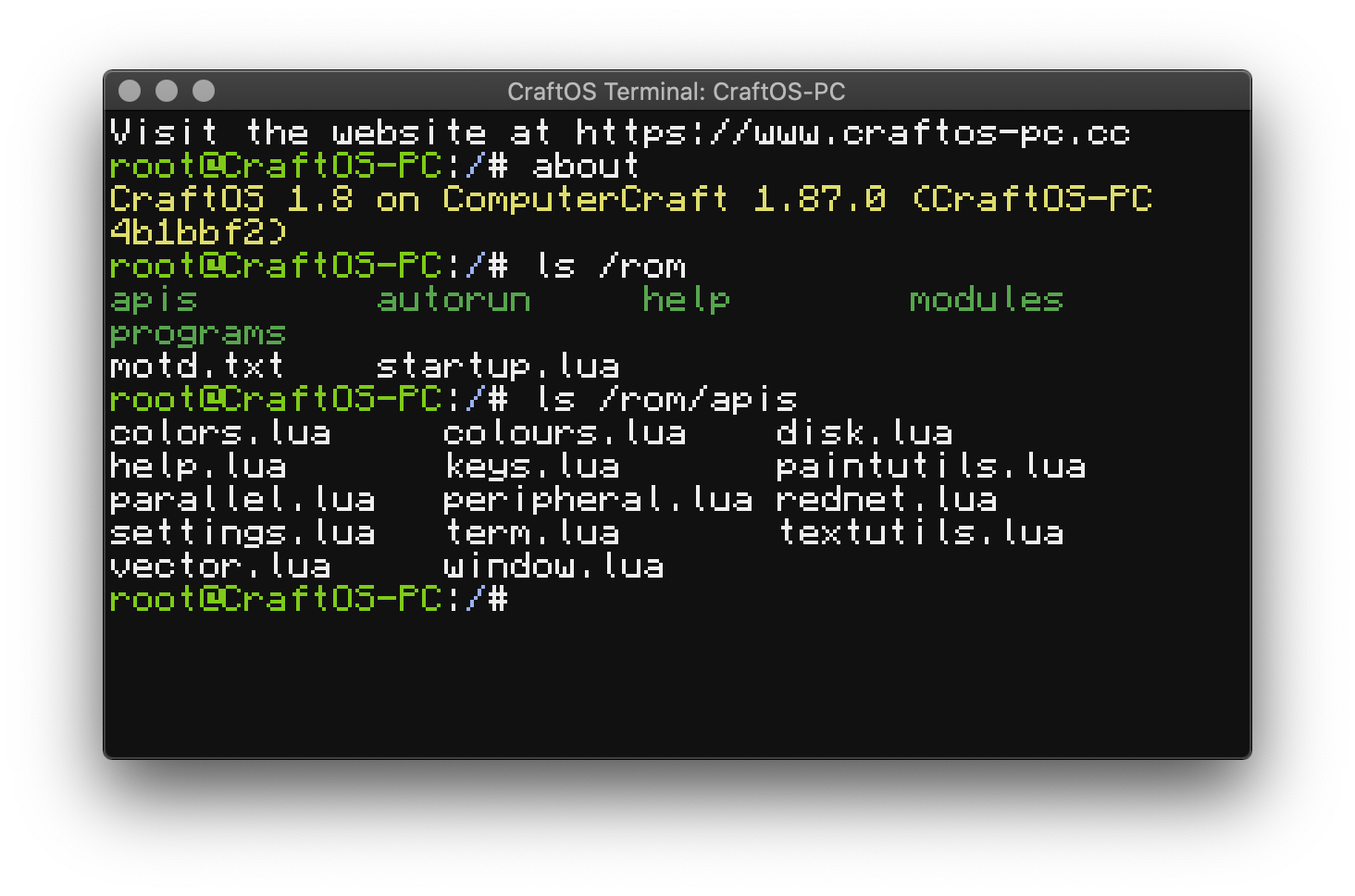
This is the exact same code running in an Emscripten environment using this SDL2 port, on the same HiDPI screen:
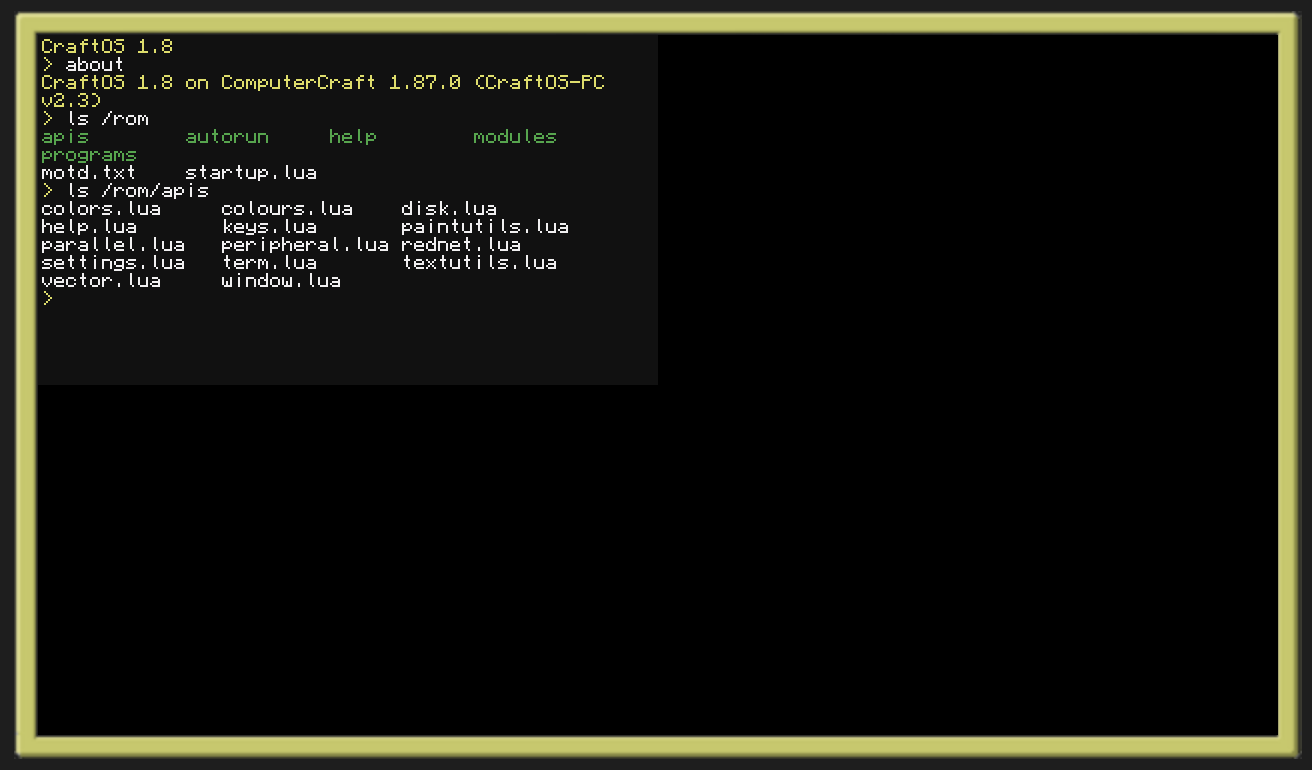
Notice how the entire screen is rendered only in the top left corner of the screen, and that everything is 2x smaller. If I change the code to render the screen at 2x resolution, it looks like this:
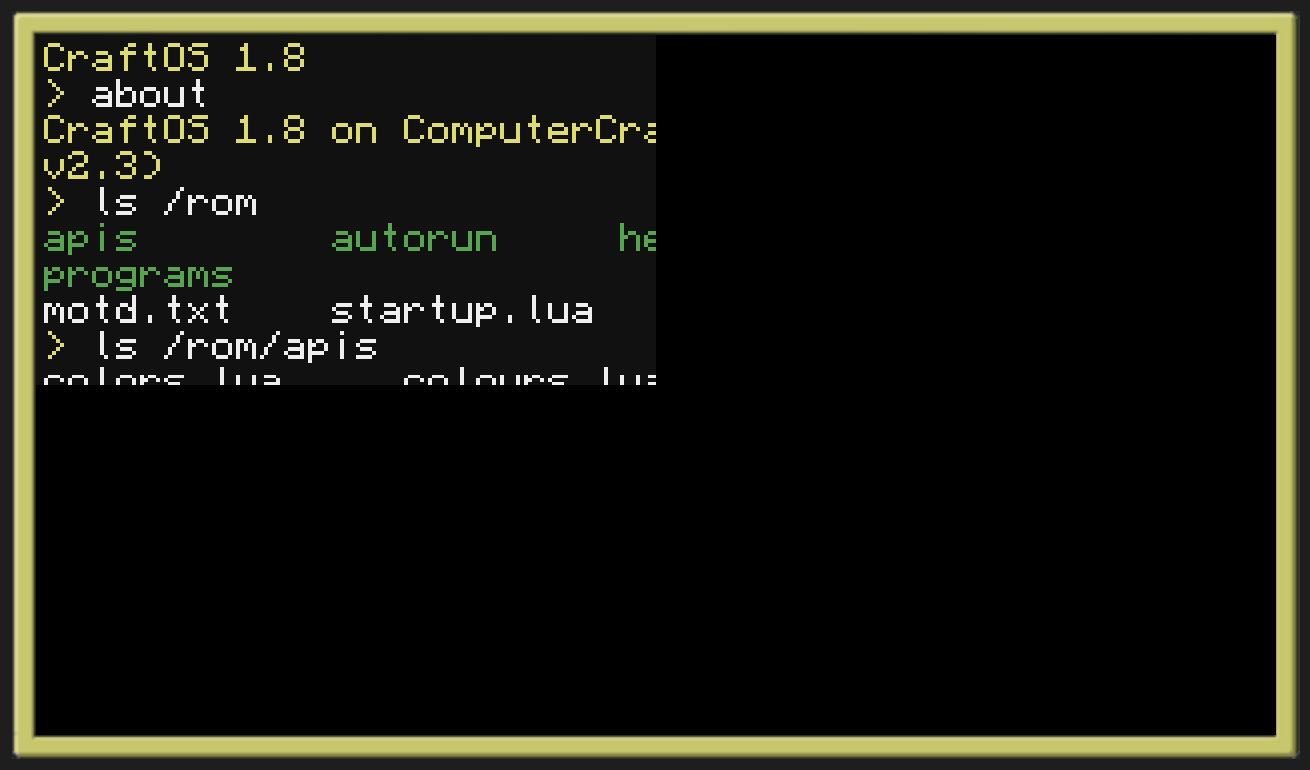
The text is at the correct size, but only a quarter of the screen is showing up. If I make the created window 2x larger, it makes the canvas much larger than the border:
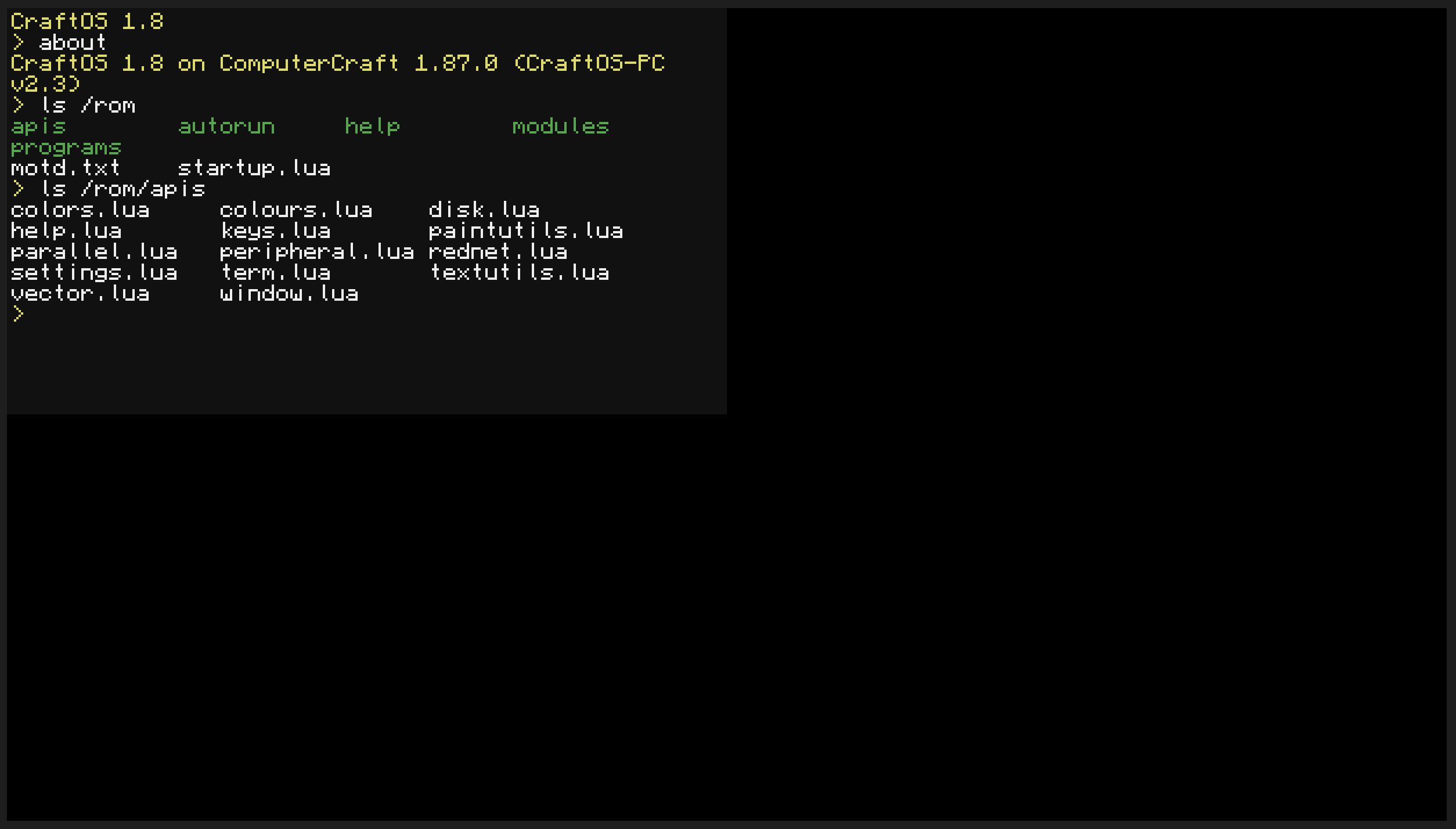
For reference, here's what it looks like without HiDPI enabled:
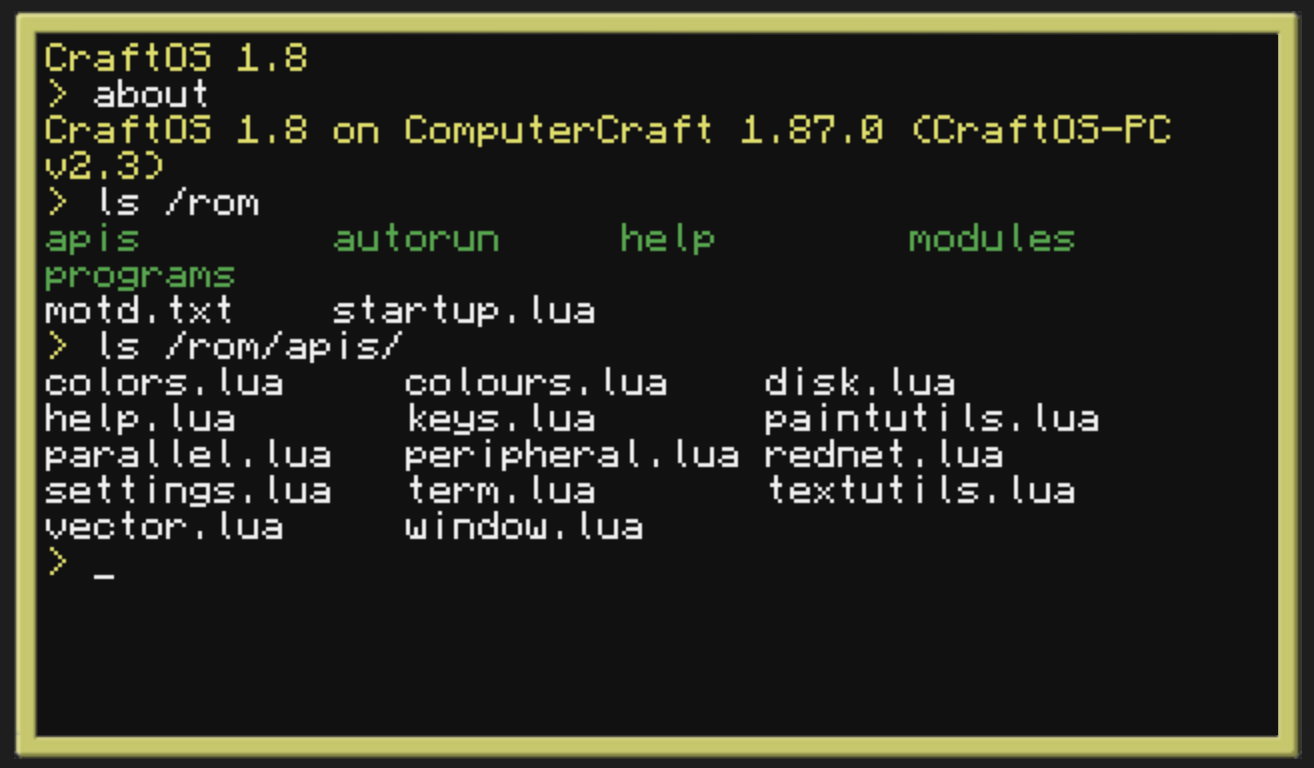
I looked at the source a bit and found that:
data->pixel_ratio
to the width and height, but I don't believe this is a portable solution since the normal SDL behavior doesn't require the application to do 2x rendering itself. (?)Emscripten_UpdateWindowFramebuffer
, which under normal circumstances I would expect, but due to the way SDL2 seems to handle HiDPI (at least from my experience) the image should be integer scaled up to the correct resolution.Note that I don't really understand how HiDPI works with normal SDL (I just messed with code until I got a result), so I may be wrong on how to handle this; but I know for sure that more than just a quarter of the canvas should be accessible with HiDPI.
The text was updated successfully, but these errors were encountered: